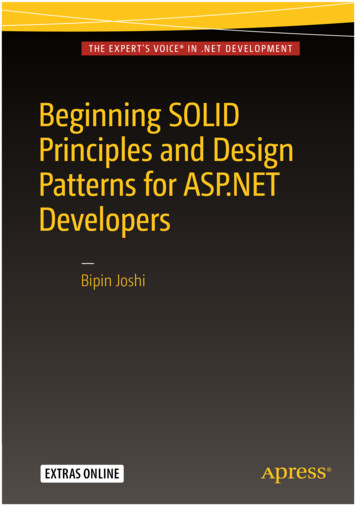
Transcription
T HE E X P ER T ’S VOIC E I N .N E T D E V E L O P M E N TBeginning SOLIDPrinciples and DesignPatterns for ASP.NETDevelopers—Bipin Joshi
Beginning SOLIDPrinciples and DesignPatterns for ASP.NETDevelopersBipin Joshi
Beginning SOLID Principles and Design Patterns for ASP.NET DevelopersBipin Joshi301 PitruchhayaThane, IndiaISBN-13 (pbk): 978-1-4842-1847-1DOI 10.1007/978-1-4842-1848-8ISBN-13 (electronic): 978-1-4842-1848-8Library of Congress Control Number: 2016937316Copyright 2016 by Bipin JoshiThis work is subject to copyright. All rights are reserved by the Publisher, whether the whole or part of the material isconcerned, specifically the rights of translation, reprinting, reuse of illustrations, recitation, broadcasting, reproductionon microfilms or in any other physical way, and transmission or information storage and retrieval, electronicadaptation, computer software, or by similar or dissimilar methodology now known or hereafter developed. Exemptedfrom this legal reservation are brief excerpts in connection with reviews or scholarly analysis or material suppliedspecifically for the purpose of being entered and executed on a computer system, for exclusive use by the purchaserof the work. Duplication of this publication or parts thereof is permitted only under the provisions of the CopyrightLaw of the Publisher's location, in its current version, and permission for use must always be obtained from Springer.Permissions for use may be obtained through RightsLink at the Copyright Clearance Center. Violations are liable toprosecution under the respective Copyright Law.Trademarked names, logos, and images may appear in this book. Rather than use a trademark symbol with everyoccurrence of a trademarked name, logo, or image we use the names, logos, and images only in an editorial fashionand to the benefit of the trademark owner, with no intention of infringement of the trademark.The use in this publication of trade names, trademarks, service marks, and similar terms, even if they are not identifiedas such, is not to be taken as an expression of opinion as to whether or not they are subject to proprietary rights.While the advice and information in this book are believed to be true and accurate at the date of publication, neitherthe authors nor the editors nor the publisher can accept any legal responsibility for any errors or omissions that maybe made. The publisher makes no warranty, express or implied, with respect to the material contained herein.Managing Director: Welmoed SpahrLead Editor: James DeWolfDevelopment Editor: Douglas PundickTechnical Reviewer: Alex ThissenEditorial Board: Steve Anglin, Pramila Balen, Louise Corrigan, James DeWolf, Jonathan Gennick,Robert Hutchinson, Celestin Suresh John, Michelle Lowman, James Markham, Susan McDermott,Matthew Moodie, Jeffrey Pepper, Douglas Pundick, Ben Renow-Clarke, Gwenan SpearingCoordinating Editor: Melissa MaldonadoCopy Editor: April RondeauCompositor: SPi GlobalIndexer: SPi GlobalArtist: SPi GlobalDistributed to the book trade worldwide by Springer Science Business Media New York, 233 Spring Street,6th Floor, New York, NY 10013. Phone 1-800-SPRINGER, fax (201) 348-4505, e-mail orders-ny@springer-sbm.com,or visit www.springer.com. Apress Media, LLC is a California LLC and the sole member (owner) is SpringerScience Business Media Finance Inc (SSBM Finance Inc). SSBM Finance Inc is a Delaware corporation.For information on translations, please e-mail rights@apress.com, or visit www.apress.com.Apress and friends of ED books may be purchased in bulk for academic, corporate, or promotional use.eBook versions and licenses are also available for most titles. For more information, reference our Special BulkSales–eBook Licensing web page at www.apress.com/bulk-sales.Any source code or other supplementary material referenced by the author in this text is available to readers atwww.apress.com. For detailed information about how to locate your book’s source code, go towww.apress.com/source-code/.Printed on acid-free paper
At the holy feet of Lord Shiva.—Bipin Joshi
Contents at a GlanceAbout the Author .xvAbout the Technical Reviewer .xviiIntroduction .xix Chapter 1: Overview of SOLID Principles and Design Patterns . 1 Chapter 2: SOLID Principles. 45 Chapter 3: Creational Patterns: Singleton, Factory Method, and Prototype . 87 Chapter 4: Creational Patterns: Abstract Factory and Builder . 111 Chapter 5: Structural Patterns: Adapter, Bridge, Composite, and Decorator .135 Chapter 6: Structural Patterns: Façade, Flyweight, and Proxy . 167 Chapter 7: Behavioral Patterns: Chain of Responsibility, Command,Interpreter, and Iterator . 201 Chapter 8: Behavioral Patterns: Mediator, Memento, and Observer . 239 Chapter 9: Behavioral Patterns: State, Strategy, Template Method,and Visitor . 275 Chapter 10: Patterns of Enterprise Application Architecture: Repository,Unit of Work, Lazy Load, and Service Layer. 309 Chapter 11: JavaScript Code-Organization Techniques and Patterns . 355 Bibliography . 391Index . 393v
ContentsAbout the Author .xvAbout the Technical Reviewer .xviiIntroduction .xix Chapter 1: Overview of SOLID Principles and Design Patterns . 1Overview of Object-Oriented Programming . 1Classes and Objects . 2Abstraction . 2Encapsulation . 3Inheritance. 6Abstract Classes and Interfaces . 7Polymorphism . 9Overview of SOLID Principles . 14Single Responsibility Principle (SRP) . 15Open/Closed Principle (OCP). 15Liskov Substitution Principle (LSP) . 15Interface Segregation Principle (ISP) . 16Dependency Inversion Principle (DIP) . 16Design Patterns . 16Gang of Four Design Patterns . 17Categorization of GoF Patterns . 17Martin Fowler’s Patterns of Enterprise Application Architecture. 19Categorization of P of EAA . 20Design Patterns in JavaScript . 20vii
CONTENTSApplying Design Principles and Patterns . 21You Are Already Using Patterns! A Few Examples . 22Creating an ASP.NET 5 Application Using MVC 6 and Entity Framework 7 . 23Creating a Web Application Using Visual Studio . 24Configuring Project Dependencies . 28Configuring Application Settings . 29Configuring Application Startup . 30Creating DbContext and Model . 33Creating the HomeController . 34Creating the Index and AddContact Views . 36Creating the ContactDb Database. 40Going Forward: From ASP.NET 5 to ASP.NET Core 1.0. 43Summary . 44 Chapter 2: SOLID Principles. 45Single Responsibility Principle (SRP) . 45Open/Closed Principle (OCP) . 54Liskov Substitution Principle (LSP) . 61Interface Segregation Principle (ISP) . 74Dependency Inversion Principle (DIP). 80Summary . 85 Chapter 3: Creational Patterns: Singleton, Factory Method, and Prototype . 87Overview of Creational Design Patterns . 87Singleton . 88Design and Explanation . 89Example . 89Factory Method . 94Design and Explanation . 94Example . 95viii
CONTENTSPrototype . 103Design and Explanation . 103Example . 104Summary . 109 Chapter 4: Creational Patterns: Abstract Factory and Builder . 111Abstract Factory . 111Design and Explanation . 111Example . 112Storing Factory Settings . 122Storing Factory Name in the Configuration File. 123Storing Factory Type Name in the Configuration File . 124Builder . 125Design and Explanation . 125Example . 126Summary . 134 Chapter 5: Structural Patterns: Adapter, Bridge, Composite, and Decorator .135An Overview of Structural Patterns . 135Adapter . 136Design and Explanation . 136Example . 137Object Adapter vs. Class Adapter. 143Bridge . 144Design and Explanation . 145Example . 146Composite . 152Design and Explanation . 153Example . 153ix
CONTENTSDecorator. 159Design and Explanation . 160Example . 161Summary . 166 Chapter 6: Structural Patterns: Façade, Flyweight, and Proxy . 167Façade . 167Design and Explanation . 168Example . 168Flyweight . 177Design and Explanation . 178Example . 179Proxy . 185Design and Explanation . 186Example . 186Summary . 200 Chapter 7: Behavioral Patterns: Chain of Responsibility, Command,Interpreter, and Iterator . 201Behavioral Patterns . 201Chain of Responsibility . 202Design and Explanation . 202Example . 203Command . 212Design and Explanation . 212Example . 213Interpreter . 221Design and Explanation . 221Example . 222x
CONTENTSIterator. 229Design and Explanation . 230Example . 230Summary . 237 Chapter 8: Behavioral Patterns: Mediator, Memento, and Observer . 239Mediator . 239Design and Explanation . 240Example . 241Memento . 253Design and Explanation . 254Example . 254Observer . 263Design and Explanation . 264Example . 264Summary . 273 Chapter 9: Behavioral Patterns: State, Strategy, Template Method,and Visitor . 275State . 275Design and Explanation . 276Example . 276Strategy . 284Design and Explanation . 285Example . 286Template Method. 292Design and Explanation . 292Example . 293Visitor . 300Design and Explanation . 301Example . 302Summary . 308xi
CONTENTS Chapter 10: Patterns of Enterprise Application Architecture: Repository,Unit of Work, Lazy Load, and Service Layer. 309Overview of P of EAA . 309Repository . 312Design and Explanation . 313Example . 313Unit of Work . 322Design and Explanation . 322Example . 323Lazy Load . 330Design and Explanation . 331Example . 332Service Layer. 340Design and Explanation . 340Example . 341Injecting Repositories Through Dependency Injection . 351Summary . 353 Chapter 11: JavaScript Code-Organization Techniques and Patterns . 355Organizing JavaScript Code Using Objects . 355Object Literals. 356Function Objects . 357Immediately Invoked Function Expressions (IIFE) . 360Namespace Pattern . 362Module Pattern . 367Revealing Module Pattern . 374Sandbox Pattern . 375xii
CONTENTSUsing Design Patterns in JavaScript . 379Singleton Pattern . 379Façade Pattern . 381Observer Pattern . 383MVC, MVVM, and MVW Patterns . 386Summary . 389 Bibliography . 391Index . 393xiii
About the AuthorBipin Joshi is a software consultant, a trainer, an author, and a yogi who writes about seemingly unrelatedtopics: software development and yoga! He conducts professional training programs to help developerslearn ASP.NET and web technologies better and faster. Currently, his focus is ASP.NET, C#, EntityFramework, JavaScript, jQuery, AngularJS, TypeScript, and design and architectural patterns. More detailsabout his training programs are available at http://www.binaryintellect.com.Bipin has been programming since 1995 and has worked with the .NET framework since itsinception. He has authored or co-authored more than ten books and numerous articles on .NETtechnologies. He regularly writes about ASP.NET and other cutting-edge web technologies on his website:http://www.binaryintellect.net. Bipin was a Microsoft Most Valuable Professional (MVP) and aMicrosoft Certified Trainer (MCT) during 2002–2008.Having embraced the yoga way of life, he enjoys the intoxicating presence of God and writes about yogaon his website: http://www.ajapayoga.in. Bipin has also penned a few books on yoga. He can be reachedthrough his websites.xv
About the Technical ReviewerAlex Thissen has been involved in application development since the late1990s and has worked as a lead developer and architect at both smallcompanies and large enterprises. He has spent a majority of h
Apress and friends of ED books may be purchased in bulk for academic, corporate, or promotional use. eBook versions and licenses are also available for most titles. For more information, reference our Special Bulk