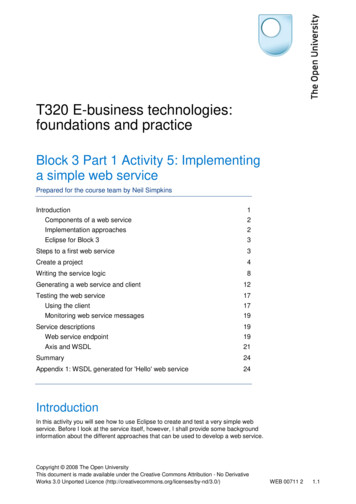
Transcription
T320 E-business technologies:foundations and practiceBlock 3 Part 1 Activity 5: Implementinga simple web servicePrepared for the course team by Neil SimpkinsIntroduction1Components of a web service2Implementation approaches2Eclipse for Block 33Steps to a first web service3Create a project4Writing the service logic8Generating a web service and client12Testing the web service17Using the client17Monitoring web service messages19Service descriptions19Web service endpoint19Axis and WSDL21Summary24Appendix 1: WSDL generated for 'Hello' web service24IntroductionIn this activity you will see how to use Eclipse to create and test a very simple webservice. Before I look at the service itself, however, I shall provide some backgroundinformation about the different approaches that can be used to develop a web service.Copyright 2008 The Open UniversityThis document is made available under the Creative Commons Attribution - No DerivativeWorks 3.0 Unported Licence B 00711 21.1
T320 E-business technologies: foundations and practiceComponents of a web serviceFrom an implementation point of view, a web service typically has two distinctcomponents: Some 'logic', which actually carries out the real work required to provide theservice functions, whatever they might be. For example, a simple service tocalculate VAT might include at its heart a piece of code that receives a monetaryvalue in sterling, such as 10, multiplies that value by 17.5% to calculate the VAT( 1.75) and returns the new total value including VAT ( 11.75). An interface, which is implemented in XML. This describes how to put a request tothe service and what the expected response or result may consist of.In addition, in order to actually make use of any web service we implement, we needalso to implement a client that constructs our request (based on the XML interface)and displays the result returned by the web service.This view of a web service should help to explain the different approaches toproducing a web service.Implementation approachesThere are two basic approaches that are used to create web services from scratch.The first approach is termed 'bottom-up'. This is where the code that implements theoperations performed by the service is written first. Then the XML description (WSDL)of the service is produced and published in UDDI. The service code is placed inside a'container' that provides the required interface for messaging (SOAP or somethingsimilar).The second approach is to produce the XML description of the service before it isimplemented. So the service is fully described in terms of what it does, how it can becalled and what result(s) it returns, but is not actually implemented. This (WSDL)specification is then used as a guide to writing the code that implements the service.This approach is termed 'top-down'.These two approaches are illustrated in Figure 1.UDDIweb cation serverbottom-updevelopmentweb servicemessage interfaceweb serviceimplementationFigure 1Bottom-up and top-down web service developmentBlock 3 Part 1 Activity 5 2
T320 E-business technologies: foundations and practiceEclipse for Block 3You should have installed the software required for the practical activities in Block 3when you were working on Block 2. If you have any problems with the installationprocess then please go to the course forums for guidance or contact your tutor.Block 3 uses Eclipse, Java, Tomcat and the following plug-ins (extensions) to Eclipse.Further information about these plug-ins is available through the 'Library resources'section of the course web site if you are interested.Axis2 plug-insTwo Axis2 plug-ins for Eclipse are used in this block. In fact you will use only one ofthese explicitly: the 'Service Archive Generator Wizard'. For now you don't need toworry about this, as it will be introduced more fully later.The other Axis2 plug-in is grandly called the 'Code Generator Wizard'. This plug-incan be used to support either top-down or bottom-up development. It will be used inthis activity, but the use will be hidden from you. If you wish, however, you can go onto use this plug-in directly yourself, either bottom-up or top-down.WTP plug-insThe Web Tools Platform (WTP) is an Eclipse project that extends Eclipse with a rangeof tools for developing, running and testing web services. In Block 2 you used the XMLeditor provided by the WTP, while in this block you will use much of the remainingfunctionality.soapUI plug-inThis plug-in has a range of functions, several of which are similar to the WTP'sfunctionality. It is up to you which you prefer to use. However, later in this block youwill use the soapUI plug-in to test web services for interoperability, which the WTPdoesn't support directly.Steps to a first web serviceThe first web service I shall demonstrate how to write is very straightforward. It willaccept a simple piece of text (intended to be a person's name) and return anotherpiece of text as a result ('Hello there' followed by the name sent to the service). This isshown in Figure 2.Eclipse"Fred"Figure 2web serviceimplementation"Hello there Fred"'Hello' web service outlineEclipse will help by automating several of the tasks that are involved in the process ofdeveloping, testing and deploying the web service.There are different sequences of steps that can be used in Eclipse to achieve thesame end result; here I will try to use the simplest sequence possible. You shouldalready have watched the Block 3 video tutorial, 'Creating a simple web service usingEclipse'. This will have given you an overall idea of the set of steps that you will needto take, as well as some idea of the 'hello there' Java code you will use as theservice's logic.Block 3 Part 1 Activity 5 3
T320 E-business technologies: foundations and practiceIn short, the steps you will take to produce and test your first web service are asfollows:1Create a project in the Eclipse workspace of type 'Dynamic Web Project', whichwill host your web service.2Write the Java code (the 'business logic') that implements your web servicefunctionality.3Use Eclipse to automatically generate the components (WSDL etc.) that willtransform the Java code into a web service, and then ask Eclipse to run that webservice for you.4Create another project of type 'Dynamic Web Project', which will host the clientapplication that you will use to access and test the web service.5Use Eclipse to automatically generate a set of web pages that function as a clientinterface to call the web service.6Use the web page client to send a request to the web service and observe the webservice's response.Application server setupBefore you can create and run an application such as a web service, you will need toset up an application server inside Eclipse. To set up the Tomcat server that the T320software installation has provided, you should follow the guide Configuring anApplication Server in Eclipse.Once you have set up the application server, you can start working through theinstructions given in the following sections.Create a projectIf Eclipse is not already open, open it by double-clicking on the 'Eclipse for Block 3'shortcut on your desktop. Ensure that you have the Project Explorer open in aJava EE perspective (Figure 3). If you are not currently in this perspective then useWindow Open Perspective and then select 'Java EE', which may be on the menu listor accessible under Other , depending on your current perspective.I shall not be going into the details of Java code and the many associatedtechnologies. You might like to know that 'Java EE' is a reference to the Javaprogramming language 'Enterprise Edition'. 'Enterprise' solutions are commonlythought of as reliable and scalable technologies.The first step is to create a project that will be used to hold all the code and otherelements that will make up your web service.Select File New Project , open up the 'Web' folder and select 'Dynamic WebProject' (Figure 4). Then click 'Next'. This will start the New Dynamic Web Projectwizard, which will guide you through the project setup stages.Block 3 Part 1 Activity 5 4
T320 E-business technologies: foundations and practiceFigure 3Eclipse Project Explorer in Java EE perspectiveFigure 4New Project dialogue boxBlock 3 Part 1 Activity 5 5
T320 E-business technologies: foundations and practiceType a name for your project in the next dialogue box. Here we will call the project'Hello' (Figure 5). At this point you may either click 'Finish' and have the projectcreated immediately, or click 'Next' to continue and see what other options areavailable in configuring a project. It's a good idea to become aware of the optionsavailable when using an Eclipse wizard, so click 'Next' to continue.Figure 5Dynamic Web Project setup dialogue boxContinue through the wizard's steps as shown in Figure 6 and Figure 7. Note thenames of the 'Content' and 'Java Source' directories (Figure 7) and then click 'Finish'.Block 3 Part 1 Activity 5 6
T320 E-business technologies: foundations and practiceFigure 6Project Facets choice dialogue boxFigure 7Web Module configuration dialogue boxBlock 3 Part 1 Activity 5 7
T320 E-business technologies: foundations and practiceAt this point, if you have not already selected the Java EE perspective then Eclipsewill offer to open this for you (Figure 8). If this happens then click 'Yes'.Figure 8Eclipse changes to the appropriate project perspectiveOnce the wizard has finished you will see that a project called 'Hello' has been createdin the Project Explorer view. You can expand the project to view the components(Figure 9).Figure 9Dynamic project createdWriting the service logicNow you will create the 'business logic' or processing code that performs the taskbehind the web service we want to deliver.The Java code is very simple. Here it is with each line numbered for referencingpurposes:1.package uk.ac.open.t320;2.public class Hello {3.4.public String helloName(String name){return "Hello there " name;}}I shall not be asking you to write Java code yourself, but you will need to understandwhat the code used in this block does, at least in high-level terms. Below is a briefline-by-line description of the code, followed by a diagrammatic view. What isimportant at this stage is that you become familiar with the terminology used.Block 3 Part 1 Activity 5 8
T320 E-business technologies: foundations and practiceLine 1specifies the Java package name to be uk.ac.open.t320 (I shall take abrief look at packages very shortly). This 'names' our code as belonging tothe T320 course within The Open University, within the 'ac' (academic)domain within the UK. It is not necessary to use a package name at all (aswas the case in the video tutorial) but it is recommended by various Javaguidelines and Eclipse.Line 2declares a publicly available object or 'class' in Java called 'Hello', whichimplements the operations that we want our web service to carry out.Line 3declares an operation that can be performed by the 'Hello' class. Operationsare called 'methods' in Java. This operation is called 'helloName' and takesan input called 'name', which is a 'String' such as "Fred" or "Nick" or "Sundayafternoon".Line 4is a single Java statement within the 'helloName' method, which producesthe method's result by returning a string. This string is created by appendingthe string that was passed into the method to the string "Hello there".The operation of the code is depicted in Figure 10, where the 'helloName' method iscalled and given a string value "Fred". It returns a result "Hello there Fred".package: uk.ac.open.t320call 'helloName' methodin 'Hello' class in package'uk.ac.open.t320'"Fred"class: Hellomethod: helloNamename "Fred""Hello there" "Fred"result returned"Hello there Fred"method: otherMethodclass: OtherClassFigure 10 Calling the 'helloName' methodNotice that, as depicted in Figure 10, a package can contain any number of classesand a class can contain any number of methods.Warning: for the purposes of coding in Eclipse, always ensure that method namesstart with a lower-case letter. Otherwise you will run into problems when attempting torun and test the web service.As I mentioned above, I have chosen to place the code in a Java package calleduk.ac.open.t320. This is a matter of style and is not really necessary, but it is goodpractice to use packages in Java. The package name I have used is based on TheOpen University's domain name (the OU web site is at http://www.open.ac.uk),which is reversed to form the first part of all OU package names. I then added thename of the course within the University, 't320'. This gives us a unique package namethat would be very difficult for any other group to legitimately claim as moreappropriately theirs – there will only ever be one T320 course within the OU. (If youwould like further information, the conventions for naming packages are described inhttp://java.sun.com/docs/books/jls/third edition/html/packages.html#7.7.)Block 3 Part 1 Activity 5 9
T320 E-business technologies: foundations and practiceNow we need to introduce the code into the 'Hello' project. To do this we could add apackage and then add a class to the package. However, a quicker approach is to adda class and specify that it belongs to a package; if the package doesn't exist thenEclipse will create the package so that it can put the class into it.In the Eclipse Project Explorer, expand the 'Hello' project and right-click on the 'JavaResources: src' component, then select New. The pop-up menu shown in Figure 11will be displayed.Figure 11 New pop-up menu items for Java Resources sourcesSelect Class and you will be presented with the New Java Class dialogue box(Figure 12).It is important that you enter a package name based on your OUCU. So if yourOUCU is 'nks34', you might enter a package name of:uk.ac.open.t320.nks34This will ensure that
soapUI plug-in This plug-in has a range of functions, several of which are similar to the WTP's functionality. It is up to you which you prefer to use. However, later in this block you will use the soapUI plug-in to test web services for interoperability, which the WTP doesn't support directly. Steps to a first web service The first web service I shall demonstrate how to write is very .