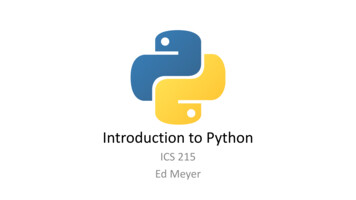
Transcription
Introduction to PythonICS 215Ed Meyer
What are people doing with Python? General ProgrammingMake games - PygameBuild web applications - DjangoData visualization - matplotlibSecurity (Packet Manipulation) - ScapyMachine learning - TensorFlow2
Language Characteristics High-level Interpreted Like Perl, code is compiled right before execution Object-oriented Created by Guido van Rossum Maintained by Python Software Foundation3
Python 2 or Python 3? Python 2.7 or Legacy Python Contains legacy librariesLast version of Python 2 was 2.7No longer receives security updatesEnd of life (EOL) was on January 1st 2020 Python 3 Current and future of Python Many legacy libraries have been converted We will use Python 3 for this class4
Getting Python Website: python.org/downloads Linux and macOS Need to install python 3 Note: May have python 2.7 already installed Windows Need to install icsprojects Server Has both5
Installing Python on Windows Run the installer Check Add Python X.X to PATH This will allow you to run python scripts directly from the commandprompt/PowerShell using the python command X.X is the version of Python you are installing6
Installing Python on WindowsCheck Add Python to PATH7
Installing Python on macOS Install as normal To run Python scripts You may need to use the command python3 to explicitly call thePython 3 interpreter8
Checking for Python Type in either of these in a command prompt/Terminal: python python3 Shows you the version and starts the interpreter Type exit() to exit the interpreter9
Verify Python on macOS If issuing the python command shows 2.7 Then explicitly use python3 command instead10
Verify Python on Windows Ensure to check Add Python X.X to PATH when installing Issue the python command11
General Syntax Differences Semicolons are optional Use spaces or tabs for code blocks Control structurestry-exceptClassesFunctions Does not use { }12
Optional: Show Indent Guide in AtomSettings/Preferences Editor Show Indent Guide13
Comments Single-line: ## This is a single line comment. Multi-line: """""" or ''' '''""" This is the first comment line.This is the second.This is the third. """14
Python Basics Creating a VariableStrings and NumbersPrintingTypecast functionsOperators Getting input Control Structures if-statements Loops Arithmetic Comparison Boolean15
Creating a variable No symbol prefixing variables PHP: Perl: , @ No variable interpolation No keyword before variables JavaScript: let Perl: my Python Syntax:variableName value; Example:message "Hello, world!";16
Variable Names Usual rules apply Contain letters, numbers, and underscoresStart with a letter or underscoreNo spacesNo keywords Coding Standard Lower camel-case17
Strings String values " and ' can be used to create strings ' can be used freely in " and vice versa Concatenate with Has methods (object-oriented)18
Some String Methods .title() Each word begins with a capital letter .upper() All characters are uppercase .lower() All characters are lowercase19
Using Some String Methodsmy name "ed meyer";print(my name.title()); # Ed Meyerprint(my name.upper()); # ED MEYERprint(my name.lower()); # ed meyerprint(my name); # ed meyer20
More String Methods .rstrip() Removes whitespace from the right side .lstrip() Removes whitespace from left side .strip() Removes whitespace from both sides21
Printing In Python 3 print is a function Parenthesis is requiredprint "Hello, it's me."; SyntaxError: Missing parenthesis.print("Hello from the other side."); Hello from the other side. Each print is like System.out.println Adds a \n at the end of each print22
Printing In Python 3 print is a function Parenthesis is requiredprint "Hello, it's me."; SyntaxError: Missing parenthesis.print("Hello from the other side."); Hello from the other side. Each print is like System.out.println Adds a \n at the end of each print23
Printing In Python 2 print is a statement Parenthesis is not required, but can be usedprint "Hello, it's me."; Hello, it's me.print("Hello from the other side."); Hello from the other side. Each print is like System.out.println Adds a \n at the end of each print24
All print is like println What if you don't want to print \n at the end? There are two approaches1. Change the ending character in the print function2. Import and use the write function25
Printing1. Change the ending character in the print function to theempty string By default, it is the newline The end must be the last argument (far right) Python 3 only# Hello World!print('Hello', end '');print(' World!');26
Printing2. Import and use the write functionimport sys;# Hello World!sys.stdout.write('Hello');sys.stdout.write(' World!');27
Print as a Function Functions take arguments Instead of concatenate, separate parts of the output intoarguments Spaces are automatically added between arguments print uses a variable amount of argumentsmy name "Ed";# Hello, Edprint("Hello,", my name);28
Numbers Number values are integers or floats Usual rules apply P ()E**M* Modulo with % DivisionD/A S- Python 2: Integer division between integers Python 3: Floating point for all numbers29
Working with Floats Sometimes you can get an arbitrary number of decimal places For example:0.2 0.1 0.300000000000000043 * 0.1 0.30000000000000004 The number 0.3 is not a nice power of 2. NOT SPECIFIC TO PYTHON30
Avoiding Type Errors From experience, we would expect:age 23;print("You are " age " years old."); To print out: You are 23 years old. But this happens: TypeError: cannot concatenate 'str' and 'int' objects31
Avoiding Type Errors Use the str() function to typecast into a stringprint("You are " str(age) " years old."); There are also: int() If the argument is a float, truncates the decimal portion If the argument is a string, must look like an integer float() The argument must look like a number32
Avoiding Type Errors Typecasting may be avoided by using arguments in printage 23;# You are 23 years old.print("You are", age, "years old.");33
Avoiding Type Errors Typecasting may be avoided by using arguments in printage 23;# You are 23 years old.print("You are", age, "years old.");No explicit space34
The input() Function Syntaxinput("Prompt: "); In Python 3 Accepts all input as a string Need to use int() or float() to convert numbers In Python 2.7 Use raw input() same behavior as Python 3 input()35
The input() Function Syntaxinput("Prompt: "); After the prompt is shown, input will be on the same line Store the input in a variable to use lateruserInput input("Say something: "); Say something: Shamwow!36
Boolean Values Uses the keywords True and False Note the T and F are capitalized 0 also evaluates to False All other numbers evaluate to True37
Comparison OperatorsOperatorDescription Equality! Not Equal Strictly greater than Strictly less than Greater than or equal to Less than or equal to38
Boolean OperatorsOperatorUsageandorx and yx or ynotnot x (in place of the !)Python does not use &&, , !39
if Statementif (condition):# Do something when condition is true.# All statements associated in the if# block must follow the same indentation# Continue with program40
if-else Statementif (condition):# When condition is trueelse:# When condition is false# Continue with program41
if-elif-else Statementif (condition1):# When condition1 is trueelif (condition2):# When condition1 is false and# condition2 is trueelse:# When condition1 and condition2 are false# Continue with program42
Bounds Checking How would you test if a number, x, is between 0 and 10,inclusive? Use the and Boolean operator Test if the number is greater than or equal to 0 Test if the number is less than or equal to 10if ((x 0) and (x 10)):43
Bounds Checking How would you test if a number, x, is between 0 and 10,inclusive? Use the and Boolean operator Test if the number is greater than or equal to 0 Test if the number is less than or equal to 10if ((x 0) and (x 10)):44
Bounds Checking How would you test if a number, x, is between 0 and 10,inclusive? Actually, it's simpler in Python:if (0 x 10):45
Looping Python supports while and for loops for loops work differently than other languages we've seen46
Incrementing Variables Python does NOT support the and -- shortcut ofincrementing/decrementing Instead, Python uses the and - for arbitrary increases ordecreasesi 1;i 1;# 2i 3;# 5print(i); # 547
while Loop Loop as long as the condition is truewhile (condition):# All lines executed as part of the loop# must have consistent indentation.48
for Looping Loops through a range or list of items Called a foreach in other languages No loop counter or condition A list is an array in Python Syntax:for dummy variable in list:# Iterates for each element in list49
for Looping Loops through a range or list of items No loop counter or condition A list is an array in Python Syntax:for dummy variable in list:# Iterates for each element in listNotice the for in : setupAt each iteration, a value in list is put intodummy variable50
The range() Function Creates a range object Represents a range of numbers with a starting number and an endingnumber Used for looping an arbitrary number of times range(end) Generates a range of numbers from 0 to less than end In other words, end - 1 range(start, end) Generates a range of numbers from start to less than end51
The Usual for Looping We can replace list with a range()for i in range(1, 5):print(i); Prints 1 thru 4 on separate lines Possible quiz question:Using a for-loop, print 1 thru 4 on the same line.1 2 3 452
The Usual for Looping We can replace list with a range()for i in range(1, 5):print(i); Prints 1 thru 4 on separate lines Possible quiz question:Using a for-loop, print 1 thru 4 on the same line.1 2 3 453
The Usual for Looping Givenfor i in range(1, 5):print(i); Similar to having Condition: i 5 Modification: i for (i 1; i 5; i )54
The Usual for Looping Givenfor i in range(1, 5):print(i); What if you want to change the i ? range(start, end, step)Step will be how much of an increaseThe default step value is 1Negative steps are possible when start end55
Installing Python on Windows Run the installer Check Add Python X.X to PATH This will allow you to run python scripts directly from the command prompt/PowerShell using the pythoncommand X.X is th