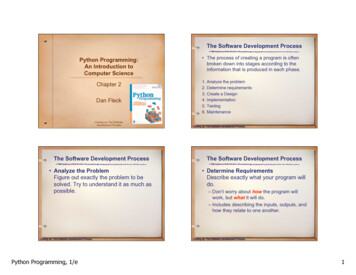
Transcription
The Software Development Process The process of creating a program is oftenbroken down into stages according to theinformation that is produced in each phase.Python Programming:An Introduction toComputer Science1. Analyze the problem2. Determine requirements3. Create a Design4. Implementation5. Testing6. MaintenanceChapter 2Dan FleckComing up: The SoftwareDevelopment Process1The Software Development ProcessThe Software Development Process Analyze the ProblemFigure out exactly the problem to besolved. Try to understand it as much aspossible. Determine RequirementsDescribe exactly what your program willdo.Python Programming, 1/e– Don’t worry about how the program willwork, but what it will do.– Includes describing the inputs, outputs, andhow they relate to one another.1
The Software Development Process Create a Design– Formulate the overall structure of theprogram.– This is where the how of the program getsworked out.– You choose or develop your own algorithmthat meets the requirements.The Software Development Process Test/Debug the Program– Try out your program to see if it worked.– If there are any errors (bugs), they need tobe located and fixed. This process is calleddebugging.– Your goal is to find errors, so try everythingthat might “break” your program! (Correctand incorrect inputs)Python Programming, 1/eThe Software Development Process Implement the Design– Translate the design into a computerlanguage.– In this course we will use Python.Why is it called debugging?The First "Computer Bug”Moth found trapped between pointsat Relay # 70, Panel F, of the MarkII Aiken Relay Calculator while itwas being tested at HarvardUniversity, 9 September 1945. Theoperators affixed the moth to thecomputer log, with the entry: "Firstactual case of bug being found".They put out the word that they had"debugged" the machine, thusintroducing the term "debugging acomputer program".Courtesy of the Naval SurfaceWarfare Center, Dahlgren, VA.,1988.U.S. Naval Historical CenterPhotograph.2
The Software Development Process Maintain the Program– Continue developing the program inresponse to the needs of your users.– In the real world, most programs are nevercompletely finished – they evolve overtime.Example : Temperature ConverterDesign Design– Input: Prompt the user for input (Celsiustemperature)– Process: Process it to convert it toFahrenheit using F 9/5(C) 32– Output: Output the result by displaying it onthe screenPython Programming, 1/eExample : Temperature ConverterAnalysis Analysis – the temperature is given inCelsius, user wants it expressed indegrees Fahrenheit. Requirements– Input – temperature in Celsius– Output – temperature in Fahrenheit– Output 9/5(input) 32Example : Temperature Converter Before we start coding, let’s write a roughdraft of the program in pseudocode Pseudocode is precise English that describeswhat a program does, step by step. However,There is no “official” syntax for pseudocode Using pseudocode, we can concentrate onthe algorithm rather than the programminglanguage.3
Temperature Converter Pseudocode Pseudocode:– Input the temperature in degrees Celsius(call it celsius)– Calculate fahrenheit as (9/5)*celsius 32– Output fahrenheit Now we need to convert this to Python!Temperature Converter Python Code#convert.py# A program to convert Celsius temps to Fahrenheit# by: Susan Computewelldef main():celsiusString raw input("What is the Celsius temperature? ”)celsius int(celsiusString) # Convert from a string to an integer (number)fahrenheit (9.0/5.0) * celsius 32print "The temperature is ",fahrenheit," degrees Fahrenheit."main()Lets try it in IDLE after the next slideUsing IDLE a Python DevelopmentEnvironment Open IDLE In the Python shell you can run dynamicPython commands (this shell is thewindow that opens) File New opens the window to write aprogram Run Run Module runs your program(or press F5)Python Programming, 1/eHow to run outside of IDLE If you have a python source file(something.py) to run it outside of IDLEon the command line: C:\python something.py C:\python c:\SomeDir\myPythonFiles\something.py WARNING: This Python is on your PATH. To add itsee this video:http://showmedo.com/videos/video?name 960000 On Mac this is typically done automatically.4
Question Does that mean I can create a Pythonsource file in anything, not just in IDLE?Like Windows Notepad? Or somethingelse?Need more IDLE Help? Try reading this webpage on usingIDLE: http://hkn.eecs.berkeley.edu/ dyoo/python/idle intro/index.html Answer: Yes! IDLE is an integrateddevelopment environment (IDE), so itmakes it EASIER, but you can use anyplain-text editor. (MS Word isn’t plaintext.)Temperature Converter TestingProgram Revisited Once we write a program, we shouldtest it!#convert.py# A program to convert Celsius temps to Fahrenheit# by: Susan Computewell What are some values with knownanswers? def main():starts a function definitioncelsiusString raw input("What is the Celsius temperature? ”)celsius int(celsiusString) # Convert from a string to an integer(number)fahrenheit (9.0/5.0) * celsius 32print "The temperature is ",fahrenheit," degrees Fahrenheit."What is the CelsiusThe temperature is main()What is the CelsiusThe temperature is main()What is the CelsiusThe temperature is Python Programming, 1/etemperature? 032.0 degrees Fahrenheit.temperature? 100212.0 degrees Fahrenheit.temperature? -40-40.0 degrees Fahrenheit.Commentsmain()5
Elements of Programs : Identifiers Names of variables: celsius, fahrenheit Names of functions: range, main, input Names of modules: convertThese names are called identifiersElements of Programs : IdentifiersIdentifiers are case sensitive. In Python, identifiers:– myVar– MYVAR– myvar Every identifier must begin with a letter orunderscore (“ ”), followed by any sequence ofletters, digits, or underscores. Good programmers use meaningful names Identifiers are case sensitive. Are all DIFFERENT because Python is casesensitiveReserved WordsUsing identifiers in expressionsSome identifiers are part of Python itself. Theseidentifiers are known as reserved words. Thismeans they are not available for you to use as aname for a variable, etc. in your program.Lets try it in IDLE x 5 x5 print x5 print spamTraceback (most recent call last):File " pyshell#15 ", line 1, in -toplevelprint spamNameError: name 'spam' is not defined NameError is the error when you try to use avariable without a value assigned to it.Python Programming, 1/e6
Math Operators– Simpler expressions can becombined using operators.– , -, *, /, **, %– Spaces are irrelevant withinan expression.– The normal mathematicalprecedence applies.– ((x1 – x2) / 2*n) (spam /k**3)Elements of Programs Output Statements– A print statement can print any number ofexpressions.– Successive print statements will display onseparate lines.– A bare print will print a blank line.– If a print statement ends with a “,”, thecursor is not advanced to the next line.Precedence is:PEMDAS - (), **, *, /, , -Elements of Programsprint 3 4print 3, 4, 3 4printprint 3, 4,print 3 4print “The answer is”,3 4Python Programming, 1/e7347347The answer is 7Assignment Statements variable expr variable is an identifier, expr is anexpression The expression on the RHS isevaluated to produce a value which isthen associated with the variablenamed on the LHS. x 3.9 * x * (1-x) fahrenheit 9.0/5.0 * celsius 32 x 57
Assignment Statements Variables can be reassigned as manytimes as you want! myVar 0 myVar0 myVar 7 myVar7 myVar myVar 1 myVar8 Converting Strings to Numbers someFloatString ‘1.343’someVar float(someFloatString)print someVarMore on this later FunctionMeaningfloat( expr )Convert expr to a floating point valueint( expr )Convert expr to an integer valuelong( expr )Convert expr to a long integer valuestr( expr )Return a string representation of expreval( string )Evaluate string as an expressionPython Programming, 1/eAssigning Input Input: gets input from the user andstores it into a variable. variable raw input( prompt ) The raw input function ALWAYSreturns a String (but you can convert itto a number)Assigning Input First the prompt is evaluated The program waits for the user to enter avalue and press enter The expression that was entered is assignedto the input variable as a string.8
How to find information yourself To the Python docs!– http://docs.python.org/ Library Reference – kinda hard to read http://docs.python.org/library/index.html Language Reference – very easy toread http://docs.python.org/reference/index.htmlString Methods you should know upperlowerreplacecountfindisdigitsplitPython Programming, 1/eUse the dot operator to call afunction that is part of a class:firstName “Dan”yellName firstName.upper()Strings: Can there be more? Lets try and see what else we can findabout them in the PythonDocumentation. What Methods are supported?– How can find if a string is all numbers?– How can I find the index of character “h” in“Python”?Using non-built in modules The math module adds more functionsto use like cosine and sine, square root,etc Lets try it! Math is NOT built-in (like String) so weneed to tell Python we want to use it9
Using Python’s Modules Python has a lot of code available inmodules for you to use Using modules, you must “import” them.Import Statement Importing a module tells the Pythoninterpreter you plan to use some or allof the function definted in that module. To use those functions though, youmust prepend the name of the module: import math Then call function XYZ defined in themath module as:– someVar math.XYZ()String Concatenation andMultiplicationWhat modules are available? Many! Find info in the module index pStr “Python”rStr “Rocks”prStr pStr rStrThis is string concatenation – adding twostrings to get a new string pMulti pStr * 5 # Use variable pMulti “Hello” * 3 # Use a literal value This is string “multiplication”, says create anew string by concatenating 5 in a rowPython Programming, 1/e10
Another way to print – Fill in the blank numCats 5Placeholder for ints numDogs 7 print "There were %d cats and %d dogs" %(numCats, numDogs)There were 5 cats and 7 dogs Printing is the SAME as creating a new string. You are really formatting theString and then printing it. This also works: myString "There were %d cats and %d dogs" %(numCats,numDogs) print myStringThere were 5 cats and 7 dogsInside the String you can put placeholders for other values. The placeholdersspecify a type.%d Signed integer%f Floating point (decimal format)%s StringString formatsString formatsYou can also specify a minimum fieldwidth like this: “%20d” . This will forcethe number to take up 20 spaces. print “Num 1 %10f” %(123.456)Num 1 123.456000To print in columns:print “Col1Col2”print “%10f%10f” %(12.23, 222.45)print “%10f%10f” %(444.55, 0A few final words on Math Shortcut operators are available:Lots of other String formats are found string-formatting–x x 1– x 1– someVariable 13 * someVariable– someVariable * 13– * / - % – Do the operation on the current variable,and save the result.Python Programming, 1/e11
Number Bases Decimal: Digits 0-9 Binary: Digits 0-1 Hexadecimal: Digit 0-9, A-F23 (2*101) (3*100) # Decimal (base 10)3001 (3*103) (0*102) (0*101) (1*100)In base 10 the red digits can be what?Binary: Base 223 2*101 3*100 # Decimal (base 10) 26 6423 16 4 2 1 25 32 (1*24) (0*23) (1*22) (1*21) (1*20) 0b10111 24 1648 (1*25) (1*24) (0*23) (0*22) (0*21) (0*20) 23 8 0b11000 22 40b101 (1*22) (0*21) (1*20) 51 2 20b1101 (1*23) (1*22) (0*21) (1*20) 13 20 1Binary: Base 223 2*101 3*100 # Decimal (base 10) 26 6423 16 4 2 1 25 32 (1*24) (0*23) (1*22) (1*21) (1*20) 0b10111 24 1648 (1*25) (1*24) (0*23) (0*22) (0*21) (0*20) 23 8 0b11000 22 40b101 (1*22) (0*21) (1*20) 5 21 20b1101 (1*23) (1*22) (0*21) (1*20) 13 20 1Python Programming, 1/eHexadecimal: Base 16Digits: 0-9, A-F: A 10, B 11, C 12,. F 15 163 4096162 256161 16160 123 16 4 (1*161) (4*160) 0x1448 (3*161) (0*160) 0x30250 (15*161) (10*160) 0xFA163 (10*161) (3*160) 0xA312
Hexadecimal: Base 16Digits: 0-9, A-F: A 10, B 11, C 12,. F 15163 4096 162 256 161 16 160 123 16 4 (1*161) (4*160) 0x1448 (3*161) (0*160) 0x30250 (15*161) (10*160) 0xFAGeneral Approach: Decimal to Hex From Base 10 to Base 161.2.3.4.5.6.7.Divide the decimal number by 16.Treat the division as an integer division.Write down the remainder (in hexadecimal).Divide the result again by 16.Treat the division as an integer division.Repeat step 2 and 3 until result is 0.The hex value is the digit sequence of theremainders from the last to first.163 (10*161) (3*160) 0xA3From here: l Approach: Decimal to Binary Same as last slide, but replace 16 with 2.In Python: Output Strings You need to display numbers as binaryor hexadecimal strings: x 32 xHex hex(32) #What data type is xHex? xBin bin(32) # Only in Python 2.6 Python Programming, 1/e13
In Python: Input From UserIt’s all about the jokes You need to input different bases.Python knows how to make a numberfrom the string using “eval”. There are 10 kinds of people in thisworld, those who know binary and thosewho don’t. x eval(‘0x10’) x eval(‘0b10’) x eval(‘10’) (I’ll be here all week.)Python Programming, 1/e# What is x? What data type?How do I ask a user fora String?14
Python Programming, 1/e 1 Coming up: The Software Development Process 1 Python Programming: An Introduction to Computer Science Chapter 2 Dan Fleck The Software Development Process The process of creating a program is often broken down into stages according to the information