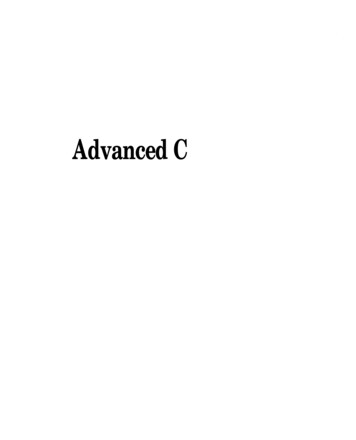
Transcription
IntroductionCCCCCCC CCCAdvanced Ci
Advanced Cii
C C CCCCCC CCIntroductionCCCCCCC CCAdvanced CPeter D. HipsonA Division of Prentice Hall Computer Publishing201 W. 103rd St., Indianapolis, Indiana 46290 USAiii
Advanced C 1992 by Sams PublishingAll rights reserved. Printed in the United States of America. No part of this book may be used orreproduced in any form or by any means, or stored in a database or retrieval system, without priorwritten permission of the publisher except in the case of brief quotations embodied in critical articlesand reviews. Making copies of any part of this book for any purpose other than your own personaluse is a violation of United States copyright laws. For information, address Sams Publishing, 201 W.103rd St., Indianapolis, IN 46290International Standard Book Number: 0-672-30168-7Library of Congress Catalog Card Number: 92-06130496 95 94 93 928 7 6 5 4 3Interpretation of the printing code: the rightmost double-digit number is the year of the book’sprinting; the rightmost single-digit number, the number of the book’s printing. For example, aprinting code of 92-1 shows that the first printing of the book occurred in 1992.Composed in AGaramond and MCPdigital by Prentice Hall Computer Publishing.Screen reproductions in this book were created by means of the program Collage Plus,from Inner Media, Inc., Hollis, NH.TrademarksAll terms mentioned in this book that are known to be trademarks or service markshave been appropriately capitalized. Sams Publishing cannot attest to the accuracy ofthis information. Use of a term in this book should not be regarded as affecting thevalidity of any trademark or service mark.iv
IntroductionPublisherCCCCCCC CCCProduction AnalystRichard K. SwadleyAcquisitions ManagerMary Beth WakefieldBook DesignJordan GoldManaging EditorMichele LaseauCover ArtNeweleen A. TrebnikAcquisitions EditorTim AmrheinGraphic Images SpecialistStacy HiquetProduction EditorDennis SheehanProductionMary CorderKaty BodenmillerChristine CookLisa DaughertyDenny HagerCarla Hall-BattonJohn KaneRoger MorganJuli PaveyAngela PozdolLinda QuigleyMichele SelfSusan ShepardGreg SimsicAlyssa YeshTechnical ReviewerTimothy C. MooreEditorial AssistantsRosemarie GrahamLori KelleyFormatterPat WhitmerProduction DirectorJeff VallerIndexProduction ManagerHilary AdamsCorinne WallsImprint ManagerMatthew MorrillProofreading/Indexing CoordinatorJoelynn Giffordv
Advanced CAbout the AuthorPeter Hipson and his wife live and work in New Hampshire. He has worked withcomputers since 1972, in hardware design and software development. He hasdeveloped numerous software programs for both PCs and larger systems. He holdspatents in the field of CPU design and has been involved with microcomputers sincetheir inception. Peter is the developer of the Windows applications STARmanager andSTARmanager A/E.You can contact Peter Hipson at P.O. Box 88, West Peterborough, NH, 03468.Enclosing an SASE greatly enhances the likelihood of a reply.To Bianca, who has shown me what great fun it is having a granddaughter.vi
IntroductionCCCCCCC CCCOverviewIntroduction . xxiiiPart I Honing Your C Skills11The C Philosophy . 32Data Types, Constants, Variables, and Arrays . 193Pointers and Indirection . 654Special Pointers and Their Usage . 995Decimal, Binary, Hex, and Octal . 1396Separate Compilation and Linking . 161Part II Managing Data in C1897C Structures . 1918Dynamic Memory Allocation . 2279Disk Files and Other I/O . 24910Data Management: Sorts, Lists, and Indexes . 321Part III Working with Others43311C and Other Langauages . 43512C and Databases . 46713All About Header Files . 497vii
Advanced CPart IV Documenting the Differences51914ANSI C’s Library Functions . 52115Preprocessor Directives . 62116Debugging and Efficiency . 641Part V Appendixes677AThe ASCII Character Set . 679BCompiler Variations . 681CIntroduction to C . 695DFunction/Header File Cross Reference . 723Index . 741viii
IntroductionCCCCCCC CCCContentsIntroduction . xxiiiPart I: Honing Your C Skills . 11The C Philosophy . 3A Brief History of C and the Standard . 3A Programming Style . 11Memory Models . 17Summary . 182Data Types, Constants, Variables, and Arrays . 19Data Types . 19Constants . 25Definitions versus Declarations . 29Declarations . 30Definitions . 33Variables . 35Variable Types and Initializing Variables . 35Scope (Or I Can See You) . 37Life Span (Or How Long Is It Going To Be Here?) . 39Type Casting . 41Arrays . 46Declaration of Arrays . 46Definition of an Array . 47Array Indexing . 48Using Array Names as Pointers . 55Strings: Character Arrays . 56Using Arrays of Pointers . 58Summary . 62ix
Advanced C3Pointers and Indirection . 65Pointers, Indirection, and Arrays . 65Pointers . 66Indirection . 69An Example of Pointers, Indirection,and Arrays . 69Character Arrays and Strings . 74Indirection to Access Character Strings . 79Protecting Strings in Memory . 90Ragged-Right String Arrays . 92Summary . 984Special Pointers and Their Use . 99Command Line Arguments . 99Function Pointers . 114Menus and Pointers. 120State Machines . 135Summary . 1375Decimal, Binary, Hex, and Octal . 139Decimal. 139Binary . 141Hex . 142Octal . 144Looking at a File . 146Bit Operators . 154Bit Fields . 155Summary . 1586Separate Compilation and Linking . 161Compiling and Linking Multiple Source Files . 162Compiling Multifile Programs . 164Linking Multifile Programs . 164Using #include . 166External Variables . 171Using an Object Library Manager . 181Using MAKE Files . 182Summary . 186x
TableIntroductionof ContentsPart II: Managing Data in C7CCCCCCC CCC189C Structures . 191Using the struct Keyword . 191Arrays of Structures . 195Structures of Arrays . 200Structures of Structures . 203Bit Fields in Structures . 206Using the typedef Keyword . 208Using the offsetof() Macro . 213Pointers to Structures . 216Understanding unions . 219Summary . 2268Dynamic Memory Allocation . 227Using the malloc( ) Function. 228Using the calloc( ) Function . 232Using the free( ) Function . 235Using the realloc( ) Function . 237Allocating Arrays . 244Global Memory versus Local Memory . 247Summary . 2489Disk Files and Other I/O . 249File I/O Basics . 250Text Files and Binary Files . 251Creating and Using Temporary Work Files . 256Stream Files and Default File Handles . 268The stdin File . 271The stdout File . 272The stderr File . 272The stdaux File . 273The stdprn File . 274Low-Level I/O and File Handles . 278Standard Low-Level File Handles . 280Console and Port I/O . 280Direct Port I/O . 288xi
Advanced CThe PC Printer Ports. 289The PC Communications Ports . 296Summary . 31810Data Management: Sorts, Lists, and Indexes . 321Sorting . 322Merging . 329Purging . 336Sorting, Merging, and Purging All in One . 343Linked Lists . 344Using Dynamic Memory . 345Disk-Based Lists . 346Double Linked Lists . 346Indexing . 367Fixed-field Disk Files . 392B-trees . 392Summary . 430Part III: Working with Others . 43311C and Other Languages . 435Other Languages . 436Assembly . 438FORTRAN . 441Pascal . 442BASIC . 443Calling Other Languages from C. 443Calling Assembly from C . 447Calling FORTRAN and Pascal from C . 449Calling C Functions from Other Languages . 450Calling C from Assembly . 451Calling C from FORTRAN and Pascal . 462All the Things that Can Go Wrong . 462Looking at Data . 463Names and Limits . 465Summary . 465xii
TableIntroductionof Contents12CCCCCCC CCCC and Databases . 467Interfacing with dBASE-Compatible Programs . 468Using dBASE Files Directly. 468Reading dBASE and dBASE-Compatible Files . 474Creating dBASE and dBASE-Compatible Files . 484Updating dBASE and dBASE-Compatible Files . 494Summary . 49413All About Header Files . 497Function Prototypes . 497The ANSI C Header Files . 500The assert.h File (ANSI) . 501The ctype.h File (ANSI) . 502The errno.h File (ANSI) . 504The float.h File (ANSI) . 506The io.h File . 508The limits.h File (ANSI) . 508The locale.h File (ANSI) . 509The malloc.h File . 510The math.h File (ANSI) . 510The memory.h File . 511The search.h File . 511The setjmp.h File (ANSI) . 512The signal.h File (ANSI) . 512The stdarg.h File (ANSI) . 513The stddef.h File (ANSI) . 515The stdio.h File (ANSI). 515The stdlib.h File (ANSI) . 516String Conversion . 516Memory Allocation . 516Random Numbers . 516Communications with the Operating System. 516Search Functions . 517Integer Math . 517Multibyte Characters . 517The string.h File (ANSI) . 517The time.h File (ANSI) . 518The varargs.h File . 518Summary . 518xiii
Advanced CPart IV: Documenting the Differences14519ANSI C’s Library Functions . 521Functions . 522abort(). 522abs() . 522acos() . 523asctime() . 523asin() . 524assert() . 524atan() . 524atan2() . 525atexit() . 525atof() . 526atoi() . 526atol() . 526bsearch() . 527calloc() . 528ceil(). 528clearerr() . 528clock() . 529cos() . 529cosh() . 530ctime() . 530difftime() . 531div() . 531exit() . 532exp() . 532fabs() . 533fclose() . 533feof() . 533ferror() . 534fflush() . 534fgetc() . 535fgetpos() . 535fgets() . 536floor() . 536xiv
TableIntroductionof ContentsCCCCCCC CCCfmod() . 537fopen() . 537fprintf() . 538fputc() . 538fputs() . 539fread() . 539free() . 540freopen() . 540frexp() . 541fscanf() . 542fseek() . 542fsetpos() . 543ftell() . 544fwrite() . 544getc(). 545getchar(). 545gets() . 546gmtime() . 546isalnum() . 547isalpha() . 547iscntrl() . 547isdigit() . 548isgraph(). 548islower() . 549isprint() . 549ispunct() . 549isspace() . 550isupper() . 551isxdigit(). 551labs() . 551ldexp() . 552ldiv() . 552localeconv() . 553localtime() . 553log() . 554log10() . 554longjmp() . 554malloc() . 556xv
Advanced Cmblen() . 556mbstowcs(). 557mbtowc() . 557memchr() . 558memcmp() . 558memcpy() . 559memmove() . 560memset() . 561mktime() . 561modf() . 562offsetof() . 562perror() . 563pow() . 564printf() . 564putc() . 564putchar() . 565puts() . 565qsort() . 566raise() . 566rand() . 567realloc() . 567remove() . 568rename() . 568rewind() . 568scanf() . 569setbuf() . 569setjmp() . 570setlocale() . 571setvbuf() .
Advanced C vi About the Author Peter Hipson and his wife live and work in New Hampshire. He has worked with computers