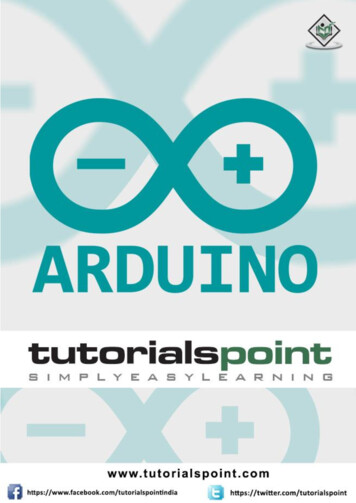
Transcription
About the TutorialArduino is a prototype platform (open-source) based on an easy-to-use hardware andsoftware. It consists of a circuit board, which can be programed (referred to asa microcontroller) and a ready-made software called Arduino IDE (IntegratedDevelopment Environment), which is used to write and upload the computer code to thephysical board.Arduino provides a standard form factor that breaks the functions of the micro-controllerinto a more accessible package.AudienceThis tutorial is intended for enthusiastic students or hobbyists. With Arduino, one can getto know the basics of micro-controllers and sensors very quickly and can start buildingprototype with very little investment.PrerequisitesBefore you start proceeding with this tutorial, we assume that you are already familiarwith the basics of C and C . If you are not well aware of these concepts, then we willsuggest you go through our short tutorials on C and C . A basic understanding ofmicrocontrollers and electronics is also expected.Copyright & Disclaimer Copyright 2016 by Tutorials Point (I) Pvt. Ltd.All the content and graphics published in this e-book are the property of Tutorials Point (I)Pvt. Ltd. The user of this e-book is prohibited to reuse, retain, copy, distribute or republishany contents or a part of contents of this e-book in any manner without written consentof the publisher.We strive to update the contents of our website and tutorials as timely and as precisely aspossible, however, the contents may contain inaccuracies or errors. Tutorials Point (I) Pvt.Ltd. provides no guarantee regarding the accuracy, timeliness or completeness of ourwebsite or its contents including this tutorial. If you discover any errors on our website orin this tutorial, please notify us at contact@tutorialspoint.comi
Table of ContentsAbout the Tutorial . iAudience . iPrerequisites . iCopyright & Disclaimer . iTable of Contents . iiARDUINO – BASICS. 1Arduino – Overview . 2Board Types . 3Arduino – Board Description . 6Arduino – Installation . 9Arduino – Program Structure . 17Arduino – Data Types . 19void . 19Boolean . 19Char . 20unsigned char . 21byte. 21int 21Unsigned int . 21Word . 21Long . 22unsigned long . 22short . 22float . 22double. 23Arduino – Variables & Constants . 24What is Variable Scope? . 24Arduino – Operators . 26Arithmetic Operators. 26Comparison Operators . 27Boolean Operators . 29Bitwise Operators . 30Compound Operators . 31Arduino – Control Statements. 33if statement . 34If else statement . 35if else if else statement . 37Switch Case Statement . 39Conditional Operator ? : . 41Rules of Conditional Operator . 41Arduino – Loops . 42ii
while loop . 42do while loop . 43for loop . 44Nested Loop . 45Infinite loop . 45Arduino - Functions. 47Function Declaration . 48Arduino – Strings . 51String Character Arrays. 51Manipulating String Arrays . 52Functions to Manipulate String Arrays . 54Array Bounds . 58Arduino – String Object . 59What is an Object? . 59When to Use a String Object . 61Arduino – Time . 62delay() function . 62delayMicroseconds() function . 63millis() function . 63micros() function . 64Arduino – Arrays . 66Declaring Arrays . 67Examples Using Arrays . 67Arduino – Passing Arrays to Functions . 70Multidimensional Arrays . 73ARDUINO – FUNCTION LIBRARIES . 78Arduino – I/O Functions . 79Pins Configured as INPUT . 79Pull-up Resistors . 79Pins Configured as OUTPUT . 80pinMode() Function . 80digitalWrite() Function . 81analogRead( ) function . 82Arduino – Advanced I/O Function . 84analogReference() Function . 84Arduino – Character Functions . 86Examples. 87Arduino – Math Library . 93Library Macros . 93Library Functions . 95Example . 99Arduino – Trigonometric Functions . 101iii
ARDUINO ADVANCED. 102Arduino – Due & Zero . 103Arduino Zero . 104Arduino – Pulse Width Modulation . 106Basic Principle of PWM . 106analogWrite() Function. 107Arduino – Random Numbers . 109randomSeed (seed) . 109random( ) . 109Bits . 110Bytes . 110Arduino – Interrupts . 112Types of Interrupts . 113Arduino – Communication . 115Parallel Communication . 115Serial Communication Modules . 115Types of Serial Communications . 116Arduino UART . 117Arduino – Inter Integrated Circuit . 119Board I2C Pins . 119Arduino I2C . 119Master Transmitter / Slave Receiver . 120Master Receiver / Slave Transmitter . 121Arduino – Serial Peripheral Interface . 123Board SPI Pins . 123SPI as MASTER . 124SPI as SLAVE . 125ARDUINO – PROJECTS . 127Arduino – Blinking LED . 128Arduino – Fading LED . 132Arduino – Reading Analog Voltage . 137Arduino – LED Bar Graph. 141Arduino – Keyboard Logout . 145Arduino – Keyboard Message . 151Arduino – Mouse Button Control . 154Arduino – Keyboard Serial . 158iv
ARDUINO SENSORS . 161Arduino – Humidity Sensor . 162Arduino – Temperature Sensor . 168Arduino – Water Detector / Sensor . 171Arduino – PIR Sensor . 174Arduino – Ultrasonic Sensor . 179Arduino – Connecting Switch . 183ARDUINO MOTOR CONTROL . 187Arduino – DC Motor . 188Motor Speed Control . 190Spin Direction Control . 192Arduino – Servo Motor . 196Arduino – Stepper Motor . 201ARDUINO AND SOUND . 205Arduino – Tone Library . 206Arduino – Wireless Communication . 212Arduino – Network Communication . 217v
Arduino – Basics6
ARDUINO – OVERVIEWArduino is a prototype platform (open-source) based on an easy-to-use hardware andsoftware. It consists of a circuit board, which can be programed (referred to asa microcontroller) and a ready-made software called Arduino IDE (Integrated DevelopmentEnvironment), which is used to write and upload the computer code to the physical board.The key features are: Arduino boards are able to read analog or digital input signals from different sensorsand turn it into an output such as activating a motor, turning LED on/off, connect tothe cloud and many other actions. You can control your board functions by sending a set of instructions to themicrocontroller on the board via Arduino IDE (referred to as uploading software). Unlike most previous programmable circuit boards, Arduino does not need an extrapiece of hardware (called a programmer) in order to load a new code onto the board.You can simply use a USB cable. Additionally, the Arduino IDE uses a simplified version of C , making it easier tolearn to program. Finally, Arduino provides a standard form factor that breaks the functions of the microcontroller into a more accessible package.7
Board TypesVarious kinds of Arduino boards are available depending on different microcontrollers used.However, all Arduino boards have one thing in common: they are programed through theArduino IDE.The differences are based on the number of inputs and outputs (the number of sensors, LEDs,and buttons you can use on a single board), speed, operating voltage, form factor etc. Someboards are designed to be embedded and have no programming interface (hardware), whichyou would need to buy separately. Some can run directly from a 3.7V battery, others need atleast 5V.8
Here is a list of different Arduino boards available.Arduino boards based on ATMEGA328 microcontrollerBoardNameArduino PWMUART5V16MHz14661Arduino UnoR3 SMD5V16MHz14661USB viaATMega16U2Red Board5V16MHz14661USB via FTDIArduino Pro3.3v/8 MHz3.3V8 MHz14661Arduino Pro5V/16MHz5V16MHz14661Arduino 146613.3V8MHz148613.3V8MHz146613.3V8MHz9450Arduino Promini3.3v/8mhzArduino Promini5v/16mhzArduinoEthernetArduino FioLilyPadArduino 328main boardLilyPadArduinosimply boardProgrammingInterfaceUSB derFTDICompatibleHeaderFTDICompatibleHeaderArduino boards based on ATMEGA32u4 microcontrollerBoard WMUARTProgrammingInterface9
ArduinoLeonardoPro micro5V/16MHzPro micro3.3V/8MHzLilyPadArduino USB5V16MHz201271Native USB5V16MHz14661Native USB5V16MHz14661Native USB3.3V8MHz14661Native USBArduino boards based on ATMEGA2560 microcontrollerBoardNameArduinoMega sPWMUART5V16MHz5416144Mega Pro3.3V3.3V8MHz5416144Mega Pro5V5V16MHz5416144Mega ProMini3.3V3.3V8MHz5416144ProgrammingInterfaceUSB derFTDICompatibleHeaderArduino boards based on AT91SAM3X8E erface3.3V84MHz5412124USB native10
ARDUINO – BOARD DESCRIPTIONIn this chapter, we will learn about the different components on the Arduino board. We willstudy the Arduino UNO board because it is the most popular board in the Arduino board family.In addition, it is the best board to get started with electronics and coding. Some boards looka bit different from the one given below, but most Arduinos have majority of thesecomponents in common.Power USB11
Arduino board can be powered by using the USB cable from your computer. All you need todo is connect the USB cable to the USB connection (1).Power (Barrel Jack)Arduino boards can be powered directly from the AC mains power supply by connecting it tothe Barrel Jack (2).Voltage RegulatorThe function of the voltage regulator is to control the voltage given to the Arduino board andstabilize the DC voltages used by the processor and other elements.Crystal OscillatorThe crystal oscillator helps Arduino in dealing with time issues. How does Arduino calculatetime? The answer is, by using the crystal oscillator. The number printed on top of the Arduinocrystal is 16.000H9H. It tells us that the frequency is 16,000,000 Hertz or 16 MHz.Arduino ResetYou can reset your Arduino board, i.e., start your program from the beginning. You can resetthe UNO board in two ways. First, by using the reset button (17) on the board. Second, youcan connect an external reset button to the Arduino pin labelled RESET (5).Pins (3.3, 5, GND, Vin) 3.3V (6): Supply 3.3 output volt Most of the components used with Arduino board works fine with 3.3 volt and5 volt.5V (7): Supply 5 output volt GND (8)(Ground): There are several GND pins on the Arduino, any of whichcan be used to ground your circuit. Vin (9): This pin also can be used to power the Arduino board from an externalpower source, like AC mains power supply. 12
Analog pinsThe Arduino UNO board has five analog input pins A0 through A5. These pins can read thesignal from an analog sensor like the humidity sensor or temperature sensor and convert itinto a digital value that can be read by the microprocessor.Main microcontrollerEach Arduino board has its own microcontroller (11). You can assume it as the brain of yourboard. The main IC (integrated circuit) on the Arduino is slightly different from board to board.The microcontrollers are usually of the ATMEL Company. You must know what IC your boardhas before loading up a new program from the Arduino IDE. This information is available onthe top of the IC. For more details about the IC construction and functions, you can refer tothe data sheet.ICSP pinMostly, ICSP (12) is an AVR, a tiny programming header for the Arduino consisting of MOSI,MISO, SCK, RESET, VCC, and GND. It is often referred to as an SPI (Serial PeripheralInterface), which could be considered as an "expansion" of the output. Actually, you areslaving the output device to the master of the SPI bus.Power LED indicatorThis LED should light up when you plug your Arduino into a power source to indicate that yourboard is powered up correctly. If this light does not turn on, then there is something wrongwith the connection.TX and RX LEDsOn your board, you will find two labels: TX (transmit) and RX (receive). They appear in twoplaces on the Arduino UNO board. First, at the digital pins 0 and 1, to indicate the pinsresponsible for serial communication. Second, the TX and RX led (13). The TX led flashes withdifferent speed while sending the serial data. The speed of flashing depends on the baud rateused by the board. RX flashes during the receiving process.Digital I / OThe Arduino UNO board has 14 digital I/O pins (15) (of which 6 provide PWM (Pulse WidthModulation) output. These pins can be configured to work as input digital pins to read logic13
values (0 or 1) or as digital output pins to drive different modules like LEDs, relays, etc. Thepins labeled “ ” can be used to generate PWM.AREFAREF stands for Analog Reference. It is sometimes, used to set an external reference voltage(between 0 and 5 Volts) as the upper limit for the analog input pins.14
ARDUINO – INSTALLATIONAfter learning about the main parts of
Programming Interface Arduino Due 3.3V 84MHz 54 12 12 4 USB native . 11 In this chapter, we will learn about the different components on the Arduino board. We will study the Arduino UNO board because it is the most popular board in the Arduino board family. In addition, it is the b