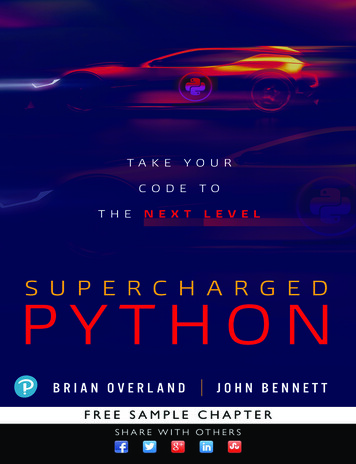
Transcription
Supercharged PythonOverland Book.indb i4/30/19 1:37 PM
This page intentionally left blankOverland Book.indb 6344/30/19 1:38 PM
Supercharged PythonBrian OverlandJohn BennettBoston Columbus New York San Francisco Amsterdam Cape TownDubai London Madrid Milan Munich Paris Montreal Toronto Delhi Mexico CitySão Paulo Sydney Hong Kong Seoul Singapore Taipei TokyoOverland Book.indb iii4/30/19 1:37 PM
Many of the designations used by manufacturers and sellers to distinguish their products areclaimed as trademarks. Where those designations appear in this book, and the publisher wasaware of a trademark claim, the designations have been printed with initial capital letters orin all capitals.The authors and publisher have taken care in the preparation of this book, but make noexpressed or implied warranty of any kind and assume no responsibility for errors or omissions. No liability is assumed for incidental or consequential damages in connection with orarising out of the use of the information or programs contained herein.For information about buying this title in bulk quantities, or for special sales opportunities(which may include electronic versions; custom cover designs; and content particular to yourbusiness, training goals, marketing focus, or branding interests), please contact our corporatesales department at corpsales@pearsoned.com or (800) 382-3419.For government sales inquiries, please contact governmentsales@pearsoned.com.For questions about sales outside the U.S., please contact intlcs@pearson.com.Visit us on the Web: informit.com/awLibrary of Congress Control Number: 2019936408Copyright 2019 Pearson Education, Inc.Cover illustration: Open Studio/ShutterstockAll rights reserved. This publication is protected by copyright, and permission must be obtainedfrom the publisher prior to any prohibited reproduction, storage in a retrieval system, or transmission in any form or by any means, electronic, mechanical, photocopying, recording, or likewise.For information regarding permissions, request forms and the appropriate contacts within thePearson Education Global Rights & Permissions Department, please visit www.pearsoned.com/permissions/.ISBN-13: 978-0-13-515994-1ISBN-10: 0-13-515994-61Overland Book.indb iv194/30/19 1:37 PM
To my beautiful and brilliant mother, Betty P. M. Overland. . . .All the world is mad except for me and thee. Stay a little.—BrianTo my parents, who did so much to shape who I am.—JohnOverland Book.indb v4/30/19 1:37 PM
This page intentionally left blankOverland Book.indb 6344/30/19 1:38 PM
ContentsChapter 1PrefacexxiiiWhat Makes Python Special?Paths to Learning: Where Do I Start?Clarity and Examples Are EverythingLearning Aids: IconsWhat You’ll LearnHave FunxxiiixxivxxivxxvxxvixxviAcknowledgmentsAbout the AuthorsxxviiReview of the Fundamentals1.11.21.31.41.51.61.71.81.91.10Python Quick StartVariables and Naming NamesCombined Assignment OperatorsSummary of Python Arithmetic OperatorsElementary Data Types: Integer and Floating PointBasic Input and OutputFunction DefinitionsThe Python “if” StatementThe Python “while” StatementA Couple of Cool Little Appsxxix11445679111214viiOverland Book.indb vii4/30/19 1:37 PM
viiiChapter 2Overland Book.indb viiiContents1.11 Summary of Python Boolean Operators1.12 Function Arguments and Return Values1.13 The Forward Reference Problem1.14 Python Strings1.15 Python Lists (and a Cool Sorting App)1.16 The “for” Statement and Ranges1.17 Tuples1.18 Dictionaries1.19 Sets1.20 Global and Local VariablesSummaryReview QuestionsSuggested Problems15161919212325262829313132Advanced String Capabilities332.1 Strings Are Immutable2.2 Numeric Conversions, Including Binary2.3 String Operators ( , , *, , etc.)2.4 Indexing and Slicing2.5 Single-Character Functions (Character Codes)2.6 Building Strings Using “join”2.7 Important String Functions2.8 Binary, Hex, and Octal Conversion Functions2.9 Simple Boolean (“is”) Methods2.10 Case Conversion Methods2.11 Search-and-Replace Methods2.12 Breaking Up Input Using “split”2.13 Stripping2.14 Justification MethodsSummaryReview QuestionsSuggested Problems33343639424446474849505354555657574/30/19 1:37 PM
ContentsChapter 3Chapter 4Advanced List Capabilities593.1 Creating and Using Python Lists3.2 Copying Lists Versus Copying List Variables3.3 Indexing3.3.1 Positive Indexes3.3.2 Negative Indexes3.3.3 Generating Index Numbers Using “enumerate”3.4 Getting Data from Slices3.5 Assigning into Slices3.6 List Operators3.7 Shallow Versus Deep Copying3.8 List Functions3.9 List Methods: Modifying a List3.10 List Methods: Getting Information on Contents3.11 List Methods: Reorganizing3.12 Lists as Stacks: RPN Application3.13 The “reduce” Function3.14 Lambda Functions3.15 List Comprehension3.16 Dictionary and Set Comprehension3.17 Passing Arguments Through a List3.18 Multidimensional Lists3.18.1 Unbalanced Matrixes3.18.2 Creating Arbitrarily Large MatrixesSummaryReview QuestionsSuggested 9191939394Shortcuts, Command Line, and Packages954.1 Overview4.2 Twenty-Two Programming Shortcuts4.2.1 Use Python Line Continuation as Needed4.2.2 Use “for” Loops Intelligently4.2.3 Understand Combined Operator Assignment ( etc.)Overland Book.indb ixix95959697984/30/19 1:37 PM
xContents4.2.4 Use Multiple Assignment4.2.5 Use Tuple Assignment4.2.6 Use Advanced Tuple Assignment4.2.7 Use List and String “Multiplication”4.2.8 Return Multiple Values4.2.9 Use Loops and the “else” Keyword4.2.10 Take Advantage of Boolean Values and “not”4.2.11 Treat Strings as Lists of Characters4.2.12 Eliminate Characters by Using “replace”4.2.13 Don’t Write Unnecessary Loops4.2.14 Use Chained Comparisons (n x m)4.2.15 Simulate “switch” with a Table of Functions4.2.16 Use the “is” Operator Correctly4.2.17 Use One-Line “for” Loops4.2.18 Squeeze Multiple Statements onto a Line4.2.19 Write One-Line if/then/else Statements4.2.20 Create Enum Values with “range”4.2.21 Reduce the Inefficiency of the “print” Function Within IDLE4.2.22 Place Underscores Inside Large Numbers4.3 Running Python from the Command Line4.3.1 Running on a Windows-Based System4.3.2 Running on a Macintosh System4.3.3 Using pip or pip3 to Download Packages4.4 Writing and Using Doc Strings4.5 Importing Packages4.6 A Guided Tour of Python Packages4.7 Functions as First-Class Objects4.8 Variable-Length Argument Lists4.8.1 The *args List4.8.2 The “**kwargs” List4.9 Decorators and Function Profilers4.10 Generators4.10.1 What’s an Iterator?4.10.2 Introducing Generators4.11 Accessing Command-Line ArgumentsSummaryQuestions for ReviewSuggested ProblemsOverland Book.indb 1331381411421424/30/19 1:37 PM
ContentsChapter 5Chapter 6Formatting Text Precisely1455.1 Formatting with the Percent Sign Operator (%)5.2 Percent Sign (%) Format Specifiers5.3 Percent Sign (%) Variable-Length Print Fields5.4 The Global “format” Function5.5 Introduction to the “format” Method5.6 Ordering by Position (Name or Number)5.7 “Repr” Versus String Conversion5.8 The “spec” Field of the “format” Function and Method5.8.1 Print-Field Width5.8.2 Text Justification: “fill” and “align” Characters5.8.3 The “sign” Character5.8.4 The Leading-Zero Character (0)5.8.5 Thousands Place Separator5.8.6 Controlling Precision5.8.7 “Precision” Used with Strings (Truncation)5.8.8 “Type” Specifiers5.8.9 Displaying in Binary Radix5.8.10 Displaying in Octal and Hex Radix5.8.11 Displaying Percentages5.8.12 Binary Radix Example5.9 Variable-Size FieldsSummaryReview QuestionsSuggested 172173174174175176176178179179Regular Expressions, Part I1816.1 Introduction to Regular Expressions6.2 A Practical Example: Phone Numbers6.3 Refining Matches6.4 How Regular Expressions Work: Compiling Versus Running6.5 Ignoring Case, and Other Function Flags6.6 Regular Expressions: Basic Syntax Summary6.6.1 Meta Characters6.6.2 Character SetsOverland Book.indb xixi1811831851881921931941954/30/19 1:37 PM
xiiChapter 7Chapter 8Contents6.6.3 Pattern Quantifiers6.6.4 Backtracking, Greedy, and Non-Greedy6.7 A Practical Regular-Expression Example6.8 Using the Match Object6.9 Searching a String for Patterns6.10 Iterative Searching (“findall”)6.11 The “findall” Method and the Grouping Problem6.12 Searching for Repeated Patterns6.13 Replacing TextSummaryReview QuestionsSuggested r Expressions, Part II2157.1 Summary of Advanced RegEx Grammar7.2 Noncapture Groups7.2.1 The Canonical Number Example7.2.2 Fixing the Tagging Problem7.3 Greedy Versus Non-Greedy Matching7.4 The Look-Ahead Feature7.5 Checking Multiple Patterns (Look-Ahead)7.6 Negative Look-Ahead7.7 Named Groups7.8 The “re.split” Function7.9 The Scanner Class and the RPN Project7.10 RPN: Doing Even More with ScannerSummaryReview QuestionsSuggested 244Text and Binary Files2458.1 Two Kinds of Files: Text and Binary8.1.1 Text Files8.1.2 Binary FilesOverland Book.indb xii2452462464/30/19 1:37 PM
ContentsChapter 98.2 Approaches to Binary Files: A Summary8.3 The File/Directory System8.4 Handling File-Opening Exceptions8.5 Using the “with” Keyword8.6 Summary of Read/Write Operations8.7 Text File Operations in Depth8.8 Using the File Pointer (“seek”)8.9 Reading Text into the RPN Project8.9.1 The RPN Interpreter to Date8.9.2 Reading RPN from a Text File8.9.3 Adding an Assignment Operator to RPN8.10 Direct Binary Read/Write8.11 Converting Data to Fixed-Length Fields (“struct”)8.11.1 Writing and Reading One Number at a Time8.11.2 Writing and Reading Several Numbers at a Time8.11.3 Writing and Reading a Fixed-Length String8.11.4 Writing and Reading a Variable-Length String8.11.5 Writing and Reading Strings and Numerics Together8.11.6 Low-Level Details: Big Endian Versus Little Endian8.12 Using the Pickling Package8.13 Using the “shelve” PackageSummaryReview QuestionsSuggested 272273274275276278280282283283Classes and Magic Methods2859.19.29.39.49.59.69.79.89.9Overland Book.indb xiiixiiiClasses and Objects: Basic SyntaxMore About Instance VariablesThe “ init ” and “ new ” MethodsClasses and the Forward Reference ProblemMethods GenerallyPublic and Private Variables and MethodsInheritanceMultiple InheritanceMagic Methods, Summarized2852872882892902922932942954/30/19 1:37 PM
xivChapter 10Overland Book.indb xivContents9.10 Magic Methods in Detail9.10.1 String Representation in Python Classes9.10.2 The Object Representation Methods9.10.3 Comparison Methods9.10.4 Arithmetic Operator Methods9.10.5 Unary Arithmetic Methods9.10.6 Reflection (Reverse-Order) Methods9.10.7 In-Place Operator Methods9.10.8 Conversion Methods9.10.9 Collection Class Methods9.10.10 Implementing “ iter ” and “ next ”9.11 Supporting Multiple Argument Types9.12 Setting and Getting Attributes DynamicallySummaryReview QuestionsSuggested 324325Decimal, Money, and Other Classes32710.1 Overview of Numeric Classes10.2 Limitations of Floating-Point Format10.3 Introducing the Decimal Class10.4 Special Operations on Decimal Objects10.5 A Decimal Class Application10.6 Designing a Money Class10.7 Writing the Basic Money Class (Containment)10.8 Displaying Money Objects (“ str ”, “ repr ”)10.9 Other Monetary Operations10.10 Demo: A Money Calculator10.11 Setting the Default Currency10.12 Money and Inheritance10.13 The Fraction Class10.14 The Complex ClassSummaryReview QuestionsSuggested 3573573584/30/19 1:37 PM
ContentsChapter 11Chapter 12Overland Book.indb xvxvThe Random and Math Packages35911.1 Overview of the Random Package11.2 A Tour of Random Functions11.3 Testing Random Behavior11.4 A Random-Integer Game11.5 Creating a Deck Object11.6 Adding Pictograms to the Deck11.7 Charting a Normal Distribution11.8 Writing Your Own Random-Number Generator11.8.1 Principles of Generating Random Numbers11.8.2 A Sample Generator11.9 Overview of the Math Package11.10 A Tour of Math Package Functions11.11 Using Special Values (pi)11.12 Trig Functions: Height of a Tree11.13 Logarithms: Number Guessing Revisited11.13.1 How Logarithms Work11.13.2 Applying a Logarithm to a Practical ProblemSummaryReview QuestionsSuggested 381381382385385386The “numpy” (Numeric Python) Package38712.1 Overview of the “array,” “numpy,” and “matplotlib” Packages12.1.1 The “array” Package12.1.2 The “numpy” Package12.1.3 The “numpy.random” Package12.1.4 The “matplotlib” Package12.2 Using the “array” Package12.3 Downloading and Importing “numpy”12.4 Introduction to “numpy”: Sum 1 to 1 Million12.5 Creating “numpy” Arrays12.5.1 The “array” Function (Conversion to an Array)12.5.2 The “arange” Function3873873873883883883903913923943964/30/19 1:37 PM
xviChapter 13Overland Book.indb xviContents12.5.3 The “linspace” Function12.5.4 The “empty” Function12.5.5 The “eye” Function12.5.6 The “ones” Function12.5.7 The “zeros” Function12.5.8 The “full” Function12.5.9 The “copy” Function12.5.10 The “fromfunction” Function12.6 Example: Creating a Multiplication Table12.7 Batch Operations on “numpy” Arrays12.8 Ordering a Slice of “numpy”12.9 Multidimensional Slicing12.10 Boolean Arrays: Mask Out That “numpy”!12.11 “numpy” and the Sieve of Eratosthenes12.12 Getting “numpy” Stats (Standard Deviation)12.13 Getting Data on “numpy” Rows and ColumnsSummaryReview QuestionsSuggested 419424429429430Advanced Uses of “numpy”43113.1 Advanced Math Operations with “numpy”13.2 Downloading “matplotlib”13.3 Plotting Lines with “numpy” and “matplotlib”13.4 Plotting More Than One Line13.5 Plotting Compound Interest13.6 Creating Histograms with “matplotlib”13.7 Circles and the Aspect Ratio13.8 Creating Pie Charts13.9 Doing Linear Algebra with “numpy”13.9.1 The Dot Product13.9.2 The Outer-Product Function13.9.3 Other Linear Algebra Functions13.10 Three-Dimensional Plotting13.11 “numpy” Financial 34644/30/19 1:37 PM
ContentsChapter 14Chapter 15Overland Book.indb xviixvii13.12 Adjusting Axes with “xticks” and “yticks”13.13 “numpy” Mixed-Data Records13.14 Reading and Writing “numpy” Data from FilesSummaryReview QuestionsSuggested Problems467469471475475476Multiple Modules and the RPN Example47714.1 Overview of Modules in Python14.2 Simple Two-Module Example14.3 Variations on the “import” Statement14.4 Using the “ all ” Symbol14.5 Public and Private Module Variables14.6 The Main Module and “ main ”14.7 Gotcha! Problems with Mutual Importing14.8 RPN Example: Breaking into Two Modules14.9 RPN Example: Adding I/O Directives14.10 Further Changes to the RPN Example14.10.1 Adding Line-Number Checking14.10.2 Adding Jump-If-Not-Zero14.10.3 Greater-Than ( ) and Get-Random-Number (!)14.11 RPN: Putting It All TogetherSummaryReview QuestionsSuggested 513514514Getting Financial Data off the 525Plan of This ChapterIntroducing the Pandas Package“stock load”: A Simple Data ReaderProducing a Simple Stock ChartAdding a Title and LegendWriting a “makeplot” Function (Refactoring)4/30/19 1:37 PM
xviiiContents15.7 Graphing Two Stocks Together15.8 Variations: Graphing Other Data15.9 Limiting the Time Period15.10 Split Charts: Subplot the Volume15.11 Adding a Moving-Average Line15.12 Giving Choices to the UserSummaryReview QuestionsSuggested Problems527530534536538540544545545Appendix APython Operator Precedence Table547Appendix BBuilt-In Python obj)bin(n)bool(obj)bytes(source, encoding)callable(obj)chr(n)compile(cmd str, filename, mode str, flags 0,dont inherit False, optimize –1)complex(real 0, imag 0)complex(complex str)delattr(obj, name str)dir([obj])divmod(a, b)enumerate(iterable, start 0)eval(expr str [, globals [, locals]] )exec(object [, global [, locals]])filter(function, iterable)float([x])format(obj, [format spec])frozenset([iterable])getattr(obj, name str [,default])Overland Book.indb 5565575585585595595605604/30/19 1:37 PM
Contentsglobals()hasattr(obj, name str)hash(obj)help([obj])hex(n)id(obj)input([prompt str])int(x, base 10)int()isinstance(obj, class)issubclass(class1, s()map(function, iterable1 [, iterable2 ])max(arg1 [, arg2] )max(iterable)min(arg1 [, arg2] )min(iterable)oct(n)open(file name str, mode 'rt')ord(char str)pow(x, y [, z])print(objects, sep '', end '\n', file sys.stdout)range(n)range(start, stop [, step])repr(obj)reversed(iterable)round(x [,ndigits])set([iterable])setattr(obj, name str, value)sorted(iterable [, key] [, reverse])str(obj '')str(obj b'' [, encoding 'utf-8'])sum(iterable [, terables)Overland Book.indb 735735735745745755755755754/30/19 1:37 PM
xxContentsAppendix CSet Methods577set obj.add(obj)set obj.clear()set obj.copy()set obj.difference(other set)set obj.difference update(other set)set obj.discard(obj)set obj.intersection(other set)set obj.intersection update(other set)set obj.isdisjoint(other set)set obj.issubset(other set)set obj.issuperset(other set)set obj.pop()set obj.remove(obj)set obj.symmetric difference(other set)set obj.symmetric difference update(other set)set obj.union(other set)set obj.union update(other 81581Dictionary Methods583dict obj.clear()dict obj.copy()dict obj.get(key obj, default val None)dict obj.items()dict obj.keys()dict obj.pop(key [, default value])dict obj.popitem()dict obj.setdefault(key, default value None)dict obj.values()dict Statement Reference587Variables and AssignmentsSpacing Issues in PythonAlphabetical Statement Referenceassert Statementbreak Statementclass Statement587589590590591591Appendix DAppendix EOverland Book.indb xx4/30/19 1:37 PM
Contentscontinue Statementdef Statementdel Statementelif Clauseelse Clauseexcept Clausefor Statementglobal Statementif Statementimport Statementnonlocal Statementpass Statementraise Statementreturn Statementtry Statementwhile Statementwith Statementyield StatementIndexOverland Book.indb 06026026036054/30/19 1:37 PM
This page intentionally left blankOverland Book.indb 6344/30/19 1:38 PM
PrefaceBooks on Python aimed for the absolute beginner have become a cottageindustry these days. Everyone and their dog, it seems, wants to chase thePython.We’re a little biased, but one book we especially recommend is PythonWithout Fear. It takes you by the hand and explains the major features oneat a time. But what do you do after you know a little of the language but notenough to call yourself an “expert”? How do you learn enough to get a job orto write major applications?That’s what this book is for: to be the second book you ever buy on Pythonand possibly the last.What Makes Python Special?It’s safe to say that many people are attracted to Python because it looks easier than C . That may be (at least in the beginning), but underneath thisso-called easy language is a tool of great power, with many shortcuts and software libraries called “packages” that—in some cases—do most of the workfor you. These let you create some really impressive software, outputtingbeautiful graphs and manipulating large amounts of data.For most people, it may take years to learn all the shortcuts and advancedfeatures. This book is written for people who want to get that knowledge now,to get closer to being a Python expert much faster.xxiiiOverland Book.indb xxiii4/30/19 1:37 PM
xxivPrefacePaths to Learning: Where Do I Start?This book offers different learning paths for different people. You’re rusty: If you’ve dabbled in Python but you’re a little rusty, you maywant to take a look at Chapter 1, “Review of the Fundamentals.” Otherwise,you may want to skip Chapter 1 or only take a brief look at it. You know the basics but are still learning: Start with Chapters 2 and 3, which survey the abilities of strings and lists. This survey includes some advanced abilitiesof these data structures that people often miss the first time they learn Python. Your understanding of Python is strong, but you don’t know everything yet:Start with Chapter 4, which lists 22 programming shortcuts unique to Python,that most people take a long time to fully learn. You want to master special features: You can start in an area of specialty. Forexample, Chapters 5, 6, and 7 deal with text formatting and regular expressions. The two chapters on regular expression syntax, Chapters 6 and 7, startwith the basics but then cover the finer points of this pattern-matching technology. Other chapters deal with other specialties. For example, Chapter 8describes the different ways of handling text and binary files. You want to learn advanced math and plotting software: If you want to doplotting, financial, or scientific applications, start with Chapter 12, “The ‘numpy’(Numeric Python) Package.” This is the basic package that provides an underlying basis for many higher-level capabilities described in Chapters 13 through 15.Clarity and Examples Are EverythingEven with advanced technology, our emphasis is on clarity, short examples,more clarity, and more examples. We emphasize an interactive approach,especially with the use of the IDLE environment, encouraging you to type instatements and see what they do. Text in bold represents lines for you to typein, or to be added or changed. print('Hello', 'my', 'world!')Hello my world!Several of the applications in this book are advanced pieces of software,including a Deck object, a fully functional “RPN” language interpreter, and amultifaceted stock-market program that presents the user with many choices.With these applications, we start with simple examples in the beginning,finally showing all the pieces in context. This approach differs from manyOverland Book.indb xxiv4/30/19 1:37 PM
xxvPrefacebooks, which give you dozens of functions all out of order, with no sense ofarchitecture. In this book, architecture is everything.You can download examples from brianoverland.com/books.Learning Aids: IconsThis book makes generous use of tables for ease of reference, as well as conceptual art (figures). Our experience is that while poorly conceived figures canbe a distraction, the best figures can be invaluable. A picture is worth a thousand words. Sometimes, more.We also believe that in discussing plotting and graphics software, there’s nosubstitute for showing all the relevant screen shots.The book itself uses a few important, typographical devices. There arethree special icons used in the text.Note Ë We sometimes use Notes to point out facts you’ll eventually want to knowbut that diverge from the main discussion. You might want to skip over Notes thefirst time you read a section, but it’s a good idea to go back later and read them.ÇKey SyntaxNoteThe Key Syntax Icon introduces general syntax displays, into which you supplysome or all of the elements. These elements are called “placeholders,” and theyappear in italics. Some of the syntax—especially keywords and punctuation—are in bold and intended to be typed in as shown. Finally, square brackets,when not in bold, indicate an optional item. For example:set([iterable])This syntax display implies that iterable is an iterable object (such as alist or a generator object) that you supply. And it’s optional.Square brackets, when in bold, are intended literally, to be typed in asshown. For example:list name [obj1, obj2, obj3, ]Ellipses ( ) indicate a language element that can be repeated any numberof times.PerformanceTipPerformance tips are like Notes in that they constitute a short digressionfrom the rest of the chapter. These tips address the question of how youcan improve software performance. If you’re interested in that topic, you’llwant to pay special attention to these notes.ÇOverland Book.indb xxvPerformance Tip4/30/19 1:37 PM
xxviPrefaceWhat You’ll LearnThe list of topics in this book that are not in Python Without Fear or other“beginner” texts is a long one, but here is a partial list of some of the majorareas: List, set, and dictionary comprehension. Regular expressions and advanced formatting techniques; how to use them inlexical analysis. Packages: the use of Python’s advanced numeric and plotting software. Also,special types such as Decimal and Fraction. Mastering all the ways of using binary file operations in Python, as well astext operations. How to use multiple modules in Python while avoiding the “gotchas.” Fine points of object-oriented programming, especially all the “magic methods,” their quirks, their special features, and their uses.Have FunWhen you master some or all of the techniques of this book, you should makea delightful discovery: Python often enables you to do a great deal with a relatively small amount of code. That’s why it’s dramatically increasing in popularity every day. Because Python is not just a time-saving device, it’s fun to beable to program this way . . . to see a few lines of code do so much.We wish you the joy of that discovery.Register your copy of Supercharged Python on the InformIT site for convenient access to updates and/or corrections as they become available. To startthe registration process, go to informit.com/register and log in or createan account. Enter the product ISBN (9780135159941) and click Submit.Look on the Registered Products tab for an Access Bonus Content linknext to this product, and follow that link to access any available bonusmaterials. If you would like to be notified of exclusive offers on new editions and updates, please check the box to receive email from us.Overland Book.indb xxvi4/30/19 1:37 PM
AcknowledgmentsFrom BrianI want to thank my coauthor, John Bennett. This book is the result of closecollaboration between the two of us over half a year, in which John was thereevery step of the way to contribute ideas, content, and sample code, so hispresence is there throughout the book. I also want to thank Greg Doench,acquisitions editor, who was a driving force behind the concept, purpose, andmarketing of this book.This book also had a wonderful supporting editorial team, includingRachel Paul and Julie Nahil. But I want to especially thank copy editor BetsyHardinger, who showed exceptional competence, cooperation, and professionalism in getting the book ready for publication.From JohnI want to thank my coauthor, Brian Overland, for inviting me to join him onthis book. This allows me to pass on many of the things I had to work hard tofind documentation for or figure out by brute-force experimentation. Hopefully this will save readers a lot of work dealing with the problems I ran into.xxviiOverland Book.indb xxvii4/30/19 1:37 PM
This page intentionally left blankOverland Book.indb 6344/30/19 1:38 PM
About the AuthorsBrian Overland started as a professional programmer back in his twenties,but also worked as a computer science, English, and math tutor. He enjoyspicking up new languages, but his specialty is explaining them to others, aswell as using programming to do games, puzzles, simulations, and math problems. Now he’s the author of over a dozen books on programming.In his ten years at Microsoft he was a software tester, programmer/writer,and manager, but his greatest achievement was in presenting Visual Basic 1.0,as lead writer and overall documentation project lead. He believes that projectchanged the world by getting people to develop for Windows, and one of thekeys to its success was showing it could be fun and easy.He’s also a playwright and actor, which has come in handy as an instructorin online classes. As a novelist, he’s twice been a finalist in the Pacific Northwest Literary Contest but is still looking for a publisher.John Bennett was a senior software engineer at Proximity Technology, FranklinElectronic Publishing, and Microsoft Corporation. More recently, he’s developed new programming languages using Python as a prototyping tool. Heholds nine U.S. patents, and his projects include a handheld spell checker andEast Asian handwriting recognition software.xxixOverland Book.indb xxix4/30/19 1:37 PM
This page intentionally left blankOverland Book.indb 6344/30/19 1:38 PM
Shortcuts,Command Line,and Packages4Master crafters need many things, but, above all, they need to master thetools of the profession. This chapter introduces tools that, even if you’re afairly experienced Python programmer, you may not have yet learned. Thesetools will make you more productive as well as increase the efficiency of yourprograms.So get ready to learn some new tips and tricks.4.1 OverviewPython is unusually gifted with shortcuts and time-saving programmingtechniques. This chapter begins with a discussion of twenty-two of thesetechniques.Another thing you can do to speed up certain programs is to take advantageof the many packages that are available with Python. Some of these—such asre (regular expressions), system, random, and math—come with the standard Python download, and all you have to do is to include an import statement. Other packages can be downloaded quite easily with the right tools.4.2 Twenty-Two Programming ShortcutsThis section lists the most common techniques for shortening and tighteningyour Python code. Most of these are new in the book, although a few of themhave been introduced b
4.3 Running Python from the Command Line 115 4.3.1 Running on a Windows-Based System 115 4.3.2 Running on a Macintosh System 116 4.3.3 Using pip or pip3 to Download Packages 117 4.4 Writing and Using Doc Strings 117 4.5 Importing Packages 119 4.6 A Guided Tour of Python