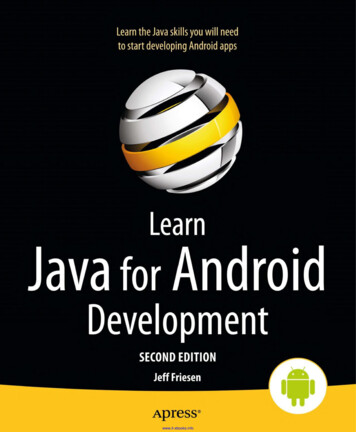
Transcription
www.it-ebooks.info
Learn Java for AndroidDevelopmentSecond EditionJeff FriesenApresswww.it-ebooks.info
Learn Java for Android DevelopmentCopyright 2013 by Jeff FriesenThis work is subject to copyright. All rights are reserved by the Publisher, whether the whole or part of the materialis concerned, specifically the rights of translation, reprinting, reuse of illustrations, recitation, broadcasting,reproduction on microfilms or in any other physical way, and transmission or information storage and retrieval,electronic adaptation, computer software, or by similar or dissimilar methodology now known or hereafter developed.Exempted from this legal reservation are brief excerpts in connection with reviews or scholarly analysis or materialsupplied specifically for the purpose of being entered and executed on a computer system, for exclusive use by thepurchaser of the work. Duplication of this publication or parts thereof is permitted only under the provisions of theCopyright Law of the Publisher's location, in its current version, and permission for use must always be obtained fromSpringer. Permissions for use may be obtained through RightsLink at the Copyright Clearance Center. Violations areliable to prosecution under the respective Copyright Law.ISBN 978-1-4302-5722-6ISBN 978-1-4302-5723-3 (eBook)Trademarked names, logos, and images may appear in this book. Rather than use a trademark symbol with everyoccurrence of a trademarked name, logo, or image we use the names, logos, and images only in an editorial fashionand to the benefit of the trademark owner, with no intention of infringement of the trademark.The use in this publication of trade names, trademarks, service marks, and similar terms, even if they are not identifiedas such, is not to be taken as an expression of opinion as to whether or not they are subject to proprietary rights.While the advice and information in this book are believed to be true and accurate at the date of publication, neitherthe authors nor the editors nor the publisher can accept any legal responsibility for any errors or omissions that maybe made. The publisher makes no warranty, express or implied, with respect to the material contained herein.President and Publisher: Paul ManningLead Editor: Steve AnglinDevelopmental Editors: Tom Welsh and Matthew MoodieTechnical Reviewers: Paul Connolly, Chad Darby and Onur CinarEditorial Board: Steve Anglin, Mark Beckner, Ewan Buckingham, Gary Cornell, Louise Corrigan, Morgan Ertel,Jonathan Gennick, Jonathan Hassell, Robert Hutchinson, Michelle Lowman, James Markham,Matthew Moodie, Jeff Olson, Jeffrey Pepper, Douglas Pundick, Ben Renow-Clarke, Dominic Shakeshaft,Gwenan Spearing, Matt Wade, Tom WelshCoordinating Editor: Katie SullivanCopy Editor: Deanna K. HegleCompositor: SPi GlobalIndexer: SPi GlobalArtist: SPi GlobalCover Designer: Anna IshchenkoDistributed to the book trade worldwide by Springer Science Business Media New York, 233 Spring Street, 6th Floor,New York, NY 10013. Phone 1-800-SPRINGER, fax (201) 348-4505, e-mail orders-ny@springer-sbm.com, or visitwww.springeronline.com. Apress Media, LLC is a California LLC and the sole member (owner) is Springer Science Business Media Finance Inc (SSBM Finance Inc). SSBM Finance Inc is a Delaware corporation.For information on translations, please e-mail rights@apress.com, or visit www.apress.com.Apress and friends of ED books may be purchased in bulk for academic, corporate, or promotional use. eBookversions and licenses are also available for most titles. For more information, reference our Special Bulk Sales–eBookLicensing web page at www.apress.com/bulk-sales.Any source code or other supplementary materials referenced by the author in this text is available to readers atwww.apress.com. For detailed information about how to locate your book’s source code, go towww.apress.com/source-code/.www.it-ebooks.info
To Amaury.www.it-ebooks.info
Contents at a GlanceAbout the Author . xviiAbout the Technical Reviewers . xixAcknowledgments . xxiIntroduction . xxiiiN Chapter 1: Getting Started With Java .1N Chapter 2: Learning Language Fundamentals .21N Chapter 3: Discovering Classes and Objects .63N Chapter 4: Discovering Inheritance, Polymorphism, and Interfaces .105N Chapter 5: Mastering Advanced Language Features Part 1 .153N Chapter 6: Mastering Advanced Language Features Part 2 .197N Chapter 7: Exploring the Basic APIs Part 1 .247N Chapter 8: Exploring the Basic APIs Part 2 .279N Chapter 9: Exploring the Collections Framework .327N Chapter 10: Exploring Additional Utility APIs .407N Chapter 11: Performing Classic I/O .449N Chapter 12: Accessing Networks .525vwww.it-ebooks.info
viContents at a GlanceN Chapter 13: Migrating to New I/O . 561N Chapter 14: Accessing Databases .603N Appendix A: Solutions to Exercises .643N Appendix B: Four of a Kind .713Index .735www.it-ebooks.info
ContentsAbout the Author . xviiAbout the Technical Reviewers . xixAcknowledgments . xxiIntroduction . xxiiiN Chapter 1: Getting Started With Java .1What Is Java? .2Java Is a Language . 2Java Is a Platform . 4Java SE, Java EE, Java ME, and Android . 5Installing and Exploring the JDK .6Installing and Exploring the Eclipse IDE .12Overview of Java APIs .16Language-Support and Other Language-Oriented APIs . 17Collections-Oriented APIs . 17Additional Utility APIs . 17Classic I/O APIs . 17Networking APIs . 18viiwww.it-ebooks.info
viiiContentsNew I/O APIs . 18Database APIs . 18Summary .19N Chapter 2: Learning Language Fundamentals .21Learning Comments .21Single-Line Comments . 22Multiline Comments. 22Javadoc Comments . 23Learning Identifiers .25Learning Types .26Primitive Types . 26User-Defined Types . 28Array Types . 28Learning Variables .29Learning Expressions .30Simple Expressions . 30Compound Expressions . 34Learning Statements .46Assignment Statements . 46Decision Statements. 47Loop Statements. 51Break and Labeled Break Statements . 56Continue and Labeled Continue Statements. 58Summary .60N Chapter 3: Discovering Classes and Objects .63Declaring Classes and Instantiating Objects .63Declaring Classes . 64Instantiating Objects with the New Operator and a Constructor . 64Specifying Constructor Parameters and Local Variables . 65www.it-ebooks.info
ContentsixEncapsulating State and Behaviors.69Representing State via Fields . 70Representing Behaviors via Methods . 75Hiding Information . 84Initializing Classes and Objects .89Class Initializers. 89Instance Initializers. 91Initialization Order . 93Collecting Garbage .96Revisiting Arrays .99Summary .104N Chapter 4: Discovering Inheritance, Polymorphism, and Interfaces .105Building Class Hierarchies.105Extending Classes . 106The Ultimate Superclass . 112Composition . 122The Trouble with Implementation Inheritance . 122Changing Form .126Upcasting and Late Binding . 127Abstract Classes and Abstract Methods . 131Downcasting and Runtime Type Identification. 133Covariant Return Types . 136Formalizing Class Interfaces .139Declaring Interfaces . 139Implementing Interfaces. 140Extending Interfaces . 144Why Use Interfaces? . 146Summary .152www.it-ebooks.info
xContentsN Chapter 5: Mastering Advanced Language Features Part 1 .153Mastering Nested Types .153Static Member Classes . 153Nonstatic Member Classes . 157Anonymous Classes. 161Local Classes . 164Interfaces within Classes . 166Mastering Packages .167What Are Packages? . 168The Package Statement. 169The Import Statement . 169Searching for Packages and Types . 170Playing with Packages. 172Packages and JAR Files . 176Mastering Static Imports .177Mastering Exceptions .179What Are Exceptions? . 179Representing Exceptions in Source Code . 179Throwing Exceptions . 184Handling Exceptions . 187Performing Cleanup . 190Summary .195N Chapter 6: Mastering Advanced Language Features Part 2 .197Mastering Assertions.197Declaring Assertions . 198Using Assertions . 199Avoiding Assertions . 205Enabling and Disabling Assertions . 206www.it-ebooks.info
ContentsxiMastering Annotations .207Discovering Annotations . 207Declaring Annotation Types and Annotating Source Code . 210Processing Annotations . 215Mastering Generics .217Collections and the Need for Type Safety . 217Generic Types . 220Generic Methods. 229Arrays and Generics . 232Mastering Enums .234The Trouble with Traditional Enumerated Types . 234The Enum Alternative . 235The Enum Class . 241Summary .245N Chapter 7: Exploring the Basic APIs Part 1 .247Exploring the Math APIs .247Math and StrictMath . 247BigDecimal . 255BigInteger . 260Exploring String Management .264String . 264StringBuffer and StringBuilder . 268Obtaining Package Information .270Summary .276N Chapter 8: Exploring the Basic APIs Part 2 .279Exploring the Primitive Type Wrapper Classes .279Boolean. 280Character . 281Float and Double. 282Integer, Long, Short, and Byte . 286Number . 288www.it-ebooks.info
xiiContentsExploring Threads.288Runnable and Thread. 289Thread Synchronization . 298Exploring System Capabilities .314System. 314Runtime and Process. 319Summary .324N Chapter 9: Exploring the Collections Framework .327Exploring Collections Framework Fundamentals .327Comparable Versus Comparator . 328Iterable and Collection . 330Exploring Lists .337ArrayList . 341LinkedList . 342Exploring Sets .344TreeSet. 344HashSet . 346EnumSet . 350Exploring Sorted Sets .353Exploring Navigable Sets.361Exploring Queues .364PriorityQueue . 365Exploring Deques .
Learn Java for Android Developm