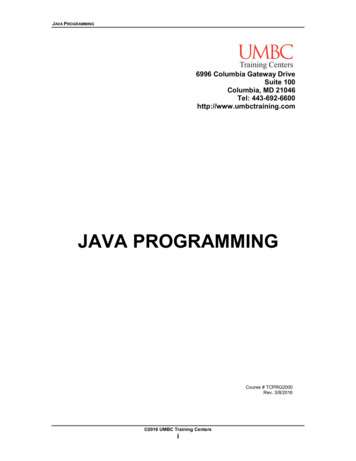
Transcription
JAVA PROGRAMMINGTraining Centers6996 Columbia Gateway DriveSuite 100Columbia, MD 21046Tel: 443-692-6600http://www.umbctraining.comJAVA PROGRAMMINGCourse # TCPRG2000Rev. 3/8/2016 2016 UMBC Training Centersi
JAVA PROGRAMMINGThis Page Intentionally Left Blank 2016 UMBC Training Centersii
JAVA PROGRAMMINGCourse Objectives At the conclusion of this course, students will be able to: Compile and run a Java application. Understand the role of the Java Virtual Machine in achievingplatform independence. Navigate through the API docs. Use the Object Oriented paradigm in Java programs. Understand the division of classes into Java packages. Use Exceptions to handle run time errors. Select the proper I/O class among those provided by the JDK. Use threads in order to create more efficient Java programs. 2016 UMBC Training Centersiii
JAVA PROGRAMMINGThis Page Intentionally Left Blank 2016 UMBC Training Centersiv
JAVA PROGRAMMINGTable of ContentsCHAPTER 1: INTRODUCTIONWhat is Java? . 1-2Versioning. 1-3The Java Virtual Machine . 1-4Writing a Java Program . 1-5Packages. 1-7Simple Java Programs. 1-11CHAPTER 2: LANGUAGE COMPONENTSPrimitive Data Types. 2-2Comments . 2-5Control Flow Statements . 2-6The if Statement. 2-7The switch Statement . 2-8The while and do while Statements. 2-9The for Statement. 2-10The break Statement . 2-11The continue Statement . 2-12Operators. 2-13Casts and Conversions. 2-14Keywords . 2-16CHAPTER 3: OBJECT-ORIENTED PROGRAMMINGDefining New Data Types . 3-2Constructors . 3-8The String Class. 3-9String Literals . 3-12Documentation. 3-14Packages. 3-16The StringBuffer Class. 3-17Naming Conventions . 3-19The Date Class . 3-20The import Statement . 3-22Deprecation . 3-23The StringTokenizer Class . 3-24The DecimalFormat Class. 3-25CHAPTER 4: METHODSIntroduction . 4-2Method Signatures. 4-3Arguments and Parameters. 4-4Passing Objects to Methods . 4-5Method Overloading . 4-8Static Methods . 4-9 2016 UMBC Training Centersv
JAVA PROGRAMMINGThe Math Class . 4-12The System Class. 4-13Wrapper Classes . 4-15CHAPTER 5: ARRAYSIntroduction . 5-2Processing Arrays. 5-3Copying Arrays . 5-4Passing Arrays to Methods. 5-6Arrays of Objects . 5-7The Arrays Class . 5-10Command Line Arguments . 5-11Multidimensional Arrays. 5-12CHAPTER 6: ENCAPSULATIONIntroduction . 6-2Constructors . 6-3The this Reference . 6-6Data Hiding . 6-10public and private Members. 6-11Access Levels. 6-15Composition. 6-16Static Data Members . 6-18CHAPTER 7: INHERITANCE & POLYMORPHISMIntroduction . 7-2A Simple Example . 7-3The Object Class. 7-6Method Overriding . 7-8Polymorphism . 7-9Additional Inheritance Examples. 7-11Other Inheritance Issues. 7-15CHAPTER 8: ABSTRACT CLASSES AND INTERFACESIntroduction . 8-2Abstract Classes. 8-3Abstract Class Example. 8-4Extending an Abstract Class. 8-5Interfaces. 8-8CHAPTER 9: EXCEPTIONSIntroduction . 9-2Exception Handling. 9-4The Exception Hierarchy . 9-6Checked Exceptions . 9-7Advertising Exceptions with throws . 9-10Developing Your Own Exception Classes . 9-11The finally Block. 9-15 2016 UMBC Training Centersvi
JAVA PROGRAMMINGCHAPTER 10: INPUT AND OUTPUT IN JAVAIntroduction . 10-2The File Class . 10-3Standard Streams. 10-4Keyboard Input . 10-5File I/O Using Byte Streams. 10-6Character Streams. 10-8File I/O Using Character Streams . 10-9Buffered Streams. 10-10File I/O Using a Buffered Stream . 10-11Keyboard Input Using a Buffered Stream . 10-12Writing Text Files . 10-13CHAPTER 11: COLLECTIONSIntroduction . 11-2Vectors . 11-3Hashtables. 11-4Enumerations. 11-6Properties . 11-9Collection Framework Hierarchy. 11-11Lists . 11-13Sets . 11-14Maps. 11-15The Collections Class . 11-16CHAPTER 12: NETWORKINGNetworking Fundamentals . 12-2The Client/Server Model . 12-4InetAddress . 12-6URLs. 12-8Sockets. 12-11A Time-of-Day Client . 12-13Writing Servers . 12-14Client/Server Example . 12-15CHAPTER 13: THREADSThreads vs. Processes . 13-2Creating Threads by Extending Thread . 13-3Creating Threads by Implementing Runnable. 13-5Advantages of Using Threads. 13-6Daemon Threads . 13-9Thread States . 13-11Thread Problems . 13-15Synchronization . 13-17 2016 UMBC Training Centersvii
JAVA PROGRAMMINGThis Page Intentionally Left Blank 2016 UMBC Training Centersviii
JAVA PROGRAMMINGCHAPTER 1: INTRODUCTIONChapter 1:Introduction1) What is Java? . 1-22) Versioning. 1-33) The Java Virtual Machine. 1-44) Writing a Java Program. 1-55) Packages. 1-76) Simple Java Programs. 1-11 2016 UMBC Training Centers1-1
JAVA PROGRAMMINGCHAPTER 1: INTRODUCTIONWhat is Java? Java is a Programming Language developed at SunMicrosystems beginning in 1991. The credit for Java is usually given to James Gosling who led agroup of engineers on a project named OAK. The project,based on C , had as its goal the control of consumer devices. The first public release of Java was in 1996 and was called theJava Development Kit (JDK). It has seen become known as theSoftware Development Kit (SDK). Java has been described in various ways. Simple - Java is simple compared to C . Object-Oriented - A style of programming emphasizing themarriage of data and methods rather than algorithms directly. Distributed - Java has built-in networking capabilities. Interpreted - The Java interpreter executes bytecodes on anymachine to which the interpreter has been ported. Robust - During bytecode generation, Java checks for anypossible errors rather than allowing an error to propagate to therun-time environment. Architecture Neutral - When a Java program is compiled, theresult is an architecture independent bytecode file. Thebytecode file is designed to run on what is called the JavaVirtual Machine (JVM). Secure - Java promises that a program cannot overwritememory outside of its own process space, or read or write localfiles when invoked through an applet in a Web Browser. Portable - Many of the problems from C and C are removed.For example, an int is always 32 bits, regardless of themachine. Strings are encoded using Unicode. Floating-pointnumbers are stored in a fixed format. 2016 UMBC Training Centers1-2
JAVA PROGRAMMINGCHAPTER 1: INTRODUCTIONVersioning SUN delivers different versions of the SDK in terms oftheir functionality. The versions are listed below. The Micro Edition (Java ME) Specifically addresses the vast consumer space. This covers the range of extremely tiny commodities such assmart cards or pagers, all the way up to the set-top box, anappliance almost as powerful as a computer. The Standard Edition (Java SE) Features a development and deployment environmentdesigned from the ground up for the Web. It provides cross-platform compatibility, safe networkdelivery, and smart card to supercomputer scalability. The Enterprise Edition (Java EE) Defines the standard for developing and deployingenterprise applications. It includes the latest versions of Enterprise JavaBeans,JavaServer Pages, Java Servlet APIs, the Java API for XMLParsing (JAXP), the Java Authentication and AuthorizationService (JAAS) API, and other API’s. 2016 UMBC Training Centers1-3
JAVA PROGRAMMINGCHAPTER 1: INTRODUCTIONThe Java Virtual Machine The Java Virtual Machine (JVM) is a computer (insoftware) with Java bytecodes as the instruction set. A developer uses an editor to create a Java source file whosename ends with the .java extension. A Java Compiler converts this to a file of bytecodes whosename ends with the .class extension. The JVM interprets and verifies the bytecodes of a file asit is loaded. If the class file passes the verifier, it is loaded and translatedinto specific OS instructions and executed on the targetmachine. The compiled .class files are architecture neutral. The language that produces the bytecode is irrelevant. Currently, any compiler can output Java bytecode. Therefore, the target platform for Java code is not anyparticular machine. It is a virtual machine (i.e., any computer that can translatethe bytecodes into native code). Performance at runtime can be somewhat slower thantraditional compilation because of verification, conversionto native machine code, array bounds checking andautomatic garbage collection. 2016 UMBC Training Centers1-4
JAVA PROGRAMMINGCHAPTER 1: INTRODUCTIONWriting a Java Program Writing a Java program requires some tools andadministration. The process generally proceeds as follows. Enter Java text into a file with any text editor. Java source files must end with the suffix .java. The name of the file must match the name of the publicclass.Hello.java1. public class Hello {2.public static void main(String args[]) {3.System.out.println("Hello World");4.}5. } Compile the source code. The Java compiler is named javac.javac Hello.java If there are no errors, the output from the compiler is a filewhose name has the name of the .java file but with the.class extension. ( Hello.class in this case.) This file contains platform independent bytecodes. Execute the program using the Java interpreter on the .classfile. The name of the interpreter is java.java HelloHello World 2016 UMBC Training Centers1-5
JAVA PROGRAMMINGCHAPTER 1: INTRODUCTIONWriting a Java Program The Hello program is intentionally simple in order to pickout the basic parts of a Java program. All Java files consist of one or more classes. Of these, exactly one of them can be made public. The name of the file must be the name of the public class. When a Java source file is compiled, a .class file is builtfor each class in the source file. A class can have more than one method. When an application is executed, the starting point for theapplication is always the following main method.public static void main(String args[]){} void: means that the method, main, does not return a value.However, some methods do return a value. public: is a keyword that controls access to this code. Codein other classes has access to a public method. There areother access levels other than public that will be introducedlater in the course. static: implies the method is not associated with an objectbut instead is associated with a class. The println method is a way of sending output to thestandard output file. 2016 UMBC Training Centers1-6
JAVA PROGRAMMINGCHAPTER 1: INTRODUCTIONPackages A package is a collection of related classes that: makes the classes easier to find and use; assists in avoiding naming conflicts; and assists in controlling access to the classes. To specify the package of a class, you put a packagestatement at the top of the source file of the class. If a package statement is not used, the class ends up in thedefault package, which is a package that has no name. The previous Hello application is an example of a class inthe default package. It is recommended that all classes generally belong in anamed package. Classes in a package need to be placed in a directorystructure that matches the package name. For example, many of the classes in this course could havebeen grouped into a package named examples. Since the course has many chapters, it might be better tosubdivide the classes into sub-packages. The classes in this chapter can be further grouped into apackage named examples.intro. Therefore, if we were to place Hello.java into this packagewe would use the following statement at the top of the class.package examples.intro; 2016 UMBC Training Centers1-7
JAVA PROGRAMMINGCHAPTER 1: INTRODUCTIONPackages If the Hello class is specified as being in theexamples.intro package, the compiled Hello.classneeds to be placed in a directory named intro, which inturn needs to be placed in a directory named examples. Although the source file, Hello.java, can be storedanywhere, it is recommended that source files be maintained inthe same package structure as the .class files. Below is a new version of Hello.java that has beenplaced in the examples.intro package.Hello.java1. package examples.intro;2. public class Hello {3.public static void main(String args[]) {4.System.out.println("Hello Again");5.}6. } Hello.java is located in the following sub-directory of thelabfiles directory: examples\intro Therefore, to compile Hello.java, we would change to theabove directory and run the compiler, resulting in a file namedHello.class in the examples\intro directory. cd javalabs\examples\intro javac Hello.java 2016 UMBC Training Centers1-8
JAVA PROGRAMMINGCHAPTER 1: INTRODUCTIONPackages Keep in mind that we now have two Hello.class files. C:\javalabs\Hello.class C:\javalabs\examples\intro\Hello.class To properly reference a class file in Java, you must supplythe fully qualified name of the class, which alwaysincludes the package name. the.package.name.ClassName To demonstrate how each of the above .class files canbe run, we will first change the command prompt to theC:\javalabs directory. Since the first Hello class we defined had no packagespecified, it is in the default package that has no name. Therefore, to run the first Hello program we would simplytype the following at the prompt.C:\javalabs java Hello This results in the following output.Hello World The second Hello class we defined specified a package ofexamples.intro. So, to run the second Hello program we would typeC:\javalabs java examples.intro.Hello This results in the following output.Hello Again 2016 UMBC Training Centers1-9
JAVA PROGRAMMINGCHAPTER 1: INTRODUCTIONPackages Both the JVM and the Java compiler rely on anenvironmental variable named CLASSPATH to locate classfiles. If the CLASSPATH is not specified, it defaults to the currentdirectory. To simplify our development environment, we will set theCLASSPATH to the directory containing the examples directory. There is a file in the javalabs directory namedsetenv.cmd, which should have the following entry added.set CLASSPATH C:\javalabs Running this script will set up our environment so that theJVM and the compiler will always begin looking for .classfiles it needs in the javalabs directory. Keep in mind that for the remainder of the course, all classeswill be organized into packages, and that to run a programyou will need to provide the fully qualified name of the classon the command line.java examples.intro.Hello The environment being set by the setenv.cmd script isonly set for that shell window. If a new window is opened, the sentenv.cmd script wouldneed to be run inside of that window as well. 2016 UMBC Training Centers1-10
JAVA PROGRAMMINGCHAPTER 1: INTRODUCTIONSimple Java Programs Below is a second program that builds upon the previousinformation by providing additional statements.AddIntegers.java1. package examples.intro;2. public class AddIntegers {3.public static void main(String args[]) {4.int x 10;5.int y 20;6.System.out.print("Sum of ");7.System.out.print(x);8.System.out.print(" and ");9.System.out.println(y);10.System.out.print("is ");11.System.out.println(x y);12.}13. } Compile the class by typing the following on the command line.javac AddIntegers.java Run the program by typing the following on the command line.java examples.intro.AddIntegers The output from executing the program is shown below:Sum of 10 and 20is 30 Note that the println method adds a newline character to theoutput, whereas the print method does not. The above example defined a few variables of type int. Soon you will see additional data types. 2016 UMBC Training Centers1-11
JAVA PROGRAMMINGCHAPTER 1: INTRODUCTIONSimple Java Programs The program on the previous page could have beenwritten in many different ways.AddAgain.java1. package examples.intro;2. public class AddAgain {3.public static void main(String args[]) {4.int x 10, y 20;5.System.out.print(x " " y);6.System.out.println(" is " ( x y));7.}8. } The output of the above program is shown below.10 20 is 30 When a String data type is added to any other datatype, the operator concatenates the values rather thanadding them as shown in the example below.Concatenation.java1. package examples.intro;2. public class Concatenation {3.public static void main(String args[]) {4.int x 10, y 20;5.System.out.println(x y " is " x y);6.}7. } The output of the above program is: 30 is 1020 Developers need to be aware of the dual purpose of the operator in Java. 2016 UMBC Training Centers1-12
JAVA PROGRAMMINGCHAPTER 1: INTRODUCTIONSimple Java Programs A method in Java cannot stand alone. It must be embedded in some class. The following code will not compile because the main methodis not embedded in a class.1. public static void main(String args[]) {2.int x 10, y 20;3.System.out.println(x y " is " x y);4. } The program below has a main method but the parameterlist is incorrect. This program will compile but cannot be executed as anapplication.Wrong.java1. package examples.intro;2. public class Wrong {3.public static void main() {4.int x 10, y x * 2;5.System.out.println(x y " is " 6.}7. } 2016 UMBC Training Centers1-13x y);
JAVA PROGRAMMINGCHAPTER 1: INTRODUCTIONExercises1. The following program contains multiple errors. Correct each of the errors until the program compiles andexecutes without any errors. A copy of this file can be found in the starters directory forthis chapter.1. package starters.intro.ex1;2. Public Class MyNewClass {3.public void static main(String s){4.integer a 55.system.out.println("a ", a);6.}7. }2. Write a program that includes in its main method, thethree lines of code shown below.int a 17, b 4, c;c a b;System.out.println(a " " b " " c); Run the program and interpret the results. Reusing the variable c, add similar statements for the / and %operators so that the results of all three calculations appear inthe output. 2016 UMBC Training Centers1-14
JAVA PROGRAMMINGCHAPTER 2: LANGUAGE COMPONENTSChapter 2:Language Components1) Primitive Data Types . 2-22) Comments . 2-53) Control Flow Statements. 2-64) The if Statement. 2-75) The switch Statement . 2-86) The while and do while Statements . 2-97) The for Statement . 2-108) The break Statement. 2-119) The continue Statement . 2-1210) Operators . 2-1311) Casts and Conversions. 2-1412) Keywords . 2-16 2016 UMBC Training Centers2-1
JAVA PROGRAMMINGCHAPTER 2: LANGUAGE COMPONENTSPrimitive Data Types Java supports a wide range of data types. The eightfundamental (or primitive) data types are shown DoublemyTruthmyCharyourCharaChar ';'\t';'\u03C0';//////////////////////8 bits1632326432641161616 Constants of any of the above types can be created byusing the keyword final.final double TAX RATE 0.06; Single quotes are used for a literal char.char letter 'A'; Local data (variables declared inside
Writing a Java Program The Hello program is intentionally simple in order to pick out the basic parts of a Java program. All Java files consist of one or more classes. Of these, exactly one of them can be made public. The name of the file must be the name of the public class. When a Java