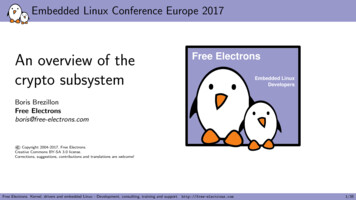
Transcription
Embedded Linux Conference Europe 2017An overview of thecrypto subsystemFree ElectronsEmbedded LinuxDevelopersBoris BrezillonFree Electronsboris@free-electrons.com Copyright 2004-2017, Free Electrons.Creative Commons BY-SA 3.0 license.Corrections, suggestions, contributions and translations are welcome!Free Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com1/35
Boris BrezillonIEmbedded Linux engineer and trainer at Free ElectronsIIIIContributionsIIIIEmbedded Linux development: kernel and driver development, system integration,boot time and power consumption optimization, consulting, etc.Embedded Linux, Linux driver development, Yocto Project / OpenEmbedded andBuildroot training courses, with materials freely available under a CreativeCommons license.http://free-electrons.comMaintainer of the NAND subsystemKernel support for various ARM SoCsContributed Marvell’s crypto engine driverLiving in Toulouse, south west of FranceFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com2/35
What is this talk about?IShort introduction to some cryptographic conceptsIOverview of services provided by the crypto subsystem and how to use itIOverview of the driver side of the crypto framework (how to implement a driverfor a simple crypto engine)IRandom thoughts about the crypto frameworkFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com3/35
An overview of the crypto subsystemIntroduction to generic cryptographic conceptsFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com4/35
Cryptography? What is this?IDo you know Alice and Bob? If you do, take a break and come back in fiveminutesICryptography is about protecting communications between two or moreparticipantsCovers three main concepts:IIIIIConfidentiality: content of the communication cannot be spied onData integrity: content of the communication cannot be altered withoutparticipants noticingAuthentication: message origin can be checkedAchieved by manipulating input/output messages and adding meta-data to themFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com5/35
Cryptography: Hash (or Digest)IUsed to guarantee Data IntegrityIOperates on a random number of input dataIGenerates a ’unique’ fixed-size signature for a specific inputIExamples: SHA1, MD5, .Main criteria for a hash algorithm:IIIIKeep the probability of collision as low as possible (ideally null)Make it impossible to re-generate data from its hashA small modification in the data should generate a completely different hashFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com6/35
Cryptography: CipherIUsed to guarantee ConfidentialityITransform the data so that someone external to the group can’t read itIRequires one or several key(s) to encrypt/decrypt dataCiphers can be stream or block orientedIIIICiphers can be symmetric or asymmetricIIIStream Ciphers: operate on a stream of dataBlock Ciphers: operate on fixed-size blocksSymmetric Ciphers: the same key (called private key) is shared among allparticipants and is used to both encrypt and decrypt dataAsymmetric Ciphers: a pair of public/private key is used. The public key can beshared with anyone and is used to encrypt messages sent to the owner of the privatekey. The private key is then used to decrypt messages.Examples: AES, RSA, .Free Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com7/35
Cryptography: Block Cipher ModeIBlock ciphers can only be used to encrypt/decrypt a single block of dataIWe need a solution to handle an arbitrary number of blocksIBlock cipher mode is just a protocol describing how to do thatIMost modes require an Initialization Vector (IV) to obfuscate the transmitteddataIExamples: ECB (Electronic Codebook), CBC (Cipher Chaining Block),.Free Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com8/35
Cryptography: MAC and HMACIMAC stands for Message Authentication CodesIMechanism used to authenticate the sender of a messageIUses a key and a transformation algorithm to generate authentication dataIMAC can be based on Hash algorithms HMACFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com9/35
Cryptography: AEADIAEAD stands for Authenticated Encryption with Associated DataICombines everything in a single step (Authentication, Confidentiality and DataIntegrity)IIs usually based on existing Cipher, Hash/HMAC algorithmsFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com10/35
Cryptography: Better introduction than mineIInterested in more advanced description of these concepts?IWatch Gilad Ben Yossef’s talk: Free Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com11/35
An overview of the crypto subsystemLinux Crypto Framework: Common PrinciplesFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com12/35
Linux Crypto Framework: Basic ConceptsIEvery crypto algorithm is about transforming input data into something elseIIITransformation implementation: represents an implementation of a specificalgorithm (struct crypto alg)Transformation object: an instance of a specific algorithm (struct crypto tfm)Everything inherits from struct crypto alg and struct crypto tfm eitherdirectly on indirectlyFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com13/35
Linux Crypto Framework: Basic ConceptsIISupports a whole bunch of algorithmsHere are some of them, spot the odd oneIIIIIIICipherHashAEADHMACCompressionA transformation algorithm can be a template using basic building blocksExamples:IIIhmac(sha1): HMAC using SHA1 hashcbc(aes): CBC using AESauthenc(hmac(sha1),cbc(aes)): AEAD using HMAC based on SHA1 and CBCbased on AESFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com14/35
An overview of the crypto subsystemLinux Crypto Framework: How to use itFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com15/35
Using the crypto frameworkFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com16/35
Dummy user exampleIDisclaimer: a lot of things have been deliberately omitted to keep the examplesimpleIIIIIIINo error checkingCode is not separated in sub-functionsWe only provide a simple example for a basic cipher: ecb(aes)Headers inclusion has been omittedThere’s no input/output parameter checking.To sum-up: do not use this example as a base for your developments, it’s justhere to show the different stepsFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com17/35
Dummy user implementationstruct encrypt ctx {struct crypto skcipher *tfm;struct skcipher request *req;struct completion complete;int err;};static void encrypt cb(struct crypto async request *req,int error){struct crypto ctx *ctx req- data;static void cleanup(struct encrypt ctx *ctx){skcipher request free(ctx- req);crypto free skcipher(ctx- tfm);}int encrypt(void *key, void *data, unsigned int size){struct encrypt ctx ctx;struct scatterlist sg;int ret;init(&ctx);if (error -EINPROGRESS)return;/* Set the private key: */crypto skcipher setkey(ctx.tfm, key, 32);ctx- err error;complete(&ctx- completion);/*Now assign the src/dst buffer and encrypt data: */sg init one(&sg, data, size);skcipher request set crypt(ctx.req, &sg, &sg, len,NULL);ret crypto skcipher encrypt(ctx.req);if (ret -EINPROGRESS ret -EBUSY) {wait for completion(&ctx.completion);ret ctx.err;}}static void init(struct encrypt ctx *ctx){/* Create a CBC(AES) algorithm instance: */ctx- tfm crypto alloc skcipher("ecb(aes)", 0, 0);/* Create a request and assign it a callback: */ctx- req skcipher request alloc(ctx- tfm,GFP KERNEL);skcipher request set callback(req,CRYPTO TFM REQ MAY BACKLOG,encrypt cb, ctx);init completion(&ctx.completion);}cleanup(&ctx);return ctx.err;}Free Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com18/35
In-kernel crypto usersIIDisk encryption: dm-cryptNetwork protocols:IIIIIIPSec802.11802.15.4Bluetooth.IFile systemsIDevice driversIgit grep "crypto alloc " and you’ll find a lot moreFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com19/35
Using kernel crypto features from userspaceIIPeople have pushed for this for quite some timeMotivation for this:IIIHave a single base of code instead of duplicating it in userspaceUse hardware crypto engines that are only exposed to the kernel worldTwo competing solutions:IIcryptodev: is an out-of-tree solution, ported from the BSD worldAF ALG: the in-tree/official solutionFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com20/35
cryptodev vs AF ALGIcryptodev:IIIIIIIIAF ALG:IIIIThe first solution to have emergedIs said to have more performance than AF ALGStill maintained as an out-of-tree kernel moduleInterfaces with the in-kernel crypto frameworkExposes a device under /dev/cryptoUses ioctls to setup the crypto contextNatively supported in OpenSSLSupported in mainline (appeared in Linux 2.6.38)Manipulated through a netlink socketSupported in OpenSSL as an out-of-tree moduleWhich one should I use?Free Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com21/35
OpenSSL Speed Test: SequentialCryptodev (Marvell CESA)AF ALG (Marvell CESA)Speed in Kbytes/sec60,00040,00020,000016b1kbFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com8kb22/35
OpenSSL Speed Test: SequentialCryptodev (Marvell CESA)AF ALG (Marvell CESA)No Hardware EngineSpeed in Kbytes/sec60,00040,00020,000016b1kbFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com8kb23/35
OpenSSL Speed Test: Parallelization (128 Threads)Speed in Kbytes/secCryptodev (Marvell CESA)AF ALG (Marvell CESA)No Hardware Engine3 · 1052 · 1051 · 105016b1kbFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com8kb24/35
cryptodev vs AF ALG: ConclusionIWhich one should I use?Icryptodev shows better results, but.I. both cryptodev and AF ALG are outperformed by the software userspacesolutionIAnd we don’t gain that much in term of CPU load when offloading to thehardware engine (60% vs 100%)IOf course those results are likely to be dependent on the crypto engine and itsdriverIn any caseIIIThink twice before using a hardware crypto engine from userspaceDo the test before taking a decisionFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com25/35
An overview of the crypto subsystemLinux Crypto Framework: How to develop acrypto engine driverFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com26/35
How to develop a crypto engine driverIThe crypto framework does not distinguish hardware engines from softwareimplementationIDeveloping a crypto engine driver is just about registering a crypto alg to thecrypto subsystemIIdentify the type of algorithm you want to add support for and the associatedcrypto alg interface (skcipher alg, ahash alg, .)IImplement the xxx alg interface and call crypto register xxx() to register itto the crypto frameworkIWe will study a simple algorithm type: cbc(aes)IGo look at other drivers or the crypto framework doc if you need information onother alg type interfacesFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com27/35
Important crypto alg Fieldsstruct skcipher alg xxx cbc aes alg {.base {/* Name used by the framework to find who is implementing what. */.cra name "cbc(aes)",/* Driver name. Can be used to request a specific implementation of an algorithm. */.cra driver name "xxx-cbc-aes",/* Priority is used when implementation auto-selection takes place:* if there are several implementers, the one with the highest priority is chosen.* By convention: HW engine ASM/arch-optimized plain C*/.cra priority 300,/* CRYPTO ALG TYPE XX: describes the type algorithm implemented here* CRYPTO ALG ASYNC: the engine is operating in an asynchronous manner* CRYPTO ALG KERN DRIVER ONLY: engine is not directly accessible to userspace*/.cra flags CRYPTO ALG TYPE SKCIPHER CRYPTO ALG KERN DRIVER ONLY CRYPTO ALG ASYNC,/* Size of the data blocks this algo operates on. */.cra blocksize AES BLOCK SIZE,/* Size of the context attached to an algorithm instance. */.cra ctxsize sizeof(struct xxx aes ctx),/* constructor/destructor methods called every time an alg instance is created/destroyed. */.cra init xxx skcipher cra init,.cra exit xxx skcipher cra exit,},};Free Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com28/35
skcipher alg Fieldsstatic int xxx encrypt(struct skcipher request *req){struct my ctx *ctx crypto tfm ctx(req- base.tfm);/* Prepare and queue the request here. Return 0 if the request has* been executed, -EINPROGRESS if it's been queued, -EBUSY if it's* been backlogged or a different error code for other kind of* errors.*/struct skcipher alg mv cesa cbc aes alg {/* Set key implementation. */.setkey xxx aes setkey,/* Encrypt/decrypt implementation. */.encrypt xxx cbc aes encrypt,.decrypt xxx cbc aes decrypt,/* Symmetric key size. */.min keysize AES MIN KEY SIZE,.max keysize AES MAX KEY SIZE,/* IV size */.ivsize AES BLOCK SIZE,.base {.},};return ret;}static int xxx decrypt(struct skcipher request *req){struct my ctx *ctx crypto tfm ctx(req- base.tfm);/* Similar to xxx encrypt() except this time we prepare and queue* a decrypt operation.*/return ret;}static int xxx setkey(struct crypto skcipher *cipher, const u8 *key,unsigned int len){struct my ctx *ctx crypto tfm ctx(req- base.tfm);/* Expand key and assing store the result in the ctx. */return ret;}Free Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com29/35
An overview of the crypto subsystemFeedback on my experience with the cryptoframeworkFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com30/35
The framework is a complex beastIGood aspects:IIIBad aspects:IIIIIICan easily be extended to support new algorithms (even non crypto related ones)It comes with an extensive testsuite to detect bad implementation or regressionsThe crypto framework is so open that you sometime have several ways to expose thesame thing (example: crypto alg ablkcipher or skcipher)The object model is not consistent (how to inherit from a base interface is notenforced even in the core)Hard to tell what the good practices are (old/existing drivers are usually notconverted to the new way of doing things)Important details can be discovered the hard way (example: completion callbackshould be called with softirqs disabled)Who am I to complain about these problems, the NAND framework is probablyworse in this regard.Getting these things addressed requires a non-negligible effort and some help fromdrivers contributorsFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com31/35
Limiting the number of interrupts: NAPI for crypto?ICrypto engines may generate a lot of interrupts (potentially one per cryptorequest, maybe more if the engine has a limited FIFO size)ICrypto can be used by the net stack which may decide to switch in polling modeunder heavy net loadIThe lack of NAPI-awareness at the crypto level defeats NAPI mode: you’ll end upwith a bunch of crypto interrupts which will prevent your system from runningsmoothly while under heavy net crypto loadICESA driver approach to limit the number of interrupts: try to queue requests atthe DMA level and use a threaded IRQ in IRQF ONESHOT mode to do somepolling instead of re-activating interrupts right awayINot a perfect solution: bigger latency than the irq softirq approachIQuestion: should we add a NAPI-like interface?Free Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com32/35
Load balancing: random thoughtsICurrent priority-based algorithm selection shows its limits:IIIISome systems come with several instances of the same engine but only one of themcan be used/exposed, unless the driver implements its own load balancing logicWe can’t distribute the load over heterogeneous engines in the system: the enginewith the highest priority gets all the requestsShould we introduce a generic load-balancing mechanism?Here is a list of things to address if we do:IIIIIntroduce the concept of crypto engine, because some engines expose several cryptoalgs, but can’t process things in parallelCalculate a per-request load based on the request type, the request length and theengine it is queued to (or something simpler load length?)Keep track of the total load of each engine registered to the system in order todecide where the next request will goAlgorithm contexts/states are driver dependent: we need anintermediate/driver-agnostic representation to allow moving the context/state fromone engine to anotherFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com33/35
Load balancing: random thoughtsFree Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com34/35
Questions? Suggestions? Comments?Boris Brezillonboris.brezillon@free-electrons.comSlides under CC-BY-SA elce/brezillon-crypto-framework/Free Electrons. Kernel, drivers and embedded Linux - Development, consulting, training and support. http://free-electrons.com35/35
Boris Brezillon I Embedded Linux engineer and trainer at Free Electrons I Embedded Linux development: kernel and driver development, system integration, boot time and power consumption optimization, consulting, etc. I Embedded Linux, Linux driver development, Yocto Project / OpenEmbedded and Buildroot training courses, with materials freely available under a Creative