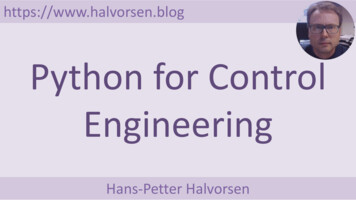
Transcription
https://www.halvorsen.blogPython for ControlEngineeringHans-Petter Halvorsen
Free Textbook with lots of Practical amming/python/
Additional Python ramming/python/
Contents Introduction to Control Engineering Python Libraries useful in ControlEngineering Applicationsβ NumPy, Matplotlibβ SciPy (especially scipy.signal)β Python Control Systems Library (control) Python Examples Additional Tutorials/Videos/Topics
Additional Tutorials/Videos/TopicsThis Tutorial is only the beginning. Some Examples of Tutorials that goes more in depth: Transfer Functions with Python State-space Models with PythonVideos available Frequency Response with Pythonon YouTube PID Control with Python Stability Analysis with Python Frequency Response Stability Analysis with Python Logging Measurement Data to File with Python Control System with Pythonβ Exemplified using Small-scale Industrial Processes and Simulators DAQ ogramming/python/
https://www.halvorsen.blogPython LibrariesHans-Petter Halvorsen
NumPy, Matplotlib In addition to Python itself, thePython libraries NumPy, Matplotlib istypically needed in all kind ofapplication If you have installed Python using theAnaconda distribution, these arealready installed
SciPy.signal An alternative to The Python Control Systems Library isSciPy.signal, i.e. the Signal Module in the SciPy Library htmlWith SciPy.signal you cancreate Transfer Functions,State-space Models, you cansimulate dynamic systems, doFrequency Response Analysis,including Bode plot, etc.
Python Control Systems Library The Python Control Systems Library (control) is aPython package that implements basic operationsfor analysis and design of feedback control systems. Existing MATLAB user? The functions and thefeatures are very similar to the MATLAB ControlSystems Toolbox. Python Control Systems Library Homepage:https://pypi.org/project/control Python Control Systems Library o
https://www.halvorsen.blogControl EngineeringHans-Petter Halvorsen
Control SystemNoise/Disturbanceππ π£The Controller istypically a PID sors
Control System π β Reference Value, SP (Set-point), SV (Set Value) π¦ β Measurement Value (MV), Process Value (PV) π β Error between the reference value and themeasurement value (π π β π¦) π£ β Disturbance, makes it more complicated to controlthe process π’ - Control Signal from the Controller
The PID Algorithm&πΎ#( πππ πΎ# π' πΜπ’ π‘ πΎ# π π %Where π’ is the controller output and π is thecontrol error:π π‘ π π‘ π¦(π‘)π is the Reference Signal or Set-pointπ¦ is the Process value, i.e., the Measured valueTuning Parameters:πΎ!π"π#Proportional GainIntegral Time [sec. ]Derivative Time [sec. ]
The PID Algorithm&πΎ#π’ π‘ πΎ# π ( πππ πΎ# π' πΜπ %PProportional GainTuning Parameters:πΎ%IIntegral Timeπ&DDerivative Timeπ'
https://www.halvorsen.blogPython ExamplesHans-Petter Halvorsen
https://www.halvorsen.blogDynamic Systems andDifferential EquationsHans-Petter Halvorsen
Dynamic Systems and Models The purpose with a Control System is to Control a DynamicSystem, e.g., an industrial process, an airplane, a self-drivencar, etc. (a Control System is βeverywhereβ). Typically, we start with a Mathematical model of such asDynamic System The mathematical model of such a system can beβ A Differential Equationβ A Transfer Functionβ A State-space Model We use the Mathematical model to create a Simulator ofthe system
1.order Dynamic SystemAssume the following general Differential Equation:Input Signalπ¦Μ ππ¦ ππ’π’(π‘)or:1π¦Μ ( π¦ πΎπ’)πWhere π !"and π DynamicSystemOutput Signalπ¦(π‘)#"Where πΎ is the Gain and π is the Time constantThis differential equation represents a 1. order dynamic systemAssume π’(π‘) is a step (π), then we can find that the solution to the differential equation is:π¦ π‘ πΎπ(1 π %")(we use Laplace)
PythonWe start by plotting the following:π¦ π‘ 0/1πΎπ(1 π )In the Python code we can set:π 1πΎ 3π 4import numpy as npimport matplotlib.pyplot as pltK 3T 4start 0stop 30increment 0.1t np.arange(start,stop,increment)y K * (1-np.exp(-t/T))plt.plot(t, y)plt.title('1.order Dynamic System')plt.xlabel('t [s]')plt.ylabel('y(t) ')plt.grid()
CommentsWe have many different options when it comes to simulation a DynamicSystem: We can solve the differential Equation(s) and then implement the thealgebraic solution and plot it.β This solution may work for simple systems. For more complicatedsystems it may be difficult to solve the differential equation(s) byhand We can use one of the βbuilt-inβ ODE solvers in Python We can make a Discrete version of the system We can convert the differential equation(s) to Transfer Function(s) etc.We will demonstrate and show examples of all these approaches
PythonDifferential Equation:Using ODE Solver1π¦Μ ( π¦ πΎπ’)πIn the Python code we can set:πΎ 3π 4import numpy as npfrom scipy.integrate import odeintimport matplotlib.pyplot as plt# InitializationK 3T 4u 1tstart 0tstop 25increment 1y0 0t np.arange(tstart,tstop 1,increment)# Function that returns dx/dtdef system1order(y, t, K, T, u):dydt (1/T) * (-y K*u)return dydt# Solve ODEx odeint(system1order, y0, t, args (K, T, u))print(x)# Plot the Resultsplt.plot(t,x)plt.title('1.order System dydt (1/T)*(-y lt.show()
DiscretizationWe start with the differential equation:π¦Μ ππ¦ ππ’We can use the Euler forward method:π¦456 π¦4π¦Μ π7This gives:8!"# /8!1 ππ¦4 ππ’4Further we get:π¦456 π¦4 π7 ππ¦4 ππ’4π¦456 π¦4 π7 ππ¦4 π7 ππ’4This gives the following discrete differentialequation:π¦ %& (1 π' π)π¦ π' ππ’
PythonLet's simulate the discrete system:π¦()* (1 π π)π¦( π ππ’(Where π !"and π #"In the Python code we can set:πΎ 3π 4import numpy as npimport matplotlib.pyplot as plt# Model ParametersK 3T 4a -1/Tb K/T# Simulation ParametersTs 0.1Tstop 30uk 1 # Step Responseyk 0 # Initial ValueN int(Tstop/Ts) # Simulation lengthdata []data.append(yk)# Simulationfor k in range(N):yk1 (1 a*Ts) * ykyk yk1data.append(yk1) Ts * b * uk# Plot the Simulation Resultst np.arange(0,Tstop Ts,Ts)plt.plot(t,data)plt.title('1.order Dynamic System')plt.xlabel('t [s]')plt.ylabel('y(t)')plt.grid()
https://www.halvorsen.blogTransfer FunctionsHans-Petter Halvorsen
Transfer Functions Transfer functions are a model formbased on the Laplace transform. Transfer functions are very useful inanalysis and design of linear dynamicsystems. You can create Transfer Functions bothwith SciPy.signal and the Python ControlSystems Library
1.order Transfer FunctionsA 1.order transfer function is given by:π¦(π )πΎπ» π π’(π ) ππ 1Where πΎ is the Gain and π is the Time constantIn the time domain we get the followingequation (using Inverse Laplace):π¦ π‘ πΎπ(1 )(π *)(After a Step π for the unput signal π’(π ))Output SignalInput Signalπ’(π )DifferentialEquationπ» π π¦(π )1π¦Μ ( π¦ πΎπ’)πWe ca find the Transfer function fromthe Differential Equation using Laplace
1.order β Step Response100%π¦(π‘)πΎππ¦ π‘ 63%0/1πΎπ(1 π )π¦(π )πΎπ» π π’(π ) ππ 1π‘π
PythonTransfer Function:3π»(π ) 4π 1import controlimport numpy as npimport matplotlib.pyplot as pltK T numden34 np.array([K]) np.array([T , 1])H control.tf(num , den)print ('H(s) ', H)t, y control.step response(H)plt.plot(t,y)plt.title("Step Response")
https://www.halvorsen.blogState-space ModelsHans-Petter Halvorsen
State-space ModelsSystemA general State-space Model is given by:π₯Μ π΄π₯ π΅π’π¦ πΆπ₯ π·π’Note that π₯Μ is the same as!"!#π’InputInternalStatesπ₯Outputπ¦π΄, π΅, πΆ and π· are matricesπ₯, π₯,Μ π’, π¦ are vectors A state-space model is a structured form or representation of a set of differentialequations. State-space models are very useful in Control theory and design. Thedifferential equations are converted in matrices and vectors. You can create State.space Models both with SciPy.signal and the Python Control SystemsLibrary
Basic ExampleGiven the following System: We want to put the equations on the following form:π₯Μ & π₯ π₯Μ π₯ π’π¦ π₯&This gives the following State-space Model:π₯Μ *0 1 π₯*0 π’π₯π₯Μ ,0 1 ,1π₯*π¦ 1 0 π₯,π₯Μ π΄π₯ π΅π’π¦ πΆπ₯ π·π’Where:0π΄ 01 1πΆ 10π₯Μ π₯Μ !π₯Μ &π΅ 01π· 0π₯!π₯ π₯&
PythonWe have the differential equations:1π₯Μ ! π₯! πΎπ’ππ₯Μ & 0π¦ π₯!The State-space Model becomes:1πΎπ₯π₯Μ !! π 0 π₯ π π’π₯Μ &&0 00π¦ 1π₯!0 π₯&Here we use the following function:t, y sig.step(sys, x0, t)import scipy.signal as sigimport matplotlib.pyplot as pltimport numpy as np#Simulation Parametersx0 [0,0]start 0stop 30step 1t np.arange(start,stop,step)K 3T 4# State-space ModelA [[-1/T, 0],[0, 0]]B [[K/T],[0]]C [[1, 0]]D 0sys sig.StateSpace(A, B, C, D)# Step Responset, y sig.step(sys, x0, t)# Plottingplt.plot(t, y)plt.title("Step plt.show()
PythonState-space Model:1π₯Μ ! ππ₯Μ "0π¦ 1πΎ0 π₯! π π’π₯"00π₯!0 π₯"We want to find the Transfer Function:π¦(π )π» π π’(π )TransferFunctionContinuous(array([0.75, 0. ]),array([1. , 0.25, 0. ]),dt: None)Python give us the following:0.75π»(π ) π 0.253π»(π ) 4π 1Which is the same asimport scipy.signal as sigimport matplotlib.pyplot as pltimport numpy as np#Simulation Parametersx0 [0,0]start 0; stop 30; step 1t np.arange(start,stop,step)K 3; T 4# State-space ModelA [[-1/T, 0],[0, 0]]B [[K/T],[0]]C [[1, 0]]D 0sys sig.StateSpace(A, B, C, D)H sys.to tf()print(H)# Step Responset, y sig.step(H, x0, t)# Plottingplt.plot(t, y)plt.title("Step Response")plt.xlabel("t"); plt.ylabel("y")plt.grid()plt.show()
https://www.halvorsen.blogFrequency ResponseHans-Petter Halvorsen
Frequency Response The Frequency Response is an important tool forAnalysis and Design of signal filters and foranalysis and design of Control Systems The frequency response can be found from atransfer function model The Bode diagram gives a simple Graphicaloverview of the Frequency Response for a givensystem The Bode Diagram is tool for Analyzing theStability properties of the Control System.
PythonTransfer Function Example:import numpy as npimport scipy.signal as signalimport matplotlib.pyplot as pltSciPy.signal3(2π 1)π» π (3π 1)(5π 1)# Define Transfer Functionnum1 np.array([3])num2 np.array([2, 1])num np.convolve(num1, num2)den1 np.array([3, 1])den2 np.array([5, 1])den np.convolve(den1, den2)H signal.TransferFunction(num, den)print ('H(s) ', H)# Frequenciesw start 0.01w stop 10step 0.01N int ((w stop-w start )/step) 1w np.linspace (w start , w stop , N)# Bode Plotw, mag, phase signal.bode(H, w)plt.figure()plt.subplot (2, 1, 1)plt.semilogx(w, mag)# Bode Magnitude Plotplt.title("Bode Plot")plt.grid(b None, which 'major', axis 'both')plt.grid(b None, which 'minor', axis 'both')plt.ylabel("Magnitude (dB)")plt.subplot (2, 1, 2)plt.semilogx(w, phase) # Bode Phase plotplt.grid(b None, which 'major', axis 'both')plt.grid(b None, which 'minor', axis 'both')plt.ylabel("Phase (deg)")plt.xlabel("Frequency (rad/sec)")plt.show()
PythonTransfer Function Example:3(2π 1)π» π (3π 1)(5π 1)Python Control Systems Libraryimport numpy as npimport control# Define Transfer Functionnum1 np.array([3])num2 np.array([2, 1])num np.convolve(num1, num2)den1 np.array([3, 1])den2 np.array([5, 1])den np.convolve(den1, den2)H control.tf(num, den)print ('H(s) ', H)# Bode Plotcontrol.bode(H, dB True)
https://www.halvorsen.blogPID ControlHans-Petter Halvorsen
Control SystemThe purpose with a Control System is to Control a Dynamic System, e.g., an industrialprocess, an airplane, a self-driven car, etc. (a Control System is βeverywhereβ).PID ControllerReferenceValueππ π¦Controllerπ’ControlSignalProcessπ¦
PID The PID Controller is the most usedcontroller today It is easy to understand andimplement There are few Tuning Parameters
The PID Algorithm&πΎ#( πππ πΎ# π' πΜπ’ π‘ πΎ# π π %Where π’ is the controller output and π is thecontrol error:π π‘ π π‘ π¦(π‘)π is the Reference Signal or Set-pointπ¦ is the Process value, i.e., the Measured valueTuning Parameters:πΎ!π"π#Proportional GainIntegral Time [sec. ]Derivative Time [sec. ]
Discrete PI ControllerWe start with the continuous PI Controller:πΎ! π’ π‘ πΎ! π ππππ" #We derive both sides in order to removethe Integral:π’Μ πΎ( πΜ πΎ(ππ)We can use the Euler Backward Discretization method:π₯Μ π₯ π π₯ π 1π%Where π' is the Sampling TimeThen we get:π’& π’&'(π& π&'( πΎ! πΎ! ππ%π%π" &Finally, we get:π’4 π’4/6 πΎC π4 π4/6Where π* π* π¦*πΎC π7 π4πD
Control System SimulationsPI Controller:Discrete Version (Ready to implement in Python):πΎ, /( ππππ’ π‘ πΎ, π π- .π4 π4 π¦4π’4 π’4/6 πΎC π4 π4/6πΎC π7 π4πDProcess (1.order system):π¦Μ ππ¦ ππ’Where π !"and π #Discrete Version (Ready to implement in Python):π¦()* (1 π π)π¦( π ππ’("In the Python code we can set πΎ 3 and π 4
import numpy as npimport matplotlib.pyplot as plt#KTabModel Parameters 3 4 -(1/T) K/T# Simulation ParametersTs 0.1 # Sampling TimeTstop 20 # End of Simulation TimeN int(Tstop/Ts) # Simulation lengthy np.zeros(N 2) # Initialization the Tout vectory[0] 0 # Initial Vaue# PI Controller SettingsKp 0.5Ti 5r 5 # Reference valuee np.zeros(N 2) # Initializationu np.zeros(N 2) # Initialization# Simulationfor k in range(N 1):e[k] r - y[k]u[k] u[k-1] Kp*(e[k] - e[k-1]) (Kp/Ti)*Ts*e[k]y[k 1] (1 Ts*a)*y[k] Ts*b*u[k]# Plot the Simulation Resultst np.arange(0,Tstop 2*Ts,Ts) #Create the Time SeriesPython# Plot Process Valueplt.figure(1)plt.plot(t,y)# Formatting the appearance of the Plotplt.title('Control of Dynamic System')plt.xlabel('t [s]')plt.ylabel('y')plt.grid()xmin 0xmax Tstopymin 0ymax 8plt.axis([xmin, xmax, ymin, ymax])plt.show()# Plot Control Signalplt.figure(2)plt.plot(t,u)# Formatting the appearance of the Plotplt.title('Control Signal')plt.xlabel('t [s]')plt.ylabel('u [V]')plt.grid()
Python
https://www.halvorsen.blogStability AnalysisHans-Petter Halvorsen
Stability AnalysisPoles:Unstable SystemImMarginally Stable SystemImAsymptotically Stable SystemImReReReStep Response:π‘lim π¦ π‘ π *Frequency Response:π π(,#π‘0 lim π¦ π‘ *π π(,#π‘lim π¦ π‘ *π π(,#
Stability Analysis ExampleππΎ! (π" π 1)π» π π" π π π¦FControllerFilterπ». π π’3π»! π 4π 11π. π 1Processπ₯Sensorπ»-1π π- π 1In Stability Analysis we use the following Transfer Functions:Loop Transfer Function: πΏ π π» (π )π»! (π )π»- (π )π». (π )Tracking Transfer Function: π(π ) /(%)2(%) 3(%)(43(%)
import numpy as npimport matplotlib.pyplot as pltimport control# Tracking transfer functionT control.feedback(L,1)print ('T(s) ', T)# Transfer Function ProcessK 3; T 4num p np.array ([K])den p np.array ([T , 1])Hp control.tf(num p , den p)print ('Hp(s) ', Hp)# Step Response Feedback System (Tracking System)t, y control.step ep Response Feedback System T(s)")plt.grid()# Transfer Function PI ControllerKp 0.4Ti 2num c np.array ([Kp*Ti, Kp])den c np.array ([Ti , 0])Hc control.tf(num c, den c)print ('Hc(s) ', Hc)# Bode Diagram with Stability Marginsplt.figure(2)control.bode(L, dB True, deg True, margins True)# Transfer Function MeasurementTm 1num m np.array ([1])den m np.array ([Tm , 1])Hm control.tf(num m , den m)print ('Hm(s) ', Hm)# Transfer Function Lowpass FilterTf 1num f np.array ([1])den f np.array ([Tf , 1])Hf control.tf(num f , den f)print ('Hf(s) ', Hf)# The Loop Transfer functionL control.series(Hc, Hp, Hf, Hm)print ('L(s) ', L)# Poles and Zeroscontrol.pzmap(T)p control.pole(T)z control.zero(T)print("poles ", p)# Calculating stability margins and crossover frequenciesgm , pm , w180 , wc control.margin(L)# Convert gm to Decibelgmdb 20 * np.log10(gm)print("wc ", f'{wc:.2f}', "rad/s")print("w180 ", f'{w180:.2f}', "rad/s")print("GM ", f'{gm:.2f}')print("GM ", f'{gmdb:.2f}', "dB")print("PM ", f'{pm:.2f}', "deg")# Find when Sysem is Marginally Stable (Kritical Gain Kc Kp*gmprint("Kc ", f'{Kc:.2f}')Kc)
πΎ( 0.4π) 2π ResultsFrequency ResponseGain Margin (GM): ΞπΎ 11. ππ΅Phase Margin (PM): Ο 30 This means that we can increaseπΎ# a bit without problemPolesStep ResponseAs you see we have an Asymptotically Stable SystemThe Critical Gain is πΎ πΎ( ΞπΎ 1.43
ConclusionsWe have an Asymptotically Stable System when πΎ! πΎ We have Poles in the left half plane lim π¦ π‘ 1 (Good Tracking) * π π(,#We have a Marginally Stable System when πΎ! πΎ We have Poles on the Imaginary Axis 0 lim π¦ π‘ * π π(,#We have an Unstable System when πΎ! πΎ We have Poles in the right half plane lim π¦ π‘ * π π(,#
Additional Tutorials/Videos/TopicsWant to learn more? Some Examples: Transfer Functions with Python State-space Models with PythonVideos available Frequency Response with Pythonon YouTube PID Control with Python Stability Analysis with Python Frequency Response Stability Analysis with Python Logging Measurement Data to File with Python Control System with Python β Exemplified using Small-scaleIndustrial Processes and Simulators DAQ Systems ng/python/
Additional Python ramming/python/
Hans-Petter HalvorsenUniversity of South-Eastern Norwaywww.usn.noE-mail: hans.p.halvorsen@usn.noWeb: https://www.halvorsen.blog
Introduction to Control Engineering Python Libraries useful in Control Engineering Applications βNumPy, Matplotlib . The Python Control Systems Library (control) is a Python package that implements basic operations for analysis