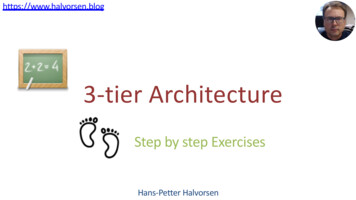
Transcription
https://www.halvorsen.blog3-tier ArchitectureStep by step ExercisesHans-Petter Halvorsen
Software Architecture2-Tier3-Tier: A way to structure your code into logical parts. Different devices orsoftware modules can share the same od Software!bAPIWeb Services: A standard way to get dataover a network/Internet using standardWeb protocols (HTTP, etc.)n-TierAPIsAPI: Application Programming Interface. Differentdevices or software modules can share the samecode. Code once, use it many times
The database-centric style. Typically, the clientscommunicate directly with the database.A three-tier style, in which clientsdo not connect directly to thedatabase.Web Services, etc.3
3-tier/layer ArchitectureNote! The different layers can beon the same computer (LogicLayers) or on different Computersin a network (Physical Layers)Presentation TierPLBusiness Logic TierBLLogic TierData Access TierDataSourceDALData Tier - DL4
Why 3-Tier (N-Tier Architecture?) Flexible applications Reusable code– Code once, use many times Modularized––––You need only to change part of the codeYou can deploy only one partYou can Test only one partMultiple Developers Different parts (Tiers) can be stored on different computers Different Platforms and Languages can be used etc.5
http://en.wikipedia.org/wiki/Multitier architecture6
3-tier/layer ArchitecturePresentation Tier This is the topmost level of the application. The presentation tier displays information related to such services as browsing merchandise, purchasingand shopping cart contents. It communicates with other tiers by which it puts out the results to the browser/client tier and all othertiers in the network. In simple terms it is a layer which users can access directly such as a web page, or an operating systemsGUIApplication tier (business logic, logic tier, data access tier, or middle tier) The logical tier is pulled out from the presentation tier and, as its own layer. It controls an application’s functionality by performing detailed processing.Data tier This tier consists of database servers. Here information is stored and retrieved. This tier keeps data neutral and independent from application servers or business logic. Giving data its own tier also improves scalability and performance.http://en.wikipedia.org/wiki/Multitier architecture7
3-tier ArchitecturePresentation TierPresentation TierPresentation TierBusiness Logic TierLogic TierData Access TierStored ProceduresDatabaseData Tier
3-tier ArchitecturePresentation TierPresentation TierPresentation TierBusiness Logic TierDifferent Devices can sharethe same Business and DataAccess CodeThe different Tiers can bephysical or logicalLogic TierData Access TierStored ProceduresDatabaseData Tier
3-tier WebService Architecture - sentationTierWebServicesBusiness/DataLogic TierStoredProceduresDataSourceDataTier
3-tier WebService Architecture - ExampleTeam Foundation ServerInstalled on one or moreWindows Servers in your LANor in the CloudWebServerWebServicesBusiness/Data Logic TierStoredProceduresDataSourceDataTierTeam Foundation ServerTFS CientPresentationTier
3-tier Architecture ScenariosClientClientClientInternetPresentation LayerFirewallPresentation LayerPresentation LayerClientClientWeb ServicePresentation LayerServerPresentation LayerPresentation LayerLocal Network (LAN)StoredDatabase ProceduresWebServerServerBusiness LogicData Access Logic
Exercises1. Create Data Tier (Database)2. Create Logic Tier (Database Communication Logic)Create Presentation Tier (User Interface Logic):3. WebApp: Using ASP.NET Web Forms (WS normally not needed)4. Desktop App: Using WinFormsA. Without Web Services (We assume the App will be used only inthe LAN and that we have direct access to the Database)B. With Web Services (We assume the App should be used onInternet outside the Firewall without direct DB access)
Android, iOS, WindowsClientWinForms8/Windows Phone,etc.MobilePresentation TierApp Presentation TierDesktop AppSeperate Presentation TierInternetPresentation Tiers for each Device AppWeb AppFirewallPresentation TierASP.NET Web FormsDevices can share the sameBusiness/Logic Tier ntAPIWeb ServerWeb ServiceLocalNetworkAPIBusiness TierLogic TierData Access Tiere.g., ADO, ADO.NETDatabaseStored ProceduresViewsTablesData Tier3-tierArchitectureScenariosNote! The different Tierscan be on the sameComputer (Logic Layers) oron different Computers in anetwork (Physical Layers)
Visual Studio ProjectsSolution with all Projects(Logic Tier, Web Service,Desktop App, Web App,Mobile App)Solution with Projectsused by Web App(Logic Tier, Web App)15
Data TierWe are going to create the Database / Data Layer/Tier,including:1. Tables2. Views3. Stored ProceduresNote! Install themin this order4. Triggers5. Script for some “Dummy” DataDownload Zip Files with Tables, Views, Stored Proceduresand Triggerse in order to create the Data Tier in SQL Server(The ZIP File is located on the same place as this File)16
Data TierTriggersStored ProceduresViewsTablesData TierSQL Server17
Database Tables18
Execute the differentScripts inside SQL ServerManagement Studio19
You are finished with the Exercise20
Create Logic TierASP.NET Web FormsPresentation TierWindows Store AppWinFormsPresentation TierLogic TierData TierPresentation TierPurpose: All the Apps should/couldshare the same Logic Tier To make your Apps easierto maintain and extend etc.Database21
Create an Empty (Blank) Solution in Visual Studio22
Add Project for Logic Tier (Data Access)Select a “Class Library” Project“LogicTier”23
Add a New Class to theProject (“StudentData.cs”)“StudentData.cs”24
Create the Code, e.g., like this (“StudentData.cs”):Create your own NamespaceA View that collects datafrom several tablesImprovements: Use Try. Catch .25
You should test the SQL Query in the SQL ServerManagement Studio first26
Code (“StudentData.cs”):using System.Data.SqlClient;using System.Data.SqlTypes;using System.Data;namespace Tuc.School.LogicTier{public class StudentData{public DataSet GetStudentDB(string connectionString){string selectSQL "select StudentName, StudentNumber, SchoolName, ClassName,Grade from StudentData order by StudentName";// Define the ADO.NET objects.SqlConnection con new SqlConnection(connectionString);SqlDataAdapter da new SqlDataAdapter(selectSQL, con);DataSet ds new DataSet();da.Fill(ds);return ds;}}}27
Create a proper name for the Assembly (.dll File)Right-click on the Project in the Solution Explorer and select PropertiesThen Build yourProject (hopefullywith no errors)This will be the Assembly for yourLogic Tier, that can be importedand used in other projects.Create once – use it many times!!28
You are finished with the Exercise29
Presentation LayerWeb App: ASP.NET WebFormsWe will create a WebForm like this where the data comes from our Logic Tier30
Add Project for Presentation Tier(ASP.NET WebForm)31
Add a New Class (“Student.cs”)32
Add Code (“Student.cs”)Note! This is our Logic TierAdd a Reference to the Assembly in the Logic Tier33
Code for Collections.Generic;System.Linq;System.Web;using System.Data;using Tuc.School.LogicTier;Since we are using the DataSet ClassOur Logic Tiernamespace Tuc.School.WebApp{public class Student{public DataSet GetStudent(string connectionString){StudentData studentData new StudentData();Get Dat from ourLogic Tierreturn studentData.GetStudentDB(connectionString);}}}34
Add a New WebForm (StudentInformation.aspx)35
Create WebForm Page (“StudentInformation.aspx”)GridView (Drag & Drop from Toolbox or createcode in .aspx file)36
HTML /ASPX Code (“StudentInformation.aspx”) %@ Page Language "C#" AutoEventWireup "true" CodeBehind "StudentInformation.aspx.cs" Inherits "WebApp.StudentInformation" % !DOCTYPE html html xmlns "http://www.w3.org/1999/xhtml" head runat "server" title Student Information /title /head body form id "form1" runat "server" div h1 Student Information /h1 /div asp:GridView ID "gridStudentData" runat "server" /asp:GridView /form /body /html 37
Code behind for the Web Form (“StudentInformation.aspx.cs”)using System.Web.Configuration;using Tuc.School.WebApp;Note!namespace WebApp{public partial class StudentInformation : System.Web.UI.Page{Web.Configprivate string connectionString onnectionString"].ConnectionString;protected void Page Load(object sender, EventArgs e){if (!IsPostBack){FillStudentGrid();}}private void FillStudentGrid(){DataSet ds new DataSet();Student studentList new Student();ds ntData.DataSource ds;gridStudentData.DataBind();}}}38
Store the “ConnectionString” for your Database in “Web.Config” ?xml version "1.0" encoding "utf-8"? !-For more information on how to configure your ASP.NET application, please visithttp://go.microsoft.com/fwlink/?LinkId 169433-- configuration connectionStrings add name "SCHOOLConnectionString" connectionString "Data Source macwin8;InitialCatalog SCHOOL;Persist Security Info True;User ID sa;Password xxxxxx"providerName "System.Data.SqlClient" / /connectionStrings system.web compilation debug "true" targetFramework "4.5" / httpRuntime targetFramework "4.5" / /system.web /configuration Then you can easly switch Databsse without changing the Code!!39
Test your Web AppNote! We have used a “View” in order to get data from several tables40
AdditionalExercise:Update GridView with NewData from DatabaseGoto New WebFormin order to Add anotherStudent41
You are finished with the Exercise42
Presentation LayerDesktop App: WinFormsPart A: Without Web Services (we assume the App will be used only in the local LAN (orlocal on the same computer where the database is located) and that we have direct accessto the Database)LabelDataGridView43
Add a WinForm Project44
Add a New Class (“StudentWinForm.cs”)45
Add Code in ClassAdd a Reference to theAssembly in the Logic Tier46
Code for Class “StudentWinForm.cs”using System.Data;Since we are using the DataSet Classusing Tuc.School.LogicTier;Reference to our Logic Tiernamespace Tuc.School.WinFormApp{class StudentWinForm{public DataSet GetStudent(string connectionString){StudentData studentData new StudentData();return studentData.GetStudentDB(connectionString);}}}Our Database Method in our Logic Tier47
Create FormLabelDataGridView48
Create Form Code49
WinForm Codeusing System.Configuration;using Tuc.School.WinFormApp;Note!namespace WinFormApp{public partial class Form1 : Form{ConnectionString isstored in App.configprivate string connectionString ectionString"].ConnectionString;public Form1(){InitializeComponent();}private void Form1 Load(object sender, EventArgs e){FillStudentGrid();}private void FillStudentGrid(){DataSet ds new DataSet();StudentWinForm studentList new StudentWinForm();ds iewStudentInformation.DataSource ds.Tables[0];}}}50
Note! Add System.Configuration Reference51
Create DB ConnectionString in App.config ?xml version "1.0" encoding "utf-8" ? configuration startup supportedRuntime version "v4.0" sku ".NETFramework,Version v4.5" / /startup connectionStrings add name "SCHOOLConnectionString" connectionString "Data Source macwin8;Initial Catalog SCHOOL;Persist SecurityInfo True;User ID sa;Password xxxxxx"providerName "System.Data.SqlClient" / /connectionStrings /configuration 52
Test itIt works!!!53
You are finished with the Exercise54
Presentation LayerDesktop App: WinFormsPart B: Using Web Services (we assume the The App should be used on Internet outsidethe Firewall)LabelDataGridView55
Step 1: Create Web Service“SchoolWS”Create an ASP.NET Project:“SchoolWS.asmx”Add Web Service:56
Web Service CodeDatabase ConnectionStringis located in Web.configWeb Service Method57
Database ConnectionString is located in Web.config58
Test Web ServiceClick to Test the Web ServiceMethod we createdIt Works!!59
Deploy/Publish Web Service to IISCopy Web Service Files (Project) to default IIS Directory: C:\inetpub\wwwroot60
61
Test if WS working:http://localhost/SchoolWS62
Step 2: Use Web Service in WinFormCreate New WinForm Project:“WinFormAppWSClient”63
Add Web Service ReferenceOur Web Service Methods64
Create GUILabelDataGridView
Create Code66
WinForm Codeusing System.Windows.Forms;namespace WinFormAppWSClient{public partial class FormWSClient : Form{public FormWSClient(){InitializeComponent();}private void FormWSClient Load(object sender, EventArgs e){FillStudentGrid();}private void FillStudentGrid(){Call the Web Service methodDataSet ds new DataSet();SchoolWSReference.SchoolWSSoapClient schoolWs newSchoolWSReference.SchoolWSSoapClient();ds on.DataSource ds.Tables[0];}}Fill GridView67
Test it:It works!!!68
You are finished with the Exercise69
Hans-Petter HalvorsenUniversity of South-Eastern Norwaywww.usn.noE-mail: hans.p.halvorsen@usn.noWeb: https://www.halvorsen.blog
API: Application Programming Interface. Different devices or software modules can share the same code. Code once, use it many times Web Services: A standard way to get data over a network/Internet using standard Web protocols (HTTP, etc.) 3-Tier: A way to str