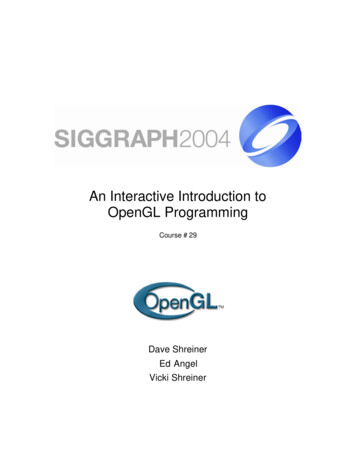
Transcription
An Interactive Introduction toOpenGL ProgrammingCourse # 29Dave ShreinerEd AngelVicki Shreiner
Table of ContentsIntroduction.ivPrerequisites .ivTopics .ivPresentation Course Notes .viAn Interactive Introduction to OpenGL Programming - Course # 29.1Welcome .2Welcome .3What Is OpenGL, and What Can It Do for Me? .4Related APIs .5OpenGL and Related APIs.6What Is Required For Your Programs .7OpenGL Command Formats .8The OpenGL Pipeline .9An Example OpenGL Program.10Sequence of Most OpenGL Programs .11An OpenGL Program.12An OpenGL Program (cont’d.) .13An OpenGL Program (cont’d.) .14GLUT Callback Functions .15Drawing with OpenGL .16What can OpenGL Draw?.17OpenGL Geometric Primitives .18Specifying Geometric Primitives.19The Power of Setting OpenGL State .20How OpenGL Works: The Conceptual Model .21Controlling OpenGL’s Drawing .22Setting OpenGL State .23Setting OpenGL State (cont’d.) .24OpenGL and Color.25Shapes Tutorial .26Animation and Depth Buffering .27Double Buffering .28Animation Using Double Buffering.29Depth Buffering and Hidden Surface Removal.30Depth Buffering Using OpenGL.31i
Transformations .32Camera Analogy .33Camera Analogy and Transformations .34Transformation Pipeline.35Coordinate Systems and Transformations .36Homogeneous Coordinates .373D Transformations .38Specifying Transformations .39Programming Transformations .40Matrix Operations .41Projection Transformation .42Applying Projection Transformations .43Viewing Transformations .44Projection Tutorial .45Modeling Transformations .46Transformation Tutorial.47Connection: Viewing and Modeling .48Common Transformation Usage .49Example 1: Perspective & LookAt .50Example 2: Ortho .51Example 2: Ortho (cont’d) .52Compositing Modeling Transformations .53Compositing Modeling Transformations .54Lighting .55Lighting Principles .56How OpenGL Simulates Lights .57Surface Normals.58Material Properties .59Light Properties.60Light Sources (cont'd.) .61Types of Lights .62Turning on the Lights.63Light Material Tutorial.64Controlling a Light’s Position.65Light Position Tutorial.66Tips for Better Lighting .67Texture Mapping .68Pixel-based primitives.69Positioning Image Primitives .70Rendering Bitmaps and Images .71Reading the Framebuffer .72Pixel Pipeline .73Texture Mapping.74Texture Example .75Applying Textures I .76ii
Texture Objects.77Texture Objects (cont'd.).78Specify the Texture Image .79Converting A Texture Image .80Mapping a Texture .81Tutorial: Texture .82Applying Textures II .83Texture Application Methods .84Filter Modes .85Mipmapped Textures .86Wrapping Mode .87Texture Functions .88Perspective Correction Hint .89Advanced OpenGL Topics .90Working with OpenGL Extensions.91Alpha: the 4th Color Component .92Blending .93Antialiasing .94Summary / Q & A .95On-Line Resources.96Books .97Thanks for Coming .98Bibliography .100Glossary.101iii
Introduction“An Interactive Introduction to OpenGL Programming” provides an overview of theOpenGL Application Programming Interface (API), a library of subroutines for drawingthree-dimensional objects and images on a computer. After the completion of the course,a programmer able to write simple programs in the “C” language will be able to create anOpenGL application that has moving 3D objects that look like they are being lit by lightsin the scene and by specifying colors or images that should be used to color those objects.Additionally, the viewpoint of the scene can be controlled by the mouse and keyboard,and can be updated interactively. Finally, the course provides references for exploringmore of the capabilities of OpenGL that aren’t covered in the class.Course PrerequisitesYou need very little experience with computer graphics or with programming to becomesuccessful using OpenGL. Our course does expect you to have a reading knowledge of aprocedural language (all of our examples are in “C”, but don’t use any advancedconcepts). We also try to explain the background of each concept as well as demonstratehow to accomplish the technique in OpenGL. A bibliography is included to aid you infinding more information or clarifying points that didn’t make sense the first time around.OpenGL and Window SystemsThe OpenGL library is platform independent with implementations available on almostevery operating system: Microsoft Windows; Apple Computer’s MAC O/S; and mostversion of UNIX, including Linux. Although the code that you write using the OpenGLAPI is easily moved between platforms, OpenGL relies on the native windowing systemof the computer you’re running the program on. Each windowing system has uniquemethods for opening windows, processing keyboard and mouse input, and enablingwindows to be able to be drawn into by OpenGL. In order to make this process simpler,this course uses the GLUT library (OpenGL Utility Toolkit, authored by Mark Kilgard)to hide the specifics required for different operating systems.TopicsOur course covers a number of topics that enable the creation of interesting OpenGLapplications : 3D object modeling – how to combine vertices to create the three geometricprimitives: points, lines, and polygons. We’ll also discuss how to constructobjects by assembling geometric primitives. By far, modeling objects is the mostlaborious task in 3D graphics. For all but the simplest shapes, or shapes derivedfrom mathematical formulas (i.e., circles, spheres, cones, etc.), most objects arecreated using a modeling program (e.g., Maya, 3D Studio Max, Houdini, etc.).The GLUT library contains routines for creating some common shapes as well,which we briefly discuss. Transformations – computer graphics relies heavily on the use of 4 4 matrices formapping our virtual three-dimensional world to the two-dimensional screen.iv
OpenGL takes care of doing all the math, and simplifies the specification and useof these matrices. We’ll find that using OpenGL, we can easily control:o how our virtual eye views our sceneo position, size, and orientation of the objects in our sceneo the creation of a complex model from the hierarchical placement of itscomponents and suitable transformations (think about a car; each wheel isbasically the same, just positioned at different points on the chassis. We’lluse transformations to put all the things in the right places, and make theentire car move as a single unit). Lighting – simulate how light illuminates the surface of our objects in our scene.In nature, what we see is the result of light reflecting off of our surroundings, andentering our eye. These interactions are quite complicated, and for an interactiveprogram, are too computationally intensive to be completely accurate. OpenGLuses a simplified lighting model to create reasonable lighting effects that usuallysuffice for interactive applications. One point that generally surprises novices toOpenGL is that shadows are not supported. Shadows require considerableknowledge of the scene and the placement of the objects, which is data that’s notgenerally available to OpenGL while it’s drawing. This may seem counterintuitive; however, OpenGL processes each primitive in isolation. Techniquesthat add shadows into an OpenGL scene have been developed. Any of the texts inthe bibliography will contain information on the topic. Depth buffering – determines which geometric primitives are closest to the eye.We take for granted that when an object is behind another object, the one farthestfrom our eye is obscured. Since OpenGL doesn’t enforce a rendering order forthe primitives you ask it to render, depth buffering is used to determine visibilityof objects in your scene. Double buffering – One of the principle goals of the course is to help you developinteractive graphics programs. To move objects around in your scene, you willhave to draw the scene multiple times, moving objects the appropriate amounteach time. In general this approach starts with a “clean slate” for each frame,which requires OpenGL to initialize its framebuffer at the start of each frame.This initialization is generally done by setting all of the pixels to the same color;however, doing this causes the animation to “flicker.” Double buffering is atechnique to remove the flickering from our sequence of frames, and provide asmooth interactive experience.v
Texture mapping – this technique allows for geometric models, perhaps composedof just a few polygons, to have much higher color fidelity. This is accomplishedby determining the color of the pixels filled by our geometric primitives not onlyby the color of that primitive, but from a texture image. The texture containsmuch more color information, and OpenGL kno ws how to extract the colors toproduce a much richer picture than the standard method for shading a polygon.Texture mapping enables a wide variety of techniques that would otherwise beprohibitively complex to do.Presentation Course NotesThe following pages include the slides presented at SIGGRAPH 2004, as well as notes toaid in use of the slides after the talk.vi
SIGGRAPH 2004 - An Interactive Introduction to OpenGL ProgrammingAn Interactive Introduction toOpenGL ProgrammingCourse # 29Dave ShreinerEd AngelVicki Shreiner-1-
SIGGRAPH 2004 - An Interactive Introduction to OpenGL ProgrammingWelcome-2-
SIGGRAPH 2004 - An Interactive Introduction to OpenGL ProgrammingWelcome Today’s Goals and Agenda– Describe OpenGL and its uses– Demonstrate and describe OpenGL’scapabilities and features– Enable you to write an interactive, 3-Dcomputer graphics program in OpenGL-3-
SIGGRAPH 2004 - An Interactive Introduction to OpenGL ProgrammingWhat Is OpenGL, and WhatCan It Do for Me? OpenGL is a computer graphics rendering API– Generate high-quality color images by rendering withgeometric and image primitives– Create interactive applications with 3D graphics OpenGL is operating system independent window system independentOpenGL is a library for drawing, or rendering, computer graphics. Byusing OpenGL, you can create interactive applications that render high-qualitycolor images composed of 3D geometric objects and images.OpenGL is window- and operating-system independent. As such, the partof your application that does rendering is platform independent. However, inorder for OpenGL to be able to render, it needs a window to draw into.Generally, this is controlled by the windowing system on whatever platformyou are working on.-4-
SIGGRAPH 2004 - An Interactive Introduction to OpenGL ProgrammingRelated APIs GLU (OpenGL Utility Library)– part of OpenGL– NURBS, tessellators, quadric shapes, etc. AGL, GLX, WGL– glue between OpenGL and windowing systems GLUT (OpenGL Utility Toolkit)– portable windowing API– not officially part of OpenGLAs mentioned, OpenGL is window and operating system independent. Tointegrate it into various window systems, additional libraries are used tomodify a native window into an OpenGL capable window. Every windowsystem has its own unique library and functions to do this. Some examplesare: GLX for the X Windows system, common on Unix platforms AGL for the Apple Macintosh WGL for Microsoft WindowsOpenGL also includes a utility library, GLU, to simplify common taskssuch as: rendering quadric surfaces (i.e. spheres, cones, cylinders, etc.),working with NURBS and curves, and concave polygon tessellation.Finally to simplify programming and window system dependence, we willbe using the freeware library, GLUT. GLUT, written by Mark Kilgard, is apublic domain window system independent toolkit for making simple OpenGLapplications. GLUT simplifies the process of creating windows, working withevents in the window system and handling animation.-5-
SIGGRAPH 2004 - An Interactive Introduction to OpenGL ProgrammingOpenGL and Related APIsapplication programOpenGL Motifwidget or similarGLUTGLX, AGLor WGLGLUGLX, Win32, Mac O/Ssoftware and/or hardwareThe above diagram illustrates the relationships of the various libraries andwindow system components.Generally, applications which require more user interface support will usea library designed to support those types of features (i.e. buttons, menu andscroll bars, etc.) such as Motif or the Win32 API.Prototype applications, or ones which do not require all the bells andwhistles of a full GUI, may choose to use GLUT instead because of itssimplified programming model and window system independence.-6-
SIGGRAPH 2004 - An Interactive Introduction to OpenGL ProgrammingWhat Is Required For YourPrograms Headers Files#include#include#include#include GL/gl.h GL/glext.h GL/glu.h GL/glut.h Libraries Enumerated Types– OpenGL defines numerous types for compatibility GLfloat, GLint, GLenum, etc.All of our discussions today will be presented in the C computer language.For C, there are a few required elements which an application must do: Header files describe all of the function calls, their parameters anddefined constant values to the compiler. OpenGL has header files forGL (the core library), GLU (the utility library), and GLUT (freewarewindowing toolkit).Note: glut.h includes gl.h and glu.h. On Microsoft Windows,including only glut.h is recommended to avoid warnings aboutredefining Windows macros. Libraries are the operating system dependent implementation ofOpenGL on the system you are using. Each operating system has itsown set of libraries. For Unix systems, the OpenGL library iscommonly named libGL.so (which is usually specified as -lGL onthe compile line) and for Microsoft Windows, it is namedopengl32.lib. Finally, enumerated types are definitions for the basic types (i.e.float, double, int, etc.) which your program uses to store variables. Tosimplify platform independence for OpenGL programs, a complete setof enumerated types are defined. Use them to simplify transferringyour programs to other operating systems.-7-
SIGGRAPH 2004 - An Interactive Introduction to OpenGL ProgrammingOpenGL CommandFormatsglVertex3fv( v )Number ofcomponents2 - (x,y)3 - (x,y,z)4 - (x,y,z,w)Data Typebubsusiuifd-byteunsigned byteshortunsigned shortintunsigned intfloatdoubleVectoromit “v” forscalar formglVertex2f( x, y )The OpenGL API calls are designed to accept almost any basic data type,which is reflected in the calls name. Knowing how the call names arestructured makes it easy to determine which call should be used for a particulardata format and size.For instance, vertices from most commercial models are stored as threecomponent, floating-point vectors. As such, the appropriate OpenGLcommand to use is glVertex3fv(coords).OpenGL considers all points to be 3D. Even if you’re drawing a simple2D line plot, OpenGL considers each vertex to have an x-, y-, and a zcoordinate. In fact, OpenGL really uses homogenous coordinates, which are aset of four numbers (a 4-tuple) that is usually written as (x, y, z, w). The wcoordinate is there to simplify the matrix multiplication that we’ll discuss inthe Transformations section. You can safely ignore w for now.For glVertex*() calls which do not specify all the coordinates (i.e.glVertex2f()), OpenGL will default z 0.0, and w 1.0 .-8-
SIGGRAPH 2004 - An Interactive Introduction to OpenGL ProgrammingThe OpenGL fer Processing is controlled by settingOpenGL’s state– colors, lights and object materials, texturemaps– drawing styles, depth testingOpenGL is a pipelined architecture, which means that the order ofoperations is fixed. In general, OpenGL operations can be partitioned into two“processing units”: vertex operations, and fragment operations.The operation of each pipeline step is controlled by what’s commonlyreferred to as state. State is just the collection of variables that OpenGL keepstrack of internally. They include colors, positions, texture maps, etc. We’lldiscuss many of these state groups during the course. Setting state comprisesabout 80% of the OpenGL functions in the library.-9-
SIGGRAPH 2004 - An Interactive Introduction to OpenGL ProgrammingAn Example OpenGL Program- 10 -
SIGGRAPH 2004 - An Interactive Introduction to OpenGL ProgrammingSequence of Most OpenGLProgramsConfigureand open awindowInitializeOpenGL’sstateProcessuser eventsUpdateOpenGL’sState(if necessary)Draw animageOpenGL was primarily designed to be able to draw high-quality imagesfast enough so that an application could draw many of them a second, andprovide the user with an interactive application, where each frame could becustomized by input from the user.The general flow of an interactive OpenGL application is:1. Configure and open a window suitable for drawing OpenGL into.2. Initialize any OpenGL state that you will need to use throughoutthe application.3. Process any events that the user might have entered. These couldinclude pressing a key on the keyboard, moving the mouse, ormoving or resizing the application’s window.4. Draw your 3D image using OpenGL with values that may havebeen entered from the user’s actions, or other data that the programhas available to it.- 11 -
SIGGRAPH 2004 - An Interactive Introduction to OpenGL ProgrammingAn OpenGL Program#include GL/glut.h #include "cube.h"void main( int argc, char *argv[] ){glutInit( &argc, argv );glutInitDisplayMode( GLUT RGBA GLUT DEPTH );glutCreateWindow( “cube” );init();glutDisplayFunc( display );glutReshapeFunc( reshape );glutMainLoop();The main part ofthe program.GLUT is used toopen the OpenGLwindow, and handleinput from the user.}This slide contains the program statements for the main() routine of a C program thatuses OpenGL and GLUT. For the most part, all of the programs you will see today, andindeed may of the programs available as examples of OpenGL programming that use GLUT,will look very similar to this program.All GLUT-based OpenGL programs begin with configuring the GLUT window to beopened.Next, in the routine init() (detailed on the following slide), we make OpenGL calls toset parameters that we’ll use later in the display() function. These parameters, commonlycalled state , are values that OpenGL uses to determine how it will draw. There’s nothingspecial about the init() routine, we just use it to logically separate the state that we need toset up only once (as compared to every frame).After initialization, we set up our GLUT callback functions, which are routines that youwrite to have OpenGL draw objects and other operations. Callback functions, if you’re notfamiliar with them, make it easy to have a generic library (like GLUT), that can easily beconfigured by providing a few routines of your own construction.Finally, as with all interactive programs, the event loop is entered. For GLUT-basedprograms, this is done by calling glutMainLoop(). As glutMainLoop() never exits (itis essentially an infinite loop), any program statements that follow glutMainLoop() willnever be executed.The header file “cube.h” contains the geometric data (vertices, colors, etc.) for the cubemodel. Cubes are a very popular shape to render in computer graphics (along with teapots we’ll explain that one later), and is a nice example to work through to get a feel for modelingcomputer graphics objects. After the class, try to figure out the geometry for a cube. We’veincluded our “cube.h” in the appendices of the notes for you to compare yours to.- 12 -
SIGGRAPH 2004 - An Interactive Introduction to OpenGL ProgrammingAn OpenGL Program(cont’d.)void init( void ){glClearColor( 0, 0, 0, 1 );gluLookAt( 2, 2, 2, 0, 0, 0, 0, 1, 0 );glEnable( GL DEPTH TEST );}Set up some initialOpenGL statevoid reshape( int width, int height ){glViewport( 0, 0, width, height );glMatrixMode( GL PROJECTION );Handle when theglLoadIdentity();user resizes thegluPerspective( 60, (GLdouble) width / height,window1.0, 10.0 );glMatrixMode( GL MODELVIEW );}First on this slide is the init() routine, which as mentioned, is where weset up the “global” OpenGL state. In this case, init() sets the color that thebackground of the window should be painted to when the window is cleared,as well as configuring where the eye should be located and enabling the depthtest. Although you may not know what these mean at the moment, we willdiscuss each of those topics. What is important to notice is that what we set ininit() remains in affect for the rest of the program’s execution. There isnothing that says we can not turn these features off later; the separation ofthese routines in this manner is purely for clarity in the program’s structure.The reshape() routine is called when the user of a program resizes theapplication’s window. We do a number of things in this routine, all of whichwill be explained in detail in the Transformations section later today.- 13 -
SIGGRAPH 2004 - An Interactive Introduction to OpenGL ProgrammingAn OpenGL Program(cont’d.)void display( void ){int i, j;glClear( GL COLOR BUFFER BIT GL DEPTH BUFFER BIT );glBegin( GL QUADS );for ( i 0; i NUM CUBE FACES; i ) {glColor3fv( faceColor[i] );for ( j 0; j NUM VERTICES PER FACE; j ) {glVertex3fv( vertex[face[i][j]] );}}glEnd();Have OpenGLdraw a cubefrom some3D points(vertices)glFlush();}Finally, we see the display() routine which is used by GLUT to callour OpenGL calls to make our image. Almost all of your OpenGL drawingcode should be called from display() (or routines that display() calls).As with most display()-like functions, a number of common thingsoccur in the following order:1. The window is cleared with a call to glClear(). This will color all ofthe pixels in the window with the color set with glClearColor() (see theprevious slide an
iv Introduction “An Interactive Introduction to OpenGL Programming” provides an overview of the OpenGL Application Programm