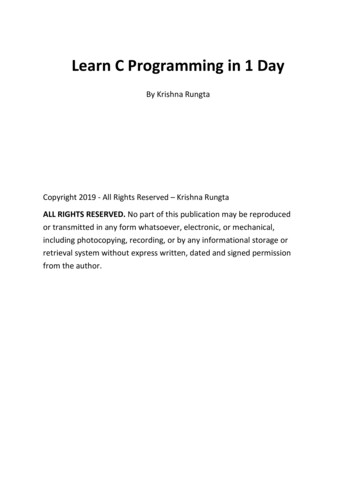
Transcription
Learn C Programming in 1 DayBy Krishna RungtaCopyright 2019 - All Rights Reserved – Krishna RungtaALL RIGHTS RESERVED. No part of this publication may be reproducedor transmitted in any form whatsoever, electronic, or mechanical,including photocopying, recording, or by any informational storage orretrieval system without express written, dated and signed permissionfrom the author.
Table Of ContentChapter 1: What is C Programming Language? Basics, Introductionand History1.2.3.4.5.What is C programming?History of C languageWhere is C used? Key ApplicationsWhy learn ‘C’?How ‘C’ Works?Chapter 2: How to Download & Install GCC Compiler for C in Windows, Linux,Mac1. Install C on Windows2. Install C in Linux3. Install C on MACChapter 3: C Hello World! Example: Your First ProgramChapter 4: How to write Comments in C Programming1.2.3.4.What Is Comment In C Language?Example Single Line CommentExample Multi Line CommentWhy do you need comments?Chapter 5: C Tokens, Keywords, Identifiers, Constants, Variables,Data Types1. What is a Character set?2. Token3. Keywords and Identifiers
4.5.6.7.8.What is a Variable?Data typesInteger data typeFloating point data typeConstantsChapter 6: C Conditional Statement: IF, IF Else and Nested IF Else withExample1.2.3.4.5.6.7.What is a Conditional Statement?If statementRelational OperatorsThe If-Else statementConditional ExpressionsNested If-else StatementsNested Else-if statementsChapter 7: C Loops: For, While, Do While, Break, Continue with Example1.2.3.4.5.6.7.8.What are Loops?Types of LoopsWhile LoopDo-While loopFor loopBreak StatementContinue StatementWhich loop to Select?Chapter 8: Switch Case Statement in C Programming with Example1. What is a Switch Statement?2. Syntax
3.4.5.6.7.Flow Chart Diagram of Switch CaseExampleNested SwitchWhy do we need a Switch case?Rules for switch statement:Chapter 9: C Strings: Declare, Initialize, Read, Print with Example1.2.3.4.5.6.What is a String?Declare and initialize a StringString Input: Read a StringString Output: Print/Display a StringThe string libraryConverting a String to a NumberChapter 10: Storage Classes in C: auto, extern, static, register with Example1.2.3.4.5.What is a Storage Class?Auto storage classExtern storage classStatic storage classRegister storage classChapter 11: C Files I/O: Create, Open, Read, Write and Close a File1.2.3.4.5.How to Create a FileHow to Close a fileWriting to a FileReading data from a FileInteractive File Read and Write with getc and putcChapter 12: Functions in C Programming with Examples:
Recursive, Inline1.2.3.4.5.6.7.8.9.10.What is a Function?Library Vs. User-defined FunctionsFunction DeclarationFunction DefinitionFunction callFunction ArgumentsVariable ScopeStatic VariablesRecursive FunctionsInline FunctionsChapter 13: Pointers in C Programming with Examples1.2.3.4.5.6.7.8.9.What is a Pointer?How does Pointer Work?Types of a pointerDirect and Indirect Access PointersPointers ArithmeticPointers and ArraysPointers and StringsAdvantages of PointersDisadvantages of PointersChapter 14: Functions Pointers in C Programming with ExamplesChapter 15: C Bitwise Operators: AND, OR, XOR, Shift & Complement(with Example)1. What are Bitwise Operators?2. Bitwise AND3. Bitwise OR
4. Bitwise Exclusive OR5. Bitwise shift operators6. Bitwise complement operatorChapter 16: C Dynamic Memory Allocation using malloc(), calloc(),realloc(), free()1.2.3.4.5.6.7.8.How Memory Management in C works?Dynamic memory allocationThe malloc FunctionThe free FunctionThe calloc Functioncalloc vs. malloc: Key DifferencesThe realloc FunctionDynamic ArraysChapter 17: TypeCasting in C: Implicit, Explicit with Example1. What is Typecasting in C?2. Implicit type casting3. Explicit type casting
Chapter 1: What is C ProgrammingLanguage? Basics, Introduction andHistoryWhat is C programming?C is a general-purpose programming language that is extremely popular,simple and flexible. It is machine-independent, structured programminglanguage which is used extensively in various applications.C was the basics language to write everything from operating systems(Windows and many others) to complex programs like the Oracledatabase, Git, Python interpreter and more.It is said that ‘C’ is a god’s programming language. One can say, C is a basefor the programming. If you know ‘C,’ you can easily grasp the knowledgeof the other programming languages that uses the concept of ‘C’It is essential to have a background in computer memory mechanismsbecause it is an important aspect when dealing with the C programminglanguage.
IEEE-the best 10 top programming language in 2018History of C languageThe base or father of programming languages is ‘ALGOL.’ It was firstintroduced in 1960. ‘ALGOL’ was used on a large basis in European countries.‘ALGOL’ introduced the concept of structured programming to the developercommunity. In 1967, a new computer programming language wasannounced called as ‘BCPL’ which stands for Basic Combined ProgrammingLanguage. BCPL was designed and developed by Martin Richards, especiallyfor writing system software. This was the era of programming languages. Justafter three years, in 1970 a new programming language called ‘B’ wasintroduced by Ken Thompson that contained multiple features of ‘BCPL.’ Thisprogramming language was created using UNIX operating system at AT&Tand Bell Laboratories. Both the ‘BCPL’ and ‘B’ were system programminglanguages.
In 1972, a great computer scientist Dennis Ritchie created a newprogramming language called ‘C’ at the Bell Laboratories. It was created from‘ALGOL’, ‘BCPL’ and ‘B’ programming languages. ‘C’ programming languagecontains all the features of these languages and many more additionalconcepts that make it unique from other languages.‘C’ is a powerful programming language which is strongly associated with theUNIX operating system. Even most of the UNIX operating system is coded in‘C’. Initially ‘C’ programming was limited to the UNIX operating system, butas it started spreading around the world, it became commercial, and manycompilers were released for cross- platform systems. Today ‘C’ runs under avariety of operating systems and hardware platforms. As it started evolvingmany different versions of the language were released. At times it becamedifficult for the developers to keep up with the latest version as the systemswere running under the older versions. To assure that ‘C’ language willremain standard, American National Standards Institute (ANSI) defined acommercial standard for ‘C’ language in 1989. Later, it was
approved by the International Standards Organization (ISO) in 1990. ‘C’programming language is also called as ‘ANSI C’.History of CLanguages such as C /Java are developed from ‘C’. These languages arewidely used in various technologies. Thus, ‘C’ forms a base for many otherlanguages that are currently in use.Where is C used? Key Applications1. ‘C’ language is widely used in embedded systems.
2. It is used for developing system applications.3. It is widely used for developing desktop applications.4. Most of the applications by Adobe are developed using ‘C’programming language.5. It is used for developing browsers and their extensions. Google’sChromium is built using ‘C’ programming language.6. It is used to develop databases. MySQL is the most populardatabase software which is built using ‘C’.7. It is used in developing an operating system. Operating systems suchas Apple’s OS X, Microsoft’s Windows, and Symbian are developedusing ‘C’ language. It is used for developing desktop as well as mobilephone’s operating system.8. It is used for compiler production.9. It is widely used in IOT applications.Why learn ‘C’?As we studied earlier, ‘C’ is a base language for many programminglanguages. So, learning ‘C’ as the main language will play an important rolewhile studying other programming languages. It shares the same conceptssuch as data types, operators, control statements and many more. ‘C’ canbe used widely in various applications. It is a simple language and providesfaster execution. There are many jobs available for a ‘C’ developer in thecurrent market.‘C’ is a structured programming language in which program is divided intovarious modules. Each module can be written separately and together itforms a single ‘C’ program. This structure makes it easy for testing,maintaining and debugging processes.‘C’ contains 32 keywords, various data types and a set of powerfulbuilt-in functions that make programming very efficient.Another feature of ‘C’ programming is that it can extend itself. A ‘C’ programcontains various functions which are part of a library. We can add ourfeatures and functions to the library. We can access and use
these functions anytime we want in our program. This feature makes itsimple while working with complex programming.Various compilers are available in the market that can be used forexecuting programs written in this language.It is a highly portable language which means programs written in ‘C’language can run on other machines. This feature is essential if we wishto use or execute the code on another computer.How ‘C’ Works?C is a compiled language. A compiler is a special tool that compiles theprogram and converts it into the object file which is machine readable. Afterthe compilation process, the linker will combine different object files andcreates a single executable file to run the program. The following diagramshows the execution of a ‘C’ programNowadays, various compilers are available online, and you can use any ofthose compilers. The functionality will never differ and most of thecompilers will provide the features required to execute both ‘C’ and ‘C ’programs.Following is the list of popular compilers available online:Clang compilerMinGW compiler (Minimalist GNU for Windows)Portable ‘C’ compilerTurbo C
Summary‘C’ was developed by Dennis Ritchie in 1972. It isa robust language.It is a low programming level language close to machine language It iswidely used in the software development field.It is a procedure and structure oriented language.It has the full support of various operating systems and hardwareplatforms.Many compilers are available for executing programs written in ‘C’.A compiler compiles the source file and generates an object file. Alinker links all the object files together and creates one executablefile.It is highly portable.
Chapter 2: How to Download & InstallGCC Compiler for C in Windows, Linux,MacIn this tutorial, we will learn to install C in Windows, Mac, and Linux.Install C on WindowsWe will use an open-source Integrated Development environment namedCode::Blocks which bundles a compiler (named gcc offered by Free SoftwareFoundation GNU), editor and debugger in a neat package.Step 1) Go to http://www.codeblocks.org/downloads and click BinaryRelease.
Step 2) Choose the installer with GCC Compiler, e.g., codeblocks17.12mingw-setup.exe which includes MinGW’s GNU GCC compiler andGNU GDB debugger with Code::Blocks source files.Step 3) Run the downloaded installer and accept the default options.Step 4) Accept the Agreement
Step 5) Keep the component selection default and click Next.Step 6) You may change the installation folder and click Next.
Step 7) To launch Code::Blocks double click on the icon.Step 8) It will detect the gcc compiler automatically, set it as default.
Associate C/C files with code::blocksStep 9) You will see the IDE Home screen.Install C in LinuxLinux operating systems mostly comes with GCC preinstalled. To verify ifthe compiler is installed on the machine, run the following command inthe terminal:gcc --versionAfter executing this command if the gcc is installed on the machine then itwill return the information about the compiler otherwise it will ask you toinstall the compiler.To set up the ‘C’ environment on Linux distributions follow the givensteps:1. Open terminal.2. For red-hat, Fedora users, type and execute this command# yum groupinstall 'Development Tools'3. For Debian and Ubuntu users, type and execute following command
sudo apt-get update sudo apt-get install build-essential manpages-dev4. To verify that the GCC has been successfully installed on themachine as we discussed earlier, execute the following commandgcc --versionInstall C on MACTo set up a ‘C’ programming environment on MAC operating system,follow the given steps:1. Visit the given tion and download.You will need an Apple developer ID“Command Line Tools for X-Code,” pick any version (latest version isalways recommended) and download the .dmg file.2. After the file is being downloaded on the machine, double click andfollow the wizard and install the file. Always keep the default settings assuggested by the installation wizard.3. After the installation process, open a terminal and run gcc -vcommand to check if everything is successfully installed.Conclusion:‘C’ program can be written and executed on any machine that has asuitable environment to run the program. Its recommended using an IDEto run C programs. An IDE includes a compiler, editor and debugger.Clanfg, MinGW compiler (Minimalist GNU for Windows), Portable ‘C’compiler, Turbo C are popular compilers available.
4. Most of the applications by Adobe are developed using ‘C’ programming language. 5. It is used for developing browsers and their extensions. Google’s Chromium is built using ‘C’ programming language. 6. It is used to develop databases. MySQL is the most popular databa