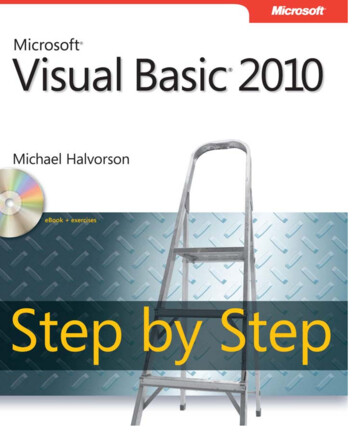
Transcription
PUBLISHED BYMicrosoft PressA Division of Microsoft CorporationOne Microsoft WayRedmond, Washington 98052-6399Copyright 2010 by Michael HalvorsonAll rights reserved. No part of the contents of this book may be reproduced or transmitted in any form or by any meanswithout the written permission of the publisher.Library of Congress Control Number: 2010924441Printed and bound in the United States of America.1 2 3 4 5 6 7 8 9 QWT 5 4 3 2 1 0Distributed in Canada by H.B. Fenn and Company Ltd.A CIP catalogue record for this book is available from the British Library.Microsoft Press books are available through booksellers and distributors worldwide. For further information aboutinternational editions, contact your local Microsoft Corporation office or contact Microsoft Press International directlyat fax (425) 936-7329. Visit our Web site at www.microsoft.com/mspress. Send comments to mspinput@microsoft.com.Microsoft, Microsoft Press, Access, ActiveX, Arc, Azure, DataTips, Excel, Expression, Halo, IntelliSense, Internet Explorer,MS, MSDN, MS-DOS, PowerPoint, SharePoint, Silverlight, SQL Server, Visual Basic, Visual C#, Visual C , VisualInterDev, Visual Studio, Windows, Windows Azure, Windows Server, Windows Vista and Zoo Tycoon are either registeredtrademarks or trademarks of Microsoft Corporation in the United States and/or other countries. Other product and companynames mentioned herein may be the trademarks of their respective owners.The example companies, organizations, products, domain names, e-mail addresses, logos, people, places, and events depictedherein are fictitious. No association with any real company, organization, product, domain name, e-mail address, logo,person, place, or event is intended or should be inferred.This book expresses the author’s views and opinions. The information contained in this book is provided without anyexpress, statutory, or implied warranties. Neither the authors, Microsoft Corporation, nor its resellers, or distributors willbe held liable for any damages caused or alleged to be caused either directly or indirectly by this book.Acquisitions Editor: Ben RyanDevelopmental Editor: Devon MusgraveProject Editor: Valerie WoolleyEditorial Production: Christian Holdener, S4Carlisle Publishing ServicesTechnical Reviewer: Technical Review services provided by Content Master, a member of CM Group, Ltd.Cover: Tom Draper DesignBody Part No. X16-88509
For Henry
Contents at a GlancePart I1234Part II5678910111213Part III14151617Part IVGetting Started with Microsoft Visual Basic 2010Exploring the Visual Studio Integrated DevelopmentEnvironment . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3Writing Your First Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 37Working with Toolbox Controls . . . . . . . . . . . . . . . . . . . . . . . . . . . . 67Working with Menus, Toolbars, and Dialog Boxes . . . . . . . . . . . . 97Programming FundamentalsVisual Basic Variables and Formulas,and the .NET Framework . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 123Using Decision Structures . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 159Using Loops and Timers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 181Debugging Visual Basic Programs . . . . . . . . . . . . . . . . . . . . . . . . 209Trapping Errors by Using Structured Error Handling . . . . . . . . . 227Creating Modules and Procedures . . . . . . . . . . . . . . . . . . . . . . . . 247Using Arrays to Manage Numeric and String Data . . . . . . . . . . . 273Working with Collections . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 297Exploring Text Files and String Processing . . . . . . . . . . . . . . . . . . 313Designing the User InterfaceManaging Windows Forms and Controls at Run Time . . . . . . . .Adding Graphics and Animation Effects . . . . . . . . . . . . . . . . . . .Inheriting Forms and Creating Base Classes . . . . . . . . . . . . . . . .Working with Printers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .351375393415Database and Web Programming18 Getting Started with ADO.NET . . . . . . . . . . . . . . . . . . . . . . . . . . . 44119 Data Presentation Using the DataGridView Control . . . . . . . . . 46720 Creating Web Sites and Web Pages by Using VisualWeb Developer and ASP.NET . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 491v
Table of ContentsAcknowledgments . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . xvIntroduction . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . xviiPart I1Getting Started with Microsoft Visual Basic 2010Exploring the Visual Studio Integrated DevelopmentEnvironment . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3The Visual Studio Development Environment . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4The Visual Studio Tools . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 7The Designer . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10Running a Visual Basic Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11The Properties Window . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13Moving and Resizing the Programming Tools . . . . . . . . . . . . . . . . . . . . . . . . . . . 17Moving and Resizing Tool Windows . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 18Docking Tool Windows . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19Hiding Tool Windows . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 21Switching Among Open Files and Toolsby Using the IDE Navigator . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 22Opening a Web Browser Within Visual Studio . . . . . . . . . . . . . . . . . . . . . . . . . . . 23Getting Help . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 24Managing Help Settings . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 25Using F1 Help . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 26Customizing IDE Settings to MatchStep-by-Step Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 29Setting the IDE for Visual Basic Development . . . . . . . . . . . . . . . . . . . . . . 29Checking Project and Compiler Settings . . . . . . . . . . . . . . . . . . . . . . . . . . 31One Step Further: Exiting Visual Studio . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 33Chapter 1 Quick Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 34What do you think of this book? We want to hear from you!Microsoft is interested in hearing your feedback so we can continually improve our books and learningresources for you. To participate in a brief online survey, please visit:www.microsoft.com/learning/booksurvey/vii
viiiTable of Contents2Writing Your First Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 37Lucky Seven: Your FirstVisual Basic Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 37Programming Steps . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 38Creating the User Interface . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 38Setting the Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 45The Picture Box Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 49Writing the Code . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 52A Look at the Button1 ClickProcedure . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 56Running Visual Basic Applications . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 58Sample Projects on Disk . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 59Building an Executable File . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 60Deploying Your Application . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 62One Step Further: Adding to a Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 63Chapter 2 Quick Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 643Working with Toolbox Controls . . . . . . . . . . . . . . . . . . . . . . . . . . . . 67The Basic Use of Controls: The HelloWorld Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 67Using the DateTimePicker Control . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 73The Birthday Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 73Controls for Gathering Input . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 78Using Group Boxes and Radio Buttons . . . . . . . . . . . . . . . . . . . . . . . . . . . . 81Processing Input with List Boxes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 84A Word About Terminology . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 89One Step Further: Using the LinkLabel Control . . . . . . . . . . . . . . . . . . . . . . . . . . 91Chapter 3 Quick Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 954Working with Menus, Toolbars, and Dialog Boxes . . . . . . . . . . . . 97Adding Menus by Using the MenuStrip Control . . . . . . . . . . . . . . . . . . . . . . . . . 97Adding Access Keys to Menu Commands . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 99Processing Menu Choices . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 102Adding Toolbars with the ToolStrip Control . . . . . . . . . . . . . . . . . . . . . . . . . . . . 107Using Dialog Box Controls . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 110Event Procedures That Manage CommonDialog Boxes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 112One Step Further: Assigning Shortcut Keys to Menus . . . . . . . . . . . . . . . . . . . 117Chapter 4 Quick Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 119
Table of ContentsPart II5Programming FundamentalsVisual Basic Variables and Formulas,and the .NET Framework . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 123The Anatomy of a Visual Basic Program Statement . . . . . . . . . . . . . . . . . . . . . 123Using Variables to Store Information . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 124Setting Aside Space for Variables: The Dim Statement . . . . . . . . . . . . . 124Implicit Variable Declaration . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 126Using Variables in a Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 127Using a Variable to Store Input . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 130Using a Variable for Output . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 133Working with Specific Data Types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 135Constants: Variables That Don’t Change . . . . . . . . . . . . . . . . . . . . . . . . . . 142Working with Visual Basic Operators . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 143Basic Math: The , –, *, and / Operators . . . . . . . . . . . . . . . . . . . . . . . . . . 144Using Advanced Operators: \, Mod, , and & . . . . . . . . . . . . . . . . . . . . . 147Working with Math Methods in the .NET Framework . . . . . . . . . . . . . . . . . . . 152One Step Further: Establishing Order of Precedence . . . . . . . . . . . . . . . . . . . . 155Using Parentheses in a Formula . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 156Chapter 5 Quick Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1566Using Decision Structures . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 159Event-Driven Programming . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 159Using Conditional Expressions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 161If . . . Then Decision Structures . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 161Testing Several Conditions in an If . . . ThenDecision Structure . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 162Using Logical Operators in Conditional Expressions . . . . . . . . . . . . . . . 167Short-Circuiting by Using AndAlso and OrElse . . . . . . . . . . . . . . . . . . . . 169Select Case Decision Structures . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 171Using Comparison Operators with a SelectCase Structure . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 173One Step Further: Detecting Mouse Events . . . . . . . . . . . . . . . . . . . . . . . . . . . . 177Chapter 6 Quick Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1797Using Loops and Timers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 181Writing For . . . Next Loops . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 181Using a Counter Variable in a Multiline TextBox Control . . . . . . . . . . . . . . . . . 183Creating Complex For . . . Next Loops . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 185Using a Counter That Has Greater Scope . . . . . . . . . . . . . . . . . . . . . . . . . 189ix
xTable of ContentsWriting Do Loops . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 192Avoiding an Endless Loop . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 193The Timer Control . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 196Creating a Digital Clock by Using a Timer Control . . . . . . . . . . . . . . . . . . . . . . 197Using a Timer Object to Set a Time Limit . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 200One Step Further: Inserting Code Snippets . . . . . . . . . . . . . . . . . . . . . . . . . . . . 203Chapter 7 Quick Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2078Debugging Visual Basic Programs . . . . . . . . . . . . . . . . . . . . . . . . 209Finding and Correcting Errors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 209Three Types of Errors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 210Identifying Logic Errors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 211Debugging 101: Using Debugging Mode . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 212Tracking Variables by Using a Watch Window . . . . . . . . . . . . . . . . . . . . . . . . . . 217Visualizers: Debugging Tools That Display Data . . . . . . . . . . . . . . . . . . . . . . . . 220Using the Immediate and Command Windows . . . . . . . . . . . . . . . . . . . . . . . . . 221Switching to the Command Window . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 223One Step Further: Removing Breakpoints . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 224Chapter 8 Quick Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2259Trapping Errors by Using Structured Error Handling . . . . . . . . . 227Processing Errors by Using the Try . . . Catch Statement . . . . . . . . . . . . . . . . . . 227When to Use Error Handlers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 228Setting the Trap: The Try . . . Catch Code Block . . . . . . . . . . . . . . . . . . . . 229Path and Disc Drive Errors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 229Writing a Disc Drive Error Handler . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 233Using the Finally Clause to Perform Cleanup Tasks . . . . . . . . . . . . . . . . . . . . . . 234More Complex Try . . . Catch Error Handlers . . . . . . . . . . . . . . . . . . . . . . . . . . . . 236The Exception Object . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 236Specifying a Retry Period . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 239Using Nested Try . . . Catch Blocks . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 242Comparing Error Handlers with DefensiveProgramming Techniques . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 242One Step Further: The Exit Try Statement . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 243Chapter 9 Quick Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 24410 Creating Modules and Procedures . . . . . . . . . . . . . . . . . . . . . . . . 247Working with Modules . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 247Creating a Module . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 248Working with Public Variables . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 251
Table of ContentsCreating Procedures . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 255Writing Function Procedures . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 256Function Syntax . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 257Calling a Function Procedure . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 258Using a Function to Perform a Calculation . . . . . . . . . . . . . . . . . . . . . . . . 258Writing Sub Procedures . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 262Sub Procedure Syntax . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 262Calling a Sub Procedure . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 263Using a Sub Procedure to Manage Input . . . . . . . . . . . . . . . . . . . . . . . . . 264One Step Further: Passing Arguments by Valueand by Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 268Chapter 10 Quick Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 27011 Using Arrays to Manage Numeric and String Data . . . . . . . . . . . 273Working with Arrays of Variables . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 273Creating an Array . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 274Declaring a Fixed-Size Array . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 275Setting Aside Memory . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 276Working with Array Elements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 277Declaring an Array and Assigning It Initial Values . . . . . . . . . . . . . . . . . . 278Creating a Fixed-Size Array to Hold Temperatures . . . . . . . . . . . . . . . . . 279Creating a Dynamic Array . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 283Preserving Array Contents by Using ReDim Preserve . . . . . . . . . . . . . . . . . . . . 287Using ReDim for Three-Dimensional Arrays . . . . . . . . . . . . . . . . . . . . . . 288One Step Further: Processing Large Arraysby Using Methods in the Array Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 288The Array Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 288Chapter 11 Quick Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 29512 Working with Collections . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 297Working with Object Collections . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 297Referencing Objects in a Collection . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 298Writing For Each . . . Next Loops . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 298Experimenting with Objects in the Controls Collection . . . . . . . . . . . . . 299Using the Name Property in a For Each . . . Next Loop . . . . . . . . . . . . . . 302Creating Your Own Collections . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 304Declaring New Collections . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 304One Step Further: VBA Collections . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 309Entering the Word Macro . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 310Chapter 12 Quick Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 311xi
xiiTable of Contents13 Exploring Text Files and String Processing . . . . . . . . . . . . . . . . . . 313Reading Text Files . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 313The My Namespace . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 314The StreamReader Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 316Using the ReadAllText Method . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 317Writing Text Files . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 321The WriteAllText Method . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 321The StreamWriter Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 322Using the WriteAllText Method . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 323Processing Strings with the String Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 326Sorting Text . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 329Working with ASCII Codes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 330Sorting Strings in a Text Box . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 331Examining the Sort Text Program Code . . . . . . . . . . . . . .
with Visual Basic ." Part I Getting Started with Microsoft Visual Basic 2010. for Visual Basic development., , . .