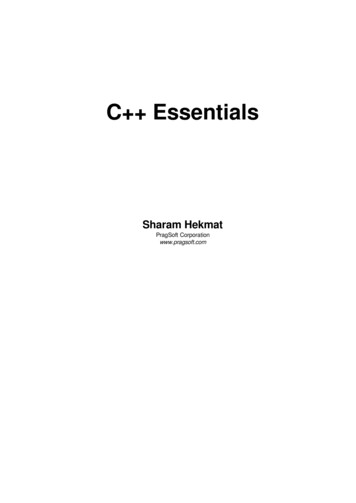
Transcription
C EssentialsSharam HekmatPragSoft Corporationwww.pragsoft.com
ContentsContentsvPrefacex1. PreliminariesA Simple C ProgramCompiling a Simple C ProgramHow C Compilation WorksVariablesSimple Input/OutputCommentsMemoryInteger NumbersReal 2131415162. ExpressionsArithmetic OperatorsRelational OperatorsLogical OperatorsBitwise OperatorsIncrement/Decrement OperatorsAssignment OperatorConditional OperatorComma OperatorThe sizeof OperatorOperator PrecedenceSimple Type ragsoft.comContentsv
vi3. StatementsSimple and Compound StatementsThe if StatementThe switch StatementThe while StatementThe do StatementThe for StatementThe continue StatementThe break StatementThe goto StatementThe return StatementExercises3031323436373840414243444. FunctionsA Simple FunctionParameters and ArgumentsGlobal and Local ScopeScope OperatorAuto VariablesRegister VariablesStatic Variables and FunctionsExtern Variables and FunctionsSymbolic ConstantsEnumerationsRuntime StackInline FunctionsRecursionDefault ArgumentsVariable Number of ArgumentsCommand Line 63645. Arrays, Pointers, and ReferencesArraysMultidimensional ArraysPointersDynamic MemoryPointer ArithmeticFunction 77980C EssentialsCopyright 2005 PragSoft
6. ClassesA Simple ClassInline Member FunctionsExample: A Set ClassConstructorsDestructorsFriendsDefault ArgumentsImplicit Member ArgumentScope OperatorMember Initialization ListConstant MembersStatic MembersMember PointersReferences MembersClass Object MembersObject ArraysClass ScopeStructures and UnionsBit 51061081101121137. OverloadingFunction OverloadingOperator OverloadingExample: Set OperatorsType ConversionExample: Binary Number ClassOverloading for OutputOverloading for InputOverloading []Overloading ()Memberwise InitializationMemberwise AssignmentOverloading new and deleteOverloading - , *, and &Overloading and 381421438. Derived ClassesAn illustrative ClassA Simple Derived Class145146150www.pragsoft.comContentsvii
Class Hierarchy NotationConstructors and DestructorsProtected Class MembersPrivate, Public, and Protected Base ClassesVirtual FunctionsMultiple InheritanceAmbiguityType ConversionInheritance and Class Object MembersVirtual Base ClassesOverloaded 651671689. TemplatesFunction Template DefinitionFunction Template InstantiationExample: Binary SearchClass Template DefinitionClass Template InstantiationNontype ParametersClass Template SpecializationClass Template MembersClass Template FriendsExample: Doubly-linked ListsDerived Class 218618710. Exception HandlingFlow ControlThe Throw ClauseThe Try Block and Catch ClausesFunction Throw ListsExercises18818919019219419511. The IO LibraryThe Role of streambufStream Output with ostreamStream Input with istreamUsing the ios ClassStream ManipulatorsFile IO with fstreamsArray IO with strstreamsExample: Program Annotation196198199201204209210212214C EssentialsCopyright 2005 PragSoft
Exercises21712. The PreprocessorPreprocessor DirectivesMacro DefinitionQuote and Concatenation OperatorsFile InclusionConditional CompilationOther DirectivesPredefined utions to Exercises230www.pragsoft.comContentsix
PrefaceSince its introduction less than a decade ago, C has experienced growingacceptance as a practical object-oriented programming language suitable forteaching, research, and commercial software development. The language has alsorapidly evolved during this period and acquired a number of new features (e.g.,templates and exception handling) which have added to its richness.This book serves as an introduction to the C language. It teaches how toprogram in C and how to properly use its features. It does not attempt to teachobject-oriented design to any depth, which I believe is best covered in a book in itsown right.In designing this book, I have strived to achieve three goals. First, to producea concise introductory text, free from unnecessary verbosity, so that beginners candevelop a good understanding of the language in a short period of time. Second, Ihave tried to combine a tutorial style (based on explanation of concepts throughexamples) with a reference style (based on a flat structure). As a result, eachchapter consists of a list of relatively short sections (mostly one or two pages), withno further subdivision. This, I hope, further simplifies the reader’s task. Finally, Ihave consciously avoided trying to present an absolutely complete description ofC . While no important topic has been omitted, descriptions of some of the minoridiosyncrasies have been avoided for the sake of clarity and to avoidoverwhelming beginners with too much information. Experience suggests that anysmall knowledge gaps left as a result, will be easily filled over time through selfdiscovery.Intended AudienceThis book introduces C as an object-oriented programming language. Noprevious knowledge of C or any other programming language is assumed. ReadersxC EssentialsCopyright 2005 PragSoft
who have already been exposed to a high-level programming language (such as Cor Pascal) will be able to skip over some of the earlier material in this book.Although the book is primarily designed for use in undergraduate computerscience courses, it will be equally useful to professional programmers and hobbyistswho intend to learn the language on their own. The entire book can be easilycovered in 10-15 lectures, making it suitable for a one-term or one-semestercourse. It can also be used as the basis of an intensive 4-5 day industrial trainingcourse.Structure of the BookThe book is divided into 12 chapters. Each chapter has a flat structure, consistingof an unnumbered sequence of sections, most of which are limited to one or twopages. The aim is to present each new topic in a confined space so that it can bequickly grasped. Each chapter ends with a list of exercises. Answers to all of theexercises are provided in an appendix. Readers are encouraged to attempt as manyof the exercises as feasible and to compare their solutions against the onesprovided.For the convenience of readers, the sample programs presented in this book(including the solutions to the exercises) and provided in electronic form.www.pragsoft.comContentsxi
1.PreliminariesThis chapter introduces the basic elements of a C program. We will use simpleexamples to show the structure of C programs and the way they are compiled.Elementary concepts such as constants, variables, and their storage in memory willalso be discussed.The following is a cursory description of the concept of programming for thebenefit of those who are new to the subject.ProgrammingA digital computer is a useful tool for solving a great variety of problems. Asolution to a problem is called an algorithm; it describes the sequence of steps tobe performed for the problem to be solved. A simple example of a problem and analgorithm for it would be:Problem:Algorithm:Sort a list of names in ascending lexicographic order.Call the given list list1; create an empty list, list2, to hold the sorted list.Repeatedly find the ‘smallest’ name in list1, remove it from list1, andmake it the next entry of list2, until list1 is empty.An algorithm is expressed in abstract terms. To be intelligible to a computer, itneeds to be expressed in a language understood by it. The only language reallyunderstood by a computer is its own machine language. Programs expressed inthe machine language are said to be executable. A program written in any otherlanguage needs to be first translated to the machine language before it can beexecuted.A machine language is far too cryptic to be suitable for the direct use ofprogrammers. A further abstraction of this language is the assembly languagewhich provides mnemonic names for the instructions and a more intelligible notationfor the data. An assembly language program is translated to machine language by atranslator called an assembler.Even assembly languages are difficult to work with. High-level languages suchas C provide a much more convenient notation for implementing algorithms.They liberate programmers from having to think in very low-level terms, and helpthem to focus on the algorithm instead. A program written in a high-level languageis translated to assembly language by a translator called a compiler. The assemblycode produced by the compiler is then assembled to produce an executableprogram.www.pragsoft.comChapter 1: Preliminaries1
A Simple C ProgramListing 1.1 shows our first C program, which when run, simply outputs themessage Hello World.Listing 1.112345#include iostream.h int main (void){cout "Hello World\n";}Annotation21This line uses the preprocessor directive #include to include the contentsof the header file iostream.h in the program. Iostream.h is a standardC header file and contains definitions for input and output.2This line defines a function called main. A function may have zero or moreparameters; these always appear after the function name, between a pair ofbrackets. The word void appearing between the brackets indicates that mainhas no parameters. A function may also have a return type; this alwaysappears before the function name. The return type for main is int (i.e., aninteger number). All C programs must have exactly one main function.Program execution always begins from main.3This brace marks the beginning of the body of main.4This line is a statement. A statement is a computation step which mayproduce a value. The end of a statement is always marked with a semicolon(;). This statement causes the string "Hello World\n" to be sent to thecout output stream. A string is any sequence of characters enclosed indouble-quotes. The last character in this string (\n) is a newline characterwhich is similar to a carriage return on a type writer. A stream is an objectwhich performs input or output. Cout is the standard output stream in C (standard output usually means your computer monitor screen). The symbol is an output operator which takes an output stream as its left operand andan expression as its right operand, and causes the value of the latter to besent to the former. In this case, the effect is that the string "Hello World\n"is sent to cout, causing it to be printed on the computer monitor screen.5This brace marks the end of the body of main.C Essentials Copyright 2005 PragSoft
Compiling a Simple C ProgramDialog 1.1 shows how the program in Listing 1.1 is compiled and run in a typicalUNIX environment. User input appears in bold and system response in plain.The UNIX command line prompt appears as a dollar symbol ( ).Dialog 1.11234 CC hello.cc a.outHello World Annotation1The command for invoking the AT&T C translator in a UNIX environmentis CC. The argument to this command (hello.cc) is the name of the file whichcontains the program. As a convention, the file name should end in .c, .C, or.cc. (This ending may be different in other systems.)2The result of compilation is an executable file which is by default nameda.out. To run the program, we just use a.out as a command.3This is the output produced by the program.4The return of the system prompt indicates that the program has completed itsexecution.The CC command accepts a variety of useful options. An option appears as name, where name is the name of the option (usually a single letter). Some optionstake arguments. For example, the output option (-o) allows you to specify a namefor the executable file produced by the compiler instead of a.out. Dialog 1.Error!Bookmark not defined. illustrates the use of this option by specifying hello asthe name of the executable file.Dialog 1.21234 CC hello.cc -o hello helloHello World Although the actual command may be different depending on the make of thecompiler, a similar compilation procedure is used under MS-DOS. Windowsbased C compilers offer a user-friendly environment where compilation is assimple as choosing a menu command. The naming convention under MS-DOS andWindows is that C source file names should end in .cpp. www.pragsoft.comChapter 1: Preliminaries3
How C Compilation WorksCompiling a C program involves a number of steps (most of which aretransparent to the user): First, the C preprocessor goes over the program text and carries out theinstructions specified by the preprocessor directives (e.g., #include). Theresult is a modified program text which no longer contains any directives.(Chapter 12 describes the preprocessor in detail.) Then, the C compiler translates the program code. The compiler may be atrue C compiler which generates native (assembly or machine) code, or justa translator which translates the code into C. In the latter case, the resulting Ccode is then passed through a C compiler to produce native object code. Ineither case, the outcome may be incomplete due to the program referring tolibrary routines which are not defined as a part of the program. For example,Listing 1.1 refers to the operator which is actually defined in a separate IOlibrary. Finally, the linker completes the object code by linking it with the object codeof any library modules that the program may have referred to. The final resultis an executable file.Figure 1.1 illustrates the above steps for both a C translator and a C nativecompiler. In practice all these steps are usually invoked by a single command (e.g.,CC) and the user will not even see the intermediate files generated.Figure 1.1 C CompilationC ProgramC TRANSLATORC ProgramC able 4C EssentialsCopyright 20
C Essentials Sharam Hekmat PragSoft Corporation www.pragsoft.com. www.pragsoft.com Contents v Contents Contents v Preface x 1. Preliminaries 1 A Simple C Program 2 Compiling a Simple C Program 3 How C Compilation Works 4 Variables 5 Simple Input/Output 7 Comments 9 Memory 10 Integer Numbers 11 Real Numbers 12 Characters 13 Strings 14 Names 15 Exercises 16 2. File Size: 540KBPage Count: 311