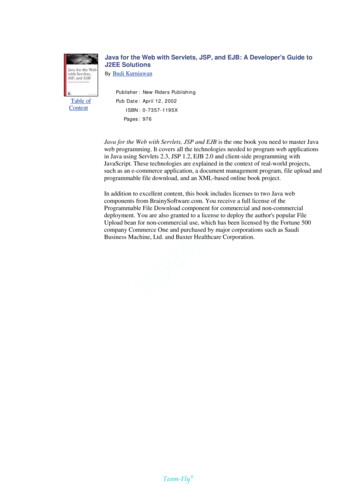
Transcription
Java for the Web with Servlets, JSP, and EJB: A Developer's Guide toJ2EE SolutionsByBudi KurniawanPublisher : New Riders PublishingPub Date : April 12, 2002ISBN : 0-7357-1195XPages : 976Java for the Web with Servlets, JSP and EJB is the one book you need to master Javaweb programming. It covers all the technologies needed to program web applicationsin Java using Servlets 2.3, JSP 1.2, EJB 2.0 and client-side programming withJavaScript. These technologies are explained in the context of real-world projects,such as an e-commerce application, a document management program, file upload andprogrammable file download, and an XML-based online book project.AMFLYIn addition to excellent content, this book includes licenses to two Java webcomponents from BrainySoftware.com. You receive a full license of theProgrammable File Download component for commercial and non-commercialdeployment. You are also granted to a license to deploy the author's popular FileUpload bean for non-commercial use, which has been licensed by the Fortune 500company Commerce One and purchased by major corporations such as SaudiBusiness Machine, Ltd. and Baxter Healthcare Corporation.TETable ofContentTeam-Fly
Table of ContentTable of Content . iiCopyright . ixCopyright 2002 by New Riders Publishing. ixTrademarks . ixWarning and Disclaimer . ixAbout the Author . xiAbout the Technical Reviewers . xiAcknowledgments . xiTell Us What You Think. xiiIntroduction. xiiThe Hypertext Transfer Protocol (HTTP) . xivSystem Architecture. xviiJava 2, Enterprise Edition (J2EE). xviiiDeveloping Web Applications in Java. xviiiOverview of Parts and Chapters. xixPart I: Building Java Web Applications. 1Chapter 1. The Servlet Technology . 2The Benefits of Servlets . 2Servlet Application Architecture. 4How a Servlet Works . 5The Tomcat Servlet Container . 5Six Steps to Running Your First Servlet . 6Summary . 11Chapter 2. Inside Servlets. 12The javax.servlet Package. 12A Servlet's Life Cycle. 13Obtaining Configuration Information . 16Preserving the ServletConfig. 18The Servlet Context . 19Sharing Information Among Servlets . 21Requests and Responses. 23The GenericServlet Wrapper Class . 28Creating Thread-Safe Servlets. 29Summary . 34Chapter 3. Writing Servlet Applications . 35The HttpServlet Class. 35The HttpServletRequest Interface . 39HttpServletResponse. 48Sending an Error Code. 51Sending Special Characters . 51Buffering the Response. 55Populating HTML Elements. 56Request Dispatching. 57Summary . 65Chapter 4. Accessing Databases with JDBC . 67The java.sql Package . 67ii
Four Steps to Getting to the Database . 71A Database-Based Login Servlet. 79The Single Quote Factor. 84Inserting Data into a Table with RegistrationServlet . 86Displaying All Records. 96Search Page . 98An Online SQL Tool. 102Should I Keep the Connection Open? . 108Transactions. 109Connection Pooling. 110Summary . 110Chapter 5. Session Management . 111What Is Session Management?. 111URL Rewriting. 113Hidden Fields . 125Cookies . 138Session Objects. 152Knowing Which Technique to Use . 160Summary . 161Chapter 6. Application and Session Events. 162Listening to Application Events . 162Listening to HttpSession Events . 171Summary . 177Chapter 7. Servlet Filtering . 178An Overview of the API . 178A Basic Filter. 180Mapping a Filter with a URL . 182A Logging Filter. 183Filter Configuration. 185A Filter that Checks User Input . 186Filtering the Response . 192Filter Chain . 195Summary . 199Chapter 8. JSP Basics . 200What's Wrong with Servlets?. 200Running Your First JSP. 202How JSP Works. 206The JSP Servlet Generated Code. 206The JSP API. 208The Generated Servlet Revisited . 210Implicit Objects . 214Summary . 217Chapter 9. JSP Syntax . 218Directives . 218Scripting Elements . 229Standard Action Elements . 239Comments . 240Converting into XML Syntax . 241Summary . 242Chapter 10. Developing JSP Beans. 243iii
Calling Your Bean from a JSP Page. 243A Brief Theory of JavaBeans. 245Making a Bean Available . 246Accessing Properties Using jsp:getProperty and jsp:setProperty . 250Setting a Property Value from a Request. 252JavaBeans Code Initialization . 254The SQLToolBean Example. 255Summary . 262Chapter 11. Using JSP Custom Tags . 264Writing Your First Custom Tag. 265The Role of the Deployment Descriptor . 269The Tag Library Descriptor. 270The Custom Tag Syntax . 272The JSP Custom Tag API. 273The Life Cycle of a Tag Handler . 274Summary . 285Chapter 12. Programmable File Download. 286Keys to Programmable File Download . 287Using the Brainysoftware.com File Download Bean . 288Summary . 288Chapter 13. File Upload . 290The HTTP Request . 290Client-Side HTML . 294HTTP Request of an Uploaded File . 295Uploading a File. 298FileUpload Bean . 302Multiple File Upload . 303Summary . 304Chapter 14. Security Configuration . 305Imposing Security Constraints . 305Allowing Multiple Roles . 312Form-Based Authentication . 313Digest Authentication. 316Methods Related to Security . 317Restricting Certain Methods . 319Summary . 319Chapter 15. Caching. 320Caching Data into a Text File . 320Caching in Memory . 325Summary . 330Chapter 16. Application Deployment . 331Application Directory Structure . 331Deployment Descriptor. 333Servlet Alias and Mapping . 351JSP Alias and Mapping . 353Packaging and Deploying a Web Application. 355Summary . 355Chapter 17. Architecting Java Web Applications . 356Model 1 Architecture. 356Model 2 Architecture. 361iv
Summary . 364Chapter 18. Developing E-Commerce Applications . 365Project Specification . 365The Database Structure . 366Page Design. 367Preparation. 367Application Design . 368Building the Project . 371Summary . 388Chapter 19. XML-Based E-Books . 389The Table of Contents. 390Translating XML into the Object Tree . 390The Project . 392Pre-Render the Table of Contents. 403Summary . 403Chapter 20. Web-Based Document Management. 404The Docman Project . 405Summary . 437Part II: Client-Side Programming with JavaScript . 439Chapter 21. JavaScript Basics . 440Introduction to JavaScript . 440Adding JavaScript Code to HTML . 450JavaScript Object Model . 452Event Handler . 453Window and String Objects . 454Summary . 457Chapter 22. Client-Side Programming Basics. 458Checking Whether JavaScript Is Enabled. 458Handling JavaScript-Unaware Browsers. 461Handling Different Versions of JavaScript. 461Including a JavaScript File. 462Checking the Operating System . 463Checking the Browser Generation . 464Checking the Browser Type . 465Checking the Browser Language . 465Handling Dynamic Variable-Names . 466Summary . 467Chapter 23. Redirection . 468Anticipating Failed Redirection . 468Using the Refresh Meta Tag . 468Using the location Object . 469Going Back to the Previous Page. 470Moving Forward. 471Navigation with a SELECT Element. 472Summary . 473Chapter 24. Client-Side Input Validation . 474The isEmpty Function. 474The trim Function . 475The trimAll Function . 477The isPositiveInteger Function. 477v
The isValidPhoneNumber Function . 478The isMoney Function . 479The isUSDate and isOZDate Functions . 480Converting Date Formats. 483Data Type Conversion: String to Numeric. 483Data Type Conversion: Numeric to String. 485Using the Validation Functions. 485Summary . 487Chapter 25. Working with Client-Side Cookies . 488Creating Cookies with a META Tag . 488Creating Cookies with document.cookie . 489Creating Cookies with the setCookie Function. 490Reading Cookies on the Browser . 492Deleting a Cookie on the Browser. 493Checking If the Browser Can Accept Cookies Using JavaScript. 494Checking If the Browser Accepts Cookies Without JavaScript. 495Summary . 495Chapter 26. Working with Object Trees. 496The Array Object . 496Truly Deleting an Array Element. 499Creating an Object . 501A Hierarchy of Objects. 501Summary . 513Chapter 27. Controlling Applets. 514Is Java Enabled?. 514Is the Applet Ready? . 515Resizing an Applet . 516Calling an Applet's Method . 516Getting an Applet's Property. 517Setting an Applet Property. 518Using Java Classes Directly . 519Applet-to-JavaScript Communication. 520Accessing the Document Object Model from an Applet . 522Invoking JavaScript Functions from an Applet . 523Evaluating a JavaScript Statement from an Applet . 524Setting the Applet Parameter . 525Applet-to-Applet Communication Through JavaScript . 526Direct Applet-to-Applet Communication . 528Summary . 530Part III: Developing Scalable Applications with EJB. 531Chapter 28. Enterprise JavaBeans . 532What Is an Enterprise JavaBean? . 532Benefits of EJB . 533EJB Application Architecture . 533The Six EJB Roles . 534Types of Enterprise Beans . 535Writing Your First Enterprise Bean. 535EJB Explained. 538Writing Client Applications . 541Creating a Bean's Instance. 544vi
Summary . 547Chapter 29. The Session Bean. 548What Is a Session Bean?. 548Stateful and Stateless Session Beans. 548Writing a Session Bean . 549The Tassie Online Bookstore Example . 554Summary . 572Chapter 30. Entity Beans . 573What Is an Entity Bean?. 573The Remote Interface. 574The Home Interface . 574The Primary Key Class.
Java for the Web with Servlets, JSP and EJB is the one book you need to master Java web programming. It covers all the technologies needed to program web applications in Java using Servlets 2.3, JSP 1.2, EJB 2.0 and client-side programming with JavaScript. These technologies are explained in the context of real-world projects,