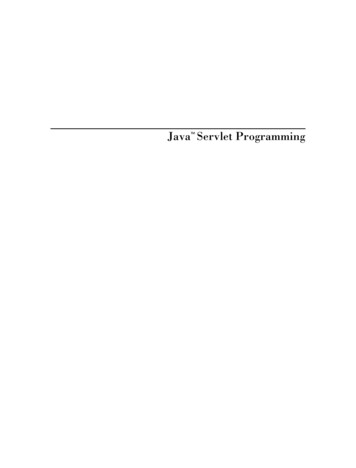
Transcription
Java Servlet Programming
Exploring Java Java ThreadsJava Network ProgrammingJava Virtual MachineJava AWT ReferenceJava Language ReferenceJava Fundamental Classes ReferenceDatabase Programming with JDBC and Java Java Distributed ComputingDeveloping Java Beans Java SecurityJava CryptographyJava SwingJava Servlet ProgrammingAlso from O’ReillyJava in a NutshellJava in a Nutshell, Deluxe EditionJava Examples in a Nutshell
Java Servlet ProgrammingJason Hunterwith William CrawfordBeijing Cambridge Farnham Köln Paris Sebastopol Taipei Tokyo
Java Servlet Programmingby Jason Hunter with William CrawfordCopyright 1998 O’Reilly & Associates, Inc. All rights reserved.Printed in the United States of America.Published by O’Reilly & Associates, Inc., 101 Morris Street, Sebastopol, CA 95472.Editor: Paula FergusonProduction Editor: Paula CarrollEditorial and Production Services: Benchmark Productions, Inc.Printing History:October 1998:First EditionNutshell Handbook, the Nutshell Handbook logo, and the O’Reilly logo are registeredtrademarks and The Java Series is a trademark of O’Reilly & Associates, Inc. The associationof the image of a copper teakettle with the topic of Java Servlet programming is a trademarkof O’Reilly & Associates, Inc. Java and all Java-based trademarks and logos are trademarksor registered trademarks of Sun Microsystems, Inc., in the United States and other countries.O’Reilly & Associates, Inc. is independent of Sun Microsystems.Many of the designations used by manufacturers and sellers to distinguish their products areclaimed as trademarks. Where those designations appear in this book, and O’Reilly &Associates, Inc. was aware of a trademark claim, the designations have been printed in capsor initial caps.While every precaution has been taken in the preparation of this book, the publisher assumesno responsibility for errors or omissions, or for damages resulting from the use of theinformation contained herein.ISBN: 1-56592-391-X[M][1/00]
opd0:Table of ContentsPreface . ix1. Introduction . 1History of Web Applications . 1Support for Servlets . 7The Power of Servlets . 102. HTTP Servlet Basics . 14HTTP Basics . 14The Servlet API . 17Page Generation . 19Server-Side Includes . 27Servlet Chaining and Filters . 30JavaServer Pages . 37Moving On . 463. The Servlet Life Cycle . 48The Servlet Alternative . 48Servlet Reloading . 55Init and Destroy . 56Single-Thread Model . 62Background Processing . 64Last Modified Times . 67vJava Servlet Programming, eMatter EditionCopyright 2000 O’Reilly & Associates, Inc. All rights reserved.0:
viTABLE OF CONTENTS4. Retrieving Information . 70Initialization Parameters . 72The Server . 74The Client . 79The Request . 845. Sending HTML Information . 124The Structure of a Response . 124Sending a Normal Response . 125Using Persistent Connections . 127HTML Generation . 129Status Codes . 142HTTP Headers . 145When Things Go Wrong . 1516. Sending Multimedia Content . 159Images . 159Compressed Content . 188Server Push . 1917. Session Tracking . 195User Authorization . 196Hidden Form Fields . 197URL Rewriting . 200Persistent Cookies . 202The Session Tracking API . 2068. Security . 221HTTP Authentication . 222Digital Certificates . 232Secure Sockets Layer (SSL) . 234Running Servlets Securely . 2379. Database Connectivity . 242Relational Databases . 243The JDBC API . 246Java Servlet Programming, eMatter EditionCopyright 2000 O’Reilly & Associates, Inc. All rights reserved.
TABLE OF CONTENTSviiReusing Database Objects . 259Transactions . 261Advanced JDBC Techniques . 27210. Applet-Servlet Communication . 277Communication Options . 277Daytime Server . 284Chat Server . 31711. Interservlet Communication . 337Servlet Manipulation . 337Servlet Reuse . 342Servlet Collaboration . 349Recap . 36312. Internationalization . 365Western European Languages . 366Conforming to Local Customs . 369Non-Western European Languages . 371Multiple Languages . 376Dynamic Language Negotiation . 379HTML Forms . 389Receiving Multilingual Input . 39513. Odds and Ends . 397Parsing Parameters . 397Sending Email . 401Using Regular Expressions . 404Executing Programs . 407Using Native Methods . 412Acting as an RMI Client . 413Debugging . 415Performance Tuning . 423Java Servlet Programming, eMatter EditionCopyright 2000 O’Reilly & Associates, Inc. All rights reserved.
viiiTABLE OF CONTENTSA. Servlet API Quick Reference . 425B. HTTP Servlet API Quick Reference . 447C. HTTP Status Codes . 472D. Character Entities . 478E. Charsets . 484Index . 487Java Servlet Programming, eMatter EditionCopyright 2000 O’Reilly & Associates, Inc. All rights reserved.
0.PrefaceIn late 1996, Java on the server side was coming on strong. Several major softwarevendors were marketing technologies specifically aimed at helping server-side Javadevelopers do their jobs more efficiently. Most of these products provided a prebuilt infrastructure that could lift the developer’s attention from the raw socketlevel into the more productive application level. For example, Netscape introduced something it named “server-side applets”; the World Wide Web Consortiumincluded extensible modules called “resources” with its Java-based Jigsaw webserver; and with its WebSite server, O’Reilly Software promoted the use of a technology it (only coincidentally) dubbed “servlets.” The drawback: each of thesetechnologies was tied to a particular server and designed for very specific tasks.Then, in early 1997, JavaSoft (a company that has since been reintegrated into SunMicrosystems as the Java Software division) finalized Java servlets. This actionconsolidated the scattered technologies into a single, standard, generic mechanism for developing modular server-side Java code. Servlets were designed to workwith both Java-based and non-Java-based servers. Support for servlets has sincebeen implemented in nearly every web server, from Apache to Zeus, and in manynon-web servers as well.Servlets have been quick to gain acceptance because, unlike many new technologies that must first explain the problem or task they were created to solve, servletsare a clear solution to a well-recognized and widespread need: generating dynamicweb content. From corporations down to individual web programmers, peoplewho struggled with the maintenance and performance problems of CGI-based webprogramming are turning to servlets for their power, portability, and efficiency.Others, who were perhaps intimidated by CGI programming’s apparent relianceon manual HTTP communication and the Perl and C languages, are looking toservlets as a manageable first step into the world of web programming.ixJava Servlet Programming, eMatter EditionCopyright 2000 O’Reilly & Associates, Inc. All rights reserved.0:
xPREFACEThis book explains everything you need to know about Java servlet programming.The first five chapters cover the basics: what servlets are, what they do, and howthey work. The following eight chapters are where the true meat is—they explorethe things you are likely to do with servlets. You’ll find numerous examples, severalsuggestions, a few warnings, and even a couple of true hacks that somehow made itpast technical review.We cover Version 2.0 of the Servlet API, which was introduced as part of the JavaWeb Server 1.1 in December 1997 and clarified by the release of the Java ServletDevelopment Kit 2.0 in April 1998. Changes in the API from Version 1.0, finalizedin June 1997, are noted throughout the text.AudienceIs this book for you? It is if you’re interested in extending the functionality of aserver—such as extending a web server to generate dynamic content. Specifically,this book was written to help:CGI programmersCGI is a popular but somewhat crude method of extending the functionalityof a web server. Servlets provide an elegant, efficient alternative.NSAPI, ISAPI, ASP, and Server-Side JavaScript programmersEach of these technologies can be used as a CGI alternative, but each has limitations regarding portability, security, and/or performance. Servlets tend toexcel in each of these areas.Java applet programmersIt has always been difficult for an applet to talk to a server. Servlets make iteasier by giving the applet an easy-to-connect-to, Java-based agent on theserver.Authors of web pages with server-side includesPages that use server-side includes to call CGI programs can use SERVLET tags to add content more efficiently to a page.Authors of web pages with different appearancesBy this we mean pages that must be available in different languages, have to beconverted for transmission over a low-bandwidth connection, or need to bemodified in some manner before they are sent to the client. Servlets providesomething called servlet chaining that can be used for processing of this type.Each servlet in a servlet chain knows how to catch, process, and return aspecific kind of content. Thus, servlets can be linked together to do languagetranslation, change large color images to small black-and-white ones, convertimages in esoteric formats to standard GIF or JPEG images, or nearly anythingelse you can think of.Java Servlet Programming, eMatter EditionCopyright 2000 O’Reilly & Associates, Inc. All rights reserved.
PREFACExiWhat You Need to KnowWhen we first started writing this book, we found to our surprise that one of thehardest things was determining what to assume about you, the reader. Are youfamiliar with Java? Have you done CGI or other web application programmingbefore? Or are you getting your feet wet with servlets? Do you understand HTTPand HTML, or do those acronyms seem perfectly interchangeable? No matterwhat experience level we imagined, it was sure to be too simplistic for some andtoo advanced for others.In the end, this book was written with the notion that it should contain predominantly original material: it could leave out exhaustive descriptions of topics andconcepts that are well described online or in other books. Scattered throughoutthe text, you’ll find several references to these external sources of information.Of course, external references only get you so far. This book expects you arecomfortable with the Java programming language and basic object-orientedprogramming techniques. If you are coming to servlets from another language, wesuggest you prepare yourself by reading a book on general Java programming,such as Exploring Java, by Patrick Niemeyer and Joshua Peck (O’Reilly). You maywant to skim quickly the sections on applets and AWT (graphical) programmingand spend extra time on network and multithreaded programming. If you want toget started with servlets right away and learn Java as you go, we suggest you readthis book with a copy of Java in a Nutshell, by David Flanagan (O’Reilly), oranother Java reference book, at your side.This book does not assume you have extensive experience with web programming,HTTP, and HTML. But neither does it provide a full introduction to or exhaustive description of these technologies. We’ll cover the basics necessary for effectiveservlet development and leave the finer points (such as a complete list of HTMLtags and HTTP 1.1 headers) to other sources.About the ExamplesIn this book you’ll find nearly 100 servlet examples. The code for these servlets isall contained within the text, but you may prefer to download the examples ratherthan type them in by hand. You can find the code online and packaged for download at http://www.oreilly.com/catalog/jservlet/. You can also see many of the servletsin action at http://www.servlets.com.All the examples have been tested using Sun’s Java Web Server 1.1.1, running inthe Java Virtual Machine (JVM) bundled with the Java Development Kit (JDK) 1.1.5, on both Windows and Unix. A few examples require alternate configurations, and this has been noted in the text. The Java Web Server is free forJava Servlet Programming, eMatter EditionCopyright 2000 O’Reilly & Associates, Inc. All rights reserved.
xiiPREFACEeducation use and has a 30-day trial period for all other use. You can download acopy from http://java.sun.com/products. The Java Development Kit is freely downloadable from http://java.sun.com/products/jdk or, for educational use, from http://www.sun.com/products-n-solutions/edu/java/. The Java Servlet Development Kit(JSDK) is available separately from the JDK; you can find it at http:// java.sun.com/products/servlet/.This book also contains a set of utility classes—they are used by the servlet examples, and you may find them helpful for your own general-purpose servletdevelopment. These classes are contained in the com.oreilly.servlet package.Among other things, there are classes to help servlets parse parameters, handle fileuploads, generate multipart responses (server push), negotiate locales for internationalization, return files, manage socket connections, and act as RMI servers.There’s even a class to help applets communicate with servlets. The source codefor the com.oreilly.servlet package is contained within the text; the latestversion is also available online (with javadoc documentation) from http://www.oreilly.com/catalog/jservlet/ and http://www.servlets.com.OrganizationThis book consists of 13 chapters and 5 appendices, as follows:Chapter 1, IntroductionExplains the role and advantage of Java servlets in web applicationdevelopment.Chapter 2, HTTP Servlet BasicsProvides a quick introduction to the things an HTTP servlet can do: pagegeneration, server-side includes, servlet chaining, and JavaServer Pages.Chapter 3, The Servlet Life CycleExplains the details of how and when a servlet is loaded, how and when it isexecuted, how threads are managed, and how to handle the synchronizationissues in a multithreaded system. Persistent state capabilities are also covered.Chapter 4, Retrieving InformationIntroduces the most common methods a servlet uses to receive information—about the client, the server, the client’s request, and itself.Chapter 5, Sending HTML InformationDescribes how a servlet can generate HTML, return errors and other statuscodes, redirect requests, write data to the server log, and send custom HTTPheader information.Chapter 6, Sending Multimedia ContentLooks at some of the interesting things a servlet can return: dynamicallygenerated images, compressed content, and multipart responses.Java Servlet Programming, eMatter EditionCopyright 2000 O’Reilly & Associates, Inc. All rights reserved.
PREFACExiiiChapter 7, Session TrackingShows how to build a sense of state on top of the stateless HTTP protocol. Thefirst half of the chapter demonstrates the traditional session-tracking techniques used by CGI developers; the second half shows how to use the built-insupport for session tracking in the Servlet API.Chapter 8, SecurityExplains the security issues involved with distributed computing and demonstrates how to maintain security with servlets.Chapter 9, Database ConnectivityShows how servlets can be used for high-performance web-databaseconnectivity.Chapter 10, Applet-Servlet CommunicationDescribes how servlets can be of use to applet developers who need to communicate with the server.Chapter 11, Interservlet CommunicationDiscusses why servlets need to communicate with each other and how it can beaccomplished.Chapter 12, InternationalizationShows how a servlet can generate multilingual content.Chapter 13, Odds and EndsPresents a junk drawer full of useful servlet examples and tips that don’t reallybelong anywhere else.Appendix A, Servlet API Quick ReferenceContains a full description of the classes, methods, and variables in thejavax.servlet package.Appendix B, HTTP Servlet API Quick ReferenceContains a full description of the classes, methods, and variables in thejavax.servlet.http package.Appendix C, HTTP Status CodesLists the status codes specified by HTTP, along with the mnemonic constantsused by servlets.Appendix D, Character EntitiesLists the character entities defined in HTML, along with their equivalentUnicode escape values.Appendix E, CharsetsLists the suggested charsets servlets may use to generate content in severaldifferent languages.Java Servlet Programming, eMatter EditionCopyright 2000 O’Reilly & Associates, Inc. All rights reserved.
xivPREFACEPlease feel free to read the chapters of this book in whatever order you like.Reading straight through from front to back ensures that you won’t encounter anysurprises, as efforts have been taken to avoid forward references. If you want toskip around, however, you can do so easily enough, especially after Chapter 5—therest of the chapters all tend to stand alone. One last suggestion: read the “Debugging” section of Chapter 13 if at any time you find a piece of code that doesn’twork as expected.Conventions Used in This BookItalic is used for: Pathnames, filenames, and program names New terms where they are defined Internet addresses, such as domain names and URLsBoldface is used for: Particular keys on a computer keyboard Names of user interface buttons and menusConstant Width is used for: Anything that appears literally in a Java program, including keywords, datatypes, constants, method names, variables, class names, and interface names Command lines and options that should be typed verbatim on the screen All Java code listings HTML documents, tags, and attributesConstant Width Italic is used for: General placeholders that indicate that an item is replaced by some actualvalue in your own programRequest for CommentsPlease help us to improve future editions of this book by reporting any errors,inaccuracies, bugs, misleading or confusing statements, and plain old typos thatyou find anywhere in this book. Email your bug reports and comments to us at:bookquestions@oreilly.com. (Before sending a bug report, however, you may want tocheck for an errata list at http://www.oreilly.com/catalog/jservlet/ to see if the bug hasalready been submitted.)Please also let us know what we can do to make this book more useful to you. Wetake your comments seriously and will try to incorporate reasonable suggestionsinto future editions.Java Servlet Programming, eMatter EditionCopyright 2000 O’Reilly & Associates, Inc. All rights reserved.
PREFACExvAcknowledgmentsThe authors would like to say a big thank you to the book’s technical reviewers,whose constructive criticism has done much to improve this work: Mike Slinn,Mike Hogarth, James Duncan Davidson, Dan Pritchett, Dave McMurdie, and RobClark. We’re still in shock that it took one reviewer just three days to read whattook us a full year to write!Jason HunterIn a sense, this book began March 20, 1997, at the Computer Literacy bookstore inSan Jose, California. There—after a hilarious talk by Larry Wall and RandallSchwartz, where Larry explained how he manages to automate his house usingPerl—I met the esteemed Tim O’Reilly for the first time. I introduced myself andbrazenly told him that some day (far in the future, I thought) I had plans to writean O’Reilly book. I felt like I was telling Steven Spielberg I planned to star in oneof his movies. To my complete and utter surprise, Tim replied, “On what topic?”So began the roller coaster ride that resulted in this book.There have been several high points I fondly remember: meeting my editor (cool,she’s young, too!), signing the official contract (did you know that all of O’Reilly’sofficial paper has animals on it?), writing the first sentence (over and over),printing the first chapter (and having it look just like an O’Reilly book), and thenwatching as the printouts piled higher and higher, until eventually there wasnothing more to write (well, except the acknowledgments).There have been a fair number of trying times as well. At one point, when thebook was about half finished, I realized the Servlet API was changing faster than Icould keep up. I believe in the saying, “If at first you don’t succeed, ask for help,”so after a quick talent search I asked William Crawford, who was already workingon Java Enterprise in a Nutshell, if he could help speed the book to completion. Hegraciously agreed and in the end wrote two chapters, as well as portions of theappendices.There are many others who have helped in the writing of this book, both directlyand indirectly. I’d like to say thank you to Paula Ferguson, the book’s editor, andMike Loukides, the Java series editor, for their efforts to ensure (and improve) thequality of this book. And to Tim O’Reilly for giving me the chance to fulfill adream.Thanks also to my managers at Silicon Graphics, Kathy Tansill and Walt Johnson,for providing me with more encouragement and flexibility than I had any right toexpect.I can’t say thank you enough to the engineers at Sun who were tremendouslyhelpful in answering questions, keeping me updated on changes in the ServletAPI, and promptly fixing almost every bug I reported: James Duncan DavidsonJava Servlet Programming, eMatter EditionCopyright 2000 O’Reilly & Associates, Inc. All rights reserved.
xviPREFACE(who looks the spitting image of James Gosling), Jim Driscoll, Rob Clark, and DaveBrownell.Thanks also to the members of the jserv-interest mailing list, whose questions andanswers have shaped the content of this book; Will Ramey, an old friend whodidn’t let friendship blind his critical eye; Mike Engber, the man to whom I turnedwhen I had run out of elegant workarounds and was ready to accept the crazythings he comes up with; Dave Vandegrift, the first person to read many of thechapters; Bill Day, author of Java Media Players, who helped intangibly by goingthrough the book writing process in parallel with me; Michael O’Connell and JillSteinberg, editors at JavaWorld, where I did my first professional writing; DougYoung, who shared with me the tricks he learned writing seven technical books ofhis own; and Shoji Kuwabara, Mieko Aono, Song Yung, Matthew Kim, and Alexandr Pashintsev for their help translating “Hello World” for Chapter 12.Finally, thanks to Mom and Dad, for their love and support and for the time theyspent long ago teaching me the basics of writing. And a special thanks to my girlfriend, Kristi Taylor, who made the small time away from work a pleasure.And Grandpa, I wish you could have seen this.Jason HunterJuly 1998William CrawfordFirst and foremost, thanks to Shelley Norton, Dr. Isaac Kohane, Dr. James Fackler,and Dr. Richard Kitz (plus a supporting cast whose contributions were invaluable), whose assistance and early support h
Java Virtual Machine Java AWT Reference Java Language Reference Java Fundamental Classes Reference Database Programming with JDBC and Java Java Distributed Computing Developing Java Beans Java Security Java Cryptography Java Swing Java Servlet Programming Also from O'Reilly Java in a Nutshell Java in a Nutshell, Deluxe Edition