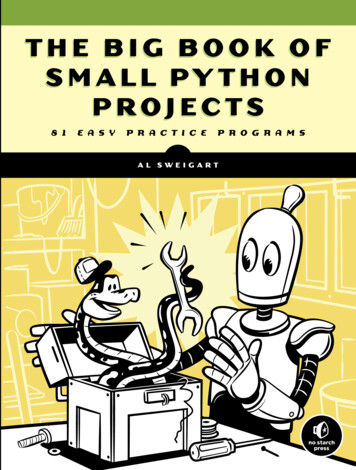
Transcription
THE BIG BOOK OFSMALL PY THONPROJECTS8 1E A S YP R A C T I C EP R O G R A M SAL SWEIGART
THE BIG BOOK OF SMALL PYTHON PROJECTS
THE BIG BOOKOF SMALLPYTHONPROJECTS81 Easy Practice ProgramsAl S w e i g a r tSan Francisco
THE BIG BOOK OF SMALL PYTHON PROJECTS. Copyright 2021 by Al Sweigart.All rights reserved. No part of this work may be reproduced or transmitted in any form or by any means,electronic or mechanical, including photocopying, recording, or by any information storage or retrievalsystem, without the prior written permission of the copyright owner and the publisher.ISBN-13: 978-1-7185-0124-9 (print)ISBN-13: 978-1-7185-0125-6 (ebook)Publisher: William PollockProduction Manager: Rachel MonaghanProduction Editor: Paula WilliamsonDevelopmental Editor: Frances SauxTechnical Reviewer: Sarah KuchinskyCover and Interior Design: Octopod StudiosCover Illustrator: Josh EllingsonCopyeditor: Bart ReedCompositor: Maureen Forys, Happenstance Type-O-RamaProofreader: Scout FestaFor information on book distributors or translations, please contact No Starch Press, Inc. directly:No Starch Press, Inc.245 8th Street, San Francisco, CA 94103phone: 1-415-863-9900; info@nostarch.comwww.nostarch.comLibrary of Congress Control Number: 2021936413No Starch Press and the No Starch Press logo are registered trademarks of No Starch Press, Inc. Otherproduct and company names mentioned herein may be the trademarks of their respective owners. Ratherthan use a trademark symbol with every occurrence of a trademarked name, we are using the names onlyin an editorial fashion and to the benefit of the trademark owner, with no intention of infringement ofthe trademark.The information in this book is distributed on an “As Is” basis, without warranty. While every precautionhas been taken in the preparation of this work, neither the author nor No Starch Press, Inc. shall have anyliability to any person or entity with respect to any loss or damage caused or alleged to be caused directlyor indirectly by the information contained in it.
About the AuthorAl Sweigart is a software developer, author, and Fellow of the PythonSoftware Foundation. He was previously the education director at Oakland,California’s video game museum, The Museum of Art and Digital Enter tainment. He has written several programming books, including Automate theBoring Stuff with Python and Invent Your Own Computer Games with Python. Hisbooks are freely available under a Creative Commons license at his websitehttps://inventwithpython.com. His cat Zophie loves eating nori seaweed snacks.About the Technical ReviewerSarah Kuchinsky, MS, is a corporate trainer and consultant. She usesPython for a variety of applications, including health systems modeling,game development, and task automation. Sarah is a co-founder of theNorth Bay Python conference, tutorials chair for PyCon US, and lead organizer for PyLadies Silicon Valley. She holds degrees in ManagementScience & Engineering and Mathematics.
CO N T E N T S I N D E TA I LIntroductionProject 1, Bagels: Deduce a secret three-digit number based on clues.xv1Practice using constants.Project 2, Birthday Paradox: Determine the probability that twopeople share the same birthday in groups of different sizes.Use Python’s datetime module.Project 3, Bitmap Message: Display a message on the screenconfigured by a 2D bitmap image.611Work with multiline strings.Project 4, Blackjack: A classic card game played against an AI dealer.15Learn about Unicode characters and code points.Project 5, Bouncing DVD Logo: Simulates the colorful bouncing DVDlogo of decades past.23Work with coordinates and colorful text.Project 6, Caesar Cipher: A simple encryption scheme used thousandsof years ago.29Convert between letters and numbers to perform math on text.Project 7, Caesar Hacker: A program to decrypt Caesar ciphermessages without the encryption key.33Implement a brute-force cryptanalysis algorithm.Project 8, Calendar Maker: Create calendar pages for a given yearand month.36Use Python’s datetime module and the timedelta data type.Project 9, Carrot in a Box: A silly bluffing game between two players.41Create ASCII art.Project 10, Cho-Han: A gambling dice game from feudal Japan.Practice using random numbers and dictionary data structures.47
Project 11, Clickbait Headline Generator: A humorous headlinegenerator for your content farm.51Practice string manipulation and text generation.Project 12, Collatz Sequence: Explore the simplest impossibleconjecture in mathematics.56Learn about the modulus operator.Project 13, Conway’s Game of Life: The classic cellular automatawhose simple rules produce complex emergent behavior.59Use dictionary data structures and screen coordinates.Project 14, Countdown: A countdown timer with a seven-segment display. 63Practice importing modules you create.Project 15, Deep Cave: A tunnel animation that descends endlesslyinto the earth.66Use string replication and simple math.Project 16, Diamonds: An algorithm for drawing diamonds ofvarious sizes.69Practice your pattern recognition skills to create drawing algorithms.Project 17, Dice Math: A visual dice-rolling math game.73Use dictionary data structures for screen coordinates.Project 18, Dice Roller: A tool for reading Dungeons & Dragons dicenotation to generate random numbers.79Parse text to identify key strings.Project 19, Digital Clock: A clock with a calculator-like display.83Generate numbers that match information from the datetime module.Project 20, Digital Stream: A scrolling screensaver that resemblesThe Matrix.86Experiment with different animation speeds.Project 21, DNA Visualization: An endless ASCII-art double helixthat demonstrates the structure of DNA.90Work with string templates and randomly generated text.Project 22, Ducklings: Mix and match strings to create a variety ofASCII-art ducks.Use object-oriented programming to create a data model for duck drawings.viiiContents in Detail94
Project 23, Etching Drawer: Move the cursor to create line drawings.100Work with screen coordinates and relative directional movements.Project 24, Factor Finder: Find all the multiplicative factors of a number. 106Use the modulus operator and Python’s math module.Project 25, Fast Draw: Test your reflexes to see if you’re the fastestkeyboard in the West.110Learn about the keyboard buffer.Project 26, Fibonacci: Generate numbers in the famous Fibonaccisequence.113Implement a rudimentary mathematics algorithm.Project 27, Fish Tank: A colorful, animated ASCII-art fish tank.117Use screen coordinates, text colors, and data structures.Project 28, Flooder: Attempt to fill the entire puzzle board with one color.125Implement the flood fill algorithm.Project 29, Forest Fire Sim: Simulate the spread of wildfires througha forest.132Create a simulation with adjustable parameters.Project 30, Four in a Row: A board game where two players tryto connect four tiles in a row.137Create a data structure that mimics gravity.Project 31, Guess the Number: The classic number guessing game.143Program basic concepts for beginners.Project 32, Gullible: A humorous program to keep gullible peoplebusy for hours.147Use input validation and loops.Project 33, Hacking Minigame: Deduce a password based on clues.150Add cosmetic features to make a basic game more interesting.Project 34, Hangman and Guillotine: The classic word guessing game.156Use string manipulation and ASCII art.Project 35, Hex Grid: Programmatically generate tiled ASCII art.162Use loops to make repeating text patterns.Contents in Detailix
Project 36, Hourglass: A simple physics engine for falling sand.165Simulate gravity and use collision detection.Project 37, Hungry Robots: Avoid killer robots in a maze.171Create a simple AI for robot movements.Project 38, J’Accuse!: A detective game to determine liars and truth-tellers. 178Use data structures to generate relationships between suspects, places, and item clues.Project 39, Langton’s Ant: A cellular automata whose ants moveaccording to simple rules.186Explore how simple rules create complex graphical patterns.Project 40, Leetspeak: Translate English messages into l33t5p34] .192Use text parsing and string manipulation.Project 41, Lucky Stars: A push-your-luck dice game.195Practice ASCII art and probability.Project 42, Magic Fortune Ball: A program to answer your yes/noquestions about the future.202Add cosmetic features to make basic text appear more interesting.Project 43, Mancala: The ancient two-player board gamefrom Mesopotamia.206Use ASCII art and string templates to draw a board game.Project 44, Maze Runner 2D: Try to escape a maze.213Read maze data from text files.Project 45, Maze Runner 3D: Try to escape a maze . . . in 3D!219Modify multiline strings to display a 3D view.Project 46, Million Dice Roll Statistics Simulator: Explore theprobability results of rolling a set of dice one million times.228Learn how computers crunch large quantities of numbers.Project 47, Mondrian Art Generator: Create geometric drawingsin the style of Piet Mondrian.231Implement an art-generating algorithm.Project 48, Monty Hall Problem: A simulation of the Monty Hallgame show problem.Examine probability with ASCII-art goats.xContents in Detail238
Project 49, Multiplication Table: Display the multiplicationtable up to 12 12.245Practice spacing text.Project 50, Ninety-Nine Bottles: Display the lyrics to a repetitive song. 248Use loops and string templates to produce text.Project 51, niNety-nniinE BoOttels: Display the lyrics to a repetitivesong that get more distorted with each verse.251Manipulate strings to introduce distortions.Project 52, Numeral Systems Counters: Examine binary andhexadecimal numbers.255Use Python’s number conversion functions.Project 53, Periodic Table of the Elements: An interactive databaseof chemical elements.259Parse CSV files to load data into a program.Project 54, Pig Latin: Translates English messages into Igpay Atinlay.263Use text parsing and string manipulation.Project 55, Powerball Lottery: Simulate losing at the lotterythousands of times.267Explore probability using random numbers.Project 56, Prime Numbers: Calculate prime numbers.272Learn math concepts and use Python’s math module.Project 57, Progress Bar: A sample progress bar animation to usein other programs.275Use the backspace-printing technique to create animations.Project 58, Rainbow: A simple rainbow animation.279Create animation for beginners.Project 59, Rock Paper Scissors: The classic hand gamefor two players.282Implement basic game rules as a program.Project 60, Rock Paper Scissors (Always-Win Version):A version of the game where the player cannot lose.286Create the illusion of randomness in a program.Contents in Detailxi
Project 61, ROT13 Cipher: The simplest cipher for encryptingand decrypting text.290Convert between letters and numbers to perform math on text.Project 62, Rotating Cube: A rotating cube animation.293Learn 3D rotation and line drawing algorithms.Project 63, Royal Game of Ur: A 5,000-year-old gamefrom Mesopotamia.300Use ASCII art and string templates to draw a board game.Project 64, Seven-Segment Display Module: A display likethose used in calculators and microwave ovens.308Create modules for use in other programs.Project 65, Shining Carpet: Programmatically generate thecarpet from The Shining.312Use loops to make repeating text patterns.Project 66, Simple Substitution Cipher: An encryption schememore advanced than the Caesar cipher.315Perform intermediate math on text.Project 67, Sine Message: Display a scrolling wave message.320Use a trigonometry function for animation.Project 68, Sliding Tile Puzzle: The classic four-by-four tile puzzle.323Employ a data structure to reflect the state of a game board.Project 69, Snail Race: Fast-paced snail racing action!329Calculate spacing for ASCII art snails.Project 70, Soroban Japanese Abacus: A computer simulationof a pre-computer calculating tool.333Use string templates to create an ASCII-art counting tool.Project 71, Sound Mimic: Memorize an increasingly longpattern of sounds.339Play sound files from a Python program.Project 72, sPoNgEcAsE: Translates English messages intosPOnGEcAsE.Change the casing of letters in strings.xiiContents in Detail343
Project 73, Sudoku Puzzle: The classic nine-by-nine newspaperdeduction puzzle.346Model a puzzle with a data structure.Project 74, Text-to-Speech Talker: Make your computertalk to you!353Use your operating system’s text-to-speech engine.Project 75, Three-Card Monte: The tricky fast-swapping cardgame that scammers play on tourists.356Manipulate a data structure based on random movements.Project 76, Tic-Tac-Toe: The classic two-player board gameof Xs and Os.361Create a data structure and helper functions.Project 77, Tower of Hanoi: The classic diskstacking puzzle.365Use stack data structures to simulate a puzzle state.Project 78, Trick Questions: A quiz of simple questions withmisleading answers.370Parse the user’s text to recognize keywords.Project 79, Twenty Forty-Eight: A casual tile matching game.377Simulate gravity to make tiles “fall” in arbitrary directions.Project 80, Vigenère Cipher: An encryption scheme so advanced itremained unbreakable for hundreds of years until computers were invented.385Perform more advanced math on text.Project 81, Water Bucket Puzzle: Obtain exactly four liters ofwater by filling and emptying three buckets.390Use string templates to generate ASCII art.Appendix A: Tag Index395Appendix B: Character Map398Contents in Detailxiii
INTRODUCTIONProgramming was so easy when it was justfollowing print('Hello, world!') tutorials.Perhaps you’ve followed a well-structuredbook or online course for beginners, workedthrough the exercises, and nodded along with its technical jargon that you (mostly) understood. However,when it came time to leave the nest to write your ownprograms, maybe you found it hard to fly on your own. You found yourselfstaring at a blank editor window and unsure of how to get started writingPython programs of your own.The problem is that following a tutorial is great for learning concepts,but that isn’t necessarily the same thing as learning to create original programs from scratch. The common advice given at this stage is to examinethe source code of open source software or to work on your own projects,but open source projects aren’t always well documented or especially accessible to newcomers. And while it’s motivating to work on your own project,you’re left completely without guidance or structure.
This book provides you with practice examples of how programmingconcepts are applied, with a collection of over 80 games, simulations, and digital art programs. These aren’t code snippets; they’re full, runnable Pythonprograms. You can copy their code to become familiar with how they work,experiment with your own changes, and then attempt to re-create them onyour own as practice. After a while, you’ll start to get ideas for your own programs and, more importantly, know how to go about creating them.How to Design Small ProgramsProgramming has proven to be a powerful skill, creating billion-dollar techcompanies and amazing technological advances. It’s easy to want to aimhigh with your own software creations, but biting off more than you canchew can leave you with half-finished programs and frustration. However,you don’t need to be a computer genius to code fun and creative programs.The Python programs in this book follow several design principles toaid new programmers in understanding their source code:Small Most of these programs are limited to 256 lines of code and areoften significantly shorter. This size limit makes them easier to comprehend. The choice of 256 is arbitrary, but 256 is also 28, and powers of 2are lucky programmer numbers.Text based Text is simpler than graphics. Since the source code andprogram output are both text, it’s easy to trace the cause and effectbetween print('Thanks for playing!') in the code and Thanks for playing!appearing on the screen.No installation needed Each program is self-contained in a singlePython source file with the .py file extension, like tictactoe.py. You don’tneed to run an installer program, and you can easily post these programs online to share with others.Numerous There are 81 programs in this book. Between board games,card games, digital artwork, simulations, mathematical puzzles, mazes,and humor programs, you’re bound to find many things you’ll love.Simple The programs have been written to be easy to understandby beginners. Whenever I had to choose between writing code usingsophisticated, high-performance algorithms or writing plain, straightforward code, I’ve chosen the latter every time.The text-based programs may seem old school, but this style of programming cuts out the distractions and potholes that downloading graphics, installing additional libraries, and managing project folders bring.Instead, you can just focus on the code.Who Is This Book For?This book is written for two groups of people. The people in the first groupare those who have already learned the basics of Python and programmingxviIntroduction
but are still unsure of how to write programs on their own. They may feelthat programming hasn’t “clicked” for them. They may be able to solve thepractice exercises from their tutorials but still struggle to picture what acomplete program “looks like.” By first copying and then later re-creatingthe games in this book, they’ll be exposed to how the programming concepts they’ve learned are assembled into a variety of real programs.The people in the second group are those who are new to programming but are excited and a bit adventurous. They want to dive right in andget started making games, simulations, and number-crunching programsright away. They’re fine with copying the code and learning along the way.Or perhaps they already know how to program in another language but arenew to Python. While it’s no substitute for a complete introductory Pythoncourse, this book contains a brief introduction to Python basics and how touse the debugger to examine the inner workings of a program as it runs.Experienced programmers might have fun with the programs in thisbook as well, but keep in mind that this book was written for beginners.About This BookWhile the bulk of this book is dedicated to the featured programs, thereare also extra resources with general programming and Python information. Here’s what’s contained in this book:Projects The 81 projects are too numerous to list here, but each oneis a self-contained mini-chapter that includes the project’s name, adescription, a sample run of the program’s output, and the source codeof the program. There are also suggestions for experimental edits youcan make to the code to customize the program.Appendix A: Tag Index Lists all of the projects categorized by theirproject tags.Appendix B: Character Map A list of character codes for symbolssuch as hearts, lines, arrows, and blocks that your programs can print.How to Learn from the Programs in This BookThis book doesn’t teach Python or programming concepts like a traditionaltutorial. It has a learn-by-doing approach, where you’re encouraged to manually copy the programs, play with them, and inspect their inner workingsby running them under a debugger.The point of this book isn’t to give a detailed explanation of programming language syntax, but to show solid examples of programs thatperform an actual activity, whether it’s a card game, an animation, or exploration of a mathematical puzzle. As such, I recommend the following steps:1. Download the program and run it to see what the program does foryourself.Introductionxvii
2. Starting from a blank file, copy the code of the game from this book bymanually typing it yourself. (Don’t use copy and paste!)3. Run the program again, and go back and fix any typos or bugs you mayhave introduced.4. Run the program under a debugger, so you can carefully execute eachline of code one at a time to understand what it does.5. Find the comments marked with (!) to find code that you can modifyand then see how this affects the program the next time you run it.6. Finally, try to re-create the program yourself from scratch. It doesn’thave to be an exact copy; you can put your own spin on the program.When copying the code from this book, you don’t necessarily have totype the comments (the text at the end of a line following the # symbol),as these are notes for human programmers and are ignored by Python.However, try to write your Python code on the same line numbers as theprograms in this book to make comparison between the two easier. If youhave trouble finding typos in your program, you can compare your code tothe code in this book with the online diff tool at ach program has been given a set of tags to describe it, such as boardgame, simulation, artistic, and two-player. An explanation of each of thesetags and a cross-index of tags and projects can be found in Appendix A.The projects are listed in alphabetical order, however.Downloading and Installing PythonPython is the name of both the programming language and the interpreter software that runs your Python code. The Python software is completely free to download and use. You can check if you already have Pythoninstalled from a command line window. On Windows, open the CommandPrompt program and then enter py --version. If you see output like the following, then Python is installed:C:\Users\Al py --versionPython 3.9.1On macOS and Linux, open the Terminal program and then enterpython3 --version. If you see output like the following, then Python isinstalled: python3 --versionPython 3.9.1This book uses Python version 3. Several backward-incompatiblechanges were made between Python 2 and 3, and the programs in this bookrequire at least Python version 3.1.1 (released in 2009) to run. If you seean error message telling you that Python cannot be found or the versionreports Python 2, you can download the latest Python installer for yourxviiiIntroduction
operating system from https://python.org/. If you’re having trouble installingPython, you can find more instructions at https://installpython3.com/.Downloading and Installing the Mu EditorWhile the Python software runs your program, you’ll type the Python codeinto a text editor or integrated development environment (IDE) application. I recommend using Mu Editor for your IDE if you are a beginnerbecause it’s simple and doesn’t distract you with an overwhelming numberof advanced options.Open https://codewith.mu/ in your browser. On Windows and macOS,download the installer for your operating system and then run it by doubleclicking the installer file. If you are on macOS, running the installer opens awindow where you must drag the Mu icon to the Applications folder icon tocontinue the installation. If you are on Ubuntu, you’ll need to install Mu asa Python package. In that case, open a new Terminal window and run pip3install mu-editor to install and mu-editor to run it. Click the Instructions button in the Python Package section of the download page for full instructiondetails.Running the Mu EditorOnce it’s installed, let’s start Mu: On Windows 7 or later, click the Start icon in the lower-left corner ofyour screen, enter mu in the search box, and select Mu when it appears.On macOS, open the Finder window, click Applications, and then clickmu-editor.On Ubuntu, press CTRL-ALT-T to open a Terminal window and thenenter python3 –m mu.The first time Mu runs, a Select Mode window appears with the following options: Adafruit CircuitPython, BBC micro:bit, Pygame Zero, andPython 3. Select Python 3. You can always change the mode later by clickingthe Mode button at the top of the editor window.You’ll be able to enter the code into Mu’s main window and then save,open, and run your files from the buttons at the top.Running IDLE and Other EditorsYou can use any number of editors for writing Python code. The IntegratedDevelopment and Learning Environment (IDLE) software installs alongwith Python, and it can serve as a second editor if for some reason you can’tget Mu installed or working. Let’s start IDLE now: On Windows 7 or later, click the Start icon in the lower-left corner ofyour screen, enter idle in the search box, and select IDLE (Python GUI).Introductionxix
On macOS, open the Finder window and click Applications Python 3.9 IDLE.On Ubuntu, select Applications Accessories Terminal and thenenter idle3. (You may also be able to click Applications at the top of thescreen, select Programming, and then click IDLE 3.)On the Raspberry Pi, click the Raspberry Pi menu button in the top-leftcorner; then click Programming and Python 3 (IDLE). You can alsoselect Thonny Python IDE from under the Programming menu.There are several other free editors you can use to enter and runPython code, such as: Thonny, a Python IDE for beginners, at https://thonny.org/.PyCharm Community Edition, a Python IDE used by professional developers, at https://www.jetbrains.com/pycharm/.Installing Python ModulesMost of the programs in this book only require the Python StandardLibrary, which is installed automatically with Python. However, some programs require third-party modules such as pyperclip, bext, playsound, andpyttsx3. All of these can be installed at once by installing the bigbookpythonmodule.For the Mu Editor, you must install the 1.1.0-alpha version (or later).As of 2020, you can find this version at the top of the download page athttps://codewith.mu/en/download under the “Try the Alpha of the Next Ver sion of Mu” section. After installation, click the gear icon in the lower-leftcorner of the window to bring up the Mu Administration window. Selectthe Third Party Packages tab, enter bigbookpython into the text field, andclick Ok. This installs all of the third-party modules used by the programsin this book.For the Visual Studio Code or IDLE editor, open the editor and run thefollowing Python code from the interactive shell: import os, sys os.system(sys.executable ' -m pip install --user bigbookpython')0The number 0 appears after the second instruction if everythingworked correctly. Otherwise, if you see an error message or another number, try running the following instructions, which don’t have the --useroption: import os, sys os.system(sys.executable ' -m pip install bigbookpython')0xxIntroduction
No matter which editor you use, you can try running import pyperclipor import bext to check if the installation worked. If these import instruction don’t produce an error message, these modules installed correctly andyou’ll be able to run the projects in this book that use these modules.Copying the Code from This BookProgramming is a skill that you improve by programming. Don’t just readthe code in this book or copy and paste it to your computer. Take the time toenter the code into the editor for yourself. Open a new file in your code editor and enter the code. Pay attention to the line numbers in this book andyour editor to make sure you aren’t accidentally skipping any lines. If youencounter errors, use the online diff tool at https://inventwithpython.com/bigbookpython/diff/ to show you any differences between your code and thecode in this book. To get a better understanding of these programs, try running them under the debugger.After entering the source code and running it a few times, try makingexperimental changes to the code. The comments marked with (!) havesuggestions for small changes you can make, and each project lists suggestions for larger modifications.Next, try re-creating the program from scratch without looking at thesource code in this book. It doesn’t have to be exactly the same as this program; you can invent your own version!Once you’ve worked through the programs in this book, you mightwant to start creating your own. Most modern video games and softwareapplications are complicated, requiring teams of programmers, artists,and designers to create. However, many board, card, and paper-and-pencilgames are often simple enough to re-create as a program. Many of thesefall under the category of “abstract strategy games.” You can find a list ofthem at https://en.wikipedia.org/wiki/List of abstract strategy games.Running Programs from the TerminalThe programming projects in this book that use the bext module have colorful text for their output. However, these colors won’t appear when yourun them from Mu, IDLE, or other editors. These programs should berun from a terminal, also called command line, window. On Windows, runthe Command Prompt program from the Start menu. On macOS, runTerminal from Spotlight. On Ubuntu Linux, run Terminal from UbuntuDash or press CTRL-ALT-T.When the terminal window appears, you should change the currentdirectory to the folder with your .py files with the cd (change directory)command. (Directory is another term for folder.) For example, if I were onWindows and saved my Python programs to the C:\Users\Al folder, I wouldenter the following line.Introductionxxi
C:\ cd C:\Users\AlC:\Users\Al Then, to run Python programs, enter python yourProgram.py on Windowsor python3 yourProgram.py on macOS and Linux, replacing yourProgram.py withthe name of your Python program:C:\Users\Al python guess.pyGuess the Number, by Al Sweigart al@inventwithpython.comI am thinking of a number between 1 and 100.You have 10 guesses left. Take a guess.--snip--You can terminate programs run from the terminal by pressing CTRLC rather than close the terminal window itself.Running Programs from a Phone or TabletIdeally you’ll have a laptop or desktop computer with a full keyboard towrite code, as tapping on a phone or even tablet keyboard can be tedious.While there are no established Python interpreters for Android or iOS,there are websites that host online Python interactive shells you can usefrom a web browser. T
Learn math concepts and use Python's math module. Project 57, Progress Bar: A sample progress bar animation to use in other programs. 275 Use the backspace-printing technique to create animations. Project 58, Rainbow: A simple rainbow animation. 279 Create animation for beginners. Project 59, Rock Paper Scissors: The classic hand game for two .