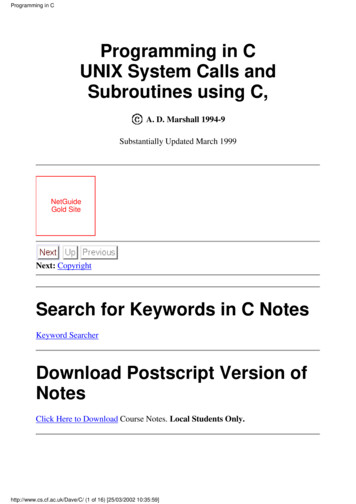
Transcription
Programming in CProgramming in CUNIX System Calls andSubroutines using C,A. D. Marshall 1994-9Substantially Updated March 1999NetGuideGold SiteNext: CopyrightSearch for Keywords in C NotesKeyword SearcherDownload Postscript Version ofNotesClick Here to Download Course Notes. Local Students Only.http://www.cs.cf.ac.uk/Dave/C/ (1 of 16) [25/03/2002 10:35:59]
Programming in CAlgorithm AnimationsDirect link to Java Algorithm Animations (C related)C COURSEWARELecture notes integrated exercises, solutions and marking Contents The Common Desktop Environment The front panel The file manager The application manager The session manager Other CDE desktop tools Application development tools Application integration Windows and the Window Manager The Root Menu ExercisesC/C Program Compilation Creating, Compiling and Running Your Program Creating the program Compilation Running the programThe C Compilation Model The Preprocessor C Compiler Assembler Link Editor Some Useful Compiler Optionshttp://www.cs.cf.ac.uk/Dave/C/ (2 of 16) [25/03/2002 10:35:59]
Programming in C Using Libraries UNIX Library Functions Finding Information about Library Functions Lint -- A C program verifier ExercisesC Basics History of C Characteristics of C C Program Structure Variables Defining Global Variables Printing Out and Inputting Variables Constants Arithmetic Operations Comparison Operators Logical Operators Order of Precedence ExercisesConditionals The if statement The ? operator The switch statement ExercisesLooping and Iteration The for statement The while statement The do-while statement break and continue ExercisesArrays and Strings Single and Multi-dimensional Arrayshttp://www.cs.cf.ac.uk/Dave/C/ (3 of 16) [25/03/2002 10:35:59]
Programming in C Strings ExercisesFunctions void functions Functions and Arrays Function Prototyping ExercisesFurther Data Types Structures Unions Coercion or Type-Casting Enumerated Types Static Variables ExercisesPointers What is a Pointer? Pointer and Functions Pointers and Arrays Arrays of Pointers Multidimensional arrays and pointers Static Initialisation of Pointer Arrays Pointers and Structures Common Pointer Pitfalls Defining New Data Types Not assigning a pointer to memory address before using it Illegal indirectionExerciseDynamic Memory Allocation and Dynamic Structures Malloc, Sizeof, and Free Calloc and Realloc Linked Listshttp://www.cs.cf.ac.uk/Dave/C/ (4 of 16) [25/03/2002 10:35:59]
Programming in C Full Program: queue.c ExercisesAdvanced Pointer Topics Pointers to Pointers Command line input Pointers to a Function ExercisesLow Level Operators and Bit Fields Bitwise Operators Bit Fields Bit Fields: Practical Example A note of caution: PortabilityExercisesThe C Preprocessor #define #undef #include #if -- Conditional inclusion Preprocessor Compiler Control Other Preprocessor Commands ExercisesC, UNIX and Standard Libraries Advantages of using UNIX with C Using UNIX System Calls and Library FunctionsInteger Functions, Random Number, String Conversion, Searching andSorting: stdlib.h Arithmetic Functions Random Numbers String Conversion Searching and Sorting ExercisesMathematics: math.h http://www.cs.cf.ac.uk/Dave/C/ (5 of 16) [25/03/2002 10:35:59]
Programming in C Math Functions Math ConstantsInput and Output (I/O):stdio.h Reporting Errors perror() errno exit()Streams Predefined Streams Basic I/O Formatted I/O scanf Files Reading and writing filesStream Status Enquiries Low Level I/O ExercisesString Handling: string.h Basic String Handling Functions Printfsprintf and sscanf RedirectionString Searching Character conversions and testing: ctype.h Memory Operations: memory.h ExercisesFile Access and Directory System Calls Directory handling functions: unistd.h Scanning and Sorting Directories: sys/types.h , sys/dir.h File Manipulation Routines: unistd.h, sys/types.h, sys/stat.h File Accesshttp://www.cs.cf.ac.uk/Dave/C/ (6 of 16) [25/03/2002 10:35:59]
Programming in C File Status File Manipulation:stdio.h, unistd.h Creating Temporary FIles: stdio.h ExercisesTime Functions Basic time functions Example time applications errno Example 1: Time (in seconds) to perform some computation Example 2: Set a random number seedExercisesProcess Control: stdlib.h , unistd.h Running UNIX Commands from C execl() fork() wait() exit() ExerisesInterprocess Communication (IPC), Pipes Piping in a C program: stdio.h popen() -- Formatted Piping pipe() -- Low level Piping ExercisesIPC:Interrupts and Signals: signal.h Sending Signals -- kill(), raise() Signal Handling -- signal() sig talk.c -- complete example program Other signal functionsIPC:Message Queues: sys/msg.h Initialising the Message Queue IPC Functions, Key Arguments, and Creation Flags: sys/ipc.h http://www.cs.cf.ac.uk/Dave/C/ (7 of 16) [25/03/2002 10:35:59]
Programming in C Controlling message queues Sending and Receiving Messages POSIX Messages: mqueue.h Example: Sending messages between two processes message send.c -- creating and sending to a simplemessage queue message rec.c -- receiving the above messageSome further example message queue programs msgget.c: Simple Program to illustrate msget() msgctl.cSample Program to Illustrate msgctl() msgop.c: Sample Program to Illustrate msgsnd() andmsgrcv()ExercisesIPC:Semaphores Initializing a Semaphore Set Controlling Semaphores Semaphore Operations POSIX Semaphores: semaphore.h semaphore.c: Illustration of simple semaphore passing Some further example semaphore programs semget.c: Illustrate the semget() function semctl.c: Illustrate the semctl() function semop() Sample Program to Illustrate semop()ExercisesIPC:Shared Memory Accessing a Shared Memory Segment Controlling a Shared Memory Segment Attaching and Detaching a Shared Memory Segment Example two processes comunicating via shared memory:shm server.c, shm client.c shm server.c shm client.chttp://www.cs.cf.ac.uk/Dave/C/ (8 of 16) [25/03/2002 10:35:59]
Programming in C POSIX Shared Memory Mapped memory Address Spaces and Mapping Coherence Creating and Using Mappings Other Memory Control FunctionsSome further example shared memory programs shmget.c:Sample Program to Illustrate shmget() shmctl.c: Sample Program to Illustrate shmctl() shmop.c: Sample Program to Illustrate shmat() andshmdt()ExercisesIPC:Sockets Socket Creation and Naming Connecting Stream Sockets Stream Data Transfer and Closing Datagram sockets Socket Options Example SocketPrograms:socket server.c,socket client socket server.c socket client.cExercisesThreads: Basic Theory and Libraries Processes and Threads Benefits of Threads vs Processes Multithreading vs. Single threading Some Example applications of threadsThread Levels User-Level Threads (ULT) Kernel-Level Threads (KLT) Combined ULT/KLT Approacheshttp://www.cs.cf.ac.uk/Dave/C/ (9 of 16) [25/03/2002 10:35:59]
Programming in C Threads libraries The POSIX Threads Library:libpthread, pthread.h Creating a (Default) Thread Wait for Thread Termination A Simple Threads Example Detaching a Thread Create a Key for Thread-Specific Data Delete the Thread-Specific Data Key Set the Thread-Specific Data Key Get the Thread-Specific Data Key Global and Private Thread-Specific Data Example Getting the Thread Identifiers Comparing Thread IDs Initializing Threads Yield Thread Execution Set the Thread Priority Get the Thread Priority Send a Signal to a Thread Access the Signal Mask of the Calling Thread Terminate a ThreadSolaris Threads: thread.h Unique Solaris Threads Functions Suspend Thread Execution Continue a Suspended Thread Set Thread Concurrency Level Readers/Writer Locks Readers/Writer Lock ExampleSimilar Solaris Threads Functions Create a Thread Get the Thread Identifier Yield Thread Executionhttp://www.cs.cf.ac.uk/Dave/C/ (10 of 16) [25/03/2002 10:35:59]
Programming in C Signals and Solaris Threads Terminating a Thread Creating a Thread-Specific Data Key Example Use of Thread Specific Data:RethinkingGlobal VariablesCompiling a Multithreaded Application Preparing for Compilation Debugging a Multithreaded ProgramFurther Threads Programming:Thread Attributes (POSIX) Attributes Initializing Thread Attributes Destroying Thread Attributes Thread's Detach State Thread's Set Scope Thread Scheduling Policy Thread Inherited Scheduling Policy Set Scheduling ParametersThread Stack Size Building Your Own Thread StackFurther Threads Programming:Synchronization Mutual Exclusion Locks Initializing a Mutex Attribute Object Destroying a Mutex Attribute Object The Scope of a Mutex Initializing a Mutex Locking a Mutex Lock with a Nonblocking Mutex Destroying a Mutex Mutex Lock Code Examples Mutex Lock Example Using Locking Hierarchies: Avoiding DeadlockNested Locking with a Singly Linked Listhttp://www.cs.cf.ac.uk/Dave/C/ (11 of 16) [25/03/2002 10:35:59]
Programming in C Solaris Mutex LocksCondition Variable Attributes Initializing a Condition Variable Attribute Destoying a Condition Variable Attribute The Scope of a Condition Variable Initializing a Condition Variable Block on a Condition Variable Destroying a Condition Variable State Solaris Condition VariablesThreads and Semaphores POSIX Semaphores Basic Solaris Semaphore FunctionsThread programming examples Using thr create() and thr join() Arrays Deadlock Signal Handler Interprocess Synchronization The Producer / Consumer Problem A Socket Server Using Many Threads Real-time Thread Example POSIX Cancellation Software Race Condition Tgrep: Threadeds version of UNIX grep Multithreaded QuicksortRemote Procedure Calls (RPC) What Is RPC How RPC Works RPC Application Development Defining the Protocolhttp://www.cs.cf.ac.uk/Dave/C/ (12 of 16) [25/03/2002 10:35:59]
Programming in C Defining Client and Server Application Code Compliling and running the applicationOverview of Interface Routines Simplified Level Routine Function Top Level RoutinesIntermediate Level Routines Expert Level Routines Bottom Level RoutinesThe Programmer's Interface to RPC Simplified Interface Passing Arbitrary Data Types Developing High Level RPC Applications Sharing the data The Server Side The Client SideExerciseProtocol Compiling and Lower Level RPC Programming What is rpcgen An rpcgen Tutorial Defining the protocolConverting Local Procedures to Remote Procedures Passing Complex Data Structures Preprocessing Directives cpp Directives Compile-Time Flags Client and Server Templates Example rpcgen compile options/templates Recommended Reading ExercisesWriting Larger Programs Header fileshttp://www.cs.cf.ac.uk/Dave/C/ (13 of 16) [25/03/2002 10:35:59]
Programming in C External variables and functions Scope of externals Advantages of Using Several Files How to Divide a Program between Several Files Organisation of Data in each File The Make Utility Make Programming Creating a makefile Make macros Running MakeProgram Listings hello.c printf.c swap.c args.c arg.c average.c cio.c factorial power.c ptr arr.c Modular Example main.c WriteMyString.c header.h Makefile static.c malloc.c queue.c bitcount.c lowio.c print.chttp://www.cs.cf.ac.uk/Dave/C/ (14 of 16) [25/03/2002 10:35:59]
Programming in C cdir.c list.c list c.c fork eg.c fork.c signal.c sig talk.c Piping plot.c plotter.c externals.h random.c time.c timer.cOnline Marking of C Programs --CEILIDH Ceilidh - On Line C Tutoring System Why Use CEILIDH ? Introduction Using Ceilidh as a Student The course and unit level The exercise level Interpreted language exercises Question/answer exercisesThe command line interface (TEXT CEILIDH ONLY) Advantages of the command line interface General points Conclusions How Ceilidh works, Ceilidh Course Notes, User Guides etc.http://www.cs.cf.ac.uk/Dave/C/ (15 of 16) [25/03/2002 10:35:59]
Programming in C ReferencesAbout this document .Dave Marshall29/3/1999http://www.cs.cf.ac.uk/Dave/C/ (16 of 16) [25/03/2002 10:35:59]
CopyrightNext: Books Up: Programming in C Previous: Programming in CCopyrightAll notes here are copyrighted. All copying etc. is not permitted.David Marshall 1994Dave.Marshall@cm.cf.ac.ukWed Sep 14 10:06:31 BST 1994http://www.cs.cf.ac.uk/Dave/C/chapter2 1.html [25/03/2002 10:36:04]
BooksNext: About This Course Up: Programming in C Previous: CopyrightBooks Brian W Kernighan and Dennis M Ritchie, The C Programming Language2nd Ed, Prentice-Hall, 1988.Kenneth E. Martin, C Through UNIX, WCB Group, 1992.Keith Tizzard, C for Professional Programmers, Ellis Horwood, 1986.Chris Carter, Structured Programming into ANSI C, Pittman, 1991.C. Charlton, P. Leng and Janet Little, A Course on C, McGraw Hill, 1992.G. Bronson and S. Menconi, A First Book on C: Fundamentals of CProgramming (2nd ed.), West Publishing, 1991.Any book on ANSI C will probably do, some UNIX may help.Dave.Marshall@cm.cf.ac.ukWed Sep 14 10:06:31 BST 1994http://www.cs.cf.ac.uk/Dave/C/chapterstar2 2.html [25/03/2002 10:36:05]
About This CourseNext: Course Material and On-line facilities Up: Programming in C Previous:BooksAbout This CourseThis course aims to teach a sound basis of C PROGRAMMING.We will start with basic ideas and hopefully extend these to include some advancedfeatures of C. We will particularly look at how C uses pointers, references low levelmemory and bytes and how it interfaces with the operating system. Course Material and On-line facilities Exercises - Using X Windows, Editing and UNIX BasicsDave.Marshall@cm.cf.ac.ukWed Sep 14 10:06:31 BST 1994http://www.cs.cf.ac.uk/Dave/C/chapterstar2 3.html [25/03/2002 10:36:06]
Course Material and On-line facilitiesNext: Exercises - Using X WindowsEditing and Up: About This Course Previous:About This CourseCourse Material and On-linefacilitiesObviously you have been provided with the course notes that you are reading.In addition several on line facilities will be employed in this course. Ceilidh - an online tutoring and program marking facility (see Appendix for details. All exercises given can be answered in Ceilidh. Some alternativeC course notes are also available. Ceilidh will mark any exercise submittedvery quickly.All program listings are available in the /well/dave/C/EXAMPLESdirectory. Feel free to copy these to help speed up your writing of programs.Mind Ceilidh helps with this also by providing skeleton programs.The course notes are also on-line. Run the mosaic program and selectcomma lecture notes.We will be using the departments Dec Workstations which are unix based.If you have not use unix or a workstation before do not worry the first tutorialsession is to be used for this purpose.Details on how to use the system are in Appendixfollow.Dave.Marshall@cm.cf.ac.ukWed Sep 14 10:06:31 BST 1994http://www.cs.cf.ac.uk/Dave/C/sectionstar2 3 1.html [25/03/2002 10:36:08]. Also try the exercises that
Exercises - Using X Windows, Editing and UNIX BasicsNext: The C Program Up: About This Course Previous: Course Material andOn-line facilitiesExercises - Using X Windows,Editing and UNIX Basics1. Practice opening, closing and moving windows around the screen and to/fromthe background/foreground. Get used to using the mouse and its buttons forsuch tasks.2. Figure out the function of each of the three mouse buttons. Pay particularattention to the different functions the buttons in different windows(applications) and also when the mouse is pointing to the background.3. Find out how to resize windows etc. and practice this.4. Fire up textedit application and practice editing text files. Create any filesyou wish for now. Figure out basic options like cut and paste of text aroundthe file, saving and loading files, searching for strings in the text andreplacing strings.Particularly pay attention in getting used to using the Key Strokes and / ormouse to perform the above tasks.5. Use Unix Commands (see Appendix ) to1. Copy a file (created by text editor or other means) to another file calledspare.2. Rename your original file to b called new.3. Delete the file spare.4. Display your original file on the terminal.5. Print your file out.6. Familiarise yourself with other UNIX functions by creating various files oftext etc. and trying out the various functions listed in handouts.Dave.Marshall@cm.cf.ac.ukWed Sep 14 10:06:31 BST 1994http://www.cs.cf.ac.uk/Dave/C/sectionstar2 3 2.html [25/03/2002 10:36:10]
Ceilidh - On Line C Tutoring SystemNext: Why Use CEILIDH ? Up: Programming in C Previous: ExercisesCeilidh - On Line C TutoringSystem Why Use CEILIDH ? Introduction Using Ceilidh as a Student The course and unit level The exercise level Interpreted language exercises Question/answer exercisesThe command line interface (TEXT CEILIDH ONLY) Advantages of the command line interface General points Conclusions How Ceilidh works, Ceilidh Course Notes, User Guides etc. ReferencesDave.Marshall@cm.cf.ac.ukWed Sep 14 10:06:31 BST 1994http://www.cs.cf.ac.uk/Dave/C/chapter2 19.html [25/03/2002 10:36:12]
Using Dec Workstations and UnixNext: About this document . Up: Programming in C Previous: timer.cUsing Dec Workstations andUnixDave.Marshall@cm.cf.ac.ukWed Sep 14 10:06:31 BST 1994http://www.cs.cf.ac.uk/Dave/C/chapter2 23.html [25/03/2002 10:36:13]
ContentsContents Contents The Common Desktop Environment The front panel The file manager The application manager The session manager Other CDE desktop tools Application development tools Application integration Windows and the Window Manager The Root Menu ExercisesC/C Program Compilation Creating, Compiling and Running Your Program Creating the program Compilation Running the programThe C Compilation Model The Preprocessor C Compiler Assembler Link Editor Some Useful Compiler Options Using Libraries UNIX Library Functions Finding Information about Library Functions Lint -- A C program verifier Exerciseshttp://www.cs.cf.ac.uk/Dave/C/node1.html (1 of 13) [25/03/2002 10:36:44]
Contents C Basics History of C Characteristics of C C Program Structure Variables Defining Global Variables Printing Out and Inputting Variables Constants Arithmetic Operations Comparison Operators Logical Operators Order of Precedence ExercisesConditionals The if statement The ? operator The switch statement ExercisesLooping and Iteration The for statement The while statement The do-while statement break and continue ExercisesArrays and Strings Single and Multi-dimensional Arrays Strings ExercisesFunctions void functions Functions and Arrayshttp://www.cs.cf.ac.uk/Dave/C/node1.html (2 of 13) [25/03/2002 10:36:44]
Contents Function Prototyping ExercisesFurther Data Types Structures Unions Coercion or Type-Casting Enumerated Types Static Variables ExercisesPointers What is a Pointer? Pointer and Functions Pointers and Arrays Arrays of Pointers Multidimensional arrays and pointers Static Initialisation of Pointer Arrays Pointers and Structures Common Pointer Pitfalls Defining New Data Types Not assigning a pointer to memory address before using it Illegal indirectionExerciseDynamic Memory Allocation and Dynamic Structures Malloc, Sizeof, and Free Calloc and Realloc Linked Lists Full Program: queue.c ExercisesAdvanced Pointer Topics Pointers to Pointers Command line inputhttp://www.cs.cf.ac.uk/Dave/C/node1.html (3 of 13) [25/03/2002 10:36:44]
Contents Pointers to a Function ExercisesLow Level Operators and Bit Fields Bitwise Operators Bit Fields Bit Fields: Practical Example A note of caution: PortabilityExercisesThe C Preprocessor #define #undef #include #if -- Conditional inclusion Preprocessor Compiler Control Other Preprocessor Commands ExercisesC, UNIX and Standard Libraries Advantages of using UNIX with C Using UNIX System Calls and Library FunctionsInteger Functions, Random Number, String Conversion, Searching andSorting: stdlib.h Arithmetic Functions Random Numbers String Conversion Searching and Sorting ExercisesMathematics: math.h Math Functions Math ConstantsInput and Output (I/O):stdio.h Reporting Errors perror()http://www.cs.cf.ac.uk/Dave/C/node1.html (4 of 13) [25/03/2002 10:36:44]
Contents errno exit()Streams Predefined Streams Basic I/O Formatted I/O scanf Files Reading and writing filesStream Status Enquiries Low Level I/O ExercisesString Handling: string.h Basic String Handling Functions Printfsprintf and sscanf RedirectionString Searching Character conversions and testing: ctype.h Memory Operations: memory.h ExercisesFile Access and Directory System Calls Directory handling functions: unistd.h Scanning and Sorting Directories: sys/types.h , sys/dir.h File Manipulation Routines: unistd.h, sys/types.h, sys/stat.h File Access errno File Status File Manipulation:stdio.h, unistd.h Creating Temporary FIles: stdio.h Exerciseshttp://www.cs.cf.ac.uk/Dave/C/node1.html (5 of 13) [25/03/2002 10:36:44]
Contents Time Functions Basic time functions Example time applications Example 1: Time (in seconds) to perform some computation Example 2: Set a random number seedExercisesProcess Control: stdlib.h , unistd.h Running UNIX Commands from C execl() fork() wait() exit() ExerisesInterprocess Communication (IPC), Pipes Piping in a C program: stdio.h popen() -- Formatted Piping pipe() -- Low level Piping ExercisesIPC:Interrupts and Signals: signal.h Sending Signals -- kill(), raise() Signal Handling -- signal() sig talk.c -- complete example program Other signal functionsIPC:Message Queues: sys/msg.h Initialising the Message Queue IPC Functions, Key Arguments, and Creation Flags: sys/ipc.h Controlling message queues Sending and Receiving Messages POSIX Messages: mqueue.h Example: Sending messages between two processes message send.c -- creating and sending to a simple messagehttp://www.cs.cf.ac.uk/Dave/C/node1.html (6 of 13) [25/03/2002 10:36:44]
Contentsqueue Some further example message queue programs msgget.c: Simple Program to illustrate msget() msgctl.cSample Program to Illustrate msgctl() msgop.c: Sample Program to Illustrate msgsnd() andmsgrcv()ExercisesIPC:Semaphores Initializing a Semaphore Set Controlling Semaphores Semaphore Operations POSIX Semaphores: semaphore.h semaphore.c: Illustration of simple semaphore passing Some further example semaphore programs message rec.c -- receiving the above message semget.c: Illustrate the semget() function semctl.c: Illustrate the semctl() function semop() Sample Program to Illustrate semop()ExercisesIPC:Shared Memory Accessing a Shared Memory Segment Controlling a Shared Memory Segment Attaching and Detaching a Shared Memory Segment Example two processes comunicating via shared memory:shm server.c, shm client.c shm server.c shm client.c POSIX Shared Memory Mapped memory Address Spaces and Mapping Coherence Creating and Using Mappingshttp://www.cs.cf.ac.uk/Dave/C/node1.html (7 of 13) [25/03/2002 10:36:44]
Contents Some further example shared memory programs shmget.c:Sample Program to Illustrate shmget() shmctl.c: Sample Program to Illustrate shmctl() shmop.c: Sample Program to Illustrate shmat() andshmdt()ExercisesIPC:Sockets Socket Creation and Naming Connecting Stream Sockets Stream Data Transfer and Closing Datagram sockets Socket Options Example Socket Programs:socket server.c,socket client Other Memory Control Functions socket server.c socket client.cExercisesThreads: Basic Theory and Libraries Processes and Threads Benefits of Threads vs Processes Multithreading vs. Single threading Some Example applications of threadsThread Levels User-Level Threads (ULT) Kernel-Level Threads (KLT) Combined ULT/KLT Approaches Threads libraries The POSIX Threads Library:libpthread, pthread.h Creating a (Default) Thread Wait for Thread Termination A Simple Threads Example Detaching a Threadhttp://www.cs.cf.ac.uk/Dave/C/node1.html (8 of 13) [25/03/2002 10:36:44]
Contents Create a Key for Thread-Specific Data Delete the Thread-Specific Data Key Set the Thread-Specific Data Key Get the Thread-Specific Data Key Global and Private Thread-Specific Data Example Getting the Thread Identifiers Comparing Thread IDs Initializing Threads Yield Thread Execution Set the Thread Priority Get the Thread Priority Send a Signal to a Thread Access the Signal Mask of the Calling Thread Terminate a ThreadSolaris Threads: thread.h Unique Solaris Threads Functions Suspend Thread Execution Continue a Suspended Thread Set Thread Concurrency Level Readers/Writer Locks Readers/Writer Lock ExampleSimilar Solaris Threads Functions Create a Thread Get the Thread Identifier Yield Thread Execution Signals and Solaris Threads Terminating a Thread Creating a Thread-Specific Data Key Example Use of Thread Specific Data:Rethinking GlobalVariablesCompiling a Multithreaded Application Preparing for l (9 of 13) [25/03/2002 10:36:44]
Contents Further Threads Programming:Thread Attributes (POSIX) Attributes Initializing Thread Attributes Destroying Thread Attributes Thread's Detach State Thread's Set Scope Thread Scheduling Policy Thread Inherited Scheduling Policy Set Scheduling ParametersThread Stack Size Debugging a Multithreaded ProgramBuilding Your Own Thread StackFurther Threads Programming:Synchronization Mutual Exclusion Locks Initializing a Mutex Attribute Object Destroying a Mutex Attribute Object The Scope of a Mutex Initializing a Mutex Locking a Mutex Lock with a Nonblocking Mutex Destroying a Mutex Mutex Lock Code Examples Mutex Lock Example Using Locking Hierarchies: Avoiding Deadlock Nested Locking with a Singly Linked List Solaris Mutex LocksCondition Variable Attributes Initializing a Condition Variable Attribute Destoying a Condition Variable Attribute The Scope of a Condition Variable Initializing a Condition Variablehttp://www.cs.cf.ac.uk/Dave/C/node1.html (10 of 13) [25/03/2002 10:36:44]
Contents Block on a Condition Variable Destroying a Condition Variable State Solaris Condition VariablesThreads and Semaphores POSIX Semaphores Basic Solaris Semaphore FunctionsThread programming examples Using thr create() and thr join() Arrays Deadlock Signal Handler Interprocess Synchronization The Producer / Consumer Problem A Socket Server Using Many Threads Real-time Thread Example POSIX Cancellation Software Race Condition Tgrep: Threadeds version of UNIX grep Multithreaded QuicksortRemote Procedure Calls (RPC) What Is RPC How RPC Works RPC Application Development Defining the Protocol Defining Client and Server Application Code Compliling and running the applicationOverview of Interface Routines Simplified Level Routine Function Top Level RoutinesIntermediate Level Routineshttp://www.cs.cf.ac.uk/Dave/C/node1.html (11 of 13) [25/03/2002 10:36:44]
Contents Expert Level Routines Bottom Level RoutinesThe Programmer's Interface to RPC Simplified Interface Passing Arbitrary Data Types Developing High Level RPC Applications Sharing the data The Server Side The Client SideExerciseProtocol Compiling and Lower Level RPC Programming What is rpcgen An rpcgen Tutorial Defining the protocolConverting Local Procedures to Remote Procedures Passing Complex Data Structures Preprocessing Directives cpp Directives Compile-Time Flags Client and Server Templates Example rpcgen compile options/templates Recommended Reading ExercisesWriting Larger Programs Header files External variables and functions Scope of externals Advantages of Using Several Files How to Divide a Program between Several Files Organisation of Data in each File The Make Utilityhttp://www.cs.cf.ac.uk/Dave/C/node1.html (12 of 13) [25/03/2002 10:36:44]
Contents Make Programming Creating a makefile Make macros Running MakeDave 1.html (13 of 13) [25/03/2002 10:36:44]
The Common Desktop EnvironmentSubsections The front panel The file manager The application manager The session manager Other CDE desktop tools Application development tools Application integration Windows and the Window Manager The Root Menu ExercisesThe Common Desktop EnvironmentIn order to use Solaris and most other Unix Systems you will need to be familiar with the CommonDesktop Environment (CDE). Before embarking on learning C with briefly introduce the mainfeatures of the CDE.Most major Unix vendors now provide the CDE as standard. Consequently, most users of the XWindow system will now be exposed to the CDE. Indeed, continuing trends in the development ofMotif and CDE will probably lead to a convergence of these technologies in the near future. Thissection highlights the key features of the CDE from a Users perspective.Upon login, the user is presented with the CDE Desktop (Fig. 1.1). The desktop includes a front panel(Fig. 1.2) , multiple virtual workspaces, and window management. CDE supports the running ofapplications from a file manager, from an application manager and from the front panel. Each of thesubcomponents of the desktop are described below.http://www.cs.cf.ac.uk/Dave/C/node2.html (1 of 9) [25/03/2002 10:37:03]
The Common Desktop EnvironmentFig. 1.1 Sample CDE DesktopThe front panelThe front panel (Fig. 1.2) contains a set of icons and popup menus (more like roll-up menus) thatappear at the bottom of the screen, by default (Fig. 1.1). The front panel contains the most regularlyused applications and tools for managing the workspace. Users can drag-and-drop application iconsfrom the file manager or application manager to the popups for addition of the application(s) to theassociated menu. The user can also manipulate the default actions and icons for the popups. The frontpanel can be locked so that users can't change it. A user can configure several virtual workspaces -each with different backgrounds and colors if desired. Each workspace can have any number ofapplications running in it. An application can be set to appear in one, more than one, or all ve/C/node2.html (2 of 9) [25/03/2002 10:37:03]
The Common Desktop EnvironmentFig. 1.2 Client
errno File Status File Manipulation:stdio.h, unistd.h Creating Temporary FIles: stdio.h Exercises Time Functions Basic time functions Example time applications Example 1: Time (in seconds) to perform some computation Example 2: Set a random number seed Exercises Process Control: stdlib.h , unistd.h Running UNIX Commands from C