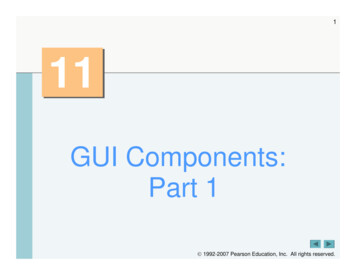
Transcription
111GUI Components:Part 1 1992-2007 Pearson Education, Inc. All rights reserved.
2Do you think I can listen all day to such stuff?— Lewis CarrollEven a minor event in the life of a child is an eventof that child’s world and thus a world event.— Gaston BachelardYou pays your money and you takes your choice.— PunchGuess if you can, choose if you dare.— Pierre Corneille 1992-2007 Pearson Education Inc. All rights reserved.
3OBJECTIVESIn this chapter you will learn: The design principles of graphical user interfaces(GUIs). To build GUIs and handle events generated byuser interactions with GUIs. To understand the packages containing GUIcomponents, event-handling classes andinterfaces. To create and manipulate buttons, labels, lists,text fields and panels. To handle mouse events and keyboard events. To use layout managers to arrange GUIcomponents 1992-2007 Pearson Education, Inc. All rights reserved.
imple GUI-Based Input/Output with JOptionPaneOverview of Swing ComponentsDisplaying Text and Images in a WindowText Fields and an Introduction to Event Handling withNested ClassesCommon GUI Event Types and Listener InterfacesHow Event Handling WorksJButtonButtons That Maintain State11.9.1 JCheckBox11.9.2 JRadioButton11.10 JComboBox and Using an Anonymous Inner Class forEvent Handling 1992-2007 Pearson Education, Inc. All rights reserved.
511.11 JList11.12 Multiple-Selection Lists11.13 Mouse Event Handling11.14 Adapter Classes11.15 JPanel Sublcass for Drawing with the Mouse11.16 Key-Event Handling11.17 Layout Managers11.17.1 FlowLayout11.17.2 BorderLayout11.17.3 GridLayout11.18 Using Panels to Manage More Complex Layouts11.19 JTextArea11.20 Wrap-Up 1992-2007 Pearson Education, Inc. All rights reserved.
611.1 Introduction Graphical user interface (GUI)– Presents a user-friendly mechanism for interacting with anapplication– Often contains title bar, menu bar containing menus,buttons and combo boxes– Built from GUI components 1992-2007 Pearson Education, Inc. All rights reserved.
7Look-and-Feel Observation 11.1Consistent user interfaces enable a user to learnnew applications faster. 1992-2007 Pearson Education, Inc. All rights reserved.
8buttonmenustitle barmenu barcombo boxscrollbarsFig. 11.1 Internet Explorer window with GUI components. 1992-2007 Pearson Education, Inc. All rights reserved.
911.2 Simple GUI-Based Input/Output withJOptionPane Dialog boxes– Used by applications to interact with the user– Provided by Java’s JOptionPane class Contains input dialogs and message dialogs 1992-2007 Pearson Education, Inc. All rights reserved.
1// Fig. 11.2: Addition.java2// Addition program that uses JOptionPane for input and output.3import javax.swing.JOptionPane; // program uses JOptionPane10Outline45public class Addition6{78public static void main( String args[] ){Show input dialog to receive firstintegerAddition.java9// obtain user input from JOptionPane input dialogs10String firstNumber 111213JOptionPane.showInputDialog( "Enter firstString secondNumber (1 of 2)Show input dialog to receiveinteger" );second integerJOptionPane.showInputDialog( "Enter second integer" );1415// convert String inputs to int values for use in a calculation16int number1 Integer.parseInt( firstNumber );17int number2 Integer.parseInt( secondNumber );18Show message dialog to outputsum to user1920int sum number1 number2; // add numbers21// display result in a JOptionPane message dialog22JOptionPane.showMessageDialog( null, "The sum is " sum,23"Sum of Two Integers", JOptionPane.PLAIN MESSAGE );24} // end method main25 } // end class Addition 1992-2007 Pearson Education, Inc. All rights reserved.
11Input dialog displayed by lines 10–11Prompt to the userOutlineText field in whichthe user types avalueWhen the user clicks OK,showInputDialogreturns to the programthe 100 typed by theuser as a String. Theprogram must convertthe String to an intAddition.java(2 of 2)Input dialog displayed by lines 12–13title barMessage dialog displayed by lines 22–23When the user clicks OK, themessage dialog is dismissed(removed from the screen) 1992-2007 Pearson Education, Inc. All rights reserved.
12Look-and-Feel Observation 11.2The prompt in an input dialog typically usessentence-style capitalization—a style thatcapitalizes only the first letter of the first wordin the text unless the word is a proper noun(for example, Deitel). 1992-2007 Pearson Education, Inc. All rights reserved.
13Look-and-Feel Observation 11.3The title bar of a window typically uses book-titlecapitalization—a style that capitalizes the firstletter of each significant word in the text anddoes not end with any punctuation (for example,Capitalization in a Book Title). 1992-2007 Pearson Education, Inc. All rights reserved.
14Message dialog typeIconDescriptionERROR MESSAGEA dialog that indicates an error to the user.INFORMATION MESSAGEA dialog with an informational message to theuser.WARNING MESSAGEA dialog warning the user of a potentialproblem.QUESTION MESSAGEPLAIN MESSAGEA dialog that poses a question to the user. Thisdialog normally requires a response, such asclicking a Yes or a No button.no icon A dialog that contains a message, but no icon.Fig. 11.3 JOptionPane static constants for message dialogs. 1992-2007 Pearson Education, Inc. All rights reserved.
1511.3 Overview of Swing Components Swing GUI components– Declared in package javax.swing– Most are pure Java components– Part of the Java Foundation Classes (JFC) 1992-2007 Pearson Education, Inc. All rights reserved.
16ComponentDescriptionJLabelDisplays uneditable text or icons.JTextFieldEnables user to enter data from the keyboard. Can also be used todisplay editable or uneditable text.JButtonTriggers an event when clicked with the mouse.JCheckBoxSpecifies an option that can be selected or not selected.JComboBoxProvides a drop-down list of items from which the user can make aselection by clicking an item or possibly by typing into the box.JListProvides a list of items from which the user can make a selection byclicking on any item in the list. Multiple elements can be selected.JPanelProvides an area in which components can be placed and organized.Can also be used as a drawing area for graphics.Fig. 11.4 Some basic GUI components. 1992-2007 Pearson Education, Inc. All rights reserved.
17Swing vs. AWT Abstract Window Toolkit (AWT)– Precursor to Swing– Declared in package java.awt– Does not provide consistent, cross-platform look-and-feel 1992-2007 Pearson Education, Inc. All rights reserved.
18Portability Tip 11.1Swing components are implemented in Java, sothey are more portable and flexible than theoriginal Java GUI components from packagejava.awt, which were based on the GUIcomponents of the underlying platform. For thisreason, Swing GUI components are generallypreferred. 1992-2007 Pearson Education, Inc. All rights reserved.
19Lightweight vs. Heavyweight GUIComponents Lightweight components– Not tied directly to GUI components supported byunderlying platform Heavyweight components– Tied directly to the local platform– AWT components– Some Swing components 1992-2007 Pearson Education, Inc. All rights reserved.
20Look-and-Feel Observation 11.4The look and feel of a GUI defined withheavyweight GUI components from packagejava.awt may vary across platforms. Becauseheavyweight components are tied to the localplatform GUI, the look and feel varies fromplatform to platform. 1992-2007 Pearson Education, Inc. All rights reserved.
21Superclasses of Swing’s Lightweight GUIComponents Class Component (package java.awt)– Subclass of Object– Declares many behaviors and attributes common to GUIcomponents Class Container (package java.awt)– Subclass of Component– Organizes Components Class JComponent (package javax.swing)– Subclass of Container– Superclass of all lightweight Swing components 1992-2007 Pearson Education, Inc. All rights reserved.
22Software Engineering Observation 11.1Study the attributes and behaviors of the classesin the class hierarchy of Fig. 11.5. These classesdeclare the features that are common to mostSwing components. 1992-2007 Pearson Education, Inc. All rights reserved.
23Fig. 11.5 Common superclasses of many of the Swing components. 1992-2007 Pearson Education, Inc. All rights reserved.
24Superclasses of Swing’s Lightweight GUIComponents Common lightweight component features– Pluggable look-and-feel to customize the appearance ofcomponents– Shortcut keys (called mnemonics)– Common event-handling capabilities– Brief description of component’s purpose (called tool tips)– Support for localization 1992-2007 Pearson Education, Inc. All rights reserved.
2511.4 Displaying Text and Images in aWindow Class JFrame– Most windows are an instance or subclass of this class– Provides title bar– Provides buttons to minimize, maximize and close theapplication 1992-2007 Pearson Education, Inc. All rights reserved.
26Labeling GUI Components Label– Text instructions or information stating the purpose ofeach component– Created with class JLabel 1992-2007 Pearson Education, Inc. All rights reserved.
27Look-and-Feel Observation 11.5Text in a JLabel normally uses sentence-stylecapitalization. 1992-2007 Pearson Education, Inc. All rights reserved.
28Specifying the Layout Laying out containers– Determines where components are placed in the container– Done in Java with layout managers One of which is class FlowLayout– Set with the setLayout method of class JFrame 1992-2007 Pearson Education, Inc. All rights reserved.
12// Fig. 11.6: LabelFrame.java// Demonstrating the JLabel class.34import java.awt.FlowLayout; // specifies how components are arrangedimport javax.swing.JFrame; // provides basic window features5import javax.swing.JLabel; // displays text and images67import javax.swing.SwingConstants; // common constants used with Swingimport javax.swing.Icon; // interface used to manipulate images89import javax.swing.ImageIcon; // loads images29OutlineLabelFrame.java(1 of 2)10 public class LabelFrame extends JFrame11 {121314private JLabel label1; // JLabel with just textprivate JLabel label2; // JLabel constructed with text and iconprivate JLabel label3; // JLabel with added text and icon1516// LabelFrame constructor adds JLabels to JFrame1718public LabelFrame(){1920super( "Testing JLabel" );setLayout( new FlowLayout() ); // set frame layout212223// JLabel constructor with a string argumentlabel1 new JLabel( "Label with text" );2425label1.setToolTipText( "This is label1" );add( label1 ); // add label1 to JFrame26 1992-2007 Pearson Education, Inc. All rights reserved.
27// JLabel constructor with string, Icon and alignment arguments28Icon bug new ImageIcon( getClass().getResource(29label2 new JLabel( "Label with text and icon", bug,30"bug1.gif" ) );OutlineSwingConstants.LEFT );31label2.setToolTipText( "This is label2" );32add( label2 ); // add label2 to JFrameLabelFrame.java3334label3 new JLabel(); // JLabel constructor no arguments35label3.setText( "Label with icon and text at bottom" );36label3.setIcon( bug ); // add icon to JLabel37label3.setHorizontalTextPosition( SwingConstants.CENTER );38label3.setVerticalTextPosition( SwingConstants.BOTTOM );3940label3.setToolTipText( "This is label3" );add( label3 ); // add label3 to JFrame4130(2 of 2)} // end LabelFrame constructor42 } // end class LabelFrame 1992-2007 Pearson Education, Inc. All rights reserved.
1// Fig. 11.7: LabelTest.java2// Testing LabelFrame.3import javax.swing.JFrame;31Outline45public class LabelTest6{LabelTest.java7public static void main( String args[] )8{9LabelFrame labelFrame new LabelFrame(); // create LabelFrame10labelFrame.setDefaultCloseOperation( JFrame.EXIT ON CLOSE );11labelFrame.setSize( 275, 180 ); // set frame size12labelFrame.setVisible( true ); // display frame13} // end main14 } // end class LabelTest 1992-2007 Pearson Education, Inc. All rights reserved.
32Creating and Attaching label1 Method setToolTipText of classJComponent– Specifies the tool tip Method add of class Container– Adds a component to a container 1992-2007 Pearson Education, Inc. All rights reserved.
33Common Programming Error 11.1If you do not explicitly add a GUI componentto a container, the GUI component will not bedisplayed when the container appears on thescreen. 1992-2007 Pearson Education, Inc. All rights reserved.
34Look-and-Feel Observation 11.6Use tool tips to add descriptive text to your GUIcomponents. This text helps the user determinethe GUI component’s purpose in the userinterface. 1992-2007 Pearson Education, Inc. All rights reserved.
35Creating and Attaching label2 Interface Icon– Can be added to a JLabel with the setIcon method– Implemented by class ImageIcon Interface SwingConstants– Declares a set of common integer constants such as thoseused to set the alignment of components– Can be used with methods setHorizontalAlignmentand setVerticalAlignment 1992-2007 Pearson Education, Inc. All rights reserved.
36Creating and Attaching label3 Other JLabel methods– getText and setText For setting and retrieving the text of a label– getIcon and setIcon For setting and retrieving the icon displayed in the label– getHorizontalTextPosition andsetHorizontalTextPosition For setting and retrieving the horizontal position of the textdisplayed in the label 1992-2007 Pearson Education, Inc. All rights reserved.
37ConstantDescriptionHorizontal-position wingConstants.RIGHTPlace text on the left.Place text in the center.Place text on the right.Vertical-position ingConstants.BOTTOMPlace text at the top.Place text in the center.Place text at the bottom.Fig. 11.8 Some basic GUI components. 1992-2007 Pearson Education, Inc. All rights reserved.
Creating and Displaying a LabelFrameWindow38 Other JFrame methods– setDefaultCloseOperation Dictates how the application reacts when the user clicks theclose button– setSize Specifies the width and height of the window– setVisible Determines whether the window is displayed (true) or not(false) 1992-2007 Pearson Education, Inc. All rights reserved.
3911.5 Text Fields and an Introduction toEvent Handling with Nested Classes GUIs are event-driven– A user interaction creates an event Common events are clicking a button, typing in a text field,selecting an item from a menu, closing and window andmoving the mouse– The event causes a call to a method called an event handler 1992-2007 Pearson Education, Inc. All rights reserved.
4011.5 Text Fields and an Introduction toEvent Handling with Nested Classes Class JTextComponent– Superclass of JTextField Superclass of JPasswordField– Adds echo character to hide text input in component– Allows user to enter text in the component whencomponent has the application’s focus 1992-2007 Pearson Education, Inc. All rights reserved.
1234// Fig. 11.9: TextFieldFrame.java// Demonstrating the JTextField class.import java.awt.FlowLayout;import wing.JOptionPane;(1 of 3)public class TextFieldFrame extends JFrame12 {1314private JTextField textField1; // text field with set sizeprivate JTextField textField2; // text field constructed with text15161718private JTextField textField3; // text field with text and sizeprivate JPasswordField passwordField; // password field with text192021public TextFieldFrame(){super( "Testing JTextField and JPasswordField" );// TextFieldFrame constructor adds JTextFields to JFrame22232425setLayout( new FlowLayout() ); // set frame layout2627add( textField1 ); // add textField1 to JFrameCreate a new JTextField// construct textfield with 10 columnstextField1 new JTextField( 10 ); 1992-2007 Pearson Education, Inc. All rights reserved.
2829303132// construct textfield with default texttextField2 new JTextField( "Enter text here" );add( textField2 ); // add textField2 to JFrame33343536textField3 new JTextField( "Uneditable text field", 21 );textField3.setEditable( false ); // disable editingadd( textField3 ); // add textField3 to JFrameMake this3738394041// construct passwordfield with default textpasswordField new JPasswordField( "Hidden text" );add( passwordField ); // add passwordField to JFrame42434445TextFieldHandler handler new TextFieldHandler();textField1.addActionListener( handler );CreatetextField2.addActionListener( handler );textField3.addActionListener( handler );464748495051525354555642OutlineCreate a new JTextField// construct textfield with default text and 21 columnsTextFieldFrame.java(2 of 3)JTextField uneditableCreate a new JPasswordField// register event handlerspasswordField.addActionListener( handler );} // end TextFieldFrame constructorevent handlerRegister event handler// private inner class for event handlingprivate class TextFieldHandler implements ActionListener{// process text field eventspublic void actionPerformed( ActionEvent event ){Create event handler class byimplementing theActionListener interfaceString string ""; // declare string to displayDeclare actionPerformedmethod 1992-2007 Pearson Education, Inc. All rights reserved.
57585960616263646566676869707172737475767778// user pressed Enter in JTextField textField1if ( event.getSource() textField1 )string String.format( "textField1: %s",event.getActionCommand() );// user pressed Enter in JTextField textField2else if ( event.getSource() textField2 )string String.format( "textField2: %s",event.getActionCommand() );// user pressed Enter in JTextField textField3else if ( event.getSource() textField3 )string String.format( "textField3: %s",event.getActionCommand() );43Test if the source of the eventis theOutlinefirst text fieldGet text from text fieldTextFieldFrameTest if the source of the eventis the.javasecond text field(3 of 3)Get text from text fieldTest if the source of the event is thethird text fieldGet// user pressed Enter in JTextField passwordFieldtext from text fieldelse if ( event.getSource() passwordField )Test if the sourcestring String.format( "passwordField: %s",password fieldnew String( passwordField.getPassword() ) );// display JTextField contentJOptionPane.showMessageDialog( null, string );of the event is theGet password from password field79} // end method actionPerformed80} // end private inner class TextFieldHandler81 } // end class TextFieldFrame 1992-2007 Pearson Education, Inc. All rights reserved.
1// Fig. 11.10: TextFieldTest.java2// Testing TextFieldFrame.3import javax.swing.JFrame;44Outline45public class TextFieldTest6{7public static void main( String args[] )8{TextFieldTest.java9TextFieldFrame textFieldFrame new peration( JFrame.EXIT ON CLOSE );11textFieldFrame.setSize( 325, 100 ); // set frame size12textFieldFrame.setVisible( true ); // display frame13(1 of 2)} // end main14 } // end class TextFieldTest 1992-2007 Pearson Education, Inc. All rights reserved.
45OutlineTextFieldTest.java(2 of 2) 1992-2007 Pearson Education, Inc. All rights reserved.
46Steps Required to Set Up Event Handlingfor a GUI Component Several coding steps are required for anapplication to respond to events– Create a class for the event handler– Implement an appropriate event-listener interface– Register the event handler 1992-2007 Pearson Education, Inc. All rights reserved.
47Using a Nested Class to Implement anEvent Handler Top-level classes– Not declared within another class Nested classes– Declared within another class– Non-static nested classes are called inner classes Frequently used for event handling 1992-2007 Pearson Education, Inc. All rights reserved.
48Software Engineering Observation 11.2An inner class is allowed to directly access itstop-level class’s variables and methods, even ifthey are private. 1992-2007 Pearson Education, Inc. All rights reserved.
49Using a Nested Class to Implement anEvent Handler JTextFields and JPasswordFields– Pressing enter within either of these fields causes anActionEvent Processed by objects that implement the ActionListenerinterface 1992-2007 Pearson Education, Inc. All rights reserved.
50Registering the Event Handler for EachText Field Registering an event handler– Call method addActionListener to register anActionListener object– ActionListener listens for events on the object 1992-2007 Pearson Education, Inc. All rights reserved.
51Software Engineering Observation 11.3The event listener for an event must implementthe appropriate event-listener interface. 1992-2007 Pearson Education, Inc. All rights reserved.
52Common Programming Error 11.2Forgetting to register an event-handler object fora particular GUI component’s event type causesevents of that type to be ignored. 1992-2007 Pearson Education, Inc. All rights reserved.
Details of Class TextFieldHandler’sactionPerformed Method53 Event source– Component from which event originates– Can be determined using method getSource– Text from a JTextField can be acquired usinggetActionCommand– Text from a JPasswordField can be acquired usinggetPassword 1992-2007 Pearson Education, Inc. All rights reserved.
5411.6 Common GUI Event Types andListener Interfaces Event types– All are subclasses of AWTEvent– Some declared in package java.awt.event– Those specific to Swing components declared injavax.swing.event 1992-2007 Pearson Education, Inc. All rights reserved.
5511.6 Common GUI Event Types andListener Interfaces Delegation event model– Event source is the component with which user interacts– Event object is created and contains information about theevent that happened– Event listener is notified when an event happens 1992-2007 Pearson Education, Inc. All rights reserved.
56Fig. 11.11 Some event classes of package java.awt.event. 1992-2007 Pearson Education, Inc. All rights reserved.
57Fig. 11.12 Some common event-listener interfaces of package java.awt.event. 1992-2007 Pearson Education, Inc. All rights reserved.
5811.7 How Event Handling Works Remaining questions– How did the event handler get registered?– How does the GUI component know to callactionPerformed rather than some other eventhandling method? 1992-2007 Pearson Education, Inc. All rights reserved.
59Registering Events Every JComponent has instance variablelistenerList– Object of type EventListenerList– Maintains references to all its registered listeners 1992-2007 Pearson Education, Inc. All rights reserved.
60Fig. 11.13 Event registration for JTextField textField1 . 1992-2007 Pearson Education, Inc. All rights reserved.
61Event-Handler Invocation Events are dispatched to only the event listenersthat match the event type– Events have a unique event ID specifying the event type MouseEvents are handled byMouseListeners andMouseMotionsListeners KeyEvents are handled by KeyListeners 1992-2007 Pearson Education, Inc. All rights reserved.
6211.8 JButton Button– Component user clicks to trigger a specific action– Can be command button, check box, toggle button or radiobutton– Button types are subclasses of class AbstractButton 1992-2007 Pearson Education, Inc. All rights reserved.
63Look-and-Feel Observation 11.7Buttons typically use book-title capitalization. 1992-2007 Pearson Education, Inc. All rights reserved.
6411.8 JButton Command button– Generates an ActionEvent when it is clicked– Created with class JButton– Text on the face of the button is called button label 1992-2007 Pearson Education, Inc. All rights reserved.
65Look-and-Feel Observation 11.8Having more than one JButton with the samelabel makes the JButtons ambiguous to the user.Provide a unique label for each button. 1992-2007 Pearson Education, Inc. All rights reserved.
66Fig. 11.14 Swing button hierarchy. 1992-2007 Pearson Education, Inc. All rights reserved.
1234// Fig. 11.15: ButtonFrame.java// Creating JButtons.import java.awt.FlowLayout;import ortimportimportButtonFrame.java910111213import javax.swing.ImageIcon;import ing.Icon;public class ButtonFrame extends JFrame{(1 of 2)Declare two JButton instancevariables14151617private JButton plainJButton; // button with just textprivate JButton fancyJButton; // button with icons18192021public ButtonFrame(){super( "Testing Buttons" );CreatesetLayout( new FlowLayout() ); // set frame layout// ButtonFrame adds JButtons to JFramenew JButton22232425plainJButton new JButton( "Plain Button" ); // Createbutton twowithImageIconstextadd( plainJButton ); // add plainJButton to JFrame26272829Icon bug1 new ImageIcon( getClass().getResource( "bug1.gif" ) );Icon bug2 new ImageIcon( getClass().getResource( "bug2.gif" ) );Set rollover icon forfancyJButton new JButton( "Fancy Button", bug1 ); // set imagefancyJButton.setRolloverIcon( bug2 ); // set rollover image30add( fancyJButton ); // add fancyJButton to JFrameCreate new JButtonJButton 1992-2007 Pearson Education, Inc. All rights reserved.
3132333468// create new ButtonHandler for button event handlingButtonHandler handler new ButtonHandler();fancyJButton.addActionListener( handler );Outline35363738plainJButton.addActionListener( handler );} // end ButtonFrame constructorCreate handler for buttons// inner class for button event handlingRegister event handlers39404142private class ButtonHandler implements ActionListener{// handle button eventpublic void actionPerformed( ActionEvent event ) Inner43444546ButtonFrame.java(2 of 2)class implements ActionListener{JOptionPane.showMessageDialog( ButtonFrame.this, String.format("You pressed: %s", event.getActionCommand() ) );} // end method actionPerformed47} // end private inner class ButtonHandler48 } // end class ButtonFrameAccess outer class’s instance usingthis referenceGet text of JButton pressed 1992-2007 Pearson Education, Inc. All rights reserved.
1// Fig. 11.16: ButtonTest.java2// Testing ButtonFrame.3import javax.swing.JFrame;69Outline45public class ButtonTest6{ButtonTest.java7public static void main( String args[] )8{(1 of 2)9ButtonFrame buttonFrame new ButtonFrame(); // create ButtonFrame10buttonFrame.setDefaultCloseOperation( JFrame.EXIT ON CLOSE );11buttonFrame.setSize( 275, 110 ); // set frame size12buttonFrame.setVisible( true ); // display frame13} // end main14 } // end class ButtonTest 1992-2007 Pearson Education, Inc. All rights reserved.
70OutlineButtonTest.java(2 of 2) 1992-2007 Pearson Education, Inc. All rights reserved.
7111.8 JButton JButtons can have a rollover icon– Appears when mouse is positioned over a button– Added to a JButton with method setRolloverIcon 1992-2007 Pearson Education, Inc. All rights reserved.
72Look-and-Feel Observation 11.9Because class AbstractButton supportsdisplaying text and images on a button, allsubclasses of AbstractButton also supportdisplaying text and images. 1992-2007 Pearson Education, Inc. All rights reserved.
73Look-and-Feel Observation 11.10Using rollover icons for JButtons providesusers with visual feedback indicating that whenthey click the mouse while the cursor ispositioned over the button, an action willoccur. 1992-2007 Pearson Education, Inc. All rights reserved.
74Software Engineering Observation 11.4When used in an inner class, keyword thisrefers to the current inner-class object beingmanipulated. An inner-class method can use itsouter-class object’s this by preceding thiswith the outer-class name and a dot, as inButtonFrame.this. 1992-2007 Pearson Education, Inc. All rights reserved.
7511.9 Buttons That Maintain State State buttons– Swing contains three types of state buttons– JToggleButton, JCheckBox and JRadioButton– JCheckBox and JRadioButton are subclasses ofJToggleButton 1992-2007 Pearson Education, Inc. All rights reserved.
7611.9.1 JCheckBox JCheckBox– Contains a check box label that appears to right of checkbox by default– Generates an ItemEvent when it is clicked ItemEvents are handled by an ItemListener Passed to method itemStateChanged– Method isSelected returns whether check box isselected (true) or not (false) 1992-2007 Pearson Education, Inc. All rights reserved.
1234// Fig. 11.17: CheckBoxFrame.java// Creating JCheckBox buttons.import java.awt.FlowLayout;import c class CheckBoxFrame extends JFrame12 {131477(1 of 3)Declare two JCheckBox instancevariablesprivate JTextField textField; // displays text in changing fontsprivate JCheckBox boldJCheckBox; // to select/deselect bold15161718private JCheckBox italicJCheckBox; // to select/deselect italic192021{222324252627// CheckBoxFrame constructor adds JCheckBoxes to JFramepublic CheckBoxFrame()super( "JCheckBox Test" );setLayout( new FlowLayout() ); // set frame layoutSet font of text field// set up JTextField and set its fonttextField new JTextField( "Watch the font style change", 20 );textField.setFont( new Font( "Serif", Font.PLAIN, 14 ) );add( textField ); // add textField to JFrame 1992-2007 Pearson Education, Inc. All rights reserved.
28293031boldJCheckBox new JCheckBox( "Bold" ); // create bold checkboxitalicJCheckBox new JCheckBox( "Italic" ); // create italicadd( boldJCheckBox ); // add bold checkbox to JFrameadd( italicJCheckBox ); // add italic checkbox to JFrame// register
Swing components are implemented in Java, so they are more portable and flexible than the original Java GUI components from package java.awt, which were based on the GUI components of the underlying platform. For this reason,