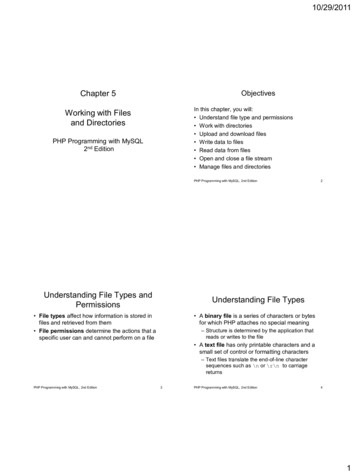
Transcription
10/29/2011Chapter 5ObjectivesIn this chapter, you will: Understand file type and permissions Work with directories Upload and download files Write data to files Read data from files Open and close a file stream Manage files and directoriesWorking with Filesand DirectoriesPHP Programming with MySQL2nd EditionPHP Programming with MySQL, 2nd EditionUnderstanding File Types andPermissions2Understanding File Types File types affect how information is stored infiles and retrieved from them File permissions determine the actions that aspecific user can and cannot perform on a file A binary file is a series of characters or bytesfor which PHP attaches no special meaning– Structure is determined by the application thatreads or writes to the file A text file has only printable characters and asmall set of control or formatting characters– Text files translate the end-of-line charactersequences such as \n or \r\n to carriagereturnsPHP Programming with MySQL, 2nd Edition3PHP Programming with MySQL, 2nd Edition41
10/29/2011Understanding File Types(continued)Understanding File Types(continued) Different operating systems use differentescape sequences to identify the end of a line:– Use the \n sequence to end a line on a UNIX/Linuxoperating system– Use the \n\r sequence to end a line on a Windowsoperating system– Use the \r sequence to end a line on a Macintoshoperating system.PHP Programming with MySQL, 2nd Edition5Understanding File Types(continued)PHP Programming with MySQL, 2nd Edition6Working with File Permissions Scripts written in a UNIX/Linux text editordisplay differently when opened in a Windowsbased text editor Files and directories have three levels of access:– User– Group– Other The three typical permissions for files anddirectories are:– Read (r)– Write (w)– Execute (x)Figure 5-1 Volunteer registration formPHP Programming with MySQL, 2nd Edition7PHP Programming with MySQL, 2nd Edition82
10/29/2011Working with File Permissions(continued)Working with File Permissions(continued) File permissions are calculated using a four-digitoctal (base 8) value– Octal values encode three bits per digit, whichmatches the three permission bits per level ofaccess– The first digit is always 0– To assign more than one value to an accesslevel, add the values of the permissions togetherPHP Programming with MySQL, 2nd Edition9Working with File Permissions(continued)10Checking Permissions The chmod() function is used to change thepermissions or modes of a file or directory The syntax for the chmod() function is The fileperms() function is used to readpermissions associated with a file– The fileperms() function takes one argumentand returns an integer bitmap of the permissionsassociated with the file– Permissions can be extracted using the arithmeticmodulus operator with an octal value of 01000chmod( filename, mode) Where filename is the name of the file tochange and mode is an integer specifying thepermissions for the filePHP Programming with MySQL, 2nd EditionPHP Programming with MySQL, 2nd Edition The dococt() function converts a decimalvalue to an octal value11PHP Programming with MySQL, 2nd Edition123
10/29/2011Reading Directories(continued)Reading Directories The opendir() function is used to iteratethrough entries in a directory A handle is a special type of variable that PHPused to represent a resource such as a file or adirectory The readdir() function returns the file anddirectory names of an open directory The directory pointer is a special type ofvariable that refers to the currently selectedrecord in a directory listing The following table lists the PHP functions thatread the names of files and directoriesPHP Programming with MySQL, 2nd Edition13Reading Directories(continued)PHP Programming with MySQL, 2nd Edition14Reading Directories(continued) The closedir() function is used to close thedirectory handle The following code lists the files in the opendirectory and closes the directory. The following Figure shows the directory listingfor three files: kitten.jpg, polarbear.jpg, andgorilla.gif Dir "/var/html/uploads"; DirOpen opendir( Dir);while ( CurFile readdir( DirOpen)) {echo CurFile . " br / \n";}closedir( DirOpen);PHP Programming with MySQL, 2nd EditionFigure 5-2 Listing of the “files” subdirectory using the opendir(),readdir(), and closedir() functions15PHP Programming with MySQL, 2nd Edition164
10/29/2011Reading Directories(continued)Reading Directories(continued) The scandir() function returns the names ofthe entries in a directory to an array sorted inascending alphabetical order The PHP scripting engine returns the navigationshortcuts (“.” and “.”) when it reads a directory The strcmp() function can be used to exclude thoseentries Dir "/var/html/uploads"; DirEntries scandir( Dir);foreach ( DirEntries as Entry) {echo Entry . " br / \n";while ( CurFile readdir( DirOpen))if ((strcmp( CurFile, '.') ! 0) &&(strcmp( CurFile, '.') ! 0))echo " a href \"files/" . CurFile . "\" " . CurFile . " /a br / ";}} PHP Programming with MySQL, 2nd Edition17Reading Directories(continued)PHP Programming with MySQL, 2nd Edition18Creating Directories The mkdir() function creates a new directory To create a new directory within the currentdirectory:– Pass just the name of the directory you want tocreate to the mkdir() functionmkdir("volunteers");Figure 5-3 Listing of the “files” subdirectoryusing the scandir() functionPHP Programming with MySQL, 2nd Edition19PHP Programming with MySQL, 2nd Edition205
10/29/2011Creating Directories (continued)Creating Directories (continued) To create a new directory in a locationother than the current directory:– Use a relative or an absolute pathmkdir("./event");Figure 5-4 Warning that appears if a directory already existsmkdir("/bin/PHP/utilities");PHP Programming with MySQL, 2nd Edition21Obtaining File and DirectoryInformationPHP Programming with MySQL, 2nd EditionPHP Programming with MySQL, 2nd Edition22Obtaining File and DirectoryInformation (continued)23PHP Programming with MySQL, 2nd Edition246
10/29/2011Obtaining File and DirectoryInformation (continued)Obtaining File and DirectoryInformation (continued) Dir "/var/html/uploads";if (is dir( Dir)) {echo " table border '1' width '100%' \n";echo " tr th Filename /th th File Size /th th File Type /th /tr \n"; DirEntries scandir( Dir);foreach ( DirEntries as Entry) { EntryFullName Dir . "/" . Entry;echo " tr td " . htmlentities( Entry) . " /td td " .filesize( EntryFullName) . " /td td " .filetype( EntryFullName) . " /td /tr \n";}echo " /table \n";}elseecho " p The directory " . htmlentities( Dir) . " does notexist. /p ";PHP Programming with MySQL, 2nd EditionFigure 5-5 Output of script with file and directoryinformation functions25Obtaining File and DirectoryInformation (continued)26Uploading and Downloading Files The following table returns additional informationabout files and directories:PHP Programming with MySQL, 2nd EditionPHP Programming with MySQL, 2nd Edition27 Web applications allow visitors to upload files toand from from their local computer (oftenreferred to as the client) The files that are uploaded and downloadedmay be simple text files or more complex filetypes, such as images, documents, orspreadsheetsPHP Programming with MySQL, 2nd Edition287
10/29/2011Selecting the File(continued)Selecting the File Files are uploaded through an XHTML formusing the “post” method An enctype attribute in the opening form tagmust have a value of “multipart/form-data,”which instructs the browser to post multiplesections – one for regular form data and one forthe file contents The file input field creates a Browse button forthe user to navigate to the appropriate file toupload input type "file" name "picture file" / The MAX FILE SIZE (uppercase) attribute of ahidden form field specifies the maximum numberof bytes allowed in the uploaded file– The MAX FILE SIZE hidden field must appearbefore the file input fieldPHP Programming with MySQL, 2nd Edition29Retrieving the File Information30Retrieving the File Information(continued)– // Contains the name of the original file When the form is posted, information for theuploaded file is stored in the FILESautoglobal array The FILES[] array contains five elements: FILES['picture file']['name']– // Contains the size of the uploadedfile in bytes FILES['picture file']['size']– FILES['picture file']['error'] //Contains the error code associated withthe file– // Contains the type of the file FILES['picture file']['type']– FILES['picture file']['tmp name'] //Contains the temporary location of thefile contentsPHP Programming with MySQL, 2nd EditionPHP Programming with MySQL, 2nd Edition31PHP Programming with MySQL, 2nd Edition328
10/29/2011Storing the Uploaded File(continued)Storing the Uploaded File Uploaded files are either public or privatedepending on whether they should beimmediately available or verified first Public files are freely available to anyone visitingthe Web site Private files are only available to authorizedvisitorsPHP Programming with MySQL, 2nd Edition33Storing the Uploaded File(continued)PHP Programming with MySQL, 2nd Edition34Downloading Files The function returns TRUE if the move succeeds,and FALSE if the move failsif(move uploaded file( FILES['picture file']['tmp name'],"uploads/" . FILES['picture file']['name']) FALSE)echo "Could not move uploaded file to \"uploads/" .htmlentities( FILES['picture file']['name']) . "\" br/ \n";elseecho "Successfully uploaded \"uploads/" .htmlentities( FILES['picture file']['name']) . "\" br/ \n";PHP Programming with MySQL, 2nd Edition The move uploaded file() function movesthe uploaded file from its temporary location to apermanent destination with the following syntax:bool move uploaded file(string filename, string destination) filename is the contents of FILES['filefield']['tmp name'] and destination is the path and filename of thelocation where the file will be stored. Files in the public XHTML directory structure canbe downloaded with an XHTML hyperlink Files outside the public XHTML directory requirea three-step process:– Tell the script which file to download– Provide the appropriate headers– Send the file The header() function is used to return headerinformation to the Web browser35PHP Programming with MySQL, 2nd Edition369
10/29/2011Downloading Files(continued)Writing an Entire File PHP supports two basic functions for writingdata to text files:– file put contents() function writes orappends a text string to a file and returns thenumber of bytes written to the file– fwrite() function incrementally writes data to atext filePHP Programming with MySQL, 2nd Edition37Writing an Entire File(continued)38Writing an Entire File(continued) The file put contents() function writes orappends a text string to a file The syntax for the file put contents()function is:file put contents (filename, string[, options])PHP Programming with MySQL, 2nd EditionPHP Programming with MySQL, 2nd Edition39 EventVolunteers " Blair, Dennis\n "; EventVolunteers . " Hernandez, Louis\n "; EventVolunteers . " Miller, Erica\n "; EventVolunteers . " Morinaga, Scott\n "; EventVolunteers . " Picard, Raymond\n "; VolunteersFile " volunteers.txt ";file put contents( VolunteersFile, EventVolunteers);PHP Programming with MySQL, 2nd Edition4010
10/29/2011Writing an Entire File(continued)Writing an Entire File(continued)) If no data was written to the file, the functionreturns a value of 0 Use the return value to determine whether datawas successfully written to the fileif (file put contents( VolunteersFile, EventVolunteers) 0)echo " p Data was successfully written to the VolunteersFile file. /p ";elseecho " p No data was written to the VolunteersFile file. /p ";PHP Programming with MySQL, 2nd Edition41 The FILE USE INCLUDE PATH constantsearches for the specified filename in the paththat is assigned to the include path directivein your php.ini configuration file The FILE APPEND constant appends data toany existing contents in the specified filenameinstead of overwriting itPHP Programming with MySQL, 2nd Edition42Reading an Entire File(continued)Reading an Entire File The readfile() function reads the entirecontents of a file into a string DailyForecast " p strong San Francisco daily weatherforecast /strong : Today: Partly cloudy. Highs from the 60s tomid 70s. West winds 5 to 15 mph. Tonight: Increasing clouds. Lowsin the mid 40s to lower 50s. West winds 5 to 10 mph. /p ";file put contents("sfweather.txt", DailyForecast); SFWeather file get contents("sfweather.txt");echo SFWeather;PHP Programming with MySQL, 2nd Edition43PHP Programming with MySQL, 2nd Edition4411
10/29/2011Reading an Entire File(continued)Reading an Entire File(continued) The readfile() function displays thecontents of a text file along with the file size to aWeb browserreadfile("sfweather.txt");PHP Programming with MySQL, 2nd Edition The file() function reads the entire contentsof a file into an indexed array Automatically recognizes whether the lines in atext file end in \n, \r, or \r\n January " 61, 42, 48\n "; January . "62, 41, 49\n "; January . " 62, 41, 49\n "; January . " 64, 40, 51\n "; January . " 69, 44, 55\n "; January . " 69, 45, 52\n "; January . " 67, 46, 54\n ";file put contents("sfjanaverages.txt", January);45Reading an Entire File(continued)PHP Programming with MySQL, 2nd Edition46Reading an Entire File(continued) JanuaryTemps file("sfjanaverages.txt");for ( i 0; i count( JanuaryTemps); i) { CurDay explode(", ", JanuaryTemps[ i]);echo " p strong Day " . ( i 1) . " /strong br / ";echo "High: { CurDay[0]} br / ";echo "Low: { CurDay[1]} br / ";echo "Mean: { CurDay[2]} /p ";}Figure 5-13 Output of individual lines in a text filePHP Programming with MySQL, 2nd Edition47PHP Programming with MySQL, 2nd Edition4812
10/29/2011Opening and Closing File Streams A handle is a special type of variable that PHPuses to represent a resource such as a file The fopen() function opens a handle to a filestream The syntax for the fopen() function is: A stream is a channel used for accessing aresource that you can read from and write to The input stream reads data from a resource(such as a file) The output stream writes data to a resource1. Open the file stream with the fopen() function2. Write data to or read data from the file stream3. Close the file stream with the fclose() functionPHP Programming with MySQL, 2nd EditionOpening a File Stream49Opening a File Stream(continued)open file fopen("text file", " mode"); A file pointer is a special type of variable thatrefers to the currently selected line or characterin a filePHP Programming with MySQL, 2nd Edition50Opening a File Stream(continued) VolunteersFile fopen(“volunteers.txt",“r ");Figure 5-15 Location of the file pointer when the fopen()function uses a mode argument of “a ”PHP Programming with MySQL, 2nd Edition51PHP Programming with MySQL, 2nd Edition5213
10/29/2011Opening a File Stream (continued)Closing a File Stream Use the fclose function when finished workingwith a file stream to save space in memory Use the statement fclose( handle); toensure that the file doesn’t keep taking up spacein your computer’s memory and allow otherprocesses to read to and write from the file VolunteersFile fopen(“volunteers.txt",“a ");Figure 5-16 Location of the file pointer when the fopen()function uses a mode argument of “a ”PHP Programming with MySQL, 2nd Edition53Writing Data IncrementallyPHP Programming with MySQL, 2nd Edition54Locking Files Use the fwrite() function to incrementallywrite data to a text file The syntax for the fwrite() function is: To prevent multiple users from modifying a filesimultaneously use the flock() function The syntax for the flock() function is:fwrite( handle, data[, length]);flock( handle, operation) The fwrite() function returns the number ofbytes that were written to the file If no data was written to the file, the functionreturns a value of 0PHP Programming with MySQL, 2nd Edition55PHP Programming with MySQL, 2nd Edition5614
10/29/2011Reading Data Incrementally(continued)Reading Data Incrementally You must use fopen() and fclose() with thefunctions listed in Table 5-10 Each time you call any of the functions in Table5-10, the file pointer automatically moves to thenext line in the text file (except for fgetc()) Each time you call the fgetc() function, the filepointer moves to the next character in the file The fgets() function uses the file pointer to iteratethrough a text filePHP Programming with MySQL, 2nd Edition57Managing Files and Directories PHP can be used to manage files and thedirectories that store them Among the file directory and management tasksfor files and directories are– Copying– Moving– Renaming– DeletingPHP Programming with MySQL, 2nd EditionPHP Programming with MySQL, 2nd Edition58Copying and Moving Files Use the copy() function to copy a file with PHP The function returns a value of TRUE if it issuccessful or FALSE if it is not The syntax for the copy() function is:copy(source, destination) For the source and destination arguments:– Include just the name of a file to make a copy inthe current directory, or– Specify the entire path for each argument59PHP Programming with MySQL, 2nd Edition6015
10/29/2011Copying and Moving Files(continued)Renaming Files and Directories Use the rename() function to rename a file ordirectory with PHP The rename() function returns a value of true ifit is successful or false if it is not The syntax for the rename() function is:if (file exists(" sfweather.txt ")) {if(is dir(" history ")) {if (copy(" sfweather.txt "," history\\sfweather01-27-2006.txt "))echo " p File copied successfully. /p ";elseecho " p Unable to copy the file! /p ";}elseecho (" p The directory does not exist! /p ");}elseecho (" p The file does not exist! /p ");PHP Programming with MySQL, 2nd Editionrename(old name, new name)61PHP Programming with MySQL, 2nd Edition62Removing Files and DirectoriesSummary Use the unlink() function to delete files andthe rmdir() function to delete directories Pass the name of a file to the unlink()function and the name of a directory to thermdir() function Both functions return a value of true if successfulor false if not Use the file exists() function to determinewhether a file or directory name exists beforeyou attempt to delete it In PHP, a file can be one of two types: binary ortext A binary file is a series of characters or bytesfor which PHP attaches no special meaning A text file has only printable characters and asmall set of control of formatting characters A text file translates the end-of-line charactersequences in code display The UNIX/Linux platforms end a line with the \nsequencePHP Programming with MySQL, 2nd EditionPHP Programming with MySQL, 2nd Edition636416
10/29/2011Summary (continued)Summary (continued) The Windows platforms end a line with the \n\rsequence The Macintosh platforms end a line with the \rsequence Files and directories have three levels of access:user, group, and other Typical file and directory permissions includeread, write, and execute PHP provides the chmod() function forchanging the permissions of a file within PHP The syntax for the chmod()function ischmod( filename, mode) The chmod() function uses a four-digit octalvalue to assign permissions The fileperms(), which takes filename asthe only parameter, returns a bitmap of thepermissions associated with a file The opendir() function iterates through theentries in a directoryPHP Programming with MySQL, 2nd EditionPHP Programming with MySQL, 2nd Edition6566Summary (continued)Summary (continued) A handle is a special type of variable thatrepresents a resource, such as a file or directory To iterate through the entries in a directory, youopen a handle to the directory with theopendir() function Use the readdir() function to return the fileand directory names from the open directory Use the closedir() function to close adirectory handle The scandir() function returns an indexedarray of the files and directories ( in ascendingalphabetical order) in a specified directory The mkdir(), with a single name argument,creates a new directory The is readable(), is writeable(), andis executable() functions check the the fileor directory to determine if the PHP scriptingengine has read, write, or execute permissions,respectivelyPHP Programming with MySQL, 2nd EditionPHP Programming with MySQL, 2nd Edition676817
10/29/2011Summary (continued)Summary (continued) A symbolic link, which is identified with theis link() is a reference to a file not on thesystem The is dir() determines if a directory exists Directory information functions provide fileaccess dates, file owner, and file type Uploading a file refers to transferring the file to aWeb server Setting the enctype attribute of the openingfrom tag to multipart/form-data instructs thebrowser to post one section for regular form dataand one section for file contents The file input type creates a browse buttonthat allows the user to navigate to a file toupload To limit the size of the file upload, above the fileinput field, insert a hidden field with an attributeMAX FILE SIZE and a value in bytesPHP Programming with MySQL, 2nd EditionPHP Programming with MySQL, 2nd Edition6970Summary (continued)Summary (continued) An uploaded file’s information (error code,temporary file name, filename, size, and type) isstored in the FILES array MIME (Multipurpose Internet Mail Extension)generally classifies the file upload as in“image.gif”, “image.jpg”, “text/plain,” or“text/html” The move uploaded file() function movesthe uploaded file to its permanent destination The file put contents() function writes orappends a text string to a file and returns thenumber of bytes written to the file The FILE APPEND constant appends data toany existing contents in the specified filenameinstead of overwriting it The file get contents() and readfile()functions read the entire contents of a file into astringPHP Programming with MySQL, 2nd EditionPHP Programming with MySQL, 2nd Edition717218
10/29/2011Summary (continued)Summary (continued) A stream is a channel that is used for accessinga resource to which you may read, and write. The input stream reads data from a resource,such as a file The output stream writes data to a resource,such as a file The fopen() opens a handle to a file streamusing the syntax open file fopen("text file", "mode"); A file pointer is a variable that refers to thecurrently selected line or character in a file Mode arguments used with the fopen()function specifies if the file is opened for reading,writing, or executing, and the indicates thelocation of the file pointer The fclose() function with a syntax offclose( handle); is used to close a filestreamPHP Programming with MySQL, 2nd EditionPHP Programming with MySQL, 2nd Edition73Summary (continued)Summary (continued) The fwrite() incrementally writes data to atext file To prevent multiple users from modifying a filesimultaneously use the flock() function A number of PHP functions are available toiterate through a text file by line or character Use the copy() function to copy a file with PHP Use the rename() function to rename a file ordirectory with PHPPHP Programming with MySQL, 2nd Edition7475 The unlink() function is used to delete filesand the rmdir() function is used to deletedirectories In lieu of a move function, the rename()function renames a file and specifies a newdirectory to store the renamed filePHP Programming with MySQL, 2nd Edition7619
PHP Programming with MySQL, 2nd Edition 3 Understanding File Types and Permissions File types affect how information is stored in files and retrieved from them File permissions determine the actions that a specific user can and cannot perform on a file PHP Programming with