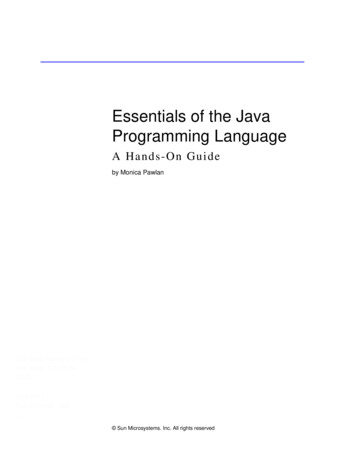
Transcription
Essentials of the JavaProgramming LanguageA Hands-On Guideby Monica Pawlan350 East Plumeria DriveSan Jose, CA 95134USAMay 2013Part Number TBDv1.0 Sun Microsystems. Inc. All rights reserved
Essentials of the Java Programming LanguageIf you are new to programming in the Java programming language (Java) and have someexperience with other languages, this tutorial could be for you. It walks through how to usethe Java Platform software to develop a basic network application that uses common Javaplatform features. This tutorial is not comprehensive, but instead takes you on a straight anduncomplicated path through the more common features available in the Java platform. Thistutorial is a learning tool and should be viewed as a stepping-stone for persons who find thecurrently available materials a little too overwhelming to start with.To reduce your learning curve, this tutorial begins with a simple program in Lesson 1,develops the program by adding new features in every lesson, and leaves you with a generalelectronic commerce application, and a basic understanding of object-oriented programmingconcepts in Lesson 15. Unlike other more reference-style texts that give you a lot ofdefinitions and concepts at the beginning, this tutorial takes a practical approach. Newfeatures and concepts are described when they are added to the example application.Please note the final application is for instructional purposes only and would need more workto make it production worthy. By the time you finish this tutorial, you should have enoughknowledge to comfortably go on to other Java programming language learning materials andcontinue your studies.If you have no programming experience at all, you might still find this tutorial helpful; but youalso might want to take an introductory programming course before you proceed.Lessons 1 through 8 explain how applications, applets, and servlets/JavaServer Pages aresimilar and different, how to build a basic user interface that handles simple user input, howto read data from and write data to files and databases, and how to send and receive dataover the network.Lessons 9 through 15 walk you through socket communications, building a user interfaceusing more components, grouping multiple data elements as one unit (collections), savingdata between program invocations (serialization), and internationalizing a program. Lesson15 concludes the series with basic object-oriented programming concepts.This tutorial covers object-oriented concepts at the end after you have had practicalexperience with the language so you can relate the object-oriented concepts to yourexperiences.Appendix A presents the complete and final code for this tutorial.JavaBean TechnologyJavaBean technology, which lets you create portable program components that followsimple naming and design conventions, is not covered here. While creating a simpleJavaBean component is easy, understanding JavaBeans features requires knowledge ofsuch things as properties, serialization, events, and inheritance. When you finish theselessons, you should have the knowledge you need to go on to a good text on JavaBeanstechnology and continue your studies.2
Essentials of the Java Programming LanguageAcknowledgementsMany Java Developer Connection (JDC) members contributed comments andsuggestions to this material when the first eight lessons appeared on the JDC website inMarch and April of 1999, and the last eight appeared the following July. With thosesuggestions and many others received from the review team at Addison-WesleyLongman, the material has evolved into an introduction to Java programming languagefeatures for persons new to the platform and unfamiliar with the terminology.I also relied on the help of co-workers, friends, and family for whose help I am verygrateful. I would like to thank my friend and co-worker, Mary Aline, for providing theFrench translations for the Chapter 13, Internationalization chapter, and my best friendand husband Jeffrey Pawlan (WA6KBL) who worked with Wolf Geihe (DJ4OA) inGermany to provide the German translations for that same chapter. I do not want toforget Stephanie Wilde, our contract editor at the JDC, who helped with copy editing onthe early versions of this material posted to the JDC website. And Dana Nourie, our JDCHTML editor, who in her quest to learn Java, provided unending enthusiasm for this workand contributed to the section on how to set the CLASSPATH environment variable on theWindows platform.Special thanks go to Allan Jacobs and Orson Alvarez who went through the examplecode and text making a number of excellent suggestions to improve them, and to CalvinAustin whose helpful suggestions at the outset made the earlier lessons moreunderstandable and accessible to novice programmers. Finally, thanks to DaneshForouhari who made some excellent suggestions to improve the graphics, and whoencouraged me to include a short section on JavaServer Pages technology. And I cannotforget my manager, Margaret Ong, who stood behind me all of the way in this effort.Lastly, I want to acknowledge various individual reviewers within Sun Microsystems, Inc.,whose expert knowledge in their respective areas was an invaluable asset to completingthe examples: Rama Roberts (object-oriented programming), Dale Green(internationalization), Alan Sommerer (JAR file format and packages), Joshua Bloch(collections), and Tony Squier (databases).3
ContentsChapter 1 Compile and Run a Simple ProgramAbout the Java Platform . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11Set Up Your Computer . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11Write a Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 12Compile the Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 12Run the Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 12Code Comments. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 12Double Slashes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13C-Style Comments . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13Doc Comments. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13API Documentation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14Chapter 2 Building ApplicationsApplication Structure and Elements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16Fields and Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 17Constructors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 20Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 21Chapter 3 Building AppletsApplication to Applet. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 23Run the Applet . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 24Applet Structure and Elements. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 24Extend a Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 24Behavior . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 25Appearance . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 27Packages . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 27Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 28Chapter 4 Building a User InterfaceProject Swing APIs . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 30Import Statements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 31Class Declaration . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 32Instance Variables . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 33Constructor . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 33Action Listening . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 35Event Handling . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 35Main Method. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 364
Essentials of the Java Programming LanguageExercises: Applets Revisited . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 37Applet and Application Differences. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 38Chapter 5 Building ServletsAbout the Example . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 40HTML Form . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 40Servlet Code. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 41Class and Method Declarations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 42Method Implementation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 43JavaServer Pages Technology. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 44HTML Form. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 44JSP Page . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 44Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 46Chapter 6 Access and PermissionsFile Access by Applications . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 48Constructor and Instance Variable Changes . . . . . . . . . . . . . . . . . . . . . 48Method Changes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 49System Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 52File.separatorChar . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 52Exception Handling. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 52File Access by Applets . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 54Grant Applets Permission . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 56Creating a Policy File . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 56Run an Applet with a Policy File . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 56Restrict Applications . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 57File Access by Servlets . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 58Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 58Code for This Lesson . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 58FileIO Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 58FileIOAppl Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 61FileIOServlet Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 63AppendIO Program. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 64Chapter 7 Database Access and PermissionsDatabase Setup . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 69Create Database Table . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 69Database Access by Applications . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 69Establish a Database Connection . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 70Database Access by Applets . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 73JDBC Driver . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 73JDBC-ODBC Bridge with ODBC Driver . . . . . . . . . . . . . . . . . . . . . . . . . 75Database Access by Servlets . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 76Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 76Code for This Lesson . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 77Dba Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 775
Essentials of the Java Programming LanguageDbaAppl Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 79DbaOdbAppl Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 82DbaServlet Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 84Chapter 8 Remote Method InvocationRMI Scenario . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 87About the Example . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 87Program Behavior. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 88File Summary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 89Compile the Example . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 90Start the RMI Registry . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 91Start the Server. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 92Run the RMIClient1 Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 93Run the RMIClient2 Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 93RemoteServer Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 94Send Interface . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 95RMIClient1 Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 96actionPerformed Method . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 96main Method . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 96RMIClient2 Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 97actionPerformed Method . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 97main Method . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 97Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 98Code for This Lesson . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 98RMIClient1 Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 98RMIClient2 Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 100RemoteServer Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 102Send Interface . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 103Chapter 9 Socket CommunicationsWhat are Sockets and Threads? . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 105About the Examples . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 105Example 1: Client-Side Behavior . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 106Example 1: Server-Side Behavior. . . . . . . . . . . . . . . . . . . . . . . . . . . . . 106Example 1: Compile and Run . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 106Example 1: Server-Side Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 107Example 1: Client-Side Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 108Example 2: Multithreaded Server Example . . . . . . . . . . . . . . . . . . . . . 110Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 113Code for This Lesson . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 113SocketClient Program. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 113SocketServer Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 115SocketThrdServer Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 117Chapter 10 Object-Oriented ProgrammingObject-Oriented Programming . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1226
Essentials of the Java Programming LanguageClasses . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 122Objects . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 123Well-Defined Boundaries and Cooperation . . . . . . . . . . . . . . . . . . . . . 123Inheritance and Polymorphism . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 124Data Access Levels . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 126Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 126Fields and Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 126Global Variables and Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 127Your Own Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 127Well-Defined Boundaries and Cooperation . . . . . . . . . . . . . . . . . . . . . 127Inheritance . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 128Access Levels. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 128Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 129Setting Access Levels. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 129Organizing Code into Functional Units . . . . . . . . . . . . . . . . . . . . . . . . . 129Chapter 11 User Interfaces RevisitedAbout the Example . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 131Fruit Order Client (RMIClient1) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 131Server Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 132View Order Client (RMIClient2) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 132Compile and Run the Example . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 132Fruit Order (RMIClient1) Code . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 134Instance Variables . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 135Constructor . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 135Event Handling . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 137Cursor Focus . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 139Converting Strings to Numbers and Back. . . . . . . . . . . . . . . . . . . . . . . 140Server Program Code. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 141Send Interface . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 141RemoteServer Class. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 141View Order Client (RMIClient2) Code. . . . . . . . . . . . . . . . . . . . . . . . . . . . 142Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 143Calculations and Pressing Return . . . . . . . . . . . . . . . . . . . . . . . . . . . . 143Extra Credit. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 144Code for This Lesson . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 144RMIClient1 Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 144RMIClient2 Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 149RMIClient1 Improved Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 152Chapter 12 Develop the ExampleTrack Orders. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 159sendOrder Method . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 159getOrder Method. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 160Other Changes to Server Code . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 161Maintain and Display a Customer List . . . . . . . . . . . . . . . . . . . . . . . . . . . 162About Collections . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1627
Essentials of the Java Programming LanguageCreate a Set . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 163Access Data in a Set . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 164Display Data in a Dialog Box . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 165Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 166Code for This Lesson . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 167RemoteServer Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 167RMIClient2 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 169Chapter 13 InternationalizationIdentify Culturally Dependent Data. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 174Create Keyword and Value Pair Files. . . . . . . . . . . . . . . . . . . . . . . . . . . . 175German Translations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 177French Translations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 178Internationalize Application Text. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 179Instance Variables . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 179main Method . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 179Constructor . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 181actionPerformed Method . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 182Internationalize Numbers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 182Compile and Run the Application . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 183Compile. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 183Start the RMI Registry . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 183UNIX . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 183Win32 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 183Start the Server. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 184Start the RMIClient1 Program in German. . . . . . . . . . . . . . . . . . . . . . . 184Start the RMIClient2 Program in French. . . . . . . . . . . . . . . . . . . . . . . . 184Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 185Code for This Lesson . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 186RMIClient1 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 186RMIClient2 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 191Chapter 14 Packages and JAR File FormatSet up Class Packages . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 197Create the Directories. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 197Declare the Packages. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 198Make Classes and Fields Accessible . . . . . . . . . . . . . . . . . . . . . . . . . . 198Change Client Code to Find the Properties Files . . . . . . . . . . . . . . . . . 199Compile and Run the Example. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 199Compile. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 199Start the RMI Registry . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 200Start the Server. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 200Start the RMIGermanApp Program . . . . . . . . . . . . . . . . . . . . . . . . . . . 201Start the RMIClient2 Program in French. . . . . . . . . . . . . . . . . . . . . . . . 201Using JAR Files to Deploy . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 201Fruit Order Set of Files (RMIClient1) . . . . . . . . . . . . . . . . . . . . . . . . . . 203View Order Set of Files. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2048
Essentials of the Java Programming LanguageExercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 205Appendix A Code ListingsRMIClient1 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 207RMIClient2 . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 212DataOrder. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 217Send . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 217RemoteServer . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 218RMIFrenchApp . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 220RMIGermanApp . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 225RMIEnglishApp. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 230RMIClientView Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 234RMIClientController Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 238Index9
1.Compile and Run a Simple Program1If you are new to theJava,Javayouprogrammingmight have heardlanguage,of applets,you areapplications,probably wonderingservlets, andwhatJavaServerall the talkis about.butPages,Youaremightnot surehavewhatheardtheyof applets,are and applications,how they differ.andOrservlets/JavaServermaybe you are justPages,curiousbutaboutarenot surethebasicwhatset oftheyapplicationare and whenprogrammingyou wouldinterfaceswant to write(APIs)anavailableapplet asinopposedthe platformto anandapplicationdo notor servlet.wantto readOramaybelot of pagesyou areto justlearncuriousthe basics.about the basic set of application programminginterfaces (APIs) available in the platform and do not want to read a lot of pages to find out whatThis short tutorial gives you a hands-on introduction to Java. It starts with compiling and runningis available.the simple program presented in this lesson, adds new features with explanations in eachThis short tutoriala hands-onto theinJavaprogrammingsuccessivelesson,givesand youintroducesAPIs introductioncommonly usedgeneralprograms.language. It startswith compiling and running the simple program presented in this lesson, adds new features withThis lesson covers the following topics:explanations in each successive lesson, and introduces APIs commonly used in generalprograms. About the Java PlatformSet UpYourtheComputerThis lessoncoversfollowing topics: WriteProgramAboutatheJava Platform Compilethe ProgramSet Up YourComputerRuntheProgramWrite a Program CodeCommentsCompilethe ProgramAPIDocumentationRun the Program ExercisesCode Comments API Documentation Exercises10
Essentials of the Java Programming LanguageAbout the Java PlatformBefore you can write and compile programs, you need to understand what the Java platformis and configure your computer to run the programs. The Java platform consists of the JavaAPIs and the Java Virtual Machine (JVM).Java APIs are libraries of compiled code that you can use in your programs. They enable youto add ready-made and customizable functionality to save you programming time. The simpleprogram in this lesson uses a Java API to print a line of text to the console. The printingcapability is provided in the API ready for you to use. You supply the text to be printed.Figure 1 shows the Java platform architecture. The JVM sits on top of your native operatingsystem. Your program sits on top of the JVM and calls compiled code from the API librariesthat live within the JVM. Java ProgramJava APIsJava Virtual MachineYour Computer SystemFigure 1. Java Platform ArchitecturePrograms written
Essentials of the Java Programming Language If you are new to programming in the Java programming language (Java) and have some experience with other languages, this tutorial could be for you. It walks through how to use the Java Platform software to develop a basic network application