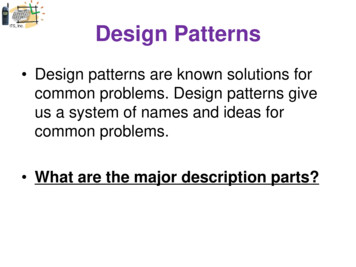
Transcription
Design Patterns Design patterns are known solutions forcommon problems. Design patterns giveus a system of names and ideas forcommon problems. What are the major description parts?
Design Patterns Descriptions Design Patterns consist of the following parts:- Problem Statement- Solution- ----------There are several Levels and Types of theDesign Patterns.What Levels and Types do you know?
Design Patterns Levels and Types There are different types and levels of design patterns. For example, theMVC is the architectural level of design pattern while the rest of thepatterns from the list above are component level design patterns. The basic types are Behavior, Creational, Structural, and Systemdesign patterns. Names are extremely important in design patterns; theyshould be clear and descriptive. More types: Enterprise and SOA Design PatternsChristopher Alexander – The first book on Design PatternsClassics: "Design Patterns: Elements of Reusable ObjectOriented Software" by Erich Gamma, Richard Helm, RalphJohnson, John Vlissides (GOF)Among other good books: “Integration-Ready Architectureand Design or Software and Knowledge Engineering”
Here is an example of creating a newDesign Pattern What: Applicationdevelopment or evenmodification require longerand longer projects Why: Growing applicationsbecome more complex andrigid; too firm and inflexible inspite of the name – SoftwareSpecial efforts are needed
Industry Lessons LearnedDesign PatternsBusiness-Driven Architecture How can technology be designed toremain in alignment with changingbusiness goals and requirements?TechnologyTechnologyBusinessGood AlignmentBusinessBad AlignmentDuplicationsCode branchesMaintenance Cost
Business-DrivenArchitecture Solution Business and architecture analysis isconducted as collaborative efforts on aregular basis Impact To keep technology in alignment with thebusiness that is changing over time, it willrequire a commitment in time and cost togovern
Design Pattern - MVCController MVC (Model – View – Controller) is well known patternModelName – MVCProblem – Complex object involves user interface and data. Need tosimplify structure Solution – Data in one part (Model), user View in another part (View),interaction logic in a third part (Controller)– Model maintains state. Notifies view of changes in state.– Controller uses state information (in Model?) and user request todetermine how to handle request, tells view what to display– View must correctly display the state of the Model Consequences– Allows "plug in" modules – eg. swap out Model to allow different waysof holding data– Requires separate engineering of the three parts, communicationbetween them through interfacesView
Factory Method Problem – Need to create a family of similar butdifferent type objects that are used in standard ways. Solution – Creator class has a "getter" method whichinstantiate the correct subclass, i.e. ConcreteProduct,Subclass is used through generic interface, i.e.Product Impact – Extra time for analysis and modeling
Factory Method & Servlet Best PracticesNew services can be added run time as new JSPs/ASPs or Java /.NET classes//serviceName and serviceDetails are to be populated// by servlet doPost() , doGet() or service() methodsString serviceName request.getParameter(“service”);Hashtable serviceDetails getServiceDetails();Service service // known or new service(Service) Class.forName(serviceName).newInstance();String content e(“text/html"); // “application/xsl” and etc.response.getWriter().println(content);XML based Service API allows us to describe any existing and future service ServiceRequest service “Mail” action “get” Param paramName1 /Param /ServiceRequest We can find both Dispatcher and Factory patterns in this example. This approach makes itpossible to create a unified API for client – server communications. Any service (includingnew, unknown design time services) can be requested by a client without code change.
Design PatternCanonical Data Model How can services be designed to avoiddata model transformation? Problem Services with disparate models for similardata impose transformation requirementsthat increase development effort, designcomplexity, and runtime performanceoverhead.
Canonical Data Model Solution Data models for common information setsare standardized across service contractswithin an inventory boundary. Application Design standards are applied to schemasused by service contracts as part of aformal design process.
Canonical Data Model Principles Standardized Service Contract Architecture Inventory, Service
Design PatternCanonical Protocol How can services be designed to avoid protocolbridging? Problem Services that support different communicationtechnologies compromise interoperability, limitthe quantity of potential consumers, andintroduce the need for undesirable protocolbridging measures.
Canonical Protocol Solution The architecture establishes a singlecommunications technology as the sole orprimary medium by which services caninteract. Application The communication protocols (includingprotocol versions) used within a serviceinventory boundary are standardized for allservices.
Design PatternConcurrent Contracts How can a service facilitate multiconsumer coupling requirements andabstraction concerns at the same time? Problem A service’s contract may not be suitable orapplicable for all of the service’s potentialconsumers.
Concurrent Contracts Solution Multiple contracts can be created for asingle service, each targeted at a specifictype of consumer. Application This pattern is ideally applied together withthe Service Façade pattern to support newcontracts as required.
Singleton Design Pattern Problem – need to be sure there is at most one objectof a given class in the system at one time Solution– Hide the class constructor– Provide a method in the class to obtain the instance– Let class manage the single instancepublic class Singleton{private static Singleton instance;private Singleton(){} // private constructor!public Singleton getInstance(){if (instance null)instance new Singleton();return instance;}}
Provider Design Pattern ContextSeparate implementations of the API from the API itselfProblemWe needed a flexible design and at the same time easily extensibleSolutionA provider implementation derives from an abstract base class, which is used todefine a contract for a particular feature.For example, to create a provider for multiple storage platforms, you create thefeature base class RDBMSProvider that derives from a commonStorageProvider base class that forces the implementation of requiredmethods and properties common to all providers.Then you create the DB2Provider, OracleProvider, MSSQLProvider, etc.classes that derived from the RDBMSProvider.In a similar manner you create the DirectoryStorageProvider derived from theStorageProvider with its subclasses ActiveDirectoryProvider,LDAPProvider, and etc.
javax.sql.DataSource able Data Service forMultiple Storage rparseXML()Providing Access to MultipleData Sources via Unified APIget(); update();delete(); insert(); DBMSXMLDataDescriptor ConnectorMultiple storage platforms can be transparentjava.sql.Connection interfaceThe same basic data operations are implemented by connectorsData structure and business rules are captured in XML descriptorsDesign Patterns: Model, Adapter, Provider
Authentication ServiceDelegation, Façade and Provider Design PatternsValidateGetRolesChangeRoles1. Delegation: application-specific rules are in a configuration file2. Façade: a single interface for all applications regardless of data source3. Provider: Works with multiple datasource providersActive Directory, LDAP and RDBMSLayered: separated Utility and Data Access LayersBusinessUtility ServicesData Layer ServicesStandard-based: Web Service and Messaging Service Standard InterfacesSecure: Protected by HTTPS and Valid Certificates
Authentication ServiceProvider, Façade and Model Design Patterns// read config & build application map on initiationAppsArray[] apps serviceConfig.getApplicationArray();// apps maps each application to its data ---------// getRoles(appName, userName);AuthServiceDao dao apps.getService(appName);// dao is one of types: LdapDao, AdDao or DbDaoString roles dao.getRoles(userName);
How Façade Design Pattern can help us toImprove Implementations of InternetServices, Increase Reuse and RemoveDuplicationsApp1App2Multiple instancesof Customer DataDB1DB2App3DB3DB4DB5App4DB6
From Project-based code to EnterpriseServices using Façade Design PatternMultiple instancesof Customer DataApp1App2App3DB1DB2DB3Customer Service(Wrapper)DB4DB5DB6App4NewNewMore Web and Internal Applications
Enterprise Services will Shield Applicationsand Enable Changes from current to betterImplementationsPortal ServicesTPSApp1GLApp2Customer Subscription ServiceEnterprise Services(Wrapper)FutureImplementationsProduct Service(Wrapper)Publish and promoteadaptation of WebServiceseLinkApp4NewNew
Design PatternDelegate Problem Business logics is often customized onclient requests creating maintenance pain Solution Delegate changeable part of businesslogic to a special component, like a rulesservice, and simplify changing this logic.
Design PatternAgnostic Context How can multi-purpose service logic bepositioned as an effective enterpriseresource? Problem Multi-purpose logic grouped together withsingle purpose logic results in programswith little or no reuse potential thatintroduce waste and redundancy into anenterprise.
Agnostic Context Solution Isolate logic that is not specific to onepurpose into separate services withdistinct agnostic contexts. Application Agnostic service contexts are defined bycarrying out service-oriented analysisand service modeling processes.
GovernanceConnect System and Enterprise ArchitecturesConnect Business and Technology ArchitectureEngage Teams in Collaborative ss requirementsConduct service-oriented analysis to re-think Enterprise Architecture
SOA with TOGAFLearn:TOGAF IntroTOGAF ADM Features to Support SOA
Why TOGAF & SOA? The Open Group Architecture Framework (TOGAF) TOGAF is a mature EA framework SOA is an architecture style Enterprises struggle to move to SOA TOGAF helps to describe EA and steps for SOA
EnterpriseContinuum
Phase A: TOGAF General Views Business Architecture viewsData Architecture viewsApplications Architecture viewsTechnology Architecture views
BusinessDataServiceMapping Business and Technology ViewsInfrastructureBusiness Architecture/Process View: Workflows & ScenariosBusiness Architecture/Product View:Product Lines, Products, FeaturesDescriptions and order termsData Architecture:Standards, RepositoriesDescriptions and ModelsService Views:Business/Utility/Data ServicesDescriptions and execution termsTechnology Architecture:Platforms/Servers/Net/Security
Questions?Please feel free to email or call Jeff:720-299-4701Looking for your feedback: what was especially helpful andwhat else you would like to know, and what are better ways towork together in a collaborative fashion
patterns from the list above are component level design patterns. The basic types are Behavior, Creational, Structural, and System design patterns. Names are extremely important in design patterns; they should be clear and descriptive. More types: Enterprise