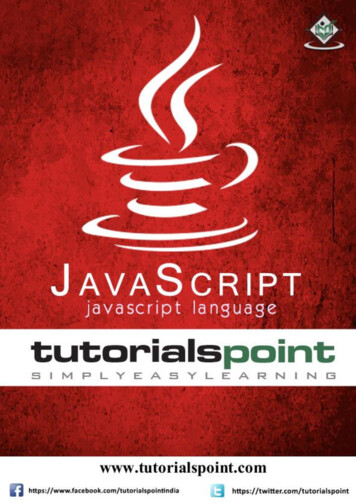
Transcription
About the TutorialJavaScript is a lightweight, interpreted programming language. It is designed forcreating network-centric applications. It is complimentary to and integrated withJava. JavaScript is very easy to implement because it is integrated with HTML. Itis open and cross-platform.AudienceThis tutorial has been prepared for JavaScript beginners to help them understandthe basic functionality of JavaScript to build dynamic web pages and webapplications.PrerequisitesFor this tutorial, it is assumed that the reader have a prior knowledge of HTMLcoding. It would help if the reader had some prior exposure to object-orientedprogramming concepts and a general idea on creating online applications.Copyright and Disclaimer Copyright 2015 by Tutorials Point (I) Pvt. Ltd.All the content and graphics published in this e-book are the property of TutorialsPoint (I) Pvt. Ltd. The user of this e-book is prohibited to reuse, retain, copy,distribute or republish any contents or a part of contents of this e-book in anymanner without written consent of the publisher.We strive to update the contents of our website and tutorials as timely and asprecisely as possible, however, the contents may contain inaccuracies or errors.Tutorials Point (I) Pvt. Ltd. provides no guarantee regarding the accuracy,timeliness or completeness of our website or its contents including this tutorial. Ifyou discover any errors on our website or in this tutorial, please notify us atcontact@tutorialspoint.comi
Table of ContentsAbout the Tutorial .Audience . iPrerequisites . iCopyright and Disclaimer . iTable of Contents . iiPART 1: JAVASCRIPT BASICS . 11.JAVASCRIPT – Overview . 2What is JavaScript? . 2Client-Side JavaScript. 2Advantages of JavaScript . 3Limitations of JavaScript . 3JavaScript Development Tools. 3Where is JavaScript Today? . 42.JAVASCRIPT – Syntax . 5Your First JavaScript Code . 5Whitespace and Line Breaks . 6Semicolons are Optional. 6Case Sensitivity . 7Comments in JavaScript . 73.JAVASCRIPT – Enabling . 9JavaScript in Internet Explorer . 9JavaScript in Firefox . 9JavaScript in Chrome . 10JavaScript in Opera . 10Warning for Non-JavaScript Browsers . 104.JAVASCRIPT – Placement . 12JavaScript in head . /head Section . 12JavaScript in body . /body Section . 13JavaScript in body and head Sections . 13JavaScript in External File . 145.JAVASCRIPT – Variables . 16JavaScript Datatypes. 16JavaScript Variables . 16JavaScript Variable Scope . 17JavaScript Variable Names . 18JavaScript Reserved Words . 196.JAVASCRIPT – Operators . 20What is an Operator? . 20Arithmetic Operators. 20Comparison Operators . 23Logical Operators. 26ii
Bitwise Operators . 28Assignment Operators . 31Miscellaneous Operators. 347.JAVASCRIPT – If-Else . 38Flow Chart of if-else. 38if Statement . 39if.else Statement . 40if.else if. Statement . 418.JAVASCRIPT – Switch-Case . 43Flow Chart . 439.JAVASCRIPT – While Loop . 47The while Loop . 47The do.while Loop . 4910. JAVASCRIPT – For Loop . 52The for Loop . 5211. JAVASCRIPT – For-in Loop . 5512. JAVASCRIPT – Loop Control. 57The break Statement . 57The continue Statement . 59Using Labels to Control the Flow . 6013. JAVASCRIPT – Functions . 64Function Definition . 64Calling a Function . 65Function Parameters . 66The return Statement . 67Nested Functions . 68Function () Constructor . 70Function Literals . 7114. JAVASCRIPT – Events. 74What is an Event? . 74onclick Event Type . 74onsubmit Event Type . 75onmouseover and onmouseout . 76HTML 5 Standard Events . 7715. JAVASCRIPT – Cookies . 82What are Cookies?. 82How It Works? . 82Storing Cookies . 83Reading Cookies . 84Setting Cookies Expiry Date . 86Deleting a Cookie . 87iii
16. JAVASCRIPT – Page Redirect . 89What is Page Redirection? . 89JavaScript Page Refresh . 89Auto Refresh . 89How Page Re-direction Works? . 9017. JAVASCRIPT – Dialog Box . 94Alert Dialog Box . 94Confirmation Dialog Box . 95Prompt Dialog Box . 9618. JAVASCRIPT – Void Keyword . 9819. JAVASCRIPT – Page Printing . 101How to Print a Page? . 102PART 2: JAVASCRIPT OBJECTS . 10320. JAVASCRIPT – Objects . 105Object Properties. 105Object Methods . 105User-Defined Objects . 106Defining Methods for an Object . 108The ‘with’ Keyword . 10921. JAVASCRIPT – Number . 112Number Properties . 112MAX VALUE . 113MIN VALUE . 114NaN . 115NEGATIVE INFINITY . 117POSITIVE INFINITY . 118Prototype . 119constructor . 121Number Methods . 121toExponential () . 122toFixed (). 124toLocaleString () . 125toPrecision () . 126toString () . 127valueOf () . 12822. JAVASCRIPT – Boolean . 130Boolean Properties . 130constructor () . 130Prototype . 131Boolean Methods . 132toSource () . 133toString () . 134valueOf () . 135iv
23. JAVASCRIPT – String . 137String Properties . 137constructor . 137Length . 138Prototype . 139String Methods . 140charAt() . 142charCodeAt (). 143contact () . 144indexOf () . 145lastIndexOf () . 147localeCompare () . 148match () . 149replace () . 150Search () . 153slice () . 154split () . 155substr (). 156substring () . 157toLocaleLowerCase() . 158toLocaleUppereCase () . 159toLowerCase (). 160toString () . 161toUpperCase () . 162valueOf () . 163String HTML Wrappers . 164anchor() . 165big(). 166blink () . 167bold () . 168fixed () . 168fontColor () . 169fontsize () . 170italics () . 171link () . 172small () . 173strike (). 174sub() . 175sup () . 17624. JAVASCRIPT – Arrays . 178Array Properties . 178constructor . 179length. 180Prototype . 181Array Methods . 182concat () . 184every (). 185filter () . 187forEach () . 190v
indexOf () . 192join () . 195lastIndexOf () . 196map () . 199pop () . 201push () .
This tutorial has been prepared for JavaScript beginners to help them understand the basic functionality of JavaScript to build dynamic web pages and web applications. Prerequisites For this tutorial, it is assumed that the reader have a prior knowledge of HTML coding. It would help if the reader had some prior exposure to object-oriented .File Size: 1014KB