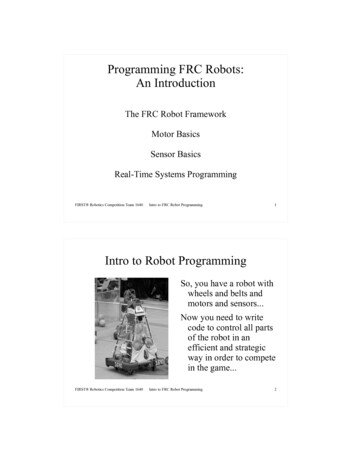
Transcription
Programming FRC Robots:An IntroductionThe FRC Robot FrameworkMotor BasicsSensor BasicsReal-Time Systems ProgrammingFIRST Robotics Competition Team 1640Intro to FRC Robot Programming1Intro to Robot ProgrammingSo, you have a robot withwheels and belts andmotors and sensors.Now you need to writecode to control all partsof the robot in anefficient and strategicway in order to competein the game.FIRST Robotics Competition Team 1640Intro to FRC Robot Programming2
The FRC Robot Framework FRC provides a framework for robot code–It is required to integrate with the Field ManagementSystem (FMS) at competitions–Teams hook in their own code in certain placesDefault code and supported libraries are available inJava, C , and LabVIEWWPI library code provides hardware integration–For motors, motor controllers, pneumatics, sensors,joysticks, and Kinect sensorsFIRST Robotics Competition Team 1640Intro to FRC Robot Programming3Java Robot /first/wpilibj/IterativeRobot.htmlimport edu.wpi.first.wpilibj.IterativeRobot;public class MyRobot extends IterativeRobot {public void robotInit() { }public void disabledInit() { }public void disabledPeriodic() { }public void autonomousInit() { }public void autonomousPeriodic() { }public void teleopInit() { }public void teleopPeriodic() { }// disabled,autonomous,teleop Continuous as well// team-specific methods}FIRST Robotics Competition Team 1640Intro to FRC Robot Programming4
C Robot ass My2011Robot : public IterativeRobot{// data member declarations public:MyRobot(void) { // constructor }void RobotInit() { // initialization }void DisabledInit() { }void AutonomousInit() { }void TeleopInit() { }void DisabledPeriodic() { }void AutonomousPeriodic() { }void TeleopPeriodic() { }// team-specific methods };START ROBOT CLASS(My2011Robot);FIRST Robotics Competition Team 1640Intro to FRC Robot Programming5LabVIEW Robot FrameworkFIRST Robotics Competition Team 1640Intro to FRC Robot Programming6
Motor Basics Motors need control signals to run– Can be simple on/off using a spike (relay) controllerMotor speed controllers give percentage of power–Two types in FRC support libraries: VictorsJaguars–Code refers to the speed controller, not the motor–Speed controller sends signals to the motorFIRST Robotics Competition Team 1640Intro to FRC Robot Programming7Motor Basics: Java Examplesimport edu.wpi.first.wpilibj.Jaguar;;// creating a Jaguar referenceJaguar motor1 new Jaguar(1);;// default digital module, channel 1// setting the desired speed (range of - 1.0 to 1.0)motor1.set(0.5);;import edu.wpi.first.wpilibj.Victor;;// creating a Victor referenceVictor motor2 new Victor(2);;// default digital module, channel 2// setting the desired speed (range of - 1.0 to 1.0)motor2.set(0.25);;import edu.wpi.first.wpilibj.Relay;;// creating a Relay reference, allowing forward direction only// default digital module, channel 1Relay motor3 new Relay(1, kForward);;// setting the motor to on and offmotor3.set(kOn);;motor3.set(kOff);;FIRST Robotics Competition Team 1640Intro to FRC Robot Programming8
Motor Basics: C Examples#include “WPILib.h”;;// creating a Jaguar referenceJaguar *motor1 new Jaguar(1);;// default digital module, channel 1// setting the desired speed (range of - 1.0 to 1.0)motor1- Set(0.5);;// creating a Victor referenceVictor *motor2 new Victor(2);; // default digital module, channel 2// setting the desired speed (range of - 1.0 to 1.0)motor2- Set(0.25);;// creating a Relay reference, allowing forward direction only// default digital module, channel 1Relay *motor3 new Relay(1, kForward);;// setting the motor to on and offmotor3- Set(kOn);;motor3- Set(kOff);;FIRST Robotics Competition Team 1640Intro to FRC Robot Programming9Motor Basics: LabVIEW ExamplesOpening a relay allowingforward and reverseand creating a referenceSetting the relay to “Off”Opening a Jaguarcontrolled motor andcreating a referenceOpening a Victorcontrolled motor andcreating a referenceSetting motor output to 50%FIRST Robotics Competition Team 1640Intro to FRC Robot ProgrammingImage courtesy of Ben Rajcan10
Sensor Basics Sensors provide data about a robot and itsenvironment– The angle of wheels, the distance from an object, etc.Sensors can provide analog or digital data–Analog sensors provide varying voltages–Digital sensors provide on/off dataSupport libraries are provided for both typesFIRST Robotics Competition Team 1640Intro to FRC Robot Programming11Sensor Basics: Java Examplesimport edu.wpi.first.wpilibj.AnalogChannel;// Create a reference to an analog sensor// default analog module, channel 1AnalogChannel sensor1 new AnalogChannel(1);// Get the average voltage from the analog sensordouble voltage sensor1.getAverageVoltage();import edu.wpi.first.wpilibj.DigitalInput;// Create a reference to a digital sensor// default digital module, channel 1DigitalInput sensor2 new DigitalInput(1);// Get the value from the sensorboolean value sensor2.get();FIRST Robotics Competition Team 1640Intro to FRC Robot Programming12
Sensor Basics: C Examples#include AnalogChannel.h // Create a reference to an analog sensor// default analog module, channel 1AnalogChannel *sensor1 new AnalogChannel(1);// Get the average voltage from the analog sensorfloat voltage sensor1- GetAverageVoltage();#include DigitalInput.h // Create a reference to a digital sensor// default digital module, channel 1DigitalInput *sensor2 new DigitalInput(1);// Get the value from the sensorUINT32 value sensor2- Get();FIRST Robotics Competition Team 1640Intro to FRC Robot Programming13Sensor Basics: LabVIEW ExamplesOpening an analogchannel andcreating a referenceGetting the average voltageOpening a digitalchannel andcreating a referenceGetting the true/false valueImage courtesy of Ben RajcanFIRST Robotics Competition Team 1640Intro to FRC Robot Programming14
Joystick BasicsOpening USB joystick ports,creating references, andthen using device referencesUsing joystick axis and button dataFIRST Robotics Competition Team 1640Intro to FRC Robot Programming15Real-Time Systems Programming Real-time system – hardware/software system withreal-time constraints– e.g. deadline for system response after an eventRobots are real-time systems–Driver controls need to be carried out in real-time–Interacting with the world has to be in real-timeAll parts of a real-time system must be time-awareor actively scheduledFIRST Robotics Competition Team 1640Intro to FRC Robot Programming16
Real-Time Constaint: Safety The robot frameworkhas built-in safetyfeatures to ensuresafe robot operation–e.g. motors will beturned off if nosignals arereceived within100ms (defaultvalue)FIRST Robotics Competition Team 1640 Best practice: Makesure to send eachmotor a signal atleast every n:Even if it is to set itto 0 powerSystemSystemSystemSystemSystemIntro to FRC Robot erStation17
How NOT to Freeze Your RobotThreading of multiple processes needs the processesto “play nicely” by having “idle time” built in–Each needs to allow other processes time to run–Putting appropriate wait times in loops is importantBarrierWheelsDriving BridgeArmFIRST Robotics Competition Team 1640MatllBa emengnaIntro to FRC Robot Programming19Summary FRC framework is required for all competition bots Default code and WPI support library is available –Java, C , and LabVIEW are supported–Other languages possible, but not supportedReal-time systems programming involves:–Handling real-time constraints (safety monitors)–Handling multiple “parallel” processes and makingsure they all share CPU time nicelyFIRST Robotics Competition Team 1640Intro to FRC Robot Programming20
FIRST Robotics Competition Team 1640Intro to FRC Robot Programming 3 The FRC Robot Framework FRC provides a framework for robot code –It is required to integrate with the Field Management System (FMS) at competitions –Teams hook in their own code in certain places Default code and su