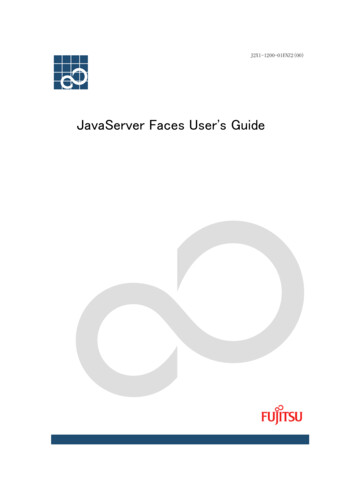
Transcription
J2X1-1200-01ENZ2(00)JavaServer Faces User's Guide
PrefacePurposeThis manual explains how to design and develop business applications when JavaServer Faces is applied to Webapplication development. Readers can get an understanding of the configuration and development of JavaServerFaces applications.Intended ReadersThis manual is intended for application designers and those who implement applications.Readers are assumed to have knowledge of the following:· Creating applications using servlets and JSPsOrganization of This ManualThe manual has the following sections:Overview of JavaServer FacesThis section explains features of JavaServer Faces applications.JavaServer Faces FunctionsThis section explains functions of JavaServer Faces.Development Using JavaServer Faces TagsThis section explains how to develop applications by using the standard tags provided by JavaServerFaces.Development Using UJI TagsThis section explains how to use UJI tags in JavaServer Faces applications.Using Apcoordinator FunctionsThis section explains how to use Apcoordinator functions in JavaServer Faces applications.Related ManualsRead also the following manuals as necessary:JavaServer Faces API ReferenceFor details on JavaServer Faces Java classes and Java interfaces, refer to this manual.JavaServer Faces Definition File ReferenceFor details on coding of the JavaServer Faces definition file (faces-config.xml), refer to this manual.JavaServer Faces Tag ReferenceFor details of the standard tags provided by JavaServer Faces, refer to this manual.Apcoordinator User's GuideFor details on each function of Apcoordinator, refer to this manual.UJI Tag ReferenceFor details of UJI tags, refer to this manual.i
ii
ContentsChapter 1 Overview of JavaServer Faces . 11.1 JavaServer Faces . 1Chapter 2 JavaServer Faces Functions. 32.1 JavaServer Faces Applications and Lifecycle. 32.2 Input-Output Pages and UIComponents. 42.3 Managed Beans . 52.4 Value Binding Expression and Method Binding Expression . 52.5 Event Processing . 72.6 Page Transitions . 82.7 Validators. 92.8 Converters. 9Chapter 3 Development of JavaServer Faces Applications . 113.1 Creating a Managed Bean . 113.2 Creating an Input-Output Page . 123.3 Creating an Event Listener. 133.4 Creating a Validator. 163.5 Creating a Converter. 193.6 Creating a Page Transition Definition . 213.7 Displaying Messages . 223.8 Character Encoding . 24Chapter 4 Development Using JavaServer Faces Tags . 27Chapter 5 Development Using UJI Tags . 335.1 Available UJI Tags . 335.2 Referring to the Properties of Managed Beans. 345.3 Using Validators . 355.4 Using Converters . 375.5 Using Events . 375.5.1 Using action methods .375.5.2 Using valueChangeListener.38Chapter 6 Using Apcoordinator Functions . 416.1 Binary File Send/Receive Function. 416.1.1 Uploading files.416.1.2 Downloading files .436.2 Application Log Function . 436.3 EJB and Web Service Invocation Function. 44Chapter 7 Setting Up the Runtime Environment . 477.1 Runtime File Mapping . 47iii
7.2 Files Required for Execution . 487.3 Web Application Environment Definition File (web.xml). 48Appendix A UIComponents Corresponding to UJI Tags. 51A.1 UIComponent Specifications . 51A.2 UIComponent Class Diagrams . 53Terms . 61iv
Chapter 1 Overview of JavaServer Faces1.1 JavaServer FacesJavaServer Faces is a set of specifications drawn up as JSR-127 by the Java Community Process (JCP). It is anapplication framework for creating Web application user interfaces.JavaServer Faces has the following features:· User interfaces (UIs) developed with JavaServer Faces in a development environment can be used todevelop Web applications through a GUI.· JavaServer Faces supports an "event model" that implements processing on a server at the time that anevent that occurred on a client is received.· Original UIs can be developed using the JavaServer Faces framework.The figure below shows an outline of an application using JavaServer Faces.As shown in the above figure, a JavaServer Faces application consists of the following components:JSPA JSP page can use Apcoordinator UJI tags and JavaServer Faces tags. It defines eventprocessing for buttons on the page and for changes in input data. For each event, theJavaServer Faces runtime engine invokes the corresponding event listener or Java classmethod.Managed beanBean for storing page valuesfaces-config.xmlFile with definitions of the JavaServer Faces environment. The environment that isdefined includes page transitions, events to be invoked, and beans to be used.Validator, value change listener, and action listenerProcessing components that are individually invoked when an applicable request isreceived from a clientThe following sections explain how to develop the components, including a JSP page, managed beans, andfaces-config.xml.1
2
Chapter 2 JavaServer Faces Functions2.1 JavaServer Faces Applications and LifecycleAs with general Java Web applications, JavaServer Faces applications run on servlet containers by using JSP,Java Beans, and event listeners.One of the unique functions of JavaServer Faces is the capability to use built-in and user-created UIComponents,event handlers, validators, and converters defined in an external environment definition file (faces-config.xml).This enables UI and event processing logic to be corrected separately, thereby improving productivity in fixingand adding new functions to an application.The lifecycle of a JavaServer Faces application is explained below. The lifecycle means the processing flow fromthe time that the JavaServer Faces application receives a request until it returns a response.JavaServer Faces processes a request in the following processing flow:The six phases indicated by solid arrows are processed sequentially unless a validation or conversion erroroccurs or event processing occurs. The dotted lines indicate error processing.Restore View phaseThe Restore View phase begins when a request is sent to a server by the clicking of a link or button. In thisphase, JavaServer Faces creates a UIComponent tree for an input-output page and associates event processingand validators with the UIComponents. The created tree is saved to the FacesContext instance.Apply Request Values phaseAfter UIComponents are created in the Restore View phase, the Apply Request Values phase begins to acquireand apply the values of a sent form. If value acquisition or application fails, an error message is set in theFacesContext instance, and the phase changes to the Render Response phase.Process Validations phaseIn this phase, all validators associated with the page to be displayed are processed. If any value is invalid, anerror message is set in the FacesContext instance, and the phase changes to the Render Response phase.Update Model Values phaseIn this phase, the values of the associated model are updated after the values are checked for validity. If3
updating of the model fails, an error message is set in the FacesContext instance, and the phase changes to theRender Response phase.Invoke Application phaseIn this phase, application level events such as page transitions are processed. If an error occurs, an errormessage is set in the FacesContext instance, and the phase changes to the Render Response phase.Render Response phaseIn this phase, a UIComponent tree is returned as a response in a format (e.g., HTML) that can be rendered bythe client.2.2 Input-Output Pages and UIComponentsJavaServer Faces composes a page by combining UIComponents.UIComponents are components such as forms,text fields, and buttons that make up a page.The corresponding JSP extension tags are provided for individualUIComponents so that a page can be created with JSP extension tags written in a JSP file. To display aUIComponent text field, for example, write the h:inputText tag in the JSP file. The JSP file used to create apage is called an input-output page.The standard JSP extension tags provided by JavaServer Faces are classified into two types: tags in theJavaServer Faces component tag library and tags in the JavaServer Faces basic tag library.The component tagsare the tags of user interface components such as text fields and command buttons. The basic tags include thef:view tag that encloses all the JavaServer Faces tags and the tags in which event listeners are set. Thus, thebasic tags specify the configuration and operation of applications and pages.These tag libraries can be used inthe same way as ordinary custom libraries.This product provides a tag library called UJI tags in addition to the standard tags provided byJavaServer Faces. For details on UJI tags, refer to "Development Using UJI Tags".A coding example of an input-output page is shown below.As indicated by comments, the example assigns a value,specifies a validator, and specifies event processing. HTML HEAD TITLE Sample1 /TITLE /HEAD !-- Declares use of the JavaServer Faces tag library. -- %@ taglib uri "http://java.sun.com/jsf/html" prefix "h" % %@ taglib uri "http://java.sun.com/jsf/core" prefix "f" % BODY !-- Writes JavaServer Faces UIComponent tags in f:view. -- f:view H2 Sample /H2 h:form !-- Assigns a value to the myBean userId property. -- h:inputText value "#{myBean.userId}" !-- Specifies the maximum number of characters with avalidator. -- f:validateLength maximum "10"/ /h:inputText !-- Invokes the myBean doAnyAction method when the button isclicked. -- 4
h:commandButton action "#{myBean.doAnyAction}" value "GO"/ /h:form /f:view /BODY /HTML The UIComponents in the above coding are converted into HTML by the renderer prepared in advance, and theresulting display is as shown below.UIComponents and renderers used to display UIComponents are designed separately in JavaServerFaces.Accordingly, simply changing renderers enables UIComponents to be displayed in differentmodes.Renderers are created so that they display UIComponents in the appropriate formats for individualclients.In JavaServer Faces, Renderers that display UIComponent in HTML are prepared in advance.For details on how to create input-output pages, refer to "Creating an Input-Output Page".2.3 Managed BeansNormally, JavaServer Faces applications store values to be displayed and data to be sent, while associatingmanaged bean properties with UIComponents.Managed beans can define event processing, validation, andmethods for page transitions.To assign a managed bean value or an event processing method to a UIComponent, specify the managed beanproperty or method using the value binding expression or the method binding expression written in #{ }.Anexample of mapping with an input-output page is shown below.[Example of using managed beans from an input-output page] !-- Specify a property for value and a method for validator. -- h:inputText id "empNo"value "#{EmployeeBean.userNumber}"validator "#{EmployeeBean.validateNumber}" / The procedure for using managed beans is as follows:1. Create a Bean class.2. Define the created Bean as a managed bean in the JavaServer Faces definition file (faces-config.xml).3. Specify the property names and method names assigned to UIComponents on an input-output page.For details on how to create and use managed beans, refer to "Creating a Managed Bean".2.4 Value Binding Expression and Method BindingExpressionThis section explains the value binding expression and the method binding expression.The value binding expression is a notation method for associating a page with a property of a managed bean.The method binding expression is a notation method for associating a page with a method of a managed bean.Both expressions are mostly used for tag attributes.As in "#{mybean.myprop}", the value binding expression and the method binding expression must begin with"#{" and end with "}".5
Value binding expressionWrite a managed bean identifier and property name by concatenating them with "." (dot) in "#{ }".If beans are nested, as many beans as the number of nested beans can be concatenated using "."A sample JSP page is shown below. f:view !-- Displaying the myprop property of the managed bean, whichis defined with the name mybean -- h:outputText value "#{mybean.myprop}" / !-- Displaying the myprop property of mybean3, while mybean1,mybean2, and mybean3 are nested -- h:outputText value "#{mybean1.mybean2.mybean3.myprop}" / /f:view If the property is an array, java.util.List, java.util.Map, refer to the value by using "[]". f:view !-- If the myArray property is an array, display the 0th elementof the array. -- h:outputText value "#{mybean.myArray[0]}" / !-- If the myList property is of the java.util.List type, displaythe 3rd element of the list. -- h:outputText value "#{mybean.myList[3]}" / !-- If the myMap property is of the java.util.Map type, displaythe value corresponding to "mykey". -- h:outputText value "#{mybean.myMap['mykey']}" / /f:view Method binding expressionWrite a managed bean identifier and method name by concatenating them with "." (dot) in "#{ }".As with referencing of values, if beans are nested, as many beans as the number of nested beans can beconcatenated using "."A sample JSP page is shown below. f:view h:form !-- Use the validate method of the managed bean defined withthe name mybean to verify the value. -- h:inputText value "#{mybean.myprop}"validator "#{mybean.validate}" / /h:form /f:view 6
Reserved variablesThe following reserved variables can be used in value binding expressions and method binding expressions.Variable nameTypeExplanationjava.util.MapCollection of all application scopevariablescookiejava.util.MapCollection of cookies in a request.The Map key is a cookie name, andthe value is .FacesContext class instanceheaderjava.util.MapCollection of all request headers.Individual values in Map are of thejava.lang.String type.headerValuesjava.util.MapCollection of all request headers.Individual values are arrays of thejava.lang.String type.initParamjava.util.MapCollection of applicationinitialization parametersparamjava.util.MapCollection of request parameters.The value of the first requestparameter of those corresponding toindividual keys is stored.paramValuesjava.util.MapCollection of request parameters.The request parametercorresponding to each key is storedin the java.lang.String array.requestScopejava.util.MapCollection of all request scopevariablessessionScopejava.util.MapCollection of all session javax.faces.component.UIViewRoot class instance corresponding tothe page being displayedapplicationScopeAn example of use of a reserved variable is shown below. f:view !-- Display for the request parameter corresponding to key"paramKey" -- h:outputText value "#{param['paramKey']}" / /f:view 2.5 Event ProcessingJavaServer Faces applications handle three types of events: ActionEvent, ValueChangeEvent, andPhaseEvent.An event listener model of JavaServer Faces receives an event class using the listener interface and processesevents occurring in UIComponents.7
JavaServer Faces supports the following three types of events:· ActionEvent occurs at such times as when the user clicks a button or hyperlink component.This eventoccurs in the Invoke Application or Apply Request Values phase.· ValueChangeEvent occurs when the user changes the value of a UIComponent. This event does notoccur at the time that a validation error is detected.This event occurs in the Process Validations or ApplyRequest Values phase.· PhaseEvent can perform processing by causing an interrupt before or after a JavaServer Faces lifecyclephase.This event can be used for unified preprocessing and postprocessing of a business logic.Applications can process these events as follows:· The application creates an event listener and assigns it to a UIComponent by using thef:valueChangeListener tag or f:actionListener tag on an input-output page.In the case of thePhaseEvent listener, the application defines it in the JavaServer Faces definition file (faces-config.xml).· The application uses the managed bean method to create event processing and specifies themethod-binding expression in the appropriate attribute of a UIComponent.For details on how to create and invoke individual events, refer to "Creating an Event Listener".2.6 Page TransitionsGenerally, each Web application, such as a JavaServer Faces application, consists of multiple input-outputpages.Therefore, transitions between the pages must be defined and implemented when an application iscreated.JavaServer Faces applications can easily define page transitions and which transition is executed according toevent processing.Page transition is a series of rules to select the transition destination page when the user clicksa button or hyperlink.Define these rules by using the navigation-rule tag in the JavaServer Faces definitionfile (faces-config.xml) written in XML.Write transition source names, action results, and transition destinationpage names for the rules.A coding example is shown below.[Coding example of the JavaServer Faces definition file (faces-config.xml)] faces-config . navigation-rule !-- Write the transition source JSP name. -- from-view-id /input.jsp /from-view-id !-- Change the transition destination according to the actionresult. -- navigation-case from-outcome success /from-outcome to-view-id /result.jsp /to-view-id /navigation-case navigation-case from-outcome fail /from-outcome to-view-id /input.jsp /to-view-id /navigation-case /navigation-rule . /faces-config 8
The following methods can be used to specify rules for selections according to the type of user operation:· Specify the character string showing the action result for the value of the action attribute of aUIComponent.· Specify the method that returns the action result by using the method binding expression as the value ofthe action attribute of a UIComponent.For details on each method, refer to "Creating a Page Transition Definition".2.7 ValidatorsJavaServer Faces supports a method for verifying data in UIComponents such as text fields in which users canenter data.Data in a UIComponent is verified before updated data is set in properties of the managed beanallocated to the UIComponent. If verification fails, properties of the managed bean are not updated.JavaServer Faces defines the classes of a series of validators that implement standard data checks. In addition,it provides basic tags corresponding to the standard validators.Developers of input-output pages can register validators in UIComponents. The standard validators provided byJavaServer Faces are available. In addition, custom validators can be created and used.The procedure for using a validator is as follows:1. Decide the validator to be used.- Use a standard validator if it satisfies the function requirements.- Otherwise, create and use a custom validator.2. When using a custom validator, define it in the JavaServer Faces definition file (faces-config.xml).3. Register the validator in a UIComponent on an input-output page.For details on the following points of validator development, refer to "Creating a Validator":· Standard validators provided by JavaServer Faces· Creating and registering a custom validator· Specifying a validator on an input-output page2.8 ConvertersWhen UIComponents are assigned to Bean properties, the data handled by JavaServer Faces is represented inthe following two formats:· Format in which data is represented in data types, such as int and long, within a model· Format in which data is represented in such a way that users can read, enter, and correct itWhen a value on an input-output page is updated or when a Bean data value is changed by event processing,conversion processing between the two formats is performed.Data is automatically converted in standard modeunless otherwise specified. However, data may need to be converted to a specified format other than thestandard format.For this reason, JavaServer Faces enables converters to be registered in UIComponents.Theconverters provided by JavaServer Faces can be used as well as custom converters created by users.The procedure for using a converter is as follows:1. Decide the converter to be used.- Use a standard converter if it satisfies the requirements.- Otherwise, create and use a custom converter.2. Register the converter in a UIComponent on an input-output page.For details on the following points of converter development, refer to "Creating a Converter":· Standard converters provided by JavaServer Faces· Creating a custom converter· Specifying a converter on an input-output page9
10
Chapter 3 Development of JavaServer FacesApplicationsThis section explains a standard development method using JavaServer Faces. An event model application hasthe following basic components:Managed bean (model)Input-output page (JSP)Event listener (business logic)ValidatorConverterPage transition definition (XML)Subsequent sections explain how to create these components.3.1 Creating a Managed BeanCreate a Bean for storing values to be displayed or data to be sent.The Bean has values in its properties, and it also has accessor methods (Setter and Getter). In addition, it canimplement event listeners.For details, refer to "Creating an Input-Output Page". Registering the Bean as a managed bean enables thefollowing types of operations through an input-output page:· Allocating input and output data to Bean properties· Allocating an input data validator to a Bean method· Allocating UIComponent event processing to a Bean methodIn the following example, a listener that operates when a value is updated and a listener that operates when abutton is clicked are implemented.package mypkg;import javax.faces.event.ActionEvent;import javax.faces.event.ValueChangeEvent;public class MyBean {protected String message "My Message";public String getMessage() {return this.message;}public void setMessage(String message) {this.message message;}public String getIdForNextPage() {// Acquires a value for a page transition.return "success";11
}public void messageChanged(ValueChangeEvent event) {// Coding of the processing executed at the time that the messageproperty value is updated}public void pushed(ActionEvent e) {// Coding of the processing executed at the time that an associatedbutton is clicked}}Specify the name and scope of the created model in the JavaServer Faces definition file (faces-config.xml). In thefollowing example, the mypkg.MyBean class is registered with the name myBean, and the scope is session. faces-config managed-bean managed-bean-name myBean /managed-bean-name managed-bean-class mypkg.MyBean /managed-bean-class managed-bean-scope session /managed-bean-scope /managed-bean /faces-config Specify the scope by selecting none, application, session, or request.If no scope is specified, none isassumed, and the Bean is not saved to any scope, i.e., the Bean exists only while it is referenced.3.2 Creating an Input-Output PageTo use JavaServer Faces UIComponents on a JSP page, use the JavaServer Faces component tag library andJavaServer Faces basic tag library.The component tags are user interface component tags. The basic tags specifyapplication and page structures and operations.These tag libraries can be used in the same way as generalcustom tag libraries.To use these tags on an input-output page, the beginning of the page must have a declaration as shown below. %@ taglib uri "http://java.sun.com/jsf/html" prefix "h" % %@ taglib uri "http://java.sun.com/jsf/core" prefix "f" % The uri attribute identifies a tag library. The prefix attribute identifies the tag library to which a tagbelongs.The above example specifies that a component tag be preceded by h: and that a basic tag be preceded byf: .The JavaServer Faces tags represent a tree of UIComponents. The root of the tree is the UIViewRootcomponent, which is represented by the f:view tag. f:view Other JavaServer Faces tags /f:view HTML and JSP tags can be written outside the f:view tags, but JavaServer Faces tags must be written within12
the scope enclosed by the f:view tags.And, f:view corresponding to one screen is only one.For details on the standard tags provided by JavaServer Faces and the display resulting from each tag, refer to"Development Using JavaServer Faces Tags".Using value binding expressions and method binding expressions, UIComponent tags can allocate Beanproperties to UIComponents and specify event processing, validators, and converters for them. An example isshown below. Note that the example provides comments with !-- -- for explanations, but no comment canactually be written between the and of a tag. f:view h:form h:inputText !-- Assigns the myBean message property value to theh:inputText value attribute. -- value "#{myBean.message}" !-- Invokes the messageChanged method of myBean when thevalue is changed. -- valueChangeListener "#{myBean.messageChanged}" !-- Specifies the maximum number of characters with avalidator. -- f:validateLength maximum "10"/ /h:inputText h:commandButton !-- Invokes the getIdForNextPage of myBean when the sendbutton is clicked. The resulting page transition is based on thereturn value. -- action "#{myBean.getIdForNextPage}"value "Send" !-- Invokes the pushed method of myBean when the send buttonis clicked. -- actionListener "#{myBean.pushed}" / /h:form /f:view For details on how to specify the following processing definitions, refer to the respective sections indicated nextto them:····Event listener - Creating an Event ListenerConverter - Creating a ConverterValidator - Creating a ValidatorPage transition - Creating a Page Transition Definition3.3 Creating an Event ListenerThere are three types of events: ActionEvent, ValueChangeEvent, and PhaseEvent.ActionEventTheA
· JavaServer Faces supports an "event model" that implements processing on a server at the time that an event that occurred on a client is received. · Original UIs can be developed using the JavaServer Faces framework. The figure below shows an outline of an application using JavaServer Faces.