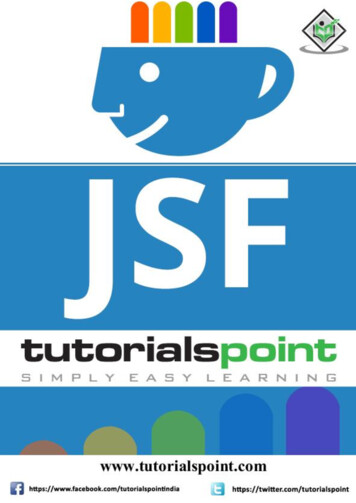
Transcription
JSF
JSFAbout the TutorialJava Server Faces (JSF) is a Java-based web application framework intended to simplifydevelopment integration of web-based user interfaces. JavaServer Faces is a standardizeddisplay technology, which was formalized in a specification through the Java CommunityProcess.This tutorial will teach you basic JSF concepts and will also take you through variousadvance concepts related to JSF framework.AudienceThis tutorial has been prepared for the beginners to help them understand basic JSFprogramming. After completing this tutorial, you will find yourself at a moderate level ofexpertise in JSF programming from where you can take yourself to the next levels.PrerequisitesBefore proceeding with this tutorial you should have a basic understanding of Javaprogramming language, text editor, and execution of programs etc. Since we are going todevelop web-based applications using JSF, it will be good if you have an understanding ofother web technologies such as HTML, CSS, AJAX, etc.Copyright & Disclaimer Copyright 2017 by Tutorials Point (I) Pvt. Ltd.All the content and graphics published in this e-book are the property of Tutorials Point (I)Pvt. Ltd. The user of this e-book is prohibited to reuse, retain, copy, distribute or republishany contents or a part of contents of this e-book in any manner without written consentof the publisher.We strive to update the contents of our website and tutorials as timely and as precisely aspossible, however, the contents may contain inaccuracies or errors. Tutorials Point (I) Pvt.Ltd. provides no guarantee regarding the accuracy, timeliness or completeness of ourwebsite or its contents including this tutorial. If you discover any errors on our website orin this tutorial, please notify us at contact@tutorialspoint.comi
JSFTable of ContentsAbout the Tutorial . iAudience . iPrerequisites . iCopyright & Disclaimer. iTable of Contents . ii1.JSF – OVERVIEW . 1What is JSF? . 1Benefits . 1JSF UI Component Model . 12.JSF – ENVIRONMENTAL SETUP . 2System Requirement . 2Environment Setup for JSF Application Development . 23.JSF – ARCHITECTURE . 10What is MVC Design Pattern?. 10JSF Architecture . 104.JSF – LIFE CYCLE. 12Phase 1: Restore view . 12Phase 2: Apply request values . 12Phase 3: Process validation . 13Phase 4: Update model values . 13Phase 5: Invoke application . 13Phase 6: Render response . 13ii
JSF5.JSF – FIRST APPLICATION . 14Create Project . 14Add JSF Capability to Project . 15Prepare Eclipse Project . 17Import Project in Eclipse . 19Configure Faces Servlet in web.xml . 19Create a Managed Bean . 21Create a JSF page. 21Build the Project. 22Deploy WAR file . 23Run Application . 236.JSF – MANAGED BEANS . 25@ManagedBean Annotation . 26Scope Annotations . 26@ManagedProperty Annotation . 267.JSF – PAGE NAVIGATION. 30Implicit Navigation . 30Auto Navigation in JSF Page . 30Auto Navigation in Managed Bean . 31Conditional Navigation . 32Resolving Navigation Based on from-action . 34Forward vs Redirect . 368.JSF – BASIC TAGS . 46h:inputText . 48h:inputSecret . 55h:inputTextarea . 60iii
JSFh:inputHidden . 66h:selectBooleanCheckbox . 72h:selectManyCheckbox . 79h:selectOneRadio . 88h:selectOneListbox . 97h:selectManyListbox . 106h:selectOneMenu . 115h:outputText . 124h:outputFormat . 126h:graphicImage . 129h:outputStylesheet . 133h:outputScript . 138h:commandButton . 143h:Link . 148h:commandLink. 155h:outputLink . 161h:panelGrid . 168h:message . 179h:messages. 185f:param . 190f:attribute . 196h:setPropertyActionListener . 2009.JSF – FACELET TAGS . 205Template Tags . 206Creating Template . 207ui:param Tag . 214Parameter to Section of a Template . 215iv
JSFParameter to Template . 215Custom Tag . 219ui:remove Tag . 22610. JSF - CONVERTOR TAGS . 229f:convertNumber. 229f:convertDateTime . 233Custom Converter . 23811. JSF - VALIDATOR TAGS . 247f:validateLength . 248f:validateLongRange . 252f:validateDoubleRange . 255f:validateRegex . 259Custom Validator . 26412. JSF – DATATABLE . 273Display DataTable . 274Add Data to DataTable . 285Edit Data of a DataTable . 290Delete Data of a DataTable . 294Using DataModel in a DataTable . 29713. JSF – COMPOSITE COMPONENTS . 301Define Custom Component . 301Use Custom Component . 30314. JSF – AJAX . 309v
JSF15. JSF – EVENT HANDLING . 315valueChangeListener . 316actionListener . 324Application Events . 33116. JSF - JDBC INTEGRATION. 33917. JSF - SPRING INTEGRATION. 34918. JSF - EXPRESSION LANGUAGE . 36019. JSF - INTERNATIONALIZATION . 364vi
1. JSF – OVERVIEWJSFWhat is JSF?JavaServer Faces (JSF) is a MVC web framework that simplifies the construction of UserInterfaces (UI) for server-based applications using reusable UI components in a page. JSFprovides a facility to connect UI widgets with data sources and to server-side event handlers.The JSF specification defines a set of standard UI components and provides an ApplicationProgramming Interface (API) for developing components. JSF enables the reuse and extensionof the existing standard UI components.BenefitsJSF reduces the effort in creating and maintaining applications, which will run on a Javaapplication server and will render application UI on to a target client. JSF facilitates Webapplication development by Providing reusable UI components Making easy data transfer between UI components Managing UI state across multiple server requests Enabling implementation of custom components Wiring client-side event to server-side application codeJSF UI Component ModelJSF provides the developers with the capability to create Web application from collections ofUI components that can render themselves in different ways for multiple client types (forexample - HTML browser, wireless, or WAP device).JSF provides Core library A set of base UI components - standard HTML input elements Extension of the base UI components to create additional UI component libraries or toextend existing components Multiple rendering capabilities that enable JSF UI components to render themselvesdifferently depending on the client types7
2. JSF – ENVIRONMENTAL SETUPJSFThis chapter will guide you on how to prepare a development environment to start your workwith JSF Framework. You will learn how to setup JDK, Eclipse, Maven, and Tomcat on yourmachine before you set up JSF Framework.System RequirementJSF requires JDK 1.5 or higher so the very first requirement is to have JDK installed on yourmachine.JDK1.5 or aboveMemoryNo minimum requirementDisk SpaceNo minimum requirementOperating SystemNo minimum requirementEnvironment Setup for JSF Application DevelopmentFollow the given steps to setup your environment to start with JSF application development.Step 1: Verify Java installation on your machine.Open console and execute the following Java command.OSTaskCommandWindowsOpen Command Consolec:\ java -versionLinuxOpen Command Terminal java -versionMacOpen Terminalmachine: joseph java -version8
JSFLet's verify the output for all the operating systems:OSGenerated Outputjava version "1.6.0 21"WindowsJava(TM) SE Runtime Environment (build 1.6.0 21-b07)Java HotSpot(TM) Client VM (build 17.0-b17, mixed mode,sharing)java version "1.6.0 21"Java(TM) SE Runtime Environment (build 1.6.0 21-b07)LinuxJava HotSpot(TM) Client VM (build 17.0-b17, mixed mode,sharing)java version "1.6.0 21"Java(TM) SE Runtime Environment (build 1.6.0 21-b07)MacJava HotSpot(TM)64-Bit Server VM (build 17.0-b17, mixedmode, sharing)Step 2: Set Up Java Development Kit (JDK).If you do not have Java installed then you can install the Java Software Development Kit(SDK) from Oracle's Java site: Java SE Downloads. You will find instructions for installing JDKin downloaded files, follow the given instructions to install and configure the setup. Finally,set PATH and JAVA HOME environment variables to refer to the directory that contains javaand javac, typically java install dir/bin and java install dir respectively.Set the JAVA HOME environment variable to point to the base directory location where Javais installed on your machine.For example OSOutput9
JSFWindowsSet the environment variable JAVA HOME to C:\Program Files\Java\jdk1.6.0 21LinuxExport JAVA HOME /usr/local/java-currentMacExport JAVA HOME /Library/Java/HomeAppend Java compiler location to System Path.OSWindowsOutputAppend the string ;%JAVA HOME%\bin to the end of the system variable, Path.LinuxExport PATH PATH: JAVA HOME/bin/MacNot requiredAlternatively, if you use an Integrated Development Environment (IDE) like Borland JBuilder,Eclipse, IntelliJ IDEA, or Sun ONE Studio, compile and run a simple program to confirm thatthe IDE knows where you installed Java. Otherwise, carry out a proper setup according to thegiven document of the IDE.Step 3: Set Up Eclipse IDE.All the examples in this tutorial have been written using Eclipse IDE. Hence, we suggest youshould have the latest version of Eclipse installed on your machine based on your operatingsystem.To install Eclipse IDE, download the latest Eclipse binaries with WTP supportfrom http://www.eclipse.org/downloads/. Once you download the installation, unpack thebinary distribution into a convenient location. For example, in C:\eclipse on Windows, or/usr/local/eclipse on Linux/Unix and finally set PATH variable appropriately.Eclipse can be started by executing the following commands on Windows machine, or you cansimply double-click on eclipse.exe%C:\eclipse\eclipse.exeEclipse can be started by executing the following commands on Unix (Solaris, Linux, etc.)machine: /usr/local/eclipse/eclipse10
JSFAfter a successful startup, if everything is fine then it will display the following result.11
JSF*Note: Install m2eclipse plugin to eclipse using the following eclipse software update sitem2eclipse Plugin - http://m2eclipse.sonatype.org/update/This plugin enables the developers to run maven commands within eclipse withembedded/external maven installation.Step 4: Download Maven archive.Download Maven 2.2.1 from ive tep 5: Extract the Maven archive.Extract the archive to the directory you wish to install Maven 2.2.1. The subdirectory apachemaven-2.2.1 will be created from the archive.OSWindowsLocation (can be different based on your installation)C:\Program Files\Apache Software e-mavenMac/usr/local/apache-mavenStep 6: Set Maven environment variables.Add M2 HOME, M2, MAVEN OPTS to environment variables.OSWindowsOutputSet the environment variables using system properties.12
JSFM2 HOME C:\Program Files\Apache Software Foundation\apachemaven-2.2.1M2 %M2 HOME%\binMAVEN OPTS -Xms256m -Xmx512mOpen command terminal and set environment variables.export M2 HOME ort M2 %M2 HOME%\binexport MAVEN OPTS -Xms256m -Xmx512mOpen command terminal and set environment variables.export M2 HOME t M2 %M2 HOME%\binexport MAVEN OPTS -Xms256m -Xmx512mStep 7: Add Maven bin directory location to system path.Now append M2 variable to System Path.OSWindowsOutputAppend the string ;%M2% to the end of the system variable, Path.Linuxexport PATH M2: PATHMacexport PATH M2: PATHStep 8: Verify Maven installation.Open console, execute the following mvn command.13
JSFOSTaskCommandWindowsOpen Command Consolec:\ mvn --versionLinuxOpen Command Terminal mvn --versionMacOpen Terminalmachine: joseph mvn --versionFinally, verify the output of the above commands, which should be as shown in the followingtable.OSOutputApache Maven 2.2.1 (r801777; 2009-08-07 00:46:01 0530)WindowsJava version: 1.6.0 21Java home: C:\Program Files\Java\jdk1.6.0 21\jreApache Maven 2.2.1 (r801777; 2009-08-07 00:46:01 0530)Java version: 1.6.0 21LinuxJava home: C:\Program Files\Java\jdk1.6.0 21\jreApache Maven 2.2.1 (r801777; 2009-08-07 00:46:01 0530)Java version: 1.6.0 21MacJava home: C:\Program Files\Java\jdk1.6.0 21\jreStep 9: Set Up Apache Tomcat.You can download the latest version of Tomcat from http://tomcat.apache.org/. Once youdownload the installation, unpack the binary distribution into a convenient location. Forexample, in C:\apache-tomcat-6.0.33 on Windows, or /usr/local/apache-tomcat-6.0.33 onLinux/Unix and set CATALINA HOME environment variable pointing to the installationlocations.Tomcat can be started by executing the following commands on Windows machine, or youcan simply double-click on startup.bat14
JSF%CATALINA \startup.batTomcat can be started by executing the following commands on Unix (Solaris, Linux, etc.)machine. CATALINA 33/bin/startup.shAfter a successful startup, the default web applications included with Tomcat will be availableby visiting http://localhost:8080/. If everything is fine, then it will display the followingresult.15
JSFFurther information about configuring and running Tomcat can be found in the documentationincluded here, as well as on the Tomcat web site: http://tomcat.apache.orgTomcat can be stopped by executing the following commands on Windows machine.%CATALINA utdownTomcat can be stopped by executing the following commands on Unix (Solaris, Linux, etc.)machine. CATALINA .29/bin/shutdown.sh16
3. JSF – ARCHITECTUREJSFJSF technology is a framework for developing, building server-side User Interface Componentsand using them in a web application. JSF technology is based on the Model View Controller(MVC) architecture for separating logic from presentation.What is MVC Design Pattern?MVC design pattern designs an application using three separate modules:ModuleDescriptionModelCarries Data and loginViewShows User InterfaceControllerHandles processing of an applicationThe purpose of MVC design pattern is to separate model and presentation enabling developersto focus on their core skills and collaborate more clearly.Web designers have to concentrate only on view layer rather than model and controller layer.Developers can change the code for model and typically need not change view layer.Controllers are used to process user actions. In this process, layer model and views may bechanged.JSF ArchitectureJSF application is similar to any other Java technology-based web application; it runs in aJava servlet container, and contains JavaBeans components as models containing application-specific functionality anddata17
JSF A custom tag library for representing event handlers and validators A custom tag library for rendering UI components UI components represented as stateful objects on the server Server-side helper classes Validators, event handlers, and navigation handlers Application configuration resource file for configuring application resourcesThere are controllers which can be used to perform user actions. UI can be created by webpage authors and the business logic can be utilized by managed beans.JSF provides several mechanisms for rendering an individual component. It is up to the webpage designer to pick the desired representation, and the application developer doesn't needto know which mechanism was used to render a JSF UI component.18
4. JSF – LIFE CYCLEJSFJSF application life cycle consists of six phases which are as follows Restore view phase Apply request values phase; process events Process validations phase; process events Update model values phase; process events Invoke application phase; process events Render response phaseThe six phases show the order in which JSF processes a form. The list shows the phases intheir likely order of execution with event processing at each phase.Phase 1: Restore viewJSF begins the restore view phase as soon as a link or a button is clicked and JSF receives arequest.During this phase, JSF builds the view, wires event handlers and validators to UI componentsand saves the view in the FacesContext instance. The FacesContext instance will now containall the information required to process a request.Phase 2: Apply request valuesAfter the component tree is created/restored, each component in the component tree usesthe decode method to extract its new value from the request parameters. Component storesthis value. If the conversion fails, an error message is generated and queued on FacesContext.19
JSFThis message will be displayed during the render response phase, along with any validationerrors.If any decode methods event listeners called renderResponse on the current FacesContextinstance, the JSF moves to the render response phase.Phase 3: Process validationDuring this phase, JSF processes all validators registered on the component tree. It examinesthe component attribute rules for the validation and compares these rules to the local valuestored for the component.If the local value is invalid, JSF adds an error message to the FacesContext instance, and thelife cycle advances to the render response phase and displays the same page again with theerror message.Phase 4: Update model valuesAfter the JSF checks that the data is valid, it walks over the component tr
JavaServer Faces(JSF) is a MVC web framework that simplifies the construction of User Interfaces (UI) for server-based applications using reusable UI components in a page. JSF provides a facility to connect UI widgets with data sources and to server-side event handlers.