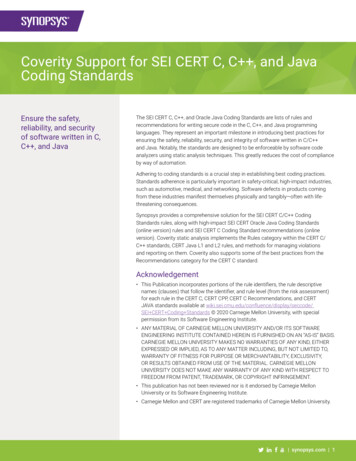
Transcription
Coverity Support for SEI CERT C, C , and JavaCoding StandardsEnsure the safety,reliability, and securityof software written in C,C , and JavaThe SEI CERT C, C , and Oracle Java Coding Standards are lists of rules andrecommendations for writing secure code in the C, C , and Java programminglanguages. They represent an important milestone in introducing best practices forensuring the safety, reliability, security, and integrity of software written in C/C and Java. Notably, the standards are designed to be enforceable by software codeanalyzers using static analysis techniques. This greatly reduces the cost of complianceby way of automation.Adhering to coding standards is a crucial step in establishing best coding practices.Standards adherence is particularly important in safety-critical, high-impact industries,such as automotive, medical, and networking. Software defects in products comingfrom these industries manifest themselves physically and tangibly—often with lifethreatening consequences.Synopsys provides a comprehensive solution for the SEI CERT C/C CodingStandards rules, along with high-impact SEI CERT Oracle Java Coding Standards(online version) rules and SEI CERT C Coding Standard recommendations (onlineversion). Coverity static analysis implements the Rules category within the CERT C/C standards, CERT Java L1 and L2 rules, and methods for managing violationsand reporting on them. Coverity also supports some of the best practices from theRecommendations category for the CERT C standard.Acknowledgement This Publication incorporates portions of the rule identifiers, the rule descriptivenames (clauses) that follow the identifier, and rule level (from the risk assessment)for each rule in the CERT C, CERT CPP, CERT C Recommendations, and CERTJAVA standards available at wiki.sei.cmu.edu/confluence/display/seccode/SEI CERT Coding Standards 2020 Carnegie Mellon University, with specialpermission from its Software Engineering Institute. ANY MATERIAL OF CARNEGIE MELLON UNIVERSITY AND/OR ITS SOFTWAREENGINEERING INSTITUTE CONTAINED HEREIN IS FURNISHED ON AN “AS-IS” BASIS.CARNEGIE MELLON UNIVERSITY MAKES NO WARRANTIES OF ANY KIND, EITHEREXPRESSED OR IMPLIED, AS TO ANY MATTER INCLUDING, BUT NOT LIMITED TO,WARRANTY OF FITNESS FOR PURPOSE OR MERCHANTABILITY, EXCLUSIVITY,OR RESULTS OBTAINED FROM USE OF THE MATERIAL. CARNEGIE MELLONUNIVERSITY DOES NOT MAKE ANY WARRANTY OF ANY KIND WITH RESPECT TOFREEDOM FROM PATENT, TRADEMARK, OR COPYRIGHT INFRINGEMENT. This publication has not been reviewed nor is it endorsed by Carnegie MellonUniversity or its Software Engineering Institute. Carnegie Mellon and CERT are registered trademarks of Carnegie Mellon University. synopsys.com 1
The “Level” column in the “supported rules” and “supported recommendations” tables below indicates the severity, remediation cost, andlikelihood of each vulnerability Level 1 vulnerabilities are the most severe, the least expensive to repair, and/or the most likely to be foundin code Meanwhile, Level 3 vulnerabilities are the least severe, the most expensive to repair, and/or the least likely to be found in code.SEI CERT C Coding Standard (2016 Edition)The SEI CERT C Coding Standard was developed specifically for the following versions of the C language: ISO/IEC 9899:2011: Information Technology—Programming Languages—C, 3rd ed ISO/IEC 9899:2011/Cor 1:2012: Information Technology—Programming Languages—C Technical Corrigendum 1These versions are commonly referred to as the C11 standard The CERT C rules may also be applied to earlier versions of the C language,such as C99The 2016 edition of the CERT C standard contains 99 coding rules There are additional CERT C rules available on the CERT Secure Codingwiki, bringing the total number of rules to 120 as of July 10, 2020 The CERT Secure Coding wiki for C is play/c/The SEI CERT C Coding Standard (2016 Edition) is vices/secure-coding-downloadcfmThe CERT C wiki also documents 185 recommendations and two platform-specific annexes (POSIX and Windows) The recommendationsand annexes are not part of the core secure coding standard and may be useful for projects with stricter requirements.CERT C rule coverageRulesSectionAllSupportedAll115121*% 7887.5POS*1417*82.4WIN010.0As of July 10, 2020, POS33-C is moved to The Void: void POS33-C Do not use vfork(), reducing the total number of POS rules to 16 and the number of CERT C rules to 120. Thus,percentage coverage is calculated as 115/120 95.8%* synopsys.com 2
CERT C supported rulesRuleDescriptionLevelPRE30-CDo not create a universal character name through concatenationL3PRE31-CAvoid side effects in arguments to unsafe macrosL3PRE32-CDo not use preprocessor directives in invocations of function-like macrosL3DCL30-CDeclare objects with appropriate storage durationsL2DCL31-CDeclare identifiers before using themL3DCL36-CDo not declare an identifier with conflicting linkage classificationsL2DCL37-CDo not declare or define a reserved identifierL3DCL38-CUse the correct syntax when declaring a flexible array memberL3DCL39-CAvoid information leakage when passing a structure across a trust boundaryL3DCL40-CDo not create incompatible declarations of the same function or objectL3DCL41-CDo not declare variables inside a switch statement before the first case labelL3EXP30-CDo not depend on order of evaluation for side effectsL2EXP32-CDo not access a volatile object through a nonvolatile referenceL2EXP33-CDo not read uninitialized memoryL1EXP34-CDo not dereference null pointersL1EXP35-CDo not modify objects with temporary lifetimeL3EXP36-CDo not cast pointers into more strictly aligned pointer typesL3EXP37-CCall functions with the correct number and type of argumentsL3EXP39-CDo not access a variable through a pointer of an incompatible typeL3EXP40-CDo not modify constant objectsL3EXP42-CDo not compare padding dataL2EXP43-CAvoid undefined behavior when using restrict-qualified pointersL3EXP44-CDo not rely on side effects in operands to sizeof, Alignof, or GenericL3EXP45-CDo not perform assignments in selection statementsL2EXP46-CDo not use a bitwise operator with a Boolean-like operandL2EXP47-CDo not call va arg with an argument of incorrect typeL2INT30-CEnsure that unsigned integer operations do not wrapL2INT31-CEnsure that integer conversions do not result in lost or misinterpreted dataL2INT32-CEnsure that operations on signed integers do not result in overflowL2INT33-CEnsure that division and modulo operations do not result in divide-by-zero errorsL2INT34-CDo not shift an expression by a negative number of bits or by greater than or equal to the number of bitsthat exist in the operandL3INT35-CUse correct integer precisionsL3INT36-CConverting a pointer to integer or integer to pointerL3FLP30-CDo not use floating-point variables as loop countersL2FLP32-CPrevent or detect domain and range errors in math functionsL2FLP34-CEnsure that floating-point conversions are within range of the new typeL3FLP36-CPreserve precision when converting integral values to floating-point typeL3FLP37-CDo not use object representations to compare floating-point valuesL3ARR30-CDo not form or use out of bounds pointers or array subscriptsL2ARR32-CEnsure size arguments for variable length arrays are in a valid rangeL2ARR36-CDo not subtract or compare two pointers that do not refer to the same arrayL2 synopsys.com 3
RuleDescriptionLevelARR37-CDo not add or subtract an integer to a pointer to a non-array objectL2ARR38-CGuarantee that library functions do not form invalid pointersL1ARR39-CDo not add or subtract a scaled integer to a pointerL2STR30-CDo not attempt to modify string literalsL2STR31-CGuarantee that storage for strings has sufficient space for character data and the null terminatorL1STR32-CDo not pass a non-null-terminated character sequence to a library function that expects a stringL1STR34-CCast characters to unsigned char before converting to larger integer sizesL2STR37-CArguments to character-handling functions must be representable as an unsigned charL3STR38-CDo not confuse narrow and wide character strings and functionsL1MEM30-CDo not access freed memoryL1MEM31-CFree dynamically allocated memory when no longer neededL2MEM33-CAllocate and copy structures containing a flexible array member dynamicallyL3MEM34-COnly free memory allocated dynamicallyL1MEM35-CAllocate sufficient memory for an objectL2MEM36-CDo not modify the alignment of objects by calling realloc()L3FIO30-CExclude user input from format stringsL1FIO32-CDo not perform operations on devices that are only appropriate for filesL3FIO34-CDistinguish between characters read from a file and EOF or WEOFL1FIO37-CDo not assume that fgets() or fgetws() returns a nonempty string when successfulL1FIO38-CDo not copy a FILE objectL3FIO39-CDo not alternately input and output from a stream without an intervening flush or positioning callL2FIO40-CReset strings on fgets() or fgetws() failureL3FIO41-CDo not call getc(), putc(), getwc(), or putwc() with a stream argument that has side effectsL3FIO42-CClose files when they are no longer neededL3FIO44-COnly use values for fsetpos() that are returned from fgetpos()L3FIO45-CAvoid TOCTOU race conditions while accessing filesL2FIO46-CDo not access a closed fileL3FIO47-CUse valid format stringsL2ENV30-CDo not modify the object referenced by the return value of certain functionsL3ENV31-CDo not rely on an environment pointer following an operation that may invalidate itL3ENV32-CAll exit handlers must return normallyL1ENV33-CDo not call system()L1ENV34-CDo not store pointers returned by certain functionsL3SIG30-CCall only asynchronous-safe functions within signal handlersL1SIG31-CDo not access shared objects in signal handlersL2SIG34-CDo not call signal() from within interruptible signal handlersL3SIG35-CDo not return from a computational exception signal handlerL3ERR30-CSet errno to zero before calling a library function known to set errno, and check errno only after thefunction returns a value indicating failureL2ERR32-CDo not rely on indeterminate values of errnoL3ERR33-CDetect and handle standard library errorsL1ERR34-CDetect errors when converting a string to a numberL3 synopsys.com 4
Rule DescriptionLevelCON30-CClean up thread-specific storageL3CON31-CDo not destroy a mutex while it is lockedL3CON32-CPrevent data races when accessing bit-fields from multiple threadsL2CON33-CAvoid race conditions when using library functionsL3CON34-CDeclare objects shared between threads with appropriate storage durationsL3CON35-CAvoid deadlock by locking in predefined orderL3CON36-CWrap functions that can spuriously wake up in a loopL3CON37-CDo not call signal() in a multithreaded programL2CON38-CPreserve thread safety and liveness when using condition variablesL3CON39-CDo not join or detach a thread that was previously joined or detachedL2CON40-CDo not refer to an atomic variable twice in an expressionL2CON41-CWrap functions that can fail spuriously in a loopL3MSC30-CDo not use the rand() function for generating pseudorandom numbersL2MSC32-CProperly seed pseudorandom number generatorsL1MSC33-CDo not pass invalid data to the asctime() functionL1MSC37-CEnsure that control never reaches the end of a non-void functionL2MSC38-CDo not treat a predefined identifier as an object if it might only be implemented as a macroL3MSC39-CDo not call va arg() on a va list that has an indeterminate valueL3MSC40-CDo not violate constraintsL3POS30-CUse the readlink() function properlyL1POS33-C Do not use vfork()L2POS34-CDo not call putenv() with a pointer to an automatic variable as the argumentL2POS35-CAvoid race conditions while checking for the existence of a symbolic linkL1POS36-CObserve correct revocation order while relinquishing privilegesL1POS37-CEnsure that privilege relinquishment is successfulL1POS38-CBeware of race conditions when using fork and file descriptorsL3POS39-CUse the correct byte ordering when transferring data between systemsL1POS44-CDo not use signals to terminate threadsL2POS47-CDo not use threads that can be canceled asynchronouslyL1POS49-CWhen data must be accessed by multiple threads, provide a mutex and guarantee no adjacent data isalso accessedL2POS50-CDeclare objects shared between POSIX threads with appropriate storage durationsL3POS52-CDo not perform operations that can block while holding a POSIX lockL3POS54-CDetect and handle POSIX library errorsL1As of July 10, 2020, POS33-C is moved to The Void: void POS33-C Do not use vfork() synopsys.com 5
CERT C L1 recommendation coverage (online % IG11100.0API11*100.0MSC3475.0*There are 26 total CERT C L1 recommendations. Apart from API10-C, which is incomplete, there are 25 validated recommendations considered in this calculation.CERT C supported recommendations (online version)RecommendationDescriptionLevelPRE09-CDo not replace secure functions with deprecated or obsolescent functionsL1PRE10-CWrap multistatement macros in a do-while loopL1PRE11-CDo not conclude macro definitions with a semicolonL1PRE12-CDo not define unsafe macrosL2DCL11-CUnderstand the type issues associated with variadic functionsL2DCL23-CGuarantee that mutually visible identifiers are uniqueL2EXP05-CDo not cast away a const qualificationL2EXP08-CEnsure pointer arithmetic is used correctlyL2EXP10-CDo not depend on the order of evaluation of subexpressions or the order in which side effectstake placeL2EXP15-CDo not place a semicolon on the same line as an if, for, or while statementL1EXP16-CDo not compare function pointers to constant valuesL2EXP19-CUse braces for the body of an if, for, or while statementL2EXP20-CPerform explicit tests to determine success, true and false, and equalityL1INT02-CUnderstand integer conversion rulesL2INT04-CEnforce limits on integer values originating from tainted sourcesL2INT07-CUse only explicitly signed or unsigned char type for numeric valuesL2INT13-CUse bitwise operators only on unsigned operandsL2INT17-CDefine integer constants in an implementation-independent mannerL1INT18-CEvaluate integer expressions in a larger size before comparing or assigning to that sizeL1ARR00-CUnderstand how arrays workL2ARR01-CDo not apply the sizeof operator to a pointer when taking the size of an arrayL1ARR02-CExplicitly specify array bounds, even if implicitly defined by an initializerL2STR00-CRepresent characters using an appropriate typeL1 synopsys.com 6
RecommendationDescriptionLevelSTR02-CSanitize data passed to complex subsystemsL1STR03-CDo not inadvertently truncate a stringL2STR06-CDo not assume that strtok() leaves the parse string unchangedL1STR07-CUse the bounds-checking interfaces for string manipulationL1STR11-CDo not specify the bound of a character array initialized with a string literalL2MEM00-CAllocate and free memory in the same module, at the same level of abstractionL1MEM01-CStore a new value in pointers immediately after free()L2MEM05-CAvoid large stack allocationsL2FIO01-CBe careful using functions that use file names for identificationL1FIO20-CAvoid unintentional truncation when using fgets() or fgetws()L1ENV01-CDo not make assumptions about the size of an environment variableL1SIG02-CAvoid using signals to implement normal functionalityL1API05-CUse conformant array parametersL1MSC15-CDo not depend on undefined behaviorL1MSC17-CFinish every set of statements associated with a case label with a break statementL1MSC18-CBe careful while handling sensitive data, such as passwords, in program codeL2MSC24-CDo not use deprecated or obsolescent functionsL1 synopsys.com 7
SEI CERT C Coding Standard (2016 Edition)The SEI CERT C Coding Standard was developed specifically for the following version of the C language: ISO/IEC 14882-2014: Information Technology---Programming Languages—C , 4th edThis version is commonly referred to as the C 14 standard.The CERT C rules may also be applied to earlier versions of the C language, such as C 11.The 2016 edition of the CERT C standard contains 83 coding rules.The CERT Secure Coding wiki for C is play/cplusplus/The SEI CERT C Coding Standard (2016 Edition) is es/secure-coding-cpp-download-2016cfmThe CERT C wiki also documents some recommendations. The recommendations are not part of the core secure coding standard.CERT C rule coverageRulesSectionSupportedAll8383All% OP99100.0CON77100.0MSC55100.0CERT C supported rulesRuleDescriptionLevelDCL50-CPPDo not define a C-style variadic functionL1DCL51-CPPDo not declare or define a reserved identifierL3DCL52-CPPNever qualify a reference type with const or volatileL3DCL53-CPPDo not write syntactically ambiguous declarationsL3DCL54-CPPOverload allocation and deallocation functions as a pair in the same scopeL2DCL55-CPPAvoid information leakage when passing a class object across a trust boundaryL3DCL56-CPPAvoid cycles during initialization of static objectsL3DCL57-CPPDo not let exceptions escape from destructors or deallocation functionsL3DCL58-CPPDo not modify the standard namespacesL2DCL59-CPPDo not define an unnamed namespace in a header fileL3DCL60-CPPObey the one-definition ruleL3EXP50-CPPDo not depend on the order of evaluation for side effectsL2 synopsys.com 8
RuleDescriptionLevelEXP51-CPPDo not delete an array through a pointer of the incorrect typeL3EXP52-CPPDo not rely on side effects in unevaluated operandsL3EXP53-CPPDo not read uninitialized memoryL1EXP54-CPPDo not access an object outside of its lifetimeL2EXP55-CPPDo not access a cv-qualified object through a cv-unqualified typeL2EXP56-CPPDo not call a function with a mismatched language linkageL3EXP57-CPPDo not cast or delete pointers to incomplete classesL3EXP58-CPPPass an object of the correct type to va startL3EXP59-CPPUse offsetof() on valid types and membersL3EXP60-CPPDo not pass a nonstandard-layout type object across execution boundariesL1EXP61-CPPA lambda object must not outlive any of its reference captured objectsL2EXP62-CPPDo not access the bits of an object representation that are not part of the object's value representationL2EXP63-CPPDo not rely on the value of a moved-from objectL2INT50-CPPDo not cast to an out-of-range enumeration valueL3CTR50-CPPGuarantee that container indices and iterators are within the valid rangeL2CTR51-CPPUse valid references, pointers, and iterators to reference elements of a containerL2CTR52-CPPGuarantee that library functions do not overflowL1CTR53-CPPUse valid iterator rangesL2CTR54-CPPDo not subtract iterators that do not refer to the same containerL2CTR55-CPPDo not use an additive operator on an iterator if the result would overflowL1CTR56-CPPDo not use pointer arithmetic on polymorphic objectsL2CTR57-CPPProvide a valid ordering predicateL3CTR58-CPPPredicate function objects should not be mutableL3STR50-CPPGuarantee that storage for strings has sufficient space for character data and the null terminatorL1STR51-CPPDo not attempt to create a std::string from a null pointerL1STR52-CPPUse valid references, pointers, and iterators to reference elements of a basic stringL2STR53-CPPRange check element accessL2MEM50-CPPDo not access freed memoryL1MEM51-CPPProperly deallocate dynamically allocated resourcesL1MEM52-CPPDetect and handle memory allocation errorsL1MEM53-CPPExplicitly construct and destruct objects when manually managing object lifetimeL1MEM54-CPPProvide placement new with properly aligned pointers to sufficient storage capacityL1MEM55-CPPHonor replacement dynamic storage management requirementsL1MEM56-CPPDo not store an already-owned pointer value in an unrelated smart pointerL1MEM57-CPPAvoid using default operator new for over-aligned typesL2FIO50-CPPDo not alternately input and output from a file stream without an intervening positioning callL2FIO51-CPPClose files when they are no longer neededL3ERR50-CPPDo not abruptly terminate the programL3ERR51-CPPHandle all exceptionsL3ERR52-CPPDo not use setjmp() or longjmp()L3ERR53-CPPDo not reference base classes or class data members in a constructor or destructor function-try-blockhandlerL3ERR54-CPPCatch handlers should order their parameter types from most derived to least derivedL1ERR55-CPPHonor exception specificationsL2 synopsys.com 9
RuleDescriptionLevelERR56-CPPGuarantee exception safetyL2ERR57-CPPDo not leak resources when handling exceptionsL3ERR58-CPPHandle all exceptions thrown before main() begins executingL2ERR59-CPPDo not throw an exception across execution boundariesL1ERR60-CPPException objects must be nothrow copy constructibleL3ERR61-CPPCatch exceptions by lvalue referenceL3ERR62-CPPDetect errors when converting a string to a numberL3OOP50-CPPDo not invoke virtual functions from constructors or destructorsL3OOP51-CPPDo not slice derived objectsL3OOP52-CPPDo not delete a polymorphic object without a virtual destructorL2OOP53-CPPWrite constructor member initializers in the canonical orderL3OOP54-CPPGracefully handle self-copy assignmentL3OOP55-CPPDo not use pointer-to-member operators to access nonexistent membersL2OOP56-CPPHonor replacement handler requirementsL3OOP57-CPPPrefer special member functions and overloaded operators to C Standard Library functionsL2OOP58-CPPCopy operations must not mutate the source objectL2CON50-CPPDo not destroy a mutex while it is lockedL3CON51-CPPEnsure actively held locks are released on exceptional conditionsL2CON52-CPPPrevent data races when accessing bit-fields from multiple threadsL2CON53-CPPAvoid deadlock by locking in a predefined orderL3CON54-CPPWrap functions that can spuriously wake up in a loopL3CON55-CPPPreserve thread safety and liveness when using condition variablesL3CON56-CPPDo no speculatively lock a non-recursive mutex that is already owned by the calling threadL3MSC50-CPPDo not use std::rand() for generating pseudorandom numbersL2MSC51-CPPEnsure your random number generator is properly seededL1MSC52-CPPValue-returning functions must return a value from all exit pathsL2MSC53-CPPDo not return from a function declared [[noreturn]]L3MSC54-CPPA signal handler must be a plain old functionL2 synopsys.com 10
SEI CERT Oracle Secure Coding Standard for Java (online version)The SEI CERT Oracle Secure Coding Standard for Java was developed primarily referring to the following version of the Java language: The Java Language Specification (3rd Edition)The SEI CERT Oracle Secure Coding Standard for Java focuses on the following Java platforms: The Java Standard Edition 6 Platform (Java SE 6) The Java Standard Edition 7 platform (Java SE 7)The SEI CERT Oracle Secure Coding Standard for Java wiki contains 182 coding rules, of which 38 are Level L1, 48 are Level L2, and 96are Level L3.The CERT Secure Coding wiki for Java is play/java/The SEI CERT Oracle Secure Coding Standard for Java (Standard Edition 6) is re-coding-standard-for-java-9780321803955The SEI CERT Oracle Secure Coding Standard for Java wiki also documents some recommendations. The recommendations are not partof the core secure coding standard.CERT rule coverage (online version)RulesSectionAllSupportedAll114159*% *60.0** There are 38 total CERT Java L1, 48 total CERT Java L2 rules and 96 total CERT Java L3 rules. Apart from five stub L1 rules (SEC08-J, SEC09-J, SEC10-J, JNI03-J, andMSC10-J), and two nonautomated L1 rules (ENV04-J and ENV05-J), there are 31 validated L1 rules considered in this calculation. Similarly, apart from two deprecated L2rules (IDS09-J and SER13-J), three stub L2 rules (IDS15-J, OBJ14-J, and MET13-J), there are 43 validated L2 rules considered in this calculation. Apart from five stub L3 rules(EXP07-J, OBJ12-J, JNI02-J, JNI04-J, and MSC08-J), five deprecated L3 rules (IDS02-J, IDS05-J, IDS10-J, IDS13-J, and FIO11-J), one nonautomated L3 rule (OBJ02-J), there are85 validated L3 rules considered in this calculation. synopsys.com 11
CERT Java supported rules (online version)RuleDescriptionLevelIDS00-JPrevent SQL injectionL1IDS01-JNormalize strings before validating themL1IDS03-JDo not log unsanitized user inputL2IDS06-JExclude unsanitized user input from format stringsL3IDS07-JSanitize untrusted data passed to the Runtimeexec() methodL1IDS08-JSanitize untrusted data included in a regular expressionL3IDS11-JPerform any string modifications before validationL1IDS14-JDo not trust the contents of hidden form fieldsL2IDS16-JPrevent XML InjectionL1IDS17-JPrevent XML External Entity AttacksL2DCL00-JPrevent class initialization cyclesL3DCL01-JDo not reuse public identifiers from the Java Standard LibraryL3DCL02-JDo not modify the collection’s elements during an enhanced for statementL3ERR00-JDo not suppress or ignore checked exceptionsL3ERR02-JPrevent exceptions while logging dataL2ERR04-JDo not complete abruptly from a finally blockL3ERR05-JDo not let checked exceptions escape from a finally blockL3ERR07-JDo not throw RuntimeException, Exception, or ThrowableL2ERR08-JDo not catch NullPointerException or any of its ancestorsL1ERR09-JDo not allow untrusted code to terminate the JVML3EXP00-JDo not ignore values returned by methodsL2EXP01-JDo not use a null in a case where an object is requiredL3EXP02-JDo not use the Object.equals() method to compare two arraysL2EXP03-JDo not use the equality operators when comparing values of boxed primitivesL2EXP06-JExpressions used in assertions must not produce side effectsL3NUM00-JDetect or prevent integer overflowL3NUM02-JEnsure that division and remainder operations do not result in divide-by-zero errorsL2NUM07-JDo not attempt comparisons with NaNL3NUM08-JCheck floating-point inputs for exceptional valuesL3NUM09-JDo not use floating-point variables as loop countersL2NUM10-JDo not construct BigDecimal objects from floating-point literalsL2NUM11-JDo not compare or inspect the string representation of floating-point valuesL2OBJ01-JLimit accessibility of fieldsL1OBJ04-JProvide mutable classes with copy functionality to safely allow passing instances to untrusted codeL2OBJ05-JDo not return references to private mutable class membersL1OBJ09-JCompare classes and not class namesL2OBJ10-JDo not use public static nonfinal fieldsL2OBJ11-JBe wary of letting constructors throw exceptionsL1OBJ13-JEnsure that references to mutable objects are not exposedL1MET00-JValidate method argumentsL2MET01-JNever use assertions to validate method argumentsL2MET02-JDo not use deprecated or obsolete classes or methodsL3 synopsys.com 12
RuleDescriptionLevelMET03-JMethods that perform a security check must be declared private or finalL2MET04-JDo not increase the accessibility of overridden or hidden methodsL2MET05-JEnsure that constructors do not call overridable methodsL2MET06-JDo not invoke overridable methods in clone()L1MET07-JNever declare a class method that hides a method declared in a superclass or superinterfaceL3MET09-JClasses that define an equals() method must also define a hashCode() methodL3MET11-JEnsure that keys used in comparison operations are immutableL3VNA00-JEnsure visibility when accessing shared primitive variablesL2VNA01-JEnsure visibility of shared references to immutable objectsL3VNA02-JEnsure that compound operations on shared variables are atomicL2VNA03-JDo not assume that a group of calls to independently atomic methods is atomicL3VNA05-JEnsure atomicity when reading and writing 64-bit valuesL3LCK00-JUse private final lock objects to synchronize classes that may interact with untrusted codeL3LCK01-JDo not synchronize on objects that may be reusedL2LCK02-JDo not synchronize on the class object returned by getClass()L2LCK03-JDo not synchronize on the intrinsic locks of high-level concurrency objectsL2LCK05-JSynchronize access to static fields that can be modified by untrusted codeL3LCK06-JDo not use an instance lock to protect shared static dataL2LCK08-JEnsure actively held locks are released on exceptional conditionsL2LCK09-JDo not perform operations that can block while holding a lockL3LCK10-JUse a correct form of the double-checked locking idiomL3THI00-JDo not invoke Thread.run()L3THI01-JDo not invoke ThreadGroup methodsL3THI02-JNotify all waiting threads rather than a single threadL3THI03-JAlways invoke wait() and await() methods inside a loopL3THI04-JEnsure that threads performing blocking operations can be terminatedL3THI05-JDo not use Thread.stop() to terminate threadsL3TPS00-JUse thread pools to enable graceful degradation of service during traffic burstsL3TPS03-JEnsure that tasks executing in a thread pool do not fail silentlyL3TPS04-JEnsure ThreadLocal variables are reinitialized when using thread poolsL3TSM00-JDo not override thread-safe methods with methods that are not thread-safeL3TSM02-JDo not use background threads during class initializationL3TSM03-JDo not publish partially initialized objectsL2FIO01-JCreate files with appropriate access permissionsL3FIO02-JDetect and handle file-related errorsL2FIO03-JRemove temporary files before terminationL2FIO05-JDo not expose buffers or their backing arrays methods to untrusted codeL1FIO06-JDo not create multiple buffered wrappers on a single byte or character streamL3FIO08-JDo not expose buffers or their backing
The 2016 edition of the CERT C standard contains 99 coding rules There are additional CERT C rules available on the CERT Secure Coding wiki, bringing the total number of rules to 120 as of July 10, 2020 The CERT Secure Coding wiki for C is here: