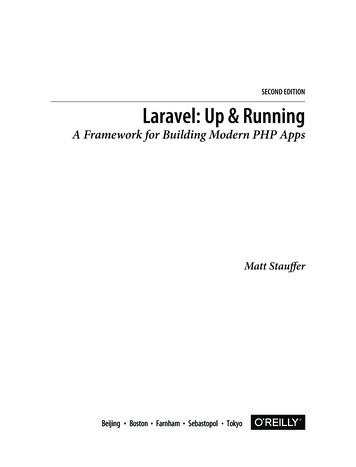
Transcription
SECOND EDITIONLaravel: Up & RunningA Framework for Building Modern PHP AppsMatt StaufferBeijingBoston Farnham SebastopolTokyo
Laravel: Up & Runningby Matt StaufferCopyright 2019 Matt Stauffer. All rights reserved.Printed in the United States of America.Published by O’Reilly Media, Inc., 1005 Gravenstein Highway North, Sebastopol, CA 95472.O’Reilly books may be purchased for educational, business, or sales promotional use. Online editions arealso available for most titles (http://oreilly.com). For more information, contact our corporate/institutionalsales department: 800-998-9938 or corporate@oreilly.com.Editor: Alicia YoungProduction Editor: Christopher FaucherCopyeditor: Rachel HeadProofreader: Amanda KerseyDecember 2016:April 2019:Indexer: WordCo Indexing Services, Inc.Interior Designer: David FutatoCover Designer: Karen MontgomeryIllustrator: Rebecca DemarestFirst EditionSecond EditionRevision History for the Second Edition2019-04-01: First ReleaseSee http://oreilly.com/catalog/errata.csp?isbn 9781492041214 for release details.The O’Reilly logo is a registered trademark of O’Reilly Media, Inc. Laravel: Up & Running, the coverimage, and related trade dress are trademarks of O’Reilly Media, Inc.The views expressed in this work are those of the author, and do not represent the publisher’s views.While the publisher and the author have used good faith efforts to ensure that the information andinstructions contained in this work are accurate, the publisher and the author disclaim all responsibilityfor errors or omissions, including without limitation responsibility for damages resulting from the use ofor reliance on this work. Use of the information and instructions contained in this work is at your ownrisk. If any code samples or other technology this work contains or describes is subject to open sourcelicenses or the intellectual property rights of others, it is your responsibility to ensure that your usethereof complies with such licenses and/or rights.978-1-492-04121-4[LSI]
This book is dedicated to my family.Mia, my little princess and bundle of joy and energy.Malachi, my little prince and adventurer and empath.Tereva, my inspiration, encourager, upgrader, pusher, rib.
This page intentionally left blank
Table of ContentsPreface. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . xvii1. Why Laravel?. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1Why Use a Framework?“I’ll Just Build It Myself ”Consistency and FlexibilityA Short History of Web and PHP FrameworksRuby on RailsThe Influx of PHP FrameworksThe Good and the Bad of CodeIgniterLaravel 1, 2, and 3Laravel 4Laravel 5What’s So Special About Laravel?The Philosophy of LaravelHow Laravel Achieves Developer HappinessThe Laravel CommunityHow It WorksWhy Laravel?12222333444556792. Setting Up a Laravel Development Environment. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11System RequirementsComposerLocal Development EnvironmentsLaravel ValetLaravel HomesteadCreating a New Laravel ProjectInstalling Laravel with the Laravel Installer Tool11121212131314v
Installing Laravel with Composer’s create-project FeatureLambo: Super-Powered “Laravel New”Laravel’s Directory StructureThe FoldersThe Loose FilesConfigurationThe .env FileUp and RunningTestingTL;DR141415161718192121223. Routing and Controllers. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 23A Quick Introduction to MVC, the HTTP Verbs, and RESTWhat Is MVC?The HTTP VerbsWhat Is REST?Route DefinitionsRoute VerbsRoute HandlingRoute ParametersRoute NamesRoute GroupsMiddlewarePath PrefixesFallback RoutesSubdomain RoutingNamespace PrefixesName PrefixesSigned RoutesSigning a RouteModifying Routes to Allow Signed LinksViewsReturning Simple Routes Directly with Route::view()Using View Composers to Share Variables with Every ViewControllersGetting User InputInjecting Dependencies into ControllersResource ControllersAPI Resource ControllersSingle Action ControllersRoute Model BindingImplicit Route Model Bindingvi Table of 424245464749495050
Custom Route Model BindingRoute CachingForm Method SpoofingHTTP Verbs in LaravelHTTP Method Spoofing in HTML FormsCSRF ProtectionRedirectsredirect()- to()redirect()- route()redirect()- back()Other Redirect Methodsredirect()- with()Aborting the RequestCustom Responsesresponse()- make()response()- json() and - jsonp()response()- download(), - streamDownload(), and - 6061624. Blade Templating. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 63Echoing DataControl StructuresConditionalsLoopsTemplate InheritanceDefining Sections with @section/@show and @yieldIncluding View PartialsUsing StacksUsing Components and SlotsView Composers and Service InjectionBinding Data to Views Using View ComposersBlade Service InjectionCustom Blade DirectivesParameters in Custom Blade DirectivesExample: Using Custom Blade Directives for a Multitenant AppEasier Custom Directives for “if ” 828383845. Databases and Eloquent. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 87Configuration87Table of Contents vii
Database ConnectionsOther Database Configuration OptionsDefining MigrationsRunning MigrationsSeedingCreating a SeederModel FactoriesQuery BuilderBasic Usage of the DB FacadeRaw SQLChaining with the Query BuilderTransactionsIntroduction to EloquentCreating and Defining Eloquent ModelsRetrieving Data with EloquentInserts and Updates with EloquentDeleting with EloquentScopesCustomizing Field Interactions with Accessors, Mutators, and AttributeCastingEloquent CollectionsEloquent SerializationEloquent RelationshipsChild Records Updating Parent Record TimestampsEloquent 1191201221261281311351371391521541551576. Frontend Components. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 159Laravel MixMix Folder StructureRunning MixWhat Does Mix Provide?Frontend Presets and Auth ScaffoldingFrontend PresetsAuth ScaffoldingPaginationPaginating Database ResultsManually Creating PaginatorsMessage BagsNamed Error BagsString Helpers, Pluralization, and Localizationviii Table of Contents159161161162169169170170170171172174174
The String Helpers and PluralizationLocalizationTestingTesting Message and Error BagsTranslation and LocalizationTL;DR1741751791791791807. Collecting and Handling User Data. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 181Injecting a Request Object request- all() request- except() and request- only() request- has() request- input() request- method() and - isMethod()Array InputJSON Input (and request- json())Route DataFrom RequestFrom Route ParametersUploaded FilesValidationvalidate() on the Request ObjectManual ValidationCustom Rule ObjectsDisplaying Validation Error MessagesForm RequestsCreating a Form RequestUsing a Form RequestEloquent Model Mass Assignment{{ Versus 71891891921921931941941951961971971998. Artisan and Tinker. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 201An Introduction to ArtisanBasic Artisan CommandsOptionsThe Grouped CommandsWriting Custom Artisan CommandsA Sample CommandArguments and OptionsUsing Input201202203203206208209211Table of Contents ix
PromptsOutputWriting Closure-Based CommandsCalling Artisan Commands in Normal CodeTinkerLaravel Dump ServerTestingTL;DR2132142152162172182192199. User Authentication and Authorization. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 221The User Model and MigrationUsing the auth() Global Helper and the Auth FacadeThe Auth ionControllerAuth::routes()The Auth Scaffold“Remember Me”Manually Authenticating UsersManually Logging Out a UserInvalidating Sessions on Other DevicesAuth MiddlewareEmail VerificationBlade Authentication DirectivesGuardsChanging the Default GuardUsing Other Guards Without Changing the DefaultAdding a New GuardClosure Request GuardsCreating a Custom User ProviderCustom User Providers for Nonrelational DatabasesAuth EventsAuthorization (ACL) and RolesDefining Authorization RulesThe Gate Facade (and Injecting Gate)Resource GatesThe Authorize MiddlewareController AuthorizationChecking on the User Instancex Table of 3245
Blade ChecksIntercepting ChecksPoliciesTestingTL;DR24624624724925210. Requests, Responses, and Middleware. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 253Laravel’s Request LifecycleBootstrapping the ApplicationService ProvidersThe Request ObjectGetting a Request Object in LaravelGetting Basic Information About a RequestThe Response ObjectUsing and Creating Response Objects in ControllersSpecialized Response TypesLaravel and MiddlewareAn Introduction to MiddlewareCreating Custom MiddlewareBinding MiddlewarePassing Parameters to MiddlewareTrusted 6927027227527627727811. The Container. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 279A Quick Introduction to Dependency InjectionDependency Injection and LaravelThe app() Global HelperHow the Container Is WiredBinding Classes to the ContainerBinding to a ClosureBinding to Singletons, Aliases, and InstancesBinding a Concrete Instance to an InterfaceContextual BindingConstructor Injection in Laravel Framework FilesMethod InjectionFacades and the ContainerHow Facades WorkReal-Time FacadesService 89289291291292Table of Contents xi
TL;DR29312. Testing. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 295Testing BasicsNaming TestsThe Testing EnvironmentThe Testing ationsDatabaseTransactionsSimple Unit TestsApplication Testing: How It WorksTestCaseHTTP TestsTesting Basic Pages with this- get() and Other HTTP CallsTesting JSON APIs with this- getJson() and Other JSON HTTP CallsAssertions Against responseAuthenticating ResponsesA Few Other Customizations to Your HTTP TestsHandling Exceptions in Application TestsDatabase TestsUsing Model Factories in TestsSeeding in TestsTesting Other Laravel SystemsEvent FakesBus and Queue FakesMail FakesNotification FakesStorage FakesMockingA Quick Introduction to MockingA Quick Introduction to MockeryFaking Other FacadesTesting Artisan CommandsAsserting Against Artisan Command SyntaxBrowser TestsChoosing a ToolTesting with DuskTL;DRxii Table of 1322322323324324335
13. Writing APIs. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 337The Basics of REST-Like JSON APIsController Organization and JSON ReturnsReading and Sending HeadersSending Response Headers in LaravelReading Request Headers in LaravelEloquent PaginationSorting and FilteringSorting Your API ResultsFiltering Your API ResultsTransforming ResultsWriting Your Own TransformerNesting and Relationships with Custom TransformersAPI ResourcesCreating a Resource ClassResource CollectionsNesting RelationshipsUsing Pagination with API ResourcesConditionally Applying AttributesMore Customizations for API ResourcesAPI Authentication with Laravel PassportA Brief Introduction to OAuth 2.0Installing PassportPassport’s APIPassport’s Available Grant TypesManaging Clients and Tokens with the Passport API and Vue ComponentsPassport ScopesDeploying PassportAPI Token AuthenticationCustomizing 404 ResponsesTriggering the Fallback RouteTestingTesting 37437437537514. Storage and Retrieval. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 377Local and Cloud File ManagersConfiguring File AccessUsing the Storage FacadeAdding Additional Flysystem ProvidersBasic File Uploads and ManipulationSimple File Downloads377377378380380382Table of Contents xiii
SessionsAccessing the SessionMethods Available on Session InstancesFlash Session StorageCacheAccessing the CacheMethods Available on Cache InstancesCookiesCookies in LaravelAccessing the Cookie ToolsLoggingWhen and Why to Use LogsWriting to the LogsLog ChannelsFull-Text Search with Laravel ScoutInstalling ScoutMarking Your Model for IndexingSearching Your IndexQueues and ScoutPerforming Operations Without IndexingConditionally Indexing ModelsManually Triggering Indexing via CodeManually Triggering Indexing via the CLITestingFile 9839839839939940040140240340340415. Mail and Notifications. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 405Mail“Classic” MailBasic “Mailable” Mail UsageMail TemplatesMethods Available in build()Attachments and Inline ImagesMarkdown MailablesRendering Mailables to the BrowserQueuesxiv Table of Contents405406406409410410411413414
Local DevelopmentNotificationsDefining the via() Method for Your NotifiablesSending NotificationsQueueing NotificationsOut-of-the-Box Notification 2142442542542616. Queues, Jobs, Events, Broadcasting, and the Scheduler. . . . . . . . . . . . . . . . . . . . . . . . . 427QueuesWhy Queues?Basic Queue ConfigurationQueued JobsControlling the QueueQueues Supporting Other FunctionsLaravel HorizonEventsFiring an EventListening for an EventBroadcasting Events over WebSockets, and Laravel EchoConfiguration and SetupBroadcasting an EventReceiving the MessageAdvanced Broadcasting ToolsLaravel Echo (the JavaScript Side)SchedulerAvailable Task TypesAvailable Time FramesDefining Time Zones for Scheduled CommandsBlocking and OverlapHandling Task OutputTask 44344344644845245745745845946046046146146317. Helpers and Collections. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 465HelpersArraysStrings465465467Table of Contents xv
Application PathsURLsMiscellaneousCollectionsThe BasicsA Few MethodsTL;DR46947047247547547748118. The Laravel Ecosystem. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 483Tools Covered in This BookValetHomesteadThe Laravel InstallerMixDuskPassportHorizonEchoTools Not Covered in This yTelescopeOther 6486487487487488488Glossary. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 489Index. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 497xvi Table of Contents
PrefaceThe story of how I got started with Laravel is a common one: I had written PHP foryears, but I was on my way out the door, pursuing the power of Rails and othermodern web frameworks. Rails in particular had a lively community, a perfect combi‐nation of opinionated defaults and flexibility, and the power of Ruby Gems to lever‐age prepackaged common code.Something kept me from jumping ship, and I was glad for that when I found Laravel.It offered everything I was drawn to in Rails, but it wasn’t just a Rails clone; this wasan innovative framework with incredible documentation, a welcoming community,and clear influences from many languages and frameworks.Since that day I’ve been able to share my journey of learning Laravel through blog‐ging, podcasting, and speaking at conferences; I’ve written dozens of apps in Laravelfor work and side projects; and I’ve met thousands of Laravel developers online andin person. I have plenty of tools in my development toolkit, but I am honestly happi‐est when I sit down in front of a command line and type laravel new projectName.What This Book Is AboutThis is not the first book about Laravel, and it won’t be the last. I don’t intend for thisto be a book that covers every line of code or every implementation pattern. I don’twant this to be the sort of book that goes out of date when a new version of Laravel isreleased. Instead, its primary purpose is to provide developers with a high-level over‐view and concrete examples to learn what they need to work in any Laravel codebasewith any and every Laravel feature and subsystem. Rather than mirroring the docs, Iwant to help you understand the foundational concepts behind Laravel.Laravel is a powerful and flexible PHP framework. It has a thriving community and awide ecosystem of tools, and as a result it’s growing in appeal and reach. This book isfor developers who already know how to make websites and applications and want tolearn how to do so well in Laravel.xvii
Laravel’s documentation is thorough and excellent. If you find that I don’t cover anyparticular topic deeply enough for your liking, I encourage you to visit the onlinedocumentation and dig deeper into that particular topic.I think you will find the book a comfortable balance between high-level introductionand concrete application, and by the end you should feel comfortable writing anentire application in Laravel, from scratch. And, if I did my job well, you’ll be excitedto try.Who This Book Is ForThis book assumes knowledge of basic object-oriented programming practices, PHP(or at least the general syntax of C-family languages), and the basic concepts of theModel–View–Controller (MVC) pattern and templating. If you’ve never made awebsite before, you may find yourself in over your head. But as long as you havesome programming experience, you don’t have to know anything about Laravelbefore you read this book—we’ll cover everything you need to know, from the sim‐plest “Hello, world!”Laravel can run on any operating system, but there will be some bash (shell) com‐mands in the book that are easiest to run on Linux/macOS. Windows users may havea harder time with these commands and with modern PHP development, but if youfollow the instructions to get Homestead (a Linux virtual machine) running, you’ll beable to run all of the commands from there.How This Book Is StructuredThis book is structured in what I imagine to be a chronological order: if you’re build‐ing your first web app with Laravel, the early chapters cover the foundational compo‐nents you’ll need to get started, and the later chapters cover less foundational or moreesoteric features.Each section of the book can be read on its own, but for someone new to the frame‐work, I’ve tried to structure the chapters so that it’s actually very reasonable to startfrom the beginning and read until the end.Where applicable, each chapter will end with two sections: “Testing” and “TL;DR.” Ifyou’re not familiar, “TL;DR” means “too long; didn’t read.” These final sections willshow you how to write tests for the features covered in each chapter and will give ahigh-level overview of what was covered.The book is written for Laravel 5.8, but will cover features and syntax changes back toLaravel 5.1.xviii Preface
About the Second EditionThe first edition of Laravel: Up & Running came out in November 2016 and coveredLaravel versions 5.1 to 5.3. This second edition adds coverage for 5.4 to 5.8 and Lara‐vel Dusk and Horizon, and adds an 18th chapter about community resources andother non-core Laravel packages that weren’t covered in the first 17 chapters.Conventions Used in This BookThe following typographical conventions are used in this book:ItalicIndicates new terms, URLs, email addresses, filenames, and file extensions.Constant widthUsed for program listings, as well as within paragraphs to refer to program ele‐ments such as variable or function names, databases, data types, environmentvariables, statements, and keywords.Constant width boldShows commands or other text that should be typed literally by the user.Constant width italicShows code text that should be replaced with user-supplied values or by valuesdetermined by context.{Italic in braces}Shows file names or file pathways that should be replaced with user-supplied val‐ues or by values determined by context.This element signifies a tip or suggestion.This element signifies a general note.This element indicates a warning or caution.Preface xix
Because this book covers Laravel from versions 5.1 to 5.8, you’ll find markersthroughout the book indicating version-specific comments. Generally speaking, theindicator is showing the version of Laravel a feature was introduced in (so you’ll see a5.3 next to a feature that’s only accessible in Laravel 5.3 and higher).O’Reilly Online LearningFor almost 40 years, O’Reilly Media has provided technologyand business training, knowledge, and insight to help compa‐nies succeed.Our unique network of experts and innovators share their knowledge and expertisethrough books, articles, conferences, and our online learning platform. O’Reilly’sonline learning platform gives you on-demand access to live training courses, indepth learning paths, interactive coding environments, and a vast collection of textand video from O’Reilly and 200 other publishers. For more information, pleasevisit http://oreilly.com.How to Contact UsPlease address comments and questions concerning this book to the publisher:O’Reilly Media, Inc.1005 Gravenstein Highway NorthSebastopol, CA 95472800-998-9938 (in the United States or Canada)707-829-0515 (international or local)707-829-0104 (fax)We have a web page for this book, where we list errata, examples, and any additionalinformation. You can access this page at http://bit.ly/laravel-up-and-running-2e.To comment or ask technical questions about this book, send email to bookques‐tions@oreilly.com.For more information about our books, courses, conferences, and news, see our web‐site at http://www.oreilly.com.Find us on Facebook: http://facebook.com/oreillyFollow us on Twitter: http://twitter.com/oreillymediaWatch us on YouTube: http://www.youtube.com/oreillymediaxx Preface
Acknowledgments for the First EditionThis book would not have happened without the gracious support of my amazingwife, Tereva, or the understanding (“Daddy’s writing, buddy!”) of my son Malachi.And while she wasn’t explicitly aware of it, my daughter Mia was around for almostthe entire creation of the book, so this book is dedicated to the whole family. Therewere many, many long evening hours and weekend Starbucks trips that took me awayfrom them, and I couldn’t be more grateful for their support and also their presencejust making my life awesome.Additionally, the entire Tighten family has supported and encouraged me through thewriting of the book, several colleagues even editing code samples (Keith Damiani,editor extraordinaire) and helping me with challenging ones (Adam Wathan, King ofthe Collection Pipeline). Dan Sheetz, my partner in Tighten crime, has been graciousenough to watch me while away many a work hour cranking on this book and wasnothing but supportive and encouraging; and Dave Hicking, our operations manager,helped me arrange my schedule and work responsibilities around writing time.Taylor Otwell deserves thanks and honor for creating Laravel—and therefore creatingso many jobs and helping so many developers love our lives that much more. Hedeserves appreciation for how he’s focused on developer happiness and how hard he’sworked to have empathy for developers and to build a positive and encouraging com‐munity. But I also want to thank him for being a kind, encouraging, and challengingfriend. Taylor, you’re a boss.Thanks to Jeffrey Way, who is one of the best teachers on the internet. He originallyintroduced me to Laravel and introduces more people every day. He’s also, unsurpris‐ingly, a fantastic human being whom I’m glad to call a friend.Thank you to Jess D’Amico, Shawn McCool, Ian Landsman, and Taylor for seeingvalue in me as a conference speaker early on and giving me a platform to teach from.Thanks to Dayle Rees for making it so easy for so many to learn Laravel in the earlydays.Thanks to every person who put their time and effort into writing blog posts aboutLaravel, especially early on: Eric Barnes, Chris Fidao, Matt Machuga, Jason Lewis,Ryan Tablada, Dries Vints, Maks Surguy, and so many more.And thanks to the entire community of friends on Twitter, IRC, and Slack who’veinteracted with me over the years. I wish I could name every name, but I would misssome and then feel awful about missing them. You all are brilliant, and I’m honoredto get to interact with you on a regular basis.Thanks to my O’Reilly editor, Ally MacDonald, and all of my technical editors: KeithDamiani, Michael Dyrynda, Adam Fairholm, and Myles Hyson.Preface xxi
And, of course, thanks to the rest of my family and friends, who supported medirectly or indirectly through this process—my parents and siblings, the Gainesvillecommunity, other business owners and authors, other conference speakers, and theinimitable DCB. I need to stop writing because by the time I run out of space here I’llbe thanking my Starbucks baristas.Acknowledgments for the Second EditionThe second edition is very similar to the first, so all of the previous acknowledgmentsare still valid. But I’ve gotten help from a few new people this time around. My tech‐nical proofreaders have been Tate Peñaranda, Andy Swick, Mohamed Said, andSamantha Geitz, and my new O’Reilly editor has been Alicia Young, who’s kept me ontask through a lot of changes in my life and the Laravel community over the last year.Matt Hacker on the Atlas team answered all my stupid AsciiDoc formatting ques‐tions, including about the surprisingly difficult formatting for the () method.And I couldn’t have made it through the process of writing a second edition withoutthe help of my research assistant, Wilbur Powery. Wilbur was willing to sift throughthe last several years’ worth of changelogs and pull requests and announcements andmatch each feature up with the current structure of the book, and he even testedevery single code example in the book in Laravel 5.7 (and then, later, 5.8) so that Icould focus my limited time and energy on writing the new and updated segments.Also, my daughter, Mia, is out of her mama’s belly now. So, let’s just add her joy andenergy and love and cuteness and adventurous spirit to my list of sources of inspira‐tion.xxii Preface
This book is dedicated to my family. Mia, my little princess and bundle of joy and energy. Malachi, my little prince and adventurer and empath. . Laravel's Request Lifecycle 253 Bootstrapping the Application 254 .