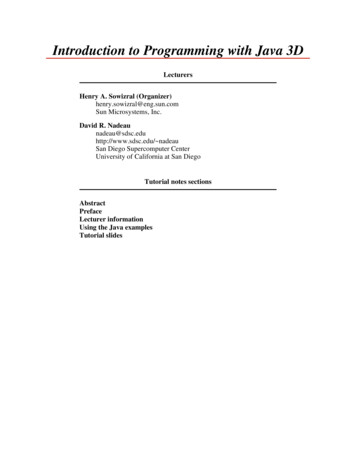
Transcription
Introduction to Programming with Java 3DLecturersHenry A. Sowizral (Organizer)henry.sowizral@eng.sun.comSun Microsystems, Inc.David R. Nadeaunadeau@sdsc.eduhttp://www.sdsc.edu/ nadeauSan Diego Supercomputer CenterUniversity of California at San DiegoTutorial notes sectionsAbstractPrefaceLecturer informationUsing the Java examplesTutorial slides
Introduction to Programming with Java 3DAbstractJava 3D is a new cross-platform API for developing 3D graphics applications in Java. Its feature set isdesigned to enable quick development of complex 3D applications and, at the same time, enable fast andefficient implementation on a variety of platforms, from PCs to workstations. Using Java 3D, softwaredevelopers can build cross-platform applications that build 3D scenes programmatically, or via loading3D content from VRML, OBJ, and/or other external files. The Java 3D API includes a rich feature setfor building shapes, composing behaviors, interacting with the user, and controlling rendering details.In this tutorial, participants learn the concepts behind Java 3D, the Java 3D class hierarchy, typical usagepatterns, ways of avoiding common mistakes, animation and scene design techniques, and tricks forincreasing performance and realism.
Introduction to Programming with Java 3DPrefaceWelcome to these tutorial notes! These tutorial notes have been written to give you a quick,practical, example-driven overview of Java 3D, the cross-platform 3D graphics API for Java. Todo this, we’ve included almost 600 pages of tutorial material with nearly 100 images and over 50Java 3D examples.To use these tutorial notes you will need:An HTML Web browserJava JDK 1.2 (Java 2 Platform) or laterJava 3D 1.1 or laterInformation on Java JDKs and Java 3D is available at:http://www.javasoft.comWhat’s included in these notesThese tutorial notes primarily contain two types of information:1. General information, such as this preface2. Tutorial slides and examplesThe tutorial slides are arranged as a sequence of 600 hyper-linked pages containing Java 3Dsyntax notes, Java 3D usage comments, or images of sample Java 3D applications. Clicking on thefile name underneath an image brings up a window showing the Java source file that generated theimage. The Java source files contain extensive comments providing information about thetechniques the file illustrates.Compiling and executing the Java example file from the command-line brings up a Javaapplication illustrating a Java 3D feature. Most such applications include menus and otherinteraction options with which you can explore Java 3D features.The tutorial notes provide a necessarily terse overview of Java 3D. We recommend that you investin a Java 3D book to get thorough coverage of the language. One of the course lecturers is anauthor of the Java 3D specification, available from Addison-Wesley: The Java 3D APISpecification, ISBN 0-201-32576-4, 1997.Use of these tutorial notesWe are often asked if there are any restrictions on use of these tutorial notes. The answer is:Parts of these tutorial notes are copyright (c) 1999 by Henry A. Sowizral, and copyright (c)1999 by David R. Nadeau. Users and possessors of these tutorial notes are hereby granted a
nonexclusive, royalty-free copyright and design patent license to use this material inindividual applications. License is not granted for commercial resale, in whole or in part,without prior written permission from the authors. This material is provided "AS IS" withoutexpress or implied warranty of any kind.You are free to use these tutorial notes in whole or in part to help you teach your own Java 3Dtutorial. You may translate these notes into other languages and you may post copies of these noteson your own Web site, as long as the above copyright notice is included as well. You may not,however, sell these tutorial notes for profit or include them on a CD-ROM or other media productwithout written permission.If you use these tutorial notes, we ask that you:1. Give us credit for the original material2. Tell us since we like hearing about the use of our material!If you find bugs in the notes, please tell us. We have worked hard to try and make the notesbug-free, but if something slipped by, we’d like to fix it before others are confused by our mistake.ContactDavid R. NadeauUniversity of CaliforniaNPACI/SDSC, MC 05059500 Gilman DriveLa Jolla, CA 92093-0505(619) 534-5062FAX: (619) 534-5152nadeau@sdsc.eduhttp://www.sdsc.edu/ nadeau
Introduction to Programming with Java 3DLecturer informationHenry A. Sowizral (Organizer)TitleDistinguished EngineerAffiliation Sun Microsystems, Inc.Address 901 San Antonio Road, MS UMPK14-202Palo Alto, CA 94303-4900EmailUPS, Fed Ex: 14 Network CircleMenlo Park, CA, 94025henry.sowizral@eng.sun.comHenry Sowizral is a Distinguished Engineer at Sun Microsystems where he is the chief architect ofthe Java 3D API. His areas of interest include virtual reality, large model visualization, anddistributed and concurrent simulation. He has taught tutorials on topics including expert systemsand virtual reality at conferences including COMPCON, Supercomputing, VRAIS, andSIGGRAPH. Henry has taught Java 3D at SIGGRAPH, Eurographics, Visualization, JavaOne,VRAIS, and other conferences.Henry is a co-author of the book The Java 3D API Specification, published by Addison-Wesley.He holds a B.S. in Information and Computer Science from the University of California, Irvine,and an M.Phil. and Ph.D. in Computer Science from Yale University.David R. NadeauTitlePrincipal ScientistAffiliation San Diego Supercomputer Center (SDSC)University of California, San Diego (UCSD)AddressNPACI/SDSC, MC 05059500 Gilman DriveLa Jolla, CA 92093-0505Emailnadeau@sdsc.eduHome page http://www.sdsc.edu/ nadeauDave Nadeau is a principal scientist at the San Diego Supercomputer Center, a national researchcenter specializing in computational science and engineering, located on the campus of theUniversity of California, San Diego. His areas of interest include scientific visualization andvirtual reality, He has taught Java 3D and VRML at multiple conferences including SIGGRAPH,Eurographics, Supercomputing, WebNet, WMC/SCS, VRAIS, and Visualization.Dave is a co-author of The VRML 2.0 Sourcebook published by John Wiley & Sons. He holds aB.S. in Aerospace Engineering from the University of Colorado, Boulder, an M.S. in MechanicalEngineering from Purdue University, and is in the Ph.D. program in Electrical and ComputerEngineering at the University of California, San Diego.
Introduction to Programming with Java 3DUsing the Java examplesThese tutorial notes include dozens of separate Java applications illustrating the use of Java 3D.The source code for these applications is included in files with .java file name extensions.Compiled byte-code for these Java files is not included! To use these examples, you will need tocompile the applications first.Compiling JavaThe source code for all Java 3D examples is in the examples folder. Images, sound, and geometryfiles used by these examples are also contained within the same folder. A README.txt file in thefolder lists the Java 3D applications included therein.To compile the Java examples, you will need:The Java 3D API 1.1 class files (or later)The Java JDK 1.2 (Java 2 Platform) class files (or later)A Java compilerThe JDK 1.2 class files are available for free from JavaSoft at http://www.javasoft.com.The Java 3D class files are available for free from Sun Microsystems athttp://www.sun.com/desktop/java3d.There are multiple Java compilers available for most platforms. JavaSoft provides the JavaDevelopment Kit (JDK) for free from its Web site at http://www.javasoft.com. The JDK includesthe javac compiler and instructions on how to use it. Multiple commercial Java developmentenvironments are available from Microsoft, Symantec, and others. An up to date list of availableJava products is available at Developer.com’s Web site ava.html.Once you have the Java API class files and a Java compiler, you may compile the supplied Javafiles. Unfortunately, we can’t give you explicit directions on how to do this. Each platform andJava compiler is different. You’ll have to consult your software’s manuals.Running the Java 3D ExamplesTo run a Java application, you must run the Java interpreter and give it the Java class file as anargument, like this:java MyClassThe Java interpreter looks for the file MyClass.class in the current directory and loads it, and anyadditional files needed by that class.
Title PagePicking shapesCreating backgroundsWorking with fogConclusionsIntroduction to Programming with Java 3DTable of contents448469489516Extended notesMorningSection 5 - Text geometry, Raster geometry, Advanced texture mappingSection 1 - Introduction, Scene graphs, Shapes, AppearanceWelcomeIntroductionBuilding 3D content with a scene graphBuilding 3D shapesControlling appearanceBuilding text shapesControlling the appearance of texturesAdding soundControlling the sound environment152465103519535552587Section 2 - Groups, Transforms, Texture mapping, Lighting138149171196212235245Grouping shapesTransforming shapesUsing special-purpose groupsIntroducing texture mappingUsing texture coordinatesUsing raster geometryLighting the environmentAfternoonSection 3 - Universes, Viewing, Input, Behaviors272283321360366381Building a virtual universeIntroducing the view modelViewing the sceneBuilding a simple universeUsing input devicesCreating behaviorsSection 4 - Interpolators, Picking, Backgrounds, Fog409437Creating interpolator behaviorsUsing specialized behaviors12WelcomeWelcomeIntroduction to Programming with Java 3DTutorial scheduleTutorial scopeIntroduction to Programming with Java 3D234Welcome to the tutorial!
34WelcomeWelcomeTutorial scheduleTutorial scopeMorningSection 1 Introduction, Scene graphs, Shapes, AppearanceSection 2 Groups, Transforms, Texture mapping, LightingAfternoonSection 3 Universes, Viewing, Input, BehaviorsSection 4 Interpolators, Picking, Backgrounds, FogThis tutorial will:Introduce Java 3D concepts and terminologyDiscuss important Java 3D classesIllustrate how to write a Java 3D application or appletDiscuss typical usage patterns, techniques, and tricksExtended notesSection 5 Text geometry, Advanced texture mapping, Sound,Sound environment56IntroductionIntroductionWhat is Java 3D?What is Java 3D?What does Java 3D do?What does Java 3D do?What application areas can use Java 3D?Examples: Scientific VisualizationExamples: Abstract Data (Financial)Examples: Medical EducationExamples: CADExamples: AnalysisExamples: AnimationsExamples: 3D LogosExamples: Scientific VisualizationWhat software do I need to use Java 3D?What hardware do I need to use Java 3D?How do I run a Java 3D application/applet?How does Java 3D compare with other APIs?SummaryWhat is Java 3D?67891011121314151617181920212223Java 3D is an interactive 3D graphics Application ProgrammingInterface (API) for building applications and applets in JavaA means for developing and presenting 3D contentDesigned for Write once, run anywhereMultiple platforms (processors and pipes)Multiple display environmentsMultiple input devices
78IntroductionIntroductionWhat is Java 3D?What does Java 3D do?Raise the programming floorProvide a vendor-neutral, platform-independent API within JavaIntegrates with other Java APIs: image processing, fonts, 2Ddrawing, user interfaces, etc.Think objects . . . not verticesThink content . . . not rendering processEnable high level application developmentAuthors focus upon content, not renderingJava 3D handles optimal rendering910IntroductionIntroductionWhat does Java 3D do?What application areas can use Java 3D?Perform rendering optimizationsScene managementContent culling based upon visibility (frustum)Efficient pipeline use (sorting, batching)Parallel renderingScene compilation (reorganization, combination, etc.)And achieve high performanceDraw via OpenGL/Direct3DUses 3D graphics hardware acceleration where availableScientific visualizationInformation visualizationMedical visualizationGeographical information systems (GIS)Computer-aided design (CAD)AnimationEducation
1112IntroductionIntroductionExamples: Scientific VisualizationExamples: Abstract Data (Financial)1314IntroductionIntroductionExamples: Medical EducationExamples: CAD
1516IntroductionIntroductionExamples: AnalysisExamples: Animations1718IntroductionIntroductionExamples: 3D LogosExamples: Scientific VisualizationAnatomy BrowserUniversity of MassachusetsandBrigham and Women’sHospitalCollaborative VisualizationSpace Science andEngineering Center (SSEC)
1920IntroductionIntroductionWhat software do I need to use Java 3D?What hardware do I need to use Java 3D?Java development kitJava 2 platformFree from http://java.sun.comJava 3D development kitJava 3D 1.1Free from http://www.sun.com/desktop/java3DSun provides Windows 9x/NT and Solaris portsLinux port is availableYou will need a 3D graphics acceleratorOn PCs:PC cards are widely availableShould support OpenGL 1.1 featuresA Direct3D version is in progressLinux port uses MesaOn Suns:Creator 3D or Elite 3D hardwareSupport OpenGL 1.2Other ports come from platform vendors2122IntroductionIntroductionHow do I run a Java 3D application/applet?How does Java 3D compare with other APIs?Java 3D applications:Run like any other Java applicationprompt java myapplicationJava 3D applets:Use the Java plug-in in Netscape or Internet ExplorerEmbeds the applet in a Web pageJava plug-in automatically downloads JDK and Java 3D if notalready installed"Older" APIs enable only low-level hardware state controlProvide and require detailed controlOpenGL, Direct3D, low-level game engines"Newer" APIs focus upon high-level content controlProvide some rendering optimizationJava 3DVRMLSGI OpenInventor, Optimizer/Cosmo3D (being phased out)SGI-Microsoft "Fahrenheit"
2324IntroductionBuilding 3D content with a scene graphSummaryJava 3D is a high-level API for building interactive 3Dapplications and applets in JavaWrite once, run anywhere . . . in 3DBuilding a scene graphScene graph exampleSketching a scene graph diagramExamples of creating large scenesBuilding a scene graphProcessing a scene graphExamples of Java 3D featuresExamples of Java 3D featuresExamples of Java 3D featuresUsing scene graph terminologyScene graph base class hierarchyBuilding a scene graphBuilding a scene graphUsing universe terminologyUsing branch terminologySketching a universe diagramSuperstructure class hierarchyBuilding a universeBuilding a universeBuilding scene contentLoading scene content from filesBuilding scene graph superstructureSketching a simple universe diagramHelloWorld exampleHelloWorld example codeHelloWorld example codeHelloWorld example codeHelloWorld example codeHelloWorld exampleMaking a node liveChecking if a node is liveCompiling a scene graphCompiling a scene graphControlling access 444546474849505152535455565758Controlling access capabilitiesControlling access capabilitiesControlling access capabilitiesSummarySummarySummary2526Building 3D content with a scene graphBuilding 3D content with a scene graphBuilding a scene graphScene graph exampleA scene graph is a "family tree" containing scene data"Children" are shapes, lights, sounds, etc."Parents" are groups of children and other parentsThis defines a hierarchical grouping of shapesFor example, imagine building a toy airplane:The application builds a scene graph using Java 3D classes andmethodsStart with parts on the table Assemble related partsJava 3D renders that scene graph onto the screenAssemble those into the final plane596061626364
2728Building 3D content with a scene graphBuilding 3D content with a scene graphSketching a scene graph diagramExamples of creating large scenesSketching a scene graph diagram can clarify a design and easesoftware developmentJava 3D scene graphs may include large numbers of shapesLanding gear192 shapesBoom box11,000 shapes2930Building 3D content with a scene graphBuilding 3D content with a scene graphBuilding a scene graphProcessing a scene graphScene graphs are built from components including:Shapes (geometry and appearance)Groups and transformsLightsFog and backgroundsSounds and sound environments (reverb)BehaviorsView platforms (viewpoints)Java 3D renders the scene graphScene graph specifies content, not rendering orderRendering order is up to Java 3DJava 3D uses separate, independent and asynchronous threadsGraphics renderingSound "rendering"Animation "behavior execution"Input device managementEvent generation (collision detection)
3132Building 3D content with a scene graphBuilding 3D content with a scene graphExamples of Java 3D featuresExamples of Java 3D featuresYou can control shape coloration and texture . . . . . lighting and fog effects . . .MonumentColonade3334Building 3D content with a scene graphBuilding 3D content with a scene graphExamples of Java 3D featuresUsing scene graph terminology. . . shape position, orientation, and size and how those changeover time, and moreBut first, some terminology . . .Node: an item in a scene graphLeaf nodes: nodes with no childrenShapes, lights, sounds, etc.Animation behaviorsGroup nodes: nodes with childrenTransforms, switches, etc.Jetsons-VisLogoNode component: a bundle of attributes for a nodeGeometry of a shapeColor of a shapeSound data to playCar SuspensionDuke Treadmill
3536Building 3D content with a scene graphBuilding 3D content with a scene graphScene graph base class hierarchyBuilding a scene graphLeaf nodes, group nodes, node components, and different typesof all of these lead to . . . a Java 3D class hierarchyBuild nodes by instantiating Java 3D classesShape3D myShape1 new Shape3D( myGeom1, myAppear1 );Shape3D myShape2 new Shape3D( myGeom2 );Class ify nodes by calling methods on an instancemyShape2.setAppearance( newAppear2 );Build groups of nodesGroup myGroup new Group( );myGroup.addChild( myShape1 );myGroup.addChild( myShape2 );3738Building 3D content with a scene graphBuilding 3D content with a scene graphBuilding a scene graphUsing universe terminologyWe need to assemble chunks of content, each in its own scenegraphBuild components separatelyAssemble them into a common container: a virtual universeA way to combine scene graphsA place to root the scene graphVirtual universe: a collection of scene graphsTypically one universe per applicationLocale: a position in the universe at which to put scene graphsTypically one locale per universeBranch graph: a scene graphTypically several branch graphs per locale
3940Building 3D content with a scene graphBuilding 3D content with a scene graphUsing branch terminologySketching a universe diagramScene graphs are typically divided into two types of branchgraphs:A universe builds superstructure to contain scene graphsContent branch: shapes, lights, and other contentTypically multiple branches per localeView branch: viewing informationTypically one per universeThis division is optional:Content and viewing information can be interleaved in thesame branch (and sometimes should be)4142Building 3D content with a scene graphBuilding 3D content with a scene graphSuperstructure class hierarchyBuilding a universeUniverses and locales are superstructure classes for organizingcontentBuild a universeVirtualUniverse myUniverse new VirtualUniverse( );Class d a localeLocale myLocale new Locale( myUniverse );Build a branch groupBranchGroup myBranch new BranchGroup( );
4344Building 3D content with a scene graphBuilding 3D content with a scene graphBuilding a universeBuilding scene contentBuild nodes and groups of nodesShape3D myShape new Shape3D( myGeom, myAppear );Group myGroup new Group( );myGroup.addChild( myShape );Java 3D’s rich feature set enables you to build complex 3DcontentBuild content directly within your Java applicationLoad content from filesDo bothAdd them to the branch groupmyBranch.addChild( myGroup );Add the branch graph to the localemyLocale.addBranchGraph( myBranch );File loader classes enable reading content from files in standardformatsVRML (Virtual Reality Modeling Language)OBJ (Alias Wavefront object)LW3D (Lightwave 3D scene)others . . .4546Building 3D content with a scene graphBuilding 3D content with a scene graphLoading scene content from filesBuilding scene graph superstructureLoad an OBJ file describing a shipUtility classes help automate common operationsImplemented atop Java 3DThe SimpleUniverse utility builds a common arrangement of auniverse, locale, and viewing classesSimpleUniverse mySimple new SimpleUniverse( myCanvasmySimple.addBranchGraph( myBranch );[ A3DApplet ]
4748Building 3D content with a scene graphBuilding 3D content with a scene graphSketching a simple universe diagramHelloWorld exampleA SimpleUniverse encapsulates a common superstructureLet’s build a multi-colored 3D cube and spin it about the verticalaxis[ HelloWorld ]4950Building 3D content with a scene graphBuilding 3D content with a scene graphHelloWorld example codeHelloWorld example codeImport the Java 3D classes . . e.*;Build a frame, 3D canvas, and simple universe . . .public static void main( String[] args ) {Frame frame new Frame( );frame.setSize( 640, 480 );frame.setLayout( new BorderLayout( ) );Canvas3D canvas new Canvas3D( null );frame.add( "Center", canvas );public class HelloWorld{. . .}SimpleUniverse univ new SimpleUniverse( canvas )univ.getViewingPlatform( ).setNominalViewingTransfBranchGroup scene createSceneGraph( );scene.compile( );univ.addBranchGraph( scene );frame.show( );}
5152Building 3D content with a scene graphBuilding 3D content with a scene graphHelloWorld example codeHelloWorld example codeBuild 3D shapes within a BranchGroup . . .Set up an animation behavior to spin the shapes . . .public BranchGroup createSceneGraph( ){BranchGroup branch new BranchGroup( );// Make a behavor to spin the shapeAlpha spinAlpha new Alpha( -1, 4000 );RotationInterpolator spinner new RotationInterpolator( spinAlpha, trans );spinner.setSchedulingBounds(new BoundingSphere( new Point3d( ), 1000.0trans.addChild( spinner );// Make a changeable 3D transformTransformGroup trans new TransformGroup( );trans.setCapability( TransformGroup.ALLOW TRANSFORbranch.addChild( trans );return branch;// Make a shapeColorCube demo new ColorCube( 0.4 );trans.addChild( demo );}. . .5354Building 3D content with a scene graphBuilding 3D content with a scene graphHelloWorld exampleMaking a node liveWhich produces a spinning multi-colored 3D cube . . .Adding a branch graph into a locale (or simple universe) makesits nodes live (drawable)BranchGroup myBranch new BranchGroup( );myBranch.addChild( myShape );myLocale.addBranchGraph( myBranch ); // make live!Removing the branch graph from the locale reverses the effectmyLocale.removeBranchGraph( myBranch );// not live[ HelloWorld ]
5556Building 3D content with a scene graphBuilding 3D content with a scene graphChecking if a node is liveCompiling a scene graphA method on SceneGraphObject queries if a node is liveA method on BranchGroup compiles the branch, optimizing it forfaster renderingMethodMethodboolean isLive( )void compile( )5758Building 3D content with a scene graphBuilding 3D content with a scene graphCompiling a scene graphControlling access capabilitiesCompile a branch graph before making it liveBranchGroup myBranch new BranchGroup( );myBranch.addChild( myShape );myBranch.compile( );myLocale.addBranchGraph( myBranch );Node capabilities (permissions) control read and write accessRead or write any attribute before a node is live or compiledCapabilities control access while a node is live or compiledKeep the number of capabilities small so Java 3D can make moreoptimizations during compilation
5960Building 3D content with a scene graphBuilding 3D content with a scene graphControlling access capabilitiesControlling access capabilitiesMethods on the SceneGraphObject set/clear capabilitiesMethodvoid setCapability( int bit )void clearCapability( int bit )boolean getCapability( int bit )Each node has its own read and write capabilitiesUsually a separate capability for each attribute of a nodeNode’s also inherit parent class capabilitiesEach capability has an upper-case nameFor example, Shape3D capabilities include:ALLOW APPEARANCE READALLOW APPEARANCE WRITEALLOW GEOMETRY READALLOW GEOMETRY WRITEALLOW COLLISION BOUNDS READALLOW COLLISION BOUNDS WRITEPlus capabilities from the parent Node class, including:ALLOW BOUNDS READALLOW BOUNDS WRITEALLOW PICKABLE READALLOW PICKABLE WRITE. . . and others6162Building 3D content with a scene graphBuilding 3D content with a scene graphControlling access capabilitiesSummarySet capabilities while you build your contentA scene graph is a hierarchy of groups of shapes, lights, sounds,etc.Shape3D myShape new Shape3D( myGeom, myAppear );myShape.setCapability( Shape3D.ALLOW APPEARANCE WRITEAfter a node is live, change attributes that have enabledcapabilitiesmyShape.setAppearance( newAppear );// allowedBut you cannot change attributes for which you do not havecapabilities setmyShape.setGeometry( newGeom );// error!Your application builds the scene graph using Java 3D classesand methodsThe Java 3D implementation uses the scene graph behind thescene to render shapes, play sounds, execute animations, etc.
6364Building 3D content with a scene graphBuilding 3D content with a scene graphSummarySummaryA virtual universe holds everythingAdding a branch graph to a locale makes it live and drawableA locale positions a branch graph in a universeCompiling a branch graph optimizes it for faster renderingA branch graph is a scene graphCapabilities control access to node attributes after a node is liveor compiledFewer capabilities enables more optimizationsA node is an item in a scene graphA node component is a bundle of attributes for a node6566Building 3D shapesBuilding 3D shapesMotivationExampleShape3D class hierarchyShape3D class methodsBuilding geometry using coordinatesBuilding geometry using coordinatesUsing a right-handed coordinate systemUsing coordinate orderUsing coordinate orderDefining verticesDefining verticesBuilding geometryGeometryArray class hierarchyGeometryArray class methodsGeometryArray class methodsBuilding different types of geometryBuilding a PointArrayPointArray example codeBuilding a LineArrayLineArray example codeBuilding a TriangleArrayTriangleArray example codeBuilding a QuadArrayQuadArray example codeBuilding geometry stripsGeometryStripArray class hierarchyBuilding a LineStripArrayBuilding a TriangleFanArrayBuilding a TriangleStripArrayBuilding indexed geometryIndexedGeometryArray class hierarchyIndexedGeometryArray class methodsIndexedGeometryArray class methodsGearbox vation100101102A Shape3D leaf node builds a 3D object with:Geometry:The form or structure of a shapeAppearance:The coloration, transparency, and shading of a shapeJava 3D supports multiple geometry and appearance featuresWe’ll talk about geometry first, then appearance
6768Building 3D shapesBuilding 3D shapesExampleShape3D class hierarchyThe Shape3D class extends the Leaf classClass ax.media.j3d.Shape3D[ GearBox ]6970Building 3D shapesBuilding 3D shapesShape3D class methodsBuilding geometry using coordinatesMethods on Shape3D set geometry and appearance attributesMethodShape3D( )Shape3D( Geometry geometry, Appearance appearance )void setGeometry( Geometry geometry )void setAppearance( Appearance appearance )Building shape geometry is like a 3D connect-the-dots gamePlace "dots" at 3D coordinatesConnect-the-dots to form 3D shapesFor example, to build a pyramid start with five coordinates
7172Building 3D shapesBuilding 3D shapesBuilding geometry using coordinatesUsing a right-handed coordinate systemFinish the pyramid by connecting the dots to form triangles3D coordinates are given in a right-handed coordinate systemX left-to-rightY bottom-to-topZ back-to-frontDistances are conventionally in meters1.2.3.4.7374Building 3D shapesBuilding 3D shapesUsing coordinate orderUsing coordinate orderPolygons have a front and back:By default, only the front side of a polygon is renderedA polygon’s winding order determines which side is the frontMost polygons only need one side renderedYou can turn on double-sided rendering, at a performancecostUse the right-hand rule:Curl your right-hand fingers around the polygon perimeter inthe order vertices are given (counter-clockwise)Your thumb sticks out the front of the polygon
7576Building 3D shapesBuilding 3D shapesDefining verticesDefining verticesA vertex describes a polygon corner and contains:A 3D coordinateA colorA texture coordinateA lighting normal vectorThe 3D coordinate in a vertex is required, the rest are optionalA vertex normal defines surface information for lightingBut the coordinate winding order defines the polygon’s frontand back, and thus the side that is drawnIf you want to light your geometry, you must specify vertexlighting normalsLighting normals must be unit length7778Building 3D shapesBuilding 3D shapesBuilding geometryGeometryArray class hierarchyJava 3D has multiple types of geometry that use 3D coordinates:Points, lines, triangles, and quadrilaterals3D extruded textRa
Introduction to Programming with Java 3D Lecturers Henry A. Sowizral (Organizer) henry.sowizral@eng.sun.com Sun Microsystems, Inc. David R. Nadeau . In this tutorial, participants learn the concepts behind Java 3D, the Java 3D class hierarchy, typical usage patterns, ways of avoiding common mistakes, animation and scene design techniques, and .