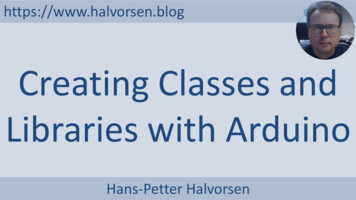
Transcription
https://www.halvorsen.blogCreating Classes andLibraries with ArduinoHans-Petter Halvorsen
Contents We will learn how we can create ourown Arduino Libraries from Scratch Why create your own Libraries?– Better Code structure– Reuse your Code in different Applications– Distribute to others
Fahrenheit Example We will create code that convert from degreesCelsius to degrees Fahrenheit (and theopposite)
void setup(){float Tf;float Tc;Serial.begin(9600);Tc 0;Tf Tc * 9/5 32;Serial.println(Tf);Tf 32;Tc (Tf-32)*((float)5/9);Serial.println(Tc);}void loop(){}The StartSerial Monitor:
Creating FunctionsWhy Creating Functions? In order to structure your code better You can reuse your Code
Creating Functionsvoid setup(){float c;float f;Serial.begin(9600);c 0;f c2f(c);Serial.println(f);f 32;c f2c(f);Serial.println(c);float c2f(float Tc){float Tf;Tf Tc * 9/5 32;return Tf;}}void loop(){}float f2c(float Tf){float Tc;Tc (Tf-32)*((float)5/9);return Tc;}
Creating Classes Next, I will show how you can group yourfunctions into a Class A class is simply a collection of functions andvariables that are all kept together in oneplace
The functions andvariables can be eitherprivate and publicCreating Classesclass Fahrenheit{public:void setup(){float f;float c;Fahrenheit(){Serial.begin(9600);};Fahrenheit fahr;float c2f(float Tc){float Tf;Tf Tc * 9/5 32;return Tf;}c 0;f fahr.c2f(c);Serial.println(f);float f2c(float Tf){float Tc;Tc (Tf-32)*((float)5/9);return Tc;}f 32;c fahr.f2c(f);Serial.println(c);}void loop(){};} public: they can be accessed bypeople using your library private: meaning they can onlybe accessed from within theclass itself Each class has a specialfunction known asa constructor, which is usedto create an instance of theclass. The constructor has the samename as the class, and noreturn type.
Running the Program
Arduino Libraries Libraries are a collection of code that makes iteasy for you to connect to a sensor, display,module, etc. There are hundreds of additional librariesavailable on the Internet for download. You can also create your own Libraries fromscratch – Thats what we will show her
Arduino LibrariesWhy create your own Libraries? Better Code structure Reuse your Code in different Applications Distribute to others
Arduino LibrariesYou need at least two files for a library: Header file (.h) - The header file has definitionsfor the library Source file (.cpp) – The Functions within the ClassNote the Library Name, Folder name, .h and .cppfiles all need to have the same name
Arduino LibrariesLocation: s macOS: /Users/hansha/Documents/Arduino
Fahrenheit.hCreating Libraries/*Fahrenheit.h - Library convertingbetween Celsius and Fahrenheit.Created by Hans-Petter Halvorsen, 2018*/#ifndef Fahrenheit h#define Fahrenheit hFahrenheit.cpp/*Fahrenheit.cpp - Library converting betweenCelsius and Fahrenheit.Created by Hans-Petter Halvorsen, 2018*/#include "Fahrenheit.h"Fahrenheit::Fahrenheit(){#include "Arduino.h"}class Fahrenheit{public:Fahrenheit();float c2f(float Tc);float f2c(float Tf);};#endiffloat Fahrenheit::c2f(float Tc){float Tf;Tf Tc * 9/5 32;return Tf;}float Fahrenheit::f2c(float Tf){float Tc;Tc (Tf-32)*((float)5/9);return Tc;}
Creating Libraries
Testing the Library#include Fahrenheit.h Fahrenheit fahr;void setup(){float f;float c;Serial.begin(9600);c 0;f fahr.c2f(c);Serial.println(f);f 32;c fahr.f2c(f);Serial.println(c);}void loop(){}
Deploying the LibraryThe Arduino Libraries need to be in the following folder (but can be changed from \librariesHere you need to put your .h and .cppfiles.You should also create an “examples”folder where you include one or moreexamples showing how to use yourLibrary.
Using the LibraryWhen the Library hasbeen installedproperly, you shouldsee your Library under“Sketch- IncludeLibrary”Your Library Examplescan be found underFile- Examples
Using the Library
References Installing Additional Arduino s Writing a Library for utorial How to write libraries for the Arduino?http://playground.arduino.cc/Code/Library
Hans-Petter HalvorsenUniversity of South-Eastern Norwaywww.usn.noE-mail: hans.p.halvorsen@usn.noWeb: https://www.halvorsen.blog
C:\Users\hansha\Documents\Arduino\libraries The Arduino Libraries need to be in the following folder (but can be changed from File-Preferences): Here you need to put your .h and .cpp files. You should also create an “examples” folder where you incl