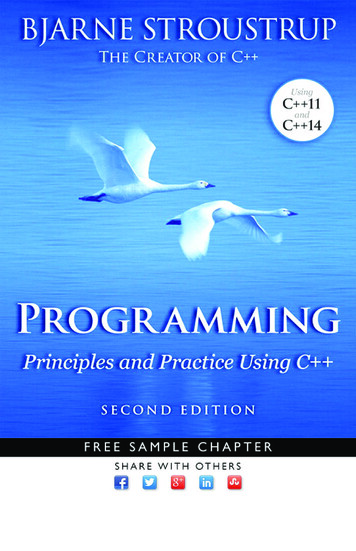
Transcription
ProgrammingSecond Edition
This page intentionally left blank
ProgrammingPrinciples and PracticeUsing C Second EditionBjarne StroustrupUpper Saddle River, NJ Boston Indianapolis San FranciscoNew York Toronto Montreal London Munich Paris MadridCapetown Sydney Tokyo Singapore Mexico City
Many of the designations used by manufacturers and sellers to distinguish their products areclaimed as trademarks. Where those designations appear in this book, and the publisher wasaware of a trademark claim, the designations have been printed with initial capital letters or inall capitals.A complete list of photo sources and credits appears on pages 1273–1274.The author and publisher have taken care in the preparation of this book, but make no expressed or implied warranty of any kind and assume no responsibility for errors or omissions.No liability is assumed for incidental or consequential damages in connection with or arisingout of the use of the information or programs contained herein.For information about buying this title in bulk quantities, or for special sales opportunities(which may include electronic versions; custom cover designs; and content particular to yourbusiness, training goals, marketing focus, or branding interests), please contact our corporatesales department at corpsales@pearsoned.com or (800) 382-3419.For government sales inquiries, please contact governmentsales@pearsoned.com.For questions about sales outside the United States, please contact international@pearsoned.com.Visit us on the Web: informit.com/awLibrary of Congress Cataloging-in-Publication DataStroustrup, Bjarne, author.Programming : principles and practice using C / Bjarne Stroustrup. — Second edition.pages cmIncludes bibliographical references and index.ISBN 978-0-321-99278-9 (pbk. : alk. paper)1. C (Computer program language) I. Title.QA76.73.C153S82 2014005.13'3—dc232014004197Copyright 2014 Pearson Education, Inc.All rights reserved. Printed in the United States of America. This publication is protectedby copyright, and permission must be obtained from the publisher prior to any prohibitedreproduction, storage in a retrieval system, or transmission in any form or by any means,electronic, mechanical, photocopying, recording, or likewise. To obtain permission to use material from this work, please submit a written request to Pearson Education, Inc., PermissionsDepartment, One Lake Street, Upper Saddle River, New Jersey 07458, or you may fax yourrequest to (201) 236-3290.ISBN-13: 978-0-321-99278-9ISBN-10: 0-321-99278-4Text printed in the United States on recycled paper at RR Donnelley in Crawfordsville, Indiana.Second printing, January 2015
ContentsPreface xxvChapter 0Notes to the Reader 10.1 The structure of this book0.1.10.1.20.1.30.2The order of topics 9Programming and programming languagePortability 1110Programming and computer science 12Creativity and problem solving 12Request for feedback 12References 13Biographies 13Bjarne Stroustrup 14Lawrence “Pete” PetersenChapter 15A philosophy of teaching and learning 60.2.10.2.20.2.30.30.40.50.60.72General approach 3Drills, exercises, etc. 4What comes after this book?15Computers, People, and Programming 171.1 Introduction 181.2 Software 191.3 People 211.4 Computer science 241.5 Computers are everywhere 251.5.11.5.21.5.31.5.4Screens and no screens 26Shipping 26Telecommunications 28Medicine 30v
CONTENTSvi1.5.51.5.61.5.71.6Part I The BasicsInformation 31A vertical view 33So what? 34Ideals for programmers3441Chapter 2Hello, World! 432.1 Programs 442.2 The classic first program 452.3 Compilation 472.4 Linking 512.5 Programming environments 52Chapter 3Objects, Types, and Values 593.1 Input 603.2 Variables 623.3 Input and type 643.4 Operations and operators 663.5 Assignment and initialization 693.5.13.63.6.13.73.83.9An example: find repeated wordsNames 74Types and objectsType safety 783.9.13.9.2Chapter 4An example: detect repeated words77Safe conversions 79Unsafe conversions 80Computation 894.1 Computation 904.2 Objectives and tools 924.3 Expressions 944.3.14.3.24.3.34.4Constant expressions 95Operators 97Conversions 99Statements 1004.4.14.4.24.571Composite assignment operators 73Selection 102Iteration 109Functions4.5.14.5.2113Why bother with functions?Function declarations 11711573
CONTENTSvii4.64.7Chapter 5vector4.6.14.6.24.6.34.6.4117Traversing a vector 119Growing a vector 119A numeric example 120A text example 123Language features125Errors 1335.1 Introduction 1345.2 Sources of errors 1365.3 Compile-time errors 1365.3.15.3.25.3.35.45.5Link-time errors 139Run-time errors 1405.5.15.5.25.5.35.6The caller deals with errors 142The callee deals with errors 143Error reporting 145Exceptions 1465.6.15.6.25.6.35.6.45.75.85.9Syntax errors 137Type errors 138Non-errors 139Bad arguments 147Range errors 148Bad input 150Narrowing errors 153Logic errors 154Estimation 157Debugging 1585.9.1Practical debug advice5.10 Pre- and post-conditions5.10.15.11 TestingChapter 6Post-conditions159163165166Writing a Program 1736.1 A problem 1746.2 Thinking about the problem 1756.2.16.2.26.3Stages of developmentStrategy 176176Back to the calculator! 1786.3.16.3.26.3.36.3.46.3.5First attempt 179Tokens 181Implementing tokens 183Using tokens 185Back to the drawing board 186
CONTENTSviii6.4Grammars 1886.4.16.4.26.5Turning a grammar into code6.5.16.5.26.5.36.5.46.66.76.8Chapter 7195Implementing grammar rulesExpressions 197Terms 200Primary expressions 202Implementing Token streamReading tokens 212Reading numbers 214Program structure196211215Completing a Program 2217.1Introduction 2227.2Input and output 2227.3Error handling 2247.4Negative numbers 2297.5Remainder: % 2307.6Cleaning up the code 2327.6.17.6.27.6.37.6.47.77.8Symbolic constants 232Use of functions 234Code layout 235Commenting 237Recovering from errorsVariables 2427.8.17.8.27.8.37.8.4Chapter 8193Trying the first version 203Trying the second version 208Token streams 2096.8.16.8.26.8.36.9A detour: English grammarWriting a grammar 194239Variables and definitions 242Introducing names 247Predefined names 250Are we there yet? 250Technicalities: Functions, etc. 2558.1 Technicalities 2568.2 Declarations and definitions 2578.2.18.2.28.2.3Kinds of declarations 261Variable and constant declarationsDefault initialization 263262
CONTENTSix8.38.48.5Header files 264Scope 266Function call and 98.68.7291Expression evaluation 292Global initialization 293Namespaces 2948.7.1Chapter 9Declaring arguments and return type 272Returning a value 274Pass-by-value 275Pass-by-const-reference 276Pass-by-reference 279Pass-by-value vs. pass-by-reference 281Argument checking and conversion 284Function call implementation 285constexpr functions 290Order of evaluation8.6.18.6.2272using declarations and using directives296Technicalities: Classes, etc. 3039.1 User-defined types 3049.2 Classes and members 3059.3 Interface and implementation 3069.4 Evolving a class 3089.4.19.4.29.4.39.4.49.4.59.4.6struct and functions 308Member functions and constructors 310Keep details private 312Defining member functions 314Referring to the current object 317Reporting errors 3179.5Enumerations 3189.69.7Operator overloadingClass interfaces 3239.5.19.7.19.7.29.7.39.7.49.7.59.8“Plain” enumerations 320321Argument types 324Copying 326Default constructors 327const member functions 330Members and “helper functions” 332The Date class 334
CONTENTSxPart II Input and OutputChapter 10343Input and Output Streams 34510.1 Input and output 34610.2 The I/O stream model 34710.3 Files 34910.4 Opening a file 35010.5 Reading and writing a file 35210.6 I/O error handling 35410.7 Reading a single value 35810.7.110.7.210.810.910.1010.11Breaking the problem into manageable partsSeparating dialog from function 362User-defined output operators 363User-defined input operators 365A standard input loop 365Reading a structured file 36710.11.1 In-memory representation 36810.11.2 Reading structured values 37010.11.3 Changing representations 374Chapter 11Customizing Input and Output 37911.1 Regularity and irregularity 38011.2 Output formatting 38011.2.111.2.211.2.311.2.411.2.5Integer output 381Integer input 383Floating-point outputPrecision 385Fields 38738411.3 File opening and hapter 12388File open modes 388Binary files 390Positioning in files 393String streams 394Line-oriented input 395Character classification 396Using nonstandard separators 398And there is so much more 406A Display Model 41112.1 Why graphics? 41212.2 A display model 41312.3 A first example 414359
CONTENTSxi12.412.512.612.7Using a GUI library 418Coordinates 419Shapes 420Using Shape primitives 812.7.912.7.10Graphics headers and main 421An almost blank window 422Axis 424Graphing a function 426Polygons 427Rectangles 428Fill 431Text 431Images 433And much more 43412.8 Getting this to run 43512.8.1Source files437Chapter 13Graphics Classes 44113.1 Overview of graphics classes 44213.2 Point and Line 44413.3 Lines 44713.4 Color 45013.5 Line style 45213.6 Open polyline 45513.7 Closed polyline 45613.8 Polygon 45813.9 Rectangle 46013.10 Managing unnamed objects 46513.11 Text 46713.12 Circle 47013.13 Ellipse 47213.14 Marked polyline 47413.15 Marks 47613.16 Mark 47813.17 Images 479Chapter 14Graphics Class Design 48714.1 Design principles 48814.1.114.1.214.1.314.1.4Types 488Operations 490Naming 491Mutability 492
CONTENTSxii14.2 Shape 49314.2.114.2.214.2.314.2.4An abstract class 495Access control 496Drawing shapes 500Copying and mutability 50314.3 Base and derived classes 50414.3.114.3.214.3.314.3.414.3.5Object layout 506Deriving classes and defining virtual functions 507Overriding 508Access 511Pure virtual functions 51214.4 Benefits of object-oriented programmingChapter 15513Graphing Functions and Data 51915.1 Introduction 52015.2 Graphing simple functions 52015.3 Function 52415.3.1 Default Arguments 52515.3.2 More examples 52715.3.3 Lambda expressions 528Axis 52915.415.5 Approximation 53215.6 Graphing data 53715.6.115.6.215.6.315.6.4Chapter 16Reading a file 539General layout 541Scaling data 542Building the graph 543Graphical User Interfaces 55116.1 User interface alternatives 55216.2 The “Next” button 55316.3 A simple window 55416.416.3.1 A callback function 55616.3.2 A wait loop 55916.3.3 A lambda expression as a callback 560Button and other Widgets 56116.4.1 Widgets 56116.4.2 Buttons 56316.4.3 In box and Out box 56316.4.4 Menus 56416.5 An example 565
CONTENTSxiii16.6 Control inversion 56916.7 Adding a menu 57016.8 Debugging GUI code 575Part III Data and AlgorithmsChapter 17581Vector and Free Store 58317.1 Introduction 58417.2 vector basics 58617.3 Memory, addresses, and pointers17.3.117.4Free store and 8590591Free-store allocation 593Access through pointers 594Ranges 595Initialization 596The null pointer 598Free-store deallocation 598Destructors17.5.117.5.217.617.717.817.9The sizeof operator601Generated destructors 603Destructors and free store 604Access to elements 605Pointers to class objects 606Messing with types: void* and casts 608Pointers and references 61017.9.1 Pointer and reference parameters 61117.9.2 Pointers, references, and inheritance 61217.9.3 An example: lists 61317.9.4 List operations 61517.9.5 List use 61617.10 The this pointer 61817.10.1 More link use 620Chapter 18Vectors and Arrays 62718.1 Introduction 62818.2 Initialization 62918.3 Copying 63118.3.118.3.218.3.318.3.4Copy constructors 633Copy assignments 634Copy terminology 636Moving 637
CONTENTSxiv18.4 Essential operations 64018.4.118.4.218.5Explicit constructors 642Debugging constructors and destructors 643Access to vector elements 64618.5.1 Overloading on const 64718.6 Arrays18.6.118.6.218.6.318.6.4648Pointers to array elementsPointers and arrays 652Array initialization 654Pointer problems 65618.7 Examples: palindrome18.7.118.7.218.7.3Chapter 19659Palindromes using string 659Palindromes using arrays 660Palindromes using pointers 661Vector, Templates, and Exceptions19.1 The problems 66819.2 Changing size tation 671reserve and capacity 673resize 674push back 674Assignment 675Our vector so far 67719.3 7650678Types as template parameters 679Generic programming 681Concepts 683Containers and inheritance 686Integers as template parameters 687Template argument deduction 689Generalizing vector 69019.4 Range checking and exceptions 69319.4.119.4.2An aside: design considerations 694A confession: macros 69619.5 Resources and otential resource management problems 698Resource acquisition is initialization 700Guarantees 701unique ptr 703Return by moving 704RAII for vector 705
CONTENTSChapter 20xvContainers and Iterators 71120.1 Storing and processing data20.1.120.1.220.2 STL ideals 71720.3 Sequences and iterators20.3.1712Working with data 713Generalizing code 714720Back to the example20.4 Linked lists72372420.4.120.4.2List operations 726Iteration 72720.5 Generalizing vector yet again 72920.5.1 Container traversal 73220.5.2 auto 73220.6 An example: a simple text editor73420.6.1 Lines 73620.6.2 Iteration 73720.7 vector, list, and string 74120.7.1 insert and erase 74220.8 Adapting our vector to the STL74520.9 Adapting built-in arrays to the STL 74720.10 Container overview 74920.10.1 Iterator categoriesChapter 21751Algorithms and Maps 75721.1 Standard library algorithms 75821.2 The simplest algorithm: find() 75921.2.1Some generic uses76121.3 The general search: find if()21.4 Function objects 76521.4.121.4.221.4.3An abstract view of function objectsPredicates on class members 767Lambda expressions 76921.5 Numerical algorithms21.5.121.5.221.5.321.5.4770Accumulate 770Generalizing accumulate()772Inner product 774Generalizing inner product()21.6 Associative containers21.6.121.6.221.6.321.6.421.6.5763776map 776map overview 779Another map exampleunordered map 785set 787782775766
CONTENTSxvi21.7 Copying21.7.121.7.221.7.321.7.4789Copy 789Stream iterators 790Using a set to keep ordercopy if 79479321.8 Sorting and searching 79421.9 Container algorithms 797Part IV Broadening the ViewChapter 22803Ideals and History 80522.1 History, ideals, and professionalism22.1.122.1.222.1.3806Programming language aims and philosophiesProgramming ideals 808Styles/paradigms 81522.2 Programming language history 22.2.8Chapter 23The earliest languages 819The roots of modern languagesThe Algol family 826Simula 833C 836C 839Today 842Information sources 844818821Text Manipulation 84923.1 Text 85023.2 Strings 85023.3 I/O streams 85523.4 Maps 85523.4.1Implementation details86123.5 A problem 86423.6 The idea of regular expressions23.6.1Raw string literals86686823.7 Searching with regular expressions23.8 Regular expression syntax aracters and special charactersCharacter classes 873Repeats 874Grouping 876Alternation 876Character sets and ranges 877Regular expression errors 878872807
CONTENTSxvii23.9 Matching with regular expressions23.10 References 885Chapter 24Numerics 88924.1 Introduction 89024.2 Size, precision, and overflow24.2.1Numeric limits89089424.3 Arrays 89524.4 C-style multidimensional arrays24.5 The Matrix library 89724.5.124.5.224.5.324.5.424.5.5880Dimensions and access1D Matrix 9012D Matrix 904Matrix I/O 9073D Matrix 90789689824.6 An example: solving linear r 25Classical Gaussian eliminationPivoting 911Testing 912Random numbers 914The standard mathematical functionsComplex numbers 919References 920908910917Embedded Systems Programming 92525.1 Embedded systems 92625.2 Basic concepts 92925.2.125.2.225.2.3Predictability 932Ideals 932Living with failure 93325.3 Memory management25.3.125.3.225.3.325.3.4935Free-store problems 936Alternatives to the general free storePool example 940Stack example 94225.4 Addresses, pointers, and cked conversions 943A problem: dysfunctional interfacesA solution: an interface class 947Inheritance and containers 95125.5 Bits, bytes, and words954Bits and bit operationsbitset 959939955944
CONTENTSxviii25.5.325.5.425.5.525.5.6Signed and unsigned 961Bit manipulation 965Bitfields 967An example: simple encryption25.6 Coding standards25.6.125.6.225.6.3Chapter 26974What should a coding standard be?Sample rules 977Real coding standards 983Testing 98926.1 What we want26.1.1969Caveat97599099126.2 Proofs 99226.3 Testing 99226.3.126.3.226.3.326.3.426.3.5Regression tests 993Unit tests 994Algorithms and non-algorithms 1001System tests 1009Finding assumptions that do not hold 100926.4 Design for testing 101126.5 Debugging 101226.6 Performance 101226.6.1Timing26.7 ReferencesChapter 2710151016The C Programming Language 102127.1 C and C : siblings 2.327.2.427.2.527.3C/C compatibility 1024C features missing from C 1025The C standard library 10271028No function name overloading 1028Function argument type checking 1029Function definitions 1031Calling C from C and C from C 1032Pointers to functions 1034Minor language differences103627.3.127.3.227.3.327.3.41036struct tag namespaceKeywords 1037Definitions 1038C-style casts 1040
CONTENTSxix27.3.527.3.627.3.727.427.5Free store 1043C-style strings 104527.5.127.5.227.5.327.5.427.61054Function-like macros 1056Syntax macros 1058Conditional compilation 1058An example: intrusive containersPart V Appendices10591071Appendix A Language SummaryA.1 General 1074A.1.1A.1.2A.1.3A.21050Output 1050Input 1052Files 1053Constants and macrosMacros 105527.8.127.8.227.8.327.9C-style strings and const 1047Byte operations 1048An example: strcpy() 1049A style issue 1049Input/output: stdio27.6.127.6.227.6.327.727.8Conversion of void* 1041enum 1042Namespaces 10421073Terminology 1075Program start and terminationComments 10761075Literals 1077A.2.1A.2.2A.2.3A.2.4A.2.5A.2.6Integer literals 1077Floating-point-literals 1079Boolean literals 1079Character literals 1079String literals 1080The pointer literal 1081A.3Identifiers 1081A.4Scope, storage class, and lifetime 1082A.3.1A.4.1A.4.2A.4.3Keywords1081Scope 1082Storage class 1083Lifetime 1085
CONTENTSxxA.5Expressions ments 1096Declarations 1098A.7.1A.8Definitions1098Built-in types 1099A.8.1A.8.2A.8.3A.9User-defined operators 1091Implicit type conversion 1091Constant expressions 1093sizeof 1093Logical expressions 1094new and delete 1094Casts 1095Pointers 1100Arrays 1101References 1102FunctionsA.9.1A.9.2A.9.3A.9.41103Overload resolution 1104Default arguments 1105Unspecified arguments 1105Linkage specifications 1106A.10 User-defined types1106A.10.1 Operator overloading 1107A.11 Enumerations 1107A.12 Classes 1108A.12.1A.12.2A.12.3A.12.4A.12.5A.12.6Member access 1108Class member definitions 1112Construction, destruction, and copyDerived classes 1116Bitfields 1120Unions 1121A.13 Templates1121A.13.1 Template arguments 1122A.13.2 Template instantiation 1123A.13.3 Template member types 1124A.14A.15A.16A.17Exceptions 1125Namespaces 1127Aliases 1128Preprocessor directivesA.17.1A.17.2#include 1128#define 112911281112
CONTENTSxxiAppendix B Standard Library SummaryB.1 Overview 1132B.1.1B.1.2B.1.3Header files 1133Namespace std 1136Description style 1136B.2Error handling 1137B.3Iterators 1139B.2.1B.3.1B.3.2B.4Overview 1146Member types 1147Constructors, destructors, and assignments 1148Iterators 1148Element access 1149Stack and queue operations 1149List operations 1150Size and capacity 1150Other operations 1151Associative container operations 11511162Inserters 1162Function objects 1163pair and tuple 1165initializer list 1166Resource management pointersI/O streamsB.7.1B.7.2B.7.31152Nonmodifying sequence algorithms 1153Modifying sequence algorithms 1154Utility algorithms 1156Sorting and searching 1157Set algorithms 1159Heaps 1160Permutations 1160min and max 1161STL utilitiesB.6.1B.6.2B.6.3B.6.4B.6.5B.71138Iterator model 1140Iterator categories .5.8B.6ExceptionsContainers .4.10B.511311168I/O streams hierarchy 1170Error handling 1171Input operations 11721167
CONTENTSxxiiB.7.4B.7.5B.7.6B.8String manipulation 1175B.8.1B.8.2B.8.3B.9Output operations 1173Formatting 1173Standard manipulators 1173Character classification 1175String 1176Regular expression matching 1177Numerics 1180B.9.1B.9.2B.9.3B.9.4B.9.5B.9.6Numerical limits 1180Standard mathematical functions 1181Complex 1182valarray 1183Generalized numerical algorithms 1183Random numbers 1184B.10 Time 1185B.11 C standard library functions 1185B.11.1B.11.2B.11.3B.11.4B.11.5B.10.6Files 1186The printf() family 1186C-style strings 1191Memory 1192Date and time 1193Etc. 1194B.12 Other libraries 1195Appendix C Getting Started with Visual Studio 1197C.1 Getting a program to run 1198C.2 Installing Visual Studio 1198C.3 Creating and running a program 1199C.3.1C.3.2C.3.3C.3.4C.3.5C.3.6C.3.7C.4Create a new project 1199Use the std lib facilities.h header file 1199Add a C source file to the project 1200Enter your source code 1200Build an executable program 1200Execute the program 1201Save the program 1201Later 1201Appendix D Installing FLTK 1203D.1 Introduction 1204D.2 Downloading FLTK 1204D.3 Installing FLTK 1205D.4 Using FLTK in Visual Studio 1205D.5 Testing if it all worked 1206
CONTENTSAppendix E GUI Implementation 1207E.1 Callback implementation 1208E.2 Widget implementation 1209E.3 Window implementation 1210E.4 Vector ref 1212E.5 An example: manipulating WidgetsGlossary 1217Bibliography 1223Index 1227xxiii1213
This page intentionally left blank
Preface“Damn the torpedoes!Full speed ahead.”—Admiral FarragutProgramming is the art of expressing solutions to problems so that a computercan execute those solutions. Much of the effort in programming is spent findingand refining solutions. Often, a problem is only fully understood through theprocess of programming a solution for it.This book is for someone who has never programmed before but is willingto work hard to learn. It helps you understand the principles and acquire thepractical skills of programming using the C programming language. My aimis for you to gain sufficient knowledge and experience to perform simple usefulprogramming tasks using the best up-to-date techniques. How long will that take?As part of a first-year university course, you can work through this book in a semester (assuming that you have a workload of four courses of average difficulty).If you work by yourself, don’t expect to spend less time than that (maybe 15hours a week for 14 weeks).Three months may seem a long time, but there’s a lot to learn and you’llbe writing your first simple programs after about an hour. Also, all learning isgradual: each chapter introduces new useful concepts and illustrates them withexamples inspired by real-world uses. Your ability to express ideas in code — getting a computer to do what you want it to do — gradually and steadily increasesas you go along. I never say, “Learn a month’s worth of theory and then see ifyou can use it.”xxv
xxviPREFACEWhy would you want to program? Our civilization runs on software. Without understanding software you are reduced to believing in “magic” and will belocked out of many of the most interesting, profitable, and socially useful technicalfields of work. When I talk about programming, I think of the whole spectrum ofcomputer programs from personal computer applications with GUIs (graphicaluser interfaces), through engineering calculations and embedded systems controlapplications (such as digital cameras, cars, and cell phones), to text manipulationapplications as found in many humanities and business applications. Like mathematics, programming — when done well — is a valuable intellectual exercise thatsharpens our ability to think. However, thanks to feedback from the computer,programming is more concrete than most forms of math, and therefore accessibleto more people. It is a way to reach out and change the world — ideally for thebetter. Finally, programming can be great fun.Why C ? You can’t learn to program without a programming language, andC directly supports the key concepts and techniques used in real-world software. C is one of the most widely used programming languages, found in anunsurpassed range of application areas. You find C applications everywherefrom the bottom of the oceans to the surface of Mars. C is precisely and comprehensively defined by a nonproprietary international standard. Quality and/or free implementations are available on every kind of computer. Most of theprogramming concepts that you will learn using C can be used directly inother languages, such as C, C#, Fortran, and Java. Finally, I simply like C asa language for writing elegant and efficient code.This is not the easiest book on beginning programming; it is not meant tobe. I just aim for it to be the easiest book from which you can learn the basics ofreal-world programming. That’s quite an ambitious goal because much modernsoftware relies on techniques considered advanced just a few years ago.My fundamental assumption is that you want to write programs for the use ofothers, and to do so responsibly, providing a decent level of system quality; that is,I assume that you want to achieve a level of professionalism. Consequently, I chosethe topics for this book to cover what is needed to get started with real-world programming, not just what is easy to teach and learn. If you need a technique to getbasic work done right, I describe it, demonstrate concepts and language facilitiesneeded to support the technique, provide exercises for it, and expect you to workon those exercises. If you just want to understand toy programs, you can get alongwith far less than I present. On the other hand, I won’t waste your time with material of marginal practical importance. If an idea is explained here, it’s becauseyou’ll almost certainly need it.If your desire is to use the work of others without understanding how thingsare done and without adding significantly to the code yourself, this book is not foryou. If so, please consider whether you would be better served by another bookand another language. If that is approximately your view of programming, please
PREFACEalso consider from where you got that view and whether it in fact is adequate foryour needs. People often underestimate the complexity of programming as well asits value. I would hate for you to acquire a dislike for programming because of amismatch between what you need and the part of the software reality I describe.There are many parts of the “information technology” world that do not requireknowledge of programming. This book is aimed to serve those who do want towrite or understand nontrivial programs.Because of its structure and practical aims, this book can also be used as asecond book on programming for someone who already knows a bit of C orfor someone who programs in another language and wants to learn C . If youfit into one of those categories, I refrain from guessing how long it will take you toread this book, but I do encourage you to do many of the exercises. This will helpyou to counteract the common problem of writing programs in older, familiarstyles rather than adopting newer techniques where these are more appropriate. Ifyou have learned C in one of the more traditional ways, you’ll find somethingsurprising and useful before you reach Chapter 7. Unless your name is Stroustrup, what I discuss here is not “your father’s C .”Programming is learned by writing programs. In this, programming is similarto other endeavors with a practical component. You cannot learn to swim, to playa musical instrument, or to drive a car just from reading a book — you must practice. Nor can you learn to program without reading and writing lots of code. Thisbook focuses on code examples closely tied to explanatory text and diagrams. Youneed those to understand the ideals, concepts, and principles of programming andto master the language constructs used to express them. That’s essential, but byitself, it will not give you the practical skills of programming. For that, you needto do the exercises and get used to the tools for writing, compiling, and runningprograms. You need to make your own mistakes and learn to correct them. Thereis no substitute for writing code. Besides, that’s where the fun is!On the other hand, there is more to programming — much more — than following a few rules and reading the manual. This book is emphatically not focusedon “the syntax of C .” Understanding the fundamental ideals, principles, andtechniques is the essence of a good programmer. Only well-designed code has achance of becoming part of a correct, reliable, and maintainable system. Also, “thefundamentals” are what last: they will still be essential after today’s languages andtools have evolved or been replaced.What about computer science, software engineering, information technology,etc.? Is that all programming? Of course not! Programming is one of the fundamental topics that underlie everything in computer-related fields, and it has a natural place in a balanced course of computer science. I provide brief introductionsto key concepts and techniques of algorithms, data structures, user interfaces, dataprocessing, and software engineering. However, this book is not a substitute for athorough and balanced study of those topics.xxvii
PREFACExxviiiCode can be beautiful as well as useful. This book is written to help you seethat, to understand what it means for code to be beautiful, a
0.2.2 Programming and programming language 10 0.2.3 Portability 11 0.3 Programming and computer science 12 0.4 Creativity and problem solving 12 0.5 Request for feedback 12 0.6 References 13 0.7 Biographies 13 Bjarne Stroustrup 14 Lawrence “Pete” Petersen 15 Chapter 1 Computers, People, and Programming 17 1.1 Introduction 18 1.2 Software 19File Size: 1MBPage Count: 137