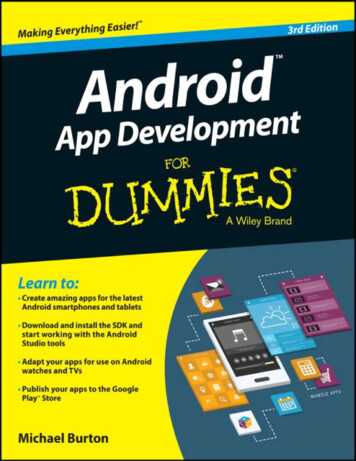
Transcription
Android Application Development For Dummies , 3rd EditionPublished by: John Wiley & Sons, Inc., 111 River Street, Hoboken, NJ 07030 5774,www.wiley.comCopyright 2015 by John Wiley & Sons, Inc., Hoboken, New JerseyPublished simultaneously in CanadaNo part of this publication may be reproduced, stored in a retrieval system or transmitted in anyform or by any means, electronic, mechanical, photocopying, recording, scanning or otherwise,except as permitted under Sections 107 or 108 of the 1976 United States Copyright Act, withoutthe prior written permission of the Publisher. Requests to the Publisher for permission should beaddressed to the Permissions Department, John Wiley & Sons, Inc., 111 River Street, Hoboken,NJ 07030, (201) 748 6011, fax (201) 748 6008, or online athttp://www.wiley.com/go/permissions.Portions of this page are based on work created and shared by the Android Open Source Projectand used according to terms described in the Creative Commons 2.5 Attribution License.Trademarks: Wiley, For Dummies, the Dummies Man logo, Dummies.com, Making EverythingEasier, and related trade dress are trademarks or registered trademarks of John Wiley & Sons, Inc.and may not be used without written permission. Android is a trademark of Google, Inc. All othertrademarks are the property of their respective owners. John Wiley & Sons, Inc. is not associatedwith any product or vendor mentioned in this book.LIMIT OF LIABILITY/DISCLAIMER OF WARRANTY: THE PUBLISHER AND THEAUTHOR MAKE NO REPRESENTATIONS OR WARRANTIES WITH RESPECT TOTHE ACCURACY OR COMPLETENESS OF THE CONTENTS OF THIS WORK ANDSPECIFICALLY DISCLAIM ALL WARRANTIES, INCLUDING WITHOUTLIMITATION WARRANTIES OF FITNESS FOR A PARTICULAR PURPOSE. NOWARRANTY MAY BE CREATED OR EXTENDED BY SALES OR PROMOTIONALMATERIALS. THE ADVICE AND STRATEGIES CONTAINED HEREIN MAY NOT BESUITABLE FOR EVERY SITUATION. THIS WORK IS SOLD WITH THEUNDERSTANDING THAT THE PUBLISHER IS NOT ENGAGED IN RENDERINGLEGAL, ACCOUNTING, OR OTHER PROFESSIONAL SERVICES. IFPROFESSIONAL ASSISTANCE IS REQUIRED, THE SERVICES OF A COMPETENTPROFESSIONAL PERSON SHOULD BE SOUGHT. NEITHER THE PUBLISHER NORTHE AUTHOR SHALL BE LIABLE FOR DAMAGES ARISING HEREFROM. THEFACT THAT AN ORGANIZATION OR WEBSITE IS REFERRED TO IN THIS WORKAS A CITATION AND/OR A POTENTIAL SOURCE OF FURTHER INFORMATIONDOES NOT MEAN THAT THE AUTHOR OR THE PUBLISHER ENDORSES THEINFORMATION THE ORGANIZATION OR WEBSITE MAY PROVIDE ORRECOMMENDATIONS IT MAY MAKE. FURTHER, READERS SHOULD BE AWARETHAT INTERNET WEBSITES LISTED IN THIS WORK MAY HAVE CHANGED ORDISAPPEARED BETWEEN WHEN THIS WORK WAS WRITTEN AND WHEN IT ISREAD.
For general information on our other products and services, please contact our Customer CareDepartment within the U.S. at 877 762 2974, outside the U.S. at 317 572 3993, or fax 317 5724002. For technical support, please visit www.wiley.com/techsupport.Wiley publishes in a variety of print and electronic formats and by print on demand. Somematerial included with standard print versions of this book may not be included in e books or inprint on demand. If this book refers to media such as a CD or DVD that is not included in theversion you purchased, you may download this material at http://booksupport.wiley.com.For more information about Wiley products, visit www.wiley.com.Library of Congress Control Number: 2014954664
Android Application Development ForDummies Visit www.dummies.com/cheatsheet/androidappdevelopment toview this book's cheat sheet.Table of ContentsCoverIntroductionAbout This BookConventions Used in This BookFoolish AssumptionsHow This Book Is OrganizedIcons Used in This BookBeyond the BookPart I: Getting Started with Your First Android ApplicationChapter 1: Developing Spectacular Android ApplicationsWhy Develop for Android?Android Development BasicsHardware ToolsSoftware ToolsChapter 2: Prepping Your Development HeadquartersDeveloping the Android Developer Inside YouAssembling Your ToolkitTuning Up Your HardwareInstalling and Configuring Your Support ToolsInstalling Android StudioInstalling Java 7Adding SDK PackagesNavigating the Android SDKSpecifying Android PlatformsUsing SDK Tools for Everyday DevelopmentPart II: Building and Publishing Your First ApplicationChapter 3: Your First Android ProjectStarting a New Project in Android StudioResponding to Errors
Setting Up an EmulatorRunning the Hello Android AppUnderstanding Project StructureChapter 4: Creating the User InterfaceCreating the Silent Mode Toggle ApplicationLaying Out the ApplicationAdding an Image to Your ApplicationCreating a Launcher Icon for the ApplicationPreviewing the Application in the Visual DesignerChapter 5: Coding Your ApplicationUnderstanding Activities and the Activity LifecycleCreating Your First ActivityWorking with the Android Framework ClassesInstalling Your ApplicationMaterial DesignUh Oh! (Responding to Errors)Thinking Beyond the Application BoundariesChapter 6: Understanding Android ResourcesUnderstanding ResourcesWorking with ResourcesDifferent Strokes for Different Folks: Using Resource Qualifier DirectoriesChapter 7: Turning Your Application into an App WidgetWorking with App Widgets in AndroidWorking with Intents and Pending IntentsCreating the App WidgetPlacing Your Widget on the Home ScreenChapter 8: Publishing Your App to the Google Play StoreCreating a Distributable FileCreating a Google Play Developer ProfilePricing Your ApplicationGetting Screen Shots for Your ApplicationUploading Your Application to the Google Play StoreWatching the Number of Installs SoarPart III: Creating a Feature Rich ApplicationChapter 9: Designing the Tasks ApplicationReviewing the Basic RequirementsCreating the Application’s ScreensChapter 10: Creating the Task Detail Page
Creating the TaskEditActivityLinking the List View to the Edit ViewCreating the TaskEditFragmentYou Put the Fragment in the Activity and Shake It All UpUpdating the StylesA Special BonusChapter 11: Going a la Carte with Your MenuUnderstanding Options and Context MenusCreating Your First MenuCreating a Long Press ActionChapter 12: Handling User InputCreating the User Input InterfaceGetting Choosy with Dates and TimesCreating an Alert DialogValidating InputChapter 13: Getting Persistent with Data StorageFinding Places to Put DataUnderstanding How the SQLite ContentProvider WorksCreating Your Application’s SQLite DatabaseUsing ContentProvider URIsDealing with CRUDImplementing the Save ButtonImplementing the List ViewReading Data into the Edit PageChapter 14: Reminding the UserSeeing Why You Need AlarmManagerAsking the User for PermissionWaking Up a Process with AlarmManagerUpdating a NotificationClearing a NotificationRebooting DevicesChapter 15: Working with Android PreferencesUnderstanding the Android Preferences FrameworkUnderstanding the PreferenceFragment ClassCreating Your Preferences ScreenWorking with the PreferenceFragment ClassWorking with Preferences in Your Activities at RuntimePart IV: Android Is More than PhonesChapter 16: Developing for Tablets
Considering the Differences between Phones and TabletsTweaking the Tasks App for TabletsConfiguring a Tablet EmulatorCreating a New Product FlavorCreating an AndroidManifest for PhonesCreating an AndroidManifest for TabletsMaking the TaskListAndEditorActivity for TabletsBuilding the Tablet AppAdding the App CallbacksOne More Thing . . .Chapter 17: Supporting Older Versions of AndroidUnderstanding AppCompatUpdating the build FileAdding the ToolbarUsing the AppCompat ThemeTesting Your AppWorking with Right to Left LanguagesFixing the Add Task MenuFixing the Window OptionsUsing Newer APIsUsing Android LintChapter 18: Wearing the Tasks AppPreparing Your Development EnvironmentCreating a New Wear AppPublishing the Data from Your PhoneRunning the App without Android StudioPackaging the AppWhat’s Next?Chapter 19: Look Ma, I’m on TV!Understanding Guidelines for Building TV AppsBuilding and Manifesting ChangesAdding the BrowseActivityCreating the TV Browse FragmentCreating the CardPresenterRunning Your AppAdding and Editing ItemsCreating BackgroundsCreating More FiltersChapter 20: Moving beyond Google
Working around Google FeaturesSetting Up the Fire SDKSetting Up Your Fire or EmulatorPublishing to Amazon Appstore for AndroidPart V: The Part of TensChapter 21: Ten Free Sample Applications and SDKsAndroid SamplesThe Google I/O AppK 9 MailGitHub Android AppFacebook SDK for AndroidNotepad TutorialU 2020Lollipop Easter EggAndroid BootstrapThe AOSPChapter 22: Ten Tools to Simplify Your Development LifeAndroid LintAndroid SystraceRoboGuice and DaggerTranslator ToolkitHierarchy ViewerUI/Application Exerciser MonkeyGit and GitHubPicasso and OkHttpMemory Analyzer ToolTravis ciAbout the AuthorCheat SheetConnect with DummiesEnd User License Agreement
IntroductionWelcome to Android Application Development For Dummies!When Android was acquired by Google in 2005 (yes, Android was a start up company at onepoint), a lot of people didn’t have much interest in it because Google hadn’t yet entered the mobilespace. Fast forward to a few years later, when Google announced its first Android phone: the G1.It was the start of something huge.The G1 was the first publicly released Android device. It didn’t match the rich feature set of theiPhone at the time, but a lot of people believed in the platform. As soon as Donut (Android 1.6)was released, it was evident that Google was putting some effort into the product. Immediatelyafter version 1.6 was released, talk of 2.0 was already on the horizon.Today, we’re on version 5.0 of the Android platform, with no signs that things are slowing down.Without doubt, this is an exciting time in Android development.About This BookAndroid Application Development For Dummies is a beginner’s guide to developing Androidapplications. You don’t need any Android application development experience under your belt toget started.The Android platform is a device independent platform, which means that you can developapplications for various devices. These devices include, but aren’t limited to phones, watches,tablets, cars, e book readers, netbooks, televisions, and GPS devices.Finding out how to develop for the Android platform opens a large variety of development optionsfor you. This book distills hundreds, if not thousands, of pages of Android documentation, tips,tricks, and tutorials into a short, digestible format that allows you to springboard into your futureas an Android developer. This book isn’t a recipe book, but it gives you the basic knowledge toassemble various pieces of the Android framework to create interactive and compellingapplications.Conventions Used in This BookThroughout the book, you use the Android framework classes, and you’ll create Java classes andXML files.Code examples in this book appear in a monospace font so that they stand out from other text in thebook. This means that the code you’ll see looks like this:public class MainActivityJava is a high level programming language that is case sensitive, so be sure to enter the text intothe editor exactly as you see it in the book. The examples follow standard Java conventions so youcan transition easily between the book examples and the example code provided by the Android
Software Development Kit (SDK). All class names, for example, appear in PascalCaseformat.All the URLs in the book appear in monospace font as well:http://d.android.comFoolish AssumptionsTo begin programming with Android, you need a computer that runs one of the following operatingsystems:Windows 2003, Vista, 7 or 8Mac OS X 10.8.5 or laterLinux GNOME or KDEYou also need to download Android Studio (which is free) and the Java Development Kit (orJDK, which is also free), if you don’t already have them on your computer. Chapter 2 outlines theentire installation process for all the tools and frameworks.Because Android applications are developed in the Java programming language, you need tounderstand the Java language. Android also uses XML quite heavily to define various resourcesinside the application, so you should understand XML too. You don’t have to be an expert in theselanguages, however.You don’t need a physical Android device, because all the applications you build in this book willwork on an emulator.
How This Book Is OrganizedAndroid Application Development For Dummies has five parts, described in the followingsections.Part I: Getting Started with Your First Android ApplicationPart I introduces the tools and frameworks that you use to develop Android applications. It alsointroduces the various SDK components and shows you how they’re used in the Androidecosystem.Part II: Building and Publishing Your First Android ApplicationPart II introduces you to building your first Android application: the Silent Mode Toggleapplication. After you build the initial application, you create an app widget for the applicationthat you can place on the Home screen of an Android device. Then you publish your application tothe Google Play Store.Part III: Creating a Feature Rich ApplicationPart III takes your development skills up a notch by walking you through the construction of theTasks application, which allows users to create various tasks with reminders. You implement anSQLite backed content provider in this multiscreen application. You also see how to use theAndroid status bar to create notifications that can help increase the usability of your application.Part IV: Android Is More than PhonesPart IV takes the phone app you built in Part III and tweaks it to work on lots of other devices,including tablets, wearables, televisions, and the Amazon Fire.Part V: The Part of TensPart V gives you a tour of sample applications that prove to be stellar launching pads for yourAndroid apps, and useful Android libraries that can make your Android development career a loteasier.Icons Used in This BookThis icon indicates a useful pointer that you shouldn’t skip.This icon represents a friendly reminder about a vital point you should keep in mind whileproceeding through a particular section of the chapter.This icon signifies that the accompanying explanation may be informative but isn’t
essential to understanding Android application development. Feel free to skip these snippets,if you like.This icon alerts you to potential problems that you may encounter along the way. Read andremember these tidbits to avoid possible trouble.This icon signifies that you’ll find additional relevant content ond the BookIn addition to the content in this book, you’ll find some extra content available at thewww.dummies.com website:The Cheat Sheet for this book at line articles covering additional topics atwww.dummies.com/extras/androidappdevelopmentHere you’ll find the articles referred to on the page that introduces each part of the book. So,feel free to visit www.dummies.com/extras/androidappdevelopment. You’ll feel at homethere . . . find coffee and donuts . . . okay, maybe not the coffee and donuts, but you can findcool supplementary information about things we couldn’t fit into the book, such as testing, GPSlocation tracking, voice control, and other fun topics.Updates to this book, if any, at t want to type all the code in the book? You can download it from the book’s website atwww.dummies.com/go/androidappdevfd.If there are ever updates to this book, you can find them atwww.dummies.com/go/androidappdevfdupdates.
Part IGetting Started with Your First AndroidApplicationVisit www.dummies.com for great Dummies content online.
In this part . . .Part I introduces you to the Android platform and describes what makes a spectacular Androidapplication. You explore various parts of the Android software development kit (SDK) and seehow to use them in your applications. You install the tools and frameworks necessary to developAndroid applications.
Chapter 1Developing Spectacular AndroidApplicationsIn This ChapterSeeing reasons to develop Android appsStarting with the basics of Android developmentWorking with the hardwareGetting familiar with the softwareGoogle rocks! Google acquired the Android platform in 2005 (see the sidebar “The roots ofAndroid,” later in this chapter) to ensure that a mobile operating system (OS) can be created andmaintained in an open platform. Google continues to pump time and resources into the Androidproject. Though devices have been available only since October 2008, over a billion Androiddevices have now been activated, and more than a million more are being added daily. In only afew years, Android has already made a huge impact.It has never been easier for Android developers to make money by developing apps. Androidusers trust Google, and because your app resides in the Google Play Store, many users will bewilling to trust your app, too.Why Develop for Android?The real question is, “Why not develop for Android?” If you want your app to be available tomillions of users worldwide or if you want to publish apps as soon as you finish writing andtesting them or if you like developing on an open platform, you have your answer. But in caseyou’re still undecided, continue reading.Market shareAs a developer, you have an opportunity to develop apps for a booming market. The number ofAndroid devices in use is greater than the number of devices on all other mobile operating systemscombined. The Google Play Store puts your app directly and easily into a user’s hands. Usersdon’t have to search the Internet to find an app to install — they can simply go to the preinstalledGoogle Play Store on their devices and have access to all your apps. Because the Google PlayStore comes preinstalled on most Android devices (see Chapter 19 for some exceptions), userstypically search the Google Play Store for all their application needs. It isn’t unusual to see anapp’s number of downloads soar in only a few days.
Time to marketBecause of all the application programming interfaces (APIs) packed into Android, you can easilydevelop full featured applications in a relatively short time frame. After you register as adeveloper at the Google Play Store, simply upload your apps and publish them. Unlike othermobile marketplaces, the Google Play Store has no app approval process. All you have to do iswrite apps and publish them.Though anyone can publish almost any type of app, maintain your good karma — and yourcompliance with the Google terms of service — by producing family friendly apps. Androidhas a diverse set of users from all over the world and of all ages.Open platformThe Android operating system is an open platform: Any hardware manufacturer or provider canmake or sell Android devices. As you can imagine, the openness of Android has allowed it to gainmarket share quickly. Feel free to dig into the Android source code to see how it works, byvisiting https://source.android.com. By using open source code, manufacturers can createcustom user interfaces (UIs) and even add new features to certain devices.Device compatibilityAndroid can run on devices of many different screen sizes and resolutions, including watches,phones, tablets, televisions, and more. Android comes supplied with tools to help you developapplications that support multiple types of devices. If your app requires a front facing camera, forexample, only devices with front facing cameras can “see” your app in the Google Play Store —an arrangement known as feature detection. (For more information on publishing your apps to theGoogle Play Store, see Chapter 8.)The roots of AndroidThough most people aren’t aware of it, Google didn’t start the Android project. The first version of the Android operatingsystem was created by Android, Inc., a small start up company in Silicon Valley that was purchased by Google inAugust 2005. The founders (who worked for various Internet technology companies, such as Danger, WildfireCommunications, T Mobile, and WebTV) became part of the Google team that helped create what is now the full fledgedAndroid mobile operating system.Mashup capabilityA mashup combines two or more services to create an application. You can create a mashup byusing the camera and the Android location services, for example, to take a photo with the exactlocation displayed on the image. Or you can use the Map API with the Contacts list to show allcontacts on a map. You can easily make apps by combining services or libraries in countless newand exciting ways. A few other types of mashups that can help your brain juices start pumping outideas include the following:
Geolocation and social networking: Suppose that you want to write an app that tweets auser’s current location every ten minutes throughout the day. Using the Android locationservices and a third party Twitter API (such as iTwitter), you can do it easily.Geolocation and gaming: Location based gaming, which is increasingly popular, is a helpfulway to inject players into the thick of a game. A game might run a background service to checka player’s current location and compare it with other players’ locations in the same area. If asecond player is within a specified distance, the first one could be notified to challenge her toa battle. All this is possible because of GPS technology on a strong platform such as Android.If you’re interested in developing games for Android, check outhttps://developers.google.com/games/services/ for more information about GooglePlay Games services.Contacts and Internet: With all the useful APIs at your disposal, you can easily make fullfeatured apps by combining the functionality of two or more APIs. You can combine theInternet and names from the Contacts list to create a greeting card app, for example. Or youmay simply want to add an easy way for users to contact you from an app or enable them tosend your app to their friends. (See “Google APIs,” later in this chapter, for more informationon the APIs.)Developers can make Android do almost anything they want, so use your best judgmentwhen creating and publishing apps for mass consumption. Just because you want livewallpaper to highlight your version of the hula in your birthday suit doesn’t mean that anyoneelse wants to see it.Android Development BasicsThank goodness you don’t have to be a member of Mensa to develop Android applications!Developing in Android is simple because its default language is Java. Though writing Androidapplications is fairly easy, writing code in general is no easy feat.If you’ve never developed applications before, this book may not be the best place tostart. Pick up a copy of Beginning Programming with Java For Dummies, by Barry Burd(John Wiley & Sons, Inc.) to learn the ropes. After you have a basic understanding of Javaunder your belt, you should be ready to tackle this book.Although the Android operating system consists primarily of Java code, some of the frameworkisn’t written in Java. Android apps use small amounts of XML in addition to Java. You need tocement your basic understanding of XML before delving into this book.If you need an introduction to XML, check out XML For Dummies, by Lucinda Dykes and
Ed Tittel (John Wiley & Sons, Inc.).If you already know how to use Java and XML, then congratulations — you’re ahead of the curve.Java: Your Android programming languageAndroid applications are written in Java — not the full blown version of Java that’s familiar todevelopers using Java Platform, Enterprise Edition (JEE), but a subset of the Java libraries thatare most useful on Android. This smaller subset of Java excludes classes that aren’t suitable formobile devices. If you have experience in Java, you should feel right at home developing apps inAndroid.Even with a Java reference book on hand, you can always search at www.google.com orwww.stackoverflow.com to find information about topics you don’t understand. Because Javaisn’t a new language, you can find plenty of examples on the web that demonstrate how to dovirtually anything.Not every class that’s available to Java programmers is also available on Android. Verifythat it’s available to you before you start trying to use it. If it’s not, an alternative is probablybundled with Android that can work for your needs.ActivitiesAn Android application can consist of one or more activities. An activity serves as a container forboth the user interface and the code that runs it. You can think of activities as pages of your app —one page in your app corresponds to one activity. Activities are discussed in more detail inChapters 3 and 5.FragmentsEvery “page” in an Android application is a separate activity. In older versions of Android, youwould place any element that you wanted to display onscreen directly into the Activity class.This arrangement works well when viewed on a phone’s small screen, on which you typicallycan’t see a lot of information at once. You may be able to see a list of tasks, or a task that you’reediting, but cramming both elements onto the screen at the same time is impossible.On a tablet, however, you’re swimming in real estate. Not only does it make sense to let users seea list of tasks and edit them on the same page, but it also looks silly not to let them do so. Thescreen size on a tablet is simply too big to fill with a single long list of items or lots of emptyspace.Android doesn’t allow you to easily put two activities on the screen at the same time. What to do?The answer is fragments.Using fragments, a single list fragment can occupy half the screen, and an edit fragment can occupythe other half. Now each page of your app can contain multiple fragments. You can find out how touse fragments in your phone application in Chapter 9 and how to scale your app to tablets inChapter 17.
You can think of fragments as miniature activities: Because every fragment has its ownlifecycle, you know when it’s being created and destroyed, among other information.Fragments go inside activities.IntentsIntents make up the core message system that runs Android. An intent is composed of twoelements:An action: The general action to be performed (such as view, edit, or dial) when the intent isreceivedData: The information that the action operates on, such as the name of a contactIntents are used to start activities and to communicate among various parts of the Androidoperating system. An application can send and receive intents.Sending messages with intentsWhen you send an intent, you send a message telling Android to make something happen. Theintent can tell Android to start a new activity from within your application or to start anotherapplication.Registering intent filtersSending an intent doesn’t make something happen automatically. You have to register an intentfilter that listens for the intent and then tells Android what to do — whether the task is starting anew activity or another app. If more than one receiver can accept a given intent, a chooser can becreated to allow the user to decide which app to use to complete the activity — such as how theYouTube app allows the user to choose whether to watch videos in the YouTube app or in abrowser.Various registered receivers, such as the Gmail and the Hangouts apps, handle image sharingintents by default. When you find more than one possible intent filter, a chooser opens with a listof options to choose from and asks what to do: Use email, messaging, or another application, asshown in Figure 1-1.
Figure 1 1: A chooser.Follow best practice and create choosers for intents that don’t target other activitieswithin your application. If the Android system cannot find a match for an intent that was sent,and if a chooser wasn’t created manually, the application crashes after experiencing aruntime exception — an unhandled error in the application. (Android expects developers toknow what they’re doing.) nding.html for moreinformation about using intent choosers.Cursorless controls
Cursorless controlsUnlike the PC, where you manipulate the mouse to move the cursor, an Android device lets youuse your fingers to do nearly anything you can do with a mouse. Rather than right click in Android,however, you long press an element until its context menu appears.As a developer, you can create and manipulate context menus. You can allow users to use twofingers on an Android device, rather than a single mouse cursor, for example. Fingers come in allsizes, so design the user interface in your apps accordingly. Buttons should be large enough (andhave sufficient spacing) so that even users with larger fingers can interact with your apps easily,whether they’re using your app on a phone or tablet.ViewsA view, which is a basic element of the Android user interface, is a rectangular area of the screenthat’s responsible for drawing and event handling. Views are a basic building block of Androiduser interfaces, much like paragraph p or anchor a tags are building blocks of an HTMLpage. Some common views you might use in an Android application might be a TextView,ImageView, Layout, and Button, but there are dozens more out there for you to explore.You can also implement your own custom views.Many more views are ready for you to use. For complete details about views, check out theandroid.widget and android.view packages in the Android documentation ckage summary.html.Background operationsThere are various ways to run multiple operations at the same time on Android without having tomanage a thread yourself (which is generally not recommended). When loading data from adatabase to show on the screen, you’ll generally find yourself using loaders. Loaders take care ofmanaging background threads for you, and they also watch your database for changes so that yourUI updates when the data changes. You can find out more about loaders in
Welcome to Android Application Development For Dummies! When Android was acquired by Google in 2005 (yes, Android was a startup company at one point), a lot of people didn’t have much interest in it because Google hadn’t yet entered the mobile space. Fastforward to a few years late