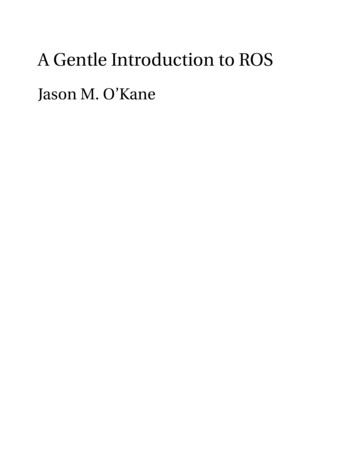
Transcription
A Gentle Introduction to ROSJason M. O’Kane
Jason M. O’KaneUniversity of South CarolinaDepartment of Computer Science and Engineering315 Main StreetColumbia, SC 29208http://www.cse.sc.edu/ jokane 2014, Jason Matthew O’Kane. All rights reserved.This is version 2.1.6(ab984b3), generated on April 24, 2018.Typeset by the author using LATEX and memoir.cls.ISBN 978-14-92143-23-9
Contents in BriefContents in BriefiiiContentsv1IntroductionIn which we introduce ROS, describe how it can be useful, and preview the remainder of the book.12Getting startedIn which we install ROS, introduce some basic ROS concepts, and interact with a working ROS system.113Writing ROS programsIn which we write ROS programs to publish and subscribe to messages.394Log messagesIn which we generate and view log messages.615Graph resource namesIn which we learn how ROS resolves the names of nodes, topics, parameters, and services.77iii
C ONTENTS IN B RIEFiv6Launch filesIn which we configure and run many nodes at once using launch files.837ParametersIn which we configure nodes using parameters.1058ServicesIn which we call services and respond to service requests.1179Recording and replaying messagesIn which we use bag files to record and replay messages.13310 ConclusionIn which we preview some additional topics.141Index145
ContentsContents in BriefiiiContentsv1.11223445577899Getting started2.1 Installing ROS . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .11112Introduction1.1 Why ROS? . . . . . . . . . . . . . . . . . . . .Distributed computation . .Software reuse . . . . . . . .Rapid testing . . . . . . . . .ROS is not . . . . . . . . . . . .1.2 What to expect . . . . . . . . . . . . . . . . .Chapters and dependenciesIntended audience . . . . .1.3 Conventions . . . . . . . . . . . . . . . . . .1.4 Getting more information . . . . . . . . . .Distributions . . . . . . . . .Build systems . . . . . . . . .1.5 Looking forward . . . . . . . . . . . . . . . .v.
C ONTENTSAdding the ROS repository . . . . . . . . . . .Installing the package authentication key . .Downloading the package lists . . . . . . . .Installing the ROS packages . . . . . . . . . .Installing turtlesim . . . . . . . . . . . . . . .Setting up rosdep systemwide . . . . . . . . .2.2 Configuring your account . . . . . . . . . . . . . . . . . . . .Setting up rosdep in a user account . . . . .Setting environment variables . . . . . . . . .2.3 A minimal example using turtlesim . . . . . . . . . . . . . . .Starting turtlesim . . . . . . . . . . . . . . . .2.4 Packages . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Listing and locating packages . . . . . . . . .Inspecting a package . . . . . . . . . . . . . .2.5 The master . . . . . . . . . . . . . . . . . . . . . . . . . . . . .2.6 Nodes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Starting nodes . . . . . . . . . . . . . . . . . .Listing nodes . . . . . . . . . . . . . . . . . . .Inspecting a node . . . . . . . . . . . . . . . .Killing a node . . . . . . . . . . . . . . . . . . .2.7 Topics and messages . . . . . . . . . . . . . . . . . . . . . . .2.7.1 Viewing the graph . . . . . . . . . . . . . . . . . . . .2.7.2 Messages and message types . . . . . . . . . . . . . .Listing topics . . . . . . . . . . . . . . . . . . .Echoing messages . . . . . . . . . . . . . . . .Measuring publication rates . . . . . . . . . .Inspecting a topic . . . . . . . . . . . . . . . .Inspecting a message type . . . . . . . . . . .Publishing messages from the command lineUnderstanding message type names . . . . .2.8 A larger example . . . . . . . . . . . . . . . . . . . . . . . . . .2.8.1 Communication via topics is many-to-many. . . . .2.8.2 Nodes are loosely coupled. . . . . . . . . . . . . . . .2.9 Checking for problems . . . . . . . . . . . . . . . . . . . . . .2.10 Looking forward . . . . . . . . . . . . . . . . . . . . . . . . . 7272828283031333435363737Writing ROS programs3.1 Creating a workspace and a package . . . . . . . . . . . . . . . . . . . . . .3939
Contents3.23.33.43.54Creating a workspace . . . . . . . . . . . .Creating a package . . . . . . . . . . . . .Editing the manifest . . . . . . . . . . . . .Hello, ROS! . . . . . . . . . . . . . . . . . . . . . . . . . . .3.2.1 A simple program . . . . . . . . . . . . . . . . . .3.2.2 Compiling the Hello program . . . . . . . . . . .Declaring dependencies . . . . . . . . . .Declaring an executable . . . . . . . . . .Building the workspace . . . . . . . . . . .Sourcing setup.bash . . . . . . . . . . . .3.2.3 Executing the hello program . . . . . . . . . . . .A publisher program . . . . . . . . . . . . . . . . . . . . .3.3.1 Publishing messages . . . . . . . . . . . . . . . . .Including the message type declaration .Creating a publisher object . . . . . . . .Creating and filling in the message objectPublishing the message . . . . . . . . . . .Formatting the output . . . . . . . . . . .3.3.2 The publishing loop . . . . . . . . . . . . . . . . .Checking for node shutdown . . . . . . .Controlling the publishing rate . . . . . .3.3.3 Compiling pubvel . . . . . . . . . . . . . . . . . .Declaring message type dependencies . .3.3.4 Executing pubvel . . . . . . . . . . . . . . . . . . .A subscriber program . . . . . . . . . . . . . . . . . . . . .Writing a callback function . . . . . . . . .Creating a subscriber object . . . . . . . .Giving ROS control . . . . . . . . . . . . .3.4.1 Compiling and executing subpose . . . . . . . . .Looking forward . . . . . . . . . . . . . . . . . . . . . . . .Log messages4.1 Severity levels . . . . . . . . . . . . . . . . . . . . .4.2 An example program . . . . . . . . . . . . . . . . .4.3 Generating log messages . . . . . . . . . . . . . . .Generating simple log messages .Generating one-time log messagesGenerating throttled log 3545454555557596060.61616262626565vii
C ONTENTS4.44.54.656viiiViewing log messages . . . . . . . . . . . . . . . . . . . . . . . . .4.4.1 Console . . . . . . . . . . . . . . . . . . . . . . . . . . . .Formatting console messages . . . . . . . . . . .4.4.2 Messages on rosout . . . . . . . . . . . . . . . . . . . . .4.4.3 Log files . . . . . . . . . . . . . . . . . . . . . . . . . . . .Finding the run id . . . . . . . . . . . . . . . . . .Checking and purging log files . . . . . . . . . . .Enabling and disabling log messages . . . . . . . . . . . . . . . .Setting the logger level from the command lineSetting the logger level from a GUI . . . . . . . .Setting the logger level from C code . . . . . .Looking forward . . . . . . . . . . . . . . . . . . . . . . . . . . . .Graph resource names5.1 Global names . . . . . . . . . . . . . . . . . . . . . . . . . . . . .5.2 Relative names . . . . . . . . . . . . . . . . . . . . . . . . . . . .Resolving relative names . . . . . . . . . . . . .Setting the default namespace . . . . . . . . . .Understanding the purpose of relative names5.3 Private names . . . . . . . . . . . . . . . . . . . . . . . . . . . .5.4 Anonymous names . . . . . . . . . . . . . . . . . . . . . . . . .5.5 Looking forward . . . . . . . . . . . . . . . . . . . . . . . . . . .Launch files6.1 Using launch files . . . . . . . . . . . . . . . . . . . . . .Executing launch files . . . . . . . . . . .Requesting verbosity . . . . . . . . . . .Ending a launched session . . . . . . . .6.2 Creating launch files . . . . . . . . . . . . . . . . . . . .6.2.1 Where to place launch files . . . . . . . . . . . .6.2.2 Basic ingredients . . . . . . . . . . . . . . . . . .Inserting the root element . . . . . . . .Launching nodes . . . . . . . . . . . . .Finding node log files . . . . . . . . . . .Directing output to the console . . . . .Requesting respawning . . . . . . . . . .Requiring nodes . . . . . . . . . . . . . .Launching nodes in their own 2.838383858586868686878888898990.
Contents6.36.46.56.678Launching nodes inside a namespace . . . . . . . . . . . . . . . .Remapping names . . . . . . . . . . . . . . . . . . . . . . . . . . . .6.4.1 Creating remappings . . . . . . . . . . . . . . . . . . . . .6.4.2 Reversing a turtle . . . . . . . . . . . . . . . . . . . . . . . .Other launch file elements . . . . . . . . . . . . . . . . . . . . . . .6.5.1 Including other files . . . . . . . . . . . . . . . . . . . . . .6.5.2 Launch arguments . . . . . . . . . . . . . . . . . . . . . . .Declaring arguments . . . . . . . . . . . . . . . . .Assigning argument values . . . . . . . . . . . . . .Accessing argument values . . . . . . . . . . . . . .Sending argument values to included launch files6.5.3 Creating groups . . . . . . . . . . . . . . . . . . . . . . . . .Looking forward . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Parameters7.1 Accessing parameters from the command line . . .Listing parameters . . . . . . . . . . .Querying parameters . . . . . . . . .Setting parameters . . . . . . . . . .Creating and loading parameter files7.2 Example: Parameters in turtlesim . . . . . . . . . . .Reading the background color . . . .Setting the background color . . . .7.3 Accessing parameters from C . . . . . . . . . . . .7.4 Setting parameters in launch files . . . . . . . . . . .Setting parameters . . . . . . . . . .Setting private parameters . . . . . .Reading parameters from a file . . .7.5 Looking forward . . . . . . . . . . . . . . . . . . . . .Services8.1 Terminology for services . . . . . . . . . . . . . . . . .8.2 Finding and calling services from the command lineListing all services . . . . . . . . . . . .Listing services by node . . . . . . . .Finding the node offering a service . .Finding the data type of a service . . .Inspecting service data types . . . . 0121.ix
C ONTENTS8.38.48.59Calling services from the command line .A client program . . . . . . . . . . . . . . . . . . . . . . . .Declaring the request and response typesCreating a client object . . . . . . . . . . .Creating request and response objects . .Calling the service . . . . . . . . . . . . . .Declaring a dependency . . . . . . . . . .A server program . . . . . . . . . . . . . . . . . . . . . . . .Writing a service callback . . . . . . . . .Creating a server object . . . . . . . . . . .Giving ROS control . . . . . . . . . . . . .8.4.1 Running and improving the server program . . .Looking ahead . . . . . . . . . . . . . . . . . . . . . . . . .Recording and replaying messages9.1 Recording and replaying bag files . . . . . .Recording bag files . . . . .Replaying bag files . . . . . .Inspecting bag files . . . . .9.2 Example: A bag of squares . . . . . . . . . .Drawing squares . . . . . . .Recording a bag of squares .Replaying the bag of squares9.3 Bags in launch files . . . . . . . . . . . . . .9.4 Looking forward . . . . . . . . . . . . . . . .10 Conclusion10.1 What next? . . . . . . . . . . . . . . . . . . . . . . . .Running ROS over a network . . . .Writing cleaner programs . . . . . .Visualizing data with rviz . . . . . .Creating message and service typesManaging coordinate frames with tfSimulating with Gazebo . . . . . . .10.2 Looking forward . . . . . . . . . . . . . . . . . . . . 142143143.145
Chapter1IntroductionIn which we introduce ROS, describe how it can be useful, and preview the remainder of the book.1.1 Why ROS?The robotics community has made impressive progress in recent years. Reliable and inexpensive robot hardware—from land-based mobile robots, to quadrotor helicopters, tohumanoids—is more widely available than ever before. Perhaps even more impressively,the community has also developed algorithms that help those robots run with increasinglevels of autonomy.In spite of (or, some might argue, because of ) this rapid progress, robots do still presentsome significant challenges for software developers. This book introduces a software platform called Robot Operating System, or ROS,1 that is intended to ease some of these difficulties. The official description of ROS is:ROS is an open-source, meta-operating system for your robot. It provides theservices you would expect from an operating system, including hardware abstraction, low-level device control, implementation of commonly-used functionality, message-passing between processes, and package management. Italso provides tools and libraries for obtaining, building, writing, and runningcode across multiple computers.Í11 When spoken aloud, the name “ROS” is nearly always pronounced as a single word that rhymes with“moss,” and almost never spelled out “arrr-oh-ess.”Í1 http://wiki.ros.org/ROS/Introduction1
1. I NTRODUCTIONThis description is accurate—and it correctly emphasizes that ROS does not replace, butinstead works alongside a traditional operating system—but it may leave you wonderingwhat the real advantages are for software that uses ROS. After all, learning to use a newframework, particularly one as complex and diverse as ROS, can take quite a lot of timeand mental energy, so one should be certain that the investment will be worthwhile. Hereare a few specific issues in the development of software for robots that ROS can help toresolve.Distributed computation Many modern robot systems rely on software that spans manydifferent processes and runs across several different computers. For example:RRRRSome robots carry multiple computers, each of which controls a subset of the robot’ssensors or actuators.Even within a single computer, it’s often a good idea to divide the robot’s softwareinto small, stand-alone parts that cooperate to achieve the overall goal. This approach is sometimes called “complexity via composition.”When multiple robots attempt to cooperate on a shared task, they often need tocommunicate with one another to coordinate their efforts.Human users often send commands to a robot from a laptop, a desktop computer,or mobile device. We can think of this human interface as an extension of the robot’ssoftware.The common thread through all of these cases is a need for communication between multiple processes that may or may not live on the same computer. ROS provides two relativelysimple, seamless mechanisms for this kind of communication. We’ll discuss the details inChapters 3 and 8.Software reuse The rapid progress of robotics research has resulted in a growing collection of good algorithms for common tasks such as navigation, motion planning, mapping,and many others. Of course, the existence of these algorithms is only truly useful if there isa way to apply them in new contexts, without the need to reimplement each algorithm foreach new system. ROS can help to prevent this kind of pain in at least two important ways.R2ROS’s standard packages provide stable, debugged implementations of many important robotics algorithms.
1.1. Why ROS?RROS’s message passing interface is becoming a de facto standard for robot softwareinteroperability, which means that ROS interfaces to both the latest hardware and toimplementations of cutting edge algorithms are quite often available. For example,the ROS website lists hundreds of publicly-available ROS packages.Í2 This sort ofuniform interface greatly reduces the need to write “glue” code to connect existingparts.As a result, developers that use ROS can expect—after, of course, climbing ROS’s initiallearning curve—to focus more time on experimenting with new ideas, and less time reinventing wheels.Rapid testing One of the reasons that software development for robots is often morechallenging than other kinds of development is that testing can be time consuming anderror-prone. Physical robots may not always be available to work with, and when theyare, the process is sometimes slow and finicky. Working with ROS provides two effectiveworkarounds to this problem.RRWell-designed ROS systems separate the low-level direct control of the hardware andhigh-level processing and decision making into separate programs. Because of thisseparation, we can temporarily replace those low-level programs (and their corresponding hardware) with a simulator, to test the behavior of the high-level part ofthe system.ROS also provides a simple way to record and play back sensor data and other kindsof messages. This facility means that we can obtain more leverage from the timewe do spend operating a physical robot. By recording the robot’s sensor data, wecan replay it many times to test different ways of processing that same data. In ROSparlance, these recordings are called “bags” and a tool called rosbag is used to recordand replay them. See Chapter 9.A crucial point for both of these features is that the change is seamless. Because the realrobot, the simulator, and the bag playback mechanism can all provide identical (or at leastvery similar) interfaces, your software does not need to be modified to operate in thesedistinct scenarios, and indeed need not even “know” whether it is talking to a real robot orto something else.Of course, ROS is not the only platform that offers these capabilities. What is uniqueabout ROS, at least in the author’s judgment, is the level of widespread support for ROSÍ2 http://www.ros.org/browse3
1. I NTRODUCTIONacross the robotics community. This “critical mass” of support makes it reasonable to predict that ROS will continue to evolve, expand, and improve in the future.ROS is not . . .ROS.RRRFinally, let’s take a moment to review a few things that are not true aboutROS is not a programming language. In fact, ROS programs are routinely writtenin C ,Í3 and this book has some explicit instructions on how to do that. Clientlibraries are also available for Python,Í4 Java,Í5 Lisp,Í6 and a handful of other languages.Í7ROS is not (only) a library. Although ROS does include client libraries, it also includes(among other things), a central server, a set of command-line tools, a set of graphicaltools, and a build system.ROS is not an integrated development environment. Although ROS does not prescribe any particular development environment, it can be used with most popularIDEs.Í8 It is also quite reasonable (and, indeed, it is the author’s personal preference) to use ROS from a text editor and the command line, without any IDE.1.2 What to expectThe goal of this book is to provide an integrated overview of the concepts and techniquesyou’ll need to know to write ROS software. This goal places a few important constraints onthe content of the book.RThis is not an introduction to programming. We won’t discuss basic programmingconcepts in any great detail. This book assumes that you’ve studied C in sufficientdepth to read, write, and understand code in that language.Í3 http://wiki.ros.org/roscppÍ4 http://wiki.ros.org/rospyÍ5 http://wiki.ros.org/rosjavaÍ6 http://wiki.ros.org/roslispÍ7 http://wiki.ros.org/ClientLibrariesÍ8 http://wiki.ros.org/IDEs4
1.2. What to expectRRThis is not a reference manual. There is plenty of detailed information about ROS,including both tutorialsÍ9 and exhaustive reference material,Í10 available online.This book makes no attempt to replace those resources. Instead, we present a selected subset of ROS features that, in the author’s view, represents a useful startingpoint for using ROS.This is not a textbook on robotics algorithms. The study of robots, especially the studyof algorithms for controlling autonomous robots, can be quite fascinating. A dizzying variety of algorithms have been developed for various parts of this problem. Thisbook will not teach you any of those algorithms.2 Our focus is on a specific tool,namely ROS, that can ease the implementation and testing of those algorithms.Chapters and dependencies Figure 1.1 shows the organization of the book. Chaptersare shown as rectangles; arrows show the major dependencies between them. It should befairly reasonable to read this book in any order that follows those constraints.Intended audience This book should be useful for both students in robotics courses andfor researchers or hobbyists that want to get a quick start with ROS. We’ll assume that readers are comfortable with Linux (including tasks like using the command line, installingsoftware, editing files, and setting environment variables), are familiar with C , and wantto write software to control robots. Generally, we’ll assume that you are using UbuntuLinux 14.04 (the newest version that is, at this writing, officially supported) and the bashshell. However, there are relatively few instances where these choices matter; other Linuxdistributions (especially those based on deb packages) and other shells will not usually beproblematic.2 . . . but you should learn them anyway.Í9 http://wiki.ros.org/ROS/TutorialsÍ10 http://wiki.ros.org/APIs5
1. I NTRODUCTION1. Introduction2. Basics3. Publish/Subscribe4. Logging6. Launch5. Names7. Parameters9. Bags8. Services10. ConclusionFigure 1.1: Dependencies between chapters.6
1.3. Conventions1.3 ConventionsThroughout the book, we’ll attempt to anticipate some of the most common sources ofproblems. These kinds of warnings, which are worthy of your attention, especially if thingsare not working as expected, are marked like this: This “dangerous bend” sign indicates a common source of problems.In addition, some sections include explanations that will be of interest to some readers,but are not crucial for understanding the concepts at hand. These comments are markedlike this:¹ This “fast forward” symbol indicates information that can be safely skipped, especially on a first reading.1.4 Getting more informationAs alluded to above, this book makes no attempt to be a comprehensive reference for ROS.It’s all but certain that you will need additional details to do anything interesting. Fortunately, online information about ROS is abundant.RRMost importantly, the developers of ROS maintain extensive documentation,Í11 including a set of tutorials. This book includes links, each marked with a Í, to manyof the corresponding pages in this documentation. If you are reading an electronicversion of the book in a reasonably modern PDF viewer, you should be able to clickthese links directly to open them in your browser.When unexpected things happen—and chances are quite good that they will—thereis a question and answer site (in the style of Stack Exchange) devoted to ROS.Í12Í11 http://wiki.ros.orgÍ12 http://answers.ros.org7
1. I NTRODUCTIONRIt may also be valuable to subscribe to the ros-users mailing list,Í13 on which announcements sometimes appear.Here are two important details that will help you make sense of some of the documentation, but are not always fully explained in context there.Distributions Major versions of ROS are called distributions, and are named using adjectives that start with with successive letters of the alphabet.Í14 (This is, for comparison, very similar to the naming schemes used for other large software projects, includingUbuntu and Android.) At the time of this writing, the current distribution is indigo. Thenext distribution, named jade, is due in May 2015.Í15 Older distributions include hydro, groovy, fuerte, electric, diamondback, C Turtle, and box turtle. These distributionnames appear in many places throughout the documentation. To keep things as simple and up-to-date as possible, this book assumes that you areusing indigo.¹ If, for some reason, you need to use hydro instead of indigo, nearly all of the book’scontent still applies without modification.The same is true for groovy as well, with one important exception: In distributionsnewer than groovy (and, therefore, in this book), velocity commands for the turtlesim simulator have been changed to use a standard message type and topic namethat happen to be shared with many real mobile robots.DistributionTopic nameMessage typegroovyindigo, hydro/turtle1/command velocity/turtle1/cmd velturtlesim/Velocitygeometry msgs/TwistRThis change has a few practical implications:When adding dependencies to your package (see page 44), you’ll need a dependency on turtlesim, instead of on geometry msgs.Í13 4 http://wiki.ros.org/DistributionsÍ15 http://wiki.ros.org/jade8
1.5. Looking forwardRThe relevant header file (see page 49) isturtlesim/Velocity.hrather thanRgeometry msgs/Twist.hThe turtlesim/Velocity message type has only two fields, called linear andangular. These fields play the same roles as the linear.x and angular.z fieldsof geometry msgs/Twist. This change applies both on the command line(see page 31) and in C code (see page 51).Build systems Starting with the groovy distribution, ROS made some major changes tothe way software is compiled. Older, pre-groovy distributions used a build system calledrosbuild, but more recent versions have begun to replace rosbuild with a new build system called catkin. It is important to know about this change because a few of the tutorialshave separate versions, depending on whether you’re using rosbuild or catkin. These separate versions are selected using a pair of buttons near the top of the tutorial. This bookdescribes catkin, but there may be some cases in which rosbuild is a better choice.Í161.5 Looking forwardIn the next chapter, we’ll get started working with ROS, learning some basic concepts andtools.Í16 http://wiki.ros.org/catkin or rosbuild9
Chapter2Getting startedIn which we install ROS, introduce some basic ROS concepts, and interact witha working ROS system.Before jumping into the details of how to write software that uses ROS, it will be valuableto get ROS up and running and to understand a few of the basic ideas that ROS uses. Thischapter lays that groundwork. After a quick discussion of how to install ROS and configureyour user account to use it, we’ll have a look at a working ROS system (specifically, aninstance of the turtlesim simulator) and learn how to interact with that system using somecommand line tools.2.1 Installing ROSBefore you can do anything with ROS, naturally you must ensure that it is installed onyour computer. (If you are working with a computer on which someone else has alreadyinstalled ROS—including the ros-indigo-turtlesim package—you can skip directly to Section 2.2.) The installation process is well documented and mostly straightforward.Í1 Í2Here’s a summary of the necessary steps.Adding the ROS repositoryAs root, create a file called/etc/apt/sources.list.d/ros-latest.listÍ1 http://wiki.ros.org/ROS/InstallationÍ2 http://wiki.ros.org/indigo/Installation/Ubuntu11
2. G ETTING STARTEDcontaining a single line:deb http://packages.ros.org/ros/ubuntu trusty main This line is specific to Ubuntu 14.04, whose codename is trusty. If you are usingUbuntu 13.10 instead, you can substitute saucy for trusty.¹ Other versions of Ubuntu—both older and and newer—are not supported bythe pre-compiled packages for the indigo distribution of ROS. However, forUbuntu versions newer than 14.04, installing ROS from its sourceÍ3 may bea reasonable option.If you are unsure of which Ubuntu version you’re using, you can find out using thiscommand:lsb release -aThe output should show both a codename and a release number.Installing the package authentication key Before installing the ROS packages, you mustacquire their package authentication key. First, download the key:wget ster/ros.keyIf this works correctly, you’ll have a small binary file called ros.key. Next, you should configure the apt package management system to use this key:sudo apt-key add ros.keyAfter completing this step (apt-key should say “OK”), you can safely delete ros.key.Downloading the package lists Once the repositories are configured, you can get thelatest lists of available packages in the usual way:Í3 http://wiki.ros.org/indigo/Installation/Source12
2.1. Installing ROSsudo apt-get updateNote that this will upd
form called Robot Operating System, or ROS,1 that is intended to ease some of these dif-ficulties. TheofficialdescriptionofROS is: ROS is an open-source, meta-operating system for you