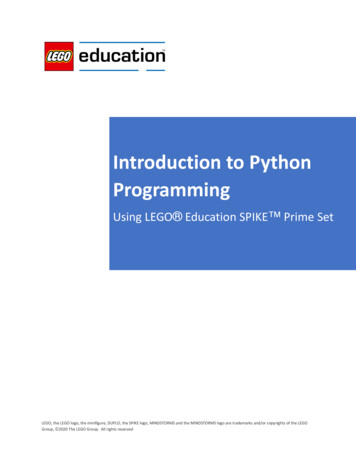
Transcription
Introduction to PythonProgrammingUsing LEGO Education SPIKE Prime SetLEGO, the LEGO logo, the minifigure, DUPLO, the SPIKE logo, MINDSTORMS and the MINDSTORMS logo are trademarks and/or copyrights of the LEGOGroup, 2020 The LEGO Group. All rights reserved
Introduction to Python ProgrammingUsing LEGO Education SPIKE Prime SetOVERVIEWIn this course, students will learn the fundamentals of thePython programming language, along with programmingbest practices, through using LEGO Education SPIKE Prime set. Through a series of scaffolded lessons, studentswill learn to important libraries, how to use the hardware andsoftware to control motors and sensors, use conditionals andloops to control the flow of your programs, and store datausing Python data types and variables. They will define anddocument their own custom programs, write scripts, and handle errors. Mostimportantly, students will have multiple and ongoing opportunities to use all of thisknowledge in authentic contexts to practice and develop their coding skills in Python.By the end of the course, students will Design, iteratively develop and program a prototype of a robot or modelWork collaboratively, give and receive feedback, and incorporate suggestionsDebug and troubleshoot both hardware and software problemsUse algorithms, data, compound conditionals, sensors, loops and Boolean logicDocument programs, feedback, testing, and debuggingArticulate flowcharts or pseudocode to address complex problemsDecompose problems and subproblems into partsDiscuss issues of bias and accessibilityCommunicate the solution to a problem, including model and programmingLEARNING PROMISEOver the duration of this course, students will develop their Python programmingknowledge by analyzing, prototyping, and communicating ideas in the areas ofabstraction, algorithms, programming, and data. Students will create artifacts and buildmodels that use motors, sensors, lights, and sounds to work effectively by creatingPython programs. They will utilize various programming techniques including writingpseudocode, using conditional statements, loops, Boolean logic, as well as linear andcomputational thinking to accomplish a variety of tasks. Students will apply their Pythonknowledge to various guided and open-ended projects which culminate in presentingsolutions to real-world problems.COURSE DESIGNThe course has been developed to address numerous Python programming skills andoutcomes. The lessons have been compiled into scaffolded experiences to increase incomplexity to enable teachers to provide their students with ongoing opportunities todevelop proficiency in Python programming.LEGO, the LEGO logo, the minifigure, DUPLO, the SPIKE logo, MINDSTORMS and the MINDSTORMS logoare trademarks and/or copyrights of the LEGO Group, 2020 The LEGO Group. All rights reserved
This course is built around the K-12 CS Framework and the CSTA standards. A matrixof the lessons, the standards covered in each lesson, and framework areas addressedis available at the end of this document.The course is broken down into five units and a culminating project. The units have 6-8lessons. The projects have between 10-12 lessons. During each 45-60-minute lesson,students will experience a high level of engagement to develop their Pythonproficiency. The course contains the following units: Unit 1: Hardware SoftwareUnit 2: MotorsUnit 3: Sensor ControlUnit 4: Loops and VariablesUnit 5: Conditions for GamesProject Unit: Mental AgilityThroughout the lessons, strategic questions and key objectives willguide students through the process of developing Python programmingproficiency. The key objectives listed on each lesson can be used to determine whetheror not each student is developing the relevant skills.The projects within the course will include specific documentation via journaling andrubrics to detail their overall understanding and application of the Python conceptspreviously covered.GETTING STARTED WITH THE SPIKE APP USING PYTHONOpening a new projectDirect students to open the software and look at library options. Open the SPIKE App Select New Project Select PYTHON Select CREATEUsing the Programming CanvasWe suggest allowing the students a few minutes in the first lesson to see the functionswithin the software and pointing out important features as shown in the image below.LEGO, the LEGO logo, the minifigure, DUPLO, the SPIKE logo, MINDSTORMS and the MINDSTORMS logoare trademarks and/or copyrights of the LEGO Group, 2020 The LEGO Group. All rights reserved
Name ProjectConnect hub andsee hub dataProgrammingareaOpen the Console for printand error messages Knowledge Basereference forgetting samplecode and APIRun programor stopThe programming canvas is the open white space starting on the top left side.When you open the software there will be a sample program already loaded inthe programming canvas.You will see the hub icon in the top left corner which is where you will connectyour hub.Along the bottom you will see the console and some functions that allow you tochange the screen size and undo actions. The console is where printed code willappear as well as error messages. If the console is not showing, click the twohorizontal bars in the center bottom of the canvas. The console will move up tobe visible.Along the right side, you will see the knowledge base. The knowledge base willprovide support and act as a reference for code.Using the Knowledge BaseThe Knowledge Base provides getting started support and easy transition to text-basedcoding for SPIKE Prime with python. The knowledge base is the API, providing a placeto find the functions linked to the hardware from the SPIKE Prime set. Additionally,sample codes that can be copied and pasted into the programming canvas, errormessage explanations, and the type and value of each function are provided in theKnowledge Base.Copy and Pasting from the Knowledge BaseTo make programming simpler, there are several sample programs provided in theKnowledge Base. Students can copy and paste these into the programming canvas atany time. To copy and paste:LEGO, the LEGO logo, the minifigure, DUPLO, the SPIKE logo, MINDSTORMS and the MINDSTORMS logoare trademarks and/or copyrights of the LEGO Group, 2020 The LEGO Group. All rights reserved
click the small blue icon in the upper right corner of the programming box withinthe Knowledge Base right click in the box that you wish to paste the code choose PASTEConnecting the Hub via BluetoothGuide students through connecting their hubs to the software. The hub can beconnected with the USB cable or through Bluetooth. To connect via Bluetooth: click the hub icon click CONNECT VIA BLUETOOTH in the upper right corner press the small circular button on the upper left part of the hub the hub name should appear at the bottom of the screen in a few seconds click on the correct hub name and it will be connected return to the programming canvas by clicking the X to close the connectiondashboard screen.Renaming a ProjectProjects will be saved in the My Projects tab. To easily locate a project, students shouldgive each project a name relevant to the task. To rename the Project: click the three small vertical dots to the right of the Project name. two choices will open in the pop-up menu, RENAME PROJECT and MOVE TO choose RENAME PROJECT the screen changes to show the current name erase what is there and type in your own title click save the menu will close and return to the programming canvasASSESSMENTIn this course, there are three types of assessment used for lessons and an additionalrubric used in the culminating project. Teachers are encouraged to use all types ofassessment as a way to provide students adequate feedback to continue grow theirknowledge and skills. The three types of assessment include: Teacher observations which encourages teachers to discuss outcomes withstudents through posing questions and listening to how students as a way tocheck for understanding. Peer feedback which allows students to learn how to provide and takeconstructive feedback that can better their solutions. Self-assessment which allows students to deeply reflect on their own learning tomake connections, think about how to work together, and complete work inpositive ways.LEGO, the LEGO logo, the minifigure, DUPLO, the SPIKE logo, MINDSTORMS and the MINDSTORMS logoare trademarks and/or copyrights of the LEGO Group, 2020 The LEGO Group. All rights reserved
Introduction to Python ProgrammingUnit 1 Hardware and SoftwareLessonImporting Libraries45 minutesObjectives Communicatingwith Light45 minutes Pair Programming45 minutes Communicatingwith Sounds45 minutes Digital Sign45-90 minutes CSTA StandardsLearn why a Pythonprogram must havelibraries imported.Import libraries.Run a program.2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.Describe the function ofhardware and software.Program the light matrixand learn how to debug asimple program.2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.Practice pairprogramming.Modify programs.2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.Describe the function ofhardware and software.Program sounds andbeeps and learn how todebug a simple program.Create a sound pattern.2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.Describe the function ofhardware and software.Program lights andsounds to communicate amessage.2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.
Introduction to Python ProgrammingUnit 1 Hardware and SoftwareLessonObjectivesCSTA Standards2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.Ideas to SupportYour Design30-45 minutes CareerConnections –Lesson Extension60-90 minutes Give specific feedbackon a peer’s project.Explore how to usefeedback to improve aproject.1B-IC-20 Seek diverse perspectives forthe purpose of improving computationalartifactsArticulate their personalinterests and goals.Relate their personalinterests and goals intopossible careerpathways.Explore various careersin career pathways.Career Ready Practice 10- Planeducation and career path aligned topersonal goals. (CCTC)
Introduction to Python ProgrammingUnit 2 MotorsLessonMaking Moveswith Motors45 minutesObjectives New Moves withMotors45 minutes Program motors to turnindividually usingparameters of time andspeedCreate a robot danceparty2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.Program a motor to moveto position using theshortest path.Program a motor to moveto a specific position.Program a motor to movea defined number ofdegrees.2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.Automating Action45 minutes Build and program amodel that automates atask.Hopper Run45 minutes Program two motors tomove simultaneously.Build and program a robotwithout wheels to moveforward. CSTA Standards
Introduction to Python ProgrammingUnit 2 MotorsLessonObjectivesCSTA Standards2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.Race Day45 minutes Ideas to Help withRace Day30-45 minutes Create a program tomove through a series ofsteps and turns.Utilize motor pair inmultiple ways.2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.Give specific feedbackon a peer’s project.Explore how to usefeedback to improve aproject.1B-IC-20 Seek diverse perspectives forthe purpose of improving computationalartifacts.
Introduction to Python ProgrammingUnit 3 Sensor ControlLessonObjectivesStart Sensing Program the force sensor.45 minutes Create conditionalstatements.Charging Rhino45 minutes Cart Control45 minutes Safe Delivery45 minutes CSTA Standards2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.Explore the force senorUnderstand effects ofpower on movement2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.Program the distancesensor.Explore movements withdistance.Understand ultrasonic.2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms.2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.Program model to movesafely using sensors.Investigate effects ofmotor power when usingsensors.2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms
Introduction to Python ProgrammingUnit 3 Sensor ControlLessonObjectivesCSTA Standards2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.GrasshopperTroubles45 minutes Ideas to Help YourGrasshopper30-45 minutes Make appropriatehardware decisionsRe-design a model to adda sensor2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.Give specific feedbackon a peer’s project.Explore how to usefeedback to improve aproject.1B-IC-20 Seek diverse perspectives forthe purpose of improving computationalartifacts.
Introduction to Python ProgrammingUnit 4 Loops and VariablesLessonObjectivesWarm Up Loop Program with loops.with Leo Build and program a sit45 minutesup machine.Counting Repswith Leo45 minutes Program a sit-up machineto count the reps and tocomplete a count down.CSTA Standards2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.
Introduction to Python ProgrammingUnit 4 Loops and VariablesLessonObjectivesDance Loop with Program a model usingCoachfor loops.45 minutes Debug four programs tolearn tips and tricks.Setting Conditionsfor Yoga45-90 minutes Investigate whilestatements.Program a model usingwhile loops.CSTA Standards2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.
Introduction to Python ProgrammingUnit 4 Loops and VariablesLessonObjectivesInfinite Moves Program infinite loops.45-90 minutes Create a model thatincludes a force sensorthat will provide acondition for the robot tomove.Leading the Teamwith Loops90-120 minutes Ideas to Help withLeading the Teamwith Loops30-45 minutes CSTA Standards2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.Design a model forrepetition.Program a model to moveusing loops.2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.Give specific feedbackon a peer’s project.Explore how to usefeedback to improve aproject.1B-IC-20 Seek diverse perspectives forthe purpose of improving computationalartifacts.
Introduction to Python ProgrammingUnit 5 Conditions for GamesLessonObjectivesControlling Motion Program the motionwith Tiltsensor.45 minutes Create conditionalstatements.Claw Machine45 minutes Charting GameDecisions45-90 minutes Guess Which Color45 minutes CSTA Standards2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.Create a basic loop.Program a grabber modelbased on set conditions.2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.Understand how to useflowcharts in planning.Create flowcharts andwrite programs that followthem.2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.Program the color sensorusing conditional code.2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.
Introduction to Python ProgrammingUnit 5 Conditions for GamesLessonObjectivesGuessing Game45 minutesCreate a game.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug. Write code that usesmultiple conditionstatements usingif/elif/else programming. Add a loop to code.Debug coding that hasincorrect/missing syntax,missing code, or incorrectindention.2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug. Score!45 minutesCSTA Standards Program movement andlight matrix.Apply knowledge ofconditional statements.
Introduction to Python ProgrammingUnit 5 Conditions for GamesLessonObjectivesGame Time Write code that includes90 minutesconditions that must be Ideas to Help withGame Time30-45 minutes met in a game formatCreate a game thatrequires a series ofevents requiring a robotto respondGive specific feedbackon a peer’s project.Explore how to usefeedback to improve aproject.CSTA Standards2-CS-02 Design projects that combinehardware and software components tocollect and exchange data.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-16 Incorporate existing code, media,and libraries into original programs, andgive attribution.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.2-IC-22 Collaborate with many contributorsthrough strategies such as crowdsourcingor surveys when creating a computationalartifact.1B-IC-20 Seek diverse perspectives forthe purpose of improving computationalartifacts.
Introduction to Python ProgrammingUnit 6 Troubleshooting and DebuggingLessonObjectives Brainstorm ideas andTesting Prototypesdevelop solutions to a45 minutes problem.Program a model.CSTA Standards2-AP-15 Seek and incorporate feedbackfrom team members and users to refine asolution that meets user needs.NGSSMS-ETS1-2. Evaluate competing designsolutions using a systematic process todetermine how well they meet the criteriaand constraints of the problem.MS-ETS1-4 Develop a model to generatedata for iterative testing and modification ofa proposed object, tool or process such thatan optimal design can be achieved.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms.2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.Break DancerBreak Down45 minutes Identify a problem anddebug the program.Dance to the Beat45 minutes Identify a problem anddebug the program.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms.2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.Testing for Trouble90 minutes Identify and repair ahardware problem in adesign.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms.2-AP-13 Decompose problems andsubproblems into parts to facilitate thedesign, implementation, and review ofprograms.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.Debug-inator45 minutes Debug a softwareproblem.2-AP-10 Use flowcharts and/or pseudocodeto address complex problems as algorithms.2-AP-13 Decompose problems andsubproblems into parts to facilitate the
Introduction to Python ProgrammingUnit 6 Troubleshooting and DebuggingLessonObjectivesCSTA Standardsdesign, implementation, and review ofprograms.2-AP-17 Systematically test and refineprograms using a range of test cases.2-AP-19 Document programs in order tomake them easier to follow, test, anddebug.Ideas to Help withthe Debug-inator30-45 minutes Give specific feedbackon a peer’s project.Explore how to usefeedback to improve apro
Over the duration of this course, students will develop their Python program ming knowledge by analyzing, prototyping, and communicating ideas in the areas of abstraction, algorithms, programming, and data. Students will create artifacts and build models that use motors, sensors, lights, and sounds to work