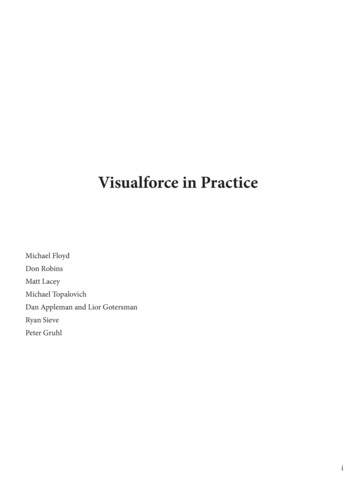
Transcription
Visualforce in PracticeMichael FloydDon RobinsMatt LaceyMichael TopalovichDan Appleman and Lior GotersmanRyan SievePeter Gruhli
Copyrights Copyright 2000–2013 salesforce.com, inc. All rights reserved. Salesforce.com is a registeredtrademark of salesforce.com, inc., as are other names and marks. Other marks appearing hereinmay be trademarks of their respective owners. Various trademarks held by their respective owners.No part of this publication may be reproduced, stored in a retrieval system, or transmitted, in anyform, or by any means, electronic, mechanical, photocopying, recording, or otherwise, without theprior consent of the publisher.Written by Michael Floyd, Don Robins, Michael Topalovich, Dan Appleman and Lior Gotersman,Ryan Sieve and Peter Gruhl.Contributions by Pat Patterson, Sandeep Bhanot, Raja Rao DV, and Michael Alderete.Cover by Domenique Sillett.Special Thanks to Nick Tran, Adam Seligman, Quinton Wall, Andrew Waite, Chris Wall, andLesley Schneiderman.ii
IntroductionThe making of this book is an interesting story. The seed of its inspiration came from developerslike you. Following one of our ELEVATE Workshops, an attendee was asked what he would like tosee next. He simply replied “A book about Visualforce.”Then my colleague Mario Korf spoke up, saying that “We have an Advanced Apex book,”authored by Dan Appleman to compliment our Introductory Apex Developer’s Guide. “We shoulddo an Advanced Visualforce.” And that’s how this book was born.But rather than follow the Beginner-Advanced documentation model, I wanted a book thatshows developers how to do real things while learning through actual apps that have beendeployed and are in practice. This is Visualforce in Practice.I also wanted readers to get the opportunity to learn from Force.com practitioners, developers inthe trenches that are earnestly building enterprise applications and deploying them to the cloud.I wanted to share the experience of those who have gone before you.And so I recruited Don Robins, a veteran Force.com Certified Salesforce Trainer and SalesforceMVP—the only developer to hold both titles. With Don’s help we recruited Matt Lacey, MichaelTopalovich, Dan Appleman, Lior Gotesman, Ryan Sieve and Peter Gruhl.Together we’ve assembled a collection of Visualforce example apps that you can use in your ownpractice. This is not documentation, and it is not intended to document every feature built intoVisualforce. This book is intended to share the experience and expertise of our authors.About the BookVisualforce in Practice is intended for intermediate Force.com developers. The book assumesyou have walked through the Visualforce Workbook tutorial, and know the basics of creating aVisualforce page.Chapter 1, Thinking in Visualforce lays the groundwork by discussing the MVC design Pattern,when you should use Visualforce and alternatives for using Visualforce under various use cases.The Standard Controller is an amazingly versatile component that changes behavior based onthe objects it’s working on. In that sense it is a polymorphic component that can do an amazingamount of work without having to write code. In Chapter 2, Don Robins walks you through theStandard Controller and sheds light on mysterious components like URLFOR().Because you’ll need to write custom controllers or controller extensions at some point, Chapter4 introduces you to “Just Enough Code.” Rather than providing introductions to Apex andSOQL, this chapter gives you the basics so you can get started writing controllers and extensionsimmediately.In Part II the rubber meets the road with chapters that will show you how to work with andpaginate lists using the StandardSetController (Chapter 4), and how to create wizards that walkusers through a process (Chapter 5). Chapter 6 shows how to create amazing looking chartsiii
to display data using the Analytics API, and Chapter 7 walks through the process for creatingdashboards.Chapter 8 uses the Streaming API to stream live data into Visualforce pages, while Chapter 9shows how to build Visualforce page templates like those in the Salesforce Mobile Templates.Chapter 10 shows you how to add JavaScript and HTML5 to your Visualforce pages, which setsup Part III covering mobile development.Chapter 11 walks you through the process of refactoring existing Force.com apps, then extendsto mobile the Warehouse App used in the Force.com Workbook. Chapter 12 introduces you tothe Salesforce Mobile Templates, the brainchild of Developer Evangelist Sandeep Bhanot.And Finally Chapter 13 presents readers with tips and tricks for optimizing Visualforce pagesto ensure peek performance.Looking back, there are many topics we would like to have covered in this book, and with theintroduction of Salesforce1 there are many new topics we would like to include in the future.Indeed, I’m looking forward to creating a second edition of Visualforce in Pracice. In themeantime, it is my sincerest hope that you find this book not only educational, but useful in youreveryday practice.Sincerely,Michael FloydEditor in Chief, Developer ForceSalesforce.comiv
ContentsIntroduction. . . iiAbout the Book. . . iiiChapter 1—Thinking in Visualforce. 9What is Visualforce. . . 10Controllers and Extensions. 10About the Markup. 11About View State. 11What is Contained in the View State?. 12Examining the View State. . 12View State in Action. 13New Addition to Visualforce. 16Salesforce1 and Visualforce. 16Five Things you should master before getting startted with Visualforce. 16Parting Words. 18Chapter 2—The Standard Controller—Pushing the Envelope. . 19What Is a Standard Controller?. 21What Does a Standard Controller Do?. 21CONTROLLING VIEWS. 22Materializing a User Interface. 22Standard User Interface Flow. 23CONTROLLING DATA. . . 24Referencing a Single Record. 24Loading Field Values. 25Loading Field Values in Apex. . . 26Referencing a List of Records. 27CONTROLLING ACTIONS AND NAVIGATION. 28Actions as Navigation. 28Stateful Actions. 28Stateful Action Mechanisms. . . 29Demo Custom sObject Actions. . . 31Stateless Actions. . . 31Stateless Action Mechanisms. . 33 Action Global Variable. 331
URLFOR() Directing to a Visualforce page. 34URLFOR() and Action Considerations. 35Adding URL parameters on Actions with URLFOR(). 36STANDARD CONTROLLERS IN APEX. 37Injecting an ApexPages.StandardController Instance. 37Fetching the ID or record. 37Leveraging Standard Controller Actions in Apex. . . 38Concurrency Considerations. 38Action Method Navigation with ApexPages.PageReference. 39CONTROLLING SECURITY. 39Security Context Variables - Standard vs. Custom. 39Summary. . . 40Chapter 3—Just Enough Code Introduction toControllers and Extensions. 41Overriding Built-in Functionality. 42Creating New Functionality. 42Overriding Permissions. . . 42Basic Extension and Custom Controller Anatomy. . . 42Objective. 42Extension Class Solution. 43Custom Controller Solution. 45When and How To Use Standard, Custom, and Extension Controllers. 47The View State. . 48The Transient Keyword. . 49Inner Classes. 49Collections of Objects. . 50Sharing Settings. 51Unit Testing. . 51Writing Unit Tests For Extensions . 52Writing Unit Tests For Custom Controllers . . . 53Conclusion. 542
Chapter 4—The Standard List Controller. 55Displaying Standard Lists of Records. . . 55Customized List Displays. 56Adding Pagination. 57Simple Filtering - Leveraging Standard List Views. . .
to mobile the Warehouse App used in the Force.com Workbook. Chapter 12 introduces you to the Salesforce Mobile Templates, the brainchild of Developer Evangelist Sandeep Bhanot. And Finally Chapter 13 presents readers with tips and tricks for optimizing Visualforce pages to ensure peek performance. Looking back, there are many topics we would like to have covered in this book, and