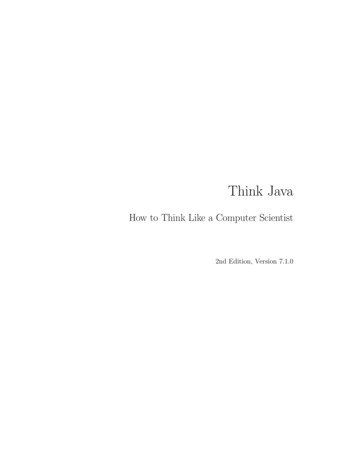
Transcription
Think JavaHow to Think Like a Computer Scientist2nd Edition, Version 7.1.0
Think JavaHow to Think Like a Computer Scientist2nd Edition, Version 7.1.0Allen B. Downey and Chris MayfieldGreen Tea PressNeedham, Massachusetts
Copyright 2020 Allen B. Downey and Chris Mayfield.Green Tea Press9 Washburn AveNeedham, MA 02492Permission is granted to copy, distribute, and/or modify this work under theterms of the Creative Commons Attribution-NonCommercial-ShareAlike 4.0International License, which is available at .The original form of this book is LATEX source code. Compiling this code hasthe effect of generating a device-independent representation of the book, whichcan be converted to other formats and printed.The LATEX source for this book is available from https://thinkjava.org/and https://github.com/ChrisMayfield/ThinkJava2.
ContentsPrefacexv1 Computer Programming11.1What Is a Computer? . . . . . . . . . . . . . . . . . . . . . .11.2What Is Programming? . . . . . . . . . . . . . . . . . . . . .21.3The Hello World Program . . . . . . . . . . . . . . . . . . .31.4Compiling Java Programs . . . . . . . . . . . . . . . . . . .51.5Displaying Two Messages . . . . . . . . . . . . . . . . . . . .61.6Formatting Source Code . . . . . . . . . . . . . . . . . . . .71.7Using Escape Sequences . . . . . . . . . . . . . . . . . . . .91.8What Is Computer Science? . . . . . . . . . . . . . . . . . .101.9Debugging Programs . . . . . . . . . . . . . . . . . . . . . .111.10Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .121.11Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . .142 Variables and Operators172.1Declaring Variables . . . . . . . . . . . . . . . . . . . . . . .172.2Assigning Variables . . . . . . . . . . . . . . . . . . . . . . .182.3Memory Diagrams . . . . . . . . . . . . . . . . . . . . . . . .192.4Printing Variables . . . . . . . . . . . . . . . . . . . . . . . .21
viCONTENTS2.5Arithmetic Operators . . . . . . . . . . . . . . . . . . . . . .222.6Floating-Point Numbers . . . . . . . . . . . . . . . . . . . .232.7Rounding Errors . . . . . . . . . . . . . . . . . . . . . . . . .242.8Operators for Strings . . . . . . . . . . . . . . . . . . . . . .252.9Compiler Error Messages . . . . . . . . . . . . . . . . . . . .272.10Other Types of Errors . . . . . . . . . . . . . . . . . . . . .282.11Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .292.12Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . .313 Input and Output333.1The System Class . . . . . . . . . . . . . . . . . . . . . . . .333.2The Scanner Class. . . . . . . . . . . . . . . . . . . . . . .343.3Language Elements . . . . . . . . . . . . . . . . . . . . . . .363.4Literals and Constants . . . . . . . . . . . . . . . . . . . . .373.5Formatting Output . . . . . . . . . . . . . . . . . . . . . . .393.6Reading Error Messages . . . . . . . . . . . . . . . . . . . .403.7Type Cast Operators . . . . . . . . . . . . . . . . . . . . . .413.8Remainder Operator . . . . . . . . . . . . . . . . . . . . . .423.9Putting It All Together . . . . . . . . . . . . . . . . . . . . .433.10The Scanner Bug . . . . . . . . . . . . . . . . . . . . . . . .453.11Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .463.12Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . .474 Methods and Testing514.1Defining New Methods . . . . . . . . . . . . . . . . . . . . .514.2Flow of Execution . . . . . . . . . . . . . . . . . . . . . . . .534.3Parameters and Arguments . . . . . . . . . . . . . . . . . . .54
CONTENTSvii4.4Multiple Parameters . . . . . . . . . . . . . . . . . . . . . .554.5Stack Diagrams . . . . . . . . . . . . . . . . . . . . . . . . .574.6Math Methods . . . . . . . . . . . . . . . . . . . . . . . . . .584.7Composition . . . . . . . . . . . . . . . . . . . . . . . . . . .594.8Return Values . . . . . . . . . . . . . . . . . . . . . . . . . .604.9Incremental Development . . . . . . . . . . . . . . . . . . . .624.10Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .644.11Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . .665 Conditionals and Logic715.1Relational Operators . . . . . . . . . . . . . . . . . . . . . .715.2The if-else Statement . . . . . . . . . . . . . . . . . . . . . .725.3Chaining and Nesting . . . . . . . . . . . . . . . . . . . . . .745.4The switch Statement . . . . . . . . . . . . . . . . . . . . . .755.5Logical Operators . . . . . . . . . . . . . . . . . . . . . . . .775.6De Morgan’s Laws. . . . . . . . . . . . . . . . . . . . . . .785.7Boolean Variables . . . . . . . . . . . . . . . . . . . . . . . .795.8Boolean Methods . . . . . . . . . . . . . . . . . . . . . . . .805.9Validating Input . . . . . . . . . . . . . . . . . . . . . . . . .815.10Example Program . . . . . . . . . . . . . . . . . . . . . . . .835.11Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .845.12Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . .856 Loops and Strings896.1The while Statement . . . . . . . . . . . . . . . . . . . . . .896.2Increment and Decrement . . . . . . . . . . . . . . . . . . .916.3The for Statement . . . . . . . . . . . . . . . . . . . . . . . .92
viiiCONTENTS6.4Nested Loops . . . . . . . . . . . . . . . . . . . . . . . . . .946.5Characters . . . . . . . . . . . . . . . . . . . . . . . . . . . .956.6Which Loop to Use . . . . . . . . . . . . . . . . . . . . . . .966.7String Iteration . . . . . . . . . . . . . . . . . . . . . . . . .976.8The indexOf Method . . . . . . . . . . . . . . . . . . . . . .986.9Substrings . . . . . . . . . . . . . . . . . . . . . . . . . . . .996.10String Comparison . . . . . . . . . . . . . . . . . . . . . . .1006.11String Formatting . . . . . . . . . . . . . . . . . . . . . . . .1016.12Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .1026.13Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . .1037 Arrays and References1077.1Creating Arrays . . . . . . . . . . . . . . . . . . . . . . . . .1087.2Accessing Elements . . . . . . . . . . . . . . . . . . . . . . .1097.3Displaying Arrays . . . . . . . . . . . . . . . . . . . . . . . .1107.4Copying Arrays . . . . . . . . . . . . . . . . . . . . . . . . .1117.5Traversing Arrays . . . . . . . . . . . . . . . . . . . . . . . .1137.6Random Numbers . . . . . . . . . . . . . . . . . . . . . . . .1157.7Building a Histogram . . . . . . . . . . . . . . . . . . . . . .1167.8The Enhanced for Loop . . . . . . . . . . . . . . . . . . . . .1187.9Counting Characters . . . . . . . . . . . . . . . . . . . . . .1197.10Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .1217.11Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . .1228 Recursive Methods1278.1Recursive Void Methods . . . . . . . . . . . . . . . . . . . .1278.2Recursive Stack Diagrams . . . . . . . . . . . . . . . . . . .129
CONTENTSix8.3Value-Returning Methods . . . . . . . . . . . . . . . . . . .1308.4The Leap of Faith . . . . . . . . . . . . . . . . . . . . . . . .1338.5Counting Up Recursively . . . . . . . . . . . . . . . . . . . .1358.6Binary Number System . . . . . . . . . . . . . . . . . . . . .1358.7Recursive Binary Method . . . . . . . . . . . . . . . . . . . .1378.8CodingBat Problems . . . . . . . . . . . . . . . . . . . . . .1388.9Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .1408.10Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . .1419 Immutable Objects1479.1Primitives vs Objects . . . . . . . . . . . . . . . . . . . . . .1479.2The null Keyword . . . . . . . . . . . . . . . . . . . . . . . .1499.3Strings Are Immutable . . . . . . . . . . . . . . . . . . . . .1509.4Wrapper Classes . . . . . . . . . . . . . . . . . . . . . . . . .1519.5Command-Line Arguments . . . . . . . . . . . . . . . . . . .1529.6Argument Validation . . . . . . . . . . . . . . . . . . . . . .1549.7BigInteger Arithmetic . . . . . . . . . . . . . . . . . . . . . .1569.8Incremental Design . . . . . . . . . . . . . . . . . . . . . . .1579.9More Generalization. . . . . . . . . . . . . . . . . . . . . .1599.10Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .1609.11Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . .16110 Mutable Objects16710.1Point Objects . . . . . . . . . . . . . . . . . . . . . . . . . .16710.2Objects as Parameters . . . . . . . . . . . . . . . . . . . . .16910.3Objects as Return Values . . . . . . . . . . . . . . . . . . . .17010.4Rectangles Are Mutable . . . . . . . . . . . . . . . . . . . .171
xCONTENTS10.5Aliasing Revisited . . . . . . . . . . . . . . . . . . . . . . . .17210.6Java Library Source . . . . . . . . . . . . . . . . . . . . . . .17310.7Class Diagrams . . . . . . . . . . . . . . . . . . . . . . . . .17410.8Scope Revisited . . . . . . . . . . . . . . . . . . . . . . . . .17510.9Garbage Collection . . . . . . . . . . . . . . . . . . . . . . .17610.10 Mutable vs Immutable . . . . . . . . . . . . . . . . . . . . .17710.11 StringBuilder Objects . . . . . . . . . . . . . . . . . . . . . .17810.12 Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .18010.13 Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . .18011 Designing Classes18311.1The Time Class . . . . . . . . . . . . . . . . . . . . . . . . .18411.2Constructors . . . . . . . . . . . . . . . . . . . . . . . . . . .18511.3Value Constructors . . . . . . . . . . . . . . . . . . . . . . .18611.4Getters and Setters . . . . . . . . . . . . . . . . . . . . . . .18811.5Displaying Objects . . . . . . . . . . . . . . . . . . . . . . .19011.6The toString Method . . . . . . . . . . . . . . . . . . . . . .19111.7The equals Method . . . . . . . . . . . . . . . . . . . . . . .19211.8Adding Times . . . . . . . . . . . . . . . . . . . . . . . . . .19411.9Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .19611.10 Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . .19712 Arrays of Objects20112.1Card Objects . . . . . . . . . . . . . . . . . . . . . . . . . .20212.2Card toString . . . . . . . . . . . . . . . . . . . . . . . . . .20312.3Class Variables . . . . . . . . . . . . . . . . . . . . . . . . .20512.4The compareTo Method . . . . . . . . . . . . . . . . . . . .206
CONTENTSxi12.5Cards Are Immutable . . . . . . . . . . . . . . . . . . . . . .20712.6Arrays of Cards . . . . . . . . . . . . . . . . . . . . . . . . .20812.7Sequential Search . . . . . . . . . . . . . . . . . . . . . . . .21012.8Binary Search . . . . . . . . . . . . . . . . . . . . . . . . . .21112.9Tracing the Code . . . . . . . . . . . . . . . . . . . . . . . .21312.10 Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .21412.11 Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . .21413 Objects of Arrays21713.1Decks of Cards . . . . . . . . . . . . . . . . . . . . . . . . .21713.2Shuffling Decks . . . . . . . . . . . . . . . . . . . . . . . . .21913.3Selection Sort . . . . . . . . . . . . . . . . . . . . . . . . . .22013.4Merge Sort . . . . . . . . . . . . . . . . . . . . . . . . . . . .22113.5Subdecks . . . . . . . . . . . . . . . . . . . . . . . . . . . . .22213.6Merging Decks . . . . . . . . . . . . . . . . . . . . . . . . . .22313.7Adding Recursion . . . . . . . . . . . . . . . . . . . . . . . .22413.8Static Context . . . . . . . . . . . . . . . . . . . . . . . . . .22513.9Piles of Cards . . . . . . . . . . . . . . . . . . . . . . . . . .22713.10 Playing War . . . . . . . . . . . . . . . . . . . . . . . . . . .22913.11 Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .23013.12 Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . .23114 Extending Classes23514.1CardCollection. . . . . . . . . . . . . . . . . . . . . . . . .23614.2Inheritance . . . . . . . . . . . . . . . . . . . . . . . . . . . .23814.3Dealing Cards . . . . . . . . . . . . . . . . . . . . . . . . . .24014.4The Player Class . . . . . . . . . . . . . . . . . . . . . . . .242
xiiCONTENTS14.5The Eights Class . . . . . . . . . . . . . . . . . . . . . . . .24414.6Class Relationships . . . . . . . . . . . . . . . . . . . . . . .24814.7Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .24914.8Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . .24915 Arrays of Arrays25115.1Conway’s Game of Life . . . . . . . . . . . . . . . . . . . . .25115.2The Cell Class . . . . . . . . . . . . . . . . . . . . . . . . . .25315.3Two-Dimensional Arrays . . . . . . . . . . . . . . . . . . . .25415.4The GridCanvas Class . . . . . . . . . . . . . . . . . . . . .25615.5Other Grid Methods . . . . . . . . . . . . . . . . . . . . . .25715.6Starting the Game . . . . . . . . . . . . . . . . . . . . . . .25815.7The Simulation Loop . . . . . . . . . . . . . . . . . . . . . .25915.8Exception Handling . . . . . . . . . . . . . . . . . . . . . . .26015.9Counting Neighbors . . . . . . . . . . . . . . . . . . . . . . .26115.10 Updating the Grid . . . . . . . . . . . . . . . . . . . . . . .26315.11 Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .26515.12 Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . .26516 Reusing Classes26916.1Langton’s Ant . . . . . . . . . . . . . . . . . . . . . . . . . .26916.2Refactoring . . . . . . . . . . . . . . . . . . . . . . . . . . .27216.3Abstract Classes . . . . . . . . . . . . . . . . . . . . . . . . .27316.4UML Diagram . . . . . . . . . . . . . . . . . . . . . . . . . .27516.5Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .27616.6Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . .277
CONTENTSxiii17 Advanced Topics27917.1Polygon Objects . . . . . . . . . . . . . . . . . . . . . . . . .27917.2Adding Color . . . . . . . . . . . . . . . . . . . . . . . . . .28017.3Regular Polygons . . . . . . . . . . . . . . . . . . . . . . . .28117.4More Constructors . . . . . . . . . . . . . . . . . . . . . . .28417.5An Initial Drawing . . . . . . . . . . . . . . . . . . . . . . .28517.6Blinking Polygons . . . . . . . . . . . . . . . . . . . . . . . .28817.7Interfaces . . . . . . . . . . . . . . . . . . . . . . . . . . . .29017.8Event Listeners . . . . . . . . . . . . . . . . . . . . . . . . .29217.9Timers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .29517.10 Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .29717.11 Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . .297A Tools299A.1Installing DrJava . . . . . . . . . . . . . . . . . . . . . . . .299A.2DrJava Interactions . . . . . . . . . . . . . . . . . . . . . . .301A.3Command-Line Interface . . . . . . . . . . . . . . . . . . . .302A.4Command-Line Testing . . . . . . . . . . . . . . . . . . . . .303A.5Running Checkstyle . . . . . . . . . . . . . . . . . . . . . . .305A.6Tracing with a Debugger . . . . . . . . . . . . . . . . . . . .306A.7Testing with JUnit . . . . . . . . . . . . . . . . . . . . . . .307A.8Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .309B Javadoc311B.1Reading Documentation . . . . . . . . . . . . . . . . . . . .312B.2Writing Documentation . . . . . . . . . . . . . . . . . . . . .314B.3Javadoc Tags . . . . . . . . . . . . . . . . . . . . . . . . . .315
xivCONTENTSB.4Example Source File . . . . . . . . . . . . . . . . . . . . . .317B.5Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .320C Graphics321C.1Creating Graphics . . . . . . . . . . . . . . . . . . . . . . . .321C.2Graphics Methods . . . . . . . . . . . . . . . . . . . . . . . .322C.3Example Drawing . . . . . . . . . . . . . . . . . . . . . . . .324C.4Vocabulary . . . . . . . . . . . . . . . . . . . . . . . . . . . .326C.5Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . .326D Debugging329D.1Compile-Time Errors . . . . . . . . . . . . . . . . . . . . . .329D.2Run-Time Errors . . . . . . . . . . . . . . . . . . . . . . . .333D.3Logic Errors . . . . . . . . . . . . . . . . . . . . . . . . . . .337Index343
PrefaceThink Java is an introduction to computer science and programming intendedfor readers with little or no experience. We start with the most basic conceptsand are careful to define all terms when they are first used. The book presentseach new idea in a logical progression. Larger topics, like control flow statements and object-oriented programming, are divided into smaller examplesand introduced over the course of several chapters.This book is intentionally concise. Each chapter is 12–14 pages and covers thematerial for one week of a college course. It is not meant to be a comprehensivepresentation of Java, but rather, an initial exposure to programming constructsand techniques. We begin with small problems and basic algorithms and workup to object-oriented design. In the vocabulary of computer science pedagogy,this book uses the “objects late” approach.The Philosophy Behind the BookHere are the guiding principles that make the book the way it is:One concept at a time: We break down topics that give beginners troubleinto a series of small steps, so that they can exercise each new conceptin isolation before continuing.Balance of Java and concepts: The book is not primarily about Java; ituses code examples to demonstrate computer science. Most chaptersstart with language features and end with concepts.
xviPREFACEConciseness: An important goal of the book is to be small enough so thatstudents can read and understand the entire text in a one-semester college or AP course.Emphasis on vocabulary: We try to introduce the minimum number ofterms and define them carefully when they are first used. We also organize them in glossaries at the end of each chapter.Program development: There are many strategies for writing programs,including bottom-up, top-down, and others. We demonstrate multipleprogram development techniques, allowing readers to choose methodsthat work best for them.Multiple learning curves: To write a program, you have to understand thealgorithm, know the programming language, and be able to debug errors.We discuss these and other aspects throughout the book and summarizeour advice in Appendix D.Object-Oriented ProgrammingSome Java books introduce classes and objects immediately; others begin withprocedural programming and transition to object-oriented more gradually.Many of Java’s object-oriented features are motivated by problems with previous languages, and their implementations are influenced by this history. Someof these features are hard to explain when people aren’t familiar with theproblems they solve.We get to object-oriented programming as quickly as possible (beginning withChapter 9). But we introduce concepts one at a time, as clearly as possible,in a way that allows readers to practice each idea in isolation before movingon. So it takes some time to get there.You can’t write Java programs (even Hello World) without encountering objectoriented features. In some cases we explain a feature briefly when it firstappears, and then explain it more deeply later on.If you read the entire book, you will see nearly every topic required for Java SEProgrammer I certification. Supplemental lessons are available in the officialJava tutorials on Oracle’s website (https://thinkjava.org/tutorial).
PREFACExviiThis book is also well suited to prepare high school students for the AP Computer Science A exam, which includes object-oriented design and implementation. (AP is a registered trademark of The College Board.) A mappingof Think Java section numbers to the AP course is available on our website:https://thinkjava.org/.Changes to the Second EditionThis new edition was written over several years, with feedback from dozensof instructors and hundreds of students. A complete history of all changes isavailable on GitHub. Here are some of the highlights:Chapters 1–4: We reordered the material in Chapter 1 to present a more interesting balance of theory and practice. Chapters 2–3 are much cleanernow too. Methods are now presented in a single chapter, along withadditional in-depth examples.Chapters 5–8: We rearranged these chapters a lot, added many examplesand new figures, and removed unnecessary details. Strings are coveredearlier (before arrays) so that readers can apply them to loop problems.The material on recursion is now a chapter, and we added new sectionsto explain binary numbers and CodingBat.Chapters 9–12: Our main goal for these chapters was to provide better explanations and more diagrams. Chapters 9–10 focus more on immutableversus mutable objects, and we added new sections on BigInteger andStringBuilder. The other content is largely the same, but it should beeasier to understand now.Chapters 13–17: We balanced the amount of content in Chapters 13–14 bymoving ArrayLists earlier, and we implement the “War” card game asanother example. Chapters 15–17 are brand new in this edition; theycover more advanced topics including 2D arrays, graphics, exceptions,abstract classes, interfaces, and events.Appendixes: We added Appendix B to explain documentation commentsand Javadoc in more detail. The other three appendixes that werepresent in the first edition have been revised for clarity and layout.
xviiiPREFACEAbout the AppendixesThe chapters of this book are meant to be read in order, because each onebuilds on the previous one. We also include several appendixes with materialthat can be read at any time:Appendix A, “Tools”This appendix explains how to download and install Java so you cancompile programs on your computer. It also provides a brief introductionto DrJava—an integrated development environment designed primarilyfor students—and other development tools, including Checkstyle for codequality and JUnit for testing.Appendix B, “Javadoc”It’s important to document your classes and methods so that other programmers (including yourself in the future) will know how to use them.This appendix explains how to read documentation, how to write documentation, and how to use the Javadoc tool.Appendix C, “Graphics”Java provides libraries for working with graphics and animation, andthese topics can be engaging for students. The libraries require objectoriented features that students will not completely understand until afterChapter 10, but they can be used much earlier.Appendix D, “Debugging”We provide debugging suggestions throughout the book, but this appendix provides many more suggestions on how to debug your programs.We recommend that you review this appendix frequently as you workthrough the book.Using the Code ExamplesMost of the code examples in this book are available from a Git repositoryat https://github.com/ChrisMayfield/ThinkJavaCode2. Git is a “versioncontrol system” that allows you to keep track of the files that make up aproject. A collection of files under Git’s control is called a “repository”.
PREFACExixGitHub is a hosting service that provides storage for Git repositories and aconvenient web interface. It provides several ways to work with the code: You can create a copy of the repository on GitHub by clicking the Forkbutton. If you don’t already have a GitHub account, you’ll need tocreate one. After forking, you’ll have your own repository on GitHubthat you can use to keep track of code you write. Then you can “clone”the repository, which downloads a copy of the files to your computer. Alternatively, you could clone the original repository without forking. Ifyou choose this option, you don’t need a GitHub account, but you won’tbe able to save your changes on GitHub. If you don’t want to use Git at all, you can download the code in aZIP archive using the Clone button on the GitHub page, or this link:https://thinkjava.org/code2zip.After you clone the repository or unzip the ZIP file, you should have a directorynamed ThinkJavaCode2 with a subdirectory for each chapter in the book.The examples in this book were developed and tested using OpenJDK 11. Ifyou are using a more recent version, everything should still work. If you areusing an older version, some of the examples might not.AcknowledgmentsMany people have sent corrections and suggestions over the years, and weappreciate their valuable feedback! This list begins with Version 4.0 of theopen source edition, so it omits those who contributed to earlier versions: Ellen Hildreth used this book to teach Data Structures at WellesleyCollege and submitted a whole stack of corrections and suggestions. Tania Passfield pointed out that some glossaries had leftover terms thatno longer appeared in the text. Elizabeth Wiethoff noticed that the series expansion of exp( x2 ) waswrong. She has also worked on a Ruby version of the book.
xxPREFACE Matt Crawford sent in a whole patch file full of corrections. Chi-Yu Li pointed out a typo and an error in one of the code examples. Doan Thanh Nam corrected an example. Muhammad Saied translated the book into Arabic and found severalerrors in the process. Marius Margowski found an inconsistency in a code example. Leslie Klein discovered another error in the series expansion of exp( x2 ),identified typos in card array figures, and helped clarify several exercises. Micah Lindstrom reported half a dozen typos and sent corrections. James Riely ported the textbook source from LaTeX to / Peter Knaggs ported the book to C#.https://www.rigwit.co.uk/think/sharp/ Heidi Gentry-Kolen recorded several video lectures that follow the book.https://www.youtube.com/user/digipipeline Waldo Ribeiro submitted a pull request that corrected a dozen typos. Michael Stewart made several suggestions for improving the first half ofthe book. Steven Richardson adapted the book for an online course and contributedmany ideas for improving the text. Fazl Rahman provided detailed feedback, chapter by chapter, and offeredmany suggestions for improving the text.We are especially grateful to the technical reviewers of the O’Reilly Mediafirst edition: Blythe Samuels, David Wisneski, and Stephen Rose. They founderrors, made many great suggestions, and helped make the book much better.Likewise, we thank Marc Loy for his thorough review of the O’Reilly Mediasecond edition. He contributed many corrections, insights, and clarifications.
PREFACExxiMany students have given exceptional feedback, including Ian Staton, TannerWernecke, Jacob Green, Rasha Abuhantash, Nick Duncan, Kylie Davidson,Shirley Jiang, Elena Trafton, Jennifer Gregorio, and Azeem Mufti.Other contributors who found one or more typos: Stijn Debrouwere, GuyDriesen, Andai Velican, Chris Kuszmaul, Daniel Kurikesu, Josh Donath, RensFindhammer, Elisa Abedrapo, Yousef BaAfif, Bruce Hill, Matt Underwood,Isaac Sultan, Dan Rice, Robert Beard, Daniel Pierce, Michael Giftthaler, ChrisFox, Min Zeng, Markus Geuss, Mauricio Gonzalez, Enrico Sartirana, KasemSatitwiwat, Jason Miller, Kevin Molloy, Cory Culbertson, Will Crawford, andShawn Brenneman.If you have additional comments or ideas about the text, please send them to:feedback@greenteapress.com.Allen Downey and Chris Mayfield
xxiiPREFACE
Chapter 1Computer ProgrammingThe goal of this book is to teach you to think like a computer scientist. Thisway of thinking combines some of the best features of mathematics, engineering, and natural science. Like mathematicians, computer scientists use formallanguages to denote ideas—specifically, computations. Like engineers, theydesign things, assembling components into systems and evaluating trade-offsamong alternatives. And like scientists, they observe the behavior of complexsystems, form hypotheses, and test predictions.An important skill for a computer scientist is problem solving. It involvesthe ability to formulate problems, think creatively about solutions, and expresssolutions clearly and accurately. As it turns out, the process of learning toprogram computers is an excellent opportunity to develop problem-solvingskills. On one level, you will be learning to write Java programs, a useful skillby itself. But on another level, you will use programming as a means to anend. As we go along, that end will become clearer.1.1What Is a Computer?When people hear the word computer, they often think of a desktop or alaptop. Not surprisingly, searching for “computer” on Google Images (https://images.google.com/) displays rows and rows of these types of machines.However, in a more general sense, a computer can be any type of device thatstores and processes data.
2Chapter 1Computer ProgrammingDictionary.com defines a computer as “a programmable electronic device designed to accept data, perform prescribed mathematical and logical operationsat high speed, and display the results of these operations. Mainframes, desktop and laptop computers, tablets, and smartphones are some of the differenttypes of computers.”Each type of computer has its own unique design, but internally they all sharethe same type of hardware. The two most important hardware componentsare processors (or CPUs) that perform simple calculations and memory(or RAM) that temporarily stores information. Figure 1.1 shows what thesecomponents look like.Figure 1.1: Example processor and memory hardware.Users generally see and interact with touchscreens, keyboards, and monitors,but it’s the processors and memory that perform the actual computation.Nowadays it’s fairly standard, even for a smartphone, to have at least eightprocessors and four gigabytes (four billion cells) of memory.1.2What Is Programming?A program is a sequence of instructions that specifies how to perform acomputation on computer hardware. The computation might be somethingmathematical, like solving a system of equations or finding the roots of a polynomial. It could also be a symbolic computation, like searching and replacingtext in a document or (strangely enough) compiling a program.The detai
The Philosophy Behind the Book Here are the guiding principles that make the book the way it is: One concept at a time: We break down topics that give beginners trouble into a series of small steps, so that they can exercise each new concept in isolation before continuing. Balance of Java and concepts: The book