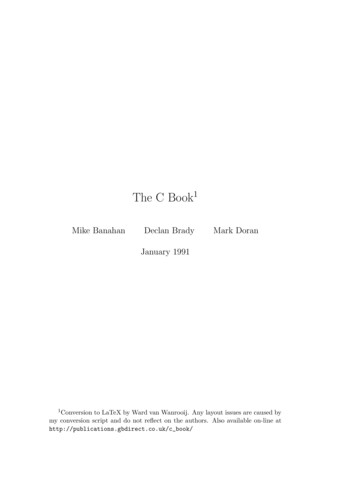
Transcription
The C Book1Mike BanahanDeclan BradyMark DoranJanuary 19911 Conversionto LaTeX by Ward van Wanrooij. Any layout issues are caused bymy conversion script and do not reflect on the authors. Also available on-line athttp://publications.gbdirect.co.uk/c book/
CONTENTSiContentsPrefaceAbout This Book . . . . . . . . . . . . .The Success of C . . . . . . . . . . . . .Standards . . . . . . . . . . . . . . . . .Hosted and Free-Standing EnvironmentsTypographical conventions . . . . . . . .Order of topics . . . . . . . . . . . . . .Example programs . . . . . . . . . . . .Deference to Higher Authority . . . . . .Address for the Standard . . . . . . . . .1 An1.11.21.3.Introduction to CThe form of a C program . . . . . . . . . .Functions . . . . . . . . . . . . . . . . . .A description of Example 1.1 . . . . . . .1.3.1 What was in it . . . . . . . . . . .1.3.2 Layout and comment . . . . . . . .1.3.3 Preprocessor statements . . . . . .1.3.3.1 Define statements . . . . .1.3.3.2 Summary . . . . . . . . .1.3.4 Function declaration and definition1.3.4.1 Declaration . . . . . . . .1.3.4.2 Definition . . . . . . . . .1.3.4.3 Summary . . . . . . . . .1.3.5 Strings . . . . . . . . . . . . . . . .1.3.6 The main function . . . . . . . . .1.3.7 Declarations . . . . . . . . . . . . .1.3.8 Assignment statement . . . . . . .1.3.9 The while statement . . . . . . . .1.3.10 The return statement . . . . . . . .1.3.10.1 Summary . . . . . . . . .1124566777.99101212121314141515151617181819192021
iiCONTENTS1.41.51.61.71.3.11 Progress so far . . . . . . . . . .Some more programs . . . . . . . . . . .1.4.1 A program to find prime numbers1.4.2 The division operators . . . . . .1.4.3 An example performing input . .1.4.4 Simple arrays . . . . . . . . . . .1.4.5 Summary . . . . . . . . . . . . .Terminology . . . . . . . . . . . . . . . .Summary . . . . . . . . . . . . . . . . .Exercises . . . . . . . . . . . . . . . . . .2 Variables and Arithmetic2.1 Some fundamentals . . . . . . . . . . . . . . . . .2.2 The alphabet of C . . . . . . . . . . . . . . . . .2.2.1 Basic Alphabet . . . . . . . . . . . . . . .2.2.2 Trigraphs . . . . . . . . . . . . . . . . . .2.2.3 Multibyte Characters . . . . . . . . . . . .2.2.4 Summary . . . . . . . . . . . . . . . . . .2.3 The Textual Structure of Programs . . . . . . . .2.3.1 Program Layout . . . . . . . . . . . . . . .2.3.2 Comment . . . . . . . . . . . . . . . . . .2.3.3 Translation phases . . . . . . . . . . . . .2.4 Keywords and identifiers . . . . . . . . . . . . . .2.4.1 Keywords . . . . . . . . . . . . . . . . . .2.4.2 Identifiers . . . . . . . . . . . . . . . . . .2.5 Declaration of variables . . . . . . . . . . . . . . .2.6 Real types . . . . . . . . . . . . . . . . . . . . . .2.6.1 Summary of real arithmatic . . . . . . . .2.6.2 Printing real numbers . . . . . . . . . . .2.7 Integral types . . . . . . . . . . . . . . . . . . . .2.7.1 Plain integers . . . . . . . . . . . . . . . .2.7.2 Character variables . . . . . . . . . . . . .2.7.3 More complicated types . . . . . . . . . .2.7.4 Summary of integral types . . . . . . . . .2.7.5 Printing the integral types . . . . . . . . .2.8 Expressions and arithmetic . . . . . . . . . . . . .2.8.1 Conversions . . . . . . . . . . . . . . . . .2.8.1.1 Integral promotions . . . . . . .2.8.1.2 Signed and unsigned integers . .2.8.1.3 Floating and integral . . . . . . .2.8.1.4 The usual arithmetic 6373838383940414444454546495151525354545555
CONTENTS2.8.1.5 Wide characters . . . . . . . . . .2.8.1.6 Casts . . . . . . . . . . . . . . . .2.8.2 Operators . . . . . . . . . . . . . . . . . . .2.8.2.1 The multiplicative operators . . . .2.8.2.2 Additive operators . . . . . . . . .2.8.2.3 The bitwise operators . . . . . . .2.8.2.4 The assignment operators . . . . .2.8.2.5 Increment and decrement operators2.8.3 Precedence and grouping . . . . . . . . . . .2.8.4 Parentheses . . . . . . . . . . . . . . . . . .2.8.5 Side Effects . . . . . . . . . . . . . . . . . .2.9 Constants . . . . . . . . . . . . . . . . . . . . . . .2.9.1 Integer constants . . . . . . . . . . . . . . .2.9.2 Real constants . . . . . . . . . . . . . . . . .2.10 Summary . . . . . . . . . . . . . . . . . . . . . . .2.11 Exercises . . . . . . . . . . . . . . . . . . . . . . . .iii.576062626263656669727373737778793 Control of Flow and Logical Expressions3.1 The Task ahead . . . . . . . . . . . . . . . . . . . . .3.1.1 Logical expressions and Relational Operators .3.2 Control of flow . . . . . . . . . . . . . . . . . . . . .3.2.1 The if statement . . . . . . . . . . . . . . . .3.2.2 The while and do statements . . . . . . . . . .3.2.2.1 Handy hints . . . . . . . . . . . . . .3.2.3 The for statement . . . . . . . . . . . . . . . .3.2.4 A brief pause . . . . . . . . . . . . . . . . . .3.2.5 The switch statement . . . . . . . . . . . . . .3.2.5.1 The major restriction . . . . . . . .3.2.5.2 Integral Constant Expression . . . .3.2.6 The break statement . . . . . . . . . . . . . .3.2.7 The continue statement . . . . . . . . . . . .3.2.8 goto and labels . . . . . . . . . . . . . . . . .3.2.9 Summary . . . . . . . . . . . . . . . . . . . .3.3 More logical expressions . . . . . . . . . . . . . . . .3.4 Strange operators . . . . . . . . . . . . . . . . . . . .3.4.1 The ?: operator . . . . . . . . . . . . . . . . .3.4.2 The comma operator . . . . . . . . . . . . . .3.5 Summary . . . . . . . . . . . . . . . . . . . . . . . .3.6 Exercises . . . . . . . . . . . . . . . . . . . . . . . . .818181838385868789899191929293949596979899100
iv4 Functions4.1 Changes . . . . . . . . . . . . . .4.1.1 Footnotes . . . . . . . . .4.2 The type of functions . . . . . . .4.2.1 Declaring functions . . . .4.2.2 The return statement . . .4.2.3 Arguments to functions . .4.2.4 Function prototypes . . .4.2.5 Argument Conversions . .4.2.6 Function definitions . . . .4.2.6.1 Summary . . . .4.2.7 Compound statements and4.2.8 Footnotes . . . . . . . . .4.3 Recursion and argument passing .4.3.1 Call by value . . . . . . .4.3.2 Call by reference . . . . .4.3.3 Recursion . . . . . . . . .4.3.4 Footnotes . . . . . . . . .4.4 Linkage . . . . . . . . . . . . . .4.4.1 Effect of scope . . . . . . .4.4.2 Internal static . . . . . . .4.4.3 Footnotes . . . . . . . . .4.5 Summary . . . . . . . . . . . . .4.5.1 Footnotes . . . . . . . . .4.6 Exercises . . . . . . . . . . . . . .4.6.1 Footnotes . . . . . . . . .CONTENTS. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .declarations. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .5 Arrays and Pointers5.1 Opening shots . . . . . . . . . . . . . .5.1.1 So why is this important? . . .5.1.2 Effect of the Standard . . . . .5.2 Arrays . . . . . . . . . . . . . . . . . .5.2.1 Multidimensional arrays . . . .5.3 Pointers . . . . . . . . . . . . . . . . .5.3.1 Declaring pointers . . . . . . .5.3.2 Arrays and pointers . . . . . . .5.3.2.1 Summary . . . . . . .5.3.3 Qualified types . . . . . . . . .5.3.4 Pointer arithmetic . . . . . . .5.3.5 void, null and dubious pointers5.4 Character handling . . . . . . . . . . .101. 101. 101. 102. 102. 104. 105. 107. 110. 111. 112. 113. 114. 115. 115. 116. 117. 120. 121. 124. 125. 126. 127. 128. 128. 129.131131131132132133135135141143143145146148
CONTENTS5.55.65.75.85.95.4.1 Strings . . . . . . . . .5.4.2 Pointers and increment5.4.3 Untyped pointers . . .Sizeof and storage allocation .5.5.1 What sizeof can’t do .5.5.2 The type of sizeof . . .Pointers to functions . . . . .Expressions involving pointers5.7.1 Conversions . . . . . .5.7.2 Arithmetic . . . . . . .5.7.3 Relational expressions5.7.4 Assignment . . . . . .5.7.5 Conditional operator .Summary . . . . . . . . . . .Exercises . . . . . . . . . . . .v. . . . . .operators. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .6 Structured Data Types6.1 History . . . . . . . . . . . . . . . . . . .6.2 Structures . . . . . . . . . . . . . . . . .6.2.1 Pointers and structures . . . . . .6.2.2 Linked lists and other structures .6.2.3 Trees . . . . . . . . . . . . . . . .6.3 Unions . . . . . . . . . . . . . . . . . . .6.4 Bitfields . . . . . . . . . . . . . . . . . .6.5 Enums . . . . . . . . . . . . . . . . . . .6.6 Qualifiers and derived types . . . . . . .6.7 Initialization . . . . . . . . . . . . . . . .6.7.1 Constant expressions . . . . . . .6.7.2 More initialization . . . . . . . .6.8 Summary . . . . . . . . . . . . . . . . .6.9 Exercises . . . . . . . . . . . . . . . . . .7 The7.17.27.3PreprocessorEffect of the Standard . . . . . . .How the preprocessor works . . . .Directives . . . . . . . . . . . . . .7.3.1 The null directive . . . . . .7.3.2 # define . . . . . . . . . . .7.3.2.1 Macro substitution7.3.2.2 Stringizing . . . .7.3.2.3 Token pasting . . . 173. 174. 178. 181. 187. 192. 195. 196. 197. 198. 198. 199. 203. 204.205. 205. 206. 208. 208. 209. 210. 211. 212
viCONTENTS.2122132152152172182182202212212228 Specialized Areas of C8.1 Government Health Warning . . . . . . . . . .8.2 Declarations, Definitions and Accessibility . .8.2.1 Storage class specifiers . . . . . . . . .8.2.1.1 Duration . . . . . . . . . . .8.2.2 Scope . . . . . . . . . . . . . . . . . .8.2.3 Linkage . . . . . . . . . . . . . . . . .8.2.4 Linkage and definitions . . . . . . . . .8.2.5 Realistic use of linkage and definitions8.3 Typedef . . . . . . . . . . . . . . . . . . . . .8.4 Const and volatile . . . . . . . . . . . . . . . .8.4.1 Const . . . . . . . . . . . . . . . . . .8.4.2 Volatile . . . . . . . . . . . . . . . . .8.4.2.1 Indivisible Operations . . . .8.5 Sequence points . . . . . . . . . . . . . . . . .8.6 Summary . . . . . . . . . . . . . . . . . . . 247. 247. 247. 248. 249. 250. 251. 252. 254. 256. 257. 2587.47.57.3.2.4 Rescanning . .7.3.2.5 Notes . . . . .7.3.3 # undef . . . . . . . . .7.3.4 # include . . . . . . . .7.3.5 Predefined names . . . .7.3.6 #line . . . . . . . . . . .7.3.7 Conditional compilation7.3.8 #pragma . . . . . . . .7.3.9 #error . . . . . . . . . .Summary . . . . . . . . . . . .Exercises . . . . . . . . . . . . .9 Libraries9.1 Introduction . . . . . . . . . . . . . . . . . . . .9.1.1 Headers and standard types . . . . . . .9.1.2 Character set and cultural dependencies9.1.3 The ¡stddef.h¿ Header . . . . . . . . . .9.1.4 The ¡errno.h¿ Header . . . . . . . . . . .9.2 Diagnostics . . . . . . . . . . . . . . . . . . . .9.3 Character handling . . . . . . . . . . . . . . . .9.4 Localization . . . . . . . . . . . . . . . . . . . .9.4.1 The setlocale function . . . . . . . . . .9.4.2 The localeconv function . . . . . . . . .9.5 Limits . . . . . . . . . . . . . . . . . . . . . . .
CONTENTS9.69.79.89.99.109.119.129.139.149.159.5.1 Limits.h . . . . . . . . . . . . . . . . . . .9.5.2 Float.h . . . . . . . . . . . . . . . . . . . .Mathematical functions . . . . . . . . . . . . . . .Non-local jumps . . . . . . . . . . . . . . . . . . .Signal handling . . . . . . . . . . . . . . . . . . .Variable numbers of arguments . . . . . . . . . .Input and output . . . . . . . . . . . . . . . . . .9.10.1 Introduction . . . . . . . . . . . . . . . . .9.10.2 The I/O model . . . . . . . . . . . . . . .9.10.2.1 Text streams . . . . . . . . . . .9.10.2.2 Binary streams . . . . . . . . . .9.10.2.3 Other streams . . . . . . . . . . .9.10.3 The stdio.h header file . . . . . . . . . . .9.10.4 Opening, closing and buffering of streams .9.10.4.1 Opening . . . . . . . . . . . . . .9.10.4.2 Closing . . . . . . . . . . . . . .9.10.4.3 Buffering . . . . . . . . . . . . .9.10.5 Direct file manipulation . . . . . . . . . .9.10.6 Opening named files . . . . . . . . . . . .9.10.7 Freopen . . . . . . . . . . . . . . . . . . .9.10.8 Closing files . . . . . . . . . . . . . . . . .9.10.9 Setbuf, setvbuf . . . . . . . . . . . . . . .9.10.10 Fflush . . . . . . . . . . . . . . . . . . . .Formatted I/O . . . . . . . . . . . . . . . . . . .9.11.1 Output: the printf family . . . . . . . . .9.11.2 Input: the scanf family . . . . . . . . . . .Character I/O . . . . . . . . . . . . . . . . . . . .9.12.1 Character input . . . . . . . . . . . . . . .9.12.2 Character output . . . . . . . . . . . . . .9.12.3 String output . . . . . . . . . . . . . . . .9.12.4 String input . . . . . . . . . . . . . . . . .Unformatted I/O . . . . . . . . . . . . . . . . . .Random access functions . . . . . . . . . . . . . .9.14.1 Error handling . . . . . . . . . . . . . . .General Utilities . . . . . . . . . . . . . . . . . . .9.15.1 String conversion functions . . . . . . . . .9.15.2 Random number generation . . . . . . . .9.15.3 Memory allocation . . . . . . . . . . . . .9.15.4 Communication with the environment . .9.15.5 Searching and sorting . . . . . . . . . . . .9.15.6 Integer arithmetic functions . . . . . . . 6287289290290292292293294295
viiiCONTENTS9.15.7 Functions using multibyte characters . .9.16 String handling . . . . . . . . . . . . . . . . . .9.16.1 Copying . . . . . . . . . . . . . . . . . .9.16.2 String and byte comparison . . . . . . .9.16.3 Character and string searching functions9.16.4 Miscellaneous functions . . . . . . . . . .9.17 Date and time . . . . . . . . . . . . . . . . . . .9.18 Summary . . . . . . . . . . . . . . . . . . . . .10 Complete Programs in C10.1 Putting it all together . . . . .10.2 Arguments to main . . . . . . .10.3 Interpreting program arguments10.4 A pattern matching program . .10.5 A more ambitious example . . .10.6 Afterword . . . . . . . . . . . .Answers to ExercisesChapter 1 . . . . . .Chapter 2 . . . . . .Chapter 3 . . . . . .Chapter 4 . . . . . .Chapter 5 . . . . . .Chapter 6 . . . . . .Chapter 7 . . . . . 3. 333. 337. 341. 342. 346. 348. 349.
CONTENTS1PrefaceAbout This BookThis book was written with two groups of readers in mind. Whether youare new to C and want to learn it, or already know the older version of thelanguage but want to find out more about the new standard, we hope thatyou will find what follows both instructive and at times entertaining too.This is not a tutorial introduction to programming. The book is designedfor programmers who already have some experience of using a modern highlevel procedural programming language. As we explain later, C isn’t reallyappropriate for complete beginners–though many have managed to use it–so the book will assume that its readers have already done battle with thenotions of statements, variables, conditional execution, arrays, procedures (orsubroutines) and so on. Instead of wasting your time by ploughing throughtedious descriptions of how to add two numbers together and explaining thatthe symbol for multiplication is *, the book concentrates on the things thatare special to C. In particular, it’s the way that C is used which is emphasized.Those who already know C will be interested in the new Standard and howit affects existing C programs. The effect on existing programs might notat first seem to be important to newcomers, but in fact the ‘old’ and newversions of the language are an issue for the beginner too. For some yearsafter the approval of the Standard, programmers will have to live in a worldwhere they can easily encounter a mixture of both the new and the oldlanguage, depending on the age of the programs that they are working with.For that reason, the book highlights where the old and new features differsignificantly. Some of the old features are no ornament to the languageand are well worth avoiding; the Standard goes so far as to consider themobsolescent and recommends that they should not be used. For that reasonthey are not described in detail, but only far enough to allow a reader tounderstand what they mean. Anybody who intends to write programs usingthese old-style features should be reading a different book.This is the second edition of the book, which has been revised to refer to the
2CONTENTSfinal, approved version of the Standard. The first edition of the book wasbased on a draft of the Standard which did contain some differences fromthe draft that was eventually approved. During the revision we have takenthe opportunity to include more summary material and an extra chapterillustrating the use of C and the Standard Library to solve a number of smallproblems.The Success of CC is a remarkable language. Designed originally by one man, Dennis Ritchie,working at AT&T Bell Laboratories in New Jersey, it has increased in useuntil now it may well be one of the most widely-written computer languagesin the world. The success of C is due to a number of factors, none of them key,but all of them important. Perhaps the most significant of all is that C wasdeveloped by real practioners of programming and was designed for practicalday-to-day use, not for show or for demonstration. Like any well-designedtool, it falls easily to the hand and feels good to use. Instead of providingconstraints, checks and rigorous boundaries, it concentrates on providing youwith power and on not getting in your way.Because of this, it’s better for professionals than beginners. In the earlystages of learning to program you need a protective environment that givesfeedback on mistakes and helps you to get results quickly–programs thatrun, even if they don’t do what you meant. C is not like that! A professionalforester would use a chain-saw to cut down trees quickly, aware of the dangersof touching the blade when the machine is running; C programmers work ina similar way. Although modern C compilers do provide a limited amount offeedback when they notice something that is out of the ordinary, you almostalways have the option of forcing the compiler to do what you said youwanted and to stop it from complaining. Provided that what you said youwanted was what you really did want, then you’ll get the result you expected.Programming in C is like eating red meat and drinking strong rum exceptyour arteries and liver are more likely to survive it.Not only is C popular and a powerful asset in the armoury of the seriousday-to-day programmer, there are other reasons for the success of this language. It has always been associated with the UNIX operating system andhas benefited from the increasing popularity of that system. Although itis not the obvious first choice for writing large commercial data processingapplications, C has the great advantage of always being available on commercial UNIX implementations. UNIX is written in C, so whenever UNIXis implemented on a new type of hardware, getting a C compiler to work for
CONTENTS3that system is the first task. As a result it is almost impossible to find aUNIX system without support for C, so the software vendors who want totarget the UNIX marketplace find that C is the best bet if they want to getwide coverage of the systems available. Realistically, C is the first choice forportability of software in the UNIX environment.C has also gained substantially in use and availability from the explosiveexpansion of the Personal Computer market. C could almost have beendesigned specifically for the development of software for the PC–developersget not only the readability and productivity of a high-level language, butalso the power to get the most out of the PC architecture without havingto resort to the use of assembly code. C is practically unique in its abilityto span two levels of programming; as well as providing high-level control offlow, data structures and procedures–all of the stuff expected in a modernhigh-level language–it also allows systems programmers to address machinewords, manipulate bits and get close to the underlying hardware if theywant to. That combination of features is very desirable in the competitivePC software markeplace and an increasing number of software developershave made C their primary language as a result.Finally, the extensibility of C has contributed in no small way to its popularity. Many other languages have failed to provide the file access and generalinput-output features that are needed for industrial-strength applications.Traditionally, in these languages I/O is built-in and is actually understoodby the compiler. A master-stroke in the design of C (and interestingly, oneof the strengths of the UNIX system too) has been to take the view that ifyou don’t know how to provide a complete solution to a generic requirement,instead of providing half a solution (which invariably pleases nobody), youshould allow the users to build their own. Software designers the world overhave something to learn from this! It’s the approach that has been takenby C, and not only for I/O. Through the use of library functions you canextend the language in many ways to provide features that the designersdidn’t think of. There’s proof of this in the so-called Standard I/O Library(stdio), which matured more slowly than the language, but had become asort of standard all of its own before the Standard Committee give it officialblessing. It proved that it is possible to develop a model of file I/O and associated features that is portable to many more systems than UNIX, whichis where it was first wrought. Despite the ability of C to provide access tolow-level hardware features, judicious style and the use of the stdio packageresults in highly portable programs; many of which are to be found runningon top of operating systems that look very different from one another. Thenice thing about this library is that if you don’t like what it does, but youhave the appropriate technical skills, you can usually extend it to do what
4CONTENTSyou do want, or bypass it altogether.StandardsRemarkably, C achieved its success in the absence of a formal standard.Even more remarkable is that during this period of increasingly widespreaduse, there has never been any serious divergence of C into the number ofdialects that has been the bane of, for example, BASIC. In fact, this is notso surprising. There has always been a “language reference manual”, thewidely-known book written by Brian Kernighan and Dennis Ritchie, usuallyreferred to as simply “K&R”.The C Programming Language,B.W. Kernighan and D. M. Ritchie,Prentice-HallEnglewood Cliffs,New Jersey,1978Further acting as a rigorous check on the expansion into numerous dialects,on UNIX systems there was only ever really one compiler for C; the so-called“Portable C Compiler”, originally written by Steve Johnson. This acted asa reference implementation for C–if the K&R reference was a bit obscurethen the behaviour of the UNIX compiler was taken as the definition of thelanguage.Despite this almost ideal situation (a reference manual and a reference implementation are extremely good ways of achieving stability at a very lowcost), the increasing number of alternative implementations of C to be foundin the PC world did begin to threaten the stability of the language.The X3J11 committee of the American National Standards Institute startedwork in the early 1980’s to produce a formal standard for C. The committeetook as its reference the K&R definition and began its lengthy and painstaking work. The job was to try to eliminate ambiguities, to define the undefined, to fix the most annoying deficiencies of the language and to preservethe spirit of C–all this as well as providing as much compatibility with existing practice as was possible. Fortunately, nearly all of the developers of thecompeting versions of C were represented on the committee, which in itselfacted as a strong force for convergence right from the beginning.Development of the Standard took a long time, as standards often do. Muchof the work is not just technical, although that is a very time-consumingpart of the job, but also procedural. It’s easy to underrate the proceduralaspects of standards work, as if it somehow dilutes the purity of the technical
CONTENTS5work, but in fact it is equally important. A standard that has no agreementor consensus in the industry is unlikely to be widely adopted and couldbe useless or even damaging. The painstaking work of obtaining consensusamong committee members is critical to the success of a practical standard,even if at times it means compromising on technical “perfection”, whateverthat might be. It is a democratic process, open to all, which occasionallyresults in aberrations just as much as can excessive indulgence by technicalpurists, and unfortunately the delivery date of the Standard was affected atthe last moment by procedural, rather than technical issues. The technicalwork was completed by December 1988, but it took a further year to resolveprocedural objections. Finally, approval to release the document as a formalAmerican National Standard was given on December 7th, 1989.Hosted and Free-Standing EnvironmentsThe dependency on the use of libraries to extend the language has an important effect on the practical use of C. Not only are the Standard I/O Libraryfunctions important to applications programmers, but there are a number ofother functions that are widely taken almost for granted as being part of thelanguage. String handling, sorting and comparison, character manipulationand similar services are invariably expected in all but the most specializedof applications areas.Because of this unusually heavy dependency on libraries to do real work, itwas most important that the Standard provided comprehensive definitionsfor the supporting functions too. The situation with the library functionswas much more complicated than the relatively simple job of providing atight definition for the language itself, because the library can be extendedor modified by a knowledgeable user and was only partially defined in K&R.In practice, this led to numerous similar but different implementations ofsupporting libraries in common use. By far the hardest part of the work ofthe Committee was to reach a good definition of the library support thatshould be provided. In terms of benefit to the final user of C, it is this workthat will prove to be by far and away the most valuable part of the Standard.However, not all C programs are used for the same type of applications. TheStandard Library is useful for ‘data processing’ types of applications, wherefile I/O and numeric and string oriented data are widely used. There is anequally important application area for C–the ‘embedded system’ area–whichincludes such things as process control, real-time and similar applications.The Standard knows this and provides for it. A large part of the Standardis the definition of the library functions that must be supplied for hosted
6CONTENTSenvironments. A hosted environment is one that provides the standardlibraries. The standard permits both hosted and freestanding environments. and goes to some length to differentiate between them. Who wouldwant to go without libraries? Well, anybody writing ‘stand alone’ programs.Operating systems, embed
level procedural programming language. As we explain later, C isn’t really appropriate for complete beginners{though many have managed to use it{so the book will assume that its readers h