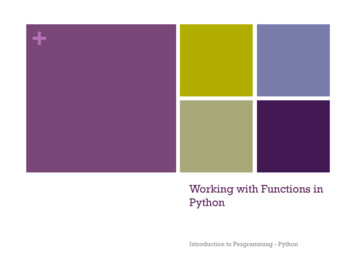
Transcription
Working with Functions inPythonIntroduction to Programming - Python
Functions
FunctionsnA function is a group of statements that exist within aprogram for the purpose of performing a specific tasknSince the beginning of the semester we have been using anumber of Python’s built-in functions, including:nnprint()range()nlen()random.randint()n etcn
FunctionsnMost programs perform tasks that are large enough to bebroken down into subtasksnBecause of this, programmers often organize their programsinto smaller, more manageable chunks by writing their ownfunctionsnInstead of writing one large set of statements we can breakdown a program into several small functions, allowing us to“divide and conquer” a programming problem
Defining FunctionsnFunctions, like variables must be named and created beforeyou can use themnThe same naming rules apply for both variables andfunctionsnnYou can’t use any of Python’s keywordsNo spacesnThe first character must be A-Z or a-z or the “ ” characterAfter the first character you can use A-Z, a-z, “ ” or 0-9nUppercase and lowercase characters are distinctn
Defining functionsdef myfunction():print (“Printed from inside a function”)# call the functionmyfunction()
Some notes on functionsnWhen you run a function you say that you “call” itnOnce a function has completed, Python will return back to theline directly after the initial function callnWhen a function is called programmers commonly say thatthe “control” of the program has been transferred to thefunction. The function is responsible for the program’sexecution.nFunctions must be defined before they can be used. InPython we generally place all of our functions at thebeginning of our programs.
Flow of Execution
Flow of Execution
Flow of Execution
Flow of Execution
Flow of Execution
Flow of Execution
Flow of Execution
Flow of Execution
Flow of Execution
Flow of Execution
Flow of Execution – WithFunctions
Flow of Execution with Functions
Flow of Execution with Functions
Flow of Execution with Functions
Flow of Execution with Functions
Flow of Execution with Functions
Flow of Execution with Functions
Flow of Execution with Functions
Flow of Execution with Functions
Flow of Execution with Functions
Flow of Execution with Functions
Multiple Functions
Multiple functionsdef hello():print (“Hello there!”)def goodbye():print (“See ya!”)hello()goodbye()
Calling functions inside functionsdef main():print (“I have a message for you.”)message()print (“Goodbye!”)def message():print (“The password is ‘foo’”)main()
Programming Challenge: HollowSquarenWrite a program that prints thepattern to the right usingfunctions
Programming Challenge:User Controlled Hollow RectanglenAsk the user for the height fora rectanglenThen draw a rectangle of thatheight
Local Variables
Local VariablesnFunctions are like “mini programs”nYou can create variables inside functions just as you would inyour main program
Local Variablesdef bugs():numbugs int(input(‘How many bugs? ‘))print (numbugs)bugs()
Local VariablesnHowever, variables that are defined inside of a function areconsidered “local” to that function.nThis means that they only exist within that function. Objectsoutside the “scope” of the function will not be able to accessthat variable
Local Variablesdef bugs():numbugs int(input(‘How many bugs? ‘))print (numbugs)bugs()print (numbugs) # error!Variable numbugs# doesn’t exist in this scope!
Local VariablesnDifferent functions can have their own local variables that usethe same variable namenThese local variables will not overwrite one another sincethey exist in different “scopes”
Local Variablesdef newjersey():numbugs 1000print (“NJ has”, numbugs, “bugs”)def newyork():numbugs 2000print (“NY has”, numbugs, “bugs”)newjersey()newyork()
Passing Arguments to aFunction
Passing Arguments to a FunctionnSometimes it’s useful to not only call a function but also sendit one or more pieces of data as an argumentnThis process is identical to what you’ve been doing with thebuilt-in functions we have studied so farnnx random.randint(1,5)y len(‘Obama’)# send 2 integers# send 1 string
Passing Arguments to a Functiondef square(num):print (num**2)# num assumes the value of the# argument that is passed to# the function (5)square(5)
Passing Arguments to a FunctionnWhen passing arguments, you need to let your function knowwhat kind of data it should expect in your function definitionnYou can do this by establishing a variable name in thefunction definition. This variable will be auto declared everytime you call your function, and will assume the value of theargument passed to the function.
Passing Multiple Arguments to aFunctionnYou can actually pass any number of arguments to a functionnOne way to do this is to pass in arguments “by position”
Passing Multiple Arguments to aFunctiondef average(num1, num2, num3):sum num1 num2 num3avg sum / 3print (avg)average(100,90,92)
Programming ChallengenWrite a function that accepts arestaurant check and a tip %nPrint out the tip that should beleft on the table as well as thetotal billnIf the tip is less than 15% youshould tell the user that theymight want to leave a littlemore on the table
Programming Challenge: DistanceFormulanWrite a program that asks theuser to enter two points on a2D plane (i.e. enter X1 & Y1,enter X2 & Y2)nCompute the distancebetween those points using afunction.nContinually ask the user fornumbers until they wish to quit
Programming ChallengenWrite a “joke” generator thatprints out a random knockknock joke.nExtension: Write a “drum roll”function that pauses theprogram for dramatic effect!Have the drum roll functionaccept a parameter thatcontrols how long it shouldpause.
Argument Mechanics
Argument MechanicsnWhen we pass an argument to a function in Python we areactually passing it’s “value” into the function, and not anactual variable
Argument Mechanicsdef change me(v):print ("function got:", v)v 10print ("argument is now:", v)myvar 5print ("starting with:", myvar)change me(myvar)print ("ending with:", myvar)
Argument MechanicsnWe call this behavior “passing by value”nWe are essentially creating two copies of the data that isbeing passed – one that stays in the main program and onethat is passed as an argument into our functionnThis behavior allows us to set up a “one way” communicationmechanism – we can send data into a function as anargument, but the function cannot communicate back byupdating or changing the argument in any wayn(we will talk about how to communicate back to the caller injust a second!)
Global Variables
Global VariablesnWhen you create a variable inside a function we say that thevariable is “local” to that functionnThis means that it can only be accessed by statements insidethe function that created itnWhen a variable is created outside all of your functions it isconsidered a “global variable”nGlobal variables can be accessed by any statement in yourprogram file, including by statements in any functionnAll of the variables we have been creating so far in class havebeen global variables
Global Variablesname 'Obama'def showname():print ("Function:", name)print ("Main program:", name)showname()
Global VariablesnIf you want to be able to change a global variable inside of afunction you must first tell Python that you wish to do thisusing the “global” keyword inside your function
Global Variablesname 'Obama’def showname():global nameprint ("Function 1:", name)name 'John’print ("Function 2:", name)print ("Main program 1:", name)showname()print ("Main program 2:", name)
Global VariablesnGlobal variables can make debugging difficultnFunctions that use global variables are generally dependenton those variables, making your code less portablenWith that said, there are many situations where using globalvariables makes a lot of sense.
Programming ChallengenWrite a very brief “chooseyour own adventure” stylegame using functionsnReference:http://thcnet.net/zork/
Programming Challenge
Value Returning Functions
Value Returning FunctionsnValue returning functions are functions that return a value tothe part of the program that initiated the function callnThey are almost identical to the type of functions we havebeen writing so far, but they have the added ability to sendback information at the end of the function callnWe have secretly been using these all semester!nnsomestring input(“Tell me your name”)somenumber random.randint(1,5)
Writing your own value returningfunctionsnYou use almost the same syntax for writing a value returningfunction as you would for writing a normal functionnThe only difference is that you need to include a “return”statement in your function to tell Python that you intend toreturn a value to the calling programnThe return statement causes a function to end immediately.It’s like the break statement for a loop.nA function will not proceed past its return statement onceencountered. Control of the program is returned back to thecaller.
Value Returning Functionsdef myfunction(arg1, arg2):statementstatement statementreturn expression# call the functionreturnvalue myfunction(10, 50)
Programming Challenge:Combined AgenWrite a function that takes twoage values as integers, addsthem up and returns the resultas an integer
Programming Challenge:Discounted PricingnPrompt the use for an item price(using a function)nApply a 20% discount to theprice (using a function)nPrint the starting price and thediscounted pricenExtension:n Don’t accept price values lessthan 0.05 – repeatedly askthe user to enter new data ifthis happensn Repeat the discountingprocess until the user elects tostop entering data
Programming Challenge: DistanceFormulanWrite a program that asks theuser to enter a point on a 2Dplane (i.e. enter X & Y)nCompute the distancebetween that point and theorigin of the plane (0,0)nIf the distance is 10, tell themthey hit a bullseye. If thedistance is 10, they missed!
IPO NotationnAs you start writing more advanced functions you shouldthink about documenting them based on their Input,Processing and Output (IPO)nExample:# function: add ages# input: age1 (integer), age2 (integer)# processing: combines the two integers# output: returns the combined valuedef add ages(age1, age2):sum age1 age2return sum
Returning Boolean ValuesnBoolean values can drasticallysimplify decision and repetitionstructuresnExample:n Write a program that asks the userfor a part numbern Only accept part #’s that are onthe following list:n 100n 200n 300n 400n 500n Continually prompt the user for apart # until they enter a correctvalue
Returning multiple valuesnFunctions can also return multiple values using the followingsyntax:def testfunction():x 5y 10return x, yp, q testfunction()
Programming Challenge: Two DicenWrite a function that simulates therolling of two dicenThe function should accept a sizeparameter (i.e. how many sides doeseach die have)nThe function should return twovalues which represent the result ofeach rollnExtension:n Make sure both numbers that youreturn are different (i.e. you can’troll doubles or snake eyes)n Build in an argument that lets youspecify whether you want toenforce the no doubles policy
Programming Challenge: Feet toInchesnWrite a function that convertsfeet to inches. It should accepta number of feet as anargument and return theequivalent number of inches.
Programming Challenge:Maximum of two valuesnWrite a function named“maximum” that accepts twointeger values and returns theone that the greater of the twoto the calling program
Modules
ModulesnAll programming languages come pre-packaged with astandard library of functions that are designed to make yourjob as a programmer easiernSome of these functions are built right into the “core” ofPython (print, input, range, etc)nOther more specialized functions are stored in a series offiles called “modules” that Python can access upon requestby using the “import” statementnnimport randomimport time
ModulesnOn a Mac you can actually see these files ns/3.2/lib/python3.2/To see information about a module, you can do the followingin IDLE:nnimport modulenamehelp(modulename)
ModulesnThe import statement tells Python to load the functions thatexist within a specific module into memory and make themavailable in your codenBecause you don’t see the inner workings of a function insidea module we sometimes call them “black boxes”nA “black box” describes a mechanism that accepts input,performs an operation that can’t be seen using that input, andproduces some kind of output
“Black Box” model
Functions in modulesnWe call functions that exist within a module by using “dotnotation” to tell Python to run a function that exists in thatmodulenExample:nnum random.randint(1,5)
Listing functions in a modulenYou can list the functions that exist in a particular module byusing the help() functionnThe help() function takes one argument (a string thatrepresents the name of the module) and returns the usermanual for that module
Creating your own modulesnYou can easily create your own modules that you canpopulate with your own functions. Here’s how:nnnnCreate a new python script (i.e. “myfunctions.py”)Place your function definitions in this scriptCreate a second python script (i.e “myprogram.py”)Import your function module using the import statement:import myfunctionsnCall your functions using dot hingelse()
Programming ChallengenCreate a module called“geometry helper”nWrite two functions in thismodule:nnArea of circlenPerimeter of circleEach of these functions willaccept one argument (aradius) and will print out theresult to the user.
Some additional functions insidethe random modulenFloating point random #’snnum random.random()# generates a float# between 0 and 1nnum random.uniform(1,10)# generates a float# between 1 and 10
Seeding the random numbergeneratornAs we mentioned in an earlier class, the computer does nothave the ability to generate a truly random #nIt uses a series of complicated mathematical formulas thatare based on a known value (usually the system clock)nThe seed function in the random module allows you tospecify the “seed” value for the random #’s that the variousrandom number functions generatenYou can seed the random # generator with whatever valueyou want – and you can even reproduce a random range thisway!
Defining Functions n Functions, like variables must be named and created before you can use them n The same naming rules apply for both variables and functions n You can’t use any of Python’s keywords n No spaces n The first character must be A-Z or a-z or the “_” character n A