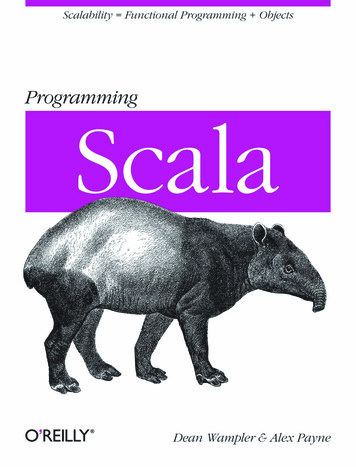
Transcription
Programming Scala
Programming ScalaDean Wampler and Alex PayneBeijing Cambridge Farnham Köln Sebastopol Taipei Tokyo
Programming Scalaby Dean Wampler and Alex PayneCopyright 2009 Dean Wampler and Alex Payne. All rights reserved.Printed in the United States of America.Published by O’Reilly Media, Inc., 1005 Gravenstein Highway North, Sebastopol, CA 95472.O’Reilly books may be purchased for educational, business, or sales promotional use. Online editionsare also available for most titles (http://my.safaribooksonline.com). For more information, contact ourcorporate/institutional sales department: 800-998-9938 or corporate@oreilly.com.Editor: Mike LoukidesProduction Editor: Sarah SchneiderProofreader: Sarah SchneiderIndexer: Ellen Troutman ZaigCover Designer: Karen MontgomeryInterior Designer: David FutatoIllustrator: Robert RomanoPrinting History:September 2009:First Edition.O’Reilly and the O’Reilly logo are registered trademarks of O’Reilly Media, Inc. Programming Scala, theimage of a Malayan tapir, and related trade dress are trademarks of O’Reilly Media, Inc.Many of the designations used by manufacturers and sellers to distinguish their products are claimed astrademarks. Where those designations appear in this book, and O’Reilly Media, Inc. was aware of atrademark claim, the designations have been printed in caps or initial caps.While every precaution has been taken in the preparation of this book, the publisher and authors assumeno responsibility for errors or omissions, or for damages resulting from the use of the information contained herein. This work has been released under the Creative Commons Attribution-Noncommerciallicense.ISBN: 978-0-596-15595-7[M]1252446332
To Dad and Mom, who always believed in me.To Ann, who was always there for me.—DeanTo my mother, who gave me an appreciation forgood writing and the accompanying intellectualtools with which to attempt to produce it.To Kristen, for her unending patience, love, andkindness.—Alex
Table of ContentsForeword . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . xvPreface . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . xvii1. Zero to Sixty: Introducing Scala . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1Why Scala?If You Are a Java Programmer If You Are a Ruby, Python, etc. Programmer Introducing ScalaThe Seductions of ScalaInstalling ScalaFor More InformationA Taste of ScalaA Taste of ConcurrencyRecap and What’s Next112478101016212. Type Less, Do More . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 23In This ChapterSemicolonsVariable DeclarationsMethod DeclarationsMethod Default and Named Arguments (Scala Version 2.8)Nesting Method DefinitionsInferring Type InformationLiteralsInteger LiteralsFloating-Point LiteralsBoolean LiteralsCharacter LiteralsString LiteralsSymbol Literals2323242526282936363738383939vii
TuplesOption, Some, and None: Avoiding nullsOrganizing Code in Files and NamespacesImporting Types and Their MembersImports are RelativeAbstract Types And Parameterized TypesReserved WordsRecap and What’s Next40414445464749523. Rounding Out the Essentials . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 53Operator? Operator?Syntactic SugarMethods Without Parentheses and DotsPrecedence RulesDomain-Specific LanguagesScala if StatementsScala for ComprehensionsA Dog-Simple ExampleFilteringYieldingExpanded ScopeOther Looping ConstructsScala while LoopsScala do-while LoopsGenerator ExpressionsConditional OperatorsPattern MatchingA Simple MatchVariables in MatchesMatching on TypeMatching on SequencesMatching on Tuples (and Guards)Matching on Case ClassesMatching on Regular ExpressionsBinding Nested Variables in Case ClausesUsing try, catch, and finally ClausesConcluding Remarks on Pattern MatchingEnumerationsRecap and What’s 6869707172744. Traits . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 75Introducing TraitsTraits As Mixinsviii Table of Contents7576
Stackable TraitsConstructing TraitsClass or Trait?Recap and What’s Next828687885. Basic Object-Oriented Programming in Scala . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 89Class and Object BasicsParent ClassesConstructors in ScalaCalling Parent Class ConstructorsNested ClassesVisibility RulesPublic VisibilityProtected VisibilityPrivate VisibilityScoped Private and Protected VisibilityFinal Thoughts on VisibilityRecap and What’s Next89919194959698991001021101106. Advanced Object-Oriented Programming In Scala . . . . . . . . . . . . . . . . . . . . . . . . . . 111Overriding Members of Classes and TraitsAttempting to Override final DeclarationsOverriding Abstract and Concrete MethodsOverriding Abstract and Concrete FieldsOverriding Abstract and Concrete Fields in TraitsOverriding Abstract and Concrete Fields in ClassesOverriding Abstract TypesWhen Accessor Methods and Fields Are Indistinguishable: The Uniform Access PrincipleCompanion ObjectsApplyUnapplyApply and UnapplySeq for CollectionsCompanion Objects and Java Static MethodsCase ClassesSyntactic Sugar for Binary OperationsThe copy Method in Scala Version 2.8Case Class InheritanceEquality of ObjectsThe equals MethodThe and ! MethodsThe ne and eq MethodsArray Equality and the sameElements 9140140142143143143143Table of Contents ix
Recap and What’s Next1447. The Scala Object System . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 145The Predef ObjectClasses and Objects: Where Are the Statics?Package ObjectsSealed Class HierarchiesThe Scala Type HierarchyLinearization of an Object’s HierarchyRecap and What’s Next1451481501511551591648. Functional Programming in Scala . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 165What Is Functional Programming?Functions in MathematicsVariables that Aren’tFunctional Programming in ScalaFunction Literals and ClosuresPurity Inside Versus OutsideRecursionTail Calls and Tail-Call OptimizationTrampoline for Tail CallsFunctional Data StructuresLists in Functional ProgrammingMaps in Functional ProgrammingSets in Functional ProgrammingOther Data Structures in Functional ProgrammingTraversing, Mapping, Filtering, Folding, and ReducingTraversalMappingFilteringFolding and ReducingFunctional OptionsPattern MatchingPartial FunctionsCurryingImplicitsImplicit ConversionsImplicit Function ParametersFinal Thoughts on ImplicitsCall by Name, Call by ValueLazy ValsRecap: Functional Component Abstractionsx Table of 174175175178179181182183184186186188189189190192
9. Robust, Scalable Concurrency with Actors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 193The Problems of Shared, Synchronized StateActorsActors in AbstractActors in ScalaSending Messages to ActorsThe MailboxActors in DepthEffective ActorsTraditional Concurrency in Scala: Threading and EventsOne-Off ThreadsUsing java.util.concurrentEventsRecap and What’s Next19319319419419519619720220320320420421010. Herding XML in Scala . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 211Reading XMLExploring XMLLooping and Matching XMLWriting XMLA Real-World ExampleRecap and What’s Next21121221321421521611. Domain-Specific Languages in Scala . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 217Internal DSLsA Payroll Internal DSLInfix Operator NotationImplicit Conversions and User-Defined TypesApply MethodsPayroll Rules DSL ImplementationInternal DSLs: Final ThoughtsExternal DSLs with Parser CombinatorsAbout Parser CombinatorsA Payroll External DSLA Scala Implementation of the External DSL GrammarGenerating Paychecks with the External DSLInternal Versus External DSLs: Final ThoughtsRecap and What’s Next21822222322322422422923023023023323924424512. The Scala Type System . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 247Reflecting on TypesUnderstanding Parameterized TypesManifests248249250Table of Contents xi
Parameterized MethodsVariance Under InheritanceVariance of Mutable TypesVariance In Scala Versus JavaImplementation NotesType BoundsUpper Type BoundsLower Type BoundsA Closer Look at ListsViews and View BoundsNothing and NullUnderstanding Abstract TypesParameterized Types Versus Abstract TypesPath-Dependent TypesC.thisC.superpath.xValue TypesType DesignatorsTuplesParameterized TypesAnnotated TypesCompound TypesInfix TypesFunction TypesType ProjectionsSingleton TypesSelf-Type AnnotationsStructural TypesExistential TypesInfinite Data Structures and LazinessRecap and What’s 13. Application Design . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 289AnnotationsEnumerations Versus Pattern MatchingThoughts On Annotations and EnumerationsEnumerations Versus Case Classes and Pattern MatchingUsing Nulls Versus OptionsOptions and for ComprehensionsExceptions and the AlternativesScalable AbstractionsFine-Grained Visibility Rulesxii Table of Contents289300304304306308311313314
Mixin CompositionSelf-Type Annotations and Abstract Type MembersEffective Design of TraitsDesign PatternsThe Visitor Pattern: A Better AlternativeDependency Injection in Scala: The Cake PatternBetter Design with Design By ContractRecap and What’s Next31631732132532633434034214. Scala Tools, Libraries, and IDE Support . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 343Command-Line Toolsscalac Command-Line ToolThe scala Command-Line ToolThe scalap, javap, and jad Command-Line ToolsThe scaladoc Command-Line ToolThe sbaz Command-Line ToolThe fsc Command-Line ToolBuild ToolsIntegration with IDEsEclipseIntelliJNetBeansText EditorsTest-Driven Development in ScalaScalaTestSpecsScalaCheckOther Notable Scala Libraries and us Smaller LibrariesJava InteroperabilityJava and Scala GenericsUsing Scala Functions in JavaJavaBean PropertiesAnyVal Types and Java PrimitivesScala Names in Java CodeJava Library InteroperabilityAspectJThe Spring 77377381Table of Contents xiii
TerracottaHadoopRecap and What’s Next384384385Appendix: References . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 387Glossary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 393Index . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 407xiv Table of Contents
ForewordIf there has been a common theme throughout my career as a programmer, it has beenthe quest for better abstractions and better tools to support the craft of writing software.Over the years, I have come to value one trait more than any other: composability. Ifone can write code with good composability, it usually means that other traits we software developers value—such as orthogonality, loose coupling, and high cohesion—are already present. It is all connected.When I discovered Scala some years ago, the thing that made the biggest impressionon me was its composability. Through some very elegant design choices and simple yetpowerful abstractions that were taken from the object-oriented and functionalprogramming worlds, Martin Odersky has managed to create a language with highcohesion and orthogonal, deep abstractions that invites composability in all dimensionsof software design. Scala is truly a SCAlable LAnguage that scales with usage, fromscripting all the way up to large-scale enterprise applications and middleware. Scalawas born out of academia, but it has grown into a pragmatic and practical languagethat is very much ready for real-world production use.What excites me most about this book is that it’s so practical. Dean and Alex have donea fantastic job, not only by explaining the language through interesting discussions andsamples, but also by putting it in the context of the real world. Itʼs written for theprogrammer who wants to get things done. I had the pleasure of getting to know Deansome years ago when we were both part of the aspect-oriented programming community. Dean holds a rare mix of deep analytical academic thinking and a pragmatic,get-things-done kind of mentality. Alex, whom I’ve had the pleasure to meet once, isleading the API team at Twitter, Inc. Alex has played a leading role in moving Twitter’scode and infrastructure to Scala, making it one on the first companies to successfullydeploy Scala in production.xv
You are about to learn how to write reusable components using mixin and functioncomposition; how to write concurrent applications using Scala’s Actors; how to makeeffective use of Scala’s XML/XPath support; how to utilize Scalaʼs rich, flexible, andexpressive syntax to build Domain-Specific Languages; how to effectively test yourScala code; how to use Scala with popular frameworks such as Spring, Hadoop, andTerracotta; and much, much more. Enjoy the ride. I sure did.—Jonas BonérIndependent Consultant, Scalable Solutions ABAugust, 2009xvi Foreword
PrefaceProgramming Scala introduces an exciting new language that offers all the benefits ofa modern object model, functional programming, and an advanced type system. Packedwith code examples, this comprehensive book teaches you how to be productive withScala quickly, and explains what makes this language ideal for today’s scalable, distributed, component-based applications that support concurrency and distribution.You’ll also learn how Scala takes advantage of the advanced Java Virtual Machine as aplatform for programming languages.Learn more at http://programmingscala.com or at the book’s catalog page.Welcome to Programming ScalaProgramming languages become popular for many reasons. Sometimes, programmerson a given platform prefer a particular language, or one is institutionalized by a vendor.Most Mac OS programmers use Objective-C. Most Windows programmers use C and .NET languages. Most embedded-systems developers use C and C .Sometimes, popularity derived from technical merit gives way to fashion and fanaticism. C , Java, and Ruby have been the objects of fanatical devotion amongprogrammers.Sometimes, a language becomes popular because it fits the needs of its era. Java wasinitially seen as a perfect fit for browser-based, rich client applications. Smalltalkcaptured the essence of object-oriented programming (OOP) as that model of programming entered the mainstream.Today, concurrency, heterogeneity, always-on services, and ever-shrinking development schedules are driving interest in functional programming (FP). It appears that thedominance of object-oriented programming may be over. Mixing paradigms is becoming popular, even necessary.We gravitated to Scala from other languages because Scala embodies many of the optimal qualities we want in a general-purpose programming language for the kinds ofapplications we build today: reliable, high-performance, highly concurrent Internet andenterprise applications.xvii
Scala is a multi-paradigm language, supporting both object-oriented and functionalprogramming approaches. Scala is scalable, suitable for everything from short scriptsup to large-scale, component-based applications. Scala is sophisticated, incorporatingstate-of-the-art ideas from the halls of computer science departments worldwide. YetScala is practical. Its creator, Martin Odersky, participated in the development of Javafor years and understands the needs of professional developers.Both of us were seduced by Scala, by its concise, elegant, and expressive syntax and bythe breadth of tools it put at our disposal. In this book, we strive to demonstrate whyall these qualities make Scala a compelling and indispensable programming language.If you are an experienced developer who wants a fast, thorough introduction to Scala,this book is for you. You may be evaluating Scala as a replacement for or complementto your current languages. Maybe you have already decided to use Scala, and you needto learn its features and how to use it well. Either way, we hope to illuminate thispowerful language for you in an accessible way.We assume that you are well versed in object-oriented programming, but we don’tassume that you have prior exposure to functional programming. We assume that youare experienced in one or more other programming languages. We draw parallels tofeatures in Java, C#, Ruby, and other languages. If you know any of these languages,we’ll point out similar features in Scala, as well as many features that are new.Whether you come from an object-oriented or functional programming background,you will see how Scala elegantly combines both paradigms, demonstrating their complementary nature. Based on many examples, you will understand how and when toapply OOP and FP techniques to many different design problems.In the end, we hope that you too will be seduced by Scala. Even if Scala does not endup becoming your day-to-day language, we hope you will gain insights that you canapply regardless of which language you are using.Conventions Used in This BookThe following typographical conventions are used in this book:ItalicIndicates new terms, URLs, email addresses, file names, and file extensions. Manyitalicized terms are defined in the Glossary on page 393.Constant widthUsed for program listings, as well as within paragraphs to refer to program elementssuch as variable or function names, databases, data types, environment variables,statements, and keywords.Constant width boldShows commands or other text that should be typed literally by the user.xviii Preface
Constant width italicShows text that should be replaced with user-supplied values or by values determined by context.This icon signifies a tip, suggestion, or general note.This icon indicates a warning or caution.Using Code ExamplesThis book is here to help you get your job done. In general, you may use the code inthis book in your programs and documentation. You do not need to contact us forpermission unless you’re reproducing a significant portion of the code. For example,writing a program that uses several chunks of code from this book does not requirepermission. Selling or distributing a CD-ROM of examples from O’Reilly books doesrequire permission. Answering a question by citing this book and quoting examplecode does not require permission. Incorporating a significant amount of example codefrom this book into your product’s documentation does require permission.We appreciate, but do not require, attribution. An attribution usually includes the title,author, publisher, and ISBN. For example: “Programming Scala by Dean Wampler andAlex Payne. Copyright 2009 Dean Wampler and Alex Payne, 978-0-596-15595-7.”If you feel your use of code examples falls outside fair use or the permission given above,feel free to contact us at permissions@oreilly.com.Getting the Code ExamplesYou can download the code examples from http://examples.oreilly.com/9780596155964/. Unzip the files to a convenient location. See the README.txt file inthe distribution for instructions on building and using the examples.Some of the example files can be run as scripts using the scala command. Others mustbe compiled into class files. Some files contain deliberate errors and won’t compile. Wehave adopted a file naming convention to indicate each of these cases, although as youlearn Scala it should become obvious from the contents of the files, in most cases:*-script.scalaFiles that end in -script.scala can be run on a command line using scala, e.g., scalafoo-script.scala. You can also start scala in the interpreter mode (when youPreface xix
don’t specify a script file) and load any script file in the interpreter using the :loadfilename command.*-wont-compile.scalaFiles that end in -wont-compile.scala contain deliberate errors that will cause themto fail to compile. We use this naming convention, along with one or more embedded comments about the errors, so it will be clear that they are invalid. Also,these files are skipped by the build process for the examples.sake.scalaFiles named sake.scala are used by our build tool, called sake. The README.txtfile describes this tool.*.scalaAll other Scala files must be compiled using scalac. In the distribution, they areused either by other compiled or script files, such as tests, not all of which are listedin this book.Safari Books OnlineSafari Books Online is an on-demand digital library that lets you easilysearch over 7,500 technology and creative reference books and videos tofind the answers you need quickly.With a subscription, you can read any page and watch any video from our library online.Read books on your cell phone and mobile devices. Access new titles before they areavailable for print, and get exclusive access to manuscripts in development and postfeedback for the authors. Copy and paste code samples, organize your favorites, download chapters, bookmark key sections, create notes, print out pages, and benefit fromtons of other time-saving features.O’Reilly Media has uploaded this book to the Safari Books Online service. To have fulldigital access to this book and others on similar topics from O’Reilly and other publishers, sign up for free at http://my.safaribooksonline.com.How to Contact UsPlease address comments and questions concerning this book to the publisher:O’Reilly Media, Inc.1005 Gravenstein Highway NorthSebastopol, CA 95472800-998-9938 (in the United States or Canada)xx Preface
707-829-0515 (international or local)707-829-0104 (fax)We have a web page for this book, where we list errata, examples, and any additionalinformation. You can access this page at:http://oreilly.com/catalog/9780596155957/To comment or ask technical questions about this book, send email to:bookquestions@oreilly.comFor more information about our books, conferences, Resource Centers, and theO’Reilly Network, see our website at:http://oreilly.comAcknowledgmentsAs we developed this book, many people read early drafts and suggested numerousimprovements to the text, for which we are eternally grateful. We are especially gratefulto Steve Jensen, Ramnivas Laddad, Marcel Molina, Bill Venners, and Jonas Bonér fortheir extensive feedback.Much of the feedback we received came through the Safari Rough Cuts releases andthe online edition available at http://programmingscala.com. We are grateful for thefeedback provided by (in no particular order) Iulian Dragos, Nikolaj Lindberg, MattHellige, David Vydra, Ricky Clarkson, Alex Cruise, Josh Cronemeyer, Tyler Jennings,Alan Supynuk, Tony Hillerson, Roger Vaughn, Arbi Sookazian, Bruce Leidl, DanielSobral, Eder Andres Avila, Marek Kubica, Henrik Huttunen, Bhaskar Maddala, GedByrne, Derek Mahar, Geoffrey Wiseman, Peter Rawsthorne, Geoffrey Wiseman, JoeBowbeer, Alexander Battisti, Rob Dickens, Tim MacEachern, Jason Harris, StevenGrady, Bob Follek, Ariel Ortiz, Parth Malwankar, Reid Hochstedler, Jason Zaugg, JonHanson, Mario Gleichmann, David Gates, Zef Hemel, Michael Yee, Marius Kreis,Martin Süsskraut, Javier Vegas, Tobias Hauth, Francesco Bochicchio, Stephen DuncanJr., Patrik Dudits, Jan Niehusmann, Bill Burdick, David Holbrook, Shalom Deitch,Jesper Nordenberg, Esa Laine, Gleb Frank, Simon Andersson, Patrik Dudits, ChrisLewis, Julian Howarth, Dirk Kuzemczak, Henri Gerrits, John Heintz, Stuart Roebuck,and Jungho Kim. Many other readers for whom we only have usernames also providedfeedback. We wish to thank Zack, JoshG, ewilligers, abcoates, brad, teto, pjcj, mkleint,dandoyon, Arek, rue, acangiano, vkelman, bryanl, Jeff, mbaxter, pjb3, kxen, hipertracker, ctran, Ram R., cody, Nolan, Joshua, Ajay, Joe, and anonymous contributors.We apologize if we have overlooked anyone!Our editor, Mike Loukides, knows how to push and prod gentle. He’s been a great helpthroughout this crazy process. Many other people at O’Reilly were always there toanswer our questions and help us move forward.Preface xxi
We thank Jonas Bonér for writing the Foreword for the book. Jonas is a longtime friendand collaborator from the aspect-oriented programming (AOP) community. For years,he has done pioneering work in the Java community. Now he is applying his energiesto promoting Scala and growing that community.Bill Venners graciously provided the quote on the back cover. The first published bookon Scala, Programming in Scala (Artima), that he cowrote with Martin Odersky andLex Spoon, is indispensable for the Scala developer. Bill has also created the wonderfulScalaTest library.We have learned a lot from fellow developers around the world. Besides Jonas and Bill,Debasish Ghosh, James Iry, Daniel Spiewak, David Pollack, Paul Snively, Ola Bini,Daniel Sobral, Josh Suereth, Robey Pointer, Nathan Hamblen, Jorge Ortiz, and othershave illuminated dark corners with their blog entries, forum discussions, and personalconversations.Dean thanks his colleagues at Object Mentor and several developers at client sites formany stimulating discussions on languages, software design, and the pragmatic issuesfacing developers in industry. The members of the Chicago Area Scala Enthusiasts(CASE) group have also been a source of valuable feedback and inspiration.Alex thanks his colleagues at Twitter for their encouragement and superb work indemonstrating Scala’s effectiveness as a language. He also thanks the Bay Area ScalaEnthusiasts (BASE) for their motivation and community.Most of all, we thank Martin Odersky and his team for creating Scala.xxii Preface
CHAPTER 1Zero to Sixty: Introducing ScalaWhy Scala?Today’s enterprise and Internet applications must balance a number of concerns. Theymust be implemented quickly and reliably. New features must be added in short, incremental cycles. Beyond simply providing business logic, applications must supportsecure access, persistence of data, transactional behavior, and other advanced features.Applications must be highly available and scalable, requiring designs that supportconcurrency and distribution. Applications are networked and provide interfaces forboth people and other applications to use.To meet these challenges, many developers are looking for new languages and tools.Venerable standbys like Java, C#, and C are no longer optimal for developing thenext generation of applications.If You Are a Java Programmer Java was officially introduced by Sun Microsystems in May of 1995, at the advent ofwidespread interest in the Internet. Java was immediately hailed as an ideal languagefor writing browser-based applets, where a secure, portable, and developer-friendlyapplication language was needed. The reigning language of the day, C , was notsuitable for this domain.Today, Java is more often used for server-side applications. It is one of the most popularlanguages in use for the development of web and enterprise applications.However, Java was a child of its time. Now it shows its age. In 1995, Java provided asyntax similar enough to C to entice C developers, while avoiding many of thatlanguage’s deficiencies and “sharp edges.” Java adopted the most useful ideas for thedevelopment problems of its era, such as object-oriented programming (OOP), whilediscarding more troublesome techniques, such as manual memory management. Thesedesign choices struck an excellent balance that minimized complexity and maximizeddeveloper productivity, while trading-off performance compared to natively compiled1
code. While Java has evolved since its birth, many people believe it has grown toocomplex without adequately addressing some newer development challenges.Developers want languages that are more succinct and flexible to improve their productivity. This is one reason why so-called scripting languages like Ruby and Pythonhave become more popular recently.The never-ending need to scale is driving architectures toward pervasive concurrency.However, Java’s concurrency model, which is based on synchronized access to shared,mutable state, results in complex and error-prone programs.While the Java language is showing its age, the Java Virtual Machine (JVM) on whichit runs continues to shine. The optimizations performed by today’s JVM are extraordinary, allowing byte code to outperform natively compiled code in many cases. Today,many developers believe that using the JVM with new languages is the path forward.Sun is embracing this trend by employing many of the lead developers of JRuby andJython, which are JVM ports of Ruby and Python, respectively.The appeal of Scala for the Java developer is that it gives you a newer, more modernlanguage, while leveraging the JVM’s amazing performance and the wealth of Javalibraries that have been developed for over a decade.If You Are a Ruby, Python, etc. Programmer Dynamically typed languages like Ruby, Python, Groovy, JavaScript, and Smalltalkoffer very high productivity due to their flexibility, powerful metaprogramming, andelegance.Statically Typed Versus Dynamically Typed LanguagesOne of the fundamental language design choices is static versus dynamic typing.The word “typing” is used in many contexts in software. The following is a “plausible”definition that is useful for our purposes.A type system is a
What Is Functional Programming? 165 Functions in Mathematics 166 Variables that Aren't 166 Functional Programming in Scala 167 Function Literals and Closures 169 Purity Inside Versus Outside 169 Recursion 170 Tail Calls and Tail-Call Optimization 171 Trampoline for Tail Calls 172 Functional Data Structures 172 Lists in Functional Programming 173