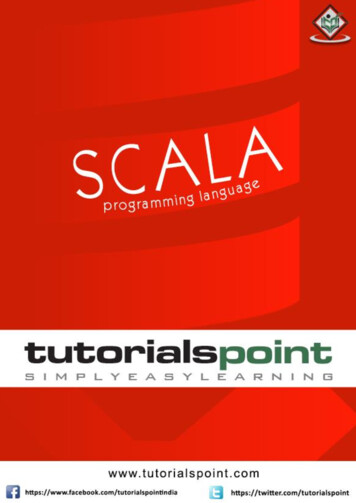
Transcription
ScalaAbout the TutorialScala is a modern multi-paradigm programming language designed to express commonprogramming patterns in a concise, elegant, and type-safe way. Scala has been createdby Martin Odersky and he released the first version in 2003.Scala smoothly integrates the features of object-oriented and functional languages. Thistutorial explains the basics of Scala in a simple and reader-friendly way.AudienceThis tutorial has been prepared for beginners to help them understand the basics of Scalain simple and easy steps. After completing this tutorial, you will find yourself at a moderatelevel of expertise in using Scala from where you can take yourself to next levels.PrerequisitesScala Programming is based on Java, so if you are aware of Java syntax, then it's prettyeasy to learn Scala. Further if you do not have expertise in Java but if you know any otherprogramming language like C, C or Python then it will also help in grasping Scalaconcepts very quickly.Disclaimer & Copyright Copyright 2015 by Tutorials Point (I) Pvt. Ltd.All the content and graphics published in this e-book are the property of Tutorials Point (I)Pvt. Ltd. The user of this e-book is prohibited to reuse, retain, copy, distribute or republishany contents or a part of contents of this e-book in any manner without written consentof the publisher.We strive to update the contents of our website and tutorials as timely and as precisely aspossible, however, the contents may contain inaccuracies or errors. Tutorials Point (I) Pvt.Ltd. provides no guarantee regarding the accuracy, timeliness or completeness of ourwebsite or its contents including this tutorial. If you discover any errors on our website orin this tutorial, please notify us at contact@tutorialspoint.com.i
ScalaTable of ContentsAbout this Tutorial . iAudience . iPrerequisites . iDisclaimer & Copyright . iTable of Contents . ii1.SCALA – OVERVIEW. 1Scala vs Java . 2Scala Web Frameworks . 22.SCALA – ENVIRONMENT . 3Step 1: Verify your Java Installation . 3Step 2: Set your Java Environment . 4Step 3: Install Scala . 43.SCALA – BASICS . 7First Scala Program . 7Script Mode . 8Basic Syntax . 9Scala Identifiers . 9Scala Keywords. 10Comments in Scala . 11Blank Lines and Whitespace . 11Newline Characters . 12Scala Packages . 12Apply Dynamic . 12ii
Scala4.SCALA – DATA . 14Scala Basic Literals . 14Escape Sequences . 165.SCALA – VARIABLES . 18Variable Declaration . 18Variable Data Types . 18Variable Type Inference . 19Multiple assignments . 19Example Program . 19Variable Scope . 206.SCALA – CLASSES & OBJECTS. 22Basic Class . 22Extending a class . 24Implicit Classes . 26Singleton Objects. 287.SCALA – ACCESS MODIFIERS . 30Private Members . 30Protected Members. 30Public Members. 31Scope of Protection . 328.SCALA – OPERATORS . 34Arithmetic Operators. 34Relational Operators . 35Logical Operators . 37Bitwise Operators . 39iii
ScalaAssignment Operators . 42Operators Precedence in Scala . 469.SCALA – IF ELSE STATEMENT . 48if Statement. 48If-else Statement . 49If-else-if-else Statement . 50Nested if-else Statement . 5210.SCALA – LOOP STATEMENTS . 54While loop . 55do-while loop . 57for Loop . 59Loop Control Statements . 66Break Statement. 66Breaking Nested Loops . 68The infinite Loop. 7011.SCALA – FUNCTIONS . 72Function Declarations . 72Function Definitions . 72Calling Functions . 73Function Call-by-Name . 74Function with Variable Arguments . 75Function Default parameter values . 76Nested Functions . 77Partially Applied Functions . 78Function with Named arguments . 80Recursion Functions . 81iv
ScalaHigher-Order Functions . 82Anonymous Functions . 83Currying Functions . 8412.SCALA – CLOSURES . 8613.SCALA – STRINGS . 88Creating a String . 88String Length . 89Concatenating Strings . 89Creating Format Strings . 90String Interpolation . 91The ‘f’ Interpolator . 92String Methods . 9414.SCALA – ARRAYS. 98Declaring Array Variables . 98Processing Arrays . 99Multi-Dimensional Arrays . 100Concatenate Arrays . 102Create Array with Range . 103Scala Array Methods . 10415.SCALA – COLLECTIONS . 106Scala Lists . 106Creating Uniform Lists . 109Tabulating a Function . 110Scala List Methods . 111Scala Sets . 114v
ScalaBasic Operations on set . 115Find max, min elements in set . 117Find Common Values Insets . 118Scala Map [K, V] . 122Concatenating Maps . 124Print Keys and Values from a Map . 125Check for a key in Map . 126Scala Map Methods . 127Scala Tuples . 131Iterate over the Tuple . 132Converting to String. 133Scala Options . 134Using getOrElse() Method . 136Using isEmpty() Method . 137Scala Option Methods . 137Scala Iterators . 139Find Min & Max values Element . 140Find the length of the Iterator . 140Scala Iterator Methods . 14116.SCALA – TRAITS . 146Value classes and Universal traits . 148When to Use Traits? . 14817.SCALA – PATTERN MATCHING. 150Matching using case Classes . 15118.SCALA – REGULAR EXPRESSIONNS . 154Forming Regular Expressions . 156vi
ScalaRegular-Expression Examples . 15819.SCALA – EXCEPTION HANDLING . 161Throwing Exceptions . 161Catching Exceptions . 161The finally Clause . 16220.SCALA – EXTRACTORS . 164Example . 164Pattern Matching with Extractors . 16521.SCALA – FILES I/O . 167Reading a Line from Command Line . 167Reading File Content . 168vii
1. SCALA – OVERVIEWScala, short for Scalable Language, is a hybrid functional programming language. It wascreated by Martin Odersky. Scala smoothly integrates the features of object-oriented andfunctional languages. Scala is compiled to run on the Java Virtual Machine. Many existingcompanies, who depend on Java for business critical applications, are turning to Scala to boosttheir development productivity, applications scalability and overall reliability.Here we have presented a few points that makes Scala the first choice of applicationdevelopers.Scala is object-orientedScala is a pure object-oriented language in the sense that every value is an object. Types andbehavior of objects are described by classes and traits which will be explained in subsequentchapters.Classes are extended by subclassing and a flexible Mixin-based composition mechanismas a clean replacement for multiple inheritance.Scala is functionalScala is also a functional language in the sense that every function is a value and every valueis an object so ultimately every function is an object.Scala provides a lightweight syntax for defining anonymous functions, it supports higherorder functions, it allows functions to be nested, and supports currying functions. Theseconcepts will be explained in subsequent chapters.Scala is statically typedScala, unlike some of the other statically typed languages (C, Pascal, Rust, etc.), does notexpect you to provide redundant type information. You don't have to specify a type in mostcases, and you certainly don't have to repeat it.Scala runs on the JVMScala is compiled into Java Byte Code which is executed by the Java Virtual Machine (JVM).This means that Scala and Java have a common runtime platform. You can easily move fromJava to Scala.The Scala compiler compiles your Scala code into Java Byte Code, which can then be executedby the ‘scala’ command. The ‘scala’ command is similar to the java command, in that itexecutes your compiled Scala code.
ScalaScala can Execute Java CodeScala enables you to use all the classes of the Java SDK and also your own custom Javaclasses, or your favorite Java open source projects.Scala can do Concurrent & Synchronize processingScala allows you to express general programming patterns in an effective way. It reduces thenumber of lines and helps the programmer to code in a type-safe way. It allows you to writecodes in an immutable manner, which makes it easy to apply concurrency and parallelism(Synchronize).Scala vs JavaScala has a set of features that completely differ from Java. Some of these are: All types are objects Type inference Nested Functions Functions are objects Domain specific language (DSL) support Traits Closures Concurrency support inspired by ErlangScala Web FrameworksScala is being used everywhere and importantly in enterprise web applications. You can checka few of the most popular Scala web frameworks: The Lift Framework The Play framework The Bowler framework2
2. SCALA – ENVIRONMENTScalaScala can be installed on any UNIX flavored or Windows based system. Before you startinstalling Scala on your machine, you must have Java 1.8 or greater installed on yourcomputer.Follow the steps given below to install Scala.Step 1: Verify Your Java InstallationFirst of all, you need to have Java Software Development Kit (SDK) installed on your system.To verify this, execute any of the following two commands depending on the platform you areworking on.If the Java installation has been done properly, then it will display the current version andspecification of your Java installation. A sample output is given in the following table.PlatformCommandOpen Command Consoleand type:Windows\ java –versionSample OutputJava version "1.8.0 31"Java (TM) SE Run TimeEnvironment (build 1.8.0 31-b31)Java Hotspot (TM) 64-bit ServerVM (build 25.31-b07, mixed mode)Open Command terminaland type:Linux java –versionJava version "1.8.0 31"OpenJDKRuntime Environment(rhel-2.8.10.4.el6 4-x86 64)Open JDK 64-Bit Server VM (build 25.31b07, mixed mode)3
ScalaWe assume that the readers of this tutorial have Java SDK version 1.8.0 31 installed on theirsystem.In case you do not have Java SDK, download its current se/downloads/index.html and install it.fromStep 2: Set Your Java EnvironmentSet the environment variable JAVA HOME to point to the base directory location where Javais installed on your machine. For example,PlatformDescriptionWindowsSet JAVA HOME to C:\ProgramFiles\java\jdk1.7.0 60LinuxExport JAVA HOME /usr/local/java-currentAppend the full path of Java compiler location to the System Path.PlatformDescriptionWindowsAppend the String "C:\Program Files\Java\jdk1.7.0 60\bin" to the end ofthe system variable PATH.LinuxExport PATH PATH: JAVA HOME/bin/Execute the command java -version from the command prompt as explained above.Step 3: Install ScalaYou can download Scala from http://www.scala-lang.org/downloads. At the time of writingthis tutorial, I downloaded ‘scala-2.11.5-installer.jar’. Make sure you have admin privilege toproceed. Now, execute the following command at the command prompt:PlatformCommand & OutputDescription4
Scala\ java –jar scala-2.11.5-installer.jarWindows\ This command will display aninstallation wizard, which willguide you to install Scala on yourwindowsmachine.Duringinstallation, it will ask for licenseagreement, simply accept it andfurther it will ask a path whereScala will be installed. I selecteddefault given path “C:\ProgramFiles\Scala”, you can select asuitablepathasperyourconvenience.Command: java –jar scala-2.9.0.1-installer.jarOutput:Welcome to the installation of Scala2.9.0.1!The homepagelang.org/Linuxisat:http://Scala-press 1 to continue, 2 to quit, 3 toredisplay1During installation, it will ask forlicense agreement, to accept ittype 1 and it will ask a path whereScala will be installed. I entered/usr/local/share, you can select asuitablepathasperyourconvenience.[ Starting to unpack ][ Processing package: Software PackageInstallation (1/1) ][ Unpacking finished ][ Console installation done ]Finally, open a new command prompt and type Scala -version and press Enter. You shouldsee the following:PlatformCommandOutput5
ScalaWindowsLinux\ scala -versionScala code runner version2.11.5 -- Copyright 2002-2013,LAMP/EPFL scala -versionScala code runner version2.9.0.1 – Copyright 2002-2013,LAMP/EPFL6
3. SCALA – BASICSScalaIf you have a good understanding on Java, then it will be very easy for you to learn Scala.The biggest syntactic difference between Scala and Java is that the ‘;’ line end character isoptional.When we consider a Scala program, it can be defined as a collection of objects thatcommunicate via invoking each other’s methods. Let us now briefly look into what do class,object, methods and instant variables mean. Object - Objects have states and behaviors. An object is an instance of a class.Example: A dog has states - color, name, breed as well as behaviors - wagging,barking, and eating. Class - A class can be defined as a template/blueprint that describes thebehaviors/states that object of its type support. Methods - A method is basically a behavior. A class can contain many methods. It isin methods where the logics are written, data is manipulated and all the actions areexecuted. Fields - Each object has its unique set of instant variables, which are called fields. Anobject's state is created by the values assigned to these fields. Closure - A closure is a function, whose return value depends on the value of one ormore variables declared outside this function. Traits - A trait encapsulates method and field definitions, which can then be reusedby mixing them into classes. Traits are used to define object types by specifying thesignature of the supported methods.First Scala ProgramWe can execute a Scala program in two modes: one is interactive mode and another isscript mode.Interactive ModeOpen the command prompt and use the following command to open Scala.\ ScalaIf Scala is installed in your system, the following output will be displayed:7
ScalaWelcome to Scala version 2.9.0.1Type in expressions to have them evaluated.Type: help for more information.Type the following text to the right of the Scala prompt and press the Enter key:Scala println(“Hello, scala”);It will produce the following result:Hello, Scala!Script ModeUse the following instructions to write a Scala program in script mode. Open notepad and addthe following code int
Scala, short for Scalable Language, is a hybrid functional programming language. It was created by Martin Odersky. Scala smoothly integrates the features of object-oriented and functional languages. Scala is c