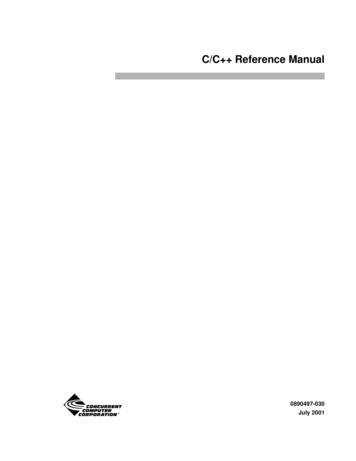
Transcription
C/C Reference Manual0890497-030July 2001
Copyright 2001 by Concurrent Computer Corporation. All rights reserved. This publication or any part thereof isintended for use with Concurrent Computer Corporation products by Concurrent Computer Corporation personnel,customers, and end–users. It may not be reproduced in any form without the written permission of the publisher.The information contained in this document is believed to be correct at the time of publication. It is subject to changewithout notice. Concurrent Computer Corporation makes no warranties, expressed or implied, concerning theinformation contained in this document.To report an error or comment on a specific portion of the manual, photocopy the page in question and mark thecorrection or comment on the copy. Mail the copy (and any additional comments) to Concurrent Computer Corporation, 2881 Gateway Drive Pompano Beach FL 33069. Mark the envelope “Attention: Publications Department.”This publication may not be reproduced for any other reason in any form without written permission of the publisher.Acknowledgment: This manual contains material contributed by Edison Design Group, Inc. Those portions are copyrighted and reproduced withpermission.The license management portion of this product is based on:Élan License ManagerCopyright 1989-1993 Elan Computer Group, Inc.All rights reserved.Élan License Manager is a trademark of Élan Computer Group, Inc.NightView and PowerMAX OS are trademarks of Concurrent Computer Corporation.POSIX is a trademark of the Institute of Electrical and Electronics Engineers, Inc.IBM and Power PC are trademarks of IBM Corp.UNIX is a registered trademark licensed exclusively by the X/Open Company Ltd.X is a trademark of The Open Group.HyperHelp is a trademark of Bristol Technology Inc.Printed in U. S. A.Revision History:Level:Effective With:Original Release -- July 1996000PowerMAX OS 3.1Current Release -- July 2001030PowerMAX OS 5.3
PrefaceScope of ManualThis manual is a reference document on Concurrent C/C , two general-purpose programming languages.Information in this manual applies to the platforms described in the latest ConcurrentComputer Corporation product catalogs.System manual page (man page) descriptions of programs, system calls and subroutinescan be found online.Syntax NotationThe following notation is used throughout this guide:italicBooks, reference cards, and items that the user must specify appearin italic type. Special terms may also appear in italic.list boldUser input appears in list bold type and must be entered exactlyas shown. Names of directories, files, commands, options and manpage references also appear in list bold type.listOperating system and program output such as prompts and messagesand listings of files and programs appears in list type.[]Brackets enclose command options and arguments that are optional.You do not type the brackets if you choose to specify such option orarguments.{}Braces enclose mutually exclusive choices separated by the pipe ( )character, where one choice must be selected. You do not type thebraces with the choice.An ellipsis follows an item that can be repeated.:: This symbol means “is defined as” in Backus-Naur Form (BNF).i
C/C Reference ManualReferenced PublicationsThe following Concurrent publications are referenced in this document:ii0890459Compilation Systems Volume 1 (Tools)0890460Compilation Systems Volume 2 (Concepts)
ContentsChapter 1 CompilationCompilation Phases . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Compiler Invocation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Program Development Environment . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Multiple Release Support . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Using Concurrent C/C with the PLDE . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .PATH Considerations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Include Files and Libraries . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .OS Versions and Target Architectures . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Shared vs. Static Linking . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Makefile Considerations. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Explicit Modification Using ec/ec . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Use of /usr/ccs/crossbin in PATH Environment Variable . . . . . . . . . . . . . .Use of CC Environment or Make Variables . . . . . . . . . . . . . . . . . . . . . . . .Invoking the Compiler . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Command Line Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Compilation Process . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Preprocessing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Key Characters . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .C Specific Features . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Error Messages. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Other. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Language Dialect. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Optimization . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Linking . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 141-141-141-161-181-191-201-241-341-41Chapter 2 Using the Program Development EnvironmentHello World - An Example . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Creating an environment . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Introducing units. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Defining a partition. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Building a partition . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Success!!! . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Let’s look around. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Listing the contents of your environment . . . . . . . . . . . . . . . . . . . . . . . . . .Viewing the source for a particular unit . . . . . . . . . . . . . . . . . . . . . . . . . . .Looking at the Environment Search Path . . . . . . . . . . . . . . . . . . . . . . . . . .What are my options? . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Hello Galaxy - The Example Continues. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Modifying an existing unit . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Building a unit with references outside the local environment . . . . . . . . . . . . .Adding an environment to the Environment Search Path . . . . . . . . . . . . . . . . .Making contact!!! . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Who resides here now?. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2-132-13iii
C/C Reference ManualConclusion . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .2-15Chapter 3 Program Development Environment ConceptsEnvironments . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Local Environments . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Foreign Environments. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Environment Search Path . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Naturalization . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Fetching . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Freezing Environments . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Environment-wide Compile Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Units . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Unit Identification. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Nationalities . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Local Units . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Foreign Units . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Artificial Units . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Unit Compile Options. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Partitions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Types of Partitions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Executable Partitions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Archives. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Shared Objects . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Lazy Versus Immediate Binding . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Position Independent Code. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Share Path . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Issues to consider . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Object Files . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Link Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Compilation and Program Generation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Compilation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Automatic Compilation Utility . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Compile Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Environment-wide Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Permanent Unit Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Temporary Unit Options. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Effective Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Compilation States. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Consistency . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Programming Hints and Caveats . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Linking Executable Programs. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Debugging . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Real-Time Debugging. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Debug Information and cprs . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Source Control Integration. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Makefile Integration. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . -103-113-113-123-133-133-133-133-133-143-14Chapter 4 Program Development Environment UtilitiesCommon Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.analyze. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Link-Time Optimizations with c.analyze . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .iv4-34-44-6
ContentsProfiling with c.analyze . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.build . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.cat . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.chmod . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.compile . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.demangle. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.edit . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.error. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.expel . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.features . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.fetch . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.freeze. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.grep . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.help . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.install. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.instantiation. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.intro . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.invalid . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.link . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.ls . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Formatting the listing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Sorting . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.lssrc . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.make . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.man . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.mkenv . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.options. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Option Sets . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Listing options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Setting options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Modifying options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Clearing options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Deleting options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Keeping temporary options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Setting options on foreign units . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.partition . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Link Options. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.path . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.prelink . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.release . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.report . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.restore . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.rmenv . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.rmsrc . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.script . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .c.touch . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Link Options . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 74-584-594-614-634-644-654-664-694-70C Dialect Accepted . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .New Language Features Accepted . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .New Language Features Not Accepted . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .5-15-25-4Chapter 5 Dialectsv
C/C Reference ManualAnachronisms Accepted . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Extensions Accepted in Normal C Mode . . . . . . . . . . . . . . . . . . . . . . . . . . . .Extensions Accepted in cfront 2.1 Compatibility Mode. . . . . . . . . . . . . . . . . . .Extensions Accepted in cfront 2.1 and 3.0 Compatibility Mode . . . . . . . . . . . .C Dialect Accepted . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .C9X Extensions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .ANSI C Extensions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .K&R/pcc Mode. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Extensions Accepted in SVR4 Compatibility Mode . . . . . . . . . . . . . . . . . . . . .5-55-65-85-95-145-145-165-205-25Chapter 6 Special Features of C Namespace Support . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Template Instantiation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Automatic Instantiation. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Instantiation Modes. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Instantiation #pragma Directives . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Implicit Inclusion . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Automatic Instantiation Issues . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .The Problem . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Solving the Problem with --prelink objects . . . . . . . . . . . . . . . . .Solving the Problem with the PDE Tools . . . . . . . . . . . . . . . . . . . . . . . . . .Solving the Problem with Makefiles and the PDE Tools . . . . . . . . . . . . . .Miscellaneous Notes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Predefined Macros . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Pragmas . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Edison Defined Pragmas. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Concurrent Defined Pragmas . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Source Listing Controls . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Optimization Directives. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Data Alignment Control Directives . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Data Alignment Rules . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .#pragma align . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .#pragma min align. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Miscellaneous Directives. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .#pragma once . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .#pragma ident . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .#pragma weak. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Template Instantiation Pragmas. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Precompiled Headers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Automatic Precompiled Header Processing . . . . . . . . . . . . . . . . . . . . . . . . . . . .Manual Precompiled Header Processing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Other Ways for Users to Control Precompiled Headers . . . . . . . . . . . . . . . . . . .Performance Issues . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Intrinsic Functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .AltiVec Technology Programming Interface . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .New Keywords for AltiVec. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .New Intrinsic Functions for AltiVec . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .New Pragma for AltiVec. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .varargs/stdarg for AltiVec . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Runtime for AltiVec . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Interoperability with Non-AltiVec for AltiVec . . . . . . . . . . . . . . . . . . . . . . . . . .Environment Variables . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . -366-366-37
ContentsDiagnostic Messages . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Termination Messages . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Response to Signals . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Exit Status . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Finding Include Files . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .6-376-396-406-406-40Chapter 7 Compilation ModesANSI C Mode . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Normal C Mode. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Strictly-Conforming Mode . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .cfront 2.1 Compatibility Mode . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .cfront 3.0 Compatibility Mode . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Transition Mode . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Old Mode . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Mode Features . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Common Features. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Differentiating Features . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Preprocessing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Type-Promotion Rules. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Binary Operator Expressions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Escape Characters . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Redeclaration of Typedefs. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Scope of Parameters . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Header File Features . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Function Prototypes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Name-Space Restrictions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Library Enhancements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Locale-Support Enhancements . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Anachronism Mode . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 7-157-157-157-167-167-187-18Chapter 8 Runtime LibrariesRuntime Library . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .General . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Language Support Library . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Linking . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Template Instantiation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Cfront Libraries . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .8-18-18-18-28-28-3Appendix A ANSI C ImplementationLexical Conventions (Chapter 2). . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Phases of Translation (2.1) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Character Literals (2.9.2) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .String Literals (2.9.4) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Basic Concepts (Chapter 3) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Types (3.9) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Main Function (3.6.1). . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Fundamental Types (3.9.1) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Standard Conversions (Chapter 4). . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
This manual is a reference document on Concurrent C/C , two general-purpose pro-gramming languages. Information in this manual applies to the platforms described in the latest Concurrent Computer Corporation product catalogs. System manual page (man page) descriptions of programs, system calls and subroutines