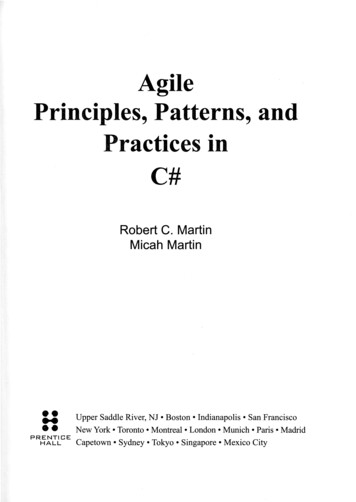
Transcription
AgilePrinciples, Patterns, andPractices inC#Robert C. MartinMicah Martin2 2 !ENTICEHALLUpper Saddle River, NJ Boston Indianapolis San FranciscoNew York Toronto Montreal London Munich Paris Madrid,„, ,„.Capetown Sydney Tokyo Smgapore Mexico City
iAboutthe AuthorsxxxiiiSection I: Agile Development1Chapter 1: Agile Practices3The Agile AllianceIndividuais and Interactions over Processes and ToolsWorking Software over Comprehensive DocumentationCustomer Collaboration over Contract NegotiationResponding to Change over Following aPlanPrinciplesConclusionBibliographyChapter 2: Overview of Extreme ProgrammingThe Practices of Extreme ProgrammingWholeTeamUser StoriesShort CyclesAcceptance TestsPair ProgrammingTest-Driven Development (TDD)45667810111314141415151617V
viContentsCollective OwnershipContinuous IntegrationSustainable PaceOpen WorkspaceThe Planning GameSimple pter3: PlanningInitial ExplorationSpiking, Splitting, and VelocityRelease PlanningIteration PlanningDefining "Done"Task pter4: TestingTest-Driven DevelopmentExample of Test-First DesignTest IsolationSerendipitous DecouplingAcceptance TestsSerendipitous 22222324242525262627282929313232333636373839
ContentsviiChapter 5: Refactoring41A Simple Example of Refactoring: Generating PrimesUnitTestingRefactoringThe Final RereadConclusionBibliographyChapter 6: A Programming EpisodeThe Bowling GameConclusionOverview of the Rules of Bowling42444549535455569899Section II: Agile Design101Chapter 7: What Is Agile Design?103Design SmellsDesign Smells—The Odors of Rotting ss ComplexityNeedless RepetitionOpacityWhy Software RotsThe Copy ProgramA Familiär ScenarioAgile Design of the Copy 106107107108108111113114
viiiContentsChapter 8: The Single-Responsibility Principle (SRP)Defining a ResponsibilitySeparating Coupled 15117119119119120Chapter 9: The Open/Closed Principle (OCP)121DescriptionofOCPThe Shape ApplicationViolatingOCPConforming to OCPAnticipation and "Natural" StructurePutting the "Hooks" InUsing Abstraction to Gain Explicit ClosureUsing a Data-Driven Approach to Achieve 131132133Chapter 10: The Liskov Substitution Principle (LSP)ViolationsofLSPA Simple ExampleA More Subtle ViolationA Real-World ExampleFactoring Instead of DerivingHeuristics and 8150151151Chapter 11: The Dependency-Inversion Principle (DIP).153LayeringOwnership InversionDependence on AbstractionsA Simple DIP ExampleFinding the Underlying AbstractionThe Furnace 162
ContentsChapter 12: The Interface Segregation Principle (ISP)Interface PollutionSeparate Clients Mean Separate InterfacesClass Interfaces versus Object InterfacesSeparation Through DelegationSeparation Through Multiple InheritanceThe ATM User Interface ExampleConclusionBibliographyChapter 13: Overview of UML for C# ProgrammersClass DiagramsObject DiagramsSequence DiagramsCollaboration DiagramsState DiagramsConclusionBibliographyChapter 14: Working with DiagramsWhy Model?Why Build Models of Software?Should We Build Comprehensive Designs Before Coding?Making Effective Use of UMLCommunicating with OthersRoadMapsBack-End DocumentationWhat to Keep and What to Throw AwayIterative RefinementBehavior FirstCheck the StructureEnvisioning the CodeEvolution of DiagramsWhen and How to Draw DiagramsWhen to Draw Diagrams and When to StopCASE ToolsBut What About 2192194194196198199200200201202202
xContentsChapter 15: State Diagrams203The BasicsSpecial EventsSuperstatesInitial and Final PseudostatesUsing FSM DiagramsConclusion204205206207208209Chapter 16: Object Diagrams211A Snapshot in TimeActive ObjectsConclusion212213217Chapter 17: Use Cases219Writing Use CasesAltemate CoursesWhatElse?Diagramming Use CasesConclusionBibliographyChapter 18: Sequence DiagramsThe BasicsObjects, Lifelines, Messages, and Other Odds and EndsCreation and DestructionSimple LoopsCases and ScenariosAdvanced ConceptsLoops and ConditionsMessages That Take TimeAsynchronous MessagesMultiple ThreadsActive ObjectsSending Messages to 228228232232233235239240240241
ContentsxiChapter 19: Class Diagrams243The BasicsClassesAssociationInheritanceAn Example Class DiagramThe DetailsClass StereotypesAbstract yAssociation StereotypesNested ClassesAssociation ClassesAssociation 249250251252253254255256256257258258Chapter 20: Heuristics and CoffeeThe Mark IV Special Coffee MakerSpecificationA Common but Hideous SolutionImaginary AbstractionAn Improved SolutionImplementing the Abstract ModelThe Benefits of This 7279292
xiiContentsSection III: The Payroll Case Study293Rudimentary Specification of the Payroll SystemExerciseUse Case 1: Add New EmployeeUse Case 2: Deleting an EmployeeUse Case 3: Post a Time CardUse Case 4: Posting a saies ReceiptUse Case 5: Posting a Union Service ChargeUse Case 6: Changing Employee DetailsUse Case 7: Run the Payroll for Today294295295295296296296296297Chapter 21: Command and Active Object: Versatilityand MultitaskingSimple CommandsTransactionsPhysical and Temporal DecouplingTemporal DecouplingUndo MethodActive 10310Chapter 22: Template Method and Strategy: Inheritanceversus Delegation311Template MethodPattern AbuseBubble SortStrategyConclusionBibliographyChapter 23: Facade and 316319324324325325327329329
ContentsChapter 24: Singleton and Monostatexiii331SingletonBenefitsCostsSingleton in ActionMonostateBenefitsCostsMonostate in 38343343Chapter 25: Null Object345DescriptionConclusionBibliographyChapter 26: The Payroll Case Study: Iteration 1Rudimentary SpeciflcationAnalysis by Use CasesAdding EmployeesDeleting EmployeesPosting Time CardsPosting Sales ReceiptsPosting a Union Service ChargeChanging Employee DetailsPaydayReflection: Finding the Underlying AbstractionsEmployee PaymentPayment SchedulePayment 3
xivContentsChapter 27: The PayroU Case Study: ImplementationTransactionsAdding EmployeesDeleting EmployeesTime Cards, Sales Receipts, and Service ChargesChanging EmployeesWhat Was I Smoking?Paying EmployeesPaying Salaried EmployeesPaying Hourly EmployeesMain ProgramTheDatabaseConclusionAbout This 08409411411412Section IV: Packaging the PayroU System413Chapter 28: Principles of Packageand Component Design415Packages and ComponentsPrinciples of Component Cohesion: GranularityThe Reuse/Release Equivalence Principle (REP)The Common Reuse Principle (CRP)The Common Closure Principle (CCP)Summary of Component CohesionPrinciples of Component Coupling: StabilityThe Acyclic Dependencies Principle (ADP)The Stable-Dependencies Principle (SDP)The Stable-Abstractions Principle (SAP)ConclusionChapter 29: FactoryA Dependency ProblemStatic versus Dynamic Typing416417417418419420420420426431435437440441
ContentsSubstitutable FactoriesUsing Factories for Test FixturesImportance of apter 30: The Payroll Case Study: Package Analysis .447Component Structure and NotationApplying the Common Closure Principle (CCP)Applying the Reuse/Release Equivalence Principle (REP)Coupling and EncapsulationMetricsApplying the Metrics to the Payroll ApplicationObject FactoriesRethinking the Cohesion BoundariesThe Final Packaging StructureConclusionBibliographyChapter 31: CompositeComposite CommandsMultiplicity or No MultiplicityConclusionChapter 32: Observer: Evolving into a PatternThe Digital ClockThe Observer PatternModelsManagement of OOD 460461463465465467469470470471472491491492493494
xviContentsChapter 33: Abstract Server, Adapter, and BridgeAbstract ServerAdapterThe Class Form of AdapterThe Modem Problem, Adapters, and LSPBridgeConclusionBibliographyChapter 34: Proxy and Gateway: ManagingThird-Party APIsProxyImplementing ProxySummaryDatabases, Middleware, and Other Third-Party InterfacesTable Data GatewayTesting and In-Memory TDGsTesting the DB GatewaysUsing Other Patterns with DatabasesConclusionBibliographyChapter 35: 526528535536539541541543544Acyclic VisitorUses of VISITORDecoratorExtension ter 36: State579Nested Switch/Case StatementsThe Internal Scope State VariableTesting the ActionsCosts andBenefits580583583583
ContentsTransition TablesUsing Table InterpretationCosts andBenefitsThe State PatternState versus StrategyCosts andBenefitsThe State Machine Compiler (SMC)Tumstile.cs Generated by SMC, and Other Support FilesClasses of State Machine ApplicationHigh-Level Application Policies for GUIsGUI Interaction ControllersDistributed ProcessingConclusionBibliographyChapter 37: The PayroU Case Study: The DatabaseBuilding the DatabaseAFlaw in the Code DesignAdding an EmployeeTransactionsLoading an EmployeeWhat Remains?Chapter 38: The PayroU User Interface: Model ViewPresenterThe InterfaceImplementationBuilding a WindowThe PayroU WindowThe 7639640650657669670670
xviiiAppendix A: A Satire of Two CompaniesRufus Inc.: Project KickoffRupert Industries: Project AlphaContents671671671Appendix B: What Is Software?687Index699
Agile Principles, Patterns, and Practices in C# Robert C. Martin Micah Martin . Agile Development 1 Chapter 1: Agile Practices 3 The Agile Alliance 4 Individuais and Interactions over Processes and Tools 5 Working Software over Comprehensive Documenta