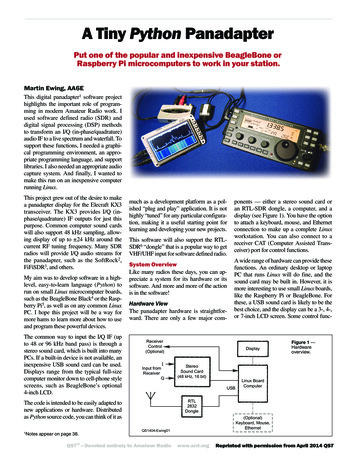
Transcription
A Tiny Python PanadapterPut one of the popular and inexpensive BeagleBone orRaspberry Pi microcomputers to work in your station.Martin Ewing, AA6EThis digital panadapter1 software projecthighlights the important role of programming in modern Amateur Radio work. Iused software defined radio (SDR) anddigital signal processing (DSP) methodsto transform an I/Q (in-phase/quadrature)audio IF to a live spectrum and waterfall. Tosupport these functions, I needed a graphical programming environment, an appropriate programming language, and supportlibraries. I also needed an appropriate audiocapture system. And finally, I wanted tomake this run on an inexpensive computerrunning Linux.This project grew out of the desire to makea panadapter display for the Elecraft KX3transceiver. The KX3 provides I/Q (inphase/quadrature) IF outputs for just thispurpose. Common computer sound cardswill also support 48 kHz sampling, allowing display of up to 24 kHz around thecurrent RF tuning frequency. Many SDRradios will provide I/Q audio streams forthe panadapter, such as the SoftRock2, FiFiSDR3, and others.My aim was to develop software in a highlevel, easy-to-learn language (Python) torun on small Linux microcomputer boards,such as the BeagleBone Black4 or the Raspberry Pi5, as well as on any common LinuxPC. I hope this project will be a way formore hams to learn more about how to useand program these powerful devices.The common way to input the I/Q IF (upto 48 or 96 kHz band pass) is through astereo sound card, which is built into manyPCs. If a built-in device is not available, aninexpensive USB sound card can be used.Displays range from the typical full-sizecomputer monitor down to cell-phone stylescreens, such as BeagleBone’s optional4-inch LCD.much as a development platform as a polished “plug and play” application. It is nothighly “tuned” for any particular configuration, making it a useful starting point forlearning and developing your new projects.This software will also support the RTLSDR6 “dongle” that is a popular way to getVHF/UHF input for software defined radio.System OverviewLike many radios these days, you can appreciate a system for its hardware or itssoftware. And more and more of the actionis in the software!Hardware ViewThe panadapter hardware is straightforward. There are only a few major com-appear on page 38.A wide range of hardware can provide thesefunctions. An ordinary desktop or laptopPC that runs Linux will do fine, and thesound card may be built in. However, it ismore interesting to use small Linux boards,like the Raspberry Pi or BeagleBone. Forthese, a USB sound card is likely to be thebest choice, and the display can be a 3-, 4-,or 7-inch LCD screen. Some control func-ReceiverControl(Optional)IInput fromReceiverQDisplayStereoSound Card(48 kHz, 16 bit)USBFigure 1 —Hardwareoverview.Linux BoardComputerRTL2832DongleThe code is intended to be easily adapted tonew applications or hardware. Distributedas Python source code, you can think of it as1Notesponents — either a stereo sound card oran RTL-SDR dongle, a computer, and adisplay (see Figure 1). You have the optionto attach a keyboard, mouse, and Ethernetconnection to make up a complete Linuxworkstation. You can also connect to areceiver CAT (Computer Assisted Transceiver) port for control functions.(Optional)Keyboard, Mouse,EthernetQS1404-Ewing01QST – Devoted entirely to Amateur Radiowww.arrl.orgReprinted with permission from April 2014 QST
Alternatively, we can get data from anRTL-SDR VHF/UHF dongle device. Thisdongle can sample at high rates (up to 2.048MHz), beyond the real-time capability ofsmaller systems. For this reason, the RTLmode uses blocking I/O and accepts someloss of data.InitializeGet I, QSamplesData in hand, we compute the log powerspectrum7 of each buffer in the chunk andaccumulate it into the chunk’s average logpower spectrum — the thing we will display. A power vs. frequency (“2D”) graphis always plotted along with a calibratedoscilloscope-like “graticule.” Optionally,a waterfall display (power vs frequency vstime, or “3D” plot) is also produced.ComputeLog PowerSpectrumDrawGraphAdd oardCommand?ProcessCommandIf requested, an overlay of diagnostic information and user help is drawn on top ofthe data displays in a way that works wellon small display devices. Several pages ofhelp are available that guide the user to setparameters using four pushbuttons. (I haveoptimized the process for the BeagleBone’s4-inch LCD4 display card, but it shouldwork on a range of processors and displays.)If there is a keyboard event (or pushbuttonpress), it is interpreted as a command tochange operating or display parameters.UpdateScreenQS1404-Ewing02Figure 2 — Software flow chart.tions are necessary, but we can implementthem with dedicated buttons without akeyboard or mouse. Some screens support atouch interface, but I have not implementedtouch in the software.The main challenge of this project is thesoftware, especially when we are aimingfor fast audio and graphics features runningin as small a processor as possible.Software ViewFigure 2 shows a simplified block diagramof the top level code of the panadapter,called iq.py.After initialization, the program gets achunk of I and Q IF (audio) data. Typically, this comes from PortAudio calls (andcall-back routines) that accept data from anALSA audio device, such as a USB soundcard. PortAudio uses non-blocking I/O sothat no data is lost during processing anddisplay, as long as there is adequate processor power.Finally, the newly constructed graphic istransmitted to the display, and the loopcontinues.Software LibrariesThe software overview in Figure 3 showsthe major code blocks and how they layeron each other and add functionality. Theseare the resources we will use in the iq.pyprogram.The bottom layer is the Linux operatingsystem, which supports the audio, graphics, numerics, and control functions. Eachcomponent may be built on simpler functions that result in the desired capabilities.For example, for the audio functions we usePyAudio (people.csail.mit.edu/hubert/pyaudio/), which is a Python form of theC/C Portaudio library (www.portaudio.com), which in turn is based onALSA, the Advanced Linux SoundArchitecture.Graphics is an important but computerintensive panadapter function. I chose thePyGame library (www.PyGame.org),which is built on SDL (Simple DirectMediaLibrary). SDL in turn calls on Xorg or directfb for the most basic screen operations.Reprinted with permission from April 2014 QSTQS1404-Ewing03Panadapter Application, g/directfbNumericsRx ControlNumpyHamlibLinuxFigure 3 — Software libraries overview.PyGame is a relatively simple graphicssystem that is easy to program and reasonably efficient.The numerical parts of my code are facilitated by the Numpy library (Numpy.org).This is a large system for scientific andengineering mathematics. I only need to useNumpy’s Fast Fourier Transforms (FFTs)and some other efficient array operations.Finally, the Hamlib (www.Hamlib.org) library provides my program with the abilityto address and control many different typesof amateur radios.Building the Operating SystemIn this section, we’ll see what needs to bedone to bring your brand new computerboard into a state that is ready for our Python application.This project is meant to run on various hardware platforms, but especially on the Raspberry Pi (Pi) and the BeagleBone Black(BBB). Other small boards, like the olderBeagleBone and BeagleBoard, should beokay, and the code should run even betteron modern Linux PCs.While all these small platforms are Linuxbased, the main software components —PyAudio, PyGame, Numpy, Hamlib, andthe Python language — are all available forWindows and MacOS. Porting this projectto Windows or Macintosh would be a finething to do, but we are focusing on theLinux OS.If you’re considering getting a new computer for this project, I would recommendthe BeagleBone Black or a card of equivalent power. The Raspberry Pi (512 MBModel B) is usable, but it requires more careto select operating modes that do not exceedits CPU capacity. On the other hand, the Pi’sRaspbian operating system can be easier toprepare for the panadapter project, and thecommunity of Pi users is very large.ARRL, the national association for Amateur Radio www.arrl.org
can follow the recipe for theBeagleBone, beginning with installation of python-pygame, etc. Ifyou are using a distribution otherthan Debian (like Fedora), yourpackages may have differentnames, and you may need to installthem with a different command.I will now describe how to makeyour Pi or BBB ready for our application. Because the two boards’suppliers configure their systemsdifferently, some preparations arespecific to each platform, but othersare common to both.Raspberry Pi: First StepsThe Pi download website (www.raspberrypi.org/downloads) recommends that first-time Pi usersinstall the “New Out of Box Software.” If you follow this procedure,you will be given a choice of operating systems. Select the Raspbianoption, and then sit back for acomplete Raspbian download.Installing and Runningthe ProgramTable 1 summarizes the suggestedhardware to support the panadapterapplication.A Raspberry Pi Model B microcomputer.If you’re familiar with the Linux/Debian way of doing things, youcan get more control and possibly savesome time by installing Raspbian directlyfrom the download page.The first thing is to set up the Pi’s operatingsystem for the panadapter project. Many ofthese operations are useful for anything youmay want to do with your Pi. Detailed stepby-step procedures are in the supplementary files in www.arrl.org/qst-in-depth. Insummary: Download Raspbian onto an SD cardusing a PC. An 8 GB class 4 card or aboveis suggested. Place the card in your Pi, attach keyboard, monitor, Ethernet, and 5Vpower. Wait for boot-up. Set up a password and other options asdesired in the raspi-config process. Set uplocalization for time zone, keyboard, etc. Reboot and go to “Continuing with yourPi or BBB” below.BeagleBone Black: First StepsThe BeagleBone Black (BBB) requiresa bit more work than the Pi to configure forour project, but in the end we will have asystem that is configured much like the Piabove. I need to bring up a Debian 7.0.0“Wheezy” (or later) Linux system on theBBB. The major steps are outlined here,while details are given the supplementaryfiles mentioned above. Follow instructions at eLinux.org/BeagleBoardDebian, building a Debiansystem on an 8 or 16 GB microSD cardusing a convenient PC. Install the microSD card on the BBB.Attach an HDMI monitor, keyboard, andmouse. Attach an Ethernet connection toyour local router. Install the LXDE desktop package fromthe network.Continuing with Your Pi or BBBMost of the following is common to bothboards. Again, more detailed steps are provided in the supplementary files. Install the packages python-pygame,python-hamlib2, python-dev, portaudio19-dev, and python-numpy from yourrepository. Obtain and install the latest pyAudiopackage. If desired, obtain, build, and install rtl-sdrand pyrtlsdr for RTL-SDR support. Install optional components, set up useraccounts, etc.Linux PCIf you have an up-to-date Linux PC, youRecent Raspberry Pis are likelyto be the Model B with 512 MBRAM. You may be able to usethe 256 MB model, but that hasnot been tested. The Pi offers anNTSC video output, but it will notprovide the needed display resolution. Usethe HDMI port if possible.The BeagleBone Black with the LCD4cape (a 4-inch LCD with five buttonsthat stack on the BBB) is a good targetplatform for a compact panadapter. I explored using the BeagleBone audio cape,but at this w riting the audio cape is notfully compatible with the BBB and LCD4.Improved audio support may eventually letus have a self-contained BBB cape system.Meanwhile, you must use an external USBsound card.If you are connecting to a receiver thatallows remote control (CAT), you maywant to use the Hamlib support, which willrequire a USB radio connection or a USBserial port adapter. If you are only using theRTL-SDR USB dongle receiver, you won’tneed a USB sound card.Table 1 — Hardware ConfigurationsDevice (Approx pricing)RequiredDesirableRaspberry Pi ( 35)Raspberry Pi (Model B)4 GB SD cardPowered USB hubUSB sound cardHDMI monitorKeyboard and mouse512 MB RAM8 GB SD cardUSB-serial port (rig control)RTL-SDR dongleBeagleBone Black ( 45)4 GB microSD cardPowered USB HubUSB sound cardLCD4 display or HDMI displayKeyboard and mouse8 GB microSD cardUSB-serial port (rig control)RTL-SDR donglePersonal ComputerRecent Linux OSBuilt-in stereo input soundcard or USB sound cardTraditional serial port orUSB-serial port (rig control)RTL-SDR dongleQST – Devoted entirely to Amateur Radiowww.arrl.orgReprinted with permission from April 2014 QST
Some points to watch out forwith any of the small Linuxboards: Be sure that your 5 V supplyhas adequate current capacityand your USB cable is good. Especially when driving externalUSB devices that take power,you can easily get unreliable operation if the voltage droops toomuch. A 2 A supply or greater isa good bet. Use a powered USB hub if youhave multiple USB devices. Thisminimizes the power drain fromthe CPU board. When it comes to poweringdown, it is preferable to use theshutdown -h now command (or A BeagleBone Black microcomputer.equivalent GUI option) and thenwait for activity to stop rather than simply The sound cards we have looked at are sumswitching off the power. If you don’t do this marized in Table 2. There are many others(and many of us don’t), there is some risk of on the market, so this is just a sampling.data corruption.It is difficult to compare published specs onSound Cardssound cards, especially the cheaper ones,Most USB sound cards have a standard because usually there are no publishedinterface that works with the ALSA drivers specs! QST reviewed sound cards in 2007.9found in typical Linux installations. Board- Those products are probably unavailablelevel plug-ins (with PCI or PCI Express now, and there were no USB sound cardsconnections) or built-in devices are also considered. Nevertheless, the article is stillsupported, and appear the same to higher- a useful review of the complications oflevel software. It is rare that special drivers evaluating sound cards. The test proceduresare needed, unless you have a complex evaluated cards as “audio” devices as ifsound card that includes special processing for human listening, using a logarithmicfrequency scale. For SDR, we want a flatand mixing options.response nearly up to the Nyquist frequencyFor this project, we have evaluated a num- (1 2 the sampling rate) and we must thinkber of stereo sound cards at different price in terms of linear frequency scales, wherelevels. The good news is that all of them the last kHz of response is as important asseem to work. The bad news is that you the first.get what you pay for. The less expensiveunits will not go above 48 kHz sampling I do not have precise measurements forand may offer poorer frequency response, sound cards, although there is some relless sensitivity, or more noise. Some offer evant data on the Internet.10 The less expenphono or microphone preamplification, and sive options are certainly usable for casualwork as a panadapter, but you can expectsome do not.high-end sound cards to performbetter for software defined radiowhere the best image rejectionand dynamic range is desired.The iConnex and UCA202 bothappear to use the TI/Burr-BrownPCM2902 chip, which has aninteresting quirk. In at leastsome versions, the left channelis delayed by exactly one samplerelative the right channel. I haveprovided the “—LAGFIX” optionin iq.py to correct for this “feature” (bug!), which would otherwise make these sound cardsunusable for SDR work.To determine the suitable operating levels for your sound card,in general, you’ll want to adjustreceiver gain (or sound card inputgain) to a level just enough that you get reasonable “counts” on receiver noise, but nomore. This will allow maximum dynamicrange (headroom) for receiving. In practice,if your typical noise level sample valuespeak at 10 counts (out of full scale 32,767 in a 16 bit converter) that shouldbe enough. (Be sure your noise is comingfrom your antenna, not your electronics!Verify that the noise goes down when youdisconnect the antenna.)If you need more gain, you may be temptedto use the sound card’s preamplifier, ifit has one. This may or may not help.If you have a “phono” preamp, it probably applies RIAA equalization to matcha p honograph’s frequency response. Thatwill attenuate high frequencies severely (by 20 dB at 20 kHz). A “mic” preamp shouldnot have this problem. Indeed, the iMic’smic preamp seems to improve the overallfrequency response of the iMic.Depending on your receiver and its grounding arrangements, it may be helpful to useaudio isolation transformers for the I andTable 2 — Some Inexpensive USB Sound Cards with Stereo InputsUnitBrief SpecsApprox PricingCommentsiKey-Audio iConnex48 kHz, phonopreamp 40PCM290x based (may require lagcorrection)Behringer UCA20248 kHz, no preamp 30Similar to iConnex without preampGriffin iMic48 kHz, mic preamp 29Spurious peaks at 5 kHz andmultiples on BBB. 114 dB SNRclaimedReprinted with permission from April 2014 QSTARRL, the national association for Amateur Radio www.arrl.org
Q signals between the radio and the soundcard. A convenient commercial unit is the“Cables to Go 40000 3.5mm Extension Stereo Audio Isolation Transformer,” whichcan be found by Internet search. Alternatively, you can wire your own if you have a1:1 audio transformer.The RTL-SDR DongleAs a byproduct of the DVB-T (digital videobroadcast — terrestrial) service in use inmany countries (but not in North America),an inexpensive USB “dongle” receiver hasbecome available for Amateur Radio applications in the VHF-UHF range. For ourpurposes, it consists of a tunable front-endand an 8-bit I/Q sampler, capable of runningat over 2 MHz sampling rate. Because it isbased on the Realtek RTL2832 quadraturedecoder chip, it is often known as the “RTLSDR” receiver. These devices are availableon eBay (www.ebay.com) for 10 and up.To our Linux boards, the RTL-SDR lookslike a USB device. It has Linux supportfor SDR, so it was a natural addition tothe panadapter project. While our softwarewill display up to a 2 MHz spectrum slicewith the RTL-SDR, we probably do nothave the CPU resources to keep up with acontinuous 2 MHz sample rate — at leastnot without extra programming. I manageby taking data in bursts only when the computer is ready. Discarding some incomingdata reduces sensitivity, but we still can geta useful spectrum display.I should note that the RTL-SDR is a verysimple receiver, with large instantaneousbandwidth and limited sensitivity. It iseasily overloaded by strong out-of-bandsignals. Serious amateur work may requirea good antenna, a preamplifier, and a bandpass filter.Downloading and Installingthe ProjectAt this point I assume you have set upyour Raspberry Pi, BeagleBone Black, orother small Linux board according to thevendor’s directions and the hints we havegiven above. You will have a Linux environment that includes Python and necessarypackages. The job now is to download andinstall the panadapter Python files.All the files for the project can be downloaded as a compressed zip or tar archiveat www.arrl.org/qst-in-depth or at www.aa6e.net. Download this file to a convenient directory and then use the commandunzip iq.zip or tar xzf iq.tar.gz, which will expand the archive into anew “iq” directory.Setting Up a Sound CardThe iq.py code needs to know which PyAudio index number to use for audio input. Ifyou have only one audio device, things aresimple. You can use the default setting (–1).If this doesn’t work, or if you have multipleaudio devices, you may need to do somemore work.In-phase andQuadrature IFsIn-phase and quadrature (I/Q)sampling refers to the technique ofmixing (multiplying) an RF or IF signalwith a local oscillator that is providedin 0 degree and 90 degree phaseshift versions. This produces two IFstreams, which may be treated asreal and imaginary parts of a complexsignal. With I/Q IFs, you can distinguish positive and negative sidebandsrelative to the local oscillator. It is thesame principle that is used in the“phasing method” for single sidebandgeneration and detection. The IFs aresampled and converted to digital formwith an analog to digital converter. Formore information, consult “DSP andSoftware Radio Design,” Chapter 15in recent editions of The ARRL Handbook for Radio lyQLocalOscillatorAn RTL-SDR dongle receiver.QS1404-EwingAFigure A — In-phase and quadrature IFs.QST – Devoted entirely to Amateur Radiowww.arrl.orgDEVICE: 0; NAME: ‘bcm2835 ALSA:bcm2835 ALSA (hw:0,0)’defaultSampleRate : 44100.0maxInputChannels : 0maxOutputChannels : 2DEVICE: 1; NAME: ‘USB Sound BlasterHD: USB Audio (hw:1,0)’defaultSampleRate : 48000.0maxInputChannels : 2maxOutputChannels : 2DEVICE: 2; NAME: ‘USB Sound BlasterHD: USB Audio #1 (hw:1,1)’defaultSampleRate : 48000.0maxInputChannels : 2maxOutputChannels : 2DEVICE: 3; NAME: ‘USB Sound BlasterHD: USB Audio #2 (hw:1,2)’defaultSampleRate : 44100.0maxInputChannels : 0maxOutputChannels : 2PyAudio’s device 0 is the Pi’s on-boardbcm2835 output-only “sound card.” Devices 1 – 3 correspond to the three logicaldevices internal to the SB1240. Becausethis card’s internal addressing is not documented, we use trial and error to find thatPyAudio device (index) #2 gives us theSB1240 input channels we need for thepanadapter.I can get similar information by using theLinux and ALSA command line. The virtual directory /proc/asound/ describes thecurrent ALSA status. For example, you cantype cat /proc/asound/cards to geta listing of all attached sound cards. Otheruseful commands include alsamixer,amixer, arecord, and aplay. Checktheir pages for details.When you use the RTL-SDR dongle forinput, it will not show up as a sound card.No index setting is required, because we donot use PyAudio. The code finds the RTLdevice automatically.0 90 I provide the special program pa.py so thatyou can see the sound devices that PyAudiosees. Type python pa.py in a terminalwindow, and examine the output. The following is a partial listing of pa.py’s outputfor a Raspberry Pi with a Sound BlasterSB1240 USB sound card:Setting Up an RTL-SDR DongleUsing the RTL-SDR dongle is simple. Youattach an antenna, and you plug in yourReprinted with permission from April 2014 QST
experimental work, we mightprefer to run on a modern LinuxPC, which will usually havemore computing and graphicspower than the small cards offer.Squeezing the application into asmall device is fun, but it doeslimit the functionality.dongle to your USB hub. Theinitial operating frequency andsample rate are set from thecommand line as shown below.Setting Up a BeagleBoneBlack and LCD4The LCD4 is one several LCDcapes that can stack on theBBB. You need to plug it in tothe CPU board, but be sure touse the right orientation: If theBBB Ethernet jack is at the left,the LCD4 pushbuttons shouldbe along the right side.My thanks to Leigh Klotz Jr,WA5ZNU, for helpful discussions during the development ofthis article.With the LCD4 installed, theBBB should boot up to anLXDE desktop on the LCD.The HDMI video output is Figure 4 — Screen shot of iq.py with help overlay.disabled automatically. Others i z e s o f L C D c a p e ( eg ,3 inch or 7 inch) can probably be used, but On the Raspberry Pi, for example, we mightthe SCREEN SIZE value in iq.py should be use:adjusted appropriately.python iq.py --index 1 --bufThe buttons on the LCD4 are labeled left, fers 15 --taking 4 --size 256.right, up, down, and enter. Pressing oneThe index setting is okay for a typical inputof these buttons is equivalent to pressingsound card.the corresponding arrow keys (or enter)on a standard keyboard. Each press of the A typical command to start receiving withENTER button produces a new help overlay an RTL-SDR dongle would be:on the LCD4. An example is shown inpython iq.py --RTL --rate Figure 4.2048000 --rtl freq 144200000.Command Line OptionsThis would set up a 2.048 MHz sample rateBecause it is designed for operating on difcentered at 144.2 MHz.ferent platforms for different kinds of operating, the iq.py code has many user-settable A complete table of options is provided inparameters and modes. You can change the supplementary files.most of them from the Linux commandConclusionline. Once you find a combination you wantTheTiny Python Panadapter is usable softto use repetitively, you can put it into a shellwarethat runs on very inexpensive hardscript (a file) or define a shell alias that hasware.It is implemented in Python, which iseverything set for you. Or, you can editaverycapable programming language thatthe source code iq opt.py and change theisrelativelyeasy to work with, althoughdefault values.lower-level approaches (like C or C )The code is normally started from the Linux could offer greater performance at the costcommand line, using the python command. of more programming effort. The PythonI assume you have started a terminal win- approach, supported by PyGame, PyAudio,dow and changed directory (cd) to the di- etc. is a good choice if we want the code torectory where you placed iq.py. If you feel be accessible to as many experimenters andlucky, you can try the command: python learners as possible.iq.py.There are many directions this project couldThis will use all the default values. If you take. It could readily support recordinghave a complicated sound card or multiple and playback of the I/Q IF signal. It couldsound cards, you will have to use pa.py (or support full SDR transceiver operation, protrial and error) to find the appropriate index viding demodulated audio on receive, andvalue, as discussed above.generating I/Q IF signals on transmit. ForReprinted with permission from April 2014 QSTNotespanadapter is a spectrum analyzerthat displays of power vs frequency,generally showing the IF passbandsurrounding an amateur’s currentoperating frequency.2SoftRock is a series of kit-form receivers and transceivers developedby Tony Parks, KB9YIG. They areavailable from fivedash.com. Thereis an active “softrock40” Yahoo!group for user support.3FiFiSDR is a kit receiver described at o28.sischa.net/fifisdr/trac and www.df3dcb.de/FiFi-SDR FA1110.pdf (in German). A partiallyassembled version is sold by FunkAmateurmagazine at www.box73.de/product info.php?products id 2425. The receiver was reviewed in the September 2013 issue of QST.4The BeagleBone Black is developed by the BeagleBoard.org Foundation. See beagleboard.org.5The Raspberry Pi is developed by the RaspberryPi Foundation (UK). See raspberrypi.org.6See Robert Nickels W9RAN, “Cheap and EasySDR,” QST, January 2013, p 30, sdr.osmocom.org/trac/wiki/rtl-sdr.7The Fourier transform (spectrum) provides a complex voltage-like value in each frequency channel. The power spectrum, the power in eachchannel, is the square of voltage (really the complex amplitude squared). Finally, the log powerspectrum is the logarithm (usually given in dB) ofpower in each channel.8Jonathan Taylor, K1RFD, “Computer Sound Cardsfor Amateur Radio,” QST, May, 2007, p 63.9For example, data from Larry Phipps, N8LP, www.telepostinc.com/sound cards.html.10Current peak values for I and Q samples are displayed on the overlay panels of the iq.py program.1AMartin Ewing, AA6E, was first licensed in1957, when Sputnik went up and SSB was stillnew. After studying physics and astronomy, hehad careers in radio astronomy and academiccomputing until retiring in 2002. He currentlyserves as an ARRL Technical Advisor and asa volunteer in the ARRL Laboratory, with particular interests in Linux software and softwaredefined radio. You can reach Martin at 28 WoodRd, Branford, CT 06405, or via e-mail at aa6e@arrl.net.For updates to this article,see the QST Feedback page atwww.arrl.org/feedback.ARRL, the national association for Amateur Radio www.arrl.org
level, easy-to-learn language (Python) to run on small Linux microcomputer boards, such as the BeagleBone Black 4 or the Rasp - berry Pi5, as well as on any common Linux PC. I hope this project will be a way for more hams to learn more about how to use and program these powerful devices