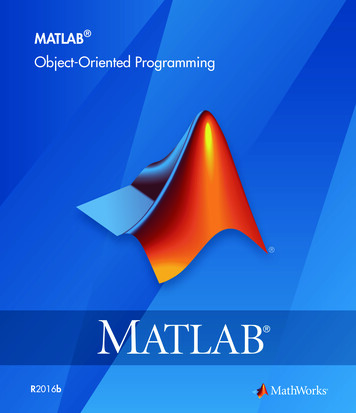
Transcription
MATLAB Object-Oriented ProgrammingR2016b
How to Contact MathWorksLatest news:www.mathworks.comSales and services:www.mathworks.com/sales and servicesUser community:www.mathworks.com/matlabcentralTechnical support:www.mathworks.com/support/contact usPhone:508-647-7000The MathWorks, Inc.3 Apple Hill DriveNatick, MA 01760-2098Object-Oriented Programming COPYRIGHT 1984–2016 by The MathWorks, Inc.The software described in this document is furnished under a license agreement. The software may be usedor copied only under the terms of the license agreement. No part of this manual may be photocopied orreproduced in any form without prior written consent from The MathWorks, Inc.FEDERAL ACQUISITION: This provision applies to all acquisitions of the Program and Documentationby, for, or through the federal government of the United States. By accepting delivery of the Programor Documentation, the government hereby agrees that this software or documentation qualifies ascommercial computer software or commercial computer software documentation as such terms are usedor defined in FAR 12.212, DFARS Part 227.72, and DFARS 252.227-7014. Accordingly, the terms andconditions of this Agreement and only those rights specified in this Agreement, shall pertain to andgovern the use, modification, reproduction, release, performance, display, and disclosure of the Programand Documentation by the federal government (or other entity acquiring for or through the federalgovernment) and shall supersede any conflicting contractual terms or conditions. If this License failsto meet the government's needs or is inconsistent in any respect with federal procurement law, thegovernment agrees to return the Program and Documentation, unused, to The MathWorks, Inc.TrademarksMATLAB and Simulink are registered trademarks of The MathWorks, Inc. Seewww.mathworks.com/trademarks for a list of additional trademarks. Other product or brandnames may be trademarks or registered trademarks of their respective holders.PatentsMathWorks products are protected by one or more U.S. patents. Please seewww.mathworks.com/patents for more information.
Revision HistoryMarch 2008October 2008March 2009September 2009March 2010September 2010April 2011September 2011March 2012September 2012March 2013September 2013March 2014October 2014March 2015September 2015March 2016September 2016Online onlyOnline onlyOnline onlyOnline onlyOnline onlyOnline onlyOnline onlyOnline onlyOnline onlyOnline onlyOnline onlyOnline onlyOnline onlyOnline onlyOnline onlyOnline onlyOnline onlyOnline onlyNew for MATLAB 7.6 (Release 2008a)Revised for MATLAB 7.7 (Release 2008b)Revised for MATLAB 7.8 (Release 2009a)Revised for MATLAB 7.9 (Release 2009b)Revised for MATLAB 7.10 (Release 2010a)Revised for Version 7.11 (Release 2010b)Revised for Version 7.12 (Release 2011a)Revised for Version 7.13 (Release 2011b)Revised for Version 7.14 (Release 2012a)Revised for Version 8.0 (Release 2012b)Revised for Version 8.1 (Release 2013a)Revised for Version 8.2 (Release 2013b)Revised for Version 8.3 (Release 2014a)Revised for Version 8.4 (Release 2014b)Revised for Version 8.5 (Release 2015a)Revised for Version 8.6 (Release 2015b)Revised for Version 9.0 (Release 2016a)Revised for Version 9.1 (Release 2016b)
Contents12Using Object-Oriented Design in MATLABWhy Use Object-Oriented Design . . . . . . . . . . . . . . . . . . . . . .Approaches to Writing MATLAB Programs . . . . . . . . . . . . . .When Should You Create Object-Oriented Programs . . . . . . .1-21-21-6Using Handles . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .What Is a Handle? . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Copies of Handles . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Handle Objects Modified in Functions . . . . . . . . . . . . . . . . .Determine If an Object Is a Handle . . . . . . . . . . . . . . . . . .Deleted Handle Objects . . . . . . . . . . . . . . . . . . . . . . . . . . . .1-121-121-121-131-151-16Basic ExampleCreate a Simple Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Design Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Create Object . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Access Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Call Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Add Constructor . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Vectorize Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Overload Functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .BasicClass Code Listing . . . . . . . . . . . . . . . . . . . . . . . . . . .2-22-22-32-32-42-42-52-62-6v
3viContentsMATLAB Classes OverviewRole of Classes in MATLAB . . . . . . . . . . . . . . . . . . . . . . . . . . .Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Some Basic Relationships . . . . . . . . . . . . . . . . . . . . . . . . . . .3-23-23-4Developing Classes — Typical Workflow . . . . . . . . . . . . . . . .Formulating a Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Specifying Class Components . . . . . . . . . . . . . . . . . . . . . . . .BankAccount Class Implementation . . . . . . . . . . . . . . . . .Formulating the AccountManager Class . . . . . . . . . . . . . .Implementing the AccountManager Class . . . . . . . . . . . . .AccountManager Class Synopsis . . . . . . . . . . . . . . . . . . . .Using BankAccount Objects . . . . . . . . . . . . . . . . . . . . . . . .3-83-83-93-103-143-153-153-16Class to Manage Writable Files . . . . . . . . . . . . . . . . . . . . . . .Flexible Workflow . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Performing a Task with an Object . . . . . . . . . . . . . . . . . . . .Defining the Filewriter Class . . . . . . . . . . . . . . . . . . . . . . .Using Filewriter Objects . . . . . . . . . . . . . . . . . . . . . . . . . . .3-193-193-193-193-21Class to Represent Structured Data . . . . . . . . . . . . . . . . . . .Objects as Data Structures . . . . . . . . . . . . . . . . . . . . . . . . .Structure of the Data . . . . . . . . . . . . . . . . . . . . . . . . . . . . .The TensileData Class . . . . . . . . . . . . . . . . . . . . . . . . . . . .Create an Instance and Assign Data . . . . . . . . . . . . . . . . . .Restrict Properties to Specific Values . . . . . . . . . . . . . . . . .Simplifying the Interface with a Constructor . . . . . . . . . . . .Calculate Data on Demand . . . . . . . . . . . . . . . . . . . . . . . . .Displaying TensileData Objects . . . . . . . . . . . . . . . . . . . .Method to Plot Stress vs. Strain . . . . . . . . . . . . . . . . . . . . .TensileData Class Synopsis . . . . . . . . . . . . . . . . . . . . . . .3-243-243-243-253-253-263-273-283-293-303-31Class to Implement Linked Lists . . . . . . . . . . . . . . . . . . . . . .Class Definition Code . . . . . . . . . . . . . . . . . . . . . . . . . . . . .dlnode Class Design . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Create Doubly Linked List . . . . . . . . . . . . . . . . . . . . . . . . .Why a Handle Class for Linked Lists? . . . . . . . . . . . . . . . .dlnode Class Synopsis . . . . . . . . . . . . . . . . . . . . . . . . . . . .Specialize the dlnode Class . . . . . . . . . . . . . . . . . . . . . . . .3-363-363-363-373-383-393-50
Class for Graphing Functions . . . . . . . . . . . . . . . . . . . . . . . .Class Definition Block . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Using the topo Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Behavior of the Handle Class . . . . . . . . . . . . . . . . . . . . . . .4Static DataStatic Data . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .What Is Static Data . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Static Variable . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Static Data Object . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Constant Data . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .53-533-533-543-554-24-24-24-34-5Class Definition—Syntax ReferenceClass Files and Folders . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Class Definition Files . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Options for Class Folders . . . . . . . . . . . . . . . . . . . . . . . . . . .Options for Class Files . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Group Classes with Package Folders . . . . . . . . . . . . . . . . . . .5-25-25-25-25-3Class Components . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Class Building Blocks . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Class Definition Block . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Properties Block . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Methods Block . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Events Block . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .A Complete Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Enumeration Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Related Information . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .5-55-55-55-65-65-75-75-85-9Classdef Block . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .How to Specify Attributes and Superclasses . . . . . . . . . . . .Class Attribute Syntax . . . . . . . . . . . . . . . . . . . . . . . . . . . .Superclass Syntax . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .5-105-105-105-11vii
Local Functions in Class File . . . . . . . . . . . . . . . . . . . . . . .viiiContents5-11Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .The Properties Block . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Access to Property Values . . . . . . . . . . . . . . . . . . . . . . . . . .5-125-125-13Methods and Functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .The Methods Block . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Method Calling Syntax . . . . . . . . . . . . . . . . . . . . . . . . . . . .Private Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .More Detailed Information On Methods . . . . . . . . . . . . . . .Class-Related Functions . . . . . . . . . . . . . . . . . . . . . . . . . . .How to Overload Functions and Operators . . . . . . . . . . . . .Rules for Defining Methods in Separate Files . . . . . . . . . . .5-155-155-155-175-175-175-185-18Events and Listeners . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Define and Trigger Events . . . . . . . . . . . . . . . . . . . . . . . . .Listen for Events . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .5-205-205-20Attribute Specification . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Attribute Syntax . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Attribute Descriptions . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Attribute Values . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Simpler Syntax for true/false Attributes . . . . . . . . . . . . . . .5-225-225-225-235-23Call Superclass Methods on Subclass Objects . . . . . . . . . . .Superclass Relation to Subclass . . . . . . . . . . . . . . . . . . . . .How to Call Superclass Methods . . . . . . . . . . . . . . . . . . . . .How to Call Superclass Constructor . . . . . . . . . . . . . . . . . .5-255-255-255-26Representative Class Code . . . . . . . . . . . . . . . . . . . . . . . . . . .Class Calculates Area . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Description of Class Definition . . . . . . . . . . . . . . . . . . . . . .5-285-285-30MATLAB Code Analyzer Warnings . . . . . . . . . . . . . . . . . . . .Syntax Warnings and Property Names . . . . . . . . . . . . . . . .Variable/Property Name Conflict Warnings . . . . . . . . . . . . .Exception to Variable/Property Name Rule . . . . . . . . . . . . .5-335-335-335-34Objects In Conditional Statements . . . . . . . . . . . . . . . . . . . .Enable Use of Objects In Conditional Statements . . . . . . . .How MATLAB Evaluates Switch Statements . . . . . . . . . . .How to Define the eq Method . . . . . . . . . . . . . . . . . . . . . . .5-355-355-355-37
Enumerations in Switch Statements . . . . . . . . . . . . . . . . . .5-39Operations on Objects . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Object Operations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Help on Objects . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Functions to Test Objects . . . . . . . . . . . . . . . . . . . . . . . . . .Functions to Query Class Components . . . . . . . . . . . . . . . .5-425-425-435-455-45Use of Editor and Debugger with Classes . . . . . . . . . . . . . .Write Class Code in the Editor . . . . . . . . . . . . . . . . . . . . . .How to Refer to Class Files . . . . . . . . . . . . . . . . . . . . . . . . .How to Debug Class Files . . . . . . . . . . . . . . . . . . . . . . . . . .5-465-465-465-47Automatic Updates for Modified Classes . . . . . . . . . . . . . . .When MATLAB Loads Class Definitions . . . . . . . . . . . . . . .Consequences of Automatic Update . . . . . . . . . . . . . . . . . . .What Happens When Class Definitions Change . . . . . . . . .Actions That Do Not Trigger Updates . . . . . . . . . . . . . . . . .Multiple Updates to Class Definitions . . . . . . . . . . . . . . . . .Object Validity with Deleted Class File . . . . . . . . . . . . . . . .When Updates Are Not Possible . . . . . . . . . . . . . . . . . . . . .Potential Consequences of Class Updates . . . . . . . . . . . . . .Updates to Class Attributes . . . . . . . . . . . . . . . . . . . . . . . .Updates to Property Definitions . . . . . . . . . . . . . . . . . . . . .Updates to Method Definitions . . . . . . . . . . . . . . . . . . . . . .Updates to Event Definitions . . . . . . . . . . . . . . . . . . . . . . -54Compatibility with Previous Versions . . . . . . . . . . . . . . . . .New Class-Definition Syntax Introduced with MATLABSoftware Version 7.6 . . . . . . . . . . . . . . . . . . . . . . . . . . . .Changes to Class Constructors . . . . . . . . . . . . . . . . . . . . . .New Features Introduced with Version 7.6 . . . . . . . . . . . . .Examples of Old and New . . . . . . . . . . . . . . . . . . . . . . . . . .5-565-565-575-575-58Comparison of MATLAB and Other OO Languages . . . . . . .Some Differences from C and Java Code . . . . . . . . . . . . .Object Modification . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Static Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Common Object-Oriented Techniques . . . . . . . . . . . . . . . . .5-595-595-605-645-65ix
6xContentsDefining and Organizing ClassesUser-Defined Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .What Is a Class Definition . . . . . . . . . . . . . . . . . . . . . . . . . .Attributes for Class Members . . . . . . . . . . . . . . . . . . . . . . . .Kinds of Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Constructing Objects . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Class Hierarchies . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .classdef Syntax . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Class Code . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .6-26-26-26-36-36-36-36-4Class Attributes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Specifying Class Attributes . . . . . . . . . . . . . . . . . . . . . . . . . .Specifying Attributes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .6-66-66-7Evaluation of Expressions in Class Definitions . . . . . . . . . . .Why Use Expressions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Where to Use Expressions in Class Definitions . . . . . . . . . . .How MATLAB Evaluates Expressions . . . . . . . . . . . . . . . . .When MATLAB Evaluates Expressions . . . . . . . . . . . . . . . .Expression Evaluation in Handle and Value Classes . . . . . .6-96-96-96-126-126-12Folders Containing Class Definitions . . . . . . . . . . . . . . . . . .Class and Path Folders . . . . . . . . . . . . . . . . . . . . . . . . . . . .Using Path Folders . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Using Class Folders . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Access to Functions Defined in Private Folders . . . . . . . . . .Class Precedence and MATLAB Path . . . . . . . . . . . . . . . . .Changing Path to Update Class Definition . . . . . . . . . . . . .6-166-166-166-176-176-186-19Class Precedence . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Use of Class Precedence . . . . . . . . . . . . . . . . . . . . . . . . . . .Why Mark Classes as Inferior . . . . . . . . . . . . . . . . . . . . . . .InferiorClasses Attribute . . . . . . . . . . . . . . . . . . . . . . . . . . .6-226-226-226-22Packages Create Namespaces . . . . . . . . . . . . . . . . . . . . . . . .Package Folders . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Internal Packages . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Referencing Package Members Within Packages . . . . . . . . .Referencing Package Members from Outside the Package . .Packages and the MATLAB Path . . . . . . . . . . . . . . . . . . . .6-246-246-256-256-266-27
Import Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Syntax for Importing Classes . . . . . . . . . . . . . . . . . . . . . . .Import Package Functions . . . . . . . . . . . . . . . . . . . . . . . . . .Package Function and Class Method Name Conflict . . . . . .Clearing Import List . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .76-296-296-296-306-30Value or Handle Class — Which to UseComparison of Handle and Value Classes . . . . . . . . . . . . . . .Basic Difference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Behavior of MATLAB Built-In Classes . . . . . . . . . . . . . . . . .User-Defined Value Classes . . . . . . . . . . . . . . . . . . . . . . . . .User-Defined Handle Classes . . . . . . . . . . . . . . . . . . . . . . . .Determining Equality of Objects . . . . . . . . . . . . . . . . . . . . . .Functionality Supported by Handle Classes . . . . . . . . . . . . .7-27-27-37-47-57-77-9Which Kind of Class to Use . . . . . . . . . . . . . . . . . . . . . . . . . .Examples of Value and Handle Classes . . . . . . . . . . . . . . . .When to Use Handle Classes . . . . . . . . . . . . . . . . . . . . . . .When to Use Value Classes . . . . . . . . . . . . . . . . . . . . . . . . .7-117-117-117-12The Handle Superclass . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Building on the Handle Class . . . . . . . . . . . . . . . . . . . . . . .Handle Class Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . .Event and Listener Methods . . . . . . . . . . . . . . . . . . . . . . . .Relational Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Test Handle Validity . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .When MATLAB Destroys Objects . . . . . . . . . . . . . . . . . . . .7-137-137-137-147-147-147-14Handle Class Destructor . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Basic Knowledge . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Syntax of Handle Class Destructor Method . . . . . . . . . . . . .Support Destruction of Partially Constructed Objects . . . . .When to Define a Destructor Method . . . . . . . . . . . . . . . . .Destructors in Class Hierarchies . . . . . . . . . . . . . . . . . . . . .Object Lifecycle . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Restrict Access to Object Delete Method . . . . . . . . . . . . . . .Nondestructor Delete Methods . . . . . . . . . . . . . . . . . . . . . .Java Objects Referencing MATLAB Objects . . . . . . . . . . . .7-167-167-167-177-187-197-207-217-227-22xi
8xiiContentsFind Handle Objects and Properties . . . . . . . . . . . . . . . . . . .Find Handle Objects . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Find Handle Object Properties . . . . . . . . . . . . . . . . . . . . . .7-257-257-25Implement Set/Get Interface for Properties . . . . . . . . . . . . .The Standard Set/Get Interface . . . . . . . . . . . . . . . . . . . . . .Subclass Syntax . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Get Method Syntax . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Set Method Syntax . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Class Derived from matlab.mixin.SetGet . . . . . . . . . . . . . . .7-277-277-277-287-287-29Customize Class Copy Behavior . . . . . . . . . . . . . . . . . . . . . .Customize Copy Operation . . . . . . . . . . . . . . . . . . . . . . . . .Copy Properties That Contain Handles . . . . . . . . . . . . . . . .Exclude Properties from Copy . . . . . . . . . . . . . . . . . . . . . . .7-347-347-357-37Control Number of Instances . . . . . . . . . . . . . . . . . . . . . . . . .Limit Instances . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Singleton Class Implementation . . . . . . . . . . . . . . . . . . . . .7-407-407-40Properties — Storing Class DataHow to Use Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .What Are Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Types of Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .8-28-28-2Property Syntax . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Property Definition Block . . . . . . . . . . . . . . . . . . . . . . . . . . .Access Property Values . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Inheritance of Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . .Specify Property Attributes . . . . . . . . . . . . . . . . . . . . . . . . . .8-48-48-58-68-6Property Attributes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Purpose of Property Attributes . . . . . . . . . . . . . . . . . . . . . . .Specifying Property Attributes . . . . . . . . . . . . . . . . . . . . . . .Table of Property Attributes . . . . . . . . . . . . . . . . . . . . . . . . .8-78-78-78-7Defining Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .What You Can Define . . . . . . . . . . . . . . . . . . . . . . . . . . . . .8-138-13
Initialize Property Values . . . . . . . . . . . . . . . . . . . . . . . . . .Property Default Values . . . . . . . . . . . . . . . . . . . . . . . . . . .Assign Property Values from Constructor . . . . . . . . . . . . . .Initialize Properties to Unique Values . . . . . . . . . . . . . . . . .Property Attributes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Property Access Methods . . . . . . . . . . . . . . . . . . . . . . . . . . .Reference Object Properties Using Variables . . . . . . . . . . . .8-138-148-148-158-158-168-17Mutable and Immutable Properties . . . . . . . . . . . . . . . . . . .Set Access to Property Values . . . . . . . . . . . . . . . . . . . . . . .Define Immutable Property . . . . . . . . . . . . . . . . . . . . . . . . .8-188-188-18Restrict Class of Properties . . . . . . . . . . . . . . . . . . . . . . . . . .Restrict Property Values to Specific Classes . . . . . . . . . . . .Property Restriction Syntax . . . . . . . . . . . . . . . . . . . . . . . .Restricted Property Implementation . . . . . . . . . . . . . . . . . .8-208-208-208-21Property Access Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . .Properties Provide Access to Class Data . . . . . . . . . . . . . . .Property Setter and Getter Methods . . . . . . . . . . . . . . . . . .Set and Get Method Execution and Property Events . . . . . .Access Methods and Properties Containing Arrays . . . . . . .Access Methods and Arrays of Objects . . . . . . . . . . . . . . . .Modify Property Values with Access Methods . . . . . . . . . . .8-238-238-238-268-278-278-27Property Set Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Overview of Property Access Methods . . . . . . . . . . . . . . . . .Property Set Method Syntax . . . . . . . . . . . . . . . . . . . . . . . .Validate Property Set Value . . . . . . . . . . . . . . . . . . . . . . . .When Set Method Is Called . . . . . . . . . . . . . . . . . . . . . . . .8-298-298-298-308-30Property Get Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Overview of Property Access Methods . . . . . . . . . . . . . . . . .Property Get Method Syntax . . . . . . . . . . . . . . . . . . . . . . . .Calculate Value for Dependent Property . . . . . . . . . . . . . . .Errors Not Returned from Get Method . . . . . . . . . . . . . . . .Get Method Behavior . . . . . . . . . . . . . . . . . . . . . . . . . . . . .8-328-328-328-328-338-33Access Methods for Dependent Properties . . . . . . . . . . . . . .Set and Get Methods for Dependent Properties . . . . . . . . . .Calculate Dependent Property Value . . . . . . . . . . . . . . . . . .When to Use Set Methods with Dependent Properties . . . . .Private Set Access with Dependent Properties . . . . . . . . . .8-348-348-358-358-36xiii
9xivContentsProperties Containing Objects . . . . . . . . . . . . . . . . . . . . . . . .Assigning Objects as Default Property Values . . . . . . . . . . .Assigning to Read-Only Properties Containing Objects . . . .Assignment Behavior . . . . . . . . . . . . . . . . . . . . . . . . . . . . .8-388-388-388-38Dynamic Properties — Adding Properties to an Instance .What Are Dynamic Properties . . . . . . . . . . . . . . . . . . . . . . .Define Dynamic Properties . . . . . . . . . . . . . . . . . . . . . . . . .8-418-418-42Access Methods for Dynamic Properties . . . . . . . . . . . . . . .Create Access Methods for Dynamic Properties . . . . . . . . . .Shared Set and Get Methods . . . . . . . . . . . . . . . . . . . . . . .8-458-458-46Dynamic Property Events . . . . . . . . . . . . . . . . . . . . . . . . . . . .Dynamic Properties and Ordinary Property Events . . . . . . .Dynamic-Property Events . . . . . . . . . . . . . . . . . . . . . . . . . .Listen for a Specific Property Name . . . . . . . . . . . . . . . . . .PropertyAdded Event Callback Execution . . . . . . . . . . . .PropertyRemoved Event Callback Execution . . . . . . . . . .How to Find meta.DynamicProperty Objects . . . . . . . . . .8-478-478-478-488-498-508-50Dynamic Properties and ConstructOnLoad . . . . . . . . . . . . .8-51Methods — Defining Class OperationsMethods in Class Design . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Class Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Kinds of Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Method Naming . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .9-29-29-29-3Method Attributes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Purpose of Method Attributes . . . . . . . . . . . . . . . . . . . . . . . .Specifying Method Attributes . . . . . . . . . . . . . . . . . . . . . . . .Table of Method Attributes . . . . . . . . . . . . . . . . . . . . . . . . . .9-59-59-59-5Ordinary Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Ordinary Methods Operate on Objects . . . . . . . . . . . . . . . . .Methods Inside classdef Block . . . . . . . . . . . . . . . . . . . . . . . .Method Files . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .9-79-79-79-8
Methods in Separate Files . . . . . . . . . . . . . . . . . . . . . . . . . . .Class Folders . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Define Method in Function File . . . . . . . . . . . . . . . . . . . . . .Specify Method Attributes in classdef File . . . . . . . . . . . .Methods You Must Define in the classdef File . . . . . . . . .9-109-109-119-119-12Method Invocation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Determining Which Method Is Invoked . . . . . . . . . . . . . . . .Referencing Names with Expressions—Dynamic Reference .Controlling Access to Methods . . . . . . . . . . . . . . . . . . . . . . .Invoking Superclass Methods in Subclass Methods . . . . . . .Invoking Built-In Functions . . . . . . . . . . . . . . . . . . . . . . . .9-149-149-169-179-189-19Class Constructor Methods . . . . . . . . . . . . . . . . . . . . . . . . . .Purpose of Class Constructor Methods . . . . . . . . . . . . . . . .Basic Structure of Constructor Methods . . . . . . . . . . . . . . .Guidelines for Constructors . . . . . . . . . . . . . . . . . . . . . . . . .Related Information . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Initializing Objects in Constructor . . . . . . . . . . . . . . . . . . .No Input Argument Constructor Requirement . . . . . . . . . . .Subclass Constructors . . . . . . . . . . . . . . . . . . . . . . . . . . . . .Errors During Class Construction . . . . . . . . . . . . . . . . . . . .Output Object Not Assigned . . . . . . . . . . . . . . . . . . . . . . . .9-209-209-209-219-229-229-239-249-279-27Static Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .What Are Static Methods . . . . . . . . . . . . . . . . . . . . . . . . . .Why Define Static Methods . . . . . . . . . . . . . . . . . . . . . . . . .Defining Static Methods . . . . . . . .
March 2008 Online only New for MATLAB 7.6 (Release 2008a) October 2008 Online only Revised for MATLAB 7.7 (Release 2008b) March 2009 Online only Revised for MATLAB 7.8 (Release 2009a) September 2009 Online only Revised for MATLAB 7.9 (Release 2009b) March 2010 Online only