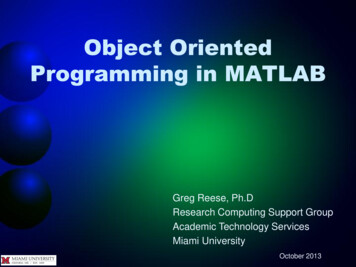
Transcription
Object OrientedProgramming in MATLABGreg Reese, Ph.DResearch Computing Support GroupAcademic Technology ServicesMiami UniversityOctober 2013
Object OrientedProgramming in MATLAB 2013 Greg Reese. All rights reserved2
The ProblemQ: What is the problem with writing largesoftware programs?A: They are very complex. As a result, theytend to be, or rather, are almost always Over time Over budget Bug ridden Brittle– Making a small change in the system causes manyand widespread problems3
The ProblemCan sum up problem this wayIf cities were builtlike software, thefirst woodpecker tocome along wouldlevel civilization4
The ProblemObject Oriented Programming One approach to managing complexity insoftware and building high-quality programs Tends to produce products that are–––––More reliableExtensibleRobust, i.e., not brittleDelivered on time and on budgetMeet customers’ expectations and requirements5
The ProblemObject oriented programming is being widelyused because it works!Object oriented programmingis the best way we currentlyknow of building large,complex software systems6
Object Oriented ProgrammingWhat is Object Oriented Programming (OOP)?It is a way of designing and developingsoftware.Its principal characteristic is to make and use(software) models of things in your problemModels hide detail and make things easier tounderstand7
Object Oriented ProgrammingProcedural programming Write software solution as data and procedures Data stored in various forms (structures) Procedures, e.g., subroutines, functions, takedata in, process it, spit out resultsIn procedural programming Pass data to first procedure, which computesone kind of output Pass data to second procedure, which computesanother kind of output etc.8
Object Oriented ProgrammingIMPORTANT CHARACTERISTIC OFPROCEDURAL PROGRAMMINGData and procedures are separate!Most MATLAB programming is procedural9
Object Oriented ProgrammingObject oriented programming Procedures and data on which they operate areput together in one bundle – an object Program “asks” object to “perform a service”– Perform a service: object does some operation on thedata stored inside itself and returns result– Program “asks” because it does not itself send datato procedures. It doesn’t know how object performsservice. Program just wants object to give it theresult, regardless of how object gets that result– “Asking an object to do something” often phrased as“Sending the object a message to do something”10
Object Oriented ProgrammingIMPORTANT CHARACTERISTIC OF OBJECTORIENTED PROGRAMMINGData and procedures are bundled together!MATLAB can do object oriented programmingI find that OOP in MATLAB Is easier than working with complex structs Makes programs cleaner, i.e., simpler and easier to read Is more enjoyable than procedural programming11
Object Oriented ProgrammingSome goals of OOP are to increase Abstraction– Provide a simplified view of something theuser is interested in– Provide all features and services userwants and nothing else Encapsulation– Keep details of items hidden within models Comprehension– Make software code easier to understand12
Object Oriented ProgrammingSome goals of OOP are to makesoftware more Correct– Meet requirements Robust– Tolerate unexpected use well Reusable– Use same code in different programs Extendable– Add new functionality Maintainable– Fix bugs13
OOP Design ProcessAnalyze domain Services? Data? Simplify by abstractingDesign Specify classes Specify interfacesWrite codeTest/use code Create/run objects14
Domain AnalysisDomain analysis – studying andunderstanding the problem and itscontext in order to make correct models Domain is often technical and/or specialized,e.g., crystallography, spectroscopy, stockderivatives, plant growth– Usually need a domain expert, i.e., someone whospecializes in the field– Programmer seldom knows domain. Domainexpert seldom knows programming!15
Domain AnalysisIn domain analysis (for models) List things that are required, e.g.,– What data?– What services (actions)?– Who will use the models? Often helps to list things that are notinterested in– Affects design of software– Makes it clear to customer and programmer thatthere are certain things the software will not do16
Domain AnalysisWORKING EXAMPLEModel of a snack vending-machineNOTE – typical OOP sequence isAllanalysisAlldesignAllclassesAllusage17
Domain AnalysisBecause we’re just learning all parts ofOOP, we’ll take it step by stepAnalyzeservice 1Analyzeservice 2Designservice 1Writeservice 1Designservice 2etc.Writeservice 2Useservice 1Useservice 218
AnalysisWORKING EXAMPLEModel of a snack vending-machineWhat is context?Possible question – How is user of modelrelated or connected to machine?––––Buyer of snacksMachine manufacturerMachine installerMachine maintenanceIn our example, only look at things from asnack buyer’s point of view19
AnalysisWhat would a buyer care about?– Imagine going to a snack machine. What do youlook at? Cost of snacks?Selection?Machine working?Machine clean?Cash?Muula?Gives change? Takes bills?Credit cards?Nutrition information?Degradable packaging?Need exact change?20
AnalysisWhat would a buyer not care about?– Imagine going to a snack machine. What do younot care about? Machine manufacturerElectrical consumptionColorInternal mechanisms21
AnalysisEven in things we do care about, there aredetails that we don’t care about. Forexample, even if it’s important to know ifthe machine takes cash, we may not careabout, or care to model––––––If it accepts foreign currencyIf it takes billsWhat denomination of bills it takesIf it accepts coinsIf it gives changeWhat happens if a coin jams22
AnalysisWORKING EXAMPLEFor brevity, will only have four services thatour snack machine must provide the user(rest of the program). It must be able to1. Tell us if it is working2. Tell us if it accepts cash3. Tell us the names of all its snacks, theircosts, and their availabilities4. Accept our money and give us our snack23
AnalysisRequirement 1 – Is it working?Suppose machine manufacturer says that ifmachine is plugged in it will work and(obviously) if it’s not plugged in it won’t work.Therefore, let’s require a service that tells usif plugged in (“yes”) or not plugged in (“no”)– Service might not literally return words “yes” and “no”,but have some binary equivalent, e.g., 1/0, true/false.Will decide in design phase24
DesignRequirement 1 – Is it working?Require a service that tells us ifplugged in or not. How do this?Various ways in MATLAB Return ‘Y’ or ‘N’ Return ‘Yes’ or ‘No’ Return 1 or 0 Return true or false25
DesignBecause only need binary response, andMATLAB logical values are inherentlybinary (keywords true and false), willchoose that way of answering question Avoids capitalization issues with “Y”, “N”,“Yes”, “No”26
DesignASIDETwo common ways in MATLAB proceduralprogramming to get some value are1. Have a function compute it2. Get it from a variableExample testResult isWaterDirty( lead, arsenic ); isDirty isWaterDirty( lead, arsenic );Longer right sideor isDirty testResult;Shorter right side27
DesignASIDEOnly two ways§ to get some value fromMATLAB object1. Have some function in object return it2. Have some variable in object provide itVariable– Pro: shorter, i.e., less typing (as in previous slide)– Con: if need to give object information so it cancompute value, can’t do soFunction – opposite pro/con§ Common ways, can get value indirectly through unusual ways, e.g., global variables28
DesignRequirement 1 – Is it working?Because don’t need to give object data inorder for it to know if it’s plugged in, will gowith simpler method, i.e., data in object29
DesignMATLAB Terminologyclass members – data or functions that arepart of a classproperty – data that is a member of a classmethod – function that is a member of a class30
DesignRequirement 1 – Is it working?Design – class will provide property called IsPluggedIn– scalar– data type is logical true if object plugged in false if object not plugged in31
CodingTo define a class called, for example, MyClass1. Make a file called MyClass.m to put the class (andnothing else) inWill discuss this later2. First line of code must beclassdef MyClass handle3. Last line of code must be corresponding endstatementend32
CodingTry ItLet’s call our class SnackMachine, socreate a file called SnackMachine.m withthe linesclassdef SnackMachine handleend% end ofclassdef.33
CodingTIPThe file is going to have a lot of “end”statements. Document what an “end”corresponds toclassdef SnackMachine handleend% end ofclassdef.34
CodingTo define a class property, add a sectionwithin the class definition that starts withpropertieslists the properties by name, e.g.,Prop1;Prop2;and ends withendBetter yet, add a comment, e.g.,end% end ofproperties35
CodingTry Itclassdef SnackMachine handlepropertiesIsPluggedIn;end % end of propertiesend% end ofclassdef.36
Testing and UsingTerminology – Class vs. ObjectClass – template from which to build apiece of software Does not exist in executable program Directions to build something, but not thatthing itselfObject – piece of software built accordingto a class Exists in executable program (memory) Built according to directions in a class37
Testing and UsingAnalogy - Class/Object : Blueprint/HouseBlueprint – plan for building a house, notthe house itselfClassBlueprintHouse – a thing built by plan in a blueprintObjectHouse38
Testing and UsingTerminology – Class vs. ObjectDistinction between class and object Often not relevant Sometimes though, very important– In these cases, will explicitly point out differences39
Testing and UsingTo create an object from a class, makea variable name, followed by the equalssign, followed by the class name, e.g., myCar Car;Accessing a property of an object Same as accessing field of struct Use the object name (not the class name),followed by a period and the property name,e.g., cost myCar.PurchasePrice; myCar.ModelYear 2013;40
CodingTry ItTo test our class, let’s make a functioncalled testSnackMachine and store it intestSnackMachine.mFirst we’ll just display value of propertyfunction testSnackMachinesnackVendor SnackMachine;% display value of propertypluggedIn snackVendor.IsPluggedInwhos pluggedIn41
CodingTry It testSnackMachinepluggedIn []NameSize BytespluggedIn 0x00ClassdoubleTwo problems already – 1) data type isdouble, not logical; 2) value is empty array,not true or falseLesson – MATLAB sets uninitialized classproperties to the empty array Must always remember to initializeproperties42
Testing and UsingTwo ways to initialize a property1. In property definition, set value, likeassigning value to a variable– When MATLAB creates object, gives it that value,but never automatically gives it a value after thatAssume that our snack machine isplugged in, so initialize IsPluggedInto true43
CodingTry ItChange class and testclassdef SnackMachine handlepropertiesIsPluggedIn true;end % end of propertiesend% end ofclassdef.44
CodingTry ItTest testSnackMachinepluggedIn 1 MATLAB displays true/false as 1/0NameSize Bytes ClasspluggedIn1x11logicalMuch better!45
CodingSecond way to initialize a propertyBecause it’s so important to initializeproperties and because it’s easy to forget todo so, MATLAB provides a convenient wayto initialize an object - the constructor46
CodingA constructor is a special method (function)of a class that MATLAB runs whenever youcreate an object of that classThe chief function of theconstructor is to return afully usable object47
CodingUse constructor to Initialize properties Perform start-up computations Acquire resources such as CPUsand files, etc.Once the constructor finishes, theobject must be completely valid, i.e.,all methods and properties functioningas defined48
CodingSome rules of MATLAB constructors Define it in a methods section Name must be exactly the same asclass name Must return one and only one outputargument – the constructed object Must never return an empty object49
CodingExcept in cases to be discussed muchlater, you don’t need to provide aconstructor for your class. If you don’t,MATLAB supplies a constructor that Takes no arguments Returns a scalar object (as opposed to an arrayof objects) whose properties are– Set to the values specified in the property definitions– Set to empty if no values are specified in definitions50
CodingYou can call the output argumentwhatever you want, but when referring tothe object’s properties or methods fromwithin the constructor, you must use theoutput-argument name. For example,51
CodingIf MyClass has a property calledProperty1 and a method calledloadData, the constructor would accessthem asfunction obj MyClassobj.Property1 49;obj.loadData( ‘myFile.txt’ );end% end of constructor52
CodingFor now, will concentrate onconstructors with no argumentsTry ItRemove value from property definition,write constructor and in it set propertyvalue, and test53
CodingTry Itclassdef SnackMachine handlepropertiesIsPluggedIn;end % end of propertiesmethodsfunction obj SnackMachineobj.IsPluggedIn true;endend % end of methodsend % end ofclassdef.54
CodingTry ItTest testSnackMachinepluggedIn 1NameSize BytespluggedIn1x11ClasslogicalEven though property value not set indefinition, it is set in constructor, soresult is good55
CodingQ: Initialize properties at definition orin constructor?A: Either or both. It’s a matter of styleAt definition– Easier to spot uninitialized property– Initializations may be scattered throughoutmany properties sectionsIn constructor– If have many properties, easy to forget toinitialize one– Good to have all initialization in one spot56
CodingScenario User wants to unplug machine Tells it that but uses ‘N’ (for “no”)instead of false Verifies that not plugged in57
Codingfunction testSnackMachinesnackVendor SnackMachine;pluggedIn snackVendor.IsPluggedIn% unplug itsnackVendor.IsPluggedIn 'N';% verify unpluggedif snackVendor.IsPluggedIndisp( 'Still plugged in' );elsedisp( 'Not plugged in' );end58
CodingTest testSnackMachinepluggedIn 1Still plugged in?Problem is lineif snackVendor.IsPluggedInMATLAB Evaluates snackVendor.IsPluggedIn to get ‘N’ ‘N’ is a character whose numerical (ASCII) value is 78 78 is nonzero, which is true59
CodingBig problem – user code expectsIsPluggedIn to be true or falseonly. Not correct expectationBigger problem – all code insideSnackMachine class expectsIsPluggedIn to be true or falseonly. Major trouble!60
CodingCan we prevent user from settingIsPluggedIn to anything but true orfalse?Yes – and will show how laterMore important question – should user evenbe able to set IsPluggedIn?In our context, no! User there to buy junk food.Not his job to tell machine if it’s plugged in ornot. Machine should handle that itself61
CodingWant user to be able to read or getIsPluggedIn but not write to or setIsPluggedIn . Can we do this?Yes. Can modify behavior of properties bysetting their attributesIn MATLAB help system, search for“property attributes” to see list of attributesand their possible values62
CodingTo set attributes of properties, after “properties”keyword and within parentheses, list attributesand their desired values like thisproperties(Attribute1 value1,Attribute2 value2)Property1;Property2;Property3; % etcend63
Coding All properties in a properties sectionhave the same attributes You must make a separate propertiessection for each different combination ofattributes that you want Any attributes not listed in propertiessection take on their default values, asdefined in the MATLAB documentation64
CodingTo control reading from and writing to aproperty To allow all class members and all users to read aproperty (get its value) set the attribute “GetAccess”to “public” To allow only class members to read a property set“GetAccess” to “private” To allow all class members and all users to write toa property (set its value) set “SetAccess” to “public” To allow only class members to write to a propertyset “SetAccess” to “private”65
CodingTry ItChange your class so that anyone canget IsPluggedIn but only classmembers can set IsPluggedInclassdef SnackMachine handleproperties(GetAccess public, SetAccess private)IsPluggedIn;end % end of properties.end % end ofclassdef.66
CodingTry ItThe default value for both “GetAccess”and “SetAccess” is “public”, so you canalso write the change asclassdef SnackMachine handleproperties(SetAccess private)IsPluggedIn;end % end of properties.end % end ofclassdef.67
CodingTry ItChange your test code to read and thenwrite IsPluggedInfunction testSnackMachinesnackVendor SnackMachine;snackVendor.IsPluggedIn % readsnackVendor.IsPluggedIn false; % write68
CodingTry ItRun the code testSnackMachineans 1Okay to readSetting the 'IsPluggedIn' property ofthe 'SnackMachine'class is not allowed.Error in testSnackMachine (line 5)snackVendor.IsPluggedIn false; % writeCrash when try to write69
CodingAllowing the user to read IsPluggedIn butnot to write to it lets him Do what he needs to do (find out if themachine is plugged in) Prevents him from doing something hedoesn’t need to do (change whether or notthe machine is plugged in)– Which has the benefit of preventing him from storingvalues that messes up the class and other users70
CodingRestricting the type of access we givea user is a special case of a usefulsoftware-development guideline called“The Principle of Least Privilege”71
CodingThe Principle of Least PrivilegeGive software only the privileges it needsto work and no moreHere, “privileges” means resources, accessrights, security rights, etc.72
CodingAlthough have fine-tuned IsPluggedIn,have lost sight of the bigger picture – theuser doesn’t really care if the vendingmachine is plugged in, he cares if it’sworking or not! For example, If internal product-delivery mechanism is broken, it’sirrelevant if machine is plugged in or not, it still won’twork If machine needs yearly parts maintenance to workand that hasn’t happened, it will shut down whether ornot it’s plugged in73
CodingLesson – make sure you provide the userthe service he wants74
CodingTry ItThe class still needs to get and setIsPluggedIn but the user shouldnot be able to do either.Change the class to effect this andverify that the user can’t read theproperty75
CodingTry ItChange classclassdef SnackMachine handleproperties(GetAccess private, SetAccess private)IsPluggedIn;end % end of properties.end % end ofclassdef.76
CodingTry ItRun revious test programfunction testSnackMachinesnackVendor SnackMachine;snackVendor.IsPluggedIn % readsnackVendor.IsPluggedIn false; % writeRun testSnackMachineGetting the 'IsPluggedIn' property ofthe 'SnackMachine‘ class is not allowed.Error in testSnackMachine (line 3)snackVendor.IsPluggedIn % read77
CodingNow Want user to be able to find out if machine is orisn’t working but not to specify whether that is so Want object to be able to find out if machine is orisn’t working and to specify whether it is or isn’tLet’s add property IsWorking to class andlet user read but not write to it, and objectread and write78
CodingTry ItChange class Add new properties section becauseattributes are different than in other section.properties(GetAccess public, SetAccess private)IsWorking;end % end of public-get, private-set propertiesproperties(GetAccess private, SetAccess private)IsPluggedIn;end % end of private-get, private-set properties.Note added detail in comments of end sections79
CodingProblem – don’t have connection betweenIsWorking and IsPluggedInBy definition, machine is working if and onlyif it is plugged in, so when code reads valueof IsWorking, want it to return value ofIsPluggedInHow do this?80
CodingMATLAB does this through dependentpropertiesDependent Property – a property of a classwhose value is computed or derived fromother propertiesRegular properties are stored in memory.Dependent properties are not stored at all –because they are computed81
CodingConcept of a dependent (computed)property is common. ExampleSuppose Point is a class that represents apoint in the plane. It has two (regular)properties X and Y, representing theobvious coordinates. If user needs to haveequivalent polar coordinates, how provideproperties for those?82
CodingOption 1 – make two new regularproperties, Radius and Angle, to storetwo polar coordinatesProblem – have now doubled theamount of memory each Point takesOption 2 – make dependent propertiesRadius and Angle When user reads Radius, computesqrt( X 2 Y 2 ) and return it When user asks for Angle, computeatan2( Y, X ) and return it83
CodingCode accesses a dependent property thesame way it accesses a regular property,e.g,if myCar.MaxSpeed 60 % read accessdisp( ‘I need a new car’ );end% paint my carmyCar.Color ‘red’; % write access84
CodingHowever, when code reads a dependentproperty, behind the scenes MATLABreplaces the property with a call to thefunction that computes that dependentproperty’s value and returns it. Thatfunction is called a property get method.85
CodingProperty get method Is a class method Must be declared in a methods sectionthat does not have any attributes Called get.PropertyNamewhere “PropertyName” is the name ofthe corresponding property86
CodingExample – if the class Car has adependent property calledMilesPerGallon, the property get methodwould be written asExactly one inputmethodsNo attributesMust be “get”Property namefunction mpg get.MilesPerGallon( obj )mpg . % code to compute outputendfunction must have end statementend % end of methods87
CodingWe’ll study methods more later. For now Define a method in a “methods” section– Define same way as ordinary function, but MUSThave end statement corresponding to function line The first input argument comes from MATLAB(not the user)– Often called “obj” A method in an object has access to allproperties in that object– Access by appending “.PropertyName” to firstmethod argument, e.g. obj.Gallons88
CodingFirst step in making dependent property Define it in a property section whose“Dependent” attribute is set to “true”– Can use other attributes also– All properties in this section will be dependent89
CodingTry ItChange class.properties(GetAccess public, .SetAccess private, Dependent true)IsWorking;end % end of public-get, private-set, dependent.Note added detail in comments of end sections90
CodingSecond step is to make the property getmethod (if you want one) Method must go in a methods section thathas no attributes Method must be called get.PropertyName Method has exactly one input argument,the one supplied by MATLAB, e.g., “obj”91
CodingTry ItChange class.methodsfunction obj SnackMachineobj.IsPluggedIn true;end % end of constructorConstructorfrom beforefunction working get.IsWorking( obj )working obj.IsPluggedIn;endend.92
CodingTry ItChange test program to read IsWorkingfunction testSnackMachinesnackVendor SnackMachine;snackVendor.IsWorkingRun testSnackMachineans 193
CodingTry ItChange initial value ofIsPluggedIn and run test programmethodsfunction obj SnackMachineobj.IsPluggedIn false;end.Run testSnackMachineans 094
CodingThird step is to make the property setmethod (if you want one) . Since we don’tneed one for IsWorking, will study later95
DesignRecapFirst requirement was to provideservice that lets user determine ifmachine is working or not. Met thisrequirement by providing twoproperties of the class, IsWorkingand IsPluggedIn96
Designproperty IsWorking Dependent - yes Read access – public Write access – none Property get method – returns value ofIsPluggedIn Property set method – none Initial value – initial value of IsPluggedIn97
Designproperty IsPluggedIn Dependent - no Read access – private Write access – private Property get method – N/A Property set method – N/A Initial state – it is plugged in98
DesignWhew! All that to answer “Is it working?”,one simple yes/no question.Since that was so much funLet’s Do It Again!99
AnalysisRequirement 2 – Does it accept cash?From before, we saw that there’s a lotmore to this question than it first appears.We can ask––––––If it accepts foreign currencyIf it takes billsWhat denomination of bills it takesIf it accepts coinsIf it gives changeWhat happens if a coin jams100
AnalysisRequirement 2 – Does it accept cash?We will greatly simplify this by asking fora yes/no type of answer. For now, willalways say it accepts cash and answers“yes” “yes” means can assume– It will take whatever coins or bills we give it– It will always be able to give us change101
DesignFrom designing IsPluggedIn, havedecided that a logical property is goodway to answer yes/no question. Let’smake AcceptsCash Dependent – ?– No: assume specified directly by manufacturer Read access – ?– Public: user needs to get this info Write access – ?– Private: user shouldn’t be able to set this Property get method – N/A Property set method – N/A Initial state – it does accept cash102
CodingTry ItDon’t have a properties section that ispublic read, private write, not dependent,so make one with AcceptsCash there.properties(GetAccess public, SetAccess private)AcceptsCash;end % end of public-get, private-set properties.103
CodingTry ItInitialize it in the constructor.methodsfunction obj SnackMachineobj.AcceptsCash true;obj.IsPluggedIn true;end % end of constructor.104
CodingTry ItEdit test program and run itfunction testSnackMachinesnackVendor SnackMachine;if snackVendor.AcceptsCashdisp( 'Takes cash!' );elsedisp( 'Doesn''t take cash' );endRun testSnackMachineTakes cash!105
CodingTry ItChange initialization to falsefunction obj SnackMachineobj.AcceptsCash false;obj.IsPluggedIn true;end % end of constructorRun testSnackMachineDoesn't take cash(Change initialization back to true)106
DesignRecapSecond requirement was to provideservice that lets user determine ifmachine accepts cash or notMet this requirement with propertyAcceptsCash––––Dependent - noRead access – publicWrite access – privateInitial state – does accept cash107
AnalysisRequirement 3 was to give us thesnack selection. Specifically, want Names of all snacks (whether in stock or not) Costs of all snacks (whether in stock or not) Availabilities (in/out of stock) of all snacksInitialize as followsSnackCostAvailableKit Kat 0.75YesCoke 1.50YesSwedish gummy fish 1.25NoGranola bars 1.25YesMonster Energy Drink 2.25Yes108
DesignTwo obvious choices for data typeStructure array Pro – easy to use in loops Pro – easier for user to move around one array than three Con – more complicated than arrays of just one data typeThree arrays Pro – easy to use in loops Con – must move three arrays instead of one Pro – less complicated than struct arrays109
DesignFurther consideration – a property inMATLAB can only have a single output. Amethod can return any number of outputs.Thus If we want to use a struct array for theoutput, we can provide it with either a propertyor a method If we want three arrays for the output, we haveto provide them through a methodLesson – technical issues of ourprogramming language can effect designof our class110
DesignThere doesn’t seem to be a compellingreason to choose one design over theother, so I’ll make an executive decisionGive the user the snack information inthree separate arrays111
DesignIMPORTANTNote that this defines the classinterface, i.e., how the class userinteracts with the class. It does notrequire us to store the informationinside the class. We will decide thatwhen we get to the coding112
DesignFor the internal storage of the snackinformation, there again doesn’t seem tobe a compelling reason to choose onedesign over the other, so for simplicitylet’s store the data in three arrays, whichwill be the properties Names, Costs, andAvailabilities113
DesignFor the internal storage of the snackinformation, there again doesn’t seem tobe a compelling reason to choose onedesign over the other, so for simplicitylet’s store the data in three arrays, whichwill be the properties Names, Costs, andAvailabilities114
Access?Design Will have method that provides all three piecesof info at once User doesn’t have use for individual pieces,e.g., what good is cost of a snack if you don’tknow its name or if it’s availableThis means all three properties shouldhave private set- and get-accessDependent?No, all three will store data, not compute it115
Try ItPut in propertiesCodingproperties( GetAccess public, SetAccess private )AcceptsCash;Availabilities;Costs;Names;end % end of public-get, private-set propertiesmethodsfunction obj SnackMachineobj.AcceptsCash false;obj.Availabilities [true, true, false, true, true];obj.Costs [ 0.75, 1.50, 1.25, 1.25, 2.25 ];obj.IsPluggedIn true;obj.Names { 'Kit Kat', 'Coke', 'Swedish gummy fish',.'Granola bars', 'Monster drink' };end % end of constructor116
DesignMethodNeed a method that returns, in threeseparate arrays, information on all snacks. Let’s call method “snacks” Since the user needs to call it, “Access”must be public It returns three arrays It has no user-provided inputs117
CodingWe’re going to use a method, let’s reviewand study MATLAB class methodsTo define a method, write a function inside amethods section, e.g.,methodsfunction obj myMethod( obj, speed, height ).end % end of myMethodend % end of methods118
Coding Define a method
MATLAB can do object oriented programming I find that OOP in MATLAB Is easier than working with complex structs Makes programs cleaner, i.e., simpler and easier to read Is more enjoyable than procedural programming . Object Oriented