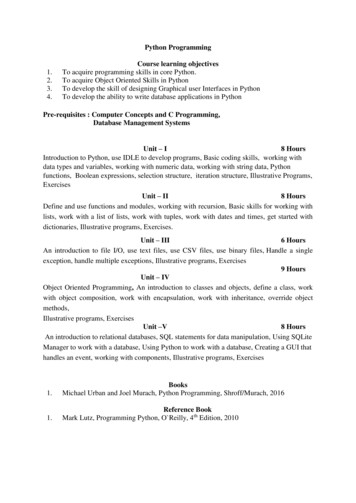
Transcription
Python Programming1.2.3.4.Course learning objectivesTo acquire programming skills in core Python.To acquire Object Oriented Skills in PythonTo develop the skill of designing Graphical user Interfaces in PythonTo develop the ability to write database applications in PythonPre-requisites : Computer Concepts and C Programming,Database Management SystemsUnit – I8 HoursIntroduction to Python, use IDLE to develop programs, Basic coding skills, working withdata types and variables, working with numeric data, working with string data, Pythonfunctions, Boolean expressions, selection structure, iteration structure, Illustrative Programs,ExercisesUnit – II8 HoursDefine and use functions and modules, working with recursion, Basic skills for working withlists, work with a list of lists, work with tuples, work with dates and times, get started withdictionaries, Illustrative programs, Exercises.Unit – III6 HoursAn introduction to file I/O, use text files, use CSV files, use binary files, Handle a singleexception, handle multiple exceptions, Illustrative programs, Exercises9 HoursUnit – IVObject Oriented Programming, An introduction to classes and objects, define a class, workwith object composition, work with encapsulation, work with inheritance, override objectmethods,Illustrative programs, ExercisesUnit –V8 HoursAn introduction to relational databases, SQL statements for data manipulation, Using SQLiteManager to work with a database, Using Python to work with a database, Creating a GUI thathandles an event, working with components, Illustrative programs, Exercises1.BooksMichael Urban and Joel Murach, Python Programming, Shroff/Murach, 20161.Reference BookMark Lutz, Programming Python, O Reilly, 4th Edition, 2010
Course Outcomes (COs)At the end of the course, the student will be able to1.2.3.1.2.3.Explain basic principles of Python programming languageImplement object oriented concepts,Implement database and GUI applications.Bloom’sLevelL2L3L3Program Outcome of this course (POs)PO No.Design/development of solutions: Design solutions for complexengineering problems and design system components or processes that meetPO3the specified needs with appropriate consideration for the public health andsafety, and the cultural, societal, and environmental considerations.Modern tool usage: Create, select, and apply appropriate techniques,resources, and modern engineering and IT tools including prediction andPO5modeling to complex engineering activities with an understanding of thelimitations.Life-long learning: Recognize the need for, and have the preparation andability to engage in independent and life-long learning in the broadest PO12context of technological change.
Unix and Shell Programming1.2.3.4.Course learning objectivesTo provide introduction to UNIX operating system and its File System.To develop the ability to formulate Regular Expressions and use them for Pattern Matching.To gain an understanding of important aspects related to the Shell and the Process.To provide a comprehensive introduction to Shell Programming, Services and Utilities.Pre-requisites: Basic knowledge of computer concepts & programming.Unit – I8 HoursIntroduction to UNIX and File-systemInside UNIX, General features of a command, PATH, Internal and External commands, Commandstructure, General purpose utilities.The File System: The File, What’s in a (File)name, The Parent-Child relationship, The UNIX FileSystem, pwd, Absolute pathnames, cd, Relative pathnames, mkdir, rmdir, cp, rm, mv, cat, file,File Attributes: ls, ls -l, ls -d, File Permissions, chmod.Unit – II9 Hoursvi/vim Editorvi Preliminaries ,Quitting vi – The Last Line Mode, Inserting and Replacing Text , Saving Text(:w) ,Exit to the UNIX Shell, The Repeat Factor, The Command Mode , Navigation , Operators , Deleting ,Moving and Yanking Text, Changing Text ( c and - ), The Dot: Repeating the Last Command ,Undoing Last Editing Instructions ( u and U), String Search, Searching with Regular Expressions,Search and Replace (:s),Unit – III8 HoursShell and Process:The Shell: The shell as command processor, Pattern matching, Redirection, Pipes, tee, Commandsubstitution.The Process: Understanding the Process, How a process is created, The Login shell, init, Internal andExternal commands, ps, running jobs in background, nice, Signals, kill.Unit – IV7 HoursFilters :SimpleFilters:wc,cmp,diff,head ,tail,sort, Filters using Regular Expressions: grep:Searchingfor pattern,grep options,regular expressions- Round one ,egrep and fgrep: The other members ,regularexpressions- Round two,sed: The Stream editor,Line addressing,context addressing.Unit – V8 HoursShell Programming:Shell variables, Shell Scripts, read: Making scripts interactive, Positional parameters, Exit status ofcommand, Logical operators && and - Conditional execution, exit: script termination, the ifconditional, test and [ ], the case conditional, expr: Computation and String handling, sleep and wait,while and until : Looping, for: Looping with a list.1.2.Text BooksSumitabha Das: “YOUR UNIX – The Ultimate Guide”, Tata McGraw Hill, 23rd reprint, 2012and onwards.Sumitabha Das: “UNIX – Concepts and Applications”, 4th Edition, Tata McGraw Hill,Copyright 2006 and onwards.
1.2.Reference BooksBehrouz A. Forouzan and Richard F. Gilberg: “UNIX and Shell Programming”, CengageLearning, 2005 and onwards.M.G. Venkateshmurthy: “UNIX & Shell Programming”, Pearson Education, 2005andonwards.Course Outcome (COs)At the end of the course, the student will be able to1.2.3.4.Describe the architecture and features of the UNIX operating system anddistinguish it from other operating systems.Demonstrate UNIX commands for file handling and process controlConstruct regular expressions for pattern matching and apply them to variousfilters for a specific task.Analyze a given problem and apply requisite facets of shell programming inorder to devise a shell script to solve the problemProgram Outcome of this course (POs)1.2.3.Engineering knowledge: Apply the knowledge of mathematics, science,engineering fundamentals, and an engineering specialization to the solutionof complex engineering problemsDesign/development of solutions: Design solutions for complexengineering problems and design system components or processes that meetthe specified needs with appropriate consideration for the public health andsafety, and the cultural, societal, and environmental considerations.Life-long learning: recognizing the need for, and have the preparation andability to engage in independent and life-long learning in the broadestcontext of technological change.Bloom’sLevelL1, L2L3L3L4PO No.1312
Object Oriented Programming with JAVACourse learning objectives (CLOs):1.Gain knowledge about basic Java language syntax and semantics to write Javaprograms and use concepts such as variables, conditional and iterative executionmethods etc.2.Understand the fundamentals of object-oriented programming in Java, includingdefining classes, objects, invoking methods etc and exception handling mechanisms.3.Understand the principles of inheritance, packages and interfaces.Prerequisites: C Programming Concepts.Unit I8 HoursOOP PARADIGM: Object-oriented programming, two paradigms, three OOP principles,overriding and exceptions, abstraction mechanisms.JAVA BASICS: History of Java, Java buzzwords, type conversion and casting. Java’sMagic: The Byte code.Unit II8 HoursINTRODUCING CLASSES : Class Fundamentals, The General Form of a Class, A SimpleClass, Declaring Objects, A Closer Look at new, Assigning Object Reference Variables,Introducing Methods, Adding a Method to the Box Class, Returning a Value, Adding aMethod That Takes Parameters, Constructors, Parameterized Constructors, The thisKeyword, Instance Variable Hiding, Garbage Collection, The finalize( ) Method.Unit III8 HoursA Closer Look at Methods and Classes: Overloading Methods, Overloading Constructors,Using Objects as Parameters, A Closer Look at Argument Passing, Returning Objects,Introducing Access Control, Understanding static, Introducing final, Introducing Nested andInner Classes.Unit IV8 HoursInheritance: Hierarchical abstractions, Base class object, subclass, subtype, substitutability,forms of inheritance- specialization, specification, construction, extension, limitation,combination, benefits of inheritance, costs of inheritance. Member access rules, super uses,using final with inheritance, polymorphism, method overriding, abstract classes, the Objectclass.Unit V8 HoursException handling: Concepts of exception handling, benefits of exception handling,exception hierarchy, usage of try, catch, throw, throws and finally, built in exceptions,creating own exception sub classes, Packages and interfaces.
Text Books:1. Herbert Schildt, “Java the Complete Reference”, TMH. 8th edition.2. Kathy Sierra & Bert Bates, “Head First Java”, O’Reilly, 2nd Edition.Reference Books:1. E Balagurusamy, “Programming with Java A Primer”, TMH, 4th edition.2. Patrick Naughton, “Java Handbook”, Osborne McGraw-HillCourse Outcome (Cos)At the end of the course, the student will be able to1.2.3.4.Identify classes, objects, members of a class and relationships among themneeded for a specific problemWrite Java application programs using OOP principles and proper programstructuringDemonstrate the concepts of polymorphism and inheritanceWrite Java programs to implement error handling techniques using exceptionhandlingProgram Outcome of this course (POs)1.2.3.Engineering knowledge: Apply the knowledge of mathematics, science,engineering fundamentals, and an engineering specialization to the solution ofcomplex engineering problems.Conduct investigations of complex problems: Use research-basedknowledge and research methods including design of experiments, analysisand interpretation of data, and synthesis of the information to provide validconclusions.Life-long learning: Recognize the need for, and have the preparation andability to engage in independent and life-long learning in the broadest contextof technological change.Bloom’sLevelL4L3L2L3PO No.PO1PO4PO12
Data StructuresCourse learning objectives(CLOs):1.To bring out the importance of data structures in a variety of applications.2.To introduce linear (arrays, linked list, doubly linked list) and non linear datastructures (Binary Tree, Heap).3.To present the advantages and applications of hashing.Prerequisites: Basic computer concepts & C programming.Unit I8 HoursBasic Concepts: Pointers and Dynamic Memory Allocation, Recursion, Arrays, DynamicallyAllocated Arrays, Structures and UnionsUnit II7 HoursStacks and queues: Stacks, Queues, Evaluation of Expressions.Unit III8 HoursLinked lists: Singly Linked lists and Chains, Representing Chains in C, Additional Listoperations, Circular Linked Lists.Unit IV8 HoursTrees: Introduction, binary trees, binary tree traversals, heaps, binary search trees.Unit V7 HoursHashing: Introduction, Hashing methods, Collision Resolution Techniques.Text Book:1. Horowitz, Sahni, Anderson-Freed: Fundamentals of Data Structures in C, 2nd Edition,Universities Press, 2007 and onwards.2. Data Structures: A Pseudocode Approach with C by Richard.F.Gilberg,Behrouz.A.Forouzan, 2nd edition 2007 and onwards.Reference Books:1. Yedidyah, Augenstein, Tannenbaum: Data Structures Using C and C , 2nd Edition,Pearson Education, 2003 and onwards.2. Debasis Samanta: Classic Data Structures, 2nd Edition, PHI, 2009 and onwards.Course Outcome (Cos)At the end of the course, the student will be able toBloom’s
Level1.2.3.4.Identify the appropriate and optimal data structure for a specified application.Employ the benefits of dynamic and static data structures implementationsIllustrate the use of different non-linear data structures and their applications.Demonstrate the use of techniques like hashing, trees and heaps in a varietyof applications.Program Outcome of this course (POs)1.2.3.Problem analysis: Identify, formulate, review research literature, and analyzecomplex engineering problems reaching substantiated conclusions using firstprinciples of mathematics, natural sciences, and engineering sciences.Design/development of solutions: Design solutions for complex engineeringproblems and design system components or processes that meet the specifiedneeds with appropriate consideration for the public health and safety, and thecultural, societal, and environmental considerations.Life-long learning: Recognize the need for, and have the preparation andability to engage in independent and life-long learning in the broadest contextof technological change.L1L3L3L3PO No.PO2PO3PO12
DATABASE MANAGEMENT SYSTEM1.2.3.4.Course learning objectivesTo discuss and realize the importance of Database Architecture Design notations, ERModeling, Mapping and Schema design.To gain the knowledge Relational algebra and learn the use of SQL and PL/SQL.To introduce formal database design approach through normalization and discussvarious normal forms.To understand the importance of Concurrent Transactions and discuss issues andtransaction control algorithms.Pre-requisites : Basic programming concepts.Unit – I8 HoursIntroduction: Introduction to database, Characteristics of Database approach, Advantages ofusing DBMS approach, When not to use a DBMS; Actors on the scene, Workers behind thescene; Three-schema architecture and data independence.Unit – II7 HoursEntity-Relationship Model: Using High-Level Conceptual Data Models for DatabaseDesign; An Example Database Application; Entity Types, Entity Sets, Attributes and Keys;Relationships, Relationship types, Roles and Structural Constraints; Weak Entity Types.Unit – III8 HoursRelational Model and Relational Algebra: Relational Model Concepts; Relational ModelConstraints and Relational Database Schemas; Update Operations, Dealing with constraintviolations; Unary Relational Operations: SELECT and PROJECT; Relational AlgebraOperations from Set Theory; Binary Relational Operations: JOIN and DIVISION;Unit – IV8 HoursDatabase Design: Informal Design Guidelines for Relation Schemas; FunctionalDependencies; Normal Forms Based on Primary Keys; General Definitions of Second andThird Normal Forms.Unit – V9 HoursSQL : SQL Data Definition and Data Types; Specifying basic constraints in SQL; Schemachange statements in SQL; Basic queries in SQL; More complex SQL Queries. Insert, Deleteand Update statements in SQL.TEXT BOOKS:1. Elmasri and Navathe: Fundamentals of Database Systems, 5th Edition, Addison-Wesley,2007 (Chapter No. 1, 2, 3, 5, 6, 7.1, 8, 10)
2. Raghu Ramakrishnan and Johannes Gehrke: Database Management Systems, 3rd Edition,McGraw-Hill, 2003. (Chapter No. 16)REFERENCE BOOKS:1. Silberschatz, Korth and Sudharshan: Data base System Concepts, 5th Edition, McGrawHill, 2006.2. C.J. Date, A. Kannan, S. Swamynatham: A Introduction to Database Systems, 8th Edition,Pearson education, 2006.Course Outcome (COs):1. Apply the database concepts and design database for given information system.[L 3]2. Create database and develop database programming skills in SQL and PL/SQL.[L 5]3. Apply the concepts of Normalization and design database which possess noanomalies.[L 3]4. Explain the issues of transaction like concurrency control, recovery and security. [L 2]Program Outcomes (POs) of the course:1. Graduates will demonstrate the ability to identify, formulate and solve computer systemsengineering problems. [PO2]2. Graduates will demonstrate the ability to design and experiment both in hardware andsoftware, analyze and interpret data. [PO3]3. Graduates will demonstrate an ability to analyze the given problems and design solutions,as per the needs and specifications. [PO4]4. Graduates will develop confidence for self education and ability for lifelong learning[PO10]5. Graduate will be capable of participating and succeeding in competitive examinations.[PO11]
Machine LearningCourse Learning Objectives1. To introduce the concept of machine learning.2. To present various Machine Learning algorithms and their applications3. To show to the implementation of Machine Learning techniques with hands on sessionsPre-requisites: Algorithms, Probability theoryUnit I5 5* HoursIntroduction, Concept Learning and Decision TreesLearning Problems – Designing Learning systems, Perspectives and Issues –Concept Learning – Version Spaces and Candidate Elimination Algorithm –Inductive bias – Decision Tree learning – Representation – Algorithm – HeuristicSpace SearchUnit II5 5* HoursNeural NetworksNeural Network Representation – Problems – Perceptrons – Multilayer Networks andBack Propagation Algorithm.Unit III5 5* HoursBayesian and Computational LearningBaye’s Theorem – Concept Learning – Maximum Likelihood – Minimum DescriptionLength principle – Bayes Optimal Classifier – Baysian Belief Network.Unit IV5 5* HoursInstance based Learning and Learning set of rulesK-nearest neighbor learning – Locally weighted regression – radial basis functions – casebased reasoning, SVM Classifier.Text Book:1. Tom M. Mitchell, “Machine Learning”, McGraw-Hill Education (INDIANEDITION), 2013.Reference Books:1. Ethem Alpaydin, “Introduction to Machine Learning”, 2nd Edition, PHI Learning Pvt.Ltd., 2013.2. T Hastie, R. Tibshirani, J.H.Fiedman, “The Elements of statistical learning”, Springer,1st Edition 2001.
Course Outcome (Cos)At the end of the course, the student will be able to1.2.Demonstrate the implementation of Machine Learning Techniquesusing tools such as Weka, R and Matlab.[Identify and apply the appropriate machine learning technique forclassification, pattern recognition, optimization and decision problemsBloom’sLevelL2L3Program Outcome of this course (POs)PO No.12345Engineering knowledge: Apply the knowledge of mathematics, science, engineeringfundamentals, and an engineering specialization to the solution of complex engineeringproblems.Problem analysis: Identify, formulate, review research literature, and analyze complexengineering problems reaching substantiated conclusions using first principles ofmathematics, natural sciences, and engineering sciences.Modern tool usage: Create, select, and apply appropriate techniques, resources, andmodern engineering and IT tools including prediction and modeling to complexengineering activities with an understanding of the limitations.Individual and team work: Function effectively as an individual, and as a member orleader in diverse teams, and in multidisciplinary settings.Communication: Communicate effectively on complex engineering activities with theengineering community and with society at large, such as, being able to comprehendand write effective reports and design documentation, make effective presentations,and give and receive clear instructions.125910
BIG DATA & HADOOP1.Course learning objectivesTo understand Big data dimensions and its applications with case studies.2.To explore Hadoop framework and architecture3.To understand basics of NoSQL4.To understand the importance of MapReduce framework5.To explore Big Data Tools and Technologies : Pig and HivePre-requisites :Database Management System, Unix Shell ProgrammingUnit – I8 HoursIntroduction: Big Data Definition, History of Data Management-Evolution of Big Data,Structuring Big Data, Elements of Big Data, Big Data Analytics, Careers in Big Data, Futureof Big Data, Use of Big Data in Social Networking, Use of Big Data in PreventingFraudulent Activities; Use of Big Data in Retail IndustryUnit – II8 HoursHadoop Ecosystem: Understanding Hadoop Ecosystem, Hadoop Distributed FileSystem:HDFS Architecture,Concept of Blocks in HDFS Architecture, NameNodes and DataNodes, The Command-Line Interface, Using HDFS Files, Hadoop-Specific File ckage,HDFSHighavailability:Features of HDFS.Unit – III8 HoursNoSQL: Introduction to NoSQL: Why NoSQL, Characteristics of NoSQL, History of NoSQL,Types of NoSQL Data Models: Key-Value Data Model, Column-Oriented Data Model,Document Data Model, Graph Databases, Schemaless Databases, Materialized views,Distribution Models: CAP Theorem, Sharding.Unit – IV8 HoursUnderstanding MapReduce: The MapReduce Framework: Exploring the Features ofMapReduce,Working of MapReduce, Exploring Map and Reduce Functions, Uses ofMapReduce.YARN Architecture: Background; Advantages of YARN;YARN Architecture
Unit – V8 HoursProperties of Pig : Introducing Hive, Getting started with Hive, Hive Services, Data types inHive, Built-in Functions in HiveAnalyzing Data with Pig: Introduction to Pig: The Pig Architecture, Benefits of Pig,Properties of PigText Book:1. DT Editorial Services,”Big Data:Black Book ,Comprehensive Problem Solver”,Dreamtech Press. 2016 Edition [ Chapters - 1,2,4,5,11,12,13,15]Reference Book:1. Paul C. Zikopoulos, Chris Eaton, Dirk deRoos, Thomas Deutsch, George Lapis,Understanding Big Data – Analytics for Enterprise Class Hadoop and Streaming Data,McGraw Hill, 20122. P. J. Sadalage and M. Fowler, "NoSQL Distilled: A Brief Guide to the EmergingWorld ofPolyglot Persistence", Addison-Wesley Professional, 2012.3. Tom White, "Hadoop: The Definitive Guide", Third Edition, O'Reilly, 2012.Course Outcome (COs)At the end of the course, the student will be able to1.2.3.4.5.1.2.3.Outline the importance of Big Data, its characteristics and use of BigData in different fields/sectors.Explain the ecosystem of HadoopInfer why to use NoSQL databases in the Big Data Scenario.Apply map reduce framework in analyzing the data and relate to YARNExplain tools in analyzing the data and managing Big DataBloom’sLevelL1Program Outcome of this course (POs)Engineering knowledge: Apply the knowledge of mathematics, science,engineering fundamentals, and an engineering specialization to the solution ofcomplex engineering problems.Problem analysis: Identify, formulate, review research literature, andanalyze complex engineering problems reaching substantiated conclusionsusing first principles of mathematics, natural sciences, and engineeringsciences.Design/development of solutions: Design solutions for complex engineeringproblems and design system components or processes that meet the specifiedneeds with appropriate consideration for the public health and safety, and thecultural, societal, and environmental considerations.L3L2L3L2PO No.123
Data Mining1.2.3.4.Course Learning ObjectivesTo introduce the fundamental concepts of data mining and Recognize various types ofdata mining tasks.To introduce mathematical and statistical models used in data Classification.To define, understand and interpret association rules.Discuss the clustering algorithms to solve real-world problems.Pre-requisites : Data Base Management Systems, Design and Analysis of AlgorithmsUnit – I7 HoursData Mining: Introduction, Challenges, Data Mining Tasks, Types of Data, Data Preprocessing, Measures of Similarity and Dissimilarity, Data Mining Applications, UsingWEKA Software.Unit – II7 HoursClassification-1 : Basics, General approach to solve classification problem, Decision TreeInduction, Rule Based Classifier, Nearest Neighbor Classifier.Unit – III8 HoursClassification-2 : Bayesian Classifiers, Artificial Neural Network, Support Vector Machine,Estimating Predictive accuracy of classification methods, Improving accuracy of classificationmethods, Evaluation criteria for classification methods.Unit – IV8 HoursAssociation Analysis: Basic Concepts and Algorithms: Frequent Itemset Generation, RuleGeneration, Compact Representation of Frequent Itemsets, Alternative Methods for GeneratingFrequent Itemsets, FP-Growth Algorithm, Evaluation of Association Patterns.Unit – V8 HoursCluster Analysis: Overview, K-means, Agglomerative Hierarchical Clustering, DBSCAN,Cluster Evaluation, Characteristics of Data, Clusters, and Clustering Algorithms.1.2.1.BooksPang-Ning Tan, Michael Steinbach, Vipin Kumar: Introduction to Data Mining,Addison-Wesley, 2007.G. K. Gupta: Introduction to Data Mining with Case Studies, 3rd Edition, PHI, NewDelhi, 2014.Reference BooksJiawei Han, Micheline Kamber , Jian Pei: Data Mining - Concepts and Techniques ,3rd Edition, Morgan Kaufmann Publishers, 2011.
Course Outcomes (COs)At the end of the course, the student will be able to1.3.4.5.Bloom’sLevel1. Explain the basic steps in data-mining.L22. Classify data by applying various clustering algorithms.L33. Evaluate the performance of various Classification algorithms.4. Recognize interesting patterns from large amounts of data toanalyze and extract patterns to make predictions of outcomes.5. Illustrate the application of clustering algorithms to real-worldproblems.L5L4Program Outcome of this course (POs)Engineering knowledge: Apply the knowledge of mathematics, science,engineering fundamentals, and an engineering specialization to the solutionof complex engineering problems.Conduct investigations of complex problems: Use research-basedknowledge and research methods including design of experiments, analysisand interpretation of data, and synthesis of the information to provide validconclusions.Modern tool usage: Create, select, and apply appropriate techniques,resources, and modern engineering and IT tools including prediction andmodelling to complex engineering activities with an understanding of thelimitations.Life-long learning: Recognize the need for, and have the preparation andability to engage in independent and life-long learning in the broadestcontext of technological change.L2PO No.14512
Python Programming Course learning objectives 1. To acquire programming skills in core Python. 2. To acquire Object Oriented Skills in Python . 1. Mark Lutz, Programming Python, O Reilly, 4th Edition, 2010 . Course Outcomes (COs) At the end of