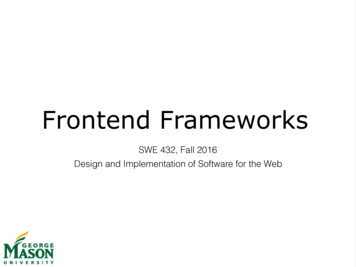
Transcription
Frontend FrameworksSWE 432, Fall 2016Design and Implementation of Software for the Web
Today How do we build a single page app without dying? MVC/MVVM (AngularJS)For further reading:Book: Learning Javascript Design Patterns, Osmani (Safari books online)Book: AngularJS in Action, Ruebbelke & Ford (Safari books online)Book: Learning AngularJS, Williamson (Safari books online)Only look at references for AngularJS (NOT Angular2)Demos (source/clone git): https://github.com/gmu-swe432/lecture10demosSource (run in browser): /BellGMU SWE 432 Fall 20162
Single Page ApplicationMany challenges DOM ManipulationeventsBrowserHTMLHistory TrackingCachingJavascriptHTML elementsHTTPRequestHTTPResponse(JSON)Presentation tierRoutingPersistence tierLoading ViewsData DomainBindinglogic tierWeb ServerHandling a ton of asyncDatabaseLaToza/BellGMU SWE 432 Fall 20163
LaToza/BellGMU SWE 432 Fall 20164
Angular to the Rescue LaToza/BellFull-featured SPA framework (can be used for nonSPA sites too!)It’s full of buzzwords! Data binding, MVC, MVVM, Routing, Testing,jqLite, Templates, History, Factories, Directives,Services, Dependency Injection, Validation, andall of their friends!There are other frameworks too, they work fine, butwe’re focusing on AngularJS: Aurelia Backbone.js Ember.jsGMU SWE 432 Fall 20165
Keeping stufforganized How do webreak apart components?
My Very Cool Drink Factory (MVC)LaToza/BellGMU SWE 432 Fall 20167
The MVC Drink Factory: A recipe Requires: 3oz coconut milk 3oz almond milk2 frozen bananas2 tbsp peanut butter1 tbsp agave nectarPlace all ingredients in blender and blend for 45seconds LaToza/BellServe in a pint glass, garnish with banana slice,cherry, and whipped cream (optional)GMU SWE 432 Fall 20168
The MVC Drink Factory: Abstract LaToza/BellWhat makes a drink? Ingredients Glasswear RecipeRecipe controls the entire processIngredients make up the content of the drinkGlass changes how you see the drink, but not itscontentsGMU SWE 432 Fall 20169
The MVC Drink Factory Can make other drinks by changing the ingredients,keeping the steps to follow and the glass Can make also keep the ingredients and steps,change the presentationSame recipe, different presentationLaToza/BellSame recipe, different ingredientsGMU SWE 432 Fall 201610
The MVC Modular Drink Factory LaToza/BellMy Very Cool factory separates concerns between recipes,ingredients, and glassesDifferent people can pull out ingredients, follow the recipe, andpour the result into the correct glass without knowing exactly whatthe other person doesCould even completely replace how the ingredients are gathered(maybe use pre-portioned), and it doesn’t effect the rest of theprocessGMU SWE 432 Fall 201611
My Very Cool Drink Factory LaToza/BellWow, this separation of concerns is just what wewant in our web apps!Because it’s so modular, we named an entiredesign pattern based on this recipe, ingredient,presentation pattern (MVC)Alternatively, we might call it Model-View-Controller Model: Ingredients Controller: Recipe View: Glass/presentationGMU SWE 432 Fall 201612
MV* Patterns LaToza/BellThe mother of them all: MVC Originally from 70's: UIs were just becomingpossible. how to separate presentation from dataand logic? Model: domain-specific data, doesn’t matter howit’s interacted with View: visual representation of current state of model Controller: Moderates user interactions, makesbusiness decisions Separation of concernsGMU SWE 432 Fall 201613
MVC & JavaScriptDOM templatesGlass/presentationJS that receives inputfrom DOM, deploysspinner, etc.RecipeIngredientsFirebase todoRef listFirebase callbacks that update view directly*Note that in drink factory, the glass doesn’t care about the ingredientsLaToza/BellGMU SWE 432 Fall 201614
MVVM LaToza/BellView does not communicate with model directlyModels are much more dumb: no formatting, etcViewModel: like a controller from MVC, but onlydoes data translation/formatting between M-VMore directly maps to MyVeryCool Drink Factorythan MVC doesGMU SWE 432 Fall 201615
MVC vs MVVMLow level controller/model codecan be easily shared (especially inserver apps)Views can have direct access tomodelLaToza/BellEasier to develop in parallel (V onlytalks to VM)View is completely “dumb” and justneeds data bindingsGMU SWE 432 Fall 201616
AngularJS LaToza/BellSupports MVC/MVVMProvides structure to organize your codeTwo-way data bindingUses plain old objects for your data - no fancystructures neededHTML templating (like react)Designed for SPAsGMU SWE 432 Fall 201617
Angular Documentation: GreatLaToza/BellGMU SWE 432 Fall 201618
Directives & Data Binding Core feature of AngularUnlike React (add HTML to code), Angular lets us directthe html to have some code in it tooLets us add code into HTMLAngular example: !DOCTYPE html html lang "en" ng-app head meta charset "UTF-8" title My Angular Demo /title script src .5.8/angular.js" /script Directives /head body What's your name? input type "text" data-ng-model "name" / {{name}} /body /html Data BindingLaToza/BellGMU SWE 432 Fall 201619
Simple Data Binding ExampleLaToza/BellGMU SWE 432 Fall 201620
Other DirectivesLaToza/BellGMU SWE 432 Fall 201621
Other Directives LaToza/Bellng-init Initialize variables within the scope of a DOMelementng-repeat Replicate a DOM element over an arrayGMU SWE 432 Fall 201622
Filters Allow you to modify the text going into data bindings Only want to make simple modifications here Syntax:{{todo.text uppercase}} (Converts the todo to uppercase) div ng-repeat "todo in todos orderBy:'-priority' " (Shows all todos ordered by key)Other uses: LaToza/Bell Select only some values in a list Order a listGMU SWE 432 Fall 201623
Partials: A "Partial" HTML documentCan be included into another with ngInclude Example: div What's your name? input type "text"data-ng-model "name"/ Hello, {{name}}! /div partials/hello.html ng-include src “‘partials/hello.html’” /ng-include index.htmlLaToza/BellGMU SWE 432 Fall 201624
Partials & ComponentsUser profile partialWho to follow partialFollow partialFeed partialFeed item partialLaToza/BellGMU SWE 432 Fall 201625
Partial Demo
Views, Controllers, Scopes LaToza/BellAngular has a lot more than just views anddirectivesLet’s focus on controllers and scopeGMU SWE 432 Fall 201627
Angular Controllers Each controller is a function that gets passed scope scope is the bridge between the controller andview scope is initially empty when the controller iscalled, and then it sets some properties on itWhen a view uses a controller, it inherits its scope function TodoController( scope){ scope.todos [{ text: "Write more demos", priority: 5},{ text : "Add some gifs", priority: 10}];}LaToza/BellGMU SWE 432 Fall 201628
Views & Controllers Select a controller for a DOM element, and it willprovide variables for everything contained in itCan have multiple controllers on one page div class "todoList" data-ng-controller "TodoController" div ng-repeat "todo in todos orderBy:'-priority' " {{todo.text uppercase}} /div /div script function TodoController( scope){ scope.todos [{ text: "Write more demos", priority: 5},{ text : "Add some gifs", priority: 10}];} /script LaToza/BellGMU SWE 432 Fall 201629
Modules as llerServiceRoutesProviderValue LaToza/BellModules contain everything that we need for asingle componentOrganize views, controllers, etc.How do we make and use them?GMU SWE 432 Fall 201630
Creating a Module Create a module and add a controller:var myApp angular.module('demoApp', []);myApp.controller("TodoController", TodoController); The empty array can instead specifydependencies Example dependency (a great one!): firebaseControllers should not stand on their own - must bepart of moduleModule name must be the name provided in ngapp html lang "en" data-ng-app “demoApp" LaToza/BellGMU SWE 432 Fall 201631
Demo: Modules,Controllers, Firebase
Modules, Routes, ServicesLaToza/BellGMU SWE 432 Fall 201633
RoutesRoutes are paths from view/controllers to others/#newLanding PageNew Todo/#categories/#landingFilter bycategoryLaToza/BellNew Category/#byCategoryGMU SWE 432 Fall 201634
Routes AngularJS makes routes read like magic!myApp.config(function( routeProvider){Dependency injection magic! routeProvider.when("/",{controller: "TodoController",templateUrl: ,{controller: "CategoryController",templateUrl: : "/"});}); LaToza/BellReads like a sentence (chaining!)GMU SWE 432 Fall 201635
Partials Easy way to have "partial" HTML documents andcombine them, magically-dynamically into one!Will be included by the route, into the containerlabeled with the directive div data-ng-view /div .myApp.config(function( routeProvider){ routeProvider.when("/",{controller: "TodoController",templateUrl: ,{controller: "CategoryController",templateUrl: : "/"});});LaToza/BellGMU SWE 432 Fall 201636
Demo: Routes Partials
Exit-Ticket ActivityGo to socrative.com and select “Student Login”Class: SWE432001 (Prof LaToza) or SWE432002 (Prof Bell)ID is your @gmu.edu email1: How well did you understand today's material2: What did you learn in today's class?For question 3: How do you think you will use React in yourHW this week?
(AngularJS) 2 For further reading: Book: Learning Javascript Design Patterns, Osmani (Safari books online) Book: AngularJS in Action, Ruebbelke & Ford (Safari books online) Book: Learning AngularJS, Williamson (Safari books online) Only look at