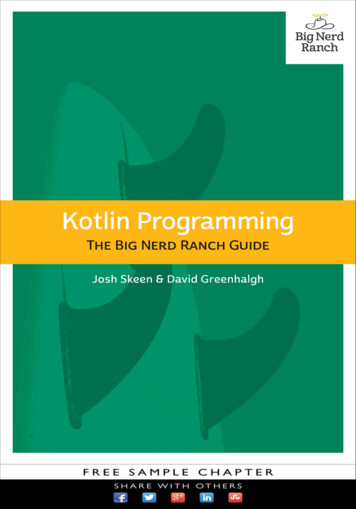
Transcription
Kotlin Programming: The Big Nerd Ranch Guideby Josh Skeen and David GreenhalghCopyright 2018 Big Nerd Ranch, LLCAll rights reserved. Printed in the United States of America. This publication is protected by copyright, andpermission must be obtained from the publisher prior to any prohibited reproduction, storage in a retrieval system,or transmission in any form or by any means, electronic, mechanical, photocopying, recording, or likewise. Forinformation regarding permissions, contactBig Nerd Ranch, LLC200 Arizona Ave NEAtlanta, GA 30307(770) bignerdranch.comThe 10-gallon hat with propeller logo is a trademark of Big Nerd Ranch, LLC.Exclusive worldwide distribution of the English edition of this book byPearson Technology Group800 East 96th StreetIndianapolis, IN 46240 USAhttp://www.informit.comThe authors and publisher have taken care in writing and printing this book but make no expressed or impliedwarranty of any kind and assume no responsibility for errors or omissions. No liability is assumed for incidentalor consequential damages in connection with or arising out of the use of the information or programs containedherein.Many of the designations used by manufacturers and sellers to distinguish their products are claimed astrademarks. Where those designations appear in this book, and the publisher was aware of a trademark claim, thedesignations have been printed with initial capital letters or in all capitals.ISBN-10 0135162386ISBN-13 978-0135162385First edition, first printing, July 2018Release D.1.1.1
DedicationFor Baker, the best little bug.— J.S.To Rebecca, a driven, patient, beautiful woman, and the reason that this book cameto be. To Mom and Dad, for valuing education above all else.— D.G.iii
AcknowledgmentsWe received a lot of help in writing this book. Without that help, this book would not be what it is, andit may never even have happened. Thanks are due.First, we need to say thank you to our colleagues at Big Nerd Ranch. Thank you to Stacy Henry andAaron Hillegass for providing us with the time and space to write this book. It has been immenselygratifying to learn and teach Kotlin. We hope that this book lives up to the trust and the support that wehave received.Particular thanks are also due to our colleagues at Big Nerd Ranch. Your careful teaching revealedmany bugs in the text, and your thoughtful recommendations led to many improvements in ourapproach. It is truly wonderful to have colleagues such as you. Thank you Kristin Marsicano, BolotKerimbaev, Brian Gardner, Chris Stewart, Paul Turner, Chris Hare, Mark Allison, Andrew Lunsford,Rafael Moreno Cesar, Eric Maxwell, Andrew Bailey, Jeremy Sherman, Christian Keur, Mikey Ward,Steve Sparks, Mark Dalrymple, CBQ, and everyone else at the Ranch who helped us with this work.Our colleagues in operations, marketing, and sales are also instrumental. Classes would literally neverbe scheduled without their work. Thank you Heather Sharpe, Mat Jackson, Rodrigo "Ram Rod" PerezVelasco, Nicholas Stolte, Justin Williams, Dan Barker, Israel Machovec, Emily Herman, PatrickFreeman, Ian Eze, and Nikki Porter. We cannot do what we do without what you do.Special thanks and an extra bit of karma are also owed to our amazing students who were adventurousenough to join us for the early access version of the course and were kind enough to help us identifyerrata. Without your feedback and insights into how to improve the course, this text would not bewhere it is today. Those students include: Santosh Katta, Abdul Hannan, Chandra Mohan, BenjaminDiGregorio, Peng Wan, Kapil Bhalla, Girish Hanchinal, Hashan Godakanda, Mithun Mahadevan,Brittany Berlanga, Natalie Ryan, Balarka Velidi, Pranay Airan, Jacob Rogers, Jean-Luc Delpech,Dennis Lin, Kristina Thai, Reid Baker, Setareh Lotfi, Harish Ravichandran, Matthew Knapp, NathanKlee, Brian Lee, Heidi Muth, Martin Davidsson, Misha Burshteyn, Kyle Summers, Cameron Hill,Vidhi Shah, Fabrice Di Meglio, Jared Burrows, Riley Brewer, Michael Krause, Tyler Holland,Gajendra Singh, Pedro Sanchez, Joe Cyboski, Zach Waldowski, Noe Arzate, Allan Caine, Zack Simon,Josh Meyers, Rick Meyers, Stephanie Guevara, Abdulrahman Alshmrani, Robert Edwards, MaribelMontejano, and Mohammad Yusuf.We want to extend a special thank you to our colleagues and members of the Android community whohelped us test the book's accuracy, clarity, and ease of use. Without your external perspective, puttingthis book together would have been even more daunting. Thank you Jon Reeve, Bill Phillips, MatthewCompton, Vishnu Rajeevan, Scott Stanlick, Alex Lumans, Shauvik Choudhary, and Jason Atwood.We also need to acknowledge the many talented folks who worked on the book with us. ElizabethHoladay, our editor, helped refine the book, crystallize its strengths, and diminish its weaknesses. AnnaBentley, our copyeditor, found and corrected errors and ultimately made us look smarter than we are.Ellie Volckhausen designed the cover. And Chris Loper designed and produced the print book and theEPUB and Kindle versions.Finally, thank you to all our students. Being your teacher offers us the opportunity to be a studentin many ways, and for that we are immensely grateful. Teaching is part of the greatest thing that wedo, and it has been a pleasure working with you. We hope that the quality of this book matches yourenthusiasm and determination.v
Table of ContentsIntroducing Kotlin . xiiiWhy Kotlin? . xiiiWho Is This Book For? . xiiiHow to Use This Book . xivFor the More Curious . xivChallenges . xivTypographical conventions . xivLooking Forward . xv1. Your First Kotlin Application . 1Installing IntelliJ IDEA . 1Your First Kotlin Project . 2Creating your first Kotlin file . 6Running your Kotlin file . 8The Kotlin REPL . 10For the More Curious: Why Use IntelliJ? . 11For the More Curious: Targeting the JVM . 12Challenge: REPL Arithmetic . 122. Variables, Constants, and Types . 13Types . 13Declaring a Variable . 14Kotlin’s Built-In Types . 16Read-Only Variables . 17Type Inference . 19Compile-Time Constants . 21Inspecting Kotlin Bytecode . 22For the More Curious: Java Primitive Types in Kotlin . 25Challenge: hasSteed . 26Challenge: The Unicorn’s Horn . 26Challenge: Magic Mirror . 263. Conditionals . 27if/else Statements . 27Adding more conditions . 30Nested if/else statements . 31More elegant conditionals . 32Ranges . 37when Expressions . 38String Templates . 40Challenge: Trying Out Some Ranges . 41Challenge: Enhancing the Aura . 42Challenge: Configurable Status Format . 424. Functions . 43Extracting Code to Functions . 43Anatomy of a Function . 46Function header . 46Function body . 49vii
Kotlin ProgrammingFunction scope . 50Calling a Function . 51Refactoring to Functions . 52Writing Your Own Functions . 54Default Arguments . 56Single-Expression Functions . 57Unit Functions . 58Named Function Arguments . 59For the More Curious: The Nothing Type . 60For the More Curious: File-Level Functions in Java . 61For the More Curious: Function Overloading . 62For the More Curious: Function Names in Backticks . 63Challenge: Single-Expression Functions . 64Challenge: Fireball Inebriation Level . 64Challenge: Inebriation Status . 645. Anonymous Functions and the Function Type . 67Anonymous Functions . 67The function type . 69Implicit returns . 70Function arguments . 71The it keyword . 72Accepting multiple arguments . 73Type Inference Support . 74Defining a Function That Accepts a Function . 75Shorthand syntax . 76Function Inlining . 77Function References . 78Function Type as Return Type . 80For the More Curious: Kotlin’s Lambdas Are Closures . 81For the More Curious: Lambdas vs Anonymous Inner Classes . 826. Null Safety and Exceptions . 83Nullability . 83Kotlin’s Explicit Null Type . 85Compile Time vs Runtime . 86Null Safety . 87Option one: the safe call operator . 88Option two: the double-bang operator . 89Option three: checking whether a value is null with if . 90Exceptions . 92Throwing an exception . 94Custom exceptions . 95Handling exceptions . 96Preconditions . 97Null: What Is It Good For? . 99For the More Curious: Checked vs Unchecked Exceptions . 100For the More Curious: How Is Nullability Enforced? . 1017. Strings . 103Extracting Substrings . 103viii
Kotlin Programmingsubstring . 103split . 106String Manipulation . 107Strings are immutable . 109String Comparison . 109For the More Curious: Unicode . 111For the More Curious: Traversing a String’s Characters . 112Challenge: Improving DragonSpeak . 1128. Numbers . 113Numeric Types . 113Integers . 114Decimal Numbers . 116Converting a String to a Numeric Type . 116Converting an Int to a Double . 117Formatting a Double . 118Converting a Double to an Int . 119For the More Curious: Bit Manipulation . 121Challenge: Remaining Pints . 122Challenge: Handling a Negative Balance . 122Challenge: Dragoncoin . 1229. Standard Functions . 123apply . 123let . 124run . 125with . 126also . 126takeIf . 127takeUnless . 127Using Standard Library Functions . 12810. Lists and Sets . 129Lists . 129Accessing a list’s elements . 130Changing a list’s contents . 133Iteration . 137Reading a File into a List . 140Destructuring . 142Sets . 142Creating a set . 143Adding elements to a set . 144while Loops . 146The break Expression . 148Collection Conversion . 149For the More Curious: Array Types . 150For the More Curious: Read-Only vs Immutable . 151Challenge: Formatted Tavern Menu . 152Challenge: Advanced Formatted Tavern Menu . 15211. Maps . 153Creating a Map . 153ix
Kotlin Programming12.13.14.15.xAccessing Map Values . 155Adding Entries to a Map . 156Modifying Map Values . 158Challenge: Tavern Bouncer . 162Defining Classes . 163Defining a Class . 163Constructing Instances . 164Class Functions . 164Visibility and Encapsulation . 166Class Properties . 167Property getters and setters . 168Property visibility . 171Computed properties . 172Refactoring NyetHack . 173Using Packages . 181For the More Curious: A Closer Look at var and val Properties . 182For the More Curious: Guarding Against Race Conditions . 184For the More Curious: Package Private . 185Initialization . 187Constructors . 187Primary constructors . 188Defining properties in a primary constructor . 189Secondary constructors . 189Default arguments . 191Named arguments . 192Initializer Blocks . 193Property Initialization . 194Initialization Order . 196Delaying Initialization . 197Late initialization . 198Lazy initialization . 198For the More Curious: Initialization Gotchas . 200Challenge: The Riddle of Excalibur . 202Inheritance . 205Defining the Room Class . 205Creating a Subclass . 206Type Checking . 212The Kotlin Type Hierarchy . 214Type casting . 215Smart casting . 216For the More Curious: Any . 217Objects . 219The object Keyword . 219Object declarations . 220Object expressions . 224Companion objects . 225Nested Classes . 226Data Classes . 228
Kotlin Programming16.17.18.19.toString .equals .copy .Destructuring declarations .Enumerated Classes .Operator Overloading .Exploring the World of NyetHack .For the More Curious: Defining Structural Comparison .For the More Curious: Algebraic Data Types .Challenge: “Quit” Command .Challenge: Implementing a World Map .Challenge: Ring the Bell .Interfaces and Abstract Classes .Defining an Interface .Implementing an Interface .Default Implementations .Abstract Classes .Combat in NyetHack .Generics .Defining Generic Types .Generic Functions .
First, we need to say thank you to our colleagues at Big Nerd Ranch. Thank you to Stacy Henry and Aaron Hillegass for providing us with the time and space to write this book. It has been immensely gratifying to learn and teach Kotlin. We hope that this book liv